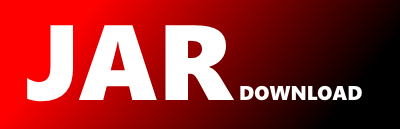
com.google.api.apikeys.v2.ApiKeysClient Maven / Gradle / Ivy
Show all versions of google-cloud-apikeys Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.api.apikeys.v2;
import com.google.api.apikeys.v2.stub.ApiKeysStub;
import com.google.api.apikeys.v2.stub.ApiKeysStubSettings;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Manages the API keys associated with projects.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* KeyName name = KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]");
* Key response = apiKeysClient.getKey(name);
* }
* }
*
* Note: close() needs to be called on the ApiKeysClient object to clean up resources such as
* threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* CreateKey
* Creates a new API key.
*
NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createKeyAsync(CreateKeyRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createKeyAsync(LocationName parent, Key key, String keyId)
*
createKeyAsync(String parent, Key key, String keyId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createKeyOperationCallable()
*
createKeyCallable()
*
*
*
*
* ListKeys
* Lists the API keys owned by a project. The key string of the API key isn't included in the response.
*
NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listKeys(ListKeysRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listKeys(LocationName parent)
*
listKeys(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listKeysPagedCallable()
*
listKeysCallable()
*
*
*
*
* GetKey
* Gets the metadata for an API key. The key string of the API key isn't included in the response.
*
NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getKey(GetKeyRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getKey(KeyName name)
*
getKey(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getKeyCallable()
*
*
*
*
* GetKeyString
* Get the key string for an API key.
*
NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getKeyString(GetKeyStringRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getKeyString(KeyName name)
*
getKeyString(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getKeyStringCallable()
*
*
*
*
* UpdateKey
* Patches the modifiable fields of an API key. The key string of the API key isn't included in the response.
*
NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateKeyAsync(UpdateKeyRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateKeyAsync(Key key, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateKeyOperationCallable()
*
updateKeyCallable()
*
*
*
*
* DeleteKey
* Deletes an API key. Deleted key can be retrieved within 30 days of deletion. Afterward, key will be purged from the project.
*
NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteKeyAsync(DeleteKeyRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteKeyAsync(KeyName name)
*
deleteKeyAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteKeyOperationCallable()
*
deleteKeyCallable()
*
*
*
*
* UndeleteKey
* Undeletes an API key which was deleted within 30 days.
*
NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* undeleteKeyAsync(UndeleteKeyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* undeleteKeyOperationCallable()
*
undeleteKeyCallable()
*
*
*
*
* LookupKey
* Find the parent project and resource name of the API key that matches the key string in the request. If the API key has been purged, resource name will not be set. The service account must have the `apikeys.keys.lookup` permission on the parent project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* lookupKey(LookupKeyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* lookupKeyCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of ApiKeysSettings to create().
* For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ApiKeysSettings apiKeysSettings =
* ApiKeysSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* ApiKeysClient apiKeysClient = ApiKeysClient.create(apiKeysSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ApiKeysSettings apiKeysSettings = ApiKeysSettings.newBuilder().setEndpoint(myEndpoint).build();
* ApiKeysClient apiKeysClient = ApiKeysClient.create(apiKeysSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ApiKeysSettings apiKeysSettings = ApiKeysSettings.newHttpJsonBuilder().build();
* ApiKeysClient apiKeysClient = ApiKeysClient.create(apiKeysSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class ApiKeysClient implements BackgroundResource {
private final ApiKeysSettings settings;
private final ApiKeysStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of ApiKeysClient with default settings. */
public static final ApiKeysClient create() throws IOException {
return create(ApiKeysSettings.newBuilder().build());
}
/**
* Constructs an instance of ApiKeysClient, using the given settings. The channels are created
* based on the settings passed in, or defaults for any settings that are not set.
*/
public static final ApiKeysClient create(ApiKeysSettings settings) throws IOException {
return new ApiKeysClient(settings);
}
/**
* Constructs an instance of ApiKeysClient, using the given stub for making calls. This is for
* advanced usage - prefer using create(ApiKeysSettings).
*/
public static final ApiKeysClient create(ApiKeysStub stub) {
return new ApiKeysClient(stub);
}
/**
* Constructs an instance of ApiKeysClient, using the given settings. This is protected so that it
* is easy to make a subclass, but otherwise, the static factory methods should be preferred.
*/
protected ApiKeysClient(ApiKeysSettings settings) throws IOException {
this.settings = settings;
this.stub = ((ApiKeysStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected ApiKeysClient(ApiKeysStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final ApiKeysSettings getSettings() {
return settings;
}
public ApiKeysStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new API key.
*
*
NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Key key = Key.newBuilder().build();
* String keyId = "keyId101944282";
* Key response = apiKeysClient.createKeyAsync(parent, key, keyId).get();
* }
* }
*
* @param parent Required. The project in which the API key is created.
* @param key Required. The API key fields to set at creation time. You can configure only the
* `display_name`, `restrictions`, and `annotations` fields.
* @param keyId User specified key id (optional). If specified, it will become the final component
* of the key resource name.
* The id must be unique within the project, must conform with RFC-1034, is restricted to
* lower-cased letters, and has a maximum length of 63 characters. In another word, the id
* must match the regular expression: `[a-z]([a-z0-9-]{0,61}[a-z0-9])?`.
*
The id must NOT be a UUID-like string.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createKeyAsync(
LocationName parent, Key key, String keyId) {
CreateKeyRequest request =
CreateKeyRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setKey(key)
.setKeyId(keyId)
.build();
return createKeyAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new API key.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Key key = Key.newBuilder().build();
* String keyId = "keyId101944282";
* Key response = apiKeysClient.createKeyAsync(parent, key, keyId).get();
* }
* }
*
* @param parent Required. The project in which the API key is created.
* @param key Required. The API key fields to set at creation time. You can configure only the
* `display_name`, `restrictions`, and `annotations` fields.
* @param keyId User specified key id (optional). If specified, it will become the final component
* of the key resource name.
* The id must be unique within the project, must conform with RFC-1034, is restricted to
* lower-cased letters, and has a maximum length of 63 characters. In another word, the id
* must match the regular expression: `[a-z]([a-z0-9-]{0,61}[a-z0-9])?`.
*
The id must NOT be a UUID-like string.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createKeyAsync(String parent, Key key, String keyId) {
CreateKeyRequest request =
CreateKeyRequest.newBuilder().setParent(parent).setKey(key).setKeyId(keyId).build();
return createKeyAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new API key.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* CreateKeyRequest request =
* CreateKeyRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setKey(Key.newBuilder().build())
* .setKeyId("keyId101944282")
* .build();
* Key response = apiKeysClient.createKeyAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createKeyAsync(CreateKeyRequest request) {
return createKeyOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new API key.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* CreateKeyRequest request =
* CreateKeyRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setKey(Key.newBuilder().build())
* .setKeyId("keyId101944282")
* .build();
* OperationFuture future =
* apiKeysClient.createKeyOperationCallable().futureCall(request);
* // Do something.
* Key response = future.get();
* }
* }
*/
public final OperationCallable createKeyOperationCallable() {
return stub.createKeyOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new API key.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* CreateKeyRequest request =
* CreateKeyRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setKey(Key.newBuilder().build())
* .setKeyId("keyId101944282")
* .build();
* ApiFuture future = apiKeysClient.createKeyCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createKeyCallable() {
return stub.createKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the API keys owned by a project. The key string of the API key isn't included in the
* response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Key element : apiKeysClient.listKeys(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Lists all API keys associated with this project.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListKeysPagedResponse listKeys(LocationName parent) {
ListKeysRequest request =
ListKeysRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listKeys(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the API keys owned by a project. The key string of the API key isn't included in the
* response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Key element : apiKeysClient.listKeys(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Lists all API keys associated with this project.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListKeysPagedResponse listKeys(String parent) {
ListKeysRequest request = ListKeysRequest.newBuilder().setParent(parent).build();
return listKeys(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the API keys owned by a project. The key string of the API key isn't included in the
* response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* ListKeysRequest request =
* ListKeysRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setShowDeleted(true)
* .build();
* for (Key element : apiKeysClient.listKeys(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListKeysPagedResponse listKeys(ListKeysRequest request) {
return listKeysPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the API keys owned by a project. The key string of the API key isn't included in the
* response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* ListKeysRequest request =
* ListKeysRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setShowDeleted(true)
* .build();
* ApiFuture future = apiKeysClient.listKeysPagedCallable().futureCall(request);
* // Do something.
