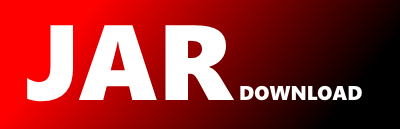
com.google.cloud.dialogflow.v2beta1.ConversationsClient Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.dialogflow.v2beta1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.dialogflow.v2beta1.stub.ConversationsStub;
import com.google.cloud.dialogflow.v2beta1.stub.ConversationsStubSettings;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.common.util.concurrent.MoreExecutors;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Service for managing
* [Conversations][google.cloud.dialogflow.v2beta1.Conversation].
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Conversation conversation = Conversation.newBuilder().build();
* Conversation response = conversationsClient.createConversation(parent, conversation);
* }
* }
*
* Note: close() needs to be called on the ConversationsClient object to clean up resources such
* as threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* CreateConversation
* Creates a new conversation. Conversations are auto-completed after 24 hours.
*
Conversation Lifecycle: There are two stages during a conversation: Automated Agent Stage and Assist Stage.
*
For Automated Agent Stage, there will be a dialogflow agent responding to user queries.
*
For Assist Stage, there's no dialogflow agent responding to user queries. But we will provide suggestions which are generated from conversation.
*
If [Conversation.conversation_profile][google.cloud.dialogflow.v2beta1.Conversation.conversation_profile] is configured for a dialogflow agent, conversation will start from `Automated Agent Stage`, otherwise, it will start from `Assist Stage`. And during `Automated Agent Stage`, once an [Intent][google.cloud.dialogflow.v2beta1.Intent] with [Intent.live_agent_handoff][google.cloud.dialogflow.v2beta1.Intent.live_agent_handoff] is triggered, conversation will transfer to Assist Stage.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createConversation(CreateConversationRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createConversation(LocationName parent, Conversation conversation)
*
createConversation(ProjectName parent, Conversation conversation)
*
createConversation(String parent, Conversation conversation)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createConversationCallable()
*
*
*
*
* ListConversations
* Returns the list of all conversations in the specified project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listConversations(ListConversationsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listConversations(LocationName parent)
*
listConversations(ProjectName parent)
*
listConversations(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listConversationsPagedCallable()
*
listConversationsCallable()
*
*
*
*
* GetConversation
* Retrieves the specific conversation.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getConversation(GetConversationRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getConversation(ConversationName name)
*
getConversation(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getConversationCallable()
*
*
*
*
* CompleteConversation
* Completes the specified conversation. Finished conversations are purged from the database after 30 days.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* completeConversation(CompleteConversationRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* completeConversation(ConversationName name)
*
completeConversation(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* completeConversationCallable()
*
*
*
*
* BatchCreateMessages
* Batch ingests messages to conversation. Customers can use this RPC to ingest historical messages to conversation.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* batchCreateMessages(BatchCreateMessagesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* batchCreateMessages(ConversationName parent)
*
batchCreateMessages(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* batchCreateMessagesCallable()
*
*
*
*
* ListMessages
* Lists messages that belong to a given conversation. `messages` are ordered by `create_time` in descending order. To fetch updates without duplication, send request with filter `create_time_epoch_microseconds > [first item's create_time of previous request]` and empty page_token.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listMessages(ListMessagesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listMessages(ConversationName parent)
*
listMessages(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listMessagesPagedCallable()
*
listMessagesCallable()
*
*
*
*
* SuggestConversationSummary
* Suggest summary for a conversation based on specific historical messages. The range of the messages to be used for summary can be specified in the request.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* suggestConversationSummary(SuggestConversationSummaryRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* suggestConversationSummary(ConversationName conversation)
*
suggestConversationSummary(String conversation)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* suggestConversationSummaryCallable()
*
*
*
*
* GenerateStatelessSummary
* Generates and returns a summary for a conversation that does not have a resource created for it.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* generateStatelessSummary(GenerateStatelessSummaryRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* generateStatelessSummaryCallable()
*
*
*
*
* SearchKnowledge
* Get answers for the given query based on knowledge documents.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* searchKnowledge(SearchKnowledgeRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* searchKnowledgeCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listLocationsPagedCallable()
*
listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getLocationCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of ConversationsSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ConversationsSettings conversationsSettings =
* ConversationsSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* ConversationsClient conversationsClient = ConversationsClient.create(conversationsSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ConversationsSettings conversationsSettings =
* ConversationsSettings.newBuilder().setEndpoint(myEndpoint).build();
* ConversationsClient conversationsClient = ConversationsClient.create(conversationsSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ConversationsSettings conversationsSettings =
* ConversationsSettings.newHttpJsonBuilder().build();
* ConversationsClient conversationsClient = ConversationsClient.create(conversationsSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class ConversationsClient implements BackgroundResource {
private final ConversationsSettings settings;
private final ConversationsStub stub;
/** Constructs an instance of ConversationsClient with default settings. */
public static final ConversationsClient create() throws IOException {
return create(ConversationsSettings.newBuilder().build());
}
/**
* Constructs an instance of ConversationsClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final ConversationsClient create(ConversationsSettings settings)
throws IOException {
return new ConversationsClient(settings);
}
/**
* Constructs an instance of ConversationsClient, using the given stub for making calls. This is
* for advanced usage - prefer using create(ConversationsSettings).
*/
public static final ConversationsClient create(ConversationsStub stub) {
return new ConversationsClient(stub);
}
/**
* Constructs an instance of ConversationsClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected ConversationsClient(ConversationsSettings settings) throws IOException {
this.settings = settings;
this.stub = ((ConversationsStubSettings) settings.getStubSettings()).createStub();
}
protected ConversationsClient(ConversationsStub stub) {
this.settings = null;
this.stub = stub;
}
public final ConversationsSettings getSettings() {
return settings;
}
public ConversationsStub getStub() {
return stub;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new conversation. Conversations are auto-completed after 24 hours.
*
*
Conversation Lifecycle: There are two stages during a conversation: Automated Agent Stage
* and Assist Stage.
*
*
For Automated Agent Stage, there will be a dialogflow agent responding to user queries.
*
*
For Assist Stage, there's no dialogflow agent responding to user queries. But we will
* provide suggestions which are generated from conversation.
*
*
If
* [Conversation.conversation_profile][google.cloud.dialogflow.v2beta1.Conversation.conversation_profile]
* is configured for a dialogflow agent, conversation will start from `Automated Agent Stage`,
* otherwise, it will start from `Assist Stage`. And during `Automated Agent Stage`, once an
* [Intent][google.cloud.dialogflow.v2beta1.Intent] with
* [Intent.live_agent_handoff][google.cloud.dialogflow.v2beta1.Intent.live_agent_handoff] is
* triggered, conversation will transfer to Assist Stage.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Conversation conversation = Conversation.newBuilder().build();
* Conversation response = conversationsClient.createConversation(parent, conversation);
* }
* }
*
* @param parent Required. Resource identifier of the project creating the conversation. Format:
* `projects/<Project ID>/locations/<Location ID>`.
* @param conversation Required. The conversation to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation createConversation(LocationName parent, Conversation conversation) {
CreateConversationRequest request =
CreateConversationRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setConversation(conversation)
.build();
return createConversation(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new conversation. Conversations are auto-completed after 24 hours.
*
* Conversation Lifecycle: There are two stages during a conversation: Automated Agent Stage
* and Assist Stage.
*
*
For Automated Agent Stage, there will be a dialogflow agent responding to user queries.
*
*
For Assist Stage, there's no dialogflow agent responding to user queries. But we will
* provide suggestions which are generated from conversation.
