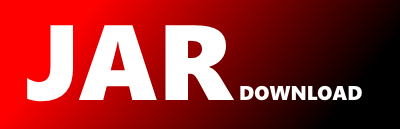
com.google.cloud.aiplatform.v1beta1.package-info Maven / Gradle / Ivy
Show all versions of google-cloud-aiplatform Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* A client to Vertex AI API
*
* The interfaces provided are listed below, along with usage samples.
*
*
======================= DatasetServiceClient =======================
*
*
Service Description: The service that manages Vertex AI Dataset and its child resources.
*
*
Sample for DatasetServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (DatasetServiceClient datasetServiceClient = DatasetServiceClient.create()) {
* DatasetName name = DatasetName.of("[PROJECT]", "[LOCATION]", "[DATASET]");
* Dataset response = datasetServiceClient.getDataset(name);
* }
* }
*
* ======================= DeploymentResourcePoolServiceClient =======================
*
*
Service Description: A service that manages the DeploymentResourcePool resource.
*
*
Sample for DeploymentResourcePoolServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (DeploymentResourcePoolServiceClient deploymentResourcePoolServiceClient =
* DeploymentResourcePoolServiceClient.create()) {
* DeploymentResourcePoolName name =
* DeploymentResourcePoolName.of("[PROJECT]", "[LOCATION]", "[DEPLOYMENT_RESOURCE_POOL]");
* DeploymentResourcePool response =
* deploymentResourcePoolServiceClient.getDeploymentResourcePool(name);
* }
* }
*
* ======================= EndpointServiceClient =======================
*
*
Service Description: A service for managing Vertex AI's Endpoints.
*
*
Sample for EndpointServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (EndpointServiceClient endpointServiceClient = EndpointServiceClient.create()) {
* EndpointName name =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]");
* Endpoint response = endpointServiceClient.getEndpoint(name);
* }
* }
*
* ======================= EvaluationServiceClient =======================
*
*
Service Description: Vertex AI Online Evaluation Service.
*
*
Sample for EvaluationServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (EvaluationServiceClient evaluationServiceClient = EvaluationServiceClient.create()) {
* EvaluateInstancesRequest request =
* EvaluateInstancesRequest.newBuilder()
* .setLocation(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .build();
* EvaluateInstancesResponse response = evaluationServiceClient.evaluateInstances(request);
* }
* }
*
* ======================= ExtensionExecutionServiceClient =======================
*
*
Service Description: A service for Extension execution.
*
*
Sample for ExtensionExecutionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ExtensionExecutionServiceClient extensionExecutionServiceClient =
* ExtensionExecutionServiceClient.create()) {
* ExtensionName name = ExtensionName.of("[PROJECT]", "[LOCATION]", "[EXTENSION]");
* String operationId = "operationId129704162";
* ExecuteExtensionResponse response =
* extensionExecutionServiceClient.executeExtension(name, operationId);
* }
* }
*
* ======================= ExtensionRegistryServiceClient =======================
*
*
Service Description: A service for managing Vertex AI's Extension registry.
*
*
Sample for ExtensionRegistryServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ExtensionRegistryServiceClient extensionRegistryServiceClient =
* ExtensionRegistryServiceClient.create()) {
* ExtensionName name = ExtensionName.of("[PROJECT]", "[LOCATION]", "[EXTENSION]");
* Extension response = extensionRegistryServiceClient.getExtension(name);
* }
* }
*
* ======================= FeatureOnlineStoreAdminServiceClient =======================
*
*
Service Description: The service that handles CRUD and List for resources for
* FeatureOnlineStore.
*
*
Sample for FeatureOnlineStoreAdminServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeatureOnlineStoreAdminServiceClient featureOnlineStoreAdminServiceClient =
* FeatureOnlineStoreAdminServiceClient.create()) {
* FeatureOnlineStoreName name =
* FeatureOnlineStoreName.of("[PROJECT]", "[LOCATION]", "[FEATURE_ONLINE_STORE]");
* FeatureOnlineStore response =
* featureOnlineStoreAdminServiceClient.getFeatureOnlineStore(name);
* }
* }
*
* ======================= FeatureOnlineStoreServiceClient =======================
*
*
Service Description: A service for fetching feature values from the online store.
*
*
Sample for FeatureOnlineStoreServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeatureOnlineStoreServiceClient featureOnlineStoreServiceClient =
* FeatureOnlineStoreServiceClient.create()) {
* FeatureViewName featureView =
* FeatureViewName.of("[PROJECT]", "[LOCATION]", "[FEATURE_ONLINE_STORE]", "[FEATURE_VIEW]");
* FeatureViewDataKey dataKey = FeatureViewDataKey.newBuilder().build();
* FetchFeatureValuesResponse response =
* featureOnlineStoreServiceClient.fetchFeatureValues(featureView, dataKey);
* }
* }
*
* ======================= FeatureRegistryServiceClient =======================
*
*
Service Description: The service that handles CRUD and List for resources for FeatureRegistry.
