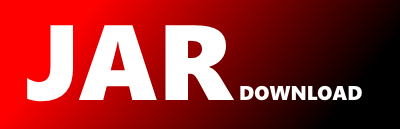
com.google.cloud.bigquery.analyticshub.v1.AnalyticsHubServiceClient Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.bigquery.analyticshub.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.api.resourcenames.ResourceName;
import com.google.cloud.bigquery.analyticshub.v1.stub.AnalyticsHubServiceStub;
import com.google.cloud.bigquery.analyticshub.v1.stub.AnalyticsHubServiceStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: The `AnalyticsHubService` API facilitates data sharing within and across
* organizations. It allows data providers to publish listings that reference shared datasets. With
* Analytics Hub, users can discover and search for listings that they have access to. Subscribers
* can view and subscribe to listings. When you subscribe to a listing, Analytics Hub creates a
* linked dataset in your project.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DataExchangeName name = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]");
* DataExchange response = analyticsHubServiceClient.getDataExchange(name);
* }
* }
*
* Note: close() needs to be called on the AnalyticsHubServiceClient object to clean up resources
* such as threads. In the example above, try-with-resources is used, which automatically calls
* close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* ListDataExchanges
* Lists all data exchanges in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listDataExchanges(ListDataExchangesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listDataExchanges(LocationName parent)
*
listDataExchanges(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listDataExchangesPagedCallable()
*
listDataExchangesCallable()
*
*
*
*
* ListOrgDataExchanges
* Lists all data exchanges from projects in a given organization and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listOrgDataExchanges(ListOrgDataExchangesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listOrgDataExchanges(String organization)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listOrgDataExchangesPagedCallable()
*
listOrgDataExchangesCallable()
*
*
*
*
* GetDataExchange
* Gets the details of a data exchange.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getDataExchange(GetDataExchangeRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getDataExchange(DataExchangeName name)
*
getDataExchange(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getDataExchangeCallable()
*
*
*
*
* CreateDataExchange
* Creates a new data exchange.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createDataExchange(CreateDataExchangeRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createDataExchange(LocationName parent, DataExchange dataExchange)
*
createDataExchange(String parent, DataExchange dataExchange)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createDataExchangeCallable()
*
*
*
*
* UpdateDataExchange
* Updates an existing data exchange.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateDataExchange(UpdateDataExchangeRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateDataExchange(DataExchange dataExchange, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateDataExchangeCallable()
*
*
*
*
* DeleteDataExchange
* Deletes an existing data exchange.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteDataExchange(DeleteDataExchangeRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteDataExchange(DataExchangeName name)
*
deleteDataExchange(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteDataExchangeCallable()
*
*
*
*
* ListListings
* Lists all listings in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listListings(ListListingsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listListings(DataExchangeName parent)
*
listListings(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listListingsPagedCallable()
*
listListingsCallable()
*
*
*
*
* GetListing
* Gets the details of a listing.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getListing(GetListingRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getListing(ListingName name)
*
getListing(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getListingCallable()
*
*
*
*
* CreateListing
* Creates a new listing.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createListing(CreateListingRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createListing(DataExchangeName parent, Listing listing)
*
createListing(String parent, Listing listing)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createListingCallable()
*
*
*
*
* UpdateListing
* Updates an existing listing.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateListing(UpdateListingRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateListing(Listing listing, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateListingCallable()
*
*
*
*
* DeleteListing
* Deletes a listing.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteListing(DeleteListingRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteListing(ListingName name)
*
deleteListing(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteListingCallable()
*
*
*
*
* SubscribeListing
* Subscribes to a listing.
*
Currently, with Analytics Hub, you can create listings that reference only BigQuery datasets. Upon subscription to a listing for a BigQuery dataset, Analytics Hub creates a linked dataset in the subscriber's project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* subscribeListing(SubscribeListingRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* subscribeListing(ListingName name)
*
subscribeListing(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* subscribeListingCallable()
*
*
*
*
* SubscribeDataExchange
* Creates a Subscription to a Data Exchange. This is a long-running operation as it will create one or more linked datasets.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* subscribeDataExchangeAsync(SubscribeDataExchangeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* subscribeDataExchangeAsync(DataExchangeName name)
*
subscribeDataExchangeAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* subscribeDataExchangeOperationCallable()
*
subscribeDataExchangeCallable()
*
*
*
*
* RefreshSubscription
* Refreshes a Subscription to a Data Exchange. A Data Exchange can become stale when a publisher adds or removes data. This is a long-running operation as it may create many linked datasets.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* refreshSubscriptionAsync(RefreshSubscriptionRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* refreshSubscriptionAsync(SubscriptionName name)
*
refreshSubscriptionAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* refreshSubscriptionOperationCallable()
*
refreshSubscriptionCallable()
*
*
*
*
* GetSubscription
* Gets the details of a Subscription.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getSubscription(GetSubscriptionRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getSubscription(SubscriptionName name)
*
getSubscription(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getSubscriptionCallable()
*
*
*
*
* ListSubscriptions
* Lists all subscriptions in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listSubscriptions(ListSubscriptionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listSubscriptions(LocationName parent)
*
listSubscriptions(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listSubscriptionsPagedCallable()
*
listSubscriptionsCallable()
*
*
*
*
* ListSharedResourceSubscriptions
* Lists all subscriptions on a given Data Exchange or Listing.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listSharedResourceSubscriptions(ListSharedResourceSubscriptionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listSharedResourceSubscriptions(ResourceName resource)
*
listSharedResourceSubscriptions(String resource)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listSharedResourceSubscriptionsPagedCallable()
*
listSharedResourceSubscriptionsCallable()
*
*
*
*
* RevokeSubscription
* Revokes a given subscription.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* revokeSubscription(RevokeSubscriptionRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* revokeSubscription(SubscriptionName name)
*
revokeSubscription(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* revokeSubscriptionCallable()
*
*
*
*
* DeleteSubscription
* Deletes a subscription.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteSubscriptionAsync(DeleteSubscriptionRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteSubscriptionAsync(SubscriptionName name)
*
deleteSubscriptionAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteSubscriptionOperationCallable()
*
deleteSubscriptionCallable()
*
*
*
*
* GetIamPolicy
* Gets the IAM policy.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getIamPolicy(GetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getIamPolicyCallable()
*
*
*
*
* SetIamPolicy
* Sets the IAM policy.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* setIamPolicy(SetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* setIamPolicyCallable()
*
*
*
*
* TestIamPermissions
* Returns the permissions that a caller has.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* testIamPermissions(TestIamPermissionsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* testIamPermissionsCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of AnalyticsHubServiceSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* AnalyticsHubServiceSettings analyticsHubServiceSettings =
* AnalyticsHubServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* AnalyticsHubServiceClient analyticsHubServiceClient =
* AnalyticsHubServiceClient.create(analyticsHubServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* AnalyticsHubServiceSettings analyticsHubServiceSettings =
* AnalyticsHubServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* AnalyticsHubServiceClient analyticsHubServiceClient =
* AnalyticsHubServiceClient.create(analyticsHubServiceSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* AnalyticsHubServiceSettings analyticsHubServiceSettings =
* AnalyticsHubServiceSettings.newHttpJsonBuilder().build();
* AnalyticsHubServiceClient analyticsHubServiceClient =
* AnalyticsHubServiceClient.create(analyticsHubServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class AnalyticsHubServiceClient implements BackgroundResource {
private final AnalyticsHubServiceSettings settings;
private final AnalyticsHubServiceStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of AnalyticsHubServiceClient with default settings. */
public static final AnalyticsHubServiceClient create() throws IOException {
return create(AnalyticsHubServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of AnalyticsHubServiceClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final AnalyticsHubServiceClient create(AnalyticsHubServiceSettings settings)
throws IOException {
return new AnalyticsHubServiceClient(settings);
}
/**
* Constructs an instance of AnalyticsHubServiceClient, using the given stub for making calls.
