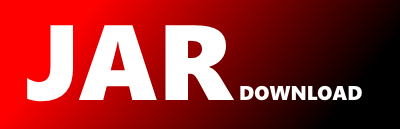
com.google.cloud.firestore.v1.FirestoreAdminClient Maven / Gradle / Ivy
Show all versions of google-cloud-firestore-admin Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.firestore.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.firestore.v1.stub.FirestoreAdminStub;
import com.google.cloud.firestore.v1.stub.FirestoreAdminStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.firestore.admin.v1.Backup;
import com.google.firestore.admin.v1.BackupName;
import com.google.firestore.admin.v1.BackupSchedule;
import com.google.firestore.admin.v1.BackupScheduleName;
import com.google.firestore.admin.v1.BulkDeleteDocumentsMetadata;
import com.google.firestore.admin.v1.BulkDeleteDocumentsRequest;
import com.google.firestore.admin.v1.BulkDeleteDocumentsResponse;
import com.google.firestore.admin.v1.CollectionGroupName;
import com.google.firestore.admin.v1.CreateBackupScheduleRequest;
import com.google.firestore.admin.v1.CreateDatabaseMetadata;
import com.google.firestore.admin.v1.CreateDatabaseRequest;
import com.google.firestore.admin.v1.CreateIndexRequest;
import com.google.firestore.admin.v1.Database;
import com.google.firestore.admin.v1.DatabaseName;
import com.google.firestore.admin.v1.DeleteBackupRequest;
import com.google.firestore.admin.v1.DeleteBackupScheduleRequest;
import com.google.firestore.admin.v1.DeleteDatabaseMetadata;
import com.google.firestore.admin.v1.DeleteDatabaseRequest;
import com.google.firestore.admin.v1.DeleteIndexRequest;
import com.google.firestore.admin.v1.ExportDocumentsMetadata;
import com.google.firestore.admin.v1.ExportDocumentsRequest;
import com.google.firestore.admin.v1.ExportDocumentsResponse;
import com.google.firestore.admin.v1.Field;
import com.google.firestore.admin.v1.FieldName;
import com.google.firestore.admin.v1.FieldOperationMetadata;
import com.google.firestore.admin.v1.GetBackupRequest;
import com.google.firestore.admin.v1.GetBackupScheduleRequest;
import com.google.firestore.admin.v1.GetDatabaseRequest;
import com.google.firestore.admin.v1.GetFieldRequest;
import com.google.firestore.admin.v1.GetIndexRequest;
import com.google.firestore.admin.v1.ImportDocumentsMetadata;
import com.google.firestore.admin.v1.ImportDocumentsRequest;
import com.google.firestore.admin.v1.Index;
import com.google.firestore.admin.v1.IndexName;
import com.google.firestore.admin.v1.IndexOperationMetadata;
import com.google.firestore.admin.v1.ListBackupSchedulesRequest;
import com.google.firestore.admin.v1.ListBackupSchedulesResponse;
import com.google.firestore.admin.v1.ListBackupsRequest;
import com.google.firestore.admin.v1.ListBackupsResponse;
import com.google.firestore.admin.v1.ListDatabasesRequest;
import com.google.firestore.admin.v1.ListDatabasesResponse;
import com.google.firestore.admin.v1.ListFieldsRequest;
import com.google.firestore.admin.v1.ListFieldsResponse;
import com.google.firestore.admin.v1.ListIndexesRequest;
import com.google.firestore.admin.v1.ListIndexesResponse;
import com.google.firestore.admin.v1.LocationName;
import com.google.firestore.admin.v1.ProjectName;
import com.google.firestore.admin.v1.RestoreDatabaseMetadata;
import com.google.firestore.admin.v1.RestoreDatabaseRequest;
import com.google.firestore.admin.v1.UpdateBackupScheduleRequest;
import com.google.firestore.admin.v1.UpdateDatabaseMetadata;
import com.google.firestore.admin.v1.UpdateDatabaseRequest;
import com.google.firestore.admin.v1.UpdateFieldRequest;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: The Cloud Firestore Admin API.
*
* This API provides several administrative services for Cloud Firestore.
*
*
Project, Database, Namespace, Collection, Collection Group, and Document are used as defined
* in the Google Cloud Firestore API.
*
*
Operation: An Operation represents work being performed in the background.
*
*
The index service manages Cloud Firestore indexes.
*
*
Index creation is performed asynchronously. An Operation resource is created for each such
* asynchronous operation. The state of the operation (including any errors encountered) may be
* queried via the Operation resource.
*
*
The Operations collection provides a record of actions performed for the specified Project
* (including any Operations in progress). Operations are not created directly but through calls on
* other collections or resources.
*
*
An Operation that is done may be deleted so that it is no longer listed as part of the
* Operation collection. Operations are garbage collected after 30 days. By default, ListOperations
* will only return in progress and failed operations. To list completed operation, issue a
* ListOperations request with the filter `done: true`.
*
*
Operations are created by service `FirestoreAdmin`, but are accessed via service
* `google.longrunning.Operations`.
*
*
This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* IndexName name = IndexName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[INDEX]");
* Index response = firestoreAdminClient.getIndex(name);
* }
* }
*
* Note: close() needs to be called on the FirestoreAdminClient object to clean up resources such
* as threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* CreateIndex
* Creates a composite index. This returns a [google.longrunning.Operation][google.longrunning.Operation] which may be used to track the status of the creation. The metadata for the operation will be the type [IndexOperationMetadata][google.firestore.admin.v1.IndexOperationMetadata].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createIndexAsync(CreateIndexRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createIndexAsync(CollectionGroupName parent, Index index)
*
createIndexAsync(String parent, Index index)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createIndexOperationCallable()
*
createIndexCallable()
*
*
*
*
* ListIndexes
* Lists composite indexes.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listIndexes(ListIndexesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listIndexes(CollectionGroupName parent)
*
listIndexes(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listIndexesPagedCallable()
*
listIndexesCallable()
*
*
*
*
* GetIndex
* Gets a composite index.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getIndex(GetIndexRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getIndex(IndexName name)
*
getIndex(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getIndexCallable()
*
*
*
*
* DeleteIndex
* Deletes a composite index.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteIndex(DeleteIndexRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteIndex(IndexName name)
*
deleteIndex(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteIndexCallable()
*
*
*
*
* GetField
* Gets the metadata and configuration for a Field.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getField(GetFieldRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getField(FieldName name)
*
getField(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getFieldCallable()
*
*
*
*
* UpdateField
* Updates a field configuration. Currently, field updates apply only to single field index configuration. However, calls to [FirestoreAdmin.UpdateField][google.firestore.admin.v1.FirestoreAdmin.UpdateField] should provide a field mask to avoid changing any configuration that the caller isn't aware of. The field mask should be specified as: `{ paths: "index_config" }`.
*
This call returns a [google.longrunning.Operation][google.longrunning.Operation] which may be used to track the status of the field update. The metadata for the operation will be the type [FieldOperationMetadata][google.firestore.admin.v1.FieldOperationMetadata].
*
To configure the default field settings for the database, use the special `Field` