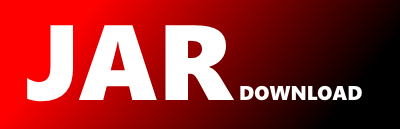
com.google.cloud.datacatalog.v1.spring.DataCatalogSpringProperties Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-cloud-datacatalog-spring-starter Show documentation
Show all versions of google-cloud-datacatalog-spring-starter Show documentation
Spring Boot Starter with AutoConfiguration for datacatalog
The newest version!
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.datacatalog.v1.spring;
import com.google.api.core.BetaApi;
import com.google.cloud.spring.core.Credentials;
import com.google.cloud.spring.core.CredentialsSupplier;
import com.google.cloud.spring.core.Retry;
import javax.annotation.Generated;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.context.properties.NestedConfigurationProperty;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/** Provides default property values for DataCatalog client bean */
@Generated("by google-cloud-spring-generator")
@BetaApi("Autogenerated Spring autoconfiguration is not yet stable")
@ConfigurationProperties("com.google.cloud.datacatalog.v1.data-catalog")
public class DataCatalogSpringProperties implements CredentialsSupplier {
/** OAuth2 credentials to authenticate and authorize calls to Google Cloud Client Libraries. */
@NestedConfigurationProperty
private final Credentials credentials =
new Credentials("https://www.googleapis.com/auth/cloud-platform");
/** Quota project to use for billing. */
private String quotaProjectId;
/** Number of threads used for executors. */
private Integer executorThreadCount;
/** Allow override of default transport channel provider to use REST instead of gRPC. */
private boolean useRest = false;
/** Allow override of retry settings at service level, applying to all of its RPC methods. */
@NestedConfigurationProperty private Retry retry;
/**
* Allow override of retry settings at method-level for searchCatalog. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry searchCatalogRetry;
/**
* Allow override of retry settings at method-level for createEntryGroup. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry createEntryGroupRetry;
/**
* Allow override of retry settings at method-level for getEntryGroup. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry getEntryGroupRetry;
/**
* Allow override of retry settings at method-level for updateEntryGroup. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry updateEntryGroupRetry;
/**
* Allow override of retry settings at method-level for deleteEntryGroup. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry deleteEntryGroupRetry;
/**
* Allow override of retry settings at method-level for listEntryGroups. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry listEntryGroupsRetry;
/**
* Allow override of retry settings at method-level for createEntry. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry createEntryRetry;
/**
* Allow override of retry settings at method-level for updateEntry. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry updateEntryRetry;
/**
* Allow override of retry settings at method-level for deleteEntry. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry deleteEntryRetry;
/**
* Allow override of retry settings at method-level for getEntry. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry getEntryRetry;
/**
* Allow override of retry settings at method-level for lookupEntry. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry lookupEntryRetry;
/**
* Allow override of retry settings at method-level for listEntries. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry listEntriesRetry;
/**
* Allow override of retry settings at method-level for modifyEntryOverview. If defined, this
* takes precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry modifyEntryOverviewRetry;
/**
* Allow override of retry settings at method-level for modifyEntryContacts. If defined, this
* takes precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry modifyEntryContactsRetry;
/**
* Allow override of retry settings at method-level for createTagTemplate. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry createTagTemplateRetry;
/**
* Allow override of retry settings at method-level for getTagTemplate. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry getTagTemplateRetry;
/**
* Allow override of retry settings at method-level for updateTagTemplate. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry updateTagTemplateRetry;
/**
* Allow override of retry settings at method-level for deleteTagTemplate. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry deleteTagTemplateRetry;
/**
* Allow override of retry settings at method-level for createTagTemplateField. If defined, this
* takes precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry createTagTemplateFieldRetry;
/**
* Allow override of retry settings at method-level for updateTagTemplateField. If defined, this
* takes precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry updateTagTemplateFieldRetry;
/**
* Allow override of retry settings at method-level for renameTagTemplateField. If defined, this
* takes precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry renameTagTemplateFieldRetry;
/**
* Allow override of retry settings at method-level for renameTagTemplateFieldEnumValue. If
* defined, this takes precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry renameTagTemplateFieldEnumValueRetry;
/**
* Allow override of retry settings at method-level for deleteTagTemplateField. If defined, this
* takes precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry deleteTagTemplateFieldRetry;
/**
* Allow override of retry settings at method-level for createTag. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry createTagRetry;
/**
* Allow override of retry settings at method-level for updateTag. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry updateTagRetry;
/**
* Allow override of retry settings at method-level for deleteTag. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry deleteTagRetry;
/**
* Allow override of retry settings at method-level for listTags. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry listTagsRetry;
/**
* Allow override of retry settings at method-level for starEntry. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry starEntryRetry;
/**
* Allow override of retry settings at method-level for unstarEntry. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry unstarEntryRetry;
/**
* Allow override of retry settings at method-level for setIamPolicy. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry setIamPolicyRetry;
/**
* Allow override of retry settings at method-level for getIamPolicy. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry getIamPolicyRetry;
/**
* Allow override of retry settings at method-level for testIamPermissions. If defined, this takes
* precedence over service-level retry configurations for that RPC method.
