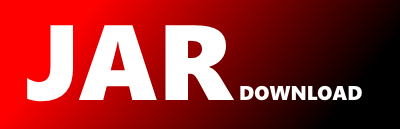
com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient Maven / Gradle / Ivy
Show all versions of google-cloud-bare-metal-solution Show documentation
/*
* Copyright 2021 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.baremetalsolution.v2;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.baremetalsolution.v2.stub.BareMetalSolutionStub;
import com.google.cloud.baremetalsolution.v2.stub.BareMetalSolutionStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.longrunning.Operation;
import com.google.longrunning.OperationsClient;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Performs management operations on Bare Metal Solution servers.
*
* The `baremetalsolution.googleapis.com` service provides management capabilities for Bare Metal
* Solution servers. To access the API methods, you must assign Bare Metal Solution IAM roles
* containing the desired permissions to your staff in your Google Cloud project. You must also
* enable the Bare Metal Solution API. Once enabled, the methods act upon specific servers in your
* Bare Metal Solution environment.
*
*
This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* InstanceName name = InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]");
* Instance response = bareMetalSolutionClient.getInstance(name);
* }
* }
*
* Note: close() needs to be called on the BareMetalSolutionClient object to clean up resources
* such as threads. In the example above, try-with-resources is used, which automatically calls
* close().
*
*
The surface of this class includes several types of Java methods for each of the API's
* methods:
*
*
* - A "flattened" method. With this type of method, the fields of the request type have been
* converted into function parameters. It may be the case that not all fields are available as
* parameters, and not every API method will have a flattened method entry point.
*
- A "request object" method. This type of method only takes one parameter, a request object,
* which must be constructed before the call. Not every API method will have a request object
* method.
*
- A "callable" method. This type of method takes no parameters and returns an immutable API
* callable object, which can be used to initiate calls to the service.
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of BareMetalSolutionSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* BareMetalSolutionSettings bareMetalSolutionSettings =
* BareMetalSolutionSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* BareMetalSolutionClient bareMetalSolutionClient =
* BareMetalSolutionClient.create(bareMetalSolutionSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* BareMetalSolutionSettings bareMetalSolutionSettings =
* BareMetalSolutionSettings.newBuilder().setEndpoint(myEndpoint).build();
* BareMetalSolutionClient bareMetalSolutionClient =
* BareMetalSolutionClient.create(bareMetalSolutionSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class BareMetalSolutionClient implements BackgroundResource {
private final BareMetalSolutionSettings settings;
private final BareMetalSolutionStub stub;
private final OperationsClient operationsClient;
/** Constructs an instance of BareMetalSolutionClient with default settings. */
public static final BareMetalSolutionClient create() throws IOException {
return create(BareMetalSolutionSettings.newBuilder().build());
}
/**
* Constructs an instance of BareMetalSolutionClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final BareMetalSolutionClient create(BareMetalSolutionSettings settings)
throws IOException {
return new BareMetalSolutionClient(settings);
}
/**
* Constructs an instance of BareMetalSolutionClient, using the given stub for making calls. This
* is for advanced usage - prefer using create(BareMetalSolutionSettings).
*/
@BetaApi("A restructuring of stub classes is planned, so this may break in the future")
public static final BareMetalSolutionClient create(BareMetalSolutionStub stub) {
return new BareMetalSolutionClient(stub);
}
/**
* Constructs an instance of BareMetalSolutionClient, using the given settings. This is protected
* so that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected BareMetalSolutionClient(BareMetalSolutionSettings settings) throws IOException {
this.settings = settings;
this.stub = ((BareMetalSolutionStubSettings) settings.getStubSettings()).createStub();
this.operationsClient = OperationsClient.create(this.stub.getOperationsStub());
}
@BetaApi("A restructuring of stub classes is planned, so this may break in the future")
protected BareMetalSolutionClient(BareMetalSolutionStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient = OperationsClient.create(this.stub.getOperationsStub());
}
public final BareMetalSolutionSettings getSettings() {
return settings;
}
@BetaApi("A restructuring of stub classes is planned, so this may break in the future")
public BareMetalSolutionStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final OperationsClient getOperationsClient() {
return operationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List servers in a given project and location.
*
*
Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Instance element : bareMetalSolutionClient.listInstances(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListInstancesRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInstancesPagedResponse listInstances(LocationName parent) {
ListInstancesRequest request =
ListInstancesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listInstances(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List servers in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Instance element : bareMetalSolutionClient.listInstances(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListInstancesRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInstancesPagedResponse listInstances(String parent) {
ListInstancesRequest request = ListInstancesRequest.newBuilder().setParent(parent).build();
return listInstances(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List servers in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListInstancesRequest request =
* ListInstancesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Instance element : bareMetalSolutionClient.listInstances(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInstancesPagedResponse listInstances(ListInstancesRequest request) {
return listInstancesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List servers in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListInstancesRequest request =
* ListInstancesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* bareMetalSolutionClient.listInstancesPagedCallable().futureCall(request);
* // Do something.
* for (Instance element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listInstancesPagedCallable() {
return stub.listInstancesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List servers in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListInstancesRequest request =
* ListInstancesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListInstancesResponse response =
* bareMetalSolutionClient.listInstancesCallable().call(request);
* for (Instance element : response.getResponsesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listInstancesCallable() {
return stub.listInstancesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details about a single server.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* InstanceName name = InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]");
* Instance response = bareMetalSolutionClient.getInstance(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Instance getInstance(InstanceName name) {
GetInstanceRequest request =
GetInstanceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getInstance(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details about a single server.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String name = InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]").toString();
* Instance response = bareMetalSolutionClient.getInstance(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Instance getInstance(String name) {
GetInstanceRequest request = GetInstanceRequest.newBuilder().setName(name).build();
return getInstance(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details about a single server.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetInstanceRequest request =
* GetInstanceRequest.newBuilder()
* .setName(InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]").toString())
* .build();
* Instance response = bareMetalSolutionClient.getInstance(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Instance getInstance(GetInstanceRequest request) {
return getInstanceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details about a single server.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetInstanceRequest request =
* GetInstanceRequest.newBuilder()
* .setName(InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]").toString())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.getInstanceCallable().futureCall(request);
* // Do something.
