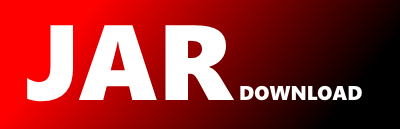
com.google.cloud.aiplatform.v1beta1.PredictionServiceClient Maven / Gradle / Ivy
Show all versions of google-cloud-aiplatform Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.aiplatform.v1beta1;
import com.google.api.HttpBody;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.BidiStreamingCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.ServerStreamingCallable;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.aiplatform.v1beta1.stub.PredictionServiceStub;
import com.google.cloud.aiplatform.v1beta1.stub.PredictionServiceStubSettings;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.protobuf.Value;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: A service for online predictions and explanations.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* EndpointName endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]");
* List instances = new ArrayList<>();
* Value parameters = Value.newBuilder().setBoolValue(true).build();
* PredictResponse response = predictionServiceClient.predict(endpoint, instances, parameters);
* }
* }
*
* Note: close() needs to be called on the PredictionServiceClient object to clean up resources
* such as threads. In the example above, try-with-resources is used, which automatically calls
* close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* Predict
* Perform an online prediction.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* predict(PredictRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* predict(EndpointName endpoint, List<Value> instances, Value parameters)
*
predict(String endpoint, List<Value> instances, Value parameters)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* predictCallable()
*
*
*
*
* RawPredict
* Perform an online prediction with an arbitrary HTTP payload.
*
The response includes the following HTTP headers:
*
* - `X-Vertex-AI-Endpoint-Id`: ID of the [Endpoint][google.cloud.aiplatform.v1beta1.Endpoint] that served this prediction.
*
*
* - `X-Vertex-AI-Deployed-Model-Id`: ID of the Endpoint's [DeployedModel][google.cloud.aiplatform.v1beta1.DeployedModel] that served this prediction.
*
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* rawPredict(RawPredictRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* rawPredict(EndpointName endpoint, HttpBody httpBody)
*
rawPredict(String endpoint, HttpBody httpBody)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* rawPredictCallable()
*
*
*
*
* StreamRawPredict
* Perform a streaming online prediction with an arbitrary HTTP payload.
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* streamRawPredictCallable()
*
*
*
*
* DirectPredict
* Perform an unary online prediction request to a gRPC model server for Vertex first-party products and frameworks.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* directPredict(DirectPredictRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* directPredictCallable()
*
*
*
*
* DirectRawPredict
* Perform an unary online prediction request to a gRPC model server for custom containers.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* directRawPredict(DirectRawPredictRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* directRawPredictCallable()
*
*
*
*
* StreamDirectPredict
* Perform a streaming online prediction request to a gRPC model server for Vertex first-party products and frameworks.
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* streamDirectPredictCallable()
*
*
*
*
* StreamDirectRawPredict
* Perform a streaming online prediction request to a gRPC model server for custom containers.
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* streamDirectRawPredictCallable()
*
*
*
*
* StreamingPredict
* Perform a streaming online prediction request for Vertex first-party products and frameworks.
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* streamingPredictCallable()
*
*
*
*
* ServerStreamingPredict
* Perform a server-side streaming online prediction request for Vertex LLM streaming.
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* serverStreamingPredictCallable()
*
*
*
*
* StreamingRawPredict
* Perform a streaming online prediction request through gRPC.
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* streamingRawPredictCallable()
*
*
*
*
* Explain
* Perform an online explanation.
*
If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is specified, the corresponding DeployModel must have [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated. If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is not specified, all DeployedModels must have [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* explain(ExplainRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* explain(EndpointName endpoint, List<Value> instances, Value parameters, String deployedModelId)
*
explain(String endpoint, List<Value> instances, Value parameters, String deployedModelId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* explainCallable()
*
*
*
*
* CountTokens
* Perform a token counting.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* countTokens(CountTokensRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* countTokens(EndpointName endpoint, List<Value> instances)
*
countTokens(String endpoint, List<Value> instances)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* countTokensCallable()
*
*
*
*
* GenerateContent
* Generate content with multimodal inputs.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* generateContent(GenerateContentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* generateContent(String model, List<Content> contents)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* generateContentCallable()
*
*
*
*
* StreamGenerateContent
* Generate content with multimodal inputs with streaming support.
