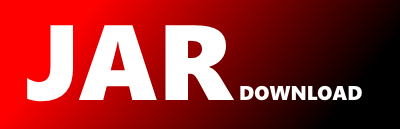
com.google.cloud.alloydb.v1alpha.AlloyDBAdminClient Maven / Gradle / Ivy
/*
* Copyright 2022 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.alloydb.v1alpha;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.alloydb.v1alpha.stub.AlloyDBAdminStub;
import com.google.cloud.alloydb.v1alpha.stub.AlloyDBAdminStubSettings;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Service describing handlers for resources
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ClusterName name = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]");
* Cluster response = alloyDBAdminClient.getCluster(name);
* }
* }
*
* Note: close() needs to be called on the AlloyDBAdminClient object to clean up resources such
* as threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
The surface of this class includes several types of Java methods for each of the API's
* methods:
*
*
* - A "flattened" method. With this type of method, the fields of the request type have been
* converted into function parameters. It may be the case that not all fields are available as
* parameters, and not every API method will have a flattened method entry point.
*
- A "request object" method. This type of method only takes one parameter, a request object,
* which must be constructed before the call. Not every API method will have a request object
* method.
*
- A "callable" method. This type of method takes no parameters and returns an immutable API
* callable object, which can be used to initiate calls to the service.
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of AlloyDBAdminSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* AlloyDBAdminSettings alloyDBAdminSettings =
* AlloyDBAdminSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create(alloyDBAdminSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* AlloyDBAdminSettings alloyDBAdminSettings =
* AlloyDBAdminSettings.newBuilder().setEndpoint(myEndpoint).build();
* AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create(alloyDBAdminSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* AlloyDBAdminSettings alloyDBAdminSettings = AlloyDBAdminSettings.newHttpJsonBuilder().build();
* AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create(alloyDBAdminSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class AlloyDBAdminClient implements BackgroundResource {
private final AlloyDBAdminSettings settings;
private final AlloyDBAdminStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of AlloyDBAdminClient with default settings. */
public static final AlloyDBAdminClient create() throws IOException {
return create(AlloyDBAdminSettings.newBuilder().build());
}
/**
* Constructs an instance of AlloyDBAdminClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final AlloyDBAdminClient create(AlloyDBAdminSettings settings) throws IOException {
return new AlloyDBAdminClient(settings);
}
/**
* Constructs an instance of AlloyDBAdminClient, using the given stub for making calls. This is
* for advanced usage - prefer using create(AlloyDBAdminSettings).
*/
public static final AlloyDBAdminClient create(AlloyDBAdminStub stub) {
return new AlloyDBAdminClient(stub);
}
/**
* Constructs an instance of AlloyDBAdminClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected AlloyDBAdminClient(AlloyDBAdminSettings settings) throws IOException {
this.settings = settings;
this.stub = ((AlloyDBAdminStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected AlloyDBAdminClient(AlloyDBAdminStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final AlloyDBAdminSettings getSettings() {
return settings;
}
public AlloyDBAdminStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Clusters in a given project and location.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Cluster element : alloyDBAdminClient.listClusters(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Cluster.name field. Additionally, you can perform an aggregated list
* operation by specifying a value with the following format: *
* projects/{project}/locations/-
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListClustersPagedResponse listClusters(LocationName parent) {
ListClustersRequest request =
ListClustersRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listClusters(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Clusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Cluster element : alloyDBAdminClient.listClusters(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Cluster.name field. Additionally, you can perform an aggregated list
* operation by specifying a value with the following format: *
* projects/{project}/locations/-
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListClustersPagedResponse listClusters(String parent) {
ListClustersRequest request = ListClustersRequest.newBuilder().setParent(parent).build();
return listClusters(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Clusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListClustersRequest request =
* ListClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Cluster element : alloyDBAdminClient.listClusters(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListClustersPagedResponse listClusters(ListClustersRequest request) {
return listClustersPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Clusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListClustersRequest request =
* ListClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* alloyDBAdminClient.listClustersPagedCallable().futureCall(request);
* // Do something.
* for (Cluster element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listClustersPagedCallable() {
return stub.listClustersPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Clusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListClustersRequest request =
* ListClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListClustersResponse response = alloyDBAdminClient.listClustersCallable().call(request);
* for (Cluster element : response.getClustersList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listClustersCallable() {
return stub.listClustersCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ClusterName name = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]");
* Cluster response = alloyDBAdminClient.getCluster(name);
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Cluster.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Cluster getCluster(ClusterName name) {
GetClusterRequest request =
GetClusterRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getCluster(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String name = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString();
* Cluster response = alloyDBAdminClient.getCluster(name);
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Cluster.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Cluster getCluster(String name) {
GetClusterRequest request = GetClusterRequest.newBuilder().setName(name).build();
return getCluster(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetClusterRequest request =
* GetClusterRequest.newBuilder()
* .setName(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .build();
* Cluster response = alloyDBAdminClient.getCluster(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Cluster getCluster(GetClusterRequest request) {
return getClusterCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetClusterRequest request =
* GetClusterRequest.newBuilder()
* .setName(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .build();
* ApiFuture future = alloyDBAdminClient.getClusterCallable().futureCall(request);
* // Do something.
* Cluster response = future.get();
* }
* }
*/
public final UnaryCallable getClusterCallable() {
return stub.getClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Cluster cluster = Cluster.newBuilder().build();
* String clusterId = "clusterId561939637";
* Cluster response = alloyDBAdminClient.createClusterAsync(parent, cluster, clusterId).get();
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Cluster.name field.
* @param cluster Required. The resource being created
* @param clusterId Required. ID of the requesting object.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createClusterAsync(
LocationName parent, Cluster cluster, String clusterId) {
CreateClusterRequest request =
CreateClusterRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setCluster(cluster)
.setClusterId(clusterId)
.build();
return createClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Cluster cluster = Cluster.newBuilder().build();
* String clusterId = "clusterId561939637";
* Cluster response = alloyDBAdminClient.createClusterAsync(parent, cluster, clusterId).get();
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Cluster.name field.
