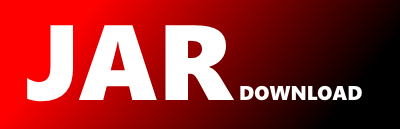
com.google.cloud.datacatalog.v1.stub.GrpcDataCatalogStub Maven / Gradle / Ivy
Show all versions of google-cloud-datacatalog Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.datacatalog.v1.stub;
import static com.google.cloud.datacatalog.v1.DataCatalogClient.ListEntriesPagedResponse;
import static com.google.cloud.datacatalog.v1.DataCatalogClient.ListEntryGroupsPagedResponse;
import static com.google.cloud.datacatalog.v1.DataCatalogClient.ListTagsPagedResponse;
import static com.google.cloud.datacatalog.v1.DataCatalogClient.SearchCatalogPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.datacatalog.v1.Contacts;
import com.google.cloud.datacatalog.v1.CreateEntryGroupRequest;
import com.google.cloud.datacatalog.v1.CreateEntryRequest;
import com.google.cloud.datacatalog.v1.CreateTagRequest;
import com.google.cloud.datacatalog.v1.CreateTagTemplateFieldRequest;
import com.google.cloud.datacatalog.v1.CreateTagTemplateRequest;
import com.google.cloud.datacatalog.v1.DeleteEntryGroupRequest;
import com.google.cloud.datacatalog.v1.DeleteEntryRequest;
import com.google.cloud.datacatalog.v1.DeleteTagRequest;
import com.google.cloud.datacatalog.v1.DeleteTagTemplateFieldRequest;
import com.google.cloud.datacatalog.v1.DeleteTagTemplateRequest;
import com.google.cloud.datacatalog.v1.Entry;
import com.google.cloud.datacatalog.v1.EntryGroup;
import com.google.cloud.datacatalog.v1.EntryOverview;
import com.google.cloud.datacatalog.v1.GetEntryGroupRequest;
import com.google.cloud.datacatalog.v1.GetEntryRequest;
import com.google.cloud.datacatalog.v1.GetTagTemplateRequest;
import com.google.cloud.datacatalog.v1.ImportEntriesMetadata;
import com.google.cloud.datacatalog.v1.ImportEntriesRequest;
import com.google.cloud.datacatalog.v1.ImportEntriesResponse;
import com.google.cloud.datacatalog.v1.ListEntriesRequest;
import com.google.cloud.datacatalog.v1.ListEntriesResponse;
import com.google.cloud.datacatalog.v1.ListEntryGroupsRequest;
import com.google.cloud.datacatalog.v1.ListEntryGroupsResponse;
import com.google.cloud.datacatalog.v1.ListTagsRequest;
import com.google.cloud.datacatalog.v1.ListTagsResponse;
import com.google.cloud.datacatalog.v1.LookupEntryRequest;
import com.google.cloud.datacatalog.v1.MigrationConfig;
import com.google.cloud.datacatalog.v1.ModifyEntryContactsRequest;
import com.google.cloud.datacatalog.v1.ModifyEntryOverviewRequest;
import com.google.cloud.datacatalog.v1.OrganizationConfig;
import com.google.cloud.datacatalog.v1.ReconcileTagsMetadata;
import com.google.cloud.datacatalog.v1.ReconcileTagsRequest;
import com.google.cloud.datacatalog.v1.ReconcileTagsResponse;
import com.google.cloud.datacatalog.v1.RenameTagTemplateFieldEnumValueRequest;
import com.google.cloud.datacatalog.v1.RenameTagTemplateFieldRequest;
import com.google.cloud.datacatalog.v1.RetrieveConfigRequest;
import com.google.cloud.datacatalog.v1.RetrieveEffectiveConfigRequest;
import com.google.cloud.datacatalog.v1.SearchCatalogRequest;
import com.google.cloud.datacatalog.v1.SearchCatalogResponse;
import com.google.cloud.datacatalog.v1.SetConfigRequest;
import com.google.cloud.datacatalog.v1.StarEntryRequest;
import com.google.cloud.datacatalog.v1.StarEntryResponse;
import com.google.cloud.datacatalog.v1.Tag;
import com.google.cloud.datacatalog.v1.TagTemplate;
import com.google.cloud.datacatalog.v1.TagTemplateField;
import com.google.cloud.datacatalog.v1.UnstarEntryRequest;
import com.google.cloud.datacatalog.v1.UnstarEntryResponse;
import com.google.cloud.datacatalog.v1.UpdateEntryGroupRequest;
import com.google.cloud.datacatalog.v1.UpdateEntryRequest;
import com.google.cloud.datacatalog.v1.UpdateTagRequest;
import com.google.cloud.datacatalog.v1.UpdateTagTemplateFieldRequest;
import com.google.cloud.datacatalog.v1.UpdateTagTemplateRequest;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import com.google.protobuf.Empty;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the DataCatalog service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public class GrpcDataCatalogStub extends DataCatalogStub {
private static final MethodDescriptor
searchCatalogMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/SearchCatalog")
.setRequestMarshaller(
ProtoUtils.marshaller(SearchCatalogRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(SearchCatalogResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
createEntryGroupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/CreateEntryGroup")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateEntryGroupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(EntryGroup.getDefaultInstance()))
.build();
private static final MethodDescriptor
getEntryGroupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/GetEntryGroup")
.setRequestMarshaller(