*/
@NestedConfigurationProperty private Retry testIamPermissionsRetry;
@Override
public Credentials getCredentials() {
return this.credentials;
}
public String getQuotaProjectId() {
return this.quotaProjectId;
}
public void setQuotaProjectId(String quotaProjectId) {
this.quotaProjectId = quotaProjectId;
}
public boolean getUseRest() {
return this.useRest;
}
public void setUseRest(boolean useRest) {
this.useRest = useRest;
}
public Integer getExecutorThreadCount() {
return this.executorThreadCount;
}
public void setExecutorThreadCount(Integer executorThreadCount) {
this.executorThreadCount = executorThreadCount;
}
public Retry getRetry() {
return this.retry;
}
public void setRetry(Retry retry) {
this.retry = retry;
}
public Retry getSearchCatalogRetry() {
return this.searchCatalogRetry;
}
public void setSearchCatalogRetry(Retry searchCatalogRetry) {
this.searchCatalogRetry = searchCatalogRetry;
}
public Retry getCreateEntryGroupRetry() {
return this.createEntryGroupRetry;
}
public void setCreateEntryGroupRetry(Retry createEntryGroupRetry) {
this.createEntryGroupRetry = createEntryGroupRetry;
}
public Retry getGetEntryGroupRetry() {
return this.getEntryGroupRetry;
}
public void setGetEntryGroupRetry(Retry getEntryGroupRetry) {
this.getEntryGroupRetry = getEntryGroupRetry;
}
public Retry getUpdateEntryGroupRetry() {
return this.updateEntryGroupRetry;
}
public void setUpdateEntryGroupRetry(Retry updateEntryGroupRetry) {
this.updateEntryGroupRetry = updateEntryGroupRetry;
}
public Retry getDeleteEntryGroupRetry() {
return this.deleteEntryGroupRetry;
}
public void setDeleteEntryGroupRetry(Retry deleteEntryGroupRetry) {
this.deleteEntryGroupRetry = deleteEntryGroupRetry;
}
public Retry getListEntryGroupsRetry() {
return this.listEntryGroupsRetry;
}
public void setListEntryGroupsRetry(Retry listEntryGroupsRetry) {
this.listEntryGroupsRetry = listEntryGroupsRetry;
}
public Retry getCreateEntryRetry() {
return this.createEntryRetry;
}
public void setCreateEntryRetry(Retry createEntryRetry) {
this.createEntryRetry = createEntryRetry;
}
public Retry getUpdateEntryRetry() {
return this.updateEntryRetry;
}
public void setUpdateEntryRetry(Retry updateEntryRetry) {
this.updateEntryRetry = updateEntryRetry;
}
public Retry getDeleteEntryRetry() {
return this.deleteEntryRetry;
}
public void setDeleteEntryRetry(Retry deleteEntryRetry) {
this.deleteEntryRetry = deleteEntryRetry;
}
public Retry getGetEntryRetry() {
return this.getEntryRetry;
}
public void setGetEntryRetry(Retry getEntryRetry) {
this.getEntryRetry = getEntryRetry;
}
public Retry getLookupEntryRetry() {
return this.lookupEntryRetry;
}
public void setLookupEntryRetry(Retry lookupEntryRetry) {
this.lookupEntryRetry = lookupEntryRetry;
}
public Retry getListEntriesRetry() {
return this.listEntriesRetry;
}
public void setListEntriesRetry(Retry listEntriesRetry) {
this.listEntriesRetry = listEntriesRetry;
}
public Retry getModifyEntryOverviewRetry() {
return this.modifyEntryOverviewRetry;
}
public void setModifyEntryOverviewRetry(Retry modifyEntryOverviewRetry) {
this.modifyEntryOverviewRetry = modifyEntryOverviewRetry;
}
public Retry getModifyEntryContactsRetry() {
return this.modifyEntryContactsRetry;
}
public void setModifyEntryContactsRetry(Retry modifyEntryContactsRetry) {
this.modifyEntryContactsRetry = modifyEntryContactsRetry;
}
public Retry getCreateTagTemplateRetry() {
return this.createTagTemplateRetry;
}
public void setCreateTagTemplateRetry(Retry createTagTemplateRetry) {