* Instance response = future.get();
* }
* }
*/
public final UnaryCallable getInstanceCallable() {
return stub.getInstanceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an ungraceful, hard reset on a server. Equivalent to shutting the power off and then
* turning it back on.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* InstanceName name = InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]");
* ResetInstanceResponse response = bareMetalSolutionClient.resetInstanceAsync(name).get();
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture resetInstanceAsync(
InstanceName name) {
ResetInstanceRequest request =
ResetInstanceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return resetInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an ungraceful, hard reset on a server. Equivalent to shutting the power off and then
* turning it back on.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String name = InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]").toString();
* ResetInstanceResponse response = bareMetalSolutionClient.resetInstanceAsync(name).get();
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture resetInstanceAsync(
String name) {
ResetInstanceRequest request = ResetInstanceRequest.newBuilder().setName(name).build();
return resetInstanceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an ungraceful, hard reset on a server. Equivalent to shutting the power off and then
* turning it back on.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ResetInstanceRequest request =
* ResetInstanceRequest.newBuilder()
* .setName(InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]").toString())
* .build();
* ResetInstanceResponse response = bareMetalSolutionClient.resetInstanceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture resetInstanceAsync(
ResetInstanceRequest request) {
return resetInstanceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an ungraceful, hard reset on a server. Equivalent to shutting the power off and then
* turning it back on.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ResetInstanceRequest request =
* ResetInstanceRequest.newBuilder()
* .setName(InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]").toString())
* .build();
* OperationFuture future =
* bareMetalSolutionClient.resetInstanceOperationCallable().futureCall(request);
* // Do something.
* ResetInstanceResponse response = future.get();
* }
* }
*/
public final OperationCallable
resetInstanceOperationCallable() {
return stub.resetInstanceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Perform an ungraceful, hard reset on a server. Equivalent to shutting the power off and then
* turning it back on.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ResetInstanceRequest request =
* ResetInstanceRequest.newBuilder()
* .setName(InstanceName.of("[PROJECT]", "[LOCATION]", "[INSTANCE]").toString())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.resetInstanceCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable resetInstanceCallable() {
return stub.resetInstanceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volumes in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Volume element : bareMetalSolutionClient.listVolumes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListVolumesRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumesPagedResponse listVolumes(LocationName parent) {
ListVolumesRequest request =
ListVolumesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listVolumes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volumes in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Volume element : bareMetalSolutionClient.listVolumes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListVolumesRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumesPagedResponse listVolumes(String parent) {
ListVolumesRequest request = ListVolumesRequest.newBuilder().setParent(parent).build();
return listVolumes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volumes in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListVolumesRequest request =
* ListVolumesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Volume element : bareMetalSolutionClient.listVolumes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumesPagedResponse listVolumes(ListVolumesRequest request) {
return listVolumesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volumes in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListVolumesRequest request =
* ListVolumesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* bareMetalSolutionClient.listVolumesPagedCallable().futureCall(request);
* // Do something.
* for (Volume element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listVolumesPagedCallable() {
return stub.listVolumesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volumes in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListVolumesRequest request =
* ListVolumesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListVolumesResponse response = bareMetalSolutionClient.listVolumesCallable().call(request);
* for (Volume element : response.getResponsesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listVolumesCallable() {
return stub.listVolumesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* VolumeName name = VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]");
* Volume response = bareMetalSolutionClient.getVolume(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Volume getVolume(VolumeName name) {
GetVolumeRequest request =
GetVolumeRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getVolume(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String name = VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString();
* Volume response = bareMetalSolutionClient.getVolume(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Volume getVolume(String name) {
GetVolumeRequest request = GetVolumeRequest.newBuilder().setName(name).build();
return getVolume(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetVolumeRequest request =
* GetVolumeRequest.newBuilder()
* .setName(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .build();
* Volume response = bareMetalSolutionClient.getVolume(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Volume getVolume(GetVolumeRequest request) {
return getVolumeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetVolumeRequest request =
* GetVolumeRequest.newBuilder()
* .setName(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .build();
* ApiFuture future = bareMetalSolutionClient.getVolumeCallable().futureCall(request);
* // Do something.
* Volume response = future.get();
* }
* }
*/
public final UnaryCallable getVolumeCallable() {
return stub.getVolumeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update details of a single storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* Volume volume = Volume.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Volume response = bareMetalSolutionClient.updateVolumeAsync(volume, updateMask).get();
* }
* }
*
* @param volume Required. The volume to update.
* The `name` field is used to identify the volume to update. Format:
* projects/{project}/locations/{location}/volumes/{volume}
* @param updateMask The list of fields to update. The only currently supported fields are:
* `snapshot_auto_delete_behavior` `snapshot_schedule_policy_name`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateVolumeAsync(
Volume volume, FieldMask updateMask) {
UpdateVolumeRequest request =
UpdateVolumeRequest.newBuilder().setVolume(volume).setUpdateMask(updateMask).build();
return updateVolumeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update details of a single storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* UpdateVolumeRequest request =
* UpdateVolumeRequest.newBuilder()
* .setVolume(Volume.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Volume response = bareMetalSolutionClient.updateVolumeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateVolumeAsync(
UpdateVolumeRequest request) {
return updateVolumeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update details of a single storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* UpdateVolumeRequest request =
* UpdateVolumeRequest.newBuilder()
* .setVolume(Volume.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* OperationFuture future =
* bareMetalSolutionClient.updateVolumeOperationCallable().futureCall(request);
* // Do something.
