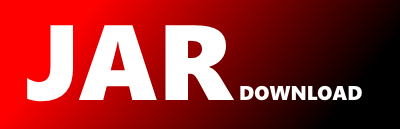
com.google.cloud.commerce.consumer.procurement.v1.ConsumerProcurementServiceClient Maven / Gradle / Ivy
Show all versions of google-cloud-cloudcommerceconsumerprocurement Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.commerce.consumer.procurement.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.commerce.consumer.procurement.v1.stub.ConsumerProcurementServiceStub;
import com.google.cloud.commerce.consumer.procurement.v1.stub.ConsumerProcurementServiceStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.longrunning.Operation;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: ConsumerProcurementService allows customers to make purchases of products
* served by the Cloud Commerce platform.
*
* When purchases are made, the
* [ConsumerProcurementService][google.cloud.commerce.consumer.procurement.v1.ConsumerProcurementService]
* programs the appropriate backends, including both Google's own infrastructure, as well as
* third-party systems, and to enable billing setup for charging for the procured item.
*
*
This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* String name = "name3373707";
* Order response = consumerProcurementServiceClient.getOrder(name);
* }
* }
*
* Note: close() needs to be called on the ConsumerProcurementServiceClient object to clean up
* resources such as threads. In the example above, try-with-resources is used, which automatically
* calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* PlaceOrder
* Creates a new [Order][google.cloud.commerce.consumer.procurement.v1.Order].
*
This API only supports GCP spend-based committed use discounts specified by GCP documentation.
*
The returned long-running operation is in-progress until the backend completes the creation of the resource. Once completed, the order is in [OrderState.ORDER_STATE_ACTIVE][google.cloud.commerce.consumer.procurement.v1.OrderState.ORDER_STATE_ACTIVE]. In case of failure, the order resource will be removed.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* placeOrderAsync(PlaceOrderRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* placeOrderOperationCallable()
*
placeOrderCallable()
*
*
*
*
* GetOrder
* Returns the requested [Order][google.cloud.commerce.consumer.procurement.v1.Order] resource.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getOrder(GetOrderRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getOrder(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getOrderCallable()
*
*
*
*
* ListOrders
* Lists [Order][google.cloud.commerce.consumer.procurement.v1.Order] resources that the user has access to, within the scope of the parent resource.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listOrders(ListOrdersRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listOrders(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listOrdersPagedCallable()
*
listOrdersCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of
* ConsumerProcurementServiceSettings to create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ConsumerProcurementServiceSettings consumerProcurementServiceSettings =
* ConsumerProcurementServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create(consumerProcurementServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ConsumerProcurementServiceSettings consumerProcurementServiceSettings =
* ConsumerProcurementServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create(consumerProcurementServiceSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ConsumerProcurementServiceSettings consumerProcurementServiceSettings =
* ConsumerProcurementServiceSettings.newHttpJsonBuilder().build();
* ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create(consumerProcurementServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class ConsumerProcurementServiceClient implements BackgroundResource {
private final ConsumerProcurementServiceSettings settings;
private final ConsumerProcurementServiceStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of ConsumerProcurementServiceClient with default settings. */
public static final ConsumerProcurementServiceClient create() throws IOException {
return create(ConsumerProcurementServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of ConsumerProcurementServiceClient, using the given settings. The
* channels are created based on the settings passed in, or defaults for any settings that are not
* set.
*/
public static final ConsumerProcurementServiceClient create(
ConsumerProcurementServiceSettings settings) throws IOException {
return new ConsumerProcurementServiceClient(settings);
}
/**
* Constructs an instance of ConsumerProcurementServiceClient, using the given stub for making
* calls. This is for advanced usage - prefer using create(ConsumerProcurementServiceSettings).
*/
public static final ConsumerProcurementServiceClient create(ConsumerProcurementServiceStub stub) {
return new ConsumerProcurementServiceClient(stub);
}
/**
* Constructs an instance of ConsumerProcurementServiceClient, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected ConsumerProcurementServiceClient(ConsumerProcurementServiceSettings settings)
throws IOException {
this.settings = settings;
this.stub = ((ConsumerProcurementServiceStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected ConsumerProcurementServiceClient(ConsumerProcurementServiceStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final ConsumerProcurementServiceSettings getSettings() {
return settings;
}
public ConsumerProcurementServiceStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new [Order][google.cloud.commerce.consumer.procurement.v1.Order].
*
*
This API only supports GCP spend-based committed use discounts specified by GCP
* documentation.
*
*
The returned long-running operation is in-progress until the backend completes the creation
* of the resource. Once completed, the order is in
* [OrderState.ORDER_STATE_ACTIVE][google.cloud.commerce.consumer.procurement.v1.OrderState.ORDER_STATE_ACTIVE].
* In case of failure, the order resource will be removed.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* PlaceOrderRequest request =
* PlaceOrderRequest.newBuilder()
* .setParent(BillingAccountName.of("[BILLING_ACCOUNT]").toString())
* .setDisplayName("displayName1714148973")
* .addAllLineItemInfo(new ArrayList())
* .setRequestId("requestId693933066")
* .build();
* Order response = consumerProcurementServiceClient.placeOrderAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture placeOrderAsync(
PlaceOrderRequest request) {
return placeOrderOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new [Order][google.cloud.commerce.consumer.procurement.v1.Order].
*
* This API only supports GCP spend-based committed use discounts specified by GCP
* documentation.
*
*
The returned long-running operation is in-progress until the backend completes the creation
* of the resource. Once completed, the order is in
* [OrderState.ORDER_STATE_ACTIVE][google.cloud.commerce.consumer.procurement.v1.OrderState.ORDER_STATE_ACTIVE].
