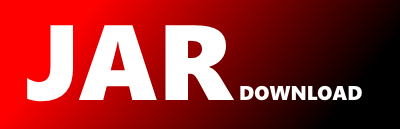
com.google.cloud.alloydb.v1alpha.stub.GrpcAlloyDBAdminStub Maven / Gradle / Ivy
Show all versions of google-cloud-alloydb Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.alloydb.v1alpha.stub;
import static com.google.cloud.alloydb.v1alpha.AlloyDBAdminClient.ListBackupsPagedResponse;
import static com.google.cloud.alloydb.v1alpha.AlloyDBAdminClient.ListClustersPagedResponse;
import static com.google.cloud.alloydb.v1alpha.AlloyDBAdminClient.ListDatabasesPagedResponse;
import static com.google.cloud.alloydb.v1alpha.AlloyDBAdminClient.ListInstancesPagedResponse;
import static com.google.cloud.alloydb.v1alpha.AlloyDBAdminClient.ListLocationsPagedResponse;
import static com.google.cloud.alloydb.v1alpha.AlloyDBAdminClient.ListSupportedDatabaseFlagsPagedResponse;
import static com.google.cloud.alloydb.v1alpha.AlloyDBAdminClient.ListUsersPagedResponse;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.alloydb.v1alpha.Backup;
import com.google.cloud.alloydb.v1alpha.BatchCreateInstancesRequest;
import com.google.cloud.alloydb.v1alpha.BatchCreateInstancesResponse;
import com.google.cloud.alloydb.v1alpha.Cluster;
import com.google.cloud.alloydb.v1alpha.ConnectionInfo;
import com.google.cloud.alloydb.v1alpha.CreateBackupRequest;
import com.google.cloud.alloydb.v1alpha.CreateClusterRequest;
import com.google.cloud.alloydb.v1alpha.CreateInstanceRequest;
import com.google.cloud.alloydb.v1alpha.CreateSecondaryClusterRequest;
import com.google.cloud.alloydb.v1alpha.CreateSecondaryInstanceRequest;
import com.google.cloud.alloydb.v1alpha.CreateUserRequest;
import com.google.cloud.alloydb.v1alpha.DeleteBackupRequest;
import com.google.cloud.alloydb.v1alpha.DeleteClusterRequest;
import com.google.cloud.alloydb.v1alpha.DeleteInstanceRequest;
import com.google.cloud.alloydb.v1alpha.DeleteUserRequest;
import com.google.cloud.alloydb.v1alpha.FailoverInstanceRequest;
import com.google.cloud.alloydb.v1alpha.GenerateClientCertificateRequest;
import com.google.cloud.alloydb.v1alpha.GenerateClientCertificateResponse;
import com.google.cloud.alloydb.v1alpha.GetBackupRequest;
import com.google.cloud.alloydb.v1alpha.GetClusterRequest;
import com.google.cloud.alloydb.v1alpha.GetConnectionInfoRequest;
import com.google.cloud.alloydb.v1alpha.GetInstanceRequest;
import com.google.cloud.alloydb.v1alpha.GetUserRequest;
import com.google.cloud.alloydb.v1alpha.InjectFaultRequest;
import com.google.cloud.alloydb.v1alpha.Instance;
import com.google.cloud.alloydb.v1alpha.ListBackupsRequest;
import com.google.cloud.alloydb.v1alpha.ListBackupsResponse;
import com.google.cloud.alloydb.v1alpha.ListClustersRequest;
import com.google.cloud.alloydb.v1alpha.ListClustersResponse;
import com.google.cloud.alloydb.v1alpha.ListDatabasesRequest;
import com.google.cloud.alloydb.v1alpha.ListDatabasesResponse;
import com.google.cloud.alloydb.v1alpha.ListInstancesRequest;
import com.google.cloud.alloydb.v1alpha.ListInstancesResponse;
import com.google.cloud.alloydb.v1alpha.ListSupportedDatabaseFlagsRequest;
import com.google.cloud.alloydb.v1alpha.ListSupportedDatabaseFlagsResponse;
import com.google.cloud.alloydb.v1alpha.ListUsersRequest;
import com.google.cloud.alloydb.v1alpha.ListUsersResponse;
import com.google.cloud.alloydb.v1alpha.OperationMetadata;
import com.google.cloud.alloydb.v1alpha.PromoteClusterRequest;
import com.google.cloud.alloydb.v1alpha.RestartInstanceRequest;
import com.google.cloud.alloydb.v1alpha.RestoreClusterRequest;
import com.google.cloud.alloydb.v1alpha.UpdateBackupRequest;
import com.google.cloud.alloydb.v1alpha.UpdateClusterRequest;
import com.google.cloud.alloydb.v1alpha.UpdateInstanceRequest;
import com.google.cloud.alloydb.v1alpha.UpdateUserRequest;
import com.google.cloud.alloydb.v1alpha.User;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import com.google.protobuf.Empty;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the AlloyDBAdmin service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class GrpcAlloyDBAdminStub extends AlloyDBAdminStub {
private static final MethodDescriptor
listClustersMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/ListClusters")
.setRequestMarshaller(ProtoUtils.marshaller(ListClustersRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListClustersResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getClusterMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/GetCluster")
.setRequestMarshaller(ProtoUtils.marshaller(GetClusterRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Cluster.getDefaultInstance()))
.build();
private static final MethodDescriptor
createClusterMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/CreateCluster")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateClusterRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateClusterMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/UpdateCluster")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateClusterRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteClusterMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/DeleteCluster")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteClusterRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
promoteClusterMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/PromoteCluster")
.setRequestMarshaller(
ProtoUtils.marshaller(PromoteClusterRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
restoreClusterMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/RestoreCluster")
.setRequestMarshaller(
ProtoUtils.marshaller(RestoreClusterRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
createSecondaryClusterMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/CreateSecondaryCluster")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateSecondaryClusterRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listInstancesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/ListInstances")
.setRequestMarshaller(
ProtoUtils.marshaller(ListInstancesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListInstancesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/GetInstance")
.setRequestMarshaller(ProtoUtils.marshaller(GetInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Instance.getDefaultInstance()))
.build();
private static final MethodDescriptor
createInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/CreateInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
createSecondaryInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.alloydb.v1alpha.AlloyDBAdmin/CreateSecondaryInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateSecondaryInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
batchCreateInstancesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/BatchCreateInstances")
.setRequestMarshaller(
ProtoUtils.marshaller(BatchCreateInstancesRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/UpdateInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/DeleteInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
failoverInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/FailoverInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(FailoverInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor injectFaultMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/InjectFault")
.setRequestMarshaller(ProtoUtils.marshaller(InjectFaultRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
restartInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/RestartInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(RestartInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listBackupsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/ListBackups")
.setRequestMarshaller(ProtoUtils.marshaller(ListBackupsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListBackupsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getBackupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/GetBackup")
.setRequestMarshaller(ProtoUtils.marshaller(GetBackupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Backup.getDefaultInstance()))
.build();
private static final MethodDescriptor
createBackupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/CreateBackup")
.setRequestMarshaller(ProtoUtils.marshaller(CreateBackupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateBackupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/UpdateBackup")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateBackupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteBackupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/DeleteBackup")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteBackupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor<
ListSupportedDatabaseFlagsRequest, ListSupportedDatabaseFlagsResponse>
listSupportedDatabaseFlagsMethodDescriptor =
MethodDescriptor
.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.alloydb.v1alpha.AlloyDBAdmin/ListSupportedDatabaseFlags")
.setRequestMarshaller(
ProtoUtils.marshaller(ListSupportedDatabaseFlagsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListSupportedDatabaseFlagsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor<
GenerateClientCertificateRequest, GenerateClientCertificateResponse>
generateClientCertificateMethodDescriptor =
MethodDescriptor
.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.alloydb.v1alpha.AlloyDBAdmin/GenerateClientCertificate")
.setRequestMarshaller(
ProtoUtils.marshaller(GenerateClientCertificateRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(GenerateClientCertificateResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getConnectionInfoMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/GetConnectionInfo")
.setRequestMarshaller(
ProtoUtils.marshaller(GetConnectionInfoRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ConnectionInfo.getDefaultInstance()))
.build();
private static final MethodDescriptor
listUsersMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/ListUsers")
.setRequestMarshaller(ProtoUtils.marshaller(ListUsersRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListUsersResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getUserMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/GetUser")
.setRequestMarshaller(ProtoUtils.marshaller(GetUserRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(User.getDefaultInstance()))