* Volume response = future.get();
* }
* }
*/
public final OperationCallable
updateVolumeOperationCallable() {
return stub.updateVolumeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update details of a single storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* UpdateVolumeRequest request =
* UpdateVolumeRequest.newBuilder()
* .setVolume(Volume.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.updateVolumeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateVolumeCallable() {
return stub.updateVolumeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List network in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Network element : bareMetalSolutionClient.listNetworks(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListNetworksRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListNetworksPagedResponse listNetworks(LocationName parent) {
ListNetworksRequest request =
ListNetworksRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listNetworks(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List network in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Network element : bareMetalSolutionClient.listNetworks(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListNetworksRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListNetworksPagedResponse listNetworks(String parent) {
ListNetworksRequest request = ListNetworksRequest.newBuilder().setParent(parent).build();
return listNetworks(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List network in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListNetworksRequest request =
* ListNetworksRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Network element : bareMetalSolutionClient.listNetworks(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListNetworksPagedResponse listNetworks(ListNetworksRequest request) {
return listNetworksPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List network in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListNetworksRequest request =
* ListNetworksRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* bareMetalSolutionClient.listNetworksPagedCallable().futureCall(request);
* // Do something.
* for (Network element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listNetworksPagedCallable() {
return stub.listNetworksPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List network in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListNetworksRequest request =
* ListNetworksRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListNetworksResponse response =
* bareMetalSolutionClient.listNetworksCallable().call(request);
* for (Network element : response.getResponsesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listNetworksCallable() {
return stub.listNetworksCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single network.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* NetworkName name = NetworkName.of("[PROJECT]", "[LOCATION]", "[NETWORK]");
* Network response = bareMetalSolutionClient.getNetwork(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Network getNetwork(NetworkName name) {
GetNetworkRequest request =
GetNetworkRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getNetwork(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single network.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String name = NetworkName.of("[PROJECT]", "[LOCATION]", "[NETWORK]").toString();
* Network response = bareMetalSolutionClient.getNetwork(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Network getNetwork(String name) {
GetNetworkRequest request = GetNetworkRequest.newBuilder().setName(name).build();
return getNetwork(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single network.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetNetworkRequest request =
* GetNetworkRequest.newBuilder()
* .setName(NetworkName.of("[PROJECT]", "[LOCATION]", "[NETWORK]").toString())
* .build();
* Network response = bareMetalSolutionClient.getNetwork(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Network getNetwork(GetNetworkRequest request) {
return getNetworkCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single network.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetNetworkRequest request =
* GetNetworkRequest.newBuilder()
* .setName(NetworkName.of("[PROJECT]", "[LOCATION]", "[NETWORK]").toString())
* .build();
* ApiFuture future = bareMetalSolutionClient.getNetworkCallable().futureCall(request);
* // Do something.
* Network response = future.get();
* }
* }
*/
public final UnaryCallable getNetworkCallable() {
return stub.getNetworkCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List snapshot schedule policies in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (SnapshotSchedulePolicy element :
* bareMetalSolutionClient.listSnapshotSchedulePolicies(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent project containing the Snapshot Schedule Policies.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSnapshotSchedulePoliciesPagedResponse listSnapshotSchedulePolicies(
LocationName parent) {
ListSnapshotSchedulePoliciesRequest request =
ListSnapshotSchedulePoliciesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listSnapshotSchedulePolicies(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List snapshot schedule policies in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (SnapshotSchedulePolicy element :
* bareMetalSolutionClient.listSnapshotSchedulePolicies(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent project containing the Snapshot Schedule Policies.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSnapshotSchedulePoliciesPagedResponse listSnapshotSchedulePolicies(
String parent) {
ListSnapshotSchedulePoliciesRequest request =
ListSnapshotSchedulePoliciesRequest.newBuilder().setParent(parent).build();
return listSnapshotSchedulePolicies(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List snapshot schedule policies in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListSnapshotSchedulePoliciesRequest request =
* ListSnapshotSchedulePoliciesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (SnapshotSchedulePolicy element :
* bareMetalSolutionClient.listSnapshotSchedulePolicies(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSnapshotSchedulePoliciesPagedResponse listSnapshotSchedulePolicies(
ListSnapshotSchedulePoliciesRequest request) {
return listSnapshotSchedulePoliciesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List snapshot schedule policies in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListSnapshotSchedulePoliciesRequest request =
* ListSnapshotSchedulePoliciesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* bareMetalSolutionClient.listSnapshotSchedulePoliciesPagedCallable().futureCall(request);
* // Do something.
* for (SnapshotSchedulePolicy element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable<
ListSnapshotSchedulePoliciesRequest, ListSnapshotSchedulePoliciesPagedResponse>
listSnapshotSchedulePoliciesPagedCallable() {
return stub.listSnapshotSchedulePoliciesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List snapshot schedule policies in a given project and location.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListSnapshotSchedulePoliciesRequest request =
* ListSnapshotSchedulePoliciesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListSnapshotSchedulePoliciesResponse response =
* bareMetalSolutionClient.listSnapshotSchedulePoliciesCallable().call(request);
* for (SnapshotSchedulePolicy element : response.getResponsesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable<
ListSnapshotSchedulePoliciesRequest, ListSnapshotSchedulePoliciesResponse>
listSnapshotSchedulePoliciesCallable() {
return stub.listSnapshotSchedulePoliciesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single snapshot schedule policy.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* SnapshotSchedulePolicyName name =
* SnapshotSchedulePolicyName.of("[PROJECT]", "[LOCATION]", "[SNAPSHOT_SCHEDULE_POLICY]");
* SnapshotSchedulePolicy response = bareMetalSolutionClient.getSnapshotSchedulePolicy(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SnapshotSchedulePolicy getSnapshotSchedulePolicy(SnapshotSchedulePolicyName name) {
GetSnapshotSchedulePolicyRequest request =
GetSnapshotSchedulePolicyRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getSnapshotSchedulePolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single snapshot schedule policy.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String name =
* SnapshotSchedulePolicyName.of("[PROJECT]", "[LOCATION]", "[SNAPSHOT_SCHEDULE_POLICY]")
* .toString();
* SnapshotSchedulePolicy response = bareMetalSolutionClient.getSnapshotSchedulePolicy(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SnapshotSchedulePolicy getSnapshotSchedulePolicy(String name) {
GetSnapshotSchedulePolicyRequest request =
GetSnapshotSchedulePolicyRequest.newBuilder().setName(name).build();
return getSnapshotSchedulePolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single snapshot schedule policy.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetSnapshotSchedulePolicyRequest request =
* GetSnapshotSchedulePolicyRequest.newBuilder()
* .setName(
* SnapshotSchedulePolicyName.of(
* "[PROJECT]", "[LOCATION]", "[SNAPSHOT_SCHEDULE_POLICY]")
* .toString())
* .build();
* SnapshotSchedulePolicy response = bareMetalSolutionClient.getSnapshotSchedulePolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SnapshotSchedulePolicy getSnapshotSchedulePolicy(
GetSnapshotSchedulePolicyRequest request) {
return getSnapshotSchedulePolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single snapshot schedule policy.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetSnapshotSchedulePolicyRequest request =
* GetSnapshotSchedulePolicyRequest.newBuilder()
* .setName(
* SnapshotSchedulePolicyName.of(
* "[PROJECT]", "[LOCATION]", "[SNAPSHOT_SCHEDULE_POLICY]")
* .toString())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.getSnapshotSchedulePolicyCallable().futureCall(request);
* // Do something.