*
*
If
* [Conversation.conversation_profile][google.cloud.dialogflow.v2beta1.Conversation.conversation_profile]
* is configured for a dialogflow agent, conversation will start from `Automated Agent Stage`,
* otherwise, it will start from `Assist Stage`. And during `Automated Agent Stage`, once an
* [Intent][google.cloud.dialogflow.v2beta1.Intent] with
* [Intent.live_agent_handoff][google.cloud.dialogflow.v2beta1.Intent.live_agent_handoff] is
* triggered, conversation will transfer to Assist Stage.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ProjectName parent = ProjectName.of("[PROJECT]");
* Conversation conversation = Conversation.newBuilder().build();
* Conversation response = conversationsClient.createConversation(parent, conversation);
* }
* }
*
* @param parent Required. Resource identifier of the project creating the conversation. Format:
* `projects/<Project ID>/locations/<Location ID>`.
* @param conversation Required. The conversation to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation createConversation(ProjectName parent, Conversation conversation) {
CreateConversationRequest request =
CreateConversationRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setConversation(conversation)
.build();
return createConversation(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new conversation. Conversations are auto-completed after 24 hours.
*
* Conversation Lifecycle: There are two stages during a conversation: Automated Agent Stage
* and Assist Stage.
*
*
For Automated Agent Stage, there will be a dialogflow agent responding to user queries.
*
*
For Assist Stage, there's no dialogflow agent responding to user queries. But we will
* provide suggestions which are generated from conversation.
*
*
If
* [Conversation.conversation_profile][google.cloud.dialogflow.v2beta1.Conversation.conversation_profile]
* is configured for a dialogflow agent, conversation will start from `Automated Agent Stage`,
* otherwise, it will start from `Assist Stage`. And during `Automated Agent Stage`, once an
* [Intent][google.cloud.dialogflow.v2beta1.Intent] with
* [Intent.live_agent_handoff][google.cloud.dialogflow.v2beta1.Intent.live_agent_handoff] is
* triggered, conversation will transfer to Assist Stage.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* String parent = ProjectName.of("[PROJECT]").toString();
* Conversation conversation = Conversation.newBuilder().build();
* Conversation response = conversationsClient.createConversation(parent, conversation);
* }
* }
*
* @param parent Required. Resource identifier of the project creating the conversation. Format:
* `projects/<Project ID>/locations/<Location ID>`.
* @param conversation Required. The conversation to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation createConversation(String parent, Conversation conversation) {
CreateConversationRequest request =
CreateConversationRequest.newBuilder()
.setParent(parent)
.setConversation(conversation)
.build();
return createConversation(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new conversation. Conversations are auto-completed after 24 hours.
*
* Conversation Lifecycle: There are two stages during a conversation: Automated Agent Stage
* and Assist Stage.
*
*
For Automated Agent Stage, there will be a dialogflow agent responding to user queries.
*
*
For Assist Stage, there's no dialogflow agent responding to user queries. But we will
* provide suggestions which are generated from conversation.
*
*
If
* [Conversation.conversation_profile][google.cloud.dialogflow.v2beta1.Conversation.conversation_profile]
* is configured for a dialogflow agent, conversation will start from `Automated Agent Stage`,
* otherwise, it will start from `Assist Stage`. And during `Automated Agent Stage`, once an
* [Intent][google.cloud.dialogflow.v2beta1.Intent] with
* [Intent.live_agent_handoff][google.cloud.dialogflow.v2beta1.Intent.live_agent_handoff] is
* triggered, conversation will transfer to Assist Stage.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* CreateConversationRequest request =
* CreateConversationRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setConversation(Conversation.newBuilder().build())
* .setConversationId("conversationId-1676095234")
* .build();
* Conversation response = conversationsClient.createConversation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation createConversation(CreateConversationRequest request) {
return createConversationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new conversation. Conversations are auto-completed after 24 hours.
*
* Conversation Lifecycle: There are two stages during a conversation: Automated Agent Stage
* and Assist Stage.
*
*
For Automated Agent Stage, there will be a dialogflow agent responding to user queries.