* In case of failure, the order resource will be removed.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* PlaceOrderRequest request =
* PlaceOrderRequest.newBuilder()
* .setParent(BillingAccountName.of("[BILLING_ACCOUNT]").toString())
* .setDisplayName("displayName1714148973")
* .addAllLineItemInfo(new ArrayList())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* consumerProcurementServiceClient.placeOrderOperationCallable().futureCall(request);
* // Do something.
* Order response = future.get();
* }
* }
*/
public final OperationCallable
placeOrderOperationCallable() {
return stub.placeOrderOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new [Order][google.cloud.commerce.consumer.procurement.v1.Order].
*
* This API only supports GCP spend-based committed use discounts specified by GCP
* documentation.
*
*
The returned long-running operation is in-progress until the backend completes the creation
* of the resource. Once completed, the order is in
* [OrderState.ORDER_STATE_ACTIVE][google.cloud.commerce.consumer.procurement.v1.OrderState.ORDER_STATE_ACTIVE].
* In case of failure, the order resource will be removed.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* PlaceOrderRequest request =
* PlaceOrderRequest.newBuilder()
* .setParent(BillingAccountName.of("[BILLING_ACCOUNT]").toString())
* .setDisplayName("displayName1714148973")
* .addAllLineItemInfo(new ArrayList())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* consumerProcurementServiceClient.placeOrderCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable placeOrderCallable() {
return stub.placeOrderCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested [Order][google.cloud.commerce.consumer.procurement.v1.Order] resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* String name = "name3373707";
* Order response = consumerProcurementServiceClient.getOrder(name);
* }
* }
*
* @param name Required. The name of the order to retrieve.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Order getOrder(String name) {
GetOrderRequest request = GetOrderRequest.newBuilder().setName(name).build();
return getOrder(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested [Order][google.cloud.commerce.consumer.procurement.v1.Order] resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* GetOrderRequest request = GetOrderRequest.newBuilder().setName("name3373707").build();
* Order response = consumerProcurementServiceClient.getOrder(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Order getOrder(GetOrderRequest request) {
return getOrderCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested [Order][google.cloud.commerce.consumer.procurement.v1.Order] resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* GetOrderRequest request = GetOrderRequest.newBuilder().setName("name3373707").build();
* ApiFuture future =
* consumerProcurementServiceClient.getOrderCallable().futureCall(request);
* // Do something.
* Order response = future.get();
* }
* }
*/
public final UnaryCallable getOrderCallable() {
return stub.getOrderCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists [Order][google.cloud.commerce.consumer.procurement.v1.Order] resources that the user has
* access to, within the scope of the parent resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* String parent = "parent-995424086";
* for (Order element : consumerProcurementServiceClient.listOrders(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource to query for orders. This field has the form
* `billingAccounts/{billing-account-id}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListOrdersPagedResponse listOrders(String parent) {
ListOrdersRequest request = ListOrdersRequest.newBuilder().setParent(parent).build();
return listOrders(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists [Order][google.cloud.commerce.consumer.procurement.v1.Order] resources that the user has
* access to, within the scope of the parent resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* ListOrdersRequest request =
* ListOrdersRequest.newBuilder()
* .setParent("parent-995424086")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* for (Order element : consumerProcurementServiceClient.listOrders(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListOrdersPagedResponse listOrders(ListOrdersRequest request) {
return listOrdersPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists [Order][google.cloud.commerce.consumer.procurement.v1.Order] resources that the user has
* access to, within the scope of the parent resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* ListOrdersRequest request =
* ListOrdersRequest.newBuilder()
* .setParent("parent-995424086")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* ApiFuture future =
* consumerProcurementServiceClient.listOrdersPagedCallable().futureCall(request);
* // Do something.
* for (Order element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listOrdersPagedCallable() {
return stub.listOrdersPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists [Order][google.cloud.commerce.consumer.procurement.v1.Order] resources that the user has
* access to, within the scope of the parent resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConsumerProcurementServiceClient consumerProcurementServiceClient =
* ConsumerProcurementServiceClient.create()) {
* ListOrdersRequest request =
* ListOrdersRequest.newBuilder()
* .setParent("parent-995424086")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* while (true) {
* ListOrdersResponse response =
* consumerProcurementServiceClient.listOrdersCallable().call(request);
* for (Order element : response.getOrdersList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listOrdersCallable() {
return stub.listOrdersCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListOrdersPagedResponse
extends AbstractPagedListResponse<
ListOrdersRequest,
ListOrdersResponse,
Order,
ListOrdersPage,
ListOrdersFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListOrdersPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListOrdersPagedResponse(input), MoreExecutors.directExecutor());
}
private ListOrdersPagedResponse(ListOrdersPage page) {
super(page, ListOrdersFixedSizeCollection.createEmptyCollection());
}
}
public static class ListOrdersPage
extends AbstractPage {
private ListOrdersPage(
PageContext context,
ListOrdersResponse response) {
super(context, response);
}
private static ListOrdersPage createEmptyPage() {
return new ListOrdersPage(null, null);
}
@Override
protected ListOrdersPage createPage(
PageContext context,
ListOrdersResponse response) {
return new ListOrdersPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListOrdersFixedSizeCollection
extends AbstractFixedSizeCollection<
ListOrdersRequest,
ListOrdersResponse,
Order,
ListOrdersPage,
ListOrdersFixedSizeCollection> {
private ListOrdersFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListOrdersFixedSizeCollection createEmptyCollection() {
return new ListOrdersFixedSizeCollection(null, 0);
}
@Override
protected ListOrdersFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListOrdersFixedSizeCollection(pages, collectionSize);
}
}
}