* @param cluster Required. The resource being created
* @param clusterId Required. ID of the requesting object.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createClusterAsync(
String parent, Cluster cluster, String clusterId) {
CreateClusterRequest request =
CreateClusterRequest.newBuilder()
.setParent(parent)
.setCluster(cluster)
.setClusterId(clusterId)
.build();
return createClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateClusterRequest request =
* CreateClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setClusterId("clusterId561939637")
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* Cluster response = alloyDBAdminClient.createClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createClusterAsync(
CreateClusterRequest request) {
return createClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateClusterRequest request =
* CreateClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setClusterId("clusterId561939637")
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.createClusterOperationCallable().futureCall(request);
* // Do something.
* Cluster response = future.get();
* }
* }
*/
public final OperationCallable
createClusterOperationCallable() {
return stub.createClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateClusterRequest request =
* CreateClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setClusterId("clusterId561939637")
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* ApiFuture future = alloyDBAdminClient.createClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createClusterCallable() {
return stub.createClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* Cluster cluster = Cluster.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Cluster response = alloyDBAdminClient.updateClusterAsync(cluster, updateMask).get();
* }
* }
*
* @param cluster Required. The resource being updated
* @param updateMask Optional. Field mask is used to specify the fields to be overwritten in the
* Cluster resource by the update. The fields specified in the update_mask are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask. If the
* user does not provide a mask then all fields will be overwritten.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateClusterAsync(
Cluster cluster, FieldMask updateMask) {
UpdateClusterRequest request =
UpdateClusterRequest.newBuilder().setCluster(cluster).setUpdateMask(updateMask).build();
return updateClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* UpdateClusterRequest request =
* UpdateClusterRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* Cluster response = alloyDBAdminClient.updateClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateClusterAsync(
UpdateClusterRequest request) {
return updateClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* UpdateClusterRequest request =
* UpdateClusterRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.updateClusterOperationCallable().futureCall(request);
* // Do something.
* Cluster response = future.get();
* }
* }
*/
public final OperationCallable
updateClusterOperationCallable() {
return stub.updateClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* UpdateClusterRequest request =
* UpdateClusterRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* ApiFuture future = alloyDBAdminClient.updateClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateClusterCallable() {
return stub.updateClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ClusterName name = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]");
* alloyDBAdminClient.deleteClusterAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Cluster.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteClusterAsync(ClusterName name) {
DeleteClusterRequest request =
DeleteClusterRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String name = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString();
* alloyDBAdminClient.deleteClusterAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Cluster.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteClusterAsync(String name) {
DeleteClusterRequest request = DeleteClusterRequest.newBuilder().setName(name).build();
return deleteClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* DeleteClusterRequest request =
* DeleteClusterRequest.newBuilder()
* .setName(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setEtag("etag3123477")
* .setValidateOnly(true)
* .setForce(true)
* .build();
* alloyDBAdminClient.deleteClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteClusterAsync(
DeleteClusterRequest request) {
return deleteClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* DeleteClusterRequest request =
* DeleteClusterRequest.newBuilder()
* .setName(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setEtag("etag3123477")
* .setValidateOnly(true)
* .setForce(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.deleteClusterOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteClusterOperationCallable() {
return stub.deleteClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* DeleteClusterRequest request =
* DeleteClusterRequest.newBuilder()
* .setName(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setEtag("etag3123477")
* .setValidateOnly(true)
* .setForce(true)
* .build();
* ApiFuture future = alloyDBAdminClient.deleteClusterCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteClusterCallable() {
return stub.deleteClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Promotes a SECONDARY cluster. This turns down replication from the PRIMARY cluster and promotes
* a secondary cluster into its own standalone cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ClusterName name = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]");
* Cluster response = alloyDBAdminClient.promoteClusterAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Cluster.name field
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture promoteClusterAsync(ClusterName name) {
PromoteClusterRequest request =
PromoteClusterRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return promoteClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Promotes a SECONDARY cluster. This turns down replication from the PRIMARY cluster and promotes
* a secondary cluster into its own standalone cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String name = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString();
* Cluster response = alloyDBAdminClient.promoteClusterAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Cluster.name field
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture promoteClusterAsync(String name) {
PromoteClusterRequest request = PromoteClusterRequest.newBuilder().setName(name).build();
return promoteClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Promotes a SECONDARY cluster. This turns down replication from the PRIMARY cluster and promotes
* a secondary cluster into its own standalone cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* PromoteClusterRequest request =
* PromoteClusterRequest.newBuilder()
* .setName(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setEtag("etag3123477")
* .setValidateOnly(true)
* .build();
* Cluster response = alloyDBAdminClient.promoteClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture promoteClusterAsync(
PromoteClusterRequest request) {
return promoteClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Promotes a SECONDARY cluster. This turns down replication from the PRIMARY cluster and promotes
* a secondary cluster into its own standalone cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* PromoteClusterRequest request =
* PromoteClusterRequest.newBuilder()
* .setName(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setEtag("etag3123477")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.promoteClusterOperationCallable().futureCall(request);
* // Do something.
* Cluster response = future.get();
* }
* }
*/
public final OperationCallable
promoteClusterOperationCallable() {
return stub.promoteClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Promotes a SECONDARY cluster. This turns down replication from the PRIMARY cluster and promotes
* a secondary cluster into its own standalone cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* PromoteClusterRequest request =
* PromoteClusterRequest.newBuilder()
* .setName(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setEtag("etag3123477")
* .setValidateOnly(true)
* .build();
* ApiFuture future = alloyDBAdminClient.promoteClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable promoteClusterCallable() {
return stub.promoteClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Cluster in a given project and location, with a volume restored from the provided
* source, either a backup ID or a point-in-time and a source cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* RestoreClusterRequest request =
* RestoreClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setClusterId("clusterId561939637")
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* Cluster response = alloyDBAdminClient.restoreClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture restoreClusterAsync(
RestoreClusterRequest request) {
return restoreClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Cluster in a given project and location, with a volume restored from the provided
* source, either a backup ID or a point-in-time and a source cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* RestoreClusterRequest request =
* RestoreClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setClusterId("clusterId561939637")
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.restoreClusterOperationCallable().futureCall(request);
* // Do something.