* This is for advanced usage - prefer using create(AnalyticsHubServiceSettings).
*/
public static final AnalyticsHubServiceClient create(AnalyticsHubServiceStub stub) {
return new AnalyticsHubServiceClient(stub);
}
/**
* Constructs an instance of AnalyticsHubServiceClient, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected AnalyticsHubServiceClient(AnalyticsHubServiceSettings settings) throws IOException {
this.settings = settings;
this.stub = ((AnalyticsHubServiceStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected AnalyticsHubServiceClient(AnalyticsHubServiceStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final AnalyticsHubServiceSettings getSettings() {
return settings;
}
public AnalyticsHubServiceStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all data exchanges in a given project and location.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (DataExchange element :
* analyticsHubServiceClient.listDataExchanges(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource path of the data exchanges. e.g.
* `projects/myproject/locations/US`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDataExchangesPagedResponse listDataExchanges(LocationName parent) {
ListDataExchangesRequest request =
ListDataExchangesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listDataExchanges(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all data exchanges in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (DataExchange element :
* analyticsHubServiceClient.listDataExchanges(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource path of the data exchanges. e.g.
* `projects/myproject/locations/US`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDataExchangesPagedResponse listDataExchanges(String parent) {
ListDataExchangesRequest request =
ListDataExchangesRequest.newBuilder().setParent(parent).build();
return listDataExchanges(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all data exchanges in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListDataExchangesRequest request =
* ListDataExchangesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (DataExchange element :
* analyticsHubServiceClient.listDataExchanges(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDataExchangesPagedResponse listDataExchanges(ListDataExchangesRequest request) {
return listDataExchangesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all data exchanges in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListDataExchangesRequest request =
* ListDataExchangesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* analyticsHubServiceClient.listDataExchangesPagedCallable().futureCall(request);
* // Do something.
* for (DataExchange element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listDataExchangesPagedCallable() {
return stub.listDataExchangesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all data exchanges in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListDataExchangesRequest request =
* ListDataExchangesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListDataExchangesResponse response =
* analyticsHubServiceClient.listDataExchangesCallable().call(request);
* for (DataExchange element : response.getDataExchangesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listDataExchangesCallable() {
return stub.listDataExchangesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all data exchanges from projects in a given organization and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String organization = "organization1178922291";
* for (DataExchange element :
* analyticsHubServiceClient.listOrgDataExchanges(organization).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param organization Required. The organization resource path of the projects containing
* DataExchanges. e.g. `organizations/myorg/locations/US`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListOrgDataExchangesPagedResponse listOrgDataExchanges(String organization) {
ListOrgDataExchangesRequest request =
ListOrgDataExchangesRequest.newBuilder().setOrganization(organization).build();
return listOrgDataExchanges(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all data exchanges from projects in a given organization and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListOrgDataExchangesRequest request =
* ListOrgDataExchangesRequest.newBuilder()
* .setOrganization("organization1178922291")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (DataExchange element :
* analyticsHubServiceClient.listOrgDataExchanges(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListOrgDataExchangesPagedResponse listOrgDataExchanges(
ListOrgDataExchangesRequest request) {
return listOrgDataExchangesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all data exchanges from projects in a given organization and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListOrgDataExchangesRequest request =
* ListOrgDataExchangesRequest.newBuilder()
* .setOrganization("organization1178922291")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* analyticsHubServiceClient.listOrgDataExchangesPagedCallable().futureCall(request);
* // Do something.
* for (DataExchange element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listOrgDataExchangesPagedCallable() {
return stub.listOrgDataExchangesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all data exchanges from projects in a given organization and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListOrgDataExchangesRequest request =
* ListOrgDataExchangesRequest.newBuilder()
* .setOrganization("organization1178922291")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListOrgDataExchangesResponse response =
* analyticsHubServiceClient.listOrgDataExchangesCallable().call(request);
* for (DataExchange element : response.getDataExchangesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listOrgDataExchangesCallable() {
return stub.listOrgDataExchangesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DataExchangeName name = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]");
* DataExchange response = analyticsHubServiceClient.getDataExchange(name);
* }
* }
*
* @param name Required. The resource name of the data exchange. e.g.
* `projects/myproject/locations/US/dataExchanges/123`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DataExchange getDataExchange(DataExchangeName name) {
GetDataExchangeRequest request =
GetDataExchangeRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getDataExchange(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString();
* DataExchange response = analyticsHubServiceClient.getDataExchange(name);
* }
* }
*
* @param name Required. The resource name of the data exchange. e.g.