* SnapshotSchedulePolicy response = future.get();
* }
* }
*/
public final UnaryCallable
getSnapshotSchedulePolicyCallable() {
return stub.getSnapshotSchedulePolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a snapshot schedule policy in the specified project.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* SnapshotSchedulePolicy snapshotSchedulePolicy = SnapshotSchedulePolicy.newBuilder().build();
* String snapshotSchedulePolicyId = "snapshotSchedulePolicyId1929948040";
* SnapshotSchedulePolicy response =
* bareMetalSolutionClient.createSnapshotSchedulePolicy(
* parent, snapshotSchedulePolicy, snapshotSchedulePolicyId);
* }
* }
*
* @param parent Required. The parent project and location containing the SnapshotSchedulePolicy.
* @param snapshotSchedulePolicy Required. The SnapshotSchedulePolicy to create.
* @param snapshotSchedulePolicyId Required. Snapshot policy ID
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SnapshotSchedulePolicy createSnapshotSchedulePolicy(
LocationName parent,
SnapshotSchedulePolicy snapshotSchedulePolicy,
String snapshotSchedulePolicyId) {
CreateSnapshotSchedulePolicyRequest request =
CreateSnapshotSchedulePolicyRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setSnapshotSchedulePolicy(snapshotSchedulePolicy)
.setSnapshotSchedulePolicyId(snapshotSchedulePolicyId)
.build();
return createSnapshotSchedulePolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a snapshot schedule policy in the specified project.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* SnapshotSchedulePolicy snapshotSchedulePolicy = SnapshotSchedulePolicy.newBuilder().build();
* String snapshotSchedulePolicyId = "snapshotSchedulePolicyId1929948040";
* SnapshotSchedulePolicy response =
* bareMetalSolutionClient.createSnapshotSchedulePolicy(
* parent, snapshotSchedulePolicy, snapshotSchedulePolicyId);
* }
* }
*
* @param parent Required. The parent project and location containing the SnapshotSchedulePolicy.
* @param snapshotSchedulePolicy Required. The SnapshotSchedulePolicy to create.
* @param snapshotSchedulePolicyId Required. Snapshot policy ID
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SnapshotSchedulePolicy createSnapshotSchedulePolicy(
String parent,
SnapshotSchedulePolicy snapshotSchedulePolicy,
String snapshotSchedulePolicyId) {
CreateSnapshotSchedulePolicyRequest request =
CreateSnapshotSchedulePolicyRequest.newBuilder()
.setParent(parent)
.setSnapshotSchedulePolicy(snapshotSchedulePolicy)
.setSnapshotSchedulePolicyId(snapshotSchedulePolicyId)
.build();
return createSnapshotSchedulePolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a snapshot schedule policy in the specified project.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* CreateSnapshotSchedulePolicyRequest request =
* CreateSnapshotSchedulePolicyRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setSnapshotSchedulePolicy(SnapshotSchedulePolicy.newBuilder().build())
* .setSnapshotSchedulePolicyId("snapshotSchedulePolicyId1929948040")
* .build();
* SnapshotSchedulePolicy response =
* bareMetalSolutionClient.createSnapshotSchedulePolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SnapshotSchedulePolicy createSnapshotSchedulePolicy(
CreateSnapshotSchedulePolicyRequest request) {
return createSnapshotSchedulePolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a snapshot schedule policy in the specified project.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* CreateSnapshotSchedulePolicyRequest request =
* CreateSnapshotSchedulePolicyRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setSnapshotSchedulePolicy(SnapshotSchedulePolicy.newBuilder().build())
* .setSnapshotSchedulePolicyId("snapshotSchedulePolicyId1929948040")
* .build();
* ApiFuture future =
* bareMetalSolutionClient.createSnapshotSchedulePolicyCallable().futureCall(request);
* // Do something.
* SnapshotSchedulePolicy response = future.get();
* }
* }
*/
public final UnaryCallable
createSnapshotSchedulePolicyCallable() {
return stub.createSnapshotSchedulePolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a snapshot schedule policy in the specified project.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* SnapshotSchedulePolicy snapshotSchedulePolicy = SnapshotSchedulePolicy.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* SnapshotSchedulePolicy response =
* bareMetalSolutionClient.updateSnapshotSchedulePolicy(snapshotSchedulePolicy, updateMask);
* }
* }
*
* @param snapshotSchedulePolicy Required. The snapshot schedule policy to update.
* The `name` field is used to identify the snapshot schedule policy to update. Format:
* projects/{project}/locations/global/snapshotSchedulePolicies/{policy}
* @param updateMask Required. The list of fields to update.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SnapshotSchedulePolicy updateSnapshotSchedulePolicy(
SnapshotSchedulePolicy snapshotSchedulePolicy, FieldMask updateMask) {
UpdateSnapshotSchedulePolicyRequest request =
UpdateSnapshotSchedulePolicyRequest.newBuilder()
.setSnapshotSchedulePolicy(snapshotSchedulePolicy)
.setUpdateMask(updateMask)
.build();
return updateSnapshotSchedulePolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a snapshot schedule policy in the specified project.