ProtoUtils.marshaller(GetEntryGroupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(EntryGroup.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateEntryGroupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/UpdateEntryGroup")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateEntryGroupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(EntryGroup.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteEntryGroupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/DeleteEntryGroup")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteEntryGroupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
listEntryGroupsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/ListEntryGroups")
.setRequestMarshaller(
ProtoUtils.marshaller(ListEntryGroupsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListEntryGroupsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor createEntryMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/CreateEntry")
.setRequestMarshaller(ProtoUtils.marshaller(CreateEntryRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Entry.getDefaultInstance()))
.build();
private static final MethodDescriptor updateEntryMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/UpdateEntry")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateEntryRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Entry.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteEntryMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/DeleteEntry")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteEntryRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor getEntryMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/GetEntry")
.setRequestMarshaller(ProtoUtils.marshaller(GetEntryRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Entry.getDefaultInstance()))
.build();
private static final MethodDescriptor lookupEntryMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/LookupEntry")
.setRequestMarshaller(ProtoUtils.marshaller(LookupEntryRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Entry.getDefaultInstance()))
.build();
private static final MethodDescriptor
listEntriesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/ListEntries")
.setRequestMarshaller(ProtoUtils.marshaller(ListEntriesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListEntriesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
modifyEntryOverviewMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/ModifyEntryOverview")
.setRequestMarshaller(
ProtoUtils.marshaller(ModifyEntryOverviewRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(EntryOverview.getDefaultInstance()))
.build();
private static final MethodDescriptor
modifyEntryContactsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/ModifyEntryContacts")
.setRequestMarshaller(
ProtoUtils.marshaller(ModifyEntryContactsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Contacts.getDefaultInstance()))
.build();
private static final MethodDescriptor
createTagTemplateMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/CreateTagTemplate")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateTagTemplateRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(TagTemplate.getDefaultInstance()))
.build();
private static final MethodDescriptor
getTagTemplateMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/GetTagTemplate")
.setRequestMarshaller(
ProtoUtils.marshaller(GetTagTemplateRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(TagTemplate.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateTagTemplateMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/UpdateTagTemplate")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateTagTemplateRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(TagTemplate.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteTagTemplateMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/DeleteTagTemplate")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteTagTemplateRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
createTagTemplateFieldMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/CreateTagTemplateField")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateTagTemplateFieldRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(TagTemplateField.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateTagTemplateFieldMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/UpdateTagTemplateField")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateTagTemplateFieldRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(TagTemplateField.getDefaultInstance()))
.build();
private static final MethodDescriptor
renameTagTemplateFieldMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/RenameTagTemplateField")
.setRequestMarshaller(
ProtoUtils.marshaller(RenameTagTemplateFieldRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(TagTemplateField.getDefaultInstance()))