* `projects/myproject/locations/US/dataExchanges/123`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DataExchange getDataExchange(String name) {
GetDataExchangeRequest request = GetDataExchangeRequest.newBuilder().setName(name).build();
return getDataExchange(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* GetDataExchangeRequest request =
* GetDataExchangeRequest.newBuilder()
* .setName(DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .build();
* DataExchange response = analyticsHubServiceClient.getDataExchange(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DataExchange getDataExchange(GetDataExchangeRequest request) {
return getDataExchangeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* GetDataExchangeRequest request =
* GetDataExchangeRequest.newBuilder()
* .setName(DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.getDataExchangeCallable().futureCall(request);
* // Do something.
* DataExchange response = future.get();
* }
* }
*/
public final UnaryCallable getDataExchangeCallable() {
return stub.getDataExchangeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* DataExchange dataExchange = DataExchange.newBuilder().build();
* DataExchange response = analyticsHubServiceClient.createDataExchange(parent, dataExchange);
* }
* }
*
* @param parent Required. The parent resource path of the data exchange. e.g.
* `projects/myproject/locations/US`.
* @param dataExchange Required. The data exchange to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DataExchange createDataExchange(LocationName parent, DataExchange dataExchange) {
CreateDataExchangeRequest request =
CreateDataExchangeRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setDataExchange(dataExchange)
.build();
return createDataExchange(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* DataExchange dataExchange = DataExchange.newBuilder().build();
* DataExchange response = analyticsHubServiceClient.createDataExchange(parent, dataExchange);
* }
* }
*
* @param parent Required. The parent resource path of the data exchange. e.g.
* `projects/myproject/locations/US`.
* @param dataExchange Required. The data exchange to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DataExchange createDataExchange(String parent, DataExchange dataExchange) {
CreateDataExchangeRequest request =
CreateDataExchangeRequest.newBuilder()
.setParent(parent)
.setDataExchange(dataExchange)
.build();
return createDataExchange(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* CreateDataExchangeRequest request =
* CreateDataExchangeRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setDataExchangeId("dataExchangeId783243752")
* .setDataExchange(DataExchange.newBuilder().build())
* .build();
* DataExchange response = analyticsHubServiceClient.createDataExchange(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DataExchange createDataExchange(CreateDataExchangeRequest request) {
return createDataExchangeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* CreateDataExchangeRequest request =
* CreateDataExchangeRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setDataExchangeId("dataExchangeId783243752")
* .setDataExchange(DataExchange.newBuilder().build())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.createDataExchangeCallable().futureCall(request);
* // Do something.
* DataExchange response = future.get();
* }
* }
*/
public final UnaryCallable createDataExchangeCallable() {
return stub.createDataExchangeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DataExchange dataExchange = DataExchange.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* DataExchange response =
* analyticsHubServiceClient.updateDataExchange(dataExchange, updateMask);
* }
* }
*
* @param dataExchange Required. The data exchange to update.
* @param updateMask Required. Field mask specifies the fields to update in the data exchange
* resource. The fields specified in the `updateMask` are relative to the resource and are not
* a full request.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DataExchange updateDataExchange(DataExchange dataExchange, FieldMask updateMask) {
UpdateDataExchangeRequest request =
UpdateDataExchangeRequest.newBuilder()
.setDataExchange(dataExchange)
.setUpdateMask(updateMask)
.build();
return updateDataExchange(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* UpdateDataExchangeRequest request =
* UpdateDataExchangeRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setDataExchange(DataExchange.newBuilder().build())
* .build();
* DataExchange response = analyticsHubServiceClient.updateDataExchange(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DataExchange updateDataExchange(UpdateDataExchangeRequest request) {
return updateDataExchangeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* UpdateDataExchangeRequest request =
* UpdateDataExchangeRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setDataExchange(DataExchange.newBuilder().build())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.updateDataExchangeCallable().futureCall(request);
* // Do something.
* DataExchange response = future.get();
* }
* }
*/
public final UnaryCallable updateDataExchangeCallable() {
return stub.updateDataExchangeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DataExchangeName name = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]");
* analyticsHubServiceClient.deleteDataExchange(name);
* }
* }
*
* @param name Required. The full name of the data exchange resource that you want to delete. For
* example, `projects/myproject/locations/US/dataExchanges/123`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteDataExchange(DataExchangeName name) {
DeleteDataExchangeRequest request =
DeleteDataExchangeRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
deleteDataExchange(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString();
* analyticsHubServiceClient.deleteDataExchange(name);
* }
* }
*
* @param name Required. The full name of the data exchange resource that you want to delete. For
* example, `projects/myproject/locations/US/dataExchanges/123`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteDataExchange(String name) {
DeleteDataExchangeRequest request =
DeleteDataExchangeRequest.newBuilder().setName(name).build();
deleteDataExchange(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DeleteDataExchangeRequest request =
* DeleteDataExchangeRequest.newBuilder()
* .setName(DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .build();
* analyticsHubServiceClient.deleteDataExchange(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteDataExchange(DeleteDataExchangeRequest request) {
deleteDataExchangeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing data exchange.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DeleteDataExchangeRequest request =
* DeleteDataExchangeRequest.newBuilder()
* .setName(DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.deleteDataExchangeCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteDataExchangeCallable() {
return stub.deleteDataExchangeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all listings in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DataExchangeName parent = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]");
* for (Listing element : analyticsHubServiceClient.listListings(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource path of the listing. e.g.
* `projects/myproject/locations/US/dataExchanges/123`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListListingsPagedResponse listListings(DataExchangeName parent) {
ListListingsRequest request =
ListListingsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listListings(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all listings in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String parent = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString();
* for (Listing element : analyticsHubServiceClient.listListings(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource path of the listing. e.g.
* `projects/myproject/locations/US/dataExchanges/123`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListListingsPagedResponse listListings(String parent) {
ListListingsRequest request = ListListingsRequest.newBuilder().setParent(parent).build();
return listListings(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all listings in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListListingsRequest request =
* ListListingsRequest.newBuilder()
* .setParent(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Listing element : analyticsHubServiceClient.listListings(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListListingsPagedResponse listListings(ListListingsRequest request) {
return listListingsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all listings in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListListingsRequest request =
* ListListingsRequest.newBuilder()
* .setParent(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* analyticsHubServiceClient.listListingsPagedCallable().futureCall(request);
* // Do something.