*
*
Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* UpdateSnapshotSchedulePolicyRequest request =
* UpdateSnapshotSchedulePolicyRequest.newBuilder()
* .setSnapshotSchedulePolicy(SnapshotSchedulePolicy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* SnapshotSchedulePolicy response =
* bareMetalSolutionClient.updateSnapshotSchedulePolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SnapshotSchedulePolicy updateSnapshotSchedulePolicy(
UpdateSnapshotSchedulePolicyRequest request) {
return updateSnapshotSchedulePolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a snapshot schedule policy in the specified project.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* UpdateSnapshotSchedulePolicyRequest request =
* UpdateSnapshotSchedulePolicyRequest.newBuilder()
* .setSnapshotSchedulePolicy(SnapshotSchedulePolicy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.updateSnapshotSchedulePolicyCallable().futureCall(request);
* // Do something.
* SnapshotSchedulePolicy response = future.get();
* }
* }
*/
public final UnaryCallable
updateSnapshotSchedulePolicyCallable() {
return stub.updateSnapshotSchedulePolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Delete a named snapshot schedule policy.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* SnapshotSchedulePolicyName name =
* SnapshotSchedulePolicyName.of("[PROJECT]", "[LOCATION]", "[SNAPSHOT_SCHEDULE_POLICY]");
* bareMetalSolutionClient.deleteSnapshotSchedulePolicy(name);
* }
* }
*
* @param name Required. The name of the snapshot schedule policy to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteSnapshotSchedulePolicy(SnapshotSchedulePolicyName name) {
DeleteSnapshotSchedulePolicyRequest request =
DeleteSnapshotSchedulePolicyRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
deleteSnapshotSchedulePolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Delete a named snapshot schedule policy.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String name =
* SnapshotSchedulePolicyName.of("[PROJECT]", "[LOCATION]", "[SNAPSHOT_SCHEDULE_POLICY]")
* .toString();
* bareMetalSolutionClient.deleteSnapshotSchedulePolicy(name);
* }
* }
*
* @param name Required. The name of the snapshot schedule policy to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteSnapshotSchedulePolicy(String name) {
DeleteSnapshotSchedulePolicyRequest request =
DeleteSnapshotSchedulePolicyRequest.newBuilder().setName(name).build();
deleteSnapshotSchedulePolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Delete a named snapshot schedule policy.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* DeleteSnapshotSchedulePolicyRequest request =
* DeleteSnapshotSchedulePolicyRequest.newBuilder()
* .setName(
* SnapshotSchedulePolicyName.of(
* "[PROJECT]", "[LOCATION]", "[SNAPSHOT_SCHEDULE_POLICY]")
* .toString())
* .build();
* bareMetalSolutionClient.deleteSnapshotSchedulePolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteSnapshotSchedulePolicy(DeleteSnapshotSchedulePolicyRequest request) {
deleteSnapshotSchedulePolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Delete a named snapshot schedule policy.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* DeleteSnapshotSchedulePolicyRequest request =
* DeleteSnapshotSchedulePolicyRequest.newBuilder()
* .setName(
* SnapshotSchedulePolicyName.of(
* "[PROJECT]", "[LOCATION]", "[SNAPSHOT_SCHEDULE_POLICY]")
* .toString())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.deleteSnapshotSchedulePolicyCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable
deleteSnapshotSchedulePolicyCallable() {
return stub.deleteSnapshotSchedulePolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a storage volume snapshot in a containing volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* VolumeName parent = VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]");
* VolumeSnapshot volumeSnapshot = VolumeSnapshot.newBuilder().build();
* VolumeSnapshot response =
* bareMetalSolutionClient.createVolumeSnapshot(parent, volumeSnapshot);
* }
* }
*
* @param parent Required. The volume to snapshot.
* @param volumeSnapshot Required. The volume snapshot to create. Only the description field may
* be specified.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeSnapshot createVolumeSnapshot(
VolumeName parent, VolumeSnapshot volumeSnapshot) {
CreateVolumeSnapshotRequest request =
CreateVolumeSnapshotRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setVolumeSnapshot(volumeSnapshot)
.build();
return createVolumeSnapshot(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a storage volume snapshot in a containing volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String parent = VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString();
* VolumeSnapshot volumeSnapshot = VolumeSnapshot.newBuilder().build();
* VolumeSnapshot response =
* bareMetalSolutionClient.createVolumeSnapshot(parent, volumeSnapshot);
* }
* }
*
* @param parent Required. The volume to snapshot.
* @param volumeSnapshot Required. The volume snapshot to create. Only the description field may
* be specified.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeSnapshot createVolumeSnapshot(String parent, VolumeSnapshot volumeSnapshot) {
CreateVolumeSnapshotRequest request =
CreateVolumeSnapshotRequest.newBuilder()
.setParent(parent)
.setVolumeSnapshot(volumeSnapshot)
.build();
return createVolumeSnapshot(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a storage volume snapshot in a containing volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* CreateVolumeSnapshotRequest request =
* CreateVolumeSnapshotRequest.newBuilder()
* .setParent(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .setVolumeSnapshot(VolumeSnapshot.newBuilder().build())
* .build();
* VolumeSnapshot response = bareMetalSolutionClient.createVolumeSnapshot(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeSnapshot createVolumeSnapshot(CreateVolumeSnapshotRequest request) {
return createVolumeSnapshotCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a storage volume snapshot in a containing volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* CreateVolumeSnapshotRequest request =
* CreateVolumeSnapshotRequest.newBuilder()
* .setParent(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .setVolumeSnapshot(VolumeSnapshot.newBuilder().build())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.createVolumeSnapshotCallable().futureCall(request);
* // Do something.