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* streamGenerateContentCallable()
*
*
*
*
* ChatCompletions
* Exposes an OpenAI-compatible endpoint for chat completions.
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* chatCompletionsCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listLocationsPagedCallable()
*
listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getLocationCallable()
*
*
*
*
* SetIamPolicy
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* setIamPolicy(SetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* setIamPolicyCallable()
*
*
*
*
* GetIamPolicy
* Gets the access control policy for a resource. Returns an empty policyif the resource exists and does not have a policy set.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getIamPolicy(GetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getIamPolicyCallable()
*
*
*
*
* TestIamPermissions
* Returns permissions that a caller has on the specified resource. If theresource does not exist, this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
Note: This operation is designed to be used for buildingpermission-aware UIs and command-line tools, not for authorizationchecking. This operation may "fail open" without warning.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* testIamPermissions(TestIamPermissionsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* testIamPermissionsCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of PredictionServiceSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* PredictionServiceSettings predictionServiceSettings =
* PredictionServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* PredictionServiceClient predictionServiceClient =
* PredictionServiceClient.create(predictionServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* PredictionServiceSettings predictionServiceSettings =
* PredictionServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* PredictionServiceClient predictionServiceClient =
* PredictionServiceClient.create(predictionServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class PredictionServiceClient implements BackgroundResource {
private final PredictionServiceSettings settings;
private final PredictionServiceStub stub;
/** Constructs an instance of PredictionServiceClient with default settings. */
public static final PredictionServiceClient create() throws IOException {
return create(PredictionServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of PredictionServiceClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final PredictionServiceClient create(PredictionServiceSettings settings)
throws IOException {
return new PredictionServiceClient(settings);
}
/**
* Constructs an instance of PredictionServiceClient, using the given stub for making calls. This
* is for advanced usage - prefer using create(PredictionServiceSettings).
*/
public static final PredictionServiceClient create(PredictionServiceStub stub) {
return new PredictionServiceClient(stub);
}
/**
* Constructs an instance of PredictionServiceClient, using the given settings. This is protected
* so that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected PredictionServiceClient(PredictionServiceSettings settings) throws IOException {
this.settings = settings;
this.stub = ((PredictionServiceStubSettings) settings.getStubSettings()).createStub();
}
protected PredictionServiceClient(PredictionServiceStub stub) {
this.settings = null;
this.stub = stub;
}
public final PredictionServiceSettings getSettings() {
return settings;
}
public PredictionServiceStub getStub() {
return stub;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online prediction.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* EndpointName endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]");
* List instances = new ArrayList<>();
* Value parameters = Value.newBuilder().setBoolValue(true).build();
* PredictResponse response = predictionServiceClient.predict(endpoint, instances, parameters);
* }
* }
*
* @param endpoint Required. The name of the Endpoint requested to serve the prediction. Format:
* `projects/{project}/locations/{location}/endpoints/{endpoint}`
* @param instances Required. The instances that are the input to the prediction call. A
* DeployedModel may have an upper limit on the number of instances it supports per request,
* and when it is exceeded the prediction call errors in case of AutoML Models, or, in case of
* customer created Models, the behaviour is as documented by that Model. The schema of any
* single instance may be specified via Endpoint's DeployedModels'
* [Model's][google.cloud.aiplatform.v1beta1.DeployedModel.model]
* [PredictSchemata's][google.cloud.aiplatform.v1beta1.Model.predict_schemata]
* [instance_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.instance_schema_uri].
* @param parameters The parameters that govern the prediction. The schema of the parameters may
* be specified via Endpoint's DeployedModels' [Model's
* ][google.cloud.aiplatform.v1beta1.DeployedModel.model]
* [PredictSchemata's][google.cloud.aiplatform.v1beta1.Model.predict_schemata]
* [parameters_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.parameters_schema_uri].