.build();
private static final MethodDescriptor
renameTagTemplateFieldEnumValueMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.datacatalog.v1.DataCatalog/RenameTagTemplateFieldEnumValue")
.setRequestMarshaller(
ProtoUtils.marshaller(
RenameTagTemplateFieldEnumValueRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(TagTemplateField.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteTagTemplateFieldMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/DeleteTagTemplateField")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteTagTemplateFieldRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor createTagMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/CreateTag")
.setRequestMarshaller(ProtoUtils.marshaller(CreateTagRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Tag.getDefaultInstance()))
.build();
private static final MethodDescriptor updateTagMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/UpdateTag")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateTagRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Tag.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteTagMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/DeleteTag")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteTagRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
listTagsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/ListTags")
.setRequestMarshaller(ProtoUtils.marshaller(ListTagsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListTagsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
reconcileTagsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/ReconcileTags")
.setRequestMarshaller(
ProtoUtils.marshaller(ReconcileTagsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
starEntryMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/StarEntry")
.setRequestMarshaller(ProtoUtils.marshaller(StarEntryRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(StarEntryResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
unstarEntryMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/UnstarEntry")
.setRequestMarshaller(ProtoUtils.marshaller(UnstarEntryRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(UnstarEntryResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor setIamPolicyMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/SetIamPolicy")
.setRequestMarshaller(ProtoUtils.marshaller(SetIamPolicyRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Policy.getDefaultInstance()))
.build();
private static final MethodDescriptor getIamPolicyMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/GetIamPolicy")
.setRequestMarshaller(ProtoUtils.marshaller(GetIamPolicyRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Policy.getDefaultInstance()))
.build();
private static final MethodDescriptor
testIamPermissionsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/TestIamPermissions")
.setRequestMarshaller(
ProtoUtils.marshaller(TestIamPermissionsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(TestIamPermissionsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
importEntriesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/ImportEntries")
.setRequestMarshaller(
ProtoUtils.marshaller(ImportEntriesRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
setConfigMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/SetConfig")
.setRequestMarshaller(ProtoUtils.marshaller(SetConfigRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(MigrationConfig.getDefaultInstance()))
.build();
private static final MethodDescriptor
retrieveConfigMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/RetrieveConfig")
.setRequestMarshaller(
ProtoUtils.marshaller(RetrieveConfigRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(OrganizationConfig.getDefaultInstance()))
.build();
private static final MethodDescriptor
retrieveEffectiveConfigMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.datacatalog.v1.DataCatalog/RetrieveEffectiveConfig")
.setRequestMarshaller(
ProtoUtils.marshaller(RetrieveEffectiveConfigRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(MigrationConfig.getDefaultInstance()))
.build();
private final UnaryCallable searchCatalogCallable;
private final UnaryCallable
searchCatalogPagedCallable;
private final UnaryCallable createEntryGroupCallable;
private final UnaryCallable getEntryGroupCallable;
private final UnaryCallable updateEntryGroupCallable;
private final UnaryCallable deleteEntryGroupCallable;
private final UnaryCallable
listEntryGroupsCallable;
private final UnaryCallable
listEntryGroupsPagedCallable;
private final UnaryCallable createEntryCallable;
private final UnaryCallable updateEntryCallable;
private final UnaryCallable deleteEntryCallable;
private final UnaryCallable getEntryCallable;