* for (Key element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listKeysPagedCallable() {
return stub.listKeysPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the API keys owned by a project. The key string of the API key isn't included in the
* response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* ListKeysRequest request =
* ListKeysRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setShowDeleted(true)
* .build();
* while (true) {
* ListKeysResponse response = apiKeysClient.listKeysCallable().call(request);
* for (Key element : response.getKeysList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listKeysCallable() {
return stub.listKeysCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the metadata for an API key. The key string of the API key isn't included in the response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* KeyName name = KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]");
* Key response = apiKeysClient.getKey(name);
* }
* }
*
* @param name Required. The resource name of the API key to get.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Key getKey(KeyName name) {
GetKeyRequest request =
GetKeyRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the metadata for an API key. The key string of the API key isn't included in the response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* String name = KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString();
* Key response = apiKeysClient.getKey(name);
* }
* }
*
* @param name Required. The resource name of the API key to get.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Key getKey(String name) {
GetKeyRequest request = GetKeyRequest.newBuilder().setName(name).build();
return getKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the metadata for an API key. The key string of the API key isn't included in the response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* GetKeyRequest request =
* GetKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .build();
* Key response = apiKeysClient.getKey(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Key getKey(GetKeyRequest request) {
return getKeyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the metadata for an API key. The key string of the API key isn't included in the response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* GetKeyRequest request =
* GetKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .build();
* ApiFuture future = apiKeysClient.getKeyCallable().futureCall(request);
* // Do something.
* Key response = future.get();
* }
* }
*/
public final UnaryCallable getKeyCallable() {
return stub.getKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get the key string for an API key.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* KeyName name = KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]");
* GetKeyStringResponse response = apiKeysClient.getKeyString(name);
* }
* }
*
* @param name Required. The resource name of the API key to be retrieved.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetKeyStringResponse getKeyString(KeyName name) {
GetKeyStringRequest request =
GetKeyStringRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getKeyString(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get the key string for an API key.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* String name = KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString();
* GetKeyStringResponse response = apiKeysClient.getKeyString(name);
* }
* }
*
* @param name Required. The resource name of the API key to be retrieved.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetKeyStringResponse getKeyString(String name) {
GetKeyStringRequest request = GetKeyStringRequest.newBuilder().setName(name).build();
return getKeyString(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get the key string for an API key.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* GetKeyStringRequest request =
* GetKeyStringRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .build();
* GetKeyStringResponse response = apiKeysClient.getKeyString(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetKeyStringResponse getKeyString(GetKeyStringRequest request) {
return getKeyStringCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get the key string for an API key.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* GetKeyStringRequest request =
* GetKeyStringRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .build();
* ApiFuture future =
* apiKeysClient.getKeyStringCallable().futureCall(request);
* // Do something.
* GetKeyStringResponse response = future.get();
* }
* }
*/
public final UnaryCallable getKeyStringCallable() {
return stub.getKeyStringCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Patches the modifiable fields of an API key. The key string of the API key isn't included in
* the response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* Key key = Key.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Key response = apiKeysClient.updateKeyAsync(key, updateMask).get();
* }
* }
*
* @param key Required. Set the `name` field to the resource name of the API key to be updated.
* You can update only the `display_name`, `restrictions`, and `annotations` fields.
* @param updateMask The field mask specifies which fields to be updated as part of this request.
* All other fields are ignored. Mutable fields are: `display_name`, `restrictions`, and
* `annotations`. If an update mask is not provided, the service treats it as an implied mask
* equivalent to all allowed fields that are set on the wire. If the field mask has a special
* value "*", the service treats it equivalent to replace all allowed mutable fields.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateKeyAsync(Key key, FieldMask updateMask) {
UpdateKeyRequest request =
UpdateKeyRequest.newBuilder().setKey(key).setUpdateMask(updateMask).build();
return updateKeyAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Patches the modifiable fields of an API key. The key string of the API key isn't included in
* the response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* UpdateKeyRequest request =
* UpdateKeyRequest.newBuilder()
* .setKey(Key.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Key response = apiKeysClient.updateKeyAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateKeyAsync(UpdateKeyRequest request) {
return updateKeyOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Patches the modifiable fields of an API key. The key string of the API key isn't included in
* the response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* UpdateKeyRequest request =
* UpdateKeyRequest.newBuilder()
* .setKey(Key.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* OperationFuture future =
* apiKeysClient.updateKeyOperationCallable().futureCall(request);
* // Do something.
* Key response = future.get();
* }
* }
*/
public final OperationCallable updateKeyOperationCallable() {
return stub.updateKeyOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Patches the modifiable fields of an API key. The key string of the API key isn't included in
* the response.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* UpdateKeyRequest request =
* UpdateKeyRequest.newBuilder()
* .setKey(Key.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = apiKeysClient.updateKeyCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateKeyCallable() {
return stub.updateKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an API key. Deleted key can be retrieved within 30 days of deletion. Afterward, key
* will be purged from the project.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* KeyName name = KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]");
* Key response = apiKeysClient.deleteKeyAsync(name).get();
* }
* }
*
* @param name Required. The resource name of the API key to be deleted.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteKeyAsync(KeyName name) {
DeleteKeyRequest request =
DeleteKeyRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteKeyAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an API key. Deleted key can be retrieved within 30 days of deletion. Afterward, key
* will be purged from the project.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* String name = KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString();
* Key response = apiKeysClient.deleteKeyAsync(name).get();
* }
* }
*
* @param name Required. The resource name of the API key to be deleted.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteKeyAsync(String name) {
DeleteKeyRequest request = DeleteKeyRequest.newBuilder().setName(name).build();
return deleteKeyAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an API key. Deleted key can be retrieved within 30 days of deletion. Afterward, key
* will be purged from the project.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* DeleteKeyRequest request =
* DeleteKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .setEtag("etag3123477")
* .build();
* Key response = apiKeysClient.deleteKeyAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteKeyAsync(DeleteKeyRequest request) {
return deleteKeyOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an API key. Deleted key can be retrieved within 30 days of deletion. Afterward, key
* will be purged from the project.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* DeleteKeyRequest request =
* DeleteKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .setEtag("etag3123477")
* .build();
* OperationFuture future =
* apiKeysClient.deleteKeyOperationCallable().futureCall(request);
* // Do something.