* Cluster response = future.get();
* }
* }
*/
public final OperationCallable
restoreClusterOperationCallable() {
return stub.restoreClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Cluster in a given project and location, with a volume restored from the provided
* source, either a backup ID or a point-in-time and a source cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* RestoreClusterRequest request =
* RestoreClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setClusterId("clusterId561939637")
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* ApiFuture future = alloyDBAdminClient.restoreClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable restoreClusterCallable() {
return stub.restoreClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a cluster of type SECONDARY in the given location using the primary cluster as the
* source.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Cluster cluster = Cluster.newBuilder().build();
* String clusterId = "clusterId561939637";
* Cluster response =
* alloyDBAdminClient.createSecondaryClusterAsync(parent, cluster, clusterId).get();
* }
* }
*
* @param parent Required. The name of the parent resource (the primary cluster). For the required
* format, see the comment on the Cluster.name field.
* @param cluster Required. Configuration of the requesting object (the secondary cluster).
* @param clusterId Required. ID of the requesting object (the secondary cluster).
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createSecondaryClusterAsync(
LocationName parent, Cluster cluster, String clusterId) {
CreateSecondaryClusterRequest request =
CreateSecondaryClusterRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setCluster(cluster)
.setClusterId(clusterId)
.build();
return createSecondaryClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a cluster of type SECONDARY in the given location using the primary cluster as the
* source.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Cluster cluster = Cluster.newBuilder().build();
* String clusterId = "clusterId561939637";
* Cluster response =
* alloyDBAdminClient.createSecondaryClusterAsync(parent, cluster, clusterId).get();
* }
* }
*
* @param parent Required. The name of the parent resource (the primary cluster). For the required
* format, see the comment on the Cluster.name field.
* @param cluster Required. Configuration of the requesting object (the secondary cluster).
* @param clusterId Required. ID of the requesting object (the secondary cluster).
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createSecondaryClusterAsync(
String parent, Cluster cluster, String clusterId) {
CreateSecondaryClusterRequest request =
CreateSecondaryClusterRequest.newBuilder()
.setParent(parent)
.setCluster(cluster)
.setClusterId(clusterId)
.build();
return createSecondaryClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a cluster of type SECONDARY in the given location using the primary cluster as the
* source.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateSecondaryClusterRequest request =
* CreateSecondaryClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setClusterId("clusterId561939637")
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* Cluster response = alloyDBAdminClient.createSecondaryClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createSecondaryClusterAsync(
CreateSecondaryClusterRequest request) {
return createSecondaryClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a cluster of type SECONDARY in the given location using the primary cluster as the
* source.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateSecondaryClusterRequest request =
* CreateSecondaryClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setClusterId("clusterId561939637")
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.createSecondaryClusterOperationCallable().futureCall(request);
* // Do something.
* Cluster response = future.get();
* }
* }
*/
public final OperationCallable
createSecondaryClusterOperationCallable() {
return stub.createSecondaryClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a cluster of type SECONDARY in the given location using the primary cluster as the
* source.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateSecondaryClusterRequest request =
* CreateSecondaryClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setClusterId("clusterId561939637")
* .setCluster(Cluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* ApiFuture future =
* alloyDBAdminClient.createSecondaryClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
createSecondaryClusterCallable() {
return stub.createSecondaryClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Instances in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ClusterName parent = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]");
* for (Instance element : alloyDBAdminClient.listInstances(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Instance.name field. Additionally, you can perform an aggregated list
* operation by specifying a value with one of the following formats: *
* projects/{project}/locations/-/clusters/- *
* projects/{project}/locations/{region}/clusters/-
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInstancesPagedResponse listInstances(ClusterName parent) {
ListInstancesRequest request =
ListInstancesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listInstances(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Instances in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString();
* for (Instance element : alloyDBAdminClient.listInstances(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Instance.name field. Additionally, you can perform an aggregated list
* operation by specifying a value with one of the following formats: *
* projects/{project}/locations/-/clusters/- *
* projects/{project}/locations/{region}/clusters/-
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInstancesPagedResponse listInstances(String parent) {
ListInstancesRequest request = ListInstancesRequest.newBuilder().setParent(parent).build();
return listInstances(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Instances in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListInstancesRequest request =
* ListInstancesRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Instance element : alloyDBAdminClient.listInstances(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInstancesPagedResponse listInstances(ListInstancesRequest request) {
return listInstancesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Instances in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListInstancesRequest request =
* ListInstancesRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* alloyDBAdminClient.listInstancesPagedCallable().futureCall(request);
* // Do something.
* for (Instance element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listInstancesPagedCallable() {
return stub.listInstancesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Instances in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListInstancesRequest request =
* ListInstancesRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListInstancesResponse response = alloyDBAdminClient.listInstancesCallable().call(request);
* for (Instance element : response.getInstancesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listInstancesCallable() {
return stub.listInstancesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* InstanceName name = InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]");
* Instance response = alloyDBAdminClient.getInstance(name);
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Instance.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Instance getInstance(InstanceName name) {
GetInstanceRequest request =
GetInstanceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getInstance(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String name =
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString();
* Instance response = alloyDBAdminClient.getInstance(name);
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Instance.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Instance getInstance(String name) {
GetInstanceRequest request = GetInstanceRequest.newBuilder().setName(name).build();
return getInstance(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetInstanceRequest request =
* GetInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setView(InstanceView.forNumber(0))
* .build();
* Instance response = alloyDBAdminClient.getInstance(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Instance getInstance(GetInstanceRequest request) {
return getInstanceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetInstanceRequest request =
* GetInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setView(InstanceView.forNumber(0))
* .build();
* ApiFuture future = alloyDBAdminClient.getInstanceCallable().futureCall(request);
* // Do something.
* Instance response = future.get();
* }
* }
*/
public final UnaryCallable getInstanceCallable() {
return stub.getInstanceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ClusterName parent = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]");
* Instance instance = Instance.newBuilder().build();
* String instanceId = "instanceId902024336";
* Instance response =
* alloyDBAdminClient.createInstanceAsync(parent, instance, instanceId).get();
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Instance.name field.
* @param instance Required. The resource being created
* @param instanceId Required. ID of the requesting object.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createInstanceAsync(
ClusterName parent, Instance instance, String instanceId) {
CreateInstanceRequest request =
CreateInstanceRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setInstance(instance)
.setInstanceId(instanceId)
.build();
return createInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString();
* Instance instance = Instance.newBuilder().build();
* String instanceId = "instanceId902024336";
* Instance response =
* alloyDBAdminClient.createInstanceAsync(parent, instance, instanceId).get();
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Instance.name field.
* @param instance Required. The resource being created
* @param instanceId Required. ID of the requesting object.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createInstanceAsync(
String parent, Instance instance, String instanceId) {
CreateInstanceRequest request =
CreateInstanceRequest.newBuilder()
.setParent(parent)
.setInstance(instance)
.setInstanceId(instanceId)
.build();
return createInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateInstanceRequest request =
* CreateInstanceRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setInstanceId("instanceId902024336")
* .setInstance(Instance.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* Instance response = alloyDBAdminClient.createInstanceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createInstanceAsync(
CreateInstanceRequest request) {
return createInstanceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateInstanceRequest request =
* CreateInstanceRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setInstanceId("instanceId902024336")
* .setInstance(Instance.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.createInstanceOperationCallable().futureCall(request);
* // Do something.
* Instance response = future.get();
* }
* }
*/
public final OperationCallable
createInstanceOperationCallable() {
return stub.createInstanceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateInstanceRequest request =
* CreateInstanceRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setInstanceId("instanceId902024336")
* .setInstance(Instance.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* ApiFuture future = alloyDBAdminClient.createInstanceCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createInstanceCallable() {
return stub.createInstanceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new SECONDARY Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ClusterName parent = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]");
* Instance instance = Instance.newBuilder().build();
* String instanceId = "instanceId902024336";
* Instance response =
* alloyDBAdminClient.createSecondaryInstanceAsync(parent, instance, instanceId).get();
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Instance.name field.
* @param instance Required. The resource being created
* @param instanceId Required. ID of the requesting object.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createSecondaryInstanceAsync(
ClusterName parent, Instance instance, String instanceId) {
CreateSecondaryInstanceRequest request =
CreateSecondaryInstanceRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setInstance(instance)
.setInstanceId(instanceId)
.build();
return createSecondaryInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new SECONDARY Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString();
* Instance instance = Instance.newBuilder().build();
* String instanceId = "instanceId902024336";
* Instance response =
* alloyDBAdminClient.createSecondaryInstanceAsync(parent, instance, instanceId).get();
* }
* }
*
* @param parent Required. The name of the parent resource. For the required format, see the
* comment on the Instance.name field.
* @param instance Required. The resource being created
* @param instanceId Required. ID of the requesting object.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createSecondaryInstanceAsync(
String parent, Instance instance, String instanceId) {
CreateSecondaryInstanceRequest request =
CreateSecondaryInstanceRequest.newBuilder()
.setParent(parent)
.setInstance(instance)
.setInstanceId(instanceId)
.build();
return createSecondaryInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new SECONDARY Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateSecondaryInstanceRequest request =
* CreateSecondaryInstanceRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setInstanceId("instanceId902024336")
* .setInstance(Instance.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* Instance response = alloyDBAdminClient.createSecondaryInstanceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createSecondaryInstanceAsync(
CreateSecondaryInstanceRequest request) {
return createSecondaryInstanceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new SECONDARY Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateSecondaryInstanceRequest request =
* CreateSecondaryInstanceRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setInstanceId("instanceId902024336")
* .setInstance(Instance.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.createSecondaryInstanceOperationCallable().futureCall(request);
* // Do something.
* Instance response = future.get();
* }
* }
*/
public final OperationCallable
createSecondaryInstanceOperationCallable() {
return stub.createSecondaryInstanceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new SECONDARY Instance in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateSecondaryInstanceRequest request =
* CreateSecondaryInstanceRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setInstanceId("instanceId902024336")
* .setInstance(Instance.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* ApiFuture future =
* alloyDBAdminClient.createSecondaryInstanceCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
createSecondaryInstanceCallable() {
return stub.createSecondaryInstanceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates new instances under the given project, location and cluster. There can be only one
* primary instance in a cluster. If the primary instance exists in the cluster as well as this
* request, then API will throw an error. The primary instance should exist before any read pool
* instance is created. If the primary instance is a part of the request payload, then the API
* will take care of creating instances in the correct order. This method is here to support
* Google-internal use cases, and is not meant for external customers to consume. Please do not
* start relying on it; its behavior is subject to change without notice.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* BatchCreateInstancesRequest request =
* BatchCreateInstancesRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequests(CreateInstanceRequests.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* BatchCreateInstancesResponse response =
* alloyDBAdminClient.batchCreateInstancesAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
batchCreateInstancesAsync(BatchCreateInstancesRequest request) {
return batchCreateInstancesOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates new instances under the given project, location and cluster. There can be only one
* primary instance in a cluster. If the primary instance exists in the cluster as well as this
* request, then API will throw an error. The primary instance should exist before any read pool
* instance is created. If the primary instance is a part of the request payload, then the API
* will take care of creating instances in the correct order. This method is here to support
* Google-internal use cases, and is not meant for external customers to consume. Please do not
* start relying on it; its behavior is subject to change without notice.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* BatchCreateInstancesRequest request =
* BatchCreateInstancesRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequests(CreateInstanceRequests.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* alloyDBAdminClient.batchCreateInstancesOperationCallable().futureCall(request);
* // Do something.
* BatchCreateInstancesResponse response = future.get();
* }
* }
*/
public final OperationCallable<
BatchCreateInstancesRequest, BatchCreateInstancesResponse, OperationMetadata>
batchCreateInstancesOperationCallable() {
return stub.batchCreateInstancesOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates new instances under the given project, location and cluster. There can be only one
* primary instance in a cluster. If the primary instance exists in the cluster as well as this
* request, then API will throw an error. The primary instance should exist before any read pool
* instance is created. If the primary instance is a part of the request payload, then the API
* will take care of creating instances in the correct order. This method is here to support
* Google-internal use cases, and is not meant for external customers to consume. Please do not
* start relying on it; its behavior is subject to change without notice.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* BatchCreateInstancesRequest request =
* BatchCreateInstancesRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequests(CreateInstanceRequests.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* alloyDBAdminClient.batchCreateInstancesCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
batchCreateInstancesCallable() {
return stub.batchCreateInstancesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* Instance instance = Instance.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Instance response = alloyDBAdminClient.updateInstanceAsync(instance, updateMask).get();
* }
* }
*
* @param instance Required. The resource being updated
* @param updateMask Optional. Field mask is used to specify the fields to be overwritten in the
* Instance resource by the update. The fields specified in the update_mask are relative to
* the resource, not the full request. A field will be overwritten if it is in the mask. If
* the user does not provide a mask then all fields will be overwritten.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateInstanceAsync(
Instance instance, FieldMask updateMask) {
UpdateInstanceRequest request =
UpdateInstanceRequest.newBuilder().setInstance(instance).setUpdateMask(updateMask).build();
return updateInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* UpdateInstanceRequest request =
* UpdateInstanceRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setInstance(Instance.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* Instance response = alloyDBAdminClient.updateInstanceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateInstanceAsync(
UpdateInstanceRequest request) {
return updateInstanceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* UpdateInstanceRequest request =
* UpdateInstanceRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setInstance(Instance.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.updateInstanceOperationCallable().futureCall(request);
* // Do something.