*
*
Sample for FeatureRegistryServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeatureRegistryServiceClient featureRegistryServiceClient =
* FeatureRegistryServiceClient.create()) {
* FeatureGroupName name = FeatureGroupName.of("[PROJECT]", "[LOCATION]", "[FEATURE_GROUP]");
* FeatureGroup response = featureRegistryServiceClient.getFeatureGroup(name);
* }
* }
*
* ======================= FeaturestoreOnlineServingServiceClient =======================
*
*
Service Description: A service for serving online feature values.
*
*
Sample for FeaturestoreOnlineServingServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeaturestoreOnlineServingServiceClient featurestoreOnlineServingServiceClient =
* FeaturestoreOnlineServingServiceClient.create()) {
* EntityTypeName entityType =
* EntityTypeName.of("[PROJECT]", "[LOCATION]", "[FEATURESTORE]", "[ENTITY_TYPE]");
* ReadFeatureValuesResponse response =
* featurestoreOnlineServingServiceClient.readFeatureValues(entityType);
* }
* }
*
* ======================= FeaturestoreServiceClient =======================
*
*
Service Description: The service that handles CRUD and List for resources for Featurestore.
*
*
Sample for FeaturestoreServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FeaturestoreServiceClient featurestoreServiceClient = FeaturestoreServiceClient.create()) {
* FeaturestoreName name = FeaturestoreName.of("[PROJECT]", "[LOCATION]", "[FEATURESTORE]");
* Featurestore response = featurestoreServiceClient.getFeaturestore(name);
* }
* }
*
* ======================= GenAiCacheServiceClient =======================
*
*
Service Description: Service for managing Vertex AI's CachedContent resource.
*
*
Sample for GenAiCacheServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (GenAiCacheServiceClient genAiCacheServiceClient = GenAiCacheServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* CachedContent cachedContent = CachedContent.newBuilder().build();
* CachedContent response = genAiCacheServiceClient.createCachedContent(parent, cachedContent);
* }
* }
*
* ======================= GenAiTuningServiceClient =======================
*
*
Service Description: A service for creating and managing GenAI Tuning Jobs.
*
*
Sample for GenAiTuningServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (GenAiTuningServiceClient genAiTuningServiceClient = GenAiTuningServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* TuningJob tuningJob = TuningJob.newBuilder().build();
* TuningJob response = genAiTuningServiceClient.createTuningJob(parent, tuningJob);
* }
* }
*
* ======================= IndexEndpointServiceClient =======================
*
*
Service Description: A service for managing Vertex AI's IndexEndpoints.
*
*
Sample for IndexEndpointServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IndexEndpointServiceClient indexEndpointServiceClient =
* IndexEndpointServiceClient.create()) {
* IndexEndpointName name = IndexEndpointName.of("[PROJECT]", "[LOCATION]", "[INDEX_ENDPOINT]");
* IndexEndpoint response = indexEndpointServiceClient.getIndexEndpoint(name);
* }
* }
*
* ======================= IndexServiceClient =======================
*
*
Service Description: A service for creating and managing Vertex AI's Index resources.
*
*
Sample for IndexServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IndexServiceClient indexServiceClient = IndexServiceClient.create()) {
* IndexName name = IndexName.of("[PROJECT]", "[LOCATION]", "[INDEX]");
* Index response = indexServiceClient.getIndex(name);
* }
* }
*
* ======================= JobServiceClient =======================
*
*
Service Description: A service for creating and managing Vertex AI's jobs.
*
*
Sample for JobServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (JobServiceClient jobServiceClient = JobServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* CustomJob customJob = CustomJob.newBuilder().build();
* CustomJob response = jobServiceClient.createCustomJob(parent, customJob);
* }
* }
*
* ======================= LlmUtilityServiceClient =======================
*
*
Service Description: Service for LLM related utility functions.
*
*
Sample for LlmUtilityServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LlmUtilityServiceClient llmUtilityServiceClient = LlmUtilityServiceClient.create()) {
* EndpointName endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]");
* List instances = new ArrayList<>();
* ComputeTokensResponse response = llmUtilityServiceClient.computeTokens(endpoint, instances);
* }
* }
*
* ======================= MatchServiceClient =======================
*
*
Service Description: MatchService is a Google managed service for efficient vector similarity
* search at scale.
