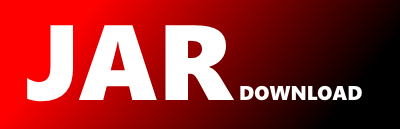
com.google.cloud.baremetalsolution.v2.stub.GrpcBareMetalSolutionStub Maven / Gradle / Ivy
Show all versions of google-cloud-bare-metal-solution Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.baremetalsolution.v2.stub;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListInstancesPagedResponse;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListLocationsPagedResponse;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListLunsPagedResponse;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListNetworksPagedResponse;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListNfsSharesPagedResponse;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListOSImagesPagedResponse;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListProvisioningQuotasPagedResponse;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListSSHKeysPagedResponse;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListVolumeSnapshotsPagedResponse;
import static com.google.cloud.baremetalsolution.v2.BareMetalSolutionClient.ListVolumesPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.baremetalsolution.v2.CreateNfsShareRequest;
import com.google.cloud.baremetalsolution.v2.CreateProvisioningConfigRequest;
import com.google.cloud.baremetalsolution.v2.CreateSSHKeyRequest;
import com.google.cloud.baremetalsolution.v2.CreateVolumeSnapshotRequest;
import com.google.cloud.baremetalsolution.v2.DeleteNfsShareRequest;
import com.google.cloud.baremetalsolution.v2.DeleteSSHKeyRequest;
import com.google.cloud.baremetalsolution.v2.DeleteVolumeSnapshotRequest;
import com.google.cloud.baremetalsolution.v2.DetachLunRequest;
import com.google.cloud.baremetalsolution.v2.DisableInteractiveSerialConsoleRequest;
import com.google.cloud.baremetalsolution.v2.DisableInteractiveSerialConsoleResponse;
import com.google.cloud.baremetalsolution.v2.EnableInteractiveSerialConsoleRequest;
import com.google.cloud.baremetalsolution.v2.EnableInteractiveSerialConsoleResponse;
import com.google.cloud.baremetalsolution.v2.EvictLunRequest;
import com.google.cloud.baremetalsolution.v2.EvictVolumeRequest;
import com.google.cloud.baremetalsolution.v2.GetInstanceRequest;
import com.google.cloud.baremetalsolution.v2.GetLunRequest;
import com.google.cloud.baremetalsolution.v2.GetNetworkRequest;
import com.google.cloud.baremetalsolution.v2.GetNfsShareRequest;
import com.google.cloud.baremetalsolution.v2.GetProvisioningConfigRequest;
import com.google.cloud.baremetalsolution.v2.GetVolumeRequest;
import com.google.cloud.baremetalsolution.v2.GetVolumeSnapshotRequest;
import com.google.cloud.baremetalsolution.v2.Instance;
import com.google.cloud.baremetalsolution.v2.ListInstancesRequest;
import com.google.cloud.baremetalsolution.v2.ListInstancesResponse;
import com.google.cloud.baremetalsolution.v2.ListLunsRequest;
import com.google.cloud.baremetalsolution.v2.ListLunsResponse;
import com.google.cloud.baremetalsolution.v2.ListNetworkUsageRequest;
import com.google.cloud.baremetalsolution.v2.ListNetworkUsageResponse;
import com.google.cloud.baremetalsolution.v2.ListNetworksRequest;
import com.google.cloud.baremetalsolution.v2.ListNetworksResponse;
import com.google.cloud.baremetalsolution.v2.ListNfsSharesRequest;
import com.google.cloud.baremetalsolution.v2.ListNfsSharesResponse;
import com.google.cloud.baremetalsolution.v2.ListOSImagesRequest;
import com.google.cloud.baremetalsolution.v2.ListOSImagesResponse;
import com.google.cloud.baremetalsolution.v2.ListProvisioningQuotasRequest;
import com.google.cloud.baremetalsolution.v2.ListProvisioningQuotasResponse;
import com.google.cloud.baremetalsolution.v2.ListSSHKeysRequest;
import com.google.cloud.baremetalsolution.v2.ListSSHKeysResponse;
import com.google.cloud.baremetalsolution.v2.ListVolumeSnapshotsRequest;
import com.google.cloud.baremetalsolution.v2.ListVolumeSnapshotsResponse;
import com.google.cloud.baremetalsolution.v2.ListVolumesRequest;
import com.google.cloud.baremetalsolution.v2.ListVolumesResponse;
import com.google.cloud.baremetalsolution.v2.Lun;
import com.google.cloud.baremetalsolution.v2.Network;
import com.google.cloud.baremetalsolution.v2.NfsShare;
import com.google.cloud.baremetalsolution.v2.OperationMetadata;
import com.google.cloud.baremetalsolution.v2.ProvisioningConfig;
import com.google.cloud.baremetalsolution.v2.RenameInstanceRequest;
import com.google.cloud.baremetalsolution.v2.RenameNetworkRequest;
import com.google.cloud.baremetalsolution.v2.RenameNfsShareRequest;
import com.google.cloud.baremetalsolution.v2.RenameVolumeRequest;
import com.google.cloud.baremetalsolution.v2.ResetInstanceRequest;
import com.google.cloud.baremetalsolution.v2.ResetInstanceResponse;
import com.google.cloud.baremetalsolution.v2.ResizeVolumeRequest;
import com.google.cloud.baremetalsolution.v2.RestoreVolumeSnapshotRequest;
import com.google.cloud.baremetalsolution.v2.SSHKey;
import com.google.cloud.baremetalsolution.v2.StartInstanceRequest;
import com.google.cloud.baremetalsolution.v2.StartInstanceResponse;
import com.google.cloud.baremetalsolution.v2.StopInstanceRequest;
import com.google.cloud.baremetalsolution.v2.StopInstanceResponse;
import com.google.cloud.baremetalsolution.v2.SubmitProvisioningConfigRequest;
import com.google.cloud.baremetalsolution.v2.SubmitProvisioningConfigResponse;
import com.google.cloud.baremetalsolution.v2.UpdateInstanceRequest;
import com.google.cloud.baremetalsolution.v2.UpdateNetworkRequest;
import com.google.cloud.baremetalsolution.v2.UpdateNfsShareRequest;
import com.google.cloud.baremetalsolution.v2.UpdateProvisioningConfigRequest;
import com.google.cloud.baremetalsolution.v2.UpdateVolumeRequest;
import com.google.cloud.baremetalsolution.v2.Volume;
import com.google.cloud.baremetalsolution.v2.VolumeSnapshot;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import com.google.protobuf.Empty;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the BareMetalSolution service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public class GrpcBareMetalSolutionStub extends BareMetalSolutionStub {