* Instance response = future.get();
* }
* }
*/
public final OperationCallable
updateInstanceOperationCallable() {
return stub.updateInstanceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* UpdateInstanceRequest request =
* UpdateInstanceRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setInstance(Instance.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* ApiFuture future = alloyDBAdminClient.updateInstanceCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateInstanceCallable() {
return stub.updateInstanceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* InstanceName name = InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]");
* alloyDBAdminClient.deleteInstanceAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Instance.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteInstanceAsync(InstanceName name) {
DeleteInstanceRequest request =
DeleteInstanceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String name =
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString();
* alloyDBAdminClient.deleteInstanceAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Instance.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteInstanceAsync(String name) {
DeleteInstanceRequest request = DeleteInstanceRequest.newBuilder().setName(name).build();
return deleteInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* DeleteInstanceRequest request =
* DeleteInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .setEtag("etag3123477")
* .setValidateOnly(true)
* .build();
* alloyDBAdminClient.deleteInstanceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteInstanceAsync(
DeleteInstanceRequest request) {
return deleteInstanceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* DeleteInstanceRequest request =
* DeleteInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .setEtag("etag3123477")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.deleteInstanceOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteInstanceOperationCallable() {
return stub.deleteInstanceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* DeleteInstanceRequest request =
* DeleteInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .setEtag("etag3123477")
* .setValidateOnly(true)
* .build();
* ApiFuture future = alloyDBAdminClient.deleteInstanceCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteInstanceCallable() {
return stub.deleteInstanceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Forces a Failover for a highly available instance. Failover promotes the HA standby instance as
* the new primary. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* InstanceName name = InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]");
* Instance response = alloyDBAdminClient.failoverInstanceAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Instance.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture failoverInstanceAsync(
InstanceName name) {
FailoverInstanceRequest request =
FailoverInstanceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return failoverInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Forces a Failover for a highly available instance. Failover promotes the HA standby instance as
* the new primary. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String name =
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString();
* Instance response = alloyDBAdminClient.failoverInstanceAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Instance.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture failoverInstanceAsync(String name) {
FailoverInstanceRequest request = FailoverInstanceRequest.newBuilder().setName(name).build();
return failoverInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Forces a Failover for a highly available instance. Failover promotes the HA standby instance as
* the new primary. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* FailoverInstanceRequest request =
* FailoverInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* Instance response = alloyDBAdminClient.failoverInstanceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture failoverInstanceAsync(
FailoverInstanceRequest request) {
return failoverInstanceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Forces a Failover for a highly available instance. Failover promotes the HA standby instance as
* the new primary. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* FailoverInstanceRequest request =
* FailoverInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.failoverInstanceOperationCallable().futureCall(request);
* // Do something.
* Instance response = future.get();
* }
* }
*/
public final OperationCallable
failoverInstanceOperationCallable() {
return stub.failoverInstanceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Forces a Failover for a highly available instance. Failover promotes the HA standby instance as
* the new primary. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* FailoverInstanceRequest request =
* FailoverInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* ApiFuture future =
* alloyDBAdminClient.failoverInstanceCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable failoverInstanceCallable() {
return stub.failoverInstanceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restart an Instance in a cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* InstanceName name = InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]");
* Instance response = alloyDBAdminClient.restartInstanceAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Instance.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture restartInstanceAsync(
InstanceName name) {
RestartInstanceRequest request =
RestartInstanceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return restartInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restart an Instance in a cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String name =
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString();
* Instance response = alloyDBAdminClient.restartInstanceAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource. For the required format, see the comment on the
* Instance.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture restartInstanceAsync(String name) {
RestartInstanceRequest request = RestartInstanceRequest.newBuilder().setName(name).build();
return restartInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restart an Instance in a cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* RestartInstanceRequest request =
* RestartInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* Instance response = alloyDBAdminClient.restartInstanceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture restartInstanceAsync(
RestartInstanceRequest request) {
return restartInstanceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restart an Instance in a cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* RestartInstanceRequest request =
* RestartInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.restartInstanceOperationCallable().futureCall(request);
* // Do something.
* Instance response = future.get();
* }
* }
*/
public final OperationCallable
restartInstanceOperationCallable() {
return stub.restartInstanceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restart an Instance in a cluster. Imperative only.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* RestartInstanceRequest request =
* RestartInstanceRequest.newBuilder()
* .setName(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* ApiFuture future =
* alloyDBAdminClient.restartInstanceCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable restartInstanceCallable() {
return stub.restartInstanceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Backups in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Backup element : alloyDBAdminClient.listBackups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListBackupsRequest
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupsPagedResponse listBackups(LocationName parent) {
ListBackupsRequest request =
ListBackupsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Backups in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Backup element : alloyDBAdminClient.listBackups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListBackupsRequest
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupsPagedResponse listBackups(String parent) {
ListBackupsRequest request = ListBackupsRequest.newBuilder().setParent(parent).build();
return listBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Backups in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListBackupsRequest request =
* ListBackupsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Backup element : alloyDBAdminClient.listBackups(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupsPagedResponse listBackups(ListBackupsRequest request) {
return listBackupsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Backups in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListBackupsRequest request =
* ListBackupsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future = alloyDBAdminClient.listBackupsPagedCallable().futureCall(request);
* // Do something.