private final UnaryCallable lookupEntryCallable;
private final UnaryCallable listEntriesCallable;
private final UnaryCallable
listEntriesPagedCallable;
private final UnaryCallable
modifyEntryOverviewCallable;
private final UnaryCallable modifyEntryContactsCallable;
private final UnaryCallable createTagTemplateCallable;
private final UnaryCallable getTagTemplateCallable;
private final UnaryCallable updateTagTemplateCallable;
private final UnaryCallable deleteTagTemplateCallable;
private final UnaryCallable
createTagTemplateFieldCallable;
private final UnaryCallable
updateTagTemplateFieldCallable;
private final UnaryCallable
renameTagTemplateFieldCallable;
private final UnaryCallable
renameTagTemplateFieldEnumValueCallable;
private final UnaryCallable deleteTagTemplateFieldCallable;
private final UnaryCallable createTagCallable;
private final UnaryCallable updateTagCallable;
private final UnaryCallable deleteTagCallable;
private final UnaryCallable listTagsCallable;
private final UnaryCallable listTagsPagedCallable;
private final UnaryCallable reconcileTagsCallable;
private final OperationCallable<
ReconcileTagsRequest, ReconcileTagsResponse, ReconcileTagsMetadata>
reconcileTagsOperationCallable;
private final UnaryCallable starEntryCallable;
private final UnaryCallable unstarEntryCallable;
private final UnaryCallable setIamPolicyCallable;
private final UnaryCallable getIamPolicyCallable;
private final UnaryCallable
testIamPermissionsCallable;
private final UnaryCallable importEntriesCallable;
private final OperationCallable<
ImportEntriesRequest, ImportEntriesResponse, ImportEntriesMetadata>
importEntriesOperationCallable;
private final UnaryCallable setConfigCallable;
private final UnaryCallable retrieveConfigCallable;
private final UnaryCallable
retrieveEffectiveConfigCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcDataCatalogStub create(DataCatalogStubSettings settings)
throws IOException {
return new GrpcDataCatalogStub(settings, ClientContext.create(settings));
}
public static final GrpcDataCatalogStub create(ClientContext clientContext) throws IOException {
return new GrpcDataCatalogStub(DataCatalogStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcDataCatalogStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcDataCatalogStub(
DataCatalogStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcDataCatalogStub, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcDataCatalogStub(DataCatalogStubSettings settings, ClientContext clientContext)
throws IOException {
this(settings, clientContext, new GrpcDataCatalogCallableFactory());
}
/**
* Constructs an instance of GrpcDataCatalogStub, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcDataCatalogStub(
DataCatalogStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings searchCatalogTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(searchCatalogMethodDescriptor)
.build();
GrpcCallSettings createEntryGroupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createEntryGroupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getEntryGroupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getEntryGroupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateEntryGroupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateEntryGroupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add(
"entry_group.name", String.valueOf(request.getEntryGroup().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteEntryGroupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteEntryGroupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listEntryGroupsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listEntryGroupsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings createEntryTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createEntryMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateEntryTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateEntryMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("entry.name", String.valueOf(request.getEntry().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteEntryTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteEntryMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getEntryTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getEntryMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings lookupEntryTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(lookupEntryMethodDescriptor)
.build();
GrpcCallSettings listEntriesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listEntriesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
modifyEntryOverviewTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(modifyEntryOverviewMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings modifyEntryContactsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(modifyEntryContactsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createTagTemplateTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createTagTemplateMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getTagTemplateTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getTagTemplateMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateTagTemplateTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateTagTemplateMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add(
"tag_template.name", String.valueOf(request.getTagTemplate().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteTagTemplateTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteTagTemplateMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
createTagTemplateFieldTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createTagTemplateFieldMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
updateTagTemplateFieldTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateTagTemplateFieldMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
renameTagTemplateFieldTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(renameTagTemplateFieldMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
renameTagTemplateFieldEnumValueTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(renameTagTemplateFieldEnumValueMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteTagTemplateFieldTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteTagTemplateFieldMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createTagTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createTagMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateTagTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateTagMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("tag.name", String.valueOf(request.getTag().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteTagTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteTagMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listTagsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listTagsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings reconcileTagsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(reconcileTagsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings starEntryTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(starEntryMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings unstarEntryTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(unstarEntryMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings setIamPolicyTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(setIamPolicyMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("resource", String.valueOf(request.getResource()));
return builder.build();
})
.build();
GrpcCallSettings getIamPolicyTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getIamPolicyMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("resource", String.valueOf(request.getResource()));
return builder.build();
})
.build();
GrpcCallSettings
testIamPermissionsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(testIamPermissionsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("resource", String.valueOf(request.getResource()));
return builder.build();
})
.build();
GrpcCallSettings importEntriesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(importEntriesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings setConfigTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(setConfigMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings retrieveConfigTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(retrieveConfigMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
retrieveEffectiveConfigTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(retrieveEffectiveConfigMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
this.searchCatalogCallable =
callableFactory.createUnaryCallable(
searchCatalogTransportSettings, settings.searchCatalogSettings(), clientContext);
this.searchCatalogPagedCallable =
callableFactory.createPagedCallable(
searchCatalogTransportSettings, settings.searchCatalogSettings(), clientContext);
this.createEntryGroupCallable =
callableFactory.createUnaryCallable(
createEntryGroupTransportSettings, settings.createEntryGroupSettings(), clientContext);
this.getEntryGroupCallable =
callableFactory.createUnaryCallable(
getEntryGroupTransportSettings, settings.getEntryGroupSettings(), clientContext);
this.updateEntryGroupCallable =
callableFactory.createUnaryCallable(
updateEntryGroupTransportSettings, settings.updateEntryGroupSettings(), clientContext);
this.deleteEntryGroupCallable =
callableFactory.createUnaryCallable(
deleteEntryGroupTransportSettings, settings.deleteEntryGroupSettings(), clientContext);
this.listEntryGroupsCallable =
callableFactory.createUnaryCallable(
listEntryGroupsTransportSettings, settings.listEntryGroupsSettings(), clientContext);