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final PredictResponse predict(
EndpointName endpoint, List instances, Value parameters) {
PredictRequest request =
PredictRequest.newBuilder()
.setEndpoint(endpoint == null ? null : endpoint.toString())
.addAllInstances(instances)
.setParameters(parameters)
.build();
return predict(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online prediction.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* String endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString();
* List instances = new ArrayList<>();
* Value parameters = Value.newBuilder().setBoolValue(true).build();
* PredictResponse response = predictionServiceClient.predict(endpoint, instances, parameters);
* }
* }
*
* @param endpoint Required. The name of the Endpoint requested to serve the prediction. Format:
* `projects/{project}/locations/{location}/endpoints/{endpoint}`
* @param instances Required. The instances that are the input to the prediction call. A
* DeployedModel may have an upper limit on the number of instances it supports per request,
* and when it is exceeded the prediction call errors in case of AutoML Models, or, in case of
* customer created Models, the behaviour is as documented by that Model. The schema of any
* single instance may be specified via Endpoint's DeployedModels'
* [Model's][google.cloud.aiplatform.v1beta1.DeployedModel.model]
* [PredictSchemata's][google.cloud.aiplatform.v1beta1.Model.predict_schemata]
* [instance_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.instance_schema_uri].
* @param parameters The parameters that govern the prediction. The schema of the parameters may
* be specified via Endpoint's DeployedModels' [Model's
* ][google.cloud.aiplatform.v1beta1.DeployedModel.model]
* [PredictSchemata's][google.cloud.aiplatform.v1beta1.Model.predict_schemata]
* [parameters_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.parameters_schema_uri].
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final PredictResponse predict(String endpoint, List instances, Value parameters) {
PredictRequest request =
PredictRequest.newBuilder()
.setEndpoint(endpoint)
.addAllInstances(instances)
.setParameters(parameters)
.build();
return predict(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online prediction.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* PredictRequest request =
* PredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllInstances(new ArrayList())
* .setParameters(Value.newBuilder().setBoolValue(true).build())
* .build();
* PredictResponse response = predictionServiceClient.predict(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final PredictResponse predict(PredictRequest request) {
return predictCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online prediction.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* PredictRequest request =
* PredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllInstances(new ArrayList())
* .setParameters(Value.newBuilder().setBoolValue(true).build())
* .build();
* ApiFuture future =
* predictionServiceClient.predictCallable().futureCall(request);
* // Do something.
* PredictResponse response = future.get();
* }
* }
*/
public final UnaryCallable predictCallable() {
return stub.predictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online prediction with an arbitrary HTTP payload.
*
* The response includes the following HTTP headers:
*
*
* - `X-Vertex-AI-Endpoint-Id`: ID of the [Endpoint][google.cloud.aiplatform.v1beta1.Endpoint]
* that served this prediction.
*
*
*
* - `X-Vertex-AI-Deployed-Model-Id`: ID of the Endpoint's
* [DeployedModel][google.cloud.aiplatform.v1beta1.DeployedModel] that served this
* prediction.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* EndpointName endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]");
* HttpBody httpBody = HttpBody.newBuilder().build();
* HttpBody response = predictionServiceClient.rawPredict(endpoint, httpBody);
* }
* }
*
* @param endpoint Required. The name of the Endpoint requested to serve the prediction. Format:
* `projects/{project}/locations/{location}/endpoints/{endpoint}`
* @param httpBody The prediction input. Supports HTTP headers and arbitrary data payload.
* A [DeployedModel][google.cloud.aiplatform.v1beta1.DeployedModel] may have an upper limit
* on the number of instances it supports per request. When this limit it is exceeded for an
* AutoML model, the
* [RawPredict][google.cloud.aiplatform.v1beta1.PredictionService.RawPredict] method returns
* an error. When this limit is exceeded for a custom-trained model, the behavior varies
* depending on the model.
*
You can specify the schema for each instance in the
* [predict_schemata.instance_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.instance_schema_uri]
* field when you create a [Model][google.cloud.aiplatform.v1beta1.Model]. This schema applies
* when you deploy the `Model` as a `DeployedModel` to an
* [Endpoint][google.cloud.aiplatform.v1beta1.Endpoint] and use the `RawPredict` method.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HttpBody rawPredict(EndpointName endpoint, HttpBody httpBody) {
RawPredictRequest request =
RawPredictRequest.newBuilder()
.setEndpoint(endpoint == null ? null : endpoint.toString())
.setHttpBody(httpBody)
.build();
return rawPredict(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online prediction with an arbitrary HTTP payload.
*
*
The response includes the following HTTP headers:
*
*
* - `X-Vertex-AI-Endpoint-Id`: ID of the [Endpoint][google.cloud.aiplatform.v1beta1.Endpoint]
* that served this prediction.