* for (Backup element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listBackupsPagedCallable() {
return stub.listBackupsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Backups in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListBackupsRequest request =
* ListBackupsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListBackupsResponse response = alloyDBAdminClient.listBackupsCallable().call(request);
* for (Backup element : response.getBackupsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listBackupsCallable() {
return stub.listBackupsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* BackupName name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]");
* Backup response = alloyDBAdminClient.getBackup(name);
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Backup getBackup(BackupName name) {
GetBackupRequest request =
GetBackupRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString();
* Backup response = alloyDBAdminClient.getBackup(name);
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Backup getBackup(String name) {
GetBackupRequest request = GetBackupRequest.newBuilder().setName(name).build();
return getBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetBackupRequest request =
* GetBackupRequest.newBuilder()
* .setName(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .build();
* Backup response = alloyDBAdminClient.getBackup(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Backup getBackup(GetBackupRequest request) {
return getBackupCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetBackupRequest request =
* GetBackupRequest.newBuilder()
* .setName(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .build();
* ApiFuture future = alloyDBAdminClient.getBackupCallable().futureCall(request);
* // Do something.
* Backup response = future.get();
* }
* }
*/
public final UnaryCallable getBackupCallable() {
return stub.getBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Backup in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Backup backup = Backup.newBuilder().build();
* String backupId = "backupId2121930365";
* Backup response = alloyDBAdminClient.createBackupAsync(parent, backup, backupId).get();
* }
* }
*
* @param parent Required. Value for parent.
* @param backup Required. The resource being created
* @param backupId Required. ID of the requesting object.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createBackupAsync(
LocationName parent, Backup backup, String backupId) {
CreateBackupRequest request =
CreateBackupRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setBackup(backup)
.setBackupId(backupId)
.build();
return createBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Backup in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Backup backup = Backup.newBuilder().build();
* String backupId = "backupId2121930365";
* Backup response = alloyDBAdminClient.createBackupAsync(parent, backup, backupId).get();
* }
* }
*
* @param parent Required. Value for parent.
* @param backup Required. The resource being created
* @param backupId Required. ID of the requesting object.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createBackupAsync(
String parent, Backup backup, String backupId) {
CreateBackupRequest request =
CreateBackupRequest.newBuilder()
.setParent(parent)
.setBackup(backup)
.setBackupId(backupId)
.build();
return createBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Backup in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateBackupRequest request =
* CreateBackupRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setBackupId("backupId2121930365")
* .setBackup(Backup.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* Backup response = alloyDBAdminClient.createBackupAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createBackupAsync(
CreateBackupRequest request) {
return createBackupOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Backup in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateBackupRequest request =
* CreateBackupRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setBackupId("backupId2121930365")
* .setBackup(Backup.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.createBackupOperationCallable().futureCall(request);
* // Do something.
* Backup response = future.get();
* }
* }
*/
public final OperationCallable
createBackupOperationCallable() {
return stub.createBackupOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Backup in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* CreateBackupRequest request =
* CreateBackupRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setBackupId("backupId2121930365")
* .setBackup(Backup.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .build();
* ApiFuture future = alloyDBAdminClient.createBackupCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createBackupCallable() {
return stub.createBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* Backup backup = Backup.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Backup response = alloyDBAdminClient.updateBackupAsync(backup, updateMask).get();
* }
* }
*
* @param backup Required. The resource being updated
* @param updateMask Optional. Field mask is used to specify the fields to be overwritten in the
* Backup resource by the update. The fields specified in the update_mask are relative to the
* resource, not the full request. A field will be overwritten if it is in the mask. If the
* user does not provide a mask then all fields will be overwritten.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateBackupAsync(
Backup backup, FieldMask updateMask) {
UpdateBackupRequest request =
UpdateBackupRequest.newBuilder().setBackup(backup).setUpdateMask(updateMask).build();
return updateBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* UpdateBackupRequest request =
* UpdateBackupRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setBackup(Backup.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* Backup response = alloyDBAdminClient.updateBackupAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateBackupAsync(
UpdateBackupRequest request) {
return updateBackupOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* UpdateBackupRequest request =
* UpdateBackupRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setBackup(Backup.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* OperationFuture future =
* alloyDBAdminClient.updateBackupOperationCallable().futureCall(request);
* // Do something.
* Backup response = future.get();
* }
* }
*/
public final OperationCallable
updateBackupOperationCallable() {
return stub.updateBackupOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* UpdateBackupRequest request =
* UpdateBackupRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setBackup(Backup.newBuilder().build())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* ApiFuture future = alloyDBAdminClient.updateBackupCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateBackupCallable() {
return stub.updateBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* BackupName name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]");
* alloyDBAdminClient.deleteBackupAsync(name).get();
* }
* }
*
* @param name Required. Name of the resource. For the required format, see the comment on the
* Backup.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteBackupAsync(BackupName name) {
DeleteBackupRequest request =
DeleteBackupRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString();
* alloyDBAdminClient.deleteBackupAsync(name).get();
* }
* }
*
* @param name Required. Name of the resource. For the required format, see the comment on the
* Backup.name field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteBackupAsync(String name) {
DeleteBackupRequest request = DeleteBackupRequest.newBuilder().setName(name).build();
return deleteBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* DeleteBackupRequest request =
* DeleteBackupRequest.newBuilder()
* .setName(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* alloyDBAdminClient.deleteBackupAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteBackupAsync(
DeleteBackupRequest request) {
return deleteBackupOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* DeleteBackupRequest request =
* DeleteBackupRequest.newBuilder()
* .setName(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* OperationFuture future =
* alloyDBAdminClient.deleteBackupOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteBackupOperationCallable() {
return stub.deleteBackupOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* DeleteBackupRequest request =
* DeleteBackupRequest.newBuilder()
* .setName(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .setRequestId("requestId693933066")
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* ApiFuture future = alloyDBAdminClient.deleteBackupCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteBackupCallable() {
return stub.deleteBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists SupportedDatabaseFlags for a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (SupportedDatabaseFlag element :
* alloyDBAdminClient.listSupportedDatabaseFlags(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the parent resource. The required format is: *
* projects/{project}/locations/{location}
* Regardless of the parent specified here, as long it is contains a valid project and
* location, the service will return a static list of supported flags resources. Note that we
* do not yet support region-specific flags.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSupportedDatabaseFlagsPagedResponse listSupportedDatabaseFlags(
LocationName parent) {
ListSupportedDatabaseFlagsRequest request =
ListSupportedDatabaseFlagsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listSupportedDatabaseFlags(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists SupportedDatabaseFlags for a given project and location.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (SupportedDatabaseFlag element :
* alloyDBAdminClient.listSupportedDatabaseFlags(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the parent resource. The required format is: *
* projects/{project}/locations/{location}
* Regardless of the parent specified here, as long it is contains a valid project and
* location, the service will return a static list of supported flags resources. Note that we
* do not yet support region-specific flags.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSupportedDatabaseFlagsPagedResponse listSupportedDatabaseFlags(String parent) {
ListSupportedDatabaseFlagsRequest request =
ListSupportedDatabaseFlagsRequest.newBuilder().setParent(parent).build();
return listSupportedDatabaseFlags(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists SupportedDatabaseFlags for a given project and location.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListSupportedDatabaseFlagsRequest request =
* ListSupportedDatabaseFlagsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (SupportedDatabaseFlag element :
* alloyDBAdminClient.listSupportedDatabaseFlags(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSupportedDatabaseFlagsPagedResponse listSupportedDatabaseFlags(
ListSupportedDatabaseFlagsRequest request) {
return listSupportedDatabaseFlagsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists SupportedDatabaseFlags for a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListSupportedDatabaseFlagsRequest request =
* ListSupportedDatabaseFlagsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* alloyDBAdminClient.listSupportedDatabaseFlagsPagedCallable().futureCall(request);
* // Do something.