* VolumeSnapshot response = future.get();
* }
* }
*/
public final UnaryCallable
createVolumeSnapshotCallable() {
return stub.createVolumeSnapshotCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restore a storage volume snapshot to its containing volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* VolumeSnapshotName volumeSnapshot =
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]");
* VolumeSnapshot response =
* bareMetalSolutionClient.restoreVolumeSnapshotAsync(volumeSnapshot).get();
* }
* }
*
* @param volumeSnapshot Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture restoreVolumeSnapshotAsync(
VolumeSnapshotName volumeSnapshot) {
RestoreVolumeSnapshotRequest request =
RestoreVolumeSnapshotRequest.newBuilder()
.setVolumeSnapshot(volumeSnapshot == null ? null : volumeSnapshot.toString())
.build();
return restoreVolumeSnapshotAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restore a storage volume snapshot to its containing volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String volumeSnapshot =
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]").toString();
* VolumeSnapshot response =
* bareMetalSolutionClient.restoreVolumeSnapshotAsync(volumeSnapshot).get();
* }
* }
*
* @param volumeSnapshot Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture restoreVolumeSnapshotAsync(
String volumeSnapshot) {
RestoreVolumeSnapshotRequest request =
RestoreVolumeSnapshotRequest.newBuilder().setVolumeSnapshot(volumeSnapshot).build();
return restoreVolumeSnapshotAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restore a storage volume snapshot to its containing volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* RestoreVolumeSnapshotRequest request =
* RestoreVolumeSnapshotRequest.newBuilder()
* .setVolumeSnapshot(
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]")
* .toString())
* .build();
* VolumeSnapshot response = bareMetalSolutionClient.restoreVolumeSnapshotAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture restoreVolumeSnapshotAsync(
RestoreVolumeSnapshotRequest request) {
return restoreVolumeSnapshotOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restore a storage volume snapshot to its containing volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* RestoreVolumeSnapshotRequest request =
* RestoreVolumeSnapshotRequest.newBuilder()
* .setVolumeSnapshot(
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]")
* .toString())
* .build();
* OperationFuture future =
* bareMetalSolutionClient.restoreVolumeSnapshotOperationCallable().futureCall(request);
* // Do something.
* VolumeSnapshot response = future.get();
* }
* }
*/
public final OperationCallable
restoreVolumeSnapshotOperationCallable() {
return stub.restoreVolumeSnapshotOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restore a storage volume snapshot to its containing volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* RestoreVolumeSnapshotRequest request =
* RestoreVolumeSnapshotRequest.newBuilder()
* .setVolumeSnapshot(
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]")
* .toString())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.restoreVolumeSnapshotCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
restoreVolumeSnapshotCallable() {
return stub.restoreVolumeSnapshotCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a storage volume snapshot for a given volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* VolumeSnapshotName name =
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]");
* bareMetalSolutionClient.deleteVolumeSnapshot(name);
* }
* }
*
* @param name Required. The name of the snapshot to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteVolumeSnapshot(VolumeSnapshotName name) {
DeleteVolumeSnapshotRequest request =
DeleteVolumeSnapshotRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
deleteVolumeSnapshot(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a storage volume snapshot for a given volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String name =
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]").toString();
* bareMetalSolutionClient.deleteVolumeSnapshot(name);
* }
* }
*
* @param name Required. The name of the snapshot to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteVolumeSnapshot(String name) {
DeleteVolumeSnapshotRequest request =
DeleteVolumeSnapshotRequest.newBuilder().setName(name).build();
deleteVolumeSnapshot(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a storage volume snapshot for a given volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* DeleteVolumeSnapshotRequest request =
* DeleteVolumeSnapshotRequest.newBuilder()
* .setName(
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]")
* .toString())
* .build();
* bareMetalSolutionClient.deleteVolumeSnapshot(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteVolumeSnapshot(DeleteVolumeSnapshotRequest request) {
deleteVolumeSnapshotCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a storage volume snapshot for a given volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* DeleteVolumeSnapshotRequest request =
* DeleteVolumeSnapshotRequest.newBuilder()
* .setName(
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]")
* .toString())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.deleteVolumeSnapshotCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteVolumeSnapshotCallable() {
return stub.deleteVolumeSnapshotCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage volume snapshot.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* VolumeSnapshotName name =
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]");
* VolumeSnapshot response = bareMetalSolutionClient.getVolumeSnapshot(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeSnapshot getVolumeSnapshot(VolumeSnapshotName name) {
GetVolumeSnapshotRequest request =
GetVolumeSnapshotRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getVolumeSnapshot(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage volume snapshot.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String name =
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]").toString();
* VolumeSnapshot response = bareMetalSolutionClient.getVolumeSnapshot(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeSnapshot getVolumeSnapshot(String name) {
GetVolumeSnapshotRequest request = GetVolumeSnapshotRequest.newBuilder().setName(name).build();
return getVolumeSnapshot(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage volume snapshot.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetVolumeSnapshotRequest request =
* GetVolumeSnapshotRequest.newBuilder()
* .setName(
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]")
* .toString())
* .build();
* VolumeSnapshot response = bareMetalSolutionClient.getVolumeSnapshot(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeSnapshot getVolumeSnapshot(GetVolumeSnapshotRequest request) {
return getVolumeSnapshotCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage volume snapshot.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetVolumeSnapshotRequest request =
* GetVolumeSnapshotRequest.newBuilder()
* .setName(
* VolumeSnapshotName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[SNAPSHOT]")
* .toString())
* .build();
* ApiFuture future =
* bareMetalSolutionClient.getVolumeSnapshotCallable().futureCall(request);
* // Do something.
* VolumeSnapshot response = future.get();
* }
* }
*/
public final UnaryCallable getVolumeSnapshotCallable() {
return stub.getVolumeSnapshotCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume snapshots for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* VolumeName parent = VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]");
* for (VolumeSnapshot element :
* bareMetalSolutionClient.listVolumeSnapshots(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListVolumesRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumeSnapshotsPagedResponse listVolumeSnapshots(VolumeName parent) {
ListVolumeSnapshotsRequest request =
ListVolumeSnapshotsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listVolumeSnapshots(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume snapshots for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String parent = VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString();
* for (VolumeSnapshot element :
* bareMetalSolutionClient.listVolumeSnapshots(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListVolumesRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumeSnapshotsPagedResponse listVolumeSnapshots(String parent) {
ListVolumeSnapshotsRequest request =
ListVolumeSnapshotsRequest.newBuilder().setParent(parent).build();
return listVolumeSnapshots(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume snapshots for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListVolumeSnapshotsRequest request =
* ListVolumeSnapshotsRequest.newBuilder()
* .setParent(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (VolumeSnapshot element :
* bareMetalSolutionClient.listVolumeSnapshots(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumeSnapshotsPagedResponse listVolumeSnapshots(
ListVolumeSnapshotsRequest request) {
return listVolumeSnapshotsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume snapshots for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListVolumeSnapshotsRequest request =
* ListVolumeSnapshotsRequest.newBuilder()
* .setParent(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* bareMetalSolutionClient.listVolumeSnapshotsPagedCallable().futureCall(request);
* // Do something.