*
*
Sample for MatchServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (MatchServiceClient matchServiceClient = MatchServiceClient.create()) {
* FindNeighborsRequest request =
* FindNeighborsRequest.newBuilder()
* .setIndexEndpoint(
* IndexEndpointName.of("[PROJECT]", "[LOCATION]", "[INDEX_ENDPOINT]").toString())
* .setDeployedIndexId("deployedIndexId-1101212953")
* .addAllQueries(new ArrayList())
* .setReturnFullDatapoint(true)
* .build();
* FindNeighborsResponse response = matchServiceClient.findNeighbors(request);
* }
* }
*
* ======================= MetadataServiceClient =======================
*
*
Service Description: Service for reading and writing metadata entries.
*
*
Sample for MetadataServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (MetadataServiceClient metadataServiceClient = MetadataServiceClient.create()) {
* MetadataStoreName name = MetadataStoreName.of("[PROJECT]", "[LOCATION]", "[METADATA_STORE]");
* MetadataStore response = metadataServiceClient.getMetadataStore(name);
* }
* }
*
* ======================= MigrationServiceClient =======================
*
*
Service Description: A service that migrates resources from automl.googleapis.com,
* datalabeling.googleapis.com and ml.googleapis.com to Vertex AI.
*
*
Sample for MigrationServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (MigrationServiceClient migrationServiceClient = MigrationServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = migrationServiceClient.getLocation(request);
* }
* }
*
* ======================= ModelGardenServiceClient =======================
*
*
Service Description: The interface of Model Garden Service.
*
*
Sample for ModelGardenServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelGardenServiceClient modelGardenServiceClient = ModelGardenServiceClient.create()) {
* PublisherModelName name = PublisherModelName.of("[PUBLISHER]", "[MODEL]");
* PublisherModel response = modelGardenServiceClient.getPublisherModel(name);
* }
* }
*
* ======================= ModelMonitoringServiceClient =======================
*
*
Service Description: A service for creating and managing Vertex AI Model moitoring. This
* includes `ModelMonitor` resources, `ModelMonitoringJob` resources.
*
*
Sample for ModelMonitoringServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelMonitoringServiceClient modelMonitoringServiceClient =
* ModelMonitoringServiceClient.create()) {
* ModelMonitorName name = ModelMonitorName.of("[PROJECT]", "[LOCATION]", "[MODEL_MONITOR]");
* ModelMonitor response = modelMonitoringServiceClient.getModelMonitor(name);
* }
* }
*
* ======================= ModelServiceClient =======================
*
*
Service Description: A service for managing Vertex AI's machine learning Models.
*
*
Sample for ModelServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ModelName name = ModelName.of("[PROJECT]", "[LOCATION]", "[MODEL]");
* Model response = modelServiceClient.getModel(name);
* }
* }
*
* ======================= NotebookServiceClient =======================
*
*
Service Description: The interface for Vertex Notebook service (a.k.a. Colab on Workbench).
*
*
Sample for NotebookServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (NotebookServiceClient notebookServiceClient = NotebookServiceClient.create()) {
* NotebookRuntimeTemplateName name =
* NotebookRuntimeTemplateName.of("[PROJECT]", "[LOCATION]", "[NOTEBOOK_RUNTIME_TEMPLATE]");
* NotebookRuntimeTemplate response = notebookServiceClient.getNotebookRuntimeTemplate(name);
* }
* }
*
* ======================= PersistentResourceServiceClient =======================
*
*
Service Description: A service for managing Vertex AI's machine learning PersistentResource.
*
*
Sample for PersistentResourceServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PersistentResourceServiceClient persistentResourceServiceClient =
* PersistentResourceServiceClient.create()) {
* PersistentResourceName name =
* PersistentResourceName.of("[PROJECT]", "[LOCATION]", "[PERSISTENT_RESOURCE]");
* PersistentResource response = persistentResourceServiceClient.getPersistentResource(name);
* }
* }
*
* ======================= PipelineServiceClient =======================
*
*
Service Description: A service for creating and managing Vertex AI's pipelines. This includes
* both `TrainingPipeline` resources (used for AutoML and custom training) and `PipelineJob`
* resources (used for Vertex AI Pipelines).
*
*
Sample for PipelineServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PipelineServiceClient pipelineServiceClient = PipelineServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* TrainingPipeline trainingPipeline = TrainingPipeline.newBuilder().build();
* TrainingPipeline response =
* pipelineServiceClient.createTrainingPipeline(parent, trainingPipeline);
* }
* }
*
* ======================= PredictionServiceClient =======================
*
*
Service Description: A service for online predictions and explanations.