* Key response = future.get();
* }
* }
*/
public final OperationCallable deleteKeyOperationCallable() {
return stub.deleteKeyOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an API key. Deleted key can be retrieved within 30 days of deletion. Afterward, key
* will be purged from the project.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* DeleteKeyRequest request =
* DeleteKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .setEtag("etag3123477")
* .build();
* ApiFuture future = apiKeysClient.deleteKeyCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable deleteKeyCallable() {
return stub.deleteKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Undeletes an API key which was deleted within 30 days.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* UndeleteKeyRequest request =
* UndeleteKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .build();
* Key response = apiKeysClient.undeleteKeyAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture undeleteKeyAsync(UndeleteKeyRequest request) {
return undeleteKeyOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Undeletes an API key which was deleted within 30 days.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* UndeleteKeyRequest request =
* UndeleteKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .build();
* OperationFuture future =
* apiKeysClient.undeleteKeyOperationCallable().futureCall(request);
* // Do something.
* Key response = future.get();
* }
* }
*/
public final OperationCallable undeleteKeyOperationCallable() {
return stub.undeleteKeyOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Undeletes an API key which was deleted within 30 days.
*
* NOTE: Key is a global resource; hence the only supported value for location is `global`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* UndeleteKeyRequest request =
* UndeleteKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[LOCATION]", "[KEY]").toString())
* .build();
* ApiFuture future = apiKeysClient.undeleteKeyCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable undeleteKeyCallable() {
return stub.undeleteKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Find the parent project and resource name of the API key that matches the key string in the
* request. If the API key has been purged, resource name will not be set. The service account
* must have the `apikeys.keys.lookup` permission on the parent project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* LookupKeyRequest request =
* LookupKeyRequest.newBuilder().setKeyString("keyString-1988270256").build();
* LookupKeyResponse response = apiKeysClient.lookupKey(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final LookupKeyResponse lookupKey(LookupKeyRequest request) {
return lookupKeyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Find the parent project and resource name of the API key that matches the key string in the
* request. If the API key has been purged, resource name will not be set. The service account
* must have the `apikeys.keys.lookup` permission on the parent project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ApiKeysClient apiKeysClient = ApiKeysClient.create()) {
* LookupKeyRequest request =
* LookupKeyRequest.newBuilder().setKeyString("keyString-1988270256").build();
* ApiFuture future = apiKeysClient.lookupKeyCallable().futureCall(request);
* // Do something.
* LookupKeyResponse response = future.get();
* }
* }
*/
public final UnaryCallable lookupKeyCallable() {
return stub.lookupKeyCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListKeysPagedResponse
extends AbstractPagedListResponse<
ListKeysRequest, ListKeysResponse, Key, ListKeysPage, ListKeysFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListKeysPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListKeysPagedResponse(input), MoreExecutors.directExecutor());
}
private ListKeysPagedResponse(ListKeysPage page) {
super(page, ListKeysFixedSizeCollection.createEmptyCollection());
}
}
public static class ListKeysPage
extends AbstractPage {
private ListKeysPage(
PageContext context, ListKeysResponse response) {
super(context, response);
}
private static ListKeysPage createEmptyPage() {
return new ListKeysPage(null, null);
}
@Override
protected ListKeysPage createPage(
PageContext context, ListKeysResponse response) {
return new ListKeysPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListKeysFixedSizeCollection
extends AbstractFixedSizeCollection<
ListKeysRequest, ListKeysResponse, Key, ListKeysPage, ListKeysFixedSizeCollection> {
private ListKeysFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListKeysFixedSizeCollection createEmptyCollection() {
return new ListKeysFixedSizeCollection(null, 0);
}
@Override
protected ListKeysFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListKeysFixedSizeCollection(pages, collectionSize);
}
}
}