* for (VolumeSnapshot element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listVolumeSnapshotsPagedCallable() {
return stub.listVolumeSnapshotsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume snapshots for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListVolumeSnapshotsRequest request =
* ListVolumeSnapshotsRequest.newBuilder()
* .setParent(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListVolumeSnapshotsResponse response =
* bareMetalSolutionClient.listVolumeSnapshotsCallable().call(request);
* for (VolumeSnapshot element : response.getResponsesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listVolumeSnapshotsCallable() {
return stub.listVolumeSnapshotsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage logical unit number(LUN).
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* LunName name = LunName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[LUN]");
* Lun response = bareMetalSolutionClient.getLun(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Lun getLun(LunName name) {
GetLunRequest request =
GetLunRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getLun(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage logical unit number(LUN).
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String name = LunName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[LUN]").toString();
* Lun response = bareMetalSolutionClient.getLun(name);
* }
* }
*
* @param name Required. Name of the resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Lun getLun(String name) {
GetLunRequest request = GetLunRequest.newBuilder().setName(name).build();
return getLun(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage logical unit number(LUN).
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetLunRequest request =
* GetLunRequest.newBuilder()
* .setName(LunName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[LUN]").toString())
* .build();
* Lun response = bareMetalSolutionClient.getLun(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Lun getLun(GetLunRequest request) {
return getLunCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get details of a single storage logical unit number(LUN).
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* GetLunRequest request =
* GetLunRequest.newBuilder()
* .setName(LunName.of("[PROJECT]", "[LOCATION]", "[VOLUME]", "[LUN]").toString())
* .build();
* ApiFuture future = bareMetalSolutionClient.getLunCallable().futureCall(request);
* // Do something.
* Lun response = future.get();
* }
* }
*/
public final UnaryCallable getLunCallable() {
return stub.getLunCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume luns for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* VolumeName parent = VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]");
* for (Lun element : bareMetalSolutionClient.listLuns(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListLunsRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLunsPagedResponse listLuns(VolumeName parent) {
ListLunsRequest request =
ListLunsRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listLuns(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume luns for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* String parent = VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString();
* for (Lun element : bareMetalSolutionClient.listLuns(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListLunsRequest.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLunsPagedResponse listLuns(String parent) {
ListLunsRequest request = ListLunsRequest.newBuilder().setParent(parent).build();
return listLuns(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume luns for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListLunsRequest request =
* ListLunsRequest.newBuilder()
* .setParent(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Lun element : bareMetalSolutionClient.listLuns(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLunsPagedResponse listLuns(ListLunsRequest request) {
return listLunsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume luns for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListLunsRequest request =
* ListLunsRequest.newBuilder()
* .setParent(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future = bareMetalSolutionClient.listLunsPagedCallable().futureCall(request);
* // Do something.
* for (Lun element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listLunsPagedCallable() {
return stub.listLunsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List storage volume luns for given storage volume.
*
* Sample code:
*
*
{@code
* try (BareMetalSolutionClient bareMetalSolutionClient = BareMetalSolutionClient.create()) {
* ListLunsRequest request =
* ListLunsRequest.newBuilder()
* .setParent(VolumeName.of("[PROJECT]", "[LOCATION]", "[VOLUME]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLunsResponse response = bareMetalSolutionClient.listLunsCallable().call(request);
* for (Lun element : response.getResponsesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLunsCallable() {
return stub.listLunsCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListInstancesPagedResponse
extends AbstractPagedListResponse<
ListInstancesRequest,
ListInstancesResponse,
Instance,
ListInstancesPage,
ListInstancesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListInstancesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListInstancesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListInstancesPagedResponse(ListInstancesPage page) {
super(page, ListInstancesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListInstancesPage
extends AbstractPage<
ListInstancesRequest, ListInstancesResponse, Instance, ListInstancesPage> {
private ListInstancesPage(
PageContext context,
ListInstancesResponse response) {
super(context, response);
}
private static ListInstancesPage createEmptyPage() {
return new ListInstancesPage(null, null);
}
@Override
protected ListInstancesPage createPage(
PageContext context,
ListInstancesResponse response) {
return new ListInstancesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListInstancesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListInstancesRequest,
ListInstancesResponse,
Instance,
ListInstancesPage,
ListInstancesFixedSizeCollection> {
private ListInstancesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListInstancesFixedSizeCollection createEmptyCollection() {
return new ListInstancesFixedSizeCollection(null, 0);
}
@Override
protected ListInstancesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListInstancesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListVolumesPagedResponse
extends AbstractPagedListResponse<
ListVolumesRequest,
ListVolumesResponse,
Volume,
ListVolumesPage,
ListVolumesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListVolumesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListVolumesPagedResponse(input), MoreExecutors.directExecutor());
}
private ListVolumesPagedResponse(ListVolumesPage page) {
super(page, ListVolumesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListVolumesPage
extends AbstractPage {
private ListVolumesPage(
PageContext context,
ListVolumesResponse response) {
super(context, response);
}
private static ListVolumesPage createEmptyPage() {
return new ListVolumesPage(null, null);
}
@Override
protected ListVolumesPage createPage(
PageContext context,
ListVolumesResponse response) {