*
*
For Assist Stage, there's no dialogflow agent responding to user queries. But we will
* provide suggestions which are generated from conversation.
*
*
If
* [Conversation.conversation_profile][google.cloud.dialogflow.v2beta1.Conversation.conversation_profile]
* is configured for a dialogflow agent, conversation will start from `Automated Agent Stage`,
* otherwise, it will start from `Assist Stage`. And during `Automated Agent Stage`, once an
* [Intent][google.cloud.dialogflow.v2beta1.Intent] with
* [Intent.live_agent_handoff][google.cloud.dialogflow.v2beta1.Intent.live_agent_handoff] is
* triggered, conversation will transfer to Assist Stage.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* CreateConversationRequest request =
* CreateConversationRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setConversation(Conversation.newBuilder().build())
* .setConversationId("conversationId-1676095234")
* .build();
* ApiFuture future =
* conversationsClient.createConversationCallable().futureCall(request);
* // Do something.
* Conversation response = future.get();
* }
* }
*/
public final UnaryCallable createConversationCallable() {
return stub.createConversationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the list of all conversations in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Conversation element : conversationsClient.listConversations(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The project from which to list all conversation. Format:
* `projects/<Project ID>/locations/<Location ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListConversationsPagedResponse listConversations(LocationName parent) {
ListConversationsRequest request =
ListConversationsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listConversations(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the list of all conversations in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ProjectName parent = ProjectName.of("[PROJECT]");
* for (Conversation element : conversationsClient.listConversations(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The project from which to list all conversation. Format:
* `projects/<Project ID>/locations/<Location ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListConversationsPagedResponse listConversations(ProjectName parent) {
ListConversationsRequest request =
ListConversationsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listConversations(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the list of all conversations in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* String parent = ProjectName.of("[PROJECT]").toString();
* for (Conversation element : conversationsClient.listConversations(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The project from which to list all conversation. Format:
* `projects/<Project ID>/locations/<Location ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListConversationsPagedResponse listConversations(String parent) {
ListConversationsRequest request =
ListConversationsRequest.newBuilder().setParent(parent).build();
return listConversations(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the list of all conversations in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ListConversationsRequest request =
* ListConversationsRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* for (Conversation element : conversationsClient.listConversations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListConversationsPagedResponse listConversations(ListConversationsRequest request) {
return listConversationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the list of all conversations in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ListConversationsRequest request =
* ListConversationsRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* ApiFuture future =
* conversationsClient.listConversationsPagedCallable().futureCall(request);
* // Do something.
* for (Conversation element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listConversationsPagedCallable() {
return stub.listConversationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the list of all conversations in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ListConversationsRequest request =
* ListConversationsRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* while (true) {
* ListConversationsResponse response =
* conversationsClient.listConversationsCallable().call(request);
* for (Conversation element : response.getConversationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listConversationsCallable() {
return stub.listConversationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the specific conversation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ConversationName name =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]");
* Conversation response = conversationsClient.getConversation(name);
* }
* }
*
* @param name Required. The name of the conversation. Format: `projects/<Project
* ID>/locations/<Location ID>/conversations/<Conversation ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation getConversation(ConversationName name) {
GetConversationRequest request =
GetConversationRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getConversation(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the specific conversation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* String name =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]").toString();
* Conversation response = conversationsClient.getConversation(name);
* }
* }
*
* @param name Required. The name of the conversation. Format: `projects/<Project
* ID>/locations/<Location ID>/conversations/<Conversation ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation getConversation(String name) {
GetConversationRequest request = GetConversationRequest.newBuilder().setName(name).build();
return getConversation(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the specific conversation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* GetConversationRequest request =
* GetConversationRequest.newBuilder()
* .setName(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .build();
* Conversation response = conversationsClient.getConversation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation getConversation(GetConversationRequest request) {
return getConversationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the specific conversation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* GetConversationRequest request =
* GetConversationRequest.newBuilder()
* .setName(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .build();
* ApiFuture future =
* conversationsClient.getConversationCallable().futureCall(request);
* // Do something.