.build();
private static final MethodDescriptor createUserMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/CreateUser")
.setRequestMarshaller(ProtoUtils.marshaller(CreateUserRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(User.getDefaultInstance()))
.build();
private static final MethodDescriptor updateUserMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/UpdateUser")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateUserRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(User.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteUserMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/DeleteUser")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteUserRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
listDatabasesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.alloydb.v1alpha.AlloyDBAdmin/ListDatabases")
.setRequestMarshaller(
ProtoUtils.marshaller(ListDatabasesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListDatabasesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
listLocationsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/ListLocations")
.setRequestMarshaller(
ProtoUtils.marshaller(ListLocationsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListLocationsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getLocationMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/GetLocation")
.setRequestMarshaller(ProtoUtils.marshaller(GetLocationRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Location.getDefaultInstance()))
.build();
private final UnaryCallable listClustersCallable;
private final UnaryCallable
listClustersPagedCallable;
private final UnaryCallable getClusterCallable;
private final UnaryCallable createClusterCallable;
private final OperationCallable
createClusterOperationCallable;
private final UnaryCallable updateClusterCallable;
private final OperationCallable
updateClusterOperationCallable;
private final UnaryCallable deleteClusterCallable;
private final OperationCallable
deleteClusterOperationCallable;
private final UnaryCallable promoteClusterCallable;
private final OperationCallable
promoteClusterOperationCallable;
private final UnaryCallable restoreClusterCallable;
private final OperationCallable
restoreClusterOperationCallable;
private final UnaryCallable
createSecondaryClusterCallable;
private final OperationCallable
createSecondaryClusterOperationCallable;
private final UnaryCallable listInstancesCallable;
private final UnaryCallable
listInstancesPagedCallable;
private final UnaryCallable getInstanceCallable;
private final UnaryCallable createInstanceCallable;
private final OperationCallable
createInstanceOperationCallable;
private final UnaryCallable
createSecondaryInstanceCallable;
private final OperationCallable
createSecondaryInstanceOperationCallable;
private final UnaryCallable batchCreateInstancesCallable;
private final OperationCallable<
BatchCreateInstancesRequest, BatchCreateInstancesResponse, OperationMetadata>
batchCreateInstancesOperationCallable;
private final UnaryCallable updateInstanceCallable;
private final OperationCallable
updateInstanceOperationCallable;
private final UnaryCallable deleteInstanceCallable;
private final OperationCallable
deleteInstanceOperationCallable;
private final UnaryCallable failoverInstanceCallable;
private final OperationCallable
failoverInstanceOperationCallable;
private final UnaryCallable injectFaultCallable;
private final OperationCallable
injectFaultOperationCallable;
private final UnaryCallable restartInstanceCallable;
private final OperationCallable
restartInstanceOperationCallable;
private final UnaryCallable listBackupsCallable;
private final UnaryCallable
listBackupsPagedCallable;
private final UnaryCallable getBackupCallable;
private final UnaryCallable createBackupCallable;
private final OperationCallable
createBackupOperationCallable;
private final UnaryCallable updateBackupCallable;
private final OperationCallable
updateBackupOperationCallable;
private final UnaryCallable deleteBackupCallable;
private final OperationCallable
deleteBackupOperationCallable;
private final UnaryCallable
listSupportedDatabaseFlagsCallable;
private final UnaryCallable<
ListSupportedDatabaseFlagsRequest, ListSupportedDatabaseFlagsPagedResponse>
listSupportedDatabaseFlagsPagedCallable;
private final UnaryCallable
generateClientCertificateCallable;
private final UnaryCallable getConnectionInfoCallable;
private final UnaryCallable listUsersCallable;
private final UnaryCallable listUsersPagedCallable;
private final UnaryCallable getUserCallable;
private final UnaryCallable createUserCallable;
private final UnaryCallable updateUserCallable;
private final UnaryCallable deleteUserCallable;
private final UnaryCallable listDatabasesCallable;
private final UnaryCallable
listDatabasesPagedCallable;
private final UnaryCallable listLocationsCallable;
private final UnaryCallable
listLocationsPagedCallable;
private final UnaryCallable getLocationCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcAlloyDBAdminStub create(AlloyDBAdminStubSettings settings)
throws IOException {
