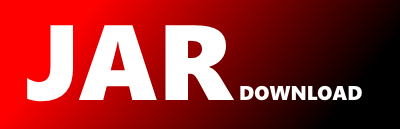
com.google.cloud.dialogflow.v2beta1.package-info Maven / Gradle / Ivy
Show all versions of google-cloud-dialogflow Show documentation
/*
* Copyright 2019 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* A client to Dialogflow API.
*
* The interfaces provided are listed below, along with usage samples.
*
*
============ AgentsClient ============
*
*
Service Description: Agents are best described as Natural Language Understanding (NLU) modules
* that transform user requests into actionable data. You can include agents in your app, product,
* or service to determine user intent and respond to the user in a natural way.
*
*
After you create an agent, you can add [Intents][google.cloud.dialogflow.v2beta1.Intents],
* [Contexts][google.cloud.dialogflow.v2beta1.Contexts], [Entity
* Types][google.cloud.dialogflow.v2beta1.EntityTypes],
* [Webhooks][google.cloud.dialogflow.v2beta1.WebhookRequest], and so on to manage the flow of a
* conversation and match user input to predefined intents and actions.
*
*
You can create an agent using both Dialogflow Standard Edition and Dialogflow Enterprise
* Edition. For details, see [Dialogflow
* Editions](https://cloud.google.com/dialogflow-enterprise/docs/editions).
*
*
You can save your agent for backup or versioning by exporting the agent by using the
* [ExportAgent][google.cloud.dialogflow.v2beta1.Agents.ExportAgent] method. You can import a saved
* agent by using the [ImportAgent][google.cloud.dialogflow.v2beta1.Agents.ImportAgent] method.
*
*
Dialogflow provides several [prebuilt agents](https://dialogflow.com/docs/prebuilt-agents) for
* common conversation scenarios such as determining a date and time, converting currency, and so
* on.
*
*
For more information about agents, see the [Dialogflow
* documentation](https://dialogflow.com/docs/agents).
*
*
Sample for AgentsClient:
*
*
*
* try (AgentsClient agentsClient = AgentsClient.create()) {
* ProjectName parent = ProjectName.of("[PROJECT]");
* Agent response = agentsClient.getAgent(parent);
* }
*
*
*
* ============== ContextsClient ==============
*
* Service Description: A context represents additional information included with user input or
* with an intent returned by the Dialogflow API. Contexts are helpful for differentiating user
* input which may be vague or have a different meaning depending on additional details from your
* application such as user setting and preferences, previous user input, where the user is in your
* application, geographic location, and so on.
*
*
You can include contexts as input parameters of a
* [DetectIntent][google.cloud.dialogflow.v2beta1.Sessions.DetectIntent] (or
* [StreamingDetectIntent][google.cloud.dialogflow.v2beta1.Sessions.StreamingDetectIntent]) request,
* or as output contexts included in the returned intent. Contexts expire when an intent is matched,
* after the number of `DetectIntent` requests specified by the `lifespan_count` parameter, or after
* 10 minutes if no intents are matched for a `DetectIntent` request.
*
*
For more information about contexts, see the [Dialogflow
* documentation](https://dialogflow.com/docs/contexts).
*
*
Sample for ContextsClient:
*
*
*
* try (ContextsClient contextsClient = ContextsClient.create()) {
* ContextName name = ContextName.of("[PROJECT]", "[SESSION]", "[CONTEXT]");
* Context response = contextsClient.getContext(name);
* }
*
*
*
* =============== DocumentsClient ===============
*
* Service Description: Manages documents of a knowledge base.
*
*
Sample for DocumentsClient:
*
*
*
* try (DocumentsClient documentsClient = DocumentsClient.create()) {
* DocumentName name = DocumentName.of("[PROJECT]", "[KNOWLEDGE_BASE]", "[DOCUMENT]");
* Document response = documentsClient.getDocument(name);
* }
*
*
*
* ================= EntityTypesClient =================
*
* Service Description: Entities are extracted from user input and represent parameters that are
* meaningful to your application. For example, a date range, a proper name such as a geographic
* location or landmark, and so on. Entities represent actionable data for your application.
*
*
When you define an entity, you can also include synonyms that all map to that entity. For
* example, "soft drink", "soda", "pop", and so on.
*
*
There are three types of entities:
*
*
* **System** - entities that are defined by the Dialogflow API for common
* data types such as date, time, currency, and so on. A system entity is represented by the
* `EntityType` type.
*
*
* **Developer** - entities that are defined by you that represent
* actionable data that is meaningful to your application. For example, you could define a
* `pizza.sauce` entity for red or white pizza sauce, a `pizza.cheese` entity for the different
* types of cheese on a pizza, a `pizza.topping` entity for different toppings, and so on. A
* developer entity is represented by the `EntityType` type.