*
*
*
* - `X-Vertex-AI-Deployed-Model-Id`: ID of the Endpoint's
* [DeployedModel][google.cloud.aiplatform.v1beta1.DeployedModel] that served this
* prediction.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* String endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString();
* HttpBody httpBody = HttpBody.newBuilder().build();
* HttpBody response = predictionServiceClient.rawPredict(endpoint, httpBody);
* }
* }
*
* @param endpoint Required. The name of the Endpoint requested to serve the prediction. Format:
* `projects/{project}/locations/{location}/endpoints/{endpoint}`
* @param httpBody The prediction input. Supports HTTP headers and arbitrary data payload.
* A [DeployedModel][google.cloud.aiplatform.v1beta1.DeployedModel] may have an upper limit
* on the number of instances it supports per request. When this limit it is exceeded for an
* AutoML model, the
* [RawPredict][google.cloud.aiplatform.v1beta1.PredictionService.RawPredict] method returns
* an error. When this limit is exceeded for a custom-trained model, the behavior varies
* depending on the model.
*
You can specify the schema for each instance in the
* [predict_schemata.instance_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.instance_schema_uri]
* field when you create a [Model][google.cloud.aiplatform.v1beta1.Model]. This schema applies
* when you deploy the `Model` as a `DeployedModel` to an
* [Endpoint][google.cloud.aiplatform.v1beta1.Endpoint] and use the `RawPredict` method.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HttpBody rawPredict(String endpoint, HttpBody httpBody) {
RawPredictRequest request =
RawPredictRequest.newBuilder().setEndpoint(endpoint).setHttpBody(httpBody).build();
return rawPredict(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online prediction with an arbitrary HTTP payload.
*
*
The response includes the following HTTP headers:
*
*
* - `X-Vertex-AI-Endpoint-Id`: ID of the [Endpoint][google.cloud.aiplatform.v1beta1.Endpoint]
* that served this prediction.
*
*
*
* - `X-Vertex-AI-Deployed-Model-Id`: ID of the Endpoint's
* [DeployedModel][google.cloud.aiplatform.v1beta1.DeployedModel] that served this
* prediction.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* RawPredictRequest request =
* RawPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setHttpBody(HttpBody.newBuilder().build())
* .build();
* HttpBody response = predictionServiceClient.rawPredict(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HttpBody rawPredict(RawPredictRequest request) {
return rawPredictCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online prediction with an arbitrary HTTP payload.
*
* The response includes the following HTTP headers:
*
*
* - `X-Vertex-AI-Endpoint-Id`: ID of the [Endpoint][google.cloud.aiplatform.v1beta1.Endpoint]
* that served this prediction.
*
*
*
* - `X-Vertex-AI-Deployed-Model-Id`: ID of the Endpoint's
* [DeployedModel][google.cloud.aiplatform.v1beta1.DeployedModel] that served this
* prediction.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* RawPredictRequest request =
* RawPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setHttpBody(HttpBody.newBuilder().build())
* .build();
* ApiFuture future = predictionServiceClient.rawPredictCallable().futureCall(request);
* // Do something.
* HttpBody response = future.get();
* }
* }
*/
public final UnaryCallable rawPredictCallable() {
return stub.rawPredictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a streaming online prediction with an arbitrary HTTP payload.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* StreamRawPredictRequest request =
* StreamRawPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setHttpBody(HttpBody.newBuilder().build())
* .build();
* ServerStream stream =
* predictionServiceClient.streamRawPredictCallable().call(request);
* for (HttpBody response : stream) {
* // Do something when a response is received.
* }
* }
* }
*/
public final ServerStreamingCallable
streamRawPredictCallable() {
return stub.streamRawPredictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an unary online prediction request to a gRPC model server for Vertex first-party
* products and frameworks.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* DirectPredictRequest request =
* DirectPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllInputs(new ArrayList())
* .setParameters(Tensor.newBuilder().build())
* .build();
* DirectPredictResponse response = predictionServiceClient.directPredict(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DirectPredictResponse directPredict(DirectPredictRequest request) {
return directPredictCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an unary online prediction request to a gRPC model server for Vertex first-party
* products and frameworks.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* DirectPredictRequest request =
* DirectPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllInputs(new ArrayList())
* .setParameters(Tensor.newBuilder().build())
* .build();
* ApiFuture future =
* predictionServiceClient.directPredictCallable().futureCall(request);
* // Do something.