* Conversation response = future.get();
* }
* }
*/
public final UnaryCallable getConversationCallable() {
return stub.getConversationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Completes the specified conversation. Finished conversations are purged from the database after
* 30 days.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ConversationName name =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]");
* Conversation response = conversationsClient.completeConversation(name);
* }
* }
*
* @param name Required. Resource identifier of the conversation to close. Format:
* `projects/<Project ID>/locations/<Location ID>/conversations/<Conversation
* ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation completeConversation(ConversationName name) {
CompleteConversationRequest request =
CompleteConversationRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return completeConversation(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Completes the specified conversation. Finished conversations are purged from the database after
* 30 days.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* String name =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]").toString();
* Conversation response = conversationsClient.completeConversation(name);
* }
* }
*
* @param name Required. Resource identifier of the conversation to close. Format:
* `projects/<Project ID>/locations/<Location ID>/conversations/<Conversation
* ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation completeConversation(String name) {
CompleteConversationRequest request =
CompleteConversationRequest.newBuilder().setName(name).build();
return completeConversation(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Completes the specified conversation. Finished conversations are purged from the database after
* 30 days.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* CompleteConversationRequest request =
* CompleteConversationRequest.newBuilder()
* .setName(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .build();
* Conversation response = conversationsClient.completeConversation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Conversation completeConversation(CompleteConversationRequest request) {
return completeConversationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Completes the specified conversation. Finished conversations are purged from the database after
* 30 days.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* CompleteConversationRequest request =
* CompleteConversationRequest.newBuilder()
* .setName(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .build();
* ApiFuture future =
* conversationsClient.completeConversationCallable().futureCall(request);
* // Do something.
* Conversation response = future.get();
* }
* }
*/
public final UnaryCallable
completeConversationCallable() {
return stub.completeConversationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Batch ingests messages to conversation. Customers can use this RPC to ingest historical
* messages to conversation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ConversationName parent =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]");
* BatchCreateMessagesResponse response = conversationsClient.batchCreateMessages(parent);
* }
* }
*
* @param parent Required. Resource identifier of the conversation to create message. Format:
* `projects/<Project ID>/locations/<Location ID>/conversations/<Conversation
* ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BatchCreateMessagesResponse batchCreateMessages(ConversationName parent) {
BatchCreateMessagesRequest request =
BatchCreateMessagesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return batchCreateMessages(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Batch ingests messages to conversation. Customers can use this RPC to ingest historical
* messages to conversation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* String parent =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]").toString();
* BatchCreateMessagesResponse response = conversationsClient.batchCreateMessages(parent);
* }
* }
*
* @param parent Required. Resource identifier of the conversation to create message. Format:
* `projects/<Project ID>/locations/<Location ID>/conversations/<Conversation
* ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BatchCreateMessagesResponse batchCreateMessages(String parent) {
BatchCreateMessagesRequest request =
BatchCreateMessagesRequest.newBuilder().setParent(parent).build();
return batchCreateMessages(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Batch ingests messages to conversation. Customers can use this RPC to ingest historical
* messages to conversation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* BatchCreateMessagesRequest request =
* BatchCreateMessagesRequest.newBuilder()
* .setParent(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .addAllRequests(new ArrayList())
* .build();
* BatchCreateMessagesResponse response = conversationsClient.batchCreateMessages(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BatchCreateMessagesResponse batchCreateMessages(BatchCreateMessagesRequest request) {
return batchCreateMessagesCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Batch ingests messages to conversation. Customers can use this RPC to ingest historical
* messages to conversation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* BatchCreateMessagesRequest request =
* BatchCreateMessagesRequest.newBuilder()
* .setParent(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .addAllRequests(new ArrayList())
* .build();
* ApiFuture future =
* conversationsClient.batchCreateMessagesCallable().futureCall(request);
* // Do something.