return new GrpcAlloyDBAdminStub(settings, ClientContext.create(settings));
}
public static final GrpcAlloyDBAdminStub create(ClientContext clientContext) throws IOException {
return new GrpcAlloyDBAdminStub(AlloyDBAdminStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcAlloyDBAdminStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcAlloyDBAdminStub(
AlloyDBAdminStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcAlloyDBAdminStub, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcAlloyDBAdminStub(AlloyDBAdminStubSettings settings, ClientContext clientContext)
throws IOException {
this(settings, clientContext, new GrpcAlloyDBAdminCallableFactory());
}
/**
* Constructs an instance of GrpcAlloyDBAdminStub, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcAlloyDBAdminStub(
AlloyDBAdminStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings listClustersTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listClustersMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getClusterTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getClusterMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createClusterTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createClusterMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateClusterTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateClusterMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("cluster.name", String.valueOf(request.getCluster().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteClusterTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteClusterMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings promoteClusterTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(promoteClusterMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings restoreClusterTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(restoreClusterMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
createSecondaryClusterTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createSecondaryClusterMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings listInstancesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listInstancesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
createSecondaryInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createSecondaryInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings batchCreateInstancesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(batchCreateInstancesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("instance.name", String.valueOf(request.getInstance().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings failoverInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(failoverInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings injectFaultTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(injectFaultMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings restartInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(restartInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listBackupsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listBackupsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getBackupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getBackupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createBackupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createBackupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateBackupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateBackupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("backup.name", String.valueOf(request.getBackup().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteBackupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteBackupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listSupportedDatabaseFlagsTransportSettings =
GrpcCallSettings
.newBuilder()
.setMethodDescriptor(listSupportedDatabaseFlagsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
generateClientCertificateTransportSettings =
GrpcCallSettings
.newBuilder()
.setMethodDescriptor(generateClientCertificateMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getConnectionInfoTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getConnectionInfoMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings listUsersTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listUsersMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getUserTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getUserMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createUserTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createUserMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateUserTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateUserMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("user.name", String.valueOf(request.getUser().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteUserTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteUserMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listDatabasesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listDatabasesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings listLocationsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listLocationsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getLocationTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getLocationMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
this.listClustersCallable =
callableFactory.createUnaryCallable(
listClustersTransportSettings, settings.listClustersSettings(), clientContext);
this.listClustersPagedCallable =
callableFactory.createPagedCallable(
listClustersTransportSettings, settings.listClustersSettings(), clientContext);
this.getClusterCallable =
callableFactory.createUnaryCallable(
getClusterTransportSettings, settings.getClusterSettings(), clientContext);
this.createClusterCallable =
callableFactory.createUnaryCallable(
createClusterTransportSettings, settings.createClusterSettings(), clientContext);
this.createClusterOperationCallable =
callableFactory.createOperationCallable(
createClusterTransportSettings,
settings.createClusterOperationSettings(),
clientContext,
operationsStub);
this.updateClusterCallable =
callableFactory.createUnaryCallable(
updateClusterTransportSettings, settings.updateClusterSettings(), clientContext);
this.updateClusterOperationCallable =
callableFactory.createOperationCallable(
updateClusterTransportSettings,
settings.updateClusterOperationSettings(),
clientContext,
operationsStub);
this.deleteClusterCallable =
callableFactory.createUnaryCallable(
deleteClusterTransportSettings, settings.deleteClusterSettings(), clientContext);
this.deleteClusterOperationCallable =
callableFactory.createOperationCallable(
deleteClusterTransportSettings,
settings.deleteClusterOperationSettings(),
clientContext,
operationsStub);
this.promoteClusterCallable =
callableFactory.createUnaryCallable(
promoteClusterTransportSettings, settings.promoteClusterSettings(), clientContext);
this.promoteClusterOperationCallable =
callableFactory.createOperationCallable(
promoteClusterTransportSettings,
settings.promoteClusterOperationSettings(),
clientContext,
operationsStub);
this.restoreClusterCallable =
callableFactory.createUnaryCallable(
restoreClusterTransportSettings, settings.restoreClusterSettings(), clientContext);
this.restoreClusterOperationCallable =
callableFactory.createOperationCallable(
restoreClusterTransportSettings,
settings.restoreClusterOperationSettings(),
clientContext,
operationsStub);
this.createSecondaryClusterCallable =
callableFactory.createUnaryCallable(
createSecondaryClusterTransportSettings,
settings.createSecondaryClusterSettings(),
clientContext);
this.createSecondaryClusterOperationCallable =
callableFactory.createOperationCallable(
createSecondaryClusterTransportSettings,
settings.createSecondaryClusterOperationSettings(),
clientContext,
operationsStub);
this.listInstancesCallable =
callableFactory.createUnaryCallable(
listInstancesTransportSettings, settings.listInstancesSettings(), clientContext);
this.listInstancesPagedCallable =
callableFactory.createPagedCallable(
listInstancesTransportSettings, settings.listInstancesSettings(), clientContext);
this.getInstanceCallable =
callableFactory.createUnaryCallable(
getInstanceTransportSettings, settings.getInstanceSettings(), clientContext);
this.createInstanceCallable =
callableFactory.createUnaryCallable(
createInstanceTransportSettings, settings.createInstanceSettings(), clientContext);
this.createInstanceOperationCallable =
callableFactory.createOperationCallable(
createInstanceTransportSettings,
settings.createInstanceOperationSettings(),
clientContext,
operationsStub);
this.createSecondaryInstanceCallable =
callableFactory.createUnaryCallable(
createSecondaryInstanceTransportSettings,
settings.createSecondaryInstanceSettings(),
clientContext);
this.createSecondaryInstanceOperationCallable =
callableFactory.createOperationCallable(
createSecondaryInstanceTransportSettings,
settings.createSecondaryInstanceOperationSettings(),
clientContext,
operationsStub);
this.batchCreateInstancesCallable =
callableFactory.createUnaryCallable(
batchCreateInstancesTransportSettings,
settings.batchCreateInstancesSettings(),
clientContext);
this.batchCreateInstancesOperationCallable =
callableFactory.createOperationCallable(
batchCreateInstancesTransportSettings,
settings.batchCreateInstancesOperationSettings(),
clientContext,
operationsStub);
this.updateInstanceCallable =
callableFactory.createUnaryCallable(
updateInstanceTransportSettings, settings.updateInstanceSettings(), clientContext);
this.updateInstanceOperationCallable =
callableFactory.createOperationCallable(
updateInstanceTransportSettings,
settings.updateInstanceOperationSettings(),
clientContext,
operationsStub);
this.deleteInstanceCallable =
callableFactory.createUnaryCallable(
deleteInstanceTransportSettings, settings.deleteInstanceSettings(), clientContext);
this.deleteInstanceOperationCallable =
callableFactory.createOperationCallable(
deleteInstanceTransportSettings,
settings.deleteInstanceOperationSettings(),
clientContext,
operationsStub);
this.failoverInstanceCallable =
callableFactory.createUnaryCallable(
failoverInstanceTransportSettings, settings.failoverInstanceSettings(), clientContext);
this.failoverInstanceOperationCallable =
callableFactory.createOperationCallable(