* for (SupportedDatabaseFlag element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable<
ListSupportedDatabaseFlagsRequest, ListSupportedDatabaseFlagsPagedResponse>
listSupportedDatabaseFlagsPagedCallable() {
return stub.listSupportedDatabaseFlagsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists SupportedDatabaseFlags for a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListSupportedDatabaseFlagsRequest request =
* ListSupportedDatabaseFlagsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListSupportedDatabaseFlagsResponse response =
* alloyDBAdminClient.listSupportedDatabaseFlagsCallable().call(request);
* for (SupportedDatabaseFlag element : response.getSupportedDatabaseFlagsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listSupportedDatabaseFlagsCallable() {
return stub.listSupportedDatabaseFlagsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generate a client certificate signed by a Cluster CA. The sole purpose of this endpoint is to
* support the Auth Proxy client and the endpoint's behavior is subject to change without notice,
* so do not rely on its behavior remaining constant. Future changes will not break the Auth Proxy
* client.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ClusterName parent = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]");
* GenerateClientCertificateResponse response =
* alloyDBAdminClient.generateClientCertificate(parent);
* }
* }
*
* @param parent Required. The name of the parent resource. The required format is: *
* projects/{project}/locations/{location}/clusters/{cluster}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateClientCertificateResponse generateClientCertificate(ClusterName parent) {
GenerateClientCertificateRequest request =
GenerateClientCertificateRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return generateClientCertificate(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generate a client certificate signed by a Cluster CA. The sole purpose of this endpoint is to
* support the Auth Proxy client and the endpoint's behavior is subject to change without notice,
* so do not rely on its behavior remaining constant. Future changes will not break the Auth Proxy
* client.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent = ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString();
* GenerateClientCertificateResponse response =
* alloyDBAdminClient.generateClientCertificate(parent);
* }
* }
*
* @param parent Required. The name of the parent resource. The required format is: *
* projects/{project}/locations/{location}/clusters/{cluster}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateClientCertificateResponse generateClientCertificate(String parent) {
GenerateClientCertificateRequest request =
GenerateClientCertificateRequest.newBuilder().setParent(parent).build();
return generateClientCertificate(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generate a client certificate signed by a Cluster CA. The sole purpose of this endpoint is to
* support the Auth Proxy client and the endpoint's behavior is subject to change without notice,
* so do not rely on its behavior remaining constant. Future changes will not break the Auth Proxy
* client.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GenerateClientCertificateRequest request =
* GenerateClientCertificateRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setPemCsr("pemCsr-991911478")
* .setCertDuration(Duration.newBuilder().build())
* .build();
* GenerateClientCertificateResponse response =
* alloyDBAdminClient.generateClientCertificate(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateClientCertificateResponse generateClientCertificate(
GenerateClientCertificateRequest request) {
return generateClientCertificateCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generate a client certificate signed by a Cluster CA. The sole purpose of this endpoint is to
* support the Auth Proxy client and the endpoint's behavior is subject to change without notice,
* so do not rely on its behavior remaining constant. Future changes will not break the Auth Proxy
* client.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GenerateClientCertificateRequest request =
* GenerateClientCertificateRequest.newBuilder()
* .setParent(ClusterName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setPemCsr("pemCsr-991911478")
* .setCertDuration(Duration.newBuilder().build())
* .build();
* ApiFuture future =
* alloyDBAdminClient.generateClientCertificateCallable().futureCall(request);
* // Do something.
* GenerateClientCertificateResponse response = future.get();
* }
* }
*/
public final UnaryCallable
generateClientCertificateCallable() {
return stub.generateClientCertificateCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get instance metadata used for a connection.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* InstanceName parent = InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]");
* ConnectionInfo response = alloyDBAdminClient.getConnectionInfo(parent);
* }
* }
*
* @param parent Required. The name of the parent resource. The required format is:
* projects/{project}/locations/{location}/clusters/{cluster}/instances/{instance}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ConnectionInfo getConnectionInfo(InstanceName parent) {
GetConnectionInfoRequest request =
GetConnectionInfoRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return getConnectionInfo(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get instance metadata used for a connection.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* String parent =
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString();
* ConnectionInfo response = alloyDBAdminClient.getConnectionInfo(parent);
* }
* }
*
* @param parent Required. The name of the parent resource. The required format is:
* projects/{project}/locations/{location}/clusters/{cluster}/instances/{instance}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ConnectionInfo getConnectionInfo(String parent) {
GetConnectionInfoRequest request =
GetConnectionInfoRequest.newBuilder().setParent(parent).build();
return getConnectionInfo(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get instance metadata used for a connection.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetConnectionInfoRequest request =
* GetConnectionInfoRequest.newBuilder()
* .setParent(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .build();
* ConnectionInfo response = alloyDBAdminClient.getConnectionInfo(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ConnectionInfo getConnectionInfo(GetConnectionInfoRequest request) {
return getConnectionInfoCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get instance metadata used for a connection.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetConnectionInfoRequest request =
* GetConnectionInfoRequest.newBuilder()
* .setParent(
* InstanceName.of("[PROJECT]", "[LOCATION]", "[CLUSTER]", "[INSTANCE]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* alloyDBAdminClient.getConnectionInfoCallable().futureCall(request);
* // Do something.