* for (Listing element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listListingsPagedCallable() {
return stub.listListingsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all listings in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListListingsRequest request =
* ListListingsRequest.newBuilder()
* .setParent(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListListingsResponse response =
* analyticsHubServiceClient.listListingsCallable().call(request);
* for (Listing element : response.getListingsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listListingsCallable() {
return stub.listListingsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListingName name = ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]");
* Listing response = analyticsHubServiceClient.getListing(name);
* }
* }
*
* @param name Required. The resource name of the listing. e.g.
* `projects/myproject/locations/US/dataExchanges/123/listings/456`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Listing getListing(ListingName name) {
GetListingRequest request =
GetListingRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getListing(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name =
* ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]").toString();
* Listing response = analyticsHubServiceClient.getListing(name);
* }
* }
*
* @param name Required. The resource name of the listing. e.g.
* `projects/myproject/locations/US/dataExchanges/123/listings/456`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Listing getListing(String name) {
GetListingRequest request = GetListingRequest.newBuilder().setName(name).build();
return getListing(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* GetListingRequest request =
* GetListingRequest.newBuilder()
* .setName(
* ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]")
* .toString())
* .build();
* Listing response = analyticsHubServiceClient.getListing(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Listing getListing(GetListingRequest request) {
return getListingCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* GetListingRequest request =
* GetListingRequest.newBuilder()
* .setName(
* ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]")
* .toString())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.getListingCallable().futureCall(request);
* // Do something.
* Listing response = future.get();
* }
* }
*/
public final UnaryCallable getListingCallable() {
return stub.getListingCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DataExchangeName parent = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]");
* Listing listing = Listing.newBuilder().build();
* Listing response = analyticsHubServiceClient.createListing(parent, listing);
* }
* }
*
* @param parent Required. The parent resource path of the listing. e.g.
* `projects/myproject/locations/US/dataExchanges/123`.
* @param listing Required. The listing to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Listing createListing(DataExchangeName parent, Listing listing) {
CreateListingRequest request =
CreateListingRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setListing(listing)
.build();
return createListing(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String parent = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString();
* Listing listing = Listing.newBuilder().build();
* Listing response = analyticsHubServiceClient.createListing(parent, listing);
* }
* }
*
* @param parent Required. The parent resource path of the listing. e.g.
* `projects/myproject/locations/US/dataExchanges/123`.
* @param listing Required. The listing to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Listing createListing(String parent, Listing listing) {
CreateListingRequest request =
CreateListingRequest.newBuilder().setParent(parent).setListing(listing).build();
return createListing(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* CreateListingRequest request =
* CreateListingRequest.newBuilder()
* .setParent(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setListingId("listingId-1215024449")
* .setListing(Listing.newBuilder().build())
* .build();
* Listing response = analyticsHubServiceClient.createListing(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Listing createListing(CreateListingRequest request) {
return createListingCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* CreateListingRequest request =
* CreateListingRequest.newBuilder()
* .setParent(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setListingId("listingId-1215024449")
* .setListing(Listing.newBuilder().build())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.createListingCallable().futureCall(request);
* // Do something.
* Listing response = future.get();
* }
* }
*/
public final UnaryCallable createListingCallable() {
return stub.createListingCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* Listing listing = Listing.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Listing response = analyticsHubServiceClient.updateListing(listing, updateMask);
* }
* }
*
* @param listing Required. The listing to update.
* @param updateMask Required. Field mask specifies the fields to update in the listing resource.
* The fields specified in the `updateMask` are relative to the resource and are not a full
* request.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Listing updateListing(Listing listing, FieldMask updateMask) {
UpdateListingRequest request =
UpdateListingRequest.newBuilder().setListing(listing).setUpdateMask(updateMask).build();
return updateListing(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* UpdateListingRequest request =
* UpdateListingRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setListing(Listing.newBuilder().build())
* .build();
* Listing response = analyticsHubServiceClient.updateListing(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Listing updateListing(UpdateListingRequest request) {
return updateListingCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* UpdateListingRequest request =
* UpdateListingRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setListing(Listing.newBuilder().build())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.updateListingCallable().futureCall(request);
* // Do something.
* Listing response = future.get();
* }
* }
*/
public final UnaryCallable updateListingCallable() {
return stub.updateListingCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListingName name = ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]");
* analyticsHubServiceClient.deleteListing(name);
* }
* }
*
* @param name Required. Resource name of the listing to delete. e.g.
* `projects/myproject/locations/US/dataExchanges/123/listings/456`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteListing(ListingName name) {
DeleteListingRequest request =
DeleteListingRequest.newBuilder().setName(name == null ? null : name.toString()).build();
deleteListing(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name =
* ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]").toString();
* analyticsHubServiceClient.deleteListing(name);
* }
* }
*
* @param name Required. Resource name of the listing to delete. e.g.
* `projects/myproject/locations/US/dataExchanges/123/listings/456`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteListing(String name) {
DeleteListingRequest request = DeleteListingRequest.newBuilder().setName(name).build();
deleteListing(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DeleteListingRequest request =
* DeleteListingRequest.newBuilder()
* .setName(
* ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]")
* .toString())
* .build();
* analyticsHubServiceClient.deleteListing(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteListing(DeleteListingRequest request) {
deleteListingCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DeleteListingRequest request =
* DeleteListingRequest.newBuilder()
* .setName(
* ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]")
* .toString())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.deleteListingCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteListingCallable() {
return stub.deleteListingCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Subscribes to a listing.
*
* Currently, with Analytics Hub, you can create listings that reference only BigQuery
* datasets. Upon subscription to a listing for a BigQuery dataset, Analytics Hub creates a linked
* dataset in the subscriber's project.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListingName name = ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]");
* SubscribeListingResponse response = analyticsHubServiceClient.subscribeListing(name);
* }
* }
*
* @param name Required. Resource name of the listing that you want to subscribe to. e.g.