private static final MethodDescriptor
listInstancesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/ListInstances")
.setRequestMarshaller(
ProtoUtils.marshaller(ListInstancesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListInstancesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/GetInstance")
.setRequestMarshaller(ProtoUtils.marshaller(GetInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Instance.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/UpdateInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
renameInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/RenameInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(RenameInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Instance.getDefaultInstance()))
.build();
private static final MethodDescriptor
resetInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/ResetInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(ResetInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
startInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/StartInstance")
.setRequestMarshaller(
ProtoUtils.marshaller(StartInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
stopInstanceMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/StopInstance")
.setRequestMarshaller(ProtoUtils.marshaller(StopInstanceRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
enableInteractiveSerialConsoleMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/EnableInteractiveSerialConsole")
.setRequestMarshaller(
ProtoUtils.marshaller(EnableInteractiveSerialConsoleRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
disableInteractiveSerialConsoleMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/DisableInteractiveSerialConsole")
.setRequestMarshaller(
ProtoUtils.marshaller(
DisableInteractiveSerialConsoleRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor detachLunMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/DetachLun")
.setRequestMarshaller(ProtoUtils.marshaller(DetachLunRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listSSHKeysMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/ListSSHKeys")
.setRequestMarshaller(ProtoUtils.marshaller(ListSSHKeysRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListSSHKeysResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor createSSHKeyMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/CreateSSHKey")
.setRequestMarshaller(ProtoUtils.marshaller(CreateSSHKeyRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(SSHKey.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteSSHKeyMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/DeleteSSHKey")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteSSHKeyRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
listVolumesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/ListVolumes")
.setRequestMarshaller(ProtoUtils.marshaller(ListVolumesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListVolumesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getVolumeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/GetVolume")
.setRequestMarshaller(ProtoUtils.marshaller(GetVolumeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Volume.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateVolumeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/UpdateVolume")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateVolumeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor renameVolumeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/RenameVolume")
.setRequestMarshaller(ProtoUtils.marshaller(RenameVolumeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Volume.getDefaultInstance()))
.build();
private static final MethodDescriptor evictVolumeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/EvictVolume")
.setRequestMarshaller(ProtoUtils.marshaller(EvictVolumeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
resizeVolumeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/ResizeVolume")
.setRequestMarshaller(ProtoUtils.marshaller(ResizeVolumeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listNetworksMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/ListNetworks")
.setRequestMarshaller(ProtoUtils.marshaller(ListNetworksRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListNetworksResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
listNetworkUsageMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/ListNetworkUsage")
.setRequestMarshaller(
ProtoUtils.marshaller(ListNetworkUsageRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListNetworkUsageResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getNetworkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/GetNetwork")
.setRequestMarshaller(ProtoUtils.marshaller(GetNetworkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Network.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateNetworkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/UpdateNetwork")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateNetworkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
createVolumeSnapshotMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/CreateVolumeSnapshot")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateVolumeSnapshotRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(VolumeSnapshot.getDefaultInstance()))
.build();
private static final MethodDescriptor
restoreVolumeSnapshotMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/RestoreVolumeSnapshot")
.setRequestMarshaller(
ProtoUtils.marshaller(RestoreVolumeSnapshotRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteVolumeSnapshotMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/DeleteVolumeSnapshot")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteVolumeSnapshotRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
getVolumeSnapshotMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/GetVolumeSnapshot")
.setRequestMarshaller(
ProtoUtils.marshaller(GetVolumeSnapshotRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(VolumeSnapshot.getDefaultInstance()))
.build();
private static final MethodDescriptor
listVolumeSnapshotsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/ListVolumeSnapshots")
.setRequestMarshaller(
ProtoUtils.marshaller(ListVolumeSnapshotsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListVolumeSnapshotsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getLunMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/GetLun")
.setRequestMarshaller(ProtoUtils.marshaller(GetLunRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Lun.getDefaultInstance()))
.build();
private static final MethodDescriptor
listLunsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/ListLuns")
.setRequestMarshaller(ProtoUtils.marshaller(ListLunsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListLunsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor evictLunMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/EvictLun")
.setRequestMarshaller(ProtoUtils.marshaller(EvictLunRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor getNfsShareMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/GetNfsShare")
.setRequestMarshaller(ProtoUtils.marshaller(GetNfsShareRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(NfsShare.getDefaultInstance()))
.build();
private static final MethodDescriptor
listNfsSharesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/ListNfsShares")
.setRequestMarshaller(
ProtoUtils.marshaller(ListNfsSharesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListNfsSharesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateNfsShareMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/UpdateNfsShare")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateNfsShareRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
createNfsShareMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/CreateNfsShare")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateNfsShareRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
renameNfsShareMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/RenameNfsShare")
.setRequestMarshaller(
ProtoUtils.marshaller(RenameNfsShareRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(NfsShare.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteNfsShareMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/DeleteNfsShare")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteNfsShareRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor<
ListProvisioningQuotasRequest, ListProvisioningQuotasResponse>
listProvisioningQuotasMethodDescriptor =
MethodDescriptor
.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/ListProvisioningQuotas")
.setRequestMarshaller(
ProtoUtils.marshaller(ListProvisioningQuotasRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListProvisioningQuotasResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor<
SubmitProvisioningConfigRequest, SubmitProvisioningConfigResponse>
submitProvisioningConfigMethodDescriptor =
MethodDescriptor
.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/SubmitProvisioningConfig")
.setRequestMarshaller(
ProtoUtils.marshaller(SubmitProvisioningConfigRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(SubmitProvisioningConfigResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getProvisioningConfigMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/GetProvisioningConfig")
.setRequestMarshaller(
ProtoUtils.marshaller(GetProvisioningConfigRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ProvisioningConfig.getDefaultInstance()))
.build();
private static final MethodDescriptor
createProvisioningConfigMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/CreateProvisioningConfig")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateProvisioningConfigRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ProvisioningConfig.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateProvisioningConfigMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/UpdateProvisioningConfig")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateProvisioningConfigRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ProvisioningConfig.getDefaultInstance()))
.build();
private static final MethodDescriptor
renameNetworkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.baremetalsolution.v2.BareMetalSolution/RenameNetwork")
.setRequestMarshaller(
ProtoUtils.marshaller(RenameNetworkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Network.getDefaultInstance()))
.build();
private static final MethodDescriptor
listOSImagesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.baremetalsolution.v2.BareMetalSolution/ListOSImages")
.setRequestMarshaller(ProtoUtils.marshaller(ListOSImagesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListOSImagesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
listLocationsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/ListLocations")
.setRequestMarshaller(
ProtoUtils.marshaller(ListLocationsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListLocationsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getLocationMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/GetLocation")
.setRequestMarshaller(ProtoUtils.marshaller(GetLocationRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Location.getDefaultInstance()))
.build();
private final UnaryCallable listInstancesCallable;
private final UnaryCallable
listInstancesPagedCallable;
private final UnaryCallable getInstanceCallable;
private final UnaryCallable updateInstanceCallable;
private final OperationCallable
updateInstanceOperationCallable;
private final UnaryCallable renameInstanceCallable;
private final UnaryCallable resetInstanceCallable;