this.createTagTemplateRetry = createTagTemplateRetry;
}
public Retry getGetTagTemplateRetry() {
return this.getTagTemplateRetry;
}
public void setGetTagTemplateRetry(Retry getTagTemplateRetry) {
this.getTagTemplateRetry = getTagTemplateRetry;
}
public Retry getUpdateTagTemplateRetry() {
return this.updateTagTemplateRetry;
}
public void setUpdateTagTemplateRetry(Retry updateTagTemplateRetry) {
this.updateTagTemplateRetry = updateTagTemplateRetry;
}
public Retry getDeleteTagTemplateRetry() {
return this.deleteTagTemplateRetry;
}
public void setDeleteTagTemplateRetry(Retry deleteTagTemplateRetry) {
this.deleteTagTemplateRetry = deleteTagTemplateRetry;
}
public Retry getCreateTagTemplateFieldRetry() {
return this.createTagTemplateFieldRetry;
}
public void setCreateTagTemplateFieldRetry(Retry createTagTemplateFieldRetry) {
this.createTagTemplateFieldRetry = createTagTemplateFieldRetry;
}
public Retry getUpdateTagTemplateFieldRetry() {
return this.updateTagTemplateFieldRetry;
}
public void setUpdateTagTemplateFieldRetry(Retry updateTagTemplateFieldRetry) {
this.updateTagTemplateFieldRetry = updateTagTemplateFieldRetry;
}
public Retry getRenameTagTemplateFieldRetry() {
return this.renameTagTemplateFieldRetry;
}
public void setRenameTagTemplateFieldRetry(Retry renameTagTemplateFieldRetry) {
this.renameTagTemplateFieldRetry = renameTagTemplateFieldRetry;
}
public Retry getRenameTagTemplateFieldEnumValueRetry() {
return this.renameTagTemplateFieldEnumValueRetry;
}
public void setRenameTagTemplateFieldEnumValueRetry(Retry renameTagTemplateFieldEnumValueRetry) {
this.renameTagTemplateFieldEnumValueRetry = renameTagTemplateFieldEnumValueRetry;
}
public Retry getDeleteTagTemplateFieldRetry() {
return this.deleteTagTemplateFieldRetry;
}
public void setDeleteTagTemplateFieldRetry(Retry deleteTagTemplateFieldRetry) {
this.deleteTagTemplateFieldRetry = deleteTagTemplateFieldRetry;
}
public Retry getCreateTagRetry() {
return this.createTagRetry;
}
public void setCreateTagRetry(Retry createTagRetry) {
this.createTagRetry = createTagRetry;
}
public Retry getUpdateTagRetry() {
return this.updateTagRetry;
}
public void setUpdateTagRetry(Retry updateTagRetry) {
this.updateTagRetry = updateTagRetry;
}
public Retry getDeleteTagRetry() {
return this.deleteTagRetry;
}
public void setDeleteTagRetry(Retry deleteTagRetry) {
this.deleteTagRetry = deleteTagRetry;
}
public Retry getListTagsRetry() {
return this.listTagsRetry;
}
public void setListTagsRetry(Retry listTagsRetry) {
this.listTagsRetry = listTagsRetry;
}
public Retry getStarEntryRetry() {
return this.starEntryRetry;
}
public void setStarEntryRetry(Retry starEntryRetry) {
this.starEntryRetry = starEntryRetry;
}
public Retry getUnstarEntryRetry() {
return this.unstarEntryRetry;
}
public void setUnstarEntryRetry(Retry unstarEntryRetry) {
this.unstarEntryRetry = unstarEntryRetry;
}
public Retry getSetIamPolicyRetry() {
return this.setIamPolicyRetry;
}
public void setSetIamPolicyRetry(Retry setIamPolicyRetry) {
this.setIamPolicyRetry = setIamPolicyRetry;
}
public Retry getGetIamPolicyRetry() {
return this.getIamPolicyRetry;
}
public void setGetIamPolicyRetry(Retry getIamPolicyRetry) {
this.getIamPolicyRetry = getIamPolicyRetry;
}
public Retry getTestIamPermissionsRetry() {
return this.testIamPermissionsRetry;
}
public void setTestIamPermissionsRetry(Retry testIamPermissionsRetry) {
this.testIamPermissionsRetry = testIamPermissionsRetry;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy