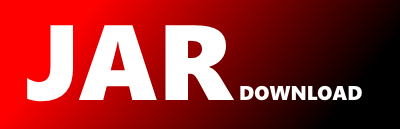
com.google.cloud.clouddms.v1.stub.DataMigrationServiceStub Maven / Gradle / Ivy
/*
* Copyright 2022 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.clouddms.v1.stub;
import static com.google.cloud.clouddms.v1.DataMigrationServiceClient.ListConnectionProfilesPagedResponse;
import static com.google.cloud.clouddms.v1.DataMigrationServiceClient.ListMigrationJobsPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.clouddms.v1.ConnectionProfile;
import com.google.cloud.clouddms.v1.CreateConnectionProfileRequest;
import com.google.cloud.clouddms.v1.CreateMigrationJobRequest;
import com.google.cloud.clouddms.v1.DeleteConnectionProfileRequest;
import com.google.cloud.clouddms.v1.DeleteMigrationJobRequest;
import com.google.cloud.clouddms.v1.GenerateSshScriptRequest;
import com.google.cloud.clouddms.v1.GetConnectionProfileRequest;
import com.google.cloud.clouddms.v1.GetMigrationJobRequest;
import com.google.cloud.clouddms.v1.ListConnectionProfilesRequest;
import com.google.cloud.clouddms.v1.ListConnectionProfilesResponse;
import com.google.cloud.clouddms.v1.ListMigrationJobsRequest;
import com.google.cloud.clouddms.v1.ListMigrationJobsResponse;
import com.google.cloud.clouddms.v1.MigrationJob;
import com.google.cloud.clouddms.v1.OperationMetadata;
import com.google.cloud.clouddms.v1.PromoteMigrationJobRequest;
import com.google.cloud.clouddms.v1.RestartMigrationJobRequest;
import com.google.cloud.clouddms.v1.ResumeMigrationJobRequest;
import com.google.cloud.clouddms.v1.SshScript;
import com.google.cloud.clouddms.v1.StartMigrationJobRequest;
import com.google.cloud.clouddms.v1.StopMigrationJobRequest;
import com.google.cloud.clouddms.v1.UpdateConnectionProfileRequest;
import com.google.cloud.clouddms.v1.UpdateMigrationJobRequest;
import com.google.cloud.clouddms.v1.VerifyMigrationJobRequest;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.OperationsStub;
import com.google.protobuf.Empty;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Base stub class for the DataMigrationService service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public abstract class DataMigrationServiceStub implements BackgroundResource {
public OperationsStub getOperationsStub() {
throw new UnsupportedOperationException("Not implemented: getOperationsStub()");
}
public UnaryCallable
listMigrationJobsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listMigrationJobsPagedCallable()");
}
public UnaryCallable
listMigrationJobsCallable() {
throw new UnsupportedOperationException("Not implemented: listMigrationJobsCallable()");
}
public UnaryCallable getMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: getMigrationJobCallable()");
}
public OperationCallable
createMigrationJobOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: createMigrationJobOperationCallable()");
}
public UnaryCallable createMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: createMigrationJobCallable()");
}
public OperationCallable
updateMigrationJobOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: updateMigrationJobOperationCallable()");
}
public UnaryCallable updateMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: updateMigrationJobCallable()");
}
public OperationCallable
deleteMigrationJobOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: deleteMigrationJobOperationCallable()");
}
public UnaryCallable deleteMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: deleteMigrationJobCallable()");
}
public OperationCallable
startMigrationJobOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: startMigrationJobOperationCallable()");
}
public UnaryCallable startMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: startMigrationJobCallable()");
}
public OperationCallable
stopMigrationJobOperationCallable() {
throw new UnsupportedOperationException("Not implemented: stopMigrationJobOperationCallable()");
}
public UnaryCallable stopMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: stopMigrationJobCallable()");
}
public OperationCallable
resumeMigrationJobOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: resumeMigrationJobOperationCallable()");
}
public UnaryCallable resumeMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: resumeMigrationJobCallable()");
}
public OperationCallable
promoteMigrationJobOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: promoteMigrationJobOperationCallable()");
}
public UnaryCallable promoteMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: promoteMigrationJobCallable()");
}
public OperationCallable
verifyMigrationJobOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: verifyMigrationJobOperationCallable()");
}
public UnaryCallable verifyMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: verifyMigrationJobCallable()");
}
public OperationCallable
restartMigrationJobOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: restartMigrationJobOperationCallable()");
}
public UnaryCallable restartMigrationJobCallable() {
throw new UnsupportedOperationException("Not implemented: restartMigrationJobCallable()");
}
public UnaryCallable generateSshScriptCallable() {
throw new UnsupportedOperationException("Not implemented: generateSshScriptCallable()");
}
public UnaryCallable
listConnectionProfilesPagedCallable() {
throw new UnsupportedOperationException(
"Not implemented: listConnectionProfilesPagedCallable()");
}
public UnaryCallable
listConnectionProfilesCallable() {
throw new UnsupportedOperationException("Not implemented: listConnectionProfilesCallable()");
}
public UnaryCallable
getConnectionProfileCallable() {
throw new UnsupportedOperationException("Not implemented: getConnectionProfileCallable()");
}
public OperationCallable
createConnectionProfileOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: createConnectionProfileOperationCallable()");
}
public UnaryCallable
createConnectionProfileCallable() {
throw new UnsupportedOperationException("Not implemented: createConnectionProfileCallable()");
}
public OperationCallable
updateConnectionProfileOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: updateConnectionProfileOperationCallable()");
}
public UnaryCallable
updateConnectionProfileCallable() {
throw new UnsupportedOperationException("Not implemented: updateConnectionProfileCallable()");
}
public OperationCallable
deleteConnectionProfileOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: deleteConnectionProfileOperationCallable()");
}
public UnaryCallable
deleteConnectionProfileCallable() {
throw new UnsupportedOperationException("Not implemented: deleteConnectionProfileCallable()");
}
@Override
public abstract void close();
}