*
*
Sample for PredictionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* EndpointName endpoint =
* EndpointName.ofProjectLocationEndpointName("[PROJECT]", "[LOCATION]", "[ENDPOINT]");
* List instances = new ArrayList<>();
* Value parameters = Value.newBuilder().setBoolValue(true).build();
* PredictResponse response = predictionServiceClient.predict(endpoint, instances, parameters);
* }
* }
*
* ======================= ReasoningEngineExecutionServiceClient =======================
*
*
Service Description: A service for executing queries on Reasoning Engine.
*
*
Sample for ReasoningEngineExecutionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ReasoningEngineExecutionServiceClient reasoningEngineExecutionServiceClient =
* ReasoningEngineExecutionServiceClient.create()) {
* QueryReasoningEngineRequest request =
* QueryReasoningEngineRequest.newBuilder()
* .setName(
* ReasoningEngineName.of("[PROJECT]", "[LOCATION]", "[REASONING_ENGINE]")
* .toString())
* .setInput(Struct.newBuilder().build())
* .build();
* QueryReasoningEngineResponse response =
* reasoningEngineExecutionServiceClient.queryReasoningEngine(request);
* }
* }
*
* ======================= ReasoningEngineServiceClient =======================
*
*
Service Description: A service for managing Vertex AI's Reasoning Engines.
*
*
Sample for ReasoningEngineServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ReasoningEngineServiceClient reasoningEngineServiceClient =
* ReasoningEngineServiceClient.create()) {
* ReasoningEngineName name =
* ReasoningEngineName.of("[PROJECT]", "[LOCATION]", "[REASONING_ENGINE]");
* ReasoningEngine response = reasoningEngineServiceClient.getReasoningEngine(name);
* }
* }
*
* ======================= ScheduleServiceClient =======================
*
*
Service Description: A service for creating and managing Vertex AI's Schedule resources to
* periodically launch shceudled runs to make API calls.
*
*
Sample for ScheduleServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ScheduleServiceClient scheduleServiceClient = ScheduleServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Schedule schedule = Schedule.newBuilder().build();
* Schedule response = scheduleServiceClient.createSchedule(parent, schedule);
* }
* }
*
* ======================= SpecialistPoolServiceClient =======================
*
*
Service Description: A service for creating and managing Customer SpecialistPools. When
* customers start Data Labeling jobs, they can reuse/create Specialist Pools to bring their own
* Specialists to label the data. Customers can add/remove Managers for the Specialist Pool on Cloud
* console, then Managers will get email notifications to manage Specialists and tasks on
* CrowdCompute console.
*
*
Sample for SpecialistPoolServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (SpecialistPoolServiceClient specialistPoolServiceClient =
* SpecialistPoolServiceClient.create()) {
* SpecialistPoolName name =
* SpecialistPoolName.of("[PROJECT]", "[LOCATION]", "[SPECIALIST_POOL]");
* SpecialistPool response = specialistPoolServiceClient.getSpecialistPool(name);
* }
* }
*
* ======================= TensorboardServiceClient =======================
*
*
Service Description: TensorboardService
*
*
Sample for TensorboardServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TensorboardServiceClient tensorboardServiceClient = TensorboardServiceClient.create()) {
* TensorboardName name = TensorboardName.of("[PROJECT]", "[LOCATION]", "[TENSORBOARD]");
* Tensorboard response = tensorboardServiceClient.getTensorboard(name);
* }
* }
*
* ======================= VertexRagDataServiceClient =======================
*
*
Service Description: A service for managing user data for RAG.
*
*
Sample for VertexRagDataServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (VertexRagDataServiceClient vertexRagDataServiceClient =
* VertexRagDataServiceClient.create()) {
* RagCorpusName name = RagCorpusName.of("[PROJECT]", "[LOCATION]", "[RAG_CORPUS]");
* RagCorpus response = vertexRagDataServiceClient.getRagCorpus(name);
* }
* }
*
* ======================= VertexRagServiceClient =======================
*
*
Service Description: A service for retrieving relevant contexts.
*
*
Sample for VertexRagServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (VertexRagServiceClient vertexRagServiceClient = VertexRagServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* RagQuery query = RagQuery.newBuilder().build();
* RetrieveContextsResponse response = vertexRagServiceClient.retrieveContexts(parent, query);
* }
* }
*
* ======================= VizierServiceClient =======================
*
*
Service Description: Vertex AI Vizier API.
*
*
Vertex AI Vizier is a service to solve blackbox optimization problems, such as tuning machine
* learning hyperparameters and searching over deep learning architectures.
*
*
Sample for VizierServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (VizierServiceClient vizierServiceClient = VizierServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Study study = Study.newBuilder().build();
* Study response = vizierServiceClient.createStudy(parent, study);
* }
* }
*/
@Generated("by gapic-generator-java")
package com.google.cloud.aiplatform.v1beta1;
import javax.annotation.Generated;