* DirectPredictResponse response = future.get();
* }
* }
*/
public final UnaryCallable directPredictCallable() {
return stub.directPredictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an unary online prediction request to a gRPC model server for custom containers.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* DirectRawPredictRequest request =
* DirectRawPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setMethodName("methodName-723163380")
* .setInput(ByteString.EMPTY)
* .build();
* DirectRawPredictResponse response = predictionServiceClient.directRawPredict(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DirectRawPredictResponse directRawPredict(DirectRawPredictRequest request) {
return directRawPredictCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an unary online prediction request to a gRPC model server for custom containers.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* DirectRawPredictRequest request =
* DirectRawPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setMethodName("methodName-723163380")
* .setInput(ByteString.EMPTY)
* .build();
* ApiFuture future =
* predictionServiceClient.directRawPredictCallable().futureCall(request);
* // Do something.
* DirectRawPredictResponse response = future.get();
* }
* }
*/
public final UnaryCallable
directRawPredictCallable() {
return stub.directRawPredictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a streaming online prediction request to a gRPC model server for Vertex first-party
* products and frameworks.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* BidiStream bidiStream =
* predictionServiceClient.streamDirectPredictCallable().call();
* StreamDirectPredictRequest request =
* StreamDirectPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllInputs(new ArrayList())
* .setParameters(Tensor.newBuilder().build())
* .build();
* bidiStream.send(request);
* for (StreamDirectPredictResponse response : bidiStream) {
* // Do something when a response is received.
* }
* }
* }
*/
public final BidiStreamingCallable
streamDirectPredictCallable() {
return stub.streamDirectPredictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a streaming online prediction request to a gRPC model server for custom containers.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* BidiStream bidiStream =
* predictionServiceClient.streamDirectRawPredictCallable().call();
* StreamDirectRawPredictRequest request =
* StreamDirectRawPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setMethodName("methodName-723163380")
* .setInput(ByteString.EMPTY)
* .build();
* bidiStream.send(request);
* for (StreamDirectRawPredictResponse response : bidiStream) {
* // Do something when a response is received.
* }
* }
* }
*/
public final BidiStreamingCallable
streamDirectRawPredictCallable() {
return stub.streamDirectRawPredictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a streaming online prediction request for Vertex first-party products and frameworks.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* BidiStream bidiStream =
* predictionServiceClient.streamingPredictCallable().call();
* StreamingPredictRequest request =
* StreamingPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllInputs(new ArrayList())
* .setParameters(Tensor.newBuilder().build())
* .build();
* bidiStream.send(request);
* for (StreamingPredictResponse response : bidiStream) {
* // Do something when a response is received.
* }
* }
* }
*/
public final BidiStreamingCallable
streamingPredictCallable() {
return stub.streamingPredictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a server-side streaming online prediction request for Vertex LLM streaming.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* StreamingPredictRequest request =
* StreamingPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllInputs(new ArrayList())
* .setParameters(Tensor.newBuilder().build())
* .build();
* ServerStream stream =
* predictionServiceClient.serverStreamingPredictCallable().call(request);
* for (StreamingPredictResponse response : stream) {
* // Do something when a response is received.
* }
* }
* }
*/
public final ServerStreamingCallable
serverStreamingPredictCallable() {
return stub.serverStreamingPredictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a streaming online prediction request through gRPC.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* BidiStream bidiStream =
* predictionServiceClient.streamingRawPredictCallable().call();
* StreamingRawPredictRequest request =
* StreamingRawPredictRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setMethodName("methodName-723163380")
* .setInput(ByteString.EMPTY)
* .build();
* bidiStream.send(request);
* for (StreamingRawPredictResponse response : bidiStream) {
* // Do something when a response is received.
* }
* }
* }
*/
public final BidiStreamingCallable
streamingRawPredictCallable() {
return stub.streamingRawPredictCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online explanation.
*
* If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is
* specified, the corresponding DeployModel must have
* [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated.