* BatchCreateMessagesResponse response = future.get();
* }
* }
*/
public final UnaryCallable
batchCreateMessagesCallable() {
return stub.batchCreateMessagesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists messages that belong to a given conversation. `messages` are ordered by `create_time` in
* descending order. To fetch updates without duplication, send request with filter
* `create_time_epoch_microseconds > [first item's create_time of previous request]` and empty
* page_token.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ConversationName parent =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]");
* for (Message element : conversationsClient.listMessages(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the conversation to list messages for. Format:
* `projects/<Project ID>/locations/<Location ID>/conversations/<Conversation
* ID>`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListMessagesPagedResponse listMessages(ConversationName parent) {
ListMessagesRequest request =
ListMessagesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listMessages(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists messages that belong to a given conversation. `messages` are ordered by `create_time` in
* descending order. To fetch updates without duplication, send request with filter
* `create_time_epoch_microseconds > [first item's create_time of previous request]` and empty
* page_token.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* String parent =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]").toString();
* for (Message element : conversationsClient.listMessages(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the conversation to list messages for. Format:
* `projects/<Project ID>/locations/<Location ID>/conversations/<Conversation
* ID>`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListMessagesPagedResponse listMessages(String parent) {
ListMessagesRequest request = ListMessagesRequest.newBuilder().setParent(parent).build();
return listMessages(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists messages that belong to a given conversation. `messages` are ordered by `create_time` in
* descending order. To fetch updates without duplication, send request with filter
* `create_time_epoch_microseconds > [first item's create_time of previous request]` and empty
* page_token.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ListMessagesRequest request =
* ListMessagesRequest.newBuilder()
* .setParent(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Message element : conversationsClient.listMessages(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListMessagesPagedResponse listMessages(ListMessagesRequest request) {
return listMessagesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists messages that belong to a given conversation. `messages` are ordered by `create_time` in
* descending order. To fetch updates without duplication, send request with filter
* `create_time_epoch_microseconds > [first item's create_time of previous request]` and empty
* page_token.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ListMessagesRequest request =
* ListMessagesRequest.newBuilder()
* .setParent(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* conversationsClient.listMessagesPagedCallable().futureCall(request);
* // Do something.
* for (Message element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listMessagesPagedCallable() {
return stub.listMessagesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists messages that belong to a given conversation. `messages` are ordered by `create_time` in
* descending order. To fetch updates without duplication, send request with filter
* `create_time_epoch_microseconds > [first item's create_time of previous request]` and empty
* page_token.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ListMessagesRequest request =
* ListMessagesRequest.newBuilder()
* .setParent(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListMessagesResponse response = conversationsClient.listMessagesCallable().call(request);
* for (Message element : response.getMessagesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listMessagesCallable() {
return stub.listMessagesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Suggest summary for a conversation based on specific historical messages. The range of the
* messages to be used for summary can be specified in the request.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ConversationName conversation =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]");
* SuggestConversationSummaryResponse response =
* conversationsClient.suggestConversationSummary(conversation);
* }
* }
*
* @param conversation Required. The conversation to fetch suggestion for. Format:
* `projects/<Project ID>/locations/<Location ID>/conversations/<Conversation
* ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SuggestConversationSummaryResponse suggestConversationSummary(
ConversationName conversation) {
SuggestConversationSummaryRequest request =
SuggestConversationSummaryRequest.newBuilder()
.setConversation(conversation == null ? null : conversation.toString())
.build();
return suggestConversationSummary(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Suggest summary for a conversation based on specific historical messages. The range of the
* messages to be used for summary can be specified in the request.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* String conversation =
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]").toString();
* SuggestConversationSummaryResponse response =
* conversationsClient.suggestConversationSummary(conversation);
* }
* }
*
* @param conversation Required. The conversation to fetch suggestion for. Format:
* `projects/<Project ID>/locations/<Location ID>/conversations/<Conversation
* ID>`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SuggestConversationSummaryResponse suggestConversationSummary(String conversation) {
SuggestConversationSummaryRequest request =
SuggestConversationSummaryRequest.newBuilder().setConversation(conversation).build();
return suggestConversationSummary(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Suggest summary for a conversation based on specific historical messages. The range of the
* messages to be used for summary can be specified in the request.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* SuggestConversationSummaryRequest request =
* SuggestConversationSummaryRequest.newBuilder()
* .setConversation(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .setLatestMessage(
* MessageName.ofProjectConversationMessageName(
* "[PROJECT]", "[CONVERSATION]", "[MESSAGE]")
* .toString())
* .setContextSize(1116903569)
* .setAssistQueryParams(AssistQueryParameters.newBuilder().build())
* .build();
* SuggestConversationSummaryResponse response =
* conversationsClient.suggestConversationSummary(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SuggestConversationSummaryResponse suggestConversationSummary(
SuggestConversationSummaryRequest request) {
return suggestConversationSummaryCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Suggest summary for a conversation based on specific historical messages. The range of the
* messages to be used for summary can be specified in the request.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* SuggestConversationSummaryRequest request =
* SuggestConversationSummaryRequest.newBuilder()
* .setConversation(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .setLatestMessage(
* MessageName.ofProjectConversationMessageName(
* "[PROJECT]", "[CONVERSATION]", "[MESSAGE]")
* .toString())
* .setContextSize(1116903569)
* .setAssistQueryParams(AssistQueryParameters.newBuilder().build())
* .build();
* ApiFuture future =
* conversationsClient.suggestConversationSummaryCallable().futureCall(request);
* // Do something.
* SuggestConversationSummaryResponse response = future.get();
* }
* }
*/
public final UnaryCallable
suggestConversationSummaryCallable() {
return stub.suggestConversationSummaryCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generates and returns a summary for a conversation that does not have a resource created for
* it.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* GenerateStatelessSummaryRequest request =
* GenerateStatelessSummaryRequest.newBuilder()
* .setStatelessConversation(
* GenerateStatelessSummaryRequest.MinimalConversation.newBuilder().build())
* .setConversationProfile(ConversationProfile.newBuilder().build())
* .setLatestMessage(
* MessageName.ofProjectConversationMessageName(
* "[PROJECT]", "[CONVERSATION]", "[MESSAGE]")
* .toString())
* .setMaxContextSize(-1134084212)
* .build();
* GenerateStatelessSummaryResponse response =
* conversationsClient.generateStatelessSummary(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateStatelessSummaryResponse generateStatelessSummary(
GenerateStatelessSummaryRequest request) {
return generateStatelessSummaryCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generates and returns a summary for a conversation that does not have a resource created for
* it.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* GenerateStatelessSummaryRequest request =
* GenerateStatelessSummaryRequest.newBuilder()
* .setStatelessConversation(
* GenerateStatelessSummaryRequest.MinimalConversation.newBuilder().build())
* .setConversationProfile(ConversationProfile.newBuilder().build())
* .setLatestMessage(
* MessageName.ofProjectConversationMessageName(
* "[PROJECT]", "[CONVERSATION]", "[MESSAGE]")
* .toString())
* .setMaxContextSize(-1134084212)
* .build();
* ApiFuture future =
* conversationsClient.generateStatelessSummaryCallable().futureCall(request);
* // Do something.