* ConnectionInfo response = future.get();
* }
* }
*/
public final UnaryCallable getConnectionInfoCallable() {
return stub.getConnectionInfoCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : alloyDBAdminClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* alloyDBAdminClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response = alloyDBAdminClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = alloyDBAdminClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AlloyDBAdminClient alloyDBAdminClient = AlloyDBAdminClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = alloyDBAdminClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListClustersPagedResponse
extends AbstractPagedListResponse<
ListClustersRequest,
ListClustersResponse,
Cluster,
ListClustersPage,
ListClustersFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListClustersPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListClustersPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListClustersPagedResponse(ListClustersPage page) {
super(page, ListClustersFixedSizeCollection.createEmptyCollection());
}
}
public static class ListClustersPage
extends AbstractPage {
private ListClustersPage(
PageContext context,
ListClustersResponse response) {
super(context, response);
}
private static ListClustersPage createEmptyPage() {
return new ListClustersPage(null, null);
}
@Override
protected ListClustersPage createPage(
PageContext context,
ListClustersResponse response) {
return new ListClustersPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListClustersFixedSizeCollection
extends AbstractFixedSizeCollection<
ListClustersRequest,
ListClustersResponse,
Cluster,
ListClustersPage,
ListClustersFixedSizeCollection> {
private ListClustersFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListClustersFixedSizeCollection createEmptyCollection() {
return new ListClustersFixedSizeCollection(null, 0);
}
@Override
protected ListClustersFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListClustersFixedSizeCollection(pages, collectionSize);
}
}
public static class ListInstancesPagedResponse
extends AbstractPagedListResponse<
ListInstancesRequest,
ListInstancesResponse,
Instance,
ListInstancesPage,
ListInstancesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListInstancesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListInstancesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListInstancesPagedResponse(ListInstancesPage page) {
super(page, ListInstancesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListInstancesPage
extends AbstractPage<
ListInstancesRequest, ListInstancesResponse, Instance, ListInstancesPage> {
private ListInstancesPage(
PageContext context,
ListInstancesResponse response) {
super(context, response);
}
private static ListInstancesPage createEmptyPage() {
return new ListInstancesPage(null, null);
}
@Override
protected ListInstancesPage createPage(
PageContext context,
ListInstancesResponse response) {
return new ListInstancesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListInstancesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListInstancesRequest,
ListInstancesResponse,
Instance,
ListInstancesPage,
ListInstancesFixedSizeCollection> {
private ListInstancesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListInstancesFixedSizeCollection createEmptyCollection() {
return new ListInstancesFixedSizeCollection(null, 0);
}
@Override
protected ListInstancesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListInstancesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListBackupsPagedResponse
extends AbstractPagedListResponse<
ListBackupsRequest,
ListBackupsResponse,
Backup,
ListBackupsPage,
ListBackupsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListBackupsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListBackupsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListBackupsPagedResponse(ListBackupsPage page) {
super(page, ListBackupsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListBackupsPage
extends AbstractPage {
private ListBackupsPage(
PageContext context,
ListBackupsResponse response) {
super(context, response);
}
private static ListBackupsPage createEmptyPage() {
return new ListBackupsPage(null, null);
}
@Override
protected ListBackupsPage createPage(
PageContext context,
ListBackupsResponse response) {
return new ListBackupsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListBackupsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListBackupsRequest,
ListBackupsResponse,
Backup,
ListBackupsPage,
ListBackupsFixedSizeCollection> {
private ListBackupsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListBackupsFixedSizeCollection createEmptyCollection() {
return new ListBackupsFixedSizeCollection(null, 0);
}
@Override
protected ListBackupsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListBackupsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListSupportedDatabaseFlagsPagedResponse
extends AbstractPagedListResponse<
ListSupportedDatabaseFlagsRequest,
ListSupportedDatabaseFlagsResponse,
SupportedDatabaseFlag,
ListSupportedDatabaseFlagsPage,
ListSupportedDatabaseFlagsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListSupportedDatabaseFlagsRequest,
ListSupportedDatabaseFlagsResponse,
SupportedDatabaseFlag>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListSupportedDatabaseFlagsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListSupportedDatabaseFlagsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListSupportedDatabaseFlagsPagedResponse(ListSupportedDatabaseFlagsPage page) {
super(page, ListSupportedDatabaseFlagsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListSupportedDatabaseFlagsPage
extends AbstractPage<
ListSupportedDatabaseFlagsRequest,
ListSupportedDatabaseFlagsResponse,
SupportedDatabaseFlag,
ListSupportedDatabaseFlagsPage> {
private ListSupportedDatabaseFlagsPage(
PageContext<
ListSupportedDatabaseFlagsRequest,
ListSupportedDatabaseFlagsResponse,
SupportedDatabaseFlag>
context,
ListSupportedDatabaseFlagsResponse response) {
super(context, response);
}
private static ListSupportedDatabaseFlagsPage createEmptyPage() {
return new ListSupportedDatabaseFlagsPage(null, null);
}
@Override
protected ListSupportedDatabaseFlagsPage createPage(
PageContext<
ListSupportedDatabaseFlagsRequest,
ListSupportedDatabaseFlagsResponse,
SupportedDatabaseFlag>
context,
ListSupportedDatabaseFlagsResponse response) {
return new ListSupportedDatabaseFlagsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListSupportedDatabaseFlagsRequest,
ListSupportedDatabaseFlagsResponse,
SupportedDatabaseFlag>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListSupportedDatabaseFlagsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListSupportedDatabaseFlagsRequest,
ListSupportedDatabaseFlagsResponse,
SupportedDatabaseFlag,
ListSupportedDatabaseFlagsPage,
ListSupportedDatabaseFlagsFixedSizeCollection> {
private ListSupportedDatabaseFlagsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListSupportedDatabaseFlagsFixedSizeCollection createEmptyCollection() {
return new ListSupportedDatabaseFlagsFixedSizeCollection(null, 0);
}
@Override
protected ListSupportedDatabaseFlagsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListSupportedDatabaseFlagsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}