this.listEntryGroupsPagedCallable =
callableFactory.createPagedCallable(
listEntryGroupsTransportSettings, settings.listEntryGroupsSettings(), clientContext);
this.createEntryCallable =
callableFactory.createUnaryCallable(
createEntryTransportSettings, settings.createEntrySettings(), clientContext);
this.updateEntryCallable =
callableFactory.createUnaryCallable(
updateEntryTransportSettings, settings.updateEntrySettings(), clientContext);
this.deleteEntryCallable =
callableFactory.createUnaryCallable(
deleteEntryTransportSettings, settings.deleteEntrySettings(), clientContext);
this.getEntryCallable =
callableFactory.createUnaryCallable(
getEntryTransportSettings, settings.getEntrySettings(), clientContext);
this.lookupEntryCallable =
callableFactory.createUnaryCallable(
lookupEntryTransportSettings, settings.lookupEntrySettings(), clientContext);
this.listEntriesCallable =
callableFactory.createUnaryCallable(
listEntriesTransportSettings, settings.listEntriesSettings(), clientContext);
this.listEntriesPagedCallable =
callableFactory.createPagedCallable(
listEntriesTransportSettings, settings.listEntriesSettings(), clientContext);
this.modifyEntryOverviewCallable =
callableFactory.createUnaryCallable(
modifyEntryOverviewTransportSettings,
settings.modifyEntryOverviewSettings(),
clientContext);
this.modifyEntryContactsCallable =
callableFactory.createUnaryCallable(
modifyEntryContactsTransportSettings,
settings.modifyEntryContactsSettings(),
clientContext);
this.createTagTemplateCallable =
callableFactory.createUnaryCallable(
createTagTemplateTransportSettings,
settings.createTagTemplateSettings(),
clientContext);
this.getTagTemplateCallable =
callableFactory.createUnaryCallable(
getTagTemplateTransportSettings, settings.getTagTemplateSettings(), clientContext);
this.updateTagTemplateCallable =
callableFactory.createUnaryCallable(
updateTagTemplateTransportSettings,
settings.updateTagTemplateSettings(),
clientContext);
this.deleteTagTemplateCallable =
callableFactory.createUnaryCallable(
deleteTagTemplateTransportSettings,
settings.deleteTagTemplateSettings(),
clientContext);
this.createTagTemplateFieldCallable =
callableFactory.createUnaryCallable(
createTagTemplateFieldTransportSettings,
settings.createTagTemplateFieldSettings(),
clientContext);
this.updateTagTemplateFieldCallable =
callableFactory.createUnaryCallable(
updateTagTemplateFieldTransportSettings,
settings.updateTagTemplateFieldSettings(),
clientContext);
this.renameTagTemplateFieldCallable =
callableFactory.createUnaryCallable(
renameTagTemplateFieldTransportSettings,
settings.renameTagTemplateFieldSettings(),
clientContext);
this.renameTagTemplateFieldEnumValueCallable =
callableFactory.createUnaryCallable(
renameTagTemplateFieldEnumValueTransportSettings,
settings.renameTagTemplateFieldEnumValueSettings(),
clientContext);
this.deleteTagTemplateFieldCallable =
callableFactory.createUnaryCallable(
deleteTagTemplateFieldTransportSettings,
settings.deleteTagTemplateFieldSettings(),
clientContext);
this.createTagCallable =
callableFactory.createUnaryCallable(
createTagTransportSettings, settings.createTagSettings(), clientContext);
this.updateTagCallable =
callableFactory.createUnaryCallable(
updateTagTransportSettings, settings.updateTagSettings(), clientContext);
this.deleteTagCallable =
callableFactory.createUnaryCallable(
deleteTagTransportSettings, settings.deleteTagSettings(), clientContext);
this.listTagsCallable =
callableFactory.createUnaryCallable(
listTagsTransportSettings, settings.listTagsSettings(), clientContext);
this.listTagsPagedCallable =
callableFactory.createPagedCallable(
listTagsTransportSettings, settings.listTagsSettings(), clientContext);
this.reconcileTagsCallable =
callableFactory.createUnaryCallable(
reconcileTagsTransportSettings, settings.reconcileTagsSettings(), clientContext);
this.reconcileTagsOperationCallable =
callableFactory.createOperationCallable(
reconcileTagsTransportSettings,
settings.reconcileTagsOperationSettings(),
clientContext,
operationsStub);
this.starEntryCallable =
callableFactory.createUnaryCallable(
starEntryTransportSettings, settings.starEntrySettings(), clientContext);
this.unstarEntryCallable =
callableFactory.createUnaryCallable(
unstarEntryTransportSettings, settings.unstarEntrySettings(), clientContext);
this.setIamPolicyCallable =
callableFactory.createUnaryCallable(
setIamPolicyTransportSettings, settings.setIamPolicySettings(), clientContext);
this.getIamPolicyCallable =
callableFactory.createUnaryCallable(
getIamPolicyTransportSettings, settings.getIamPolicySettings(), clientContext);
this.testIamPermissionsCallable =
callableFactory.createUnaryCallable(
testIamPermissionsTransportSettings,
settings.testIamPermissionsSettings(),
clientContext);
this.importEntriesCallable =
callableFactory.createUnaryCallable(