failoverInstanceTransportSettings,
settings.failoverInstanceOperationSettings(),
clientContext,
operationsStub);
this.injectFaultCallable =
callableFactory.createUnaryCallable(
injectFaultTransportSettings, settings.injectFaultSettings(), clientContext);
this.injectFaultOperationCallable =
callableFactory.createOperationCallable(
injectFaultTransportSettings,
settings.injectFaultOperationSettings(),
clientContext,
operationsStub);
this.restartInstanceCallable =
callableFactory.createUnaryCallable(
restartInstanceTransportSettings, settings.restartInstanceSettings(), clientContext);
this.restartInstanceOperationCallable =
callableFactory.createOperationCallable(
restartInstanceTransportSettings,
settings.restartInstanceOperationSettings(),
clientContext,
operationsStub);
this.listBackupsCallable =
callableFactory.createUnaryCallable(
listBackupsTransportSettings, settings.listBackupsSettings(), clientContext);
this.listBackupsPagedCallable =
callableFactory.createPagedCallable(
listBackupsTransportSettings, settings.listBackupsSettings(), clientContext);
this.getBackupCallable =
callableFactory.createUnaryCallable(
getBackupTransportSettings, settings.getBackupSettings(), clientContext);
this.createBackupCallable =
callableFactory.createUnaryCallable(
createBackupTransportSettings, settings.createBackupSettings(), clientContext);
this.createBackupOperationCallable =
callableFactory.createOperationCallable(
createBackupTransportSettings,
settings.createBackupOperationSettings(),
clientContext,
operationsStub);
this.updateBackupCallable =
callableFactory.createUnaryCallable(
updateBackupTransportSettings, settings.updateBackupSettings(), clientContext);
this.updateBackupOperationCallable =
callableFactory.createOperationCallable(
updateBackupTransportSettings,
settings.updateBackupOperationSettings(),
clientContext,
operationsStub);
this.deleteBackupCallable =
callableFactory.createUnaryCallable(
deleteBackupTransportSettings, settings.deleteBackupSettings(), clientContext);
this.deleteBackupOperationCallable =
callableFactory.createOperationCallable(
deleteBackupTransportSettings,
settings.deleteBackupOperationSettings(),
clientContext,
operationsStub);
this.listSupportedDatabaseFlagsCallable =
callableFactory.createUnaryCallable(
listSupportedDatabaseFlagsTransportSettings,
settings.listSupportedDatabaseFlagsSettings(),
clientContext);
this.listSupportedDatabaseFlagsPagedCallable =
callableFactory.createPagedCallable(
listSupportedDatabaseFlagsTransportSettings,
settings.listSupportedDatabaseFlagsSettings(),
clientContext);
this.generateClientCertificateCallable =
callableFactory.createUnaryCallable(
generateClientCertificateTransportSettings,
settings.generateClientCertificateSettings(),
clientContext);
this.getConnectionInfoCallable =
callableFactory.createUnaryCallable(
getConnectionInfoTransportSettings,
settings.getConnectionInfoSettings(),
clientContext);
this.listUsersCallable =
callableFactory.createUnaryCallable(
listUsersTransportSettings, settings.listUsersSettings(), clientContext);
this.listUsersPagedCallable =
callableFactory.createPagedCallable(
listUsersTransportSettings, settings.listUsersSettings(), clientContext);
this.getUserCallable =
callableFactory.createUnaryCallable(
getUserTransportSettings, settings.getUserSettings(), clientContext);
this.createUserCallable =
callableFactory.createUnaryCallable(
createUserTransportSettings, settings.createUserSettings(), clientContext);
this.updateUserCallable =
callableFactory.createUnaryCallable(
updateUserTransportSettings, settings.updateUserSettings(), clientContext);
this.deleteUserCallable =
callableFactory.createUnaryCallable(
deleteUserTransportSettings, settings.deleteUserSettings(), clientContext);
this.listDatabasesCallable =
callableFactory.createUnaryCallable(
listDatabasesTransportSettings, settings.listDatabasesSettings(), clientContext);
this.listDatabasesPagedCallable =
callableFactory.createPagedCallable(
listDatabasesTransportSettings, settings.listDatabasesSettings(), clientContext);
this.listLocationsCallable =
callableFactory.createUnaryCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.listLocationsPagedCallable =
callableFactory.createPagedCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.getLocationCallable =
callableFactory.createUnaryCallable(
getLocationTransportSettings, settings.getLocationSettings(), clientContext);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable listClustersCallable() {
return listClustersCallable;
}
@Override
public UnaryCallable listClustersPagedCallable() {
return listClustersPagedCallable;
}
@Override
public UnaryCallable getClusterCallable() {
return getClusterCallable;
}
@Override
public UnaryCallable createClusterCallable() {
return createClusterCallable;
}
@Override
public OperationCallable
createClusterOperationCallable() {
return createClusterOperationCallable;
}
@Override
public UnaryCallable updateClusterCallable() {
return updateClusterCallable;
}
@Override
public OperationCallable
updateClusterOperationCallable() {
return updateClusterOperationCallable;
}
@Override
public UnaryCallable deleteClusterCallable() {