* `projects/myproject/locations/US/dataExchanges/123/listings/456`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SubscribeListingResponse subscribeListing(ListingName name) {
SubscribeListingRequest request =
SubscribeListingRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return subscribeListing(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Subscribes to a listing.
*
* Currently, with Analytics Hub, you can create listings that reference only BigQuery
* datasets. Upon subscription to a listing for a BigQuery dataset, Analytics Hub creates a linked
* dataset in the subscriber's project.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name =
* ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]").toString();
* SubscribeListingResponse response = analyticsHubServiceClient.subscribeListing(name);
* }
* }
*
* @param name Required. Resource name of the listing that you want to subscribe to. e.g.
* `projects/myproject/locations/US/dataExchanges/123/listings/456`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SubscribeListingResponse subscribeListing(String name) {
SubscribeListingRequest request = SubscribeListingRequest.newBuilder().setName(name).build();
return subscribeListing(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Subscribes to a listing.
*
* Currently, with Analytics Hub, you can create listings that reference only BigQuery
* datasets. Upon subscription to a listing for a BigQuery dataset, Analytics Hub creates a linked
* dataset in the subscriber's project.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SubscribeListingRequest request =
* SubscribeListingRequest.newBuilder()
* .setName(
* ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]")
* .toString())
* .build();
* SubscribeListingResponse response = analyticsHubServiceClient.subscribeListing(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SubscribeListingResponse subscribeListing(SubscribeListingRequest request) {
return subscribeListingCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Subscribes to a listing.
*
* Currently, with Analytics Hub, you can create listings that reference only BigQuery
* datasets. Upon subscription to a listing for a BigQuery dataset, Analytics Hub creates a linked
* dataset in the subscriber's project.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SubscribeListingRequest request =
* SubscribeListingRequest.newBuilder()
* .setName(
* ListingName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]", "[LISTING]")
* .toString())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.subscribeListingCallable().futureCall(request);
* // Do something.
* SubscribeListingResponse response = future.get();
* }
* }
*/
public final UnaryCallable
subscribeListingCallable() {
return stub.subscribeListingCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Subscription to a Data Exchange. This is a long-running operation as it will create
* one or more linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DataExchangeName name = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]");
* SubscribeDataExchangeResponse response =
* analyticsHubServiceClient.subscribeDataExchangeAsync(name).get();
* }
* }
*
* @param name Required. Resource name of the Data Exchange. e.g.
* `projects/publisherproject/locations/US/dataExchanges/123`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
subscribeDataExchangeAsync(DataExchangeName name) {
SubscribeDataExchangeRequest request =
SubscribeDataExchangeRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return subscribeDataExchangeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Subscription to a Data Exchange. This is a long-running operation as it will create
* one or more linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString();
* SubscribeDataExchangeResponse response =
* analyticsHubServiceClient.subscribeDataExchangeAsync(name).get();
* }
* }
*
* @param name Required. Resource name of the Data Exchange. e.g.
* `projects/publisherproject/locations/US/dataExchanges/123`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
subscribeDataExchangeAsync(String name) {
SubscribeDataExchangeRequest request =
SubscribeDataExchangeRequest.newBuilder().setName(name).build();
return subscribeDataExchangeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Subscription to a Data Exchange. This is a long-running operation as it will create
* one or more linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SubscribeDataExchangeRequest request =
* SubscribeDataExchangeRequest.newBuilder()
* .setName(DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setDestination(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setSubscription("subscription341203229")
* .setSubscriberContact("subscriberContact-847205736")
* .build();
* SubscribeDataExchangeResponse response =
* analyticsHubServiceClient.subscribeDataExchangeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
subscribeDataExchangeAsync(SubscribeDataExchangeRequest request) {
return subscribeDataExchangeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Subscription to a Data Exchange. This is a long-running operation as it will create
* one or more linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SubscribeDataExchangeRequest request =
* SubscribeDataExchangeRequest.newBuilder()
* .setName(DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setDestination(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setSubscription("subscription341203229")
* .setSubscriberContact("subscriberContact-847205736")
* .build();
* OperationFuture future =
* analyticsHubServiceClient.subscribeDataExchangeOperationCallable().futureCall(request);
* // Do something.
* SubscribeDataExchangeResponse response = future.get();
* }
* }
*/
public final OperationCallable<
SubscribeDataExchangeRequest, SubscribeDataExchangeResponse, OperationMetadata>
subscribeDataExchangeOperationCallable() {
return stub.subscribeDataExchangeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Subscription to a Data Exchange. This is a long-running operation as it will create
* one or more linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SubscribeDataExchangeRequest request =
* SubscribeDataExchangeRequest.newBuilder()
* .setName(DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setDestination(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setSubscription("subscription341203229")
* .setSubscriberContact("subscriberContact-847205736")
* .build();
* ApiFuture future =
* analyticsHubServiceClient.subscribeDataExchangeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
subscribeDataExchangeCallable() {
return stub.subscribeDataExchangeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Refreshes a Subscription to a Data Exchange. A Data Exchange can become stale when a publisher
* adds or removes data. This is a long-running operation as it may create many linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SubscriptionName name = SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]");
* RefreshSubscriptionResponse response =
* analyticsHubServiceClient.refreshSubscriptionAsync(name).get();
* }
* }
*
* @param name Required. Resource name of the Subscription to refresh. e.g.
* `projects/subscriberproject/locations/US/subscriptions/123`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
refreshSubscriptionAsync(SubscriptionName name) {
RefreshSubscriptionRequest request =
RefreshSubscriptionRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return refreshSubscriptionAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Refreshes a Subscription to a Data Exchange. A Data Exchange can become stale when a publisher
* adds or removes data. This is a long-running operation as it may create many linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name = SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString();
* RefreshSubscriptionResponse response =
* analyticsHubServiceClient.refreshSubscriptionAsync(name).get();
* }
* }
*
* @param name Required. Resource name of the Subscription to refresh. e.g.
* `projects/subscriberproject/locations/US/subscriptions/123`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
refreshSubscriptionAsync(String name) {
RefreshSubscriptionRequest request =
RefreshSubscriptionRequest.newBuilder().setName(name).build();
return refreshSubscriptionAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Refreshes a Subscription to a Data Exchange. A Data Exchange can become stale when a publisher
* adds or removes data. This is a long-running operation as it may create many linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* RefreshSubscriptionRequest request =
* RefreshSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* RefreshSubscriptionResponse response =
* analyticsHubServiceClient.refreshSubscriptionAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
refreshSubscriptionAsync(RefreshSubscriptionRequest request) {
return refreshSubscriptionOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Refreshes a Subscription to a Data Exchange. A Data Exchange can become stale when a publisher
* adds or removes data. This is a long-running operation as it may create many linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* RefreshSubscriptionRequest request =
* RefreshSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* OperationFuture future =
* analyticsHubServiceClient.refreshSubscriptionOperationCallable().futureCall(request);
* // Do something.
* RefreshSubscriptionResponse response = future.get();
* }
* }
*/
public final OperationCallable<
RefreshSubscriptionRequest, RefreshSubscriptionResponse, OperationMetadata>
refreshSubscriptionOperationCallable() {
return stub.refreshSubscriptionOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Refreshes a Subscription to a Data Exchange. A Data Exchange can become stale when a publisher
* adds or removes data. This is a long-running operation as it may create many linked datasets.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* RefreshSubscriptionRequest request =
* RefreshSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.refreshSubscriptionCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable refreshSubscriptionCallable() {
return stub.refreshSubscriptionCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a Subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SubscriptionName name = SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]");
* Subscription response = analyticsHubServiceClient.getSubscription(name);
* }
* }
*
* @param name Required. Resource name of the subscription. e.g.
* projects/123/locations/US/subscriptions/456
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Subscription getSubscription(SubscriptionName name) {
GetSubscriptionRequest request =
GetSubscriptionRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getSubscription(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a Subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name = SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString();
* Subscription response = analyticsHubServiceClient.getSubscription(name);
* }
* }
*
* @param name Required. Resource name of the subscription. e.g.
* projects/123/locations/US/subscriptions/456
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Subscription getSubscription(String name) {
GetSubscriptionRequest request = GetSubscriptionRequest.newBuilder().setName(name).build();
return getSubscription(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a Subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* GetSubscriptionRequest request =
* GetSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* Subscription response = analyticsHubServiceClient.getSubscription(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Subscription getSubscription(GetSubscriptionRequest request) {
return getSubscriptionCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a Subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* GetSubscriptionRequest request =
* GetSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.getSubscriptionCallable().futureCall(request);
* // Do something.
* Subscription response = future.get();
* }
* }
*/
public final UnaryCallable getSubscriptionCallable() {
return stub.getSubscriptionCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Subscription element :
* analyticsHubServiceClient.listSubscriptions(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource path of the subscription. e.g.
* projects/myproject/locations/US
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSubscriptionsPagedResponse listSubscriptions(LocationName parent) {
ListSubscriptionsRequest request =
ListSubscriptionsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listSubscriptions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Subscription element :
* analyticsHubServiceClient.listSubscriptions(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource path of the subscription. e.g.
* projects/myproject/locations/US
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSubscriptionsPagedResponse listSubscriptions(String parent) {
ListSubscriptionsRequest request =
ListSubscriptionsRequest.newBuilder().setParent(parent).build();
return listSubscriptions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListSubscriptionsRequest request =
* ListSubscriptionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Subscription element :
* analyticsHubServiceClient.listSubscriptions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSubscriptionsPagedResponse listSubscriptions(ListSubscriptionsRequest request) {
return listSubscriptionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListSubscriptionsRequest request =
* ListSubscriptionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* analyticsHubServiceClient.listSubscriptionsPagedCallable().futureCall(request);
* // Do something.
* for (Subscription element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listSubscriptionsPagedCallable() {
return stub.listSubscriptionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListSubscriptionsRequest request =
* ListSubscriptionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListSubscriptionsResponse response =
* analyticsHubServiceClient.listSubscriptionsCallable().call(request);
* for (Subscription element : response.getSubscriptionsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listSubscriptionsCallable() {
return stub.listSubscriptionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions on a given Data Exchange or Listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ResourceName resource = DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]");
* for (Subscription element :
* analyticsHubServiceClient.listSharedResourceSubscriptions(resource).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param resource Required. Resource name of the requested target. This resource may be either a
* Listing or a DataExchange. e.g. projects/123/locations/US/dataExchanges/456 OR e.g.
* projects/123/locations/US/dataExchanges/456/listings/789
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSharedResourceSubscriptionsPagedResponse listSharedResourceSubscriptions(
ResourceName resource) {
ListSharedResourceSubscriptionsRequest request =
ListSharedResourceSubscriptionsRequest.newBuilder()
.setResource(resource == null ? null : resource.toString())
.build();
return listSharedResourceSubscriptions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions on a given Data Exchange or Listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String resource =
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString();
* for (Subscription element :
* analyticsHubServiceClient.listSharedResourceSubscriptions(resource).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param resource Required. Resource name of the requested target. This resource may be either a
* Listing or a DataExchange. e.g. projects/123/locations/US/dataExchanges/456 OR e.g.
* projects/123/locations/US/dataExchanges/456/listings/789
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSharedResourceSubscriptionsPagedResponse listSharedResourceSubscriptions(
String resource) {
ListSharedResourceSubscriptionsRequest request =
ListSharedResourceSubscriptionsRequest.newBuilder().setResource(resource).build();
return listSharedResourceSubscriptions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions on a given Data Exchange or Listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListSharedResourceSubscriptionsRequest request =
* ListSharedResourceSubscriptionsRequest.newBuilder()
* .setResource(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setIncludeDeletedSubscriptions(true)
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Subscription element :
* analyticsHubServiceClient.listSharedResourceSubscriptions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSharedResourceSubscriptionsPagedResponse listSharedResourceSubscriptions(
ListSharedResourceSubscriptionsRequest request) {
return listSharedResourceSubscriptionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions on a given Data Exchange or Listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListSharedResourceSubscriptionsRequest request =
* ListSharedResourceSubscriptionsRequest.newBuilder()
* .setResource(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setIncludeDeletedSubscriptions(true)
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* analyticsHubServiceClient
* .listSharedResourceSubscriptionsPagedCallable()
* .futureCall(request);
* // Do something.
* for (Subscription element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable<
ListSharedResourceSubscriptionsRequest, ListSharedResourceSubscriptionsPagedResponse>
listSharedResourceSubscriptionsPagedCallable() {
return stub.listSharedResourceSubscriptionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all subscriptions on a given Data Exchange or Listing.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* ListSharedResourceSubscriptionsRequest request =
* ListSharedResourceSubscriptionsRequest.newBuilder()
* .setResource(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setIncludeDeletedSubscriptions(true)
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListSharedResourceSubscriptionsResponse response =
* analyticsHubServiceClient.listSharedResourceSubscriptionsCallable().call(request);
* for (Subscription element : response.getSharedResourceSubscriptionsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable<
ListSharedResourceSubscriptionsRequest, ListSharedResourceSubscriptionsResponse>
listSharedResourceSubscriptionsCallable() {
return stub.listSharedResourceSubscriptionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Revokes a given subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SubscriptionName name = SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]");
* RevokeSubscriptionResponse response = analyticsHubServiceClient.revokeSubscription(name);
* }
* }
*
* @param name Required. Resource name of the subscription to revoke. e.g.
* projects/123/locations/US/subscriptions/456
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RevokeSubscriptionResponse revokeSubscription(SubscriptionName name) {
RevokeSubscriptionRequest request =
RevokeSubscriptionRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return revokeSubscription(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Revokes a given subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name = SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString();
* RevokeSubscriptionResponse response = analyticsHubServiceClient.revokeSubscription(name);
* }
* }
*
* @param name Required. Resource name of the subscription to revoke. e.g.
* projects/123/locations/US/subscriptions/456
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RevokeSubscriptionResponse revokeSubscription(String name) {
RevokeSubscriptionRequest request =
RevokeSubscriptionRequest.newBuilder().setName(name).build();
return revokeSubscription(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Revokes a given subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* RevokeSubscriptionRequest request =
* RevokeSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* RevokeSubscriptionResponse response = analyticsHubServiceClient.revokeSubscription(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RevokeSubscriptionResponse revokeSubscription(RevokeSubscriptionRequest request) {
return revokeSubscriptionCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Revokes a given subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* RevokeSubscriptionRequest request =
* RevokeSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.revokeSubscriptionCallable().futureCall(request);
* // Do something.
* RevokeSubscriptionResponse response = future.get();
* }
* }
*/
public final UnaryCallable
revokeSubscriptionCallable() {
return stub.revokeSubscriptionCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SubscriptionName name = SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]");
* analyticsHubServiceClient.deleteSubscriptionAsync(name).get();
* }
* }
*
* @param name Required. Resource name of the subscription to delete. e.g.
* projects/123/locations/US/subscriptions/456
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteSubscriptionAsync(
SubscriptionName name) {
DeleteSubscriptionRequest request =
DeleteSubscriptionRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteSubscriptionAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* String name = SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString();
* analyticsHubServiceClient.deleteSubscriptionAsync(name).get();
* }
* }
*
* @param name Required. Resource name of the subscription to delete. e.g.
* projects/123/locations/US/subscriptions/456
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteSubscriptionAsync(String name) {
DeleteSubscriptionRequest request =
DeleteSubscriptionRequest.newBuilder().setName(name).build();
return deleteSubscriptionAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DeleteSubscriptionRequest request =
* DeleteSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* analyticsHubServiceClient.deleteSubscriptionAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteSubscriptionAsync(
DeleteSubscriptionRequest request) {
return deleteSubscriptionOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DeleteSubscriptionRequest request =
* DeleteSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* OperationFuture future =
* analyticsHubServiceClient.deleteSubscriptionOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteSubscriptionOperationCallable() {
return stub.deleteSubscriptionOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a subscription.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* DeleteSubscriptionRequest request =
* DeleteSubscriptionRequest.newBuilder()
* .setName(SubscriptionName.of("[PROJECT]", "[LOCATION]", "[SUBSCRIPTION]").toString())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.deleteSubscriptionCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteSubscriptionCallable() {
return stub.deleteSubscriptionCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the IAM policy.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* Policy response = analyticsHubServiceClient.getIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(GetIamPolicyRequest request) {
return getIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the IAM policy.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.getIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable getIamPolicyCallable() {
return stub.getIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the IAM policy.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Policy response = analyticsHubServiceClient.setIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(SetIamPolicyRequest request) {
return setIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the IAM policy.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.setIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable setIamPolicyCallable() {
return stub.setIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the permissions that a caller has.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* TestIamPermissionsResponse response = analyticsHubServiceClient.testIamPermissions(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(TestIamPermissionsRequest request) {
return testIamPermissionsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the permissions that a caller has.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (AnalyticsHubServiceClient analyticsHubServiceClient = AnalyticsHubServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(
* DataExchangeName.of("[PROJECT]", "[LOCATION]", "[DATA_EXCHANGE]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* ApiFuture future =
* analyticsHubServiceClient.testIamPermissionsCallable().futureCall(request);
* // Do something.
* TestIamPermissionsResponse response = future.get();
* }
* }
*/
public final UnaryCallable
testIamPermissionsCallable() {
return stub.testIamPermissionsCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListDataExchangesPagedResponse
extends AbstractPagedListResponse<
ListDataExchangesRequest,
ListDataExchangesResponse,
DataExchange,
ListDataExchangesPage,
ListDataExchangesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListDataExchangesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListDataExchangesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListDataExchangesPagedResponse(ListDataExchangesPage page) {
super(page, ListDataExchangesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListDataExchangesPage
extends AbstractPage<
ListDataExchangesRequest,
ListDataExchangesResponse,
DataExchange,
ListDataExchangesPage> {
private ListDataExchangesPage(
PageContext context,
ListDataExchangesResponse response) {
super(context, response);
}
private static ListDataExchangesPage createEmptyPage() {
return new ListDataExchangesPage(null, null);
}
@Override
protected ListDataExchangesPage createPage(
PageContext context,
ListDataExchangesResponse response) {
return new ListDataExchangesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListDataExchangesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListDataExchangesRequest,
ListDataExchangesResponse,
DataExchange,
ListDataExchangesPage,
ListDataExchangesFixedSizeCollection> {
private ListDataExchangesFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListDataExchangesFixedSizeCollection createEmptyCollection() {
return new ListDataExchangesFixedSizeCollection(null, 0);
}
@Override
protected ListDataExchangesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListDataExchangesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListOrgDataExchangesPagedResponse
extends AbstractPagedListResponse<
ListOrgDataExchangesRequest,
ListOrgDataExchangesResponse,
DataExchange,
ListOrgDataExchangesPage,
ListOrgDataExchangesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListOrgDataExchangesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListOrgDataExchangesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListOrgDataExchangesPagedResponse(ListOrgDataExchangesPage page) {
super(page, ListOrgDataExchangesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListOrgDataExchangesPage
extends AbstractPage<
ListOrgDataExchangesRequest,
ListOrgDataExchangesResponse,
DataExchange,
ListOrgDataExchangesPage> {
private ListOrgDataExchangesPage(
PageContext
context,
ListOrgDataExchangesResponse response) {
super(context, response);
}
private static ListOrgDataExchangesPage createEmptyPage() {
return new ListOrgDataExchangesPage(null, null);
}
@Override
protected ListOrgDataExchangesPage createPage(
PageContext
context,
ListOrgDataExchangesResponse response) {
return new ListOrgDataExchangesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListOrgDataExchangesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListOrgDataExchangesRequest,
ListOrgDataExchangesResponse,
DataExchange,
ListOrgDataExchangesPage,
ListOrgDataExchangesFixedSizeCollection> {
private ListOrgDataExchangesFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListOrgDataExchangesFixedSizeCollection createEmptyCollection() {
return new ListOrgDataExchangesFixedSizeCollection(null, 0);
}
@Override
protected ListOrgDataExchangesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListOrgDataExchangesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListListingsPagedResponse
extends AbstractPagedListResponse<
ListListingsRequest,
ListListingsResponse,
Listing,
ListListingsPage,
ListListingsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListListingsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListListingsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListListingsPagedResponse(ListListingsPage page) {
super(page, ListListingsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListListingsPage
extends AbstractPage {
private ListListingsPage(
PageContext context,
ListListingsResponse response) {
super(context, response);
}
private static ListListingsPage createEmptyPage() {
return new ListListingsPage(null, null);
}
@Override
protected ListListingsPage createPage(
PageContext context,
ListListingsResponse response) {
return new ListListingsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListListingsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListListingsRequest,
ListListingsResponse,
Listing,
ListListingsPage,
ListListingsFixedSizeCollection> {
private ListListingsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListListingsFixedSizeCollection createEmptyCollection() {
return new ListListingsFixedSizeCollection(null, 0);
}
@Override
protected ListListingsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListListingsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListSubscriptionsPagedResponse
extends AbstractPagedListResponse<
ListSubscriptionsRequest,
ListSubscriptionsResponse,
Subscription,
ListSubscriptionsPage,
ListSubscriptionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListSubscriptionsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListSubscriptionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListSubscriptionsPagedResponse(ListSubscriptionsPage page) {
super(page, ListSubscriptionsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListSubscriptionsPage
extends AbstractPage<
ListSubscriptionsRequest,
ListSubscriptionsResponse,
Subscription,
ListSubscriptionsPage> {
private ListSubscriptionsPage(
PageContext context,
ListSubscriptionsResponse response) {
super(context, response);
}
private static ListSubscriptionsPage createEmptyPage() {
return new ListSubscriptionsPage(null, null);
}
@Override
protected ListSubscriptionsPage createPage(
PageContext context,
ListSubscriptionsResponse response) {
return new ListSubscriptionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListSubscriptionsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListSubscriptionsRequest,
ListSubscriptionsResponse,
Subscription,
ListSubscriptionsPage,
ListSubscriptionsFixedSizeCollection> {
private ListSubscriptionsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListSubscriptionsFixedSizeCollection createEmptyCollection() {
return new ListSubscriptionsFixedSizeCollection(null, 0);
}
@Override
protected ListSubscriptionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListSubscriptionsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListSharedResourceSubscriptionsPagedResponse
extends AbstractPagedListResponse<
ListSharedResourceSubscriptionsRequest,
ListSharedResourceSubscriptionsResponse,
Subscription,
ListSharedResourceSubscriptionsPage,
ListSharedResourceSubscriptionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListSharedResourceSubscriptionsRequest,
ListSharedResourceSubscriptionsResponse,
Subscription>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListSharedResourceSubscriptionsPage.createEmptyPage()
.createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListSharedResourceSubscriptionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListSharedResourceSubscriptionsPagedResponse(ListSharedResourceSubscriptionsPage page) {
super(page, ListSharedResourceSubscriptionsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListSharedResourceSubscriptionsPage
extends AbstractPage<
ListSharedResourceSubscriptionsRequest,
ListSharedResourceSubscriptionsResponse,
Subscription,
ListSharedResourceSubscriptionsPage> {
private ListSharedResourceSubscriptionsPage(
PageContext<
ListSharedResourceSubscriptionsRequest,
ListSharedResourceSubscriptionsResponse,
Subscription>
context,
ListSharedResourceSubscriptionsResponse response) {
super(context, response);
}
private static ListSharedResourceSubscriptionsPage createEmptyPage() {
return new ListSharedResourceSubscriptionsPage(null, null);
}
@Override
protected ListSharedResourceSubscriptionsPage createPage(
PageContext<
ListSharedResourceSubscriptionsRequest,
ListSharedResourceSubscriptionsResponse,
Subscription>
context,
ListSharedResourceSubscriptionsResponse response) {
return new ListSharedResourceSubscriptionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListSharedResourceSubscriptionsRequest,
ListSharedResourceSubscriptionsResponse,
Subscription>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListSharedResourceSubscriptionsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListSharedResourceSubscriptionsRequest,
ListSharedResourceSubscriptionsResponse,
Subscription,
ListSharedResourceSubscriptionsPage,
ListSharedResourceSubscriptionsFixedSizeCollection> {
private ListSharedResourceSubscriptionsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListSharedResourceSubscriptionsFixedSizeCollection createEmptyCollection() {
return new ListSharedResourceSubscriptionsFixedSizeCollection(null, 0);
}
@Override
protected ListSharedResourceSubscriptionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListSharedResourceSubscriptionsFixedSizeCollection(pages, collectionSize);
}
}
}