* If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is not
* specified, all DeployedModels must have
* [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* EndpointName endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]");
* List instances = new ArrayList<>();
* Value parameters = Value.newBuilder().setBoolValue(true).build();
* String deployedModelId = "deployedModelId-1817547906";
* ExplainResponse response =
* predictionServiceClient.explain(endpoint, instances, parameters, deployedModelId);
* }
* }
*
* @param endpoint Required. The name of the Endpoint requested to serve the explanation. Format:
* `projects/{project}/locations/{location}/endpoints/{endpoint}`
* @param instances Required. The instances that are the input to the explanation call. A
* DeployedModel may have an upper limit on the number of instances it supports per request,
* and when it is exceeded the explanation call errors in case of AutoML Models, or, in case
* of customer created Models, the behaviour is as documented by that Model. The schema of any
* single instance may be specified via Endpoint's DeployedModels'
* [Model's][google.cloud.aiplatform.v1beta1.DeployedModel.model]
* [PredictSchemata's][google.cloud.aiplatform.v1beta1.Model.predict_schemata]
* [instance_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.instance_schema_uri].
* @param parameters The parameters that govern the prediction. The schema of the parameters may
* be specified via Endpoint's DeployedModels' [Model's
* ][google.cloud.aiplatform.v1beta1.DeployedModel.model]
* [PredictSchemata's][google.cloud.aiplatform.v1beta1.Model.predict_schemata]
* [parameters_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.parameters_schema_uri].
* @param deployedModelId If specified, this ExplainRequest will be served by the chosen
* DeployedModel, overriding
* [Endpoint.traffic_split][google.cloud.aiplatform.v1beta1.Endpoint.traffic_split].
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ExplainResponse explain(
EndpointName endpoint, List instances, Value parameters, String deployedModelId) {
ExplainRequest request =
ExplainRequest.newBuilder()
.setEndpoint(endpoint == null ? null : endpoint.toString())
.addAllInstances(instances)
.setParameters(parameters)
.setDeployedModelId(deployedModelId)
.build();
return explain(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online explanation.
*
* If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is
* specified, the corresponding DeployModel must have
* [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated.
* If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is not
* specified, all DeployedModels must have
* [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* String endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString();
* List instances = new ArrayList<>();
* Value parameters = Value.newBuilder().setBoolValue(true).build();
* String deployedModelId = "deployedModelId-1817547906";
* ExplainResponse response =
* predictionServiceClient.explain(endpoint, instances, parameters, deployedModelId);
* }
* }
*
* @param endpoint Required. The name of the Endpoint requested to serve the explanation. Format:
* `projects/{project}/locations/{location}/endpoints/{endpoint}`
* @param instances Required. The instances that are the input to the explanation call. A
* DeployedModel may have an upper limit on the number of instances it supports per request,
* and when it is exceeded the explanation call errors in case of AutoML Models, or, in case
* of customer created Models, the behaviour is as documented by that Model. The schema of any
* single instance may be specified via Endpoint's DeployedModels'
* [Model's][google.cloud.aiplatform.v1beta1.DeployedModel.model]
* [PredictSchemata's][google.cloud.aiplatform.v1beta1.Model.predict_schemata]
* [instance_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.instance_schema_uri].
* @param parameters The parameters that govern the prediction. The schema of the parameters may
* be specified via Endpoint's DeployedModels' [Model's
* ][google.cloud.aiplatform.v1beta1.DeployedModel.model]
* [PredictSchemata's][google.cloud.aiplatform.v1beta1.Model.predict_schemata]
* [parameters_schema_uri][google.cloud.aiplatform.v1beta1.PredictSchemata.parameters_schema_uri].
* @param deployedModelId If specified, this ExplainRequest will be served by the chosen
* DeployedModel, overriding
* [Endpoint.traffic_split][google.cloud.aiplatform.v1beta1.Endpoint.traffic_split].
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ExplainResponse explain(
String endpoint, List instances, Value parameters, String deployedModelId) {
ExplainRequest request =
ExplainRequest.newBuilder()
.setEndpoint(endpoint)
.addAllInstances(instances)
.setParameters(parameters)
.setDeployedModelId(deployedModelId)
.build();
return explain(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online explanation.
*
* If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is
* specified, the corresponding DeployModel must have
* [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated.