*
*
* **User** - entities that are built for an individual user such as
* favorites, preferences, playlists, and so on. A user entity is represented by the
* [SessionEntityType][google.cloud.dialogflow.v2beta1.SessionEntityType] type.
*
*
For more information about entity types, see the [Dialogflow
* documentation](https://dialogflow.com/docs/entities).
*
*
Sample for EntityTypesClient:
*
*
*
* try (EntityTypesClient entityTypesClient = EntityTypesClient.create()) {
* EntityTypeName name = EntityTypeName.of("[PROJECT]", "[ENTITY_TYPE]");
* EntityType response = entityTypesClient.getEntityType(name);
* }
*
*
*
* ============= IntentsClient =============
*
* Service Description: An intent represents a mapping between input from a user and an action to
* be taken by your application. When you pass user input to the
* [DetectIntent][google.cloud.dialogflow.v2beta1.Sessions.DetectIntent] (or
* [StreamingDetectIntent][google.cloud.dialogflow.v2beta1.Sessions.StreamingDetectIntent]) method,
* the Dialogflow API analyzes the input and searches for a matching intent. If no match is found,
* the Dialogflow API returns a fallback intent (`is_fallback` = true).
*
*
You can provide additional information for the Dialogflow API to use to match user input to an
* intent by adding the following to your intent.
*
*
* **Contexts** - provide additional context for intent analysis. For
* example, if an intent is related to an object in your application that plays music, you can
* provide a context to determine when to match the intent if the user input is “turn it off”. You
* can include a context that matches the intent when there is previous user input of "play music",
* and not when there is previous user input of "turn on the light".
*
*
* **Events** - allow for matching an intent by using an event name instead
* of user input. Your application can provide an event name and related parameters to the
* Dialogflow API to match an intent. For example, when your application starts, you can send a
* welcome event with a user name parameter to the Dialogflow API to match an intent with a
* personalized welcome message for the user.
*
*
* **Training phrases** - provide examples of user input to train the
* Dialogflow API agent to better match intents.
*
*
For more information about intents, see the [Dialogflow
* documentation](https://dialogflow.com/docs/intents).
*
*
Sample for IntentsClient:
*
*
*
* try (IntentsClient intentsClient = IntentsClient.create()) {
* IntentName name = IntentName.of("[PROJECT]", "[INTENT]");
* Intent response = intentsClient.getIntent(name);
* }
*
*
*
* ==================== KnowledgeBasesClient ====================
*
* Service Description: Manages knowledge bases.
*
*
Allows users to setup and maintain knowledge bases with their knowledge data.
*
*
Sample for KnowledgeBasesClient:
*
*
*
* try (KnowledgeBasesClient knowledgeBasesClient = KnowledgeBasesClient.create()) {
* KnowledgeBaseName name = KnowledgeBaseName.of("[PROJECT]", "[KNOWLEDGE_BASE]");
* KnowledgeBase response = knowledgeBasesClient.getKnowledgeBase(name);
* }
*
*
*
* ======================== SessionEntityTypesClient ========================
*
* Service Description: Entities are extracted from user input and represent parameters that are
* meaningful to your application. For example, a date range, a proper name such as a geographic
* location or landmark, and so on. Entities represent actionable data for your application.
*
*
Session entity types are referred to as **User** entity types and are entities
* that are built for an individual user such as favorites, preferences, playlists, and so on. You
* can redefine a session entity type at the session level.
*
*
For more information about entity types, see the [Dialogflow
* documentation](https://dialogflow.com/docs/entities).
*
*
Sample for SessionEntityTypesClient:
*
*
*
* try (SessionEntityTypesClient sessionEntityTypesClient = SessionEntityTypesClient.create()) {
* SessionEntityTypeName name = SessionEntityTypeName.of("[PROJECT]", "[SESSION]", "[ENTITY_TYPE]");
* SessionEntityType response = sessionEntityTypesClient.getSessionEntityType(name);
* }
*
*
*
* ============== SessionsClient ==============
*
* Service Description: A session represents an interaction with a user. You retrieve user input
* and pass it to the [DetectIntent][google.cloud.dialogflow.v2beta1.Sessions.DetectIntent] (or
* [StreamingDetectIntent][google.cloud.dialogflow.v2beta1.Sessions.StreamingDetectIntent]) method
* to determine user intent and respond.
*
*
Sample for SessionsClient:
*
*
*
* try (SessionsClient sessionsClient = SessionsClient.create()) {
* SessionName session = SessionName.of("[PROJECT]", "[SESSION]");
* QueryInput queryInput = QueryInput.newBuilder().build();
* DetectIntentResponse response = sessionsClient.detectIntent(session, queryInput);
* }
*
*
*/
package com.google.cloud.dialogflow.v2beta1;