return new ListVolumesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListVolumesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListVolumesRequest,
ListVolumesResponse,
Volume,
ListVolumesPage,
ListVolumesFixedSizeCollection> {
private ListVolumesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListVolumesFixedSizeCollection createEmptyCollection() {
return new ListVolumesFixedSizeCollection(null, 0);
}
@Override
protected ListVolumesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListVolumesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListNetworksPagedResponse
extends AbstractPagedListResponse<
ListNetworksRequest,
ListNetworksResponse,
Network,
ListNetworksPage,
ListNetworksFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListNetworksPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListNetworksPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListNetworksPagedResponse(ListNetworksPage page) {
super(page, ListNetworksFixedSizeCollection.createEmptyCollection());
}
}
public static class ListNetworksPage
extends AbstractPage {
private ListNetworksPage(
PageContext context,
ListNetworksResponse response) {
super(context, response);
}
private static ListNetworksPage createEmptyPage() {
return new ListNetworksPage(null, null);
}
@Override
protected ListNetworksPage createPage(
PageContext context,
ListNetworksResponse response) {
return new ListNetworksPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListNetworksFixedSizeCollection
extends AbstractFixedSizeCollection<
ListNetworksRequest,
ListNetworksResponse,
Network,
ListNetworksPage,
ListNetworksFixedSizeCollection> {
private ListNetworksFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListNetworksFixedSizeCollection createEmptyCollection() {
return new ListNetworksFixedSizeCollection(null, 0);
}
@Override
protected ListNetworksFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListNetworksFixedSizeCollection(pages, collectionSize);
}
}
public static class ListSnapshotSchedulePoliciesPagedResponse
extends AbstractPagedListResponse<
ListSnapshotSchedulePoliciesRequest,
ListSnapshotSchedulePoliciesResponse,
SnapshotSchedulePolicy,
ListSnapshotSchedulePoliciesPage,
ListSnapshotSchedulePoliciesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListSnapshotSchedulePoliciesRequest,
ListSnapshotSchedulePoliciesResponse,
SnapshotSchedulePolicy>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListSnapshotSchedulePoliciesPage.createEmptyPage()
.createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListSnapshotSchedulePoliciesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListSnapshotSchedulePoliciesPagedResponse(ListSnapshotSchedulePoliciesPage page) {
super(page, ListSnapshotSchedulePoliciesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListSnapshotSchedulePoliciesPage
extends AbstractPage<
ListSnapshotSchedulePoliciesRequest,
ListSnapshotSchedulePoliciesResponse,
SnapshotSchedulePolicy,
ListSnapshotSchedulePoliciesPage> {
private ListSnapshotSchedulePoliciesPage(
PageContext<
ListSnapshotSchedulePoliciesRequest,
ListSnapshotSchedulePoliciesResponse,
SnapshotSchedulePolicy>
context,
ListSnapshotSchedulePoliciesResponse response) {
super(context, response);
}
private static ListSnapshotSchedulePoliciesPage createEmptyPage() {
return new ListSnapshotSchedulePoliciesPage(null, null);
}
@Override
protected ListSnapshotSchedulePoliciesPage createPage(
PageContext<
ListSnapshotSchedulePoliciesRequest,
ListSnapshotSchedulePoliciesResponse,
SnapshotSchedulePolicy>
context,
ListSnapshotSchedulePoliciesResponse response) {
return new ListSnapshotSchedulePoliciesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListSnapshotSchedulePoliciesRequest,
ListSnapshotSchedulePoliciesResponse,
SnapshotSchedulePolicy>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListSnapshotSchedulePoliciesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListSnapshotSchedulePoliciesRequest,
ListSnapshotSchedulePoliciesResponse,
SnapshotSchedulePolicy,
ListSnapshotSchedulePoliciesPage,
ListSnapshotSchedulePoliciesFixedSizeCollection> {
private ListSnapshotSchedulePoliciesFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListSnapshotSchedulePoliciesFixedSizeCollection createEmptyCollection() {
return new ListSnapshotSchedulePoliciesFixedSizeCollection(null, 0);
}
@Override
protected ListSnapshotSchedulePoliciesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListSnapshotSchedulePoliciesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListVolumeSnapshotsPagedResponse
extends AbstractPagedListResponse<
ListVolumeSnapshotsRequest,
ListVolumeSnapshotsResponse,
VolumeSnapshot,
ListVolumeSnapshotsPage,
ListVolumeSnapshotsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListVolumeSnapshotsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListVolumeSnapshotsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListVolumeSnapshotsPagedResponse(ListVolumeSnapshotsPage page) {
super(page, ListVolumeSnapshotsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListVolumeSnapshotsPage
extends AbstractPage<
ListVolumeSnapshotsRequest,
ListVolumeSnapshotsResponse,
VolumeSnapshot,
ListVolumeSnapshotsPage> {
private ListVolumeSnapshotsPage(
PageContext
context,
ListVolumeSnapshotsResponse response) {
super(context, response);
}
private static ListVolumeSnapshotsPage createEmptyPage() {
return new ListVolumeSnapshotsPage(null, null);
}
@Override
protected ListVolumeSnapshotsPage createPage(
PageContext
context,
ListVolumeSnapshotsResponse response) {
return new ListVolumeSnapshotsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListVolumeSnapshotsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListVolumeSnapshotsRequest,
ListVolumeSnapshotsResponse,
VolumeSnapshot,
ListVolumeSnapshotsPage,
ListVolumeSnapshotsFixedSizeCollection> {
private ListVolumeSnapshotsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListVolumeSnapshotsFixedSizeCollection createEmptyCollection() {
return new ListVolumeSnapshotsFixedSizeCollection(null, 0);
}
@Override
protected ListVolumeSnapshotsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListVolumeSnapshotsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLunsPagedResponse
extends AbstractPagedListResponse<
ListLunsRequest, ListLunsResponse, Lun, ListLunsPage, ListLunsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLunsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListLunsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListLunsPagedResponse(ListLunsPage page) {
super(page, ListLunsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLunsPage
extends AbstractPage {
private ListLunsPage(
PageContext context, ListLunsResponse response) {
super(context, response);
}
private static ListLunsPage createEmptyPage() {
return new ListLunsPage(null, null);
}
@Override
protected ListLunsPage createPage(
PageContext context, ListLunsResponse response) {
return new ListLunsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLunsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLunsRequest, ListLunsResponse, Lun, ListLunsPage, ListLunsFixedSizeCollection> {
private ListLunsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLunsFixedSizeCollection createEmptyCollection() {
return new ListLunsFixedSizeCollection(null, 0);
}
@Override
protected ListLunsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLunsFixedSizeCollection(pages, collectionSize);
}
}
}