* GenerateStatelessSummaryResponse response = future.get();
* }
* }
*/
public final UnaryCallable
generateStatelessSummaryCallable() {
return stub.generateStatelessSummaryCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get answers for the given query based on knowledge documents.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* SearchKnowledgeRequest request =
* SearchKnowledgeRequest.newBuilder()
* .setParent("parent-995424086")
* .setQuery(TextInput.newBuilder().build())
* .setConversationProfile(
* ConversationProfileName.ofProjectConversationProfileName(
* "[PROJECT]", "[CONVERSATION_PROFILE]")
* .toString())
* .setSessionId("sessionId607796817")
* .setConversation(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .setLatestMessage(
* MessageName.ofProjectConversationMessageName(
* "[PROJECT]", "[CONVERSATION]", "[MESSAGE]")
* .toString())
* .build();
* SearchKnowledgeResponse response = conversationsClient.searchKnowledge(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SearchKnowledgeResponse searchKnowledge(SearchKnowledgeRequest request) {
return searchKnowledgeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get answers for the given query based on knowledge documents.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* SearchKnowledgeRequest request =
* SearchKnowledgeRequest.newBuilder()
* .setParent("parent-995424086")
* .setQuery(TextInput.newBuilder().build())
* .setConversationProfile(
* ConversationProfileName.ofProjectConversationProfileName(
* "[PROJECT]", "[CONVERSATION_PROFILE]")
* .toString())
* .setSessionId("sessionId607796817")
* .setConversation(
* ConversationName.ofProjectConversationName("[PROJECT]", "[CONVERSATION]")
* .toString())
* .setLatestMessage(
* MessageName.ofProjectConversationMessageName(
* "[PROJECT]", "[CONVERSATION]", "[MESSAGE]")
* .toString())
* .build();
* ApiFuture future =
* conversationsClient.searchKnowledgeCallable().futureCall(request);
* // Do something.
* SearchKnowledgeResponse response = future.get();
* }
* }
*/
public final UnaryCallable
searchKnowledgeCallable() {
return stub.searchKnowledgeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : conversationsClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* conversationsClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response = conversationsClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = conversationsClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ConversationsClient conversationsClient = ConversationsClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = conversationsClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListConversationsPagedResponse
extends AbstractPagedListResponse<
ListConversationsRequest,
ListConversationsResponse,
Conversation,
ListConversationsPage,
ListConversationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListConversationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListConversationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListConversationsPagedResponse(ListConversationsPage page) {
super(page, ListConversationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListConversationsPage
extends AbstractPage<
ListConversationsRequest,
ListConversationsResponse,
Conversation,
ListConversationsPage> {
private ListConversationsPage(
PageContext context,
ListConversationsResponse response) {
super(context, response);
}
private static ListConversationsPage createEmptyPage() {
return new ListConversationsPage(null, null);
}
@Override
protected ListConversationsPage createPage(
PageContext context,
ListConversationsResponse response) {
return new ListConversationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListConversationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListConversationsRequest,
ListConversationsResponse,
Conversation,
ListConversationsPage,
ListConversationsFixedSizeCollection> {
private ListConversationsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListConversationsFixedSizeCollection createEmptyCollection() {
return new ListConversationsFixedSizeCollection(null, 0);
}
@Override
protected ListConversationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListConversationsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListMessagesPagedResponse
extends AbstractPagedListResponse<
ListMessagesRequest,
ListMessagesResponse,
Message,
ListMessagesPage,
ListMessagesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListMessagesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListMessagesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListMessagesPagedResponse(ListMessagesPage page) {
super(page, ListMessagesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListMessagesPage
extends AbstractPage {
private ListMessagesPage(
PageContext context,
ListMessagesResponse response) {
super(context, response);
}
private static ListMessagesPage createEmptyPage() {
return new ListMessagesPage(null, null);
}
@Override
protected ListMessagesPage createPage(
PageContext context,
ListMessagesResponse response) {
return new ListMessagesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListMessagesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListMessagesRequest,
ListMessagesResponse,
Message,
ListMessagesPage,
ListMessagesFixedSizeCollection> {
private ListMessagesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListMessagesFixedSizeCollection createEmptyCollection() {
return new ListMessagesFixedSizeCollection(null, 0);
}
@Override
protected ListMessagesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListMessagesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}