return deleteClusterCallable;
}
@Override
public OperationCallable
deleteClusterOperationCallable() {
return deleteClusterOperationCallable;
}
@Override
public UnaryCallable promoteClusterCallable() {
return promoteClusterCallable;
}
@Override
public OperationCallable
promoteClusterOperationCallable() {
return promoteClusterOperationCallable;
}
@Override
public UnaryCallable restoreClusterCallable() {
return restoreClusterCallable;
}
@Override
public OperationCallable
restoreClusterOperationCallable() {
return restoreClusterOperationCallable;
}
@Override
public UnaryCallable createSecondaryClusterCallable() {
return createSecondaryClusterCallable;
}
@Override
public OperationCallable
createSecondaryClusterOperationCallable() {
return createSecondaryClusterOperationCallable;
}
@Override
public UnaryCallable listInstancesCallable() {
return listInstancesCallable;
}
@Override
public UnaryCallable
listInstancesPagedCallable() {
return listInstancesPagedCallable;
}
@Override
public UnaryCallable getInstanceCallable() {
return getInstanceCallable;
}
@Override
public UnaryCallable createInstanceCallable() {
return createInstanceCallable;
}
@Override
public OperationCallable
createInstanceOperationCallable() {
return createInstanceOperationCallable;
}
@Override
public UnaryCallable
createSecondaryInstanceCallable() {
return createSecondaryInstanceCallable;
}
@Override
public OperationCallable
createSecondaryInstanceOperationCallable() {
return createSecondaryInstanceOperationCallable;
}
@Override
public UnaryCallable batchCreateInstancesCallable() {
return batchCreateInstancesCallable;
}
@Override
public OperationCallable<
BatchCreateInstancesRequest, BatchCreateInstancesResponse, OperationMetadata>
batchCreateInstancesOperationCallable() {
return batchCreateInstancesOperationCallable;
}
@Override
public UnaryCallable updateInstanceCallable() {
return updateInstanceCallable;
}
@Override
public OperationCallable
updateInstanceOperationCallable() {
return updateInstanceOperationCallable;
}
@Override
public UnaryCallable deleteInstanceCallable() {
return deleteInstanceCallable;
}
@Override
public OperationCallable
deleteInstanceOperationCallable() {
return deleteInstanceOperationCallable;
}
@Override
public UnaryCallable failoverInstanceCallable() {
return failoverInstanceCallable;
}
@Override
public OperationCallable
failoverInstanceOperationCallable() {
return failoverInstanceOperationCallable;
}
@Override
public UnaryCallable injectFaultCallable() {
return injectFaultCallable;
}
@Override
public OperationCallable
injectFaultOperationCallable() {
return injectFaultOperationCallable;
}
@Override
public UnaryCallable restartInstanceCallable() {
return restartInstanceCallable;
}
@Override
public OperationCallable
restartInstanceOperationCallable() {
return restartInstanceOperationCallable;
}
@Override
public UnaryCallable listBackupsCallable() {
return listBackupsCallable;
}
@Override
public UnaryCallable listBackupsPagedCallable() {
return listBackupsPagedCallable;
}
@Override
public UnaryCallable getBackupCallable() {
return getBackupCallable;
}
@Override
public UnaryCallable createBackupCallable() {
return createBackupCallable;
}
@Override
public OperationCallable
createBackupOperationCallable() {
return createBackupOperationCallable;
}
@Override
public UnaryCallable updateBackupCallable() {
return updateBackupCallable;
}
@Override
public OperationCallable
updateBackupOperationCallable() {
return updateBackupOperationCallable;
}
@Override
public UnaryCallable deleteBackupCallable() {
return deleteBackupCallable;
}
@Override
public OperationCallable
deleteBackupOperationCallable() {
return deleteBackupOperationCallable;
}
@Override
public UnaryCallable
listSupportedDatabaseFlagsCallable() {
return listSupportedDatabaseFlagsCallable;
}
@Override
public UnaryCallable
listSupportedDatabaseFlagsPagedCallable() {
return listSupportedDatabaseFlagsPagedCallable;
}
@Override
public UnaryCallable
generateClientCertificateCallable() {
return generateClientCertificateCallable;
}
@Override
public UnaryCallable getConnectionInfoCallable() {
return getConnectionInfoCallable;
}
@Override
public UnaryCallable listUsersCallable() {
return listUsersCallable;
}
@Override
public UnaryCallable listUsersPagedCallable() {
return listUsersPagedCallable;
}
@Override
public UnaryCallable getUserCallable() {
return getUserCallable;
}
@Override
public UnaryCallable createUserCallable() {
return createUserCallable;
}
@Override
public UnaryCallable updateUserCallable() {
return updateUserCallable;
}
@Override
public UnaryCallable deleteUserCallable() {
return deleteUserCallable;
}
@Override
public UnaryCallable listDatabasesCallable() {
return listDatabasesCallable;
}
@Override
public UnaryCallable
listDatabasesPagedCallable() {
return listDatabasesPagedCallable;
}
@Override
public UnaryCallable listLocationsCallable() {
return listLocationsCallable;
}
@Override
public UnaryCallable
listLocationsPagedCallable() {
return listLocationsPagedCallable;
}
@Override
public UnaryCallable getLocationCallable() {
return getLocationCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}