importEntriesTransportSettings, settings.importEntriesSettings(), clientContext);
this.importEntriesOperationCallable =
callableFactory.createOperationCallable(
importEntriesTransportSettings,
settings.importEntriesOperationSettings(),
clientContext,
operationsStub);
this.setConfigCallable =
callableFactory.createUnaryCallable(
setConfigTransportSettings, settings.setConfigSettings(), clientContext);
this.retrieveConfigCallable =
callableFactory.createUnaryCallable(
retrieveConfigTransportSettings, settings.retrieveConfigSettings(), clientContext);
this.retrieveEffectiveConfigCallable =
callableFactory.createUnaryCallable(
retrieveEffectiveConfigTransportSettings,
settings.retrieveEffectiveConfigSettings(),
clientContext);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable searchCatalogCallable() {
return searchCatalogCallable;
}
@Override
public UnaryCallable
searchCatalogPagedCallable() {
return searchCatalogPagedCallable;
}
@Override
public UnaryCallable createEntryGroupCallable() {
return createEntryGroupCallable;
}
@Override
public UnaryCallable getEntryGroupCallable() {
return getEntryGroupCallable;
}
@Override
public UnaryCallable updateEntryGroupCallable() {
return updateEntryGroupCallable;
}
@Override
public UnaryCallable deleteEntryGroupCallable() {
return deleteEntryGroupCallable;
}
@Override
public UnaryCallable listEntryGroupsCallable() {
return listEntryGroupsCallable;
}
@Override
public UnaryCallable
listEntryGroupsPagedCallable() {
return listEntryGroupsPagedCallable;
}
@Override
public UnaryCallable createEntryCallable() {
return createEntryCallable;
}
@Override
public UnaryCallable updateEntryCallable() {
return updateEntryCallable;
}
@Override
public UnaryCallable deleteEntryCallable() {
return deleteEntryCallable;
}
@Override
public UnaryCallable getEntryCallable() {
return getEntryCallable;
}
@Override
public UnaryCallable lookupEntryCallable() {
return lookupEntryCallable;
}
@Override
public UnaryCallable listEntriesCallable() {
return listEntriesCallable;
}
@Override
public UnaryCallable listEntriesPagedCallable() {
return listEntriesPagedCallable;
}
@Override
public UnaryCallable modifyEntryOverviewCallable() {
return modifyEntryOverviewCallable;
}
@Override
public UnaryCallable modifyEntryContactsCallable() {
return modifyEntryContactsCallable;
}
@Override
public UnaryCallable createTagTemplateCallable() {
return createTagTemplateCallable;
}
@Override
public UnaryCallable getTagTemplateCallable() {
return getTagTemplateCallable;
}
@Override
public UnaryCallable updateTagTemplateCallable() {
return updateTagTemplateCallable;
}
@Override
public UnaryCallable deleteTagTemplateCallable() {
return deleteTagTemplateCallable;
}
@Override
public UnaryCallable
createTagTemplateFieldCallable() {
return createTagTemplateFieldCallable;
}
@Override
public UnaryCallable
updateTagTemplateFieldCallable() {
return updateTagTemplateFieldCallable;
}
@Override
public UnaryCallable
renameTagTemplateFieldCallable() {
return renameTagTemplateFieldCallable;
}
@Override
public UnaryCallable
renameTagTemplateFieldEnumValueCallable() {
return renameTagTemplateFieldEnumValueCallable;
}
@Override
public UnaryCallable deleteTagTemplateFieldCallable() {
return deleteTagTemplateFieldCallable;
}
@Override
public UnaryCallable createTagCallable() {
return createTagCallable;
}
@Override
public UnaryCallable updateTagCallable() {
return updateTagCallable;
}
@Override
public UnaryCallable deleteTagCallable() {
return deleteTagCallable;
}
@Override
public UnaryCallable listTagsCallable() {
return listTagsCallable;
}
@Override
public UnaryCallable listTagsPagedCallable() {
return listTagsPagedCallable;
}
@Override
public UnaryCallable reconcileTagsCallable() {
return reconcileTagsCallable;
}
@Override
public OperationCallable
reconcileTagsOperationCallable() {
return reconcileTagsOperationCallable;
}
@Override
public UnaryCallable starEntryCallable() {
return starEntryCallable;
}
@Override
public UnaryCallable unstarEntryCallable() {
return unstarEntryCallable;
}
@Override
public UnaryCallable setIamPolicyCallable() {
return setIamPolicyCallable;
}
@Override
public UnaryCallable getIamPolicyCallable() {
return getIamPolicyCallable;
}
@Override
public UnaryCallable
testIamPermissionsCallable() {
return testIamPermissionsCallable;
}
@Override
public UnaryCallable importEntriesCallable() {
return importEntriesCallable;
}
@Override
public OperationCallable
importEntriesOperationCallable() {
return importEntriesOperationCallable;
}
@Override
public UnaryCallable setConfigCallable() {
return setConfigCallable;
}
@Override
public UnaryCallable retrieveConfigCallable() {
return retrieveConfigCallable;
}
@Override
public UnaryCallable
retrieveEffectiveConfigCallable() {
return retrieveEffectiveConfigCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}