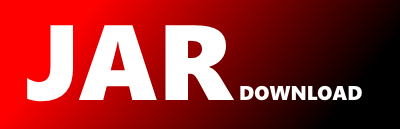
com.google.cloud.discoveryengine.v1beta.package-info Maven / Gradle / Ivy
/*
* Copyright 2023 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* A client to Discovery Engine API
*
* The interfaces provided are listed below, along with usage samples.
*
*
======================= CompletionServiceClient =======================
*
*
Service Description: Service for Auto-Completion.
*
*
Sample for CompletionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CompletionServiceClient completionServiceClient = CompletionServiceClient.create()) {
* CompleteQueryRequest request =
* CompleteQueryRequest.newBuilder()
* .setDataStore(
* DataStoreName.ofProjectLocationDataStoreName(
* "[PROJECT]", "[LOCATION]", "[DATA_STORE]")
* .toString())
* .setQuery("query107944136")
* .setQueryModel("queryModel-184930495")
* .setUserPseudoId("userPseudoId-1155274652")
* .build();
* CompleteQueryResponse response = completionServiceClient.completeQuery(request);
* }
* }
*
* ======================= DocumentServiceClient =======================
*
*
Service Description: Service for ingesting
* [Document][google.cloud.discoveryengine.v1beta.Document] information of the customer's website.
*
*
Sample for DocumentServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (DocumentServiceClient documentServiceClient = DocumentServiceClient.create()) {
* DocumentName name =
* DocumentName.ofProjectLocationDataStoreBranchDocumentName(
* "[PROJECT]", "[LOCATION]", "[DATA_STORE]", "[BRANCH]", "[DOCUMENT]");
* Document response = documentServiceClient.getDocument(name);
* }
* }
*
* ======================= RecommendationServiceClient =======================
*
*
Service Description: Service for making recommendations.
*
*
Sample for RecommendationServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RecommendationServiceClient recommendationServiceClient =
* RecommendationServiceClient.create()) {
* RecommendRequest request =
* RecommendRequest.newBuilder()
* .setServingConfig(
* ServingConfigName.ofProjectLocationDataStoreServingConfigName(
* "[PROJECT]", "[LOCATION]", "[DATA_STORE]", "[SERVING_CONFIG]")
* .toString())
* .setUserEvent(UserEvent.newBuilder().build())
* .setPageSize(883849137)
* .setFilter("filter-1274492040")
* .setValidateOnly(true)
* .putAllParams(new HashMap())
* .putAllUserLabels(new HashMap())
* .build();
* RecommendResponse response = recommendationServiceClient.recommend(request);
* }
* }
*
* ======================= SchemaServiceClient =======================
*
*
Service Description: Service for managing
* [Schema][google.cloud.discoveryengine.v1beta.Schema]s.
*
*
Sample for SchemaServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (SchemaServiceClient schemaServiceClient = SchemaServiceClient.create()) {
* SchemaName name =
* SchemaName.ofProjectLocationDataStoreSchemaName(
* "[PROJECT]", "[LOCATION]", "[DATA_STORE]", "[SCHEMA]");
* Schema response = schemaServiceClient.getSchema(name);
* }
* }
*
* ======================= SearchServiceClient =======================
*
*
Service Description: Service for search.
*
*
Sample for SearchServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (SearchServiceClient searchServiceClient = SearchServiceClient.create()) {
* SearchRequest request =
* SearchRequest.newBuilder()
* .setServingConfig(
* ServingConfigName.ofProjectLocationDataStoreServingConfigName(
* "[PROJECT]", "[LOCATION]", "[DATA_STORE]", "[SERVING_CONFIG]")
* .toString())
* .setBranch(
* BranchName.ofProjectLocationDataStoreBranchName(
* "[PROJECT]", "[LOCATION]", "[DATA_STORE]", "[BRANCH]")
* .toString())
* .setQuery("query107944136")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setOffset(-1019779949)
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .setUserInfo(UserInfo.newBuilder().build())
* .addAllFacetSpecs(new ArrayList())
* .setBoostSpec(SearchRequest.BoostSpec.newBuilder().build())
* .putAllParams(new HashMap())
* .setQueryExpansionSpec(SearchRequest.QueryExpansionSpec.newBuilder().build())
* .setSpellCorrectionSpec(SearchRequest.SpellCorrectionSpec.newBuilder().build())
* .setUserPseudoId("userPseudoId-1155274652")
* .setContentSearchSpec(SearchRequest.ContentSearchSpec.newBuilder().build())
* .setSafeSearch(true)
* .putAllUserLabels(new HashMap())
* .build();
* for (SearchResponse.SearchResult element : searchServiceClient.search(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* ======================= UserEventServiceClient =======================
*
*
Service Description: Service for ingesting end user actions on a website to Discovery Engine
* API.
*
*
Sample for UserEventServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (UserEventServiceClient userEventServiceClient = UserEventServiceClient.create()) {
* WriteUserEventRequest request =
* WriteUserEventRequest.newBuilder()
* .setParent(
* DataStoreName.ofProjectLocationDataStoreName(
* "[PROJECT]", "[LOCATION]", "[DATA_STORE]")
* .toString())
* .setUserEvent(UserEvent.newBuilder().build())
* .build();
* UserEvent response = userEventServiceClient.writeUserEvent(request);
* }
* }
*/
@Generated("by gapic-generator-java")
package com.google.cloud.discoveryengine.v1beta;
import javax.annotation.Generated;