* If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is not
* specified, all DeployedModels must have
* [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* ExplainRequest request =
* ExplainRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllInstances(new ArrayList())
* .setParameters(Value.newBuilder().setBoolValue(true).build())
* .setExplanationSpecOverride(ExplanationSpecOverride.newBuilder().build())
* .putAllConcurrentExplanationSpecOverride(
* new HashMap())
* .setDeployedModelId("deployedModelId-1817547906")
* .build();
* ExplainResponse response = predictionServiceClient.explain(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ExplainResponse explain(ExplainRequest request) {
return explainCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an online explanation.
*
* If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is
* specified, the corresponding DeployModel must have
* [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated.
* If [deployed_model_id][google.cloud.aiplatform.v1beta1.ExplainRequest.deployed_model_id] is not
* specified, all DeployedModels must have
* [explanation_spec][google.cloud.aiplatform.v1beta1.DeployedModel.explanation_spec] populated.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* ExplainRequest request =
* ExplainRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllInstances(new ArrayList())
* .setParameters(Value.newBuilder().setBoolValue(true).build())
* .setExplanationSpecOverride(ExplanationSpecOverride.newBuilder().build())
* .putAllConcurrentExplanationSpecOverride(
* new HashMap())
* .setDeployedModelId("deployedModelId-1817547906")
* .build();
* ApiFuture future =
* predictionServiceClient.explainCallable().futureCall(request);
* // Do something.
* ExplainResponse response = future.get();
* }
* }
*/
public final UnaryCallable explainCallable() {
return stub.explainCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a token counting.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* EndpointName endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]");
* List instances = new ArrayList<>();
* CountTokensResponse response = predictionServiceClient.countTokens(endpoint, instances);
* }
* }
*
* @param endpoint Required. The name of the Endpoint requested to perform token counting. Format:
* `projects/{project}/locations/{location}/endpoints/{endpoint}`
* @param instances Optional. The instances that are the input to token counting call. Schema is
* identical to the prediction schema of the underlying model.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CountTokensResponse countTokens(EndpointName endpoint, List instances) {
CountTokensRequest request =
CountTokensRequest.newBuilder()
.setEndpoint(endpoint == null ? null : endpoint.toString())
.addAllInstances(instances)
.build();
return countTokens(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a token counting.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* String endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString();
* List instances = new ArrayList<>();
* CountTokensResponse response = predictionServiceClient.countTokens(endpoint, instances);
* }
* }
*
* @param endpoint Required. The name of the Endpoint requested to perform token counting. Format:
* `projects/{project}/locations/{location}/endpoints/{endpoint}`
* @param instances Optional. The instances that are the input to token counting call. Schema is
* identical to the prediction schema of the underlying model.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CountTokensResponse countTokens(String endpoint, List instances) {
CountTokensRequest request =
CountTokensRequest.newBuilder().setEndpoint(endpoint).addAllInstances(instances).build();
return countTokens(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a token counting.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* CountTokensRequest request =
* CountTokensRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setModel("model104069929")
* .addAllInstances(new ArrayList())
* .addAllContents(new ArrayList())
* .setSystemInstruction(Content.newBuilder().build())
* .addAllTools(new ArrayList())
* .build();
* CountTokensResponse response = predictionServiceClient.countTokens(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CountTokensResponse countTokens(CountTokensRequest request) {
return countTokensCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform a token counting.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* CountTokensRequest request =
* CountTokensRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setModel("model104069929")
* .addAllInstances(new ArrayList())
* .addAllContents(new ArrayList())
* .setSystemInstruction(Content.newBuilder().build())
* .addAllTools(new ArrayList())
* .build();
* ApiFuture future =
* predictionServiceClient.countTokensCallable().futureCall(request);
* // Do something.
* CountTokensResponse response = future.get();
* }
* }
*/
public final UnaryCallable countTokensCallable() {
return stub.countTokensCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generate content with multimodal inputs.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* String model = "model104069929";
* List contents = new ArrayList<>();
* GenerateContentResponse response = predictionServiceClient.generateContent(model, contents);
* }
* }
*
* @param model Required. The name of the publisher model requested to serve the prediction.
* Format: `projects/{project}/locations/{location}/publishers/*/models/*`
* @param contents Required. The content of the current conversation with the model.
* For single-turn queries, this is a single instance. For multi-turn queries, this is a
* repeated field that contains conversation history + latest request.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateContentResponse generateContent(String model, List contents) {
GenerateContentRequest request =
GenerateContentRequest.newBuilder().setModel(model).addAllContents(contents).build();
return generateContent(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generate content with multimodal inputs.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* GenerateContentRequest request =
* GenerateContentRequest.newBuilder()
* .setModel("model104069929")
* .addAllContents(new ArrayList())
* .setSystemInstruction(Content.newBuilder().build())
* .setCachedContent(
* CachedContentName.of("[PROJECT]", "[LOCATION]", "[CACHED_CONTENT]").toString())
* .addAllTools(new ArrayList())
* .setToolConfig(ToolConfig.newBuilder().build())
* .addAllSafetySettings(new ArrayList())
* .setGenerationConfig(GenerationConfig.newBuilder().build())
* .build();
* GenerateContentResponse response = predictionServiceClient.generateContent(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateContentResponse generateContent(GenerateContentRequest request) {
return generateContentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generate content with multimodal inputs.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* GenerateContentRequest request =
* GenerateContentRequest.newBuilder()
* .setModel("model104069929")
* .addAllContents(new ArrayList())
* .setSystemInstruction(Content.newBuilder().build())
* .setCachedContent(
* CachedContentName.of("[PROJECT]", "[LOCATION]", "[CACHED_CONTENT]").toString())
* .addAllTools(new ArrayList())
* .setToolConfig(ToolConfig.newBuilder().build())
* .addAllSafetySettings(new ArrayList())
* .setGenerationConfig(GenerationConfig.newBuilder().build())
* .build();
* ApiFuture future =
* predictionServiceClient.generateContentCallable().futureCall(request);
* // Do something.
* GenerateContentResponse response = future.get();
* }
* }
*/
public final UnaryCallable
generateContentCallable() {
return stub.generateContentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generate content with multimodal inputs with streaming support.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* GenerateContentRequest request =
* GenerateContentRequest.newBuilder()
* .setModel("model104069929")
* .addAllContents(new ArrayList())
* .setSystemInstruction(Content.newBuilder().build())
* .setCachedContent(
* CachedContentName.of("[PROJECT]", "[LOCATION]", "[CACHED_CONTENT]").toString())
* .addAllTools(new ArrayList())
* .setToolConfig(ToolConfig.newBuilder().build())
* .addAllSafetySettings(new ArrayList())
* .setGenerationConfig(GenerationConfig.newBuilder().build())
* .build();
* ServerStream stream =
* predictionServiceClient.streamGenerateContentCallable().call(request);
* for (GenerateContentResponse response : stream) {
* // Do something when a response is received.
* }
* }
* }
*/
public final ServerStreamingCallable
streamGenerateContentCallable() {
return stub.streamGenerateContentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Exposes an OpenAI-compatible endpoint for chat completions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* ChatCompletionsRequest request =
* ChatCompletionsRequest.newBuilder()
* .setEndpoint(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setHttpBody(HttpBody.newBuilder().build())
* .build();
* ServerStream stream =
* predictionServiceClient.chatCompletionsCallable().call(request);
* for (HttpBody response : stream) {
* // Do something when a response is received.
* }
* }
* }
*/
public final ServerStreamingCallable chatCompletionsCallable() {
return stub.chatCompletionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : predictionServiceClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* predictionServiceClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response =
* predictionServiceClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = predictionServiceClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future =
* predictionServiceClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Policy response = predictionServiceClient.setIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(SetIamPolicyRequest request) {
return setIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = predictionServiceClient.setIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable setIamPolicyCallable() {
return stub.setIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* Policy response = predictionServiceClient.getIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(GetIamPolicyRequest request) {
return getIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* ApiFuture future = predictionServiceClient.getIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable getIamPolicyCallable() {
return stub.getIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllPermissions(new ArrayList())
* .build();
* TestIamPermissionsResponse response = predictionServiceClient.testIamPermissions(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(TestIamPermissionsRequest request) {
return testIamPermissionsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(
* EndpointName.ofProjectLocationEndpointName(
* "[PROJECT]", "[LOCATION]", "[ENDPOINT]")
* .toString())
* .addAllPermissions(new ArrayList())
* .build();
* ApiFuture future =
* predictionServiceClient.testIamPermissionsCallable().futureCall(request);
* // Do something.
* TestIamPermissionsResponse response = future.get();
* }
* }
*/
public final UnaryCallable
testIamPermissionsCallable() {
return stub.testIamPermissionsCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}