private final OperationCallable
resetInstanceOperationCallable;
private final UnaryCallable startInstanceCallable;
private final OperationCallable
startInstanceOperationCallable;
private final UnaryCallable stopInstanceCallable;
private final OperationCallable
stopInstanceOperationCallable;
private final UnaryCallable
enableInteractiveSerialConsoleCallable;
private final OperationCallable<
EnableInteractiveSerialConsoleRequest,
EnableInteractiveSerialConsoleResponse,
OperationMetadata>
enableInteractiveSerialConsoleOperationCallable;
private final UnaryCallable
disableInteractiveSerialConsoleCallable;
private final OperationCallable<
DisableInteractiveSerialConsoleRequest,
DisableInteractiveSerialConsoleResponse,
OperationMetadata>
disableInteractiveSerialConsoleOperationCallable;
private final UnaryCallable detachLunCallable;
private final OperationCallable
detachLunOperationCallable;
private final UnaryCallable listSSHKeysCallable;
private final UnaryCallable
listSSHKeysPagedCallable;
private final UnaryCallable createSSHKeyCallable;
private final UnaryCallable deleteSSHKeyCallable;
private final UnaryCallable listVolumesCallable;
private final UnaryCallable
listVolumesPagedCallable;
private final UnaryCallable getVolumeCallable;
private final UnaryCallable updateVolumeCallable;
private final OperationCallable
updateVolumeOperationCallable;
private final UnaryCallable renameVolumeCallable;
private final UnaryCallable evictVolumeCallable;
private final OperationCallable
evictVolumeOperationCallable;
private final UnaryCallable resizeVolumeCallable;
private final OperationCallable
resizeVolumeOperationCallable;
private final UnaryCallable listNetworksCallable;
private final UnaryCallable
listNetworksPagedCallable;
private final UnaryCallable
listNetworkUsageCallable;
private final UnaryCallable getNetworkCallable;
private final UnaryCallable updateNetworkCallable;
private final OperationCallable
updateNetworkOperationCallable;
private final UnaryCallable
createVolumeSnapshotCallable;
private final UnaryCallable
restoreVolumeSnapshotCallable;
private final OperationCallable
restoreVolumeSnapshotOperationCallable;
private final UnaryCallable deleteVolumeSnapshotCallable;
private final UnaryCallable getVolumeSnapshotCallable;
private final UnaryCallable
listVolumeSnapshotsCallable;
private final UnaryCallable
listVolumeSnapshotsPagedCallable;
private final UnaryCallable getLunCallable;
private final UnaryCallable listLunsCallable;
private final UnaryCallable listLunsPagedCallable;
private final UnaryCallable evictLunCallable;
private final OperationCallable
evictLunOperationCallable;
private final UnaryCallable getNfsShareCallable;
private final UnaryCallable listNfsSharesCallable;
private final UnaryCallable
listNfsSharesPagedCallable;
private final UnaryCallable updateNfsShareCallable;
private final OperationCallable
updateNfsShareOperationCallable;
private final UnaryCallable createNfsShareCallable;
private final OperationCallable
createNfsShareOperationCallable;
private final UnaryCallable renameNfsShareCallable;
private final UnaryCallable deleteNfsShareCallable;
private final OperationCallable
deleteNfsShareOperationCallable;
private final UnaryCallable
listProvisioningQuotasCallable;
private final UnaryCallable
listProvisioningQuotasPagedCallable;
private final UnaryCallable
submitProvisioningConfigCallable;
private final UnaryCallable
getProvisioningConfigCallable;
private final UnaryCallable
createProvisioningConfigCallable;
private final UnaryCallable
updateProvisioningConfigCallable;
private final UnaryCallable renameNetworkCallable;
private final UnaryCallable listOSImagesCallable;
private final UnaryCallable
listOSImagesPagedCallable;
private final UnaryCallable listLocationsCallable;
private final UnaryCallable
listLocationsPagedCallable;
private final UnaryCallable getLocationCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcBareMetalSolutionStub create(BareMetalSolutionStubSettings settings)
throws IOException {
return new GrpcBareMetalSolutionStub(settings, ClientContext.create(settings));
}
public static final GrpcBareMetalSolutionStub create(ClientContext clientContext)
throws IOException {
return new GrpcBareMetalSolutionStub(
BareMetalSolutionStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcBareMetalSolutionStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcBareMetalSolutionStub(
BareMetalSolutionStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcBareMetalSolutionStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcBareMetalSolutionStub(
BareMetalSolutionStubSettings settings, ClientContext clientContext) throws IOException {
this(settings, clientContext, new GrpcBareMetalSolutionCallableFactory());
}
/**
* Constructs an instance of GrpcBareMetalSolutionStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcBareMetalSolutionStub(
BareMetalSolutionStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings listInstancesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listInstancesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("instance.name", String.valueOf(request.getInstance().getName()));
return builder.build();
})
.build();
GrpcCallSettings renameInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(renameInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings resetInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(resetInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings startInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(startInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings stopInstanceTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(stopInstanceMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
enableInteractiveSerialConsoleTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(enableInteractiveSerialConsoleMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
disableInteractiveSerialConsoleTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(disableInteractiveSerialConsoleMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings detachLunTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(detachLunMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("instance", String.valueOf(request.getInstance()));
return builder.build();
})
.build();
GrpcCallSettings listSSHKeysTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listSSHKeysMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings createSSHKeyTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createSSHKeyMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings deleteSSHKeyTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteSSHKeyMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listVolumesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listVolumesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getVolumeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getVolumeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateVolumeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateVolumeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("volume.name", String.valueOf(request.getVolume().getName()));
return builder.build();
})
.build();
GrpcCallSettings renameVolumeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(renameVolumeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings evictVolumeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(evictVolumeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings resizeVolumeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(resizeVolumeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("volume", String.valueOf(request.getVolume()));
return builder.build();
})
.build();
GrpcCallSettings listNetworksTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listNetworksMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
listNetworkUsageTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listNetworkUsageMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("location", String.valueOf(request.getLocation()));
return builder.build();
})
.build();
GrpcCallSettings getNetworkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getNetworkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateNetworkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateNetworkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("network.name", String.valueOf(request.getNetwork().getName()));
return builder.build();
})
.build();
GrpcCallSettings
createVolumeSnapshotTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createVolumeSnapshotMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
restoreVolumeSnapshotTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(restoreVolumeSnapshotMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("volume_snapshot", String.valueOf(request.getVolumeSnapshot()));
return builder.build();
})
.build();
GrpcCallSettings deleteVolumeSnapshotTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteVolumeSnapshotMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getVolumeSnapshotTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getVolumeSnapshotMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listVolumeSnapshotsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listVolumeSnapshotsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getLunTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getLunMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listLunsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listLunsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings evictLunTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(evictLunMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getNfsShareTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getNfsShareMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listNfsSharesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listNfsSharesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateNfsShareTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateNfsShareMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("nfs_share.name", String.valueOf(request.getNfsShare().getName()));
return builder.build();
})
.build();
GrpcCallSettings createNfsShareTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createNfsShareMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings renameNfsShareTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(renameNfsShareMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteNfsShareTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteNfsShareMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listProvisioningQuotasTransportSettings =
GrpcCallSettings
.newBuilder()
.setMethodDescriptor(listProvisioningQuotasMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
submitProvisioningConfigTransportSettings =
GrpcCallSettings
.newBuilder()
.setMethodDescriptor(submitProvisioningConfigMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
getProvisioningConfigTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getProvisioningConfigMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
createProvisioningConfigTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createProvisioningConfigMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
updateProvisioningConfigTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateProvisioningConfigMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add(
"provisioning_config.name",
String.valueOf(request.getProvisioningConfig().getName()));
return builder.build();
})
.build();
GrpcCallSettings renameNetworkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(renameNetworkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listOSImagesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listOSImagesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings listLocationsTransportSettings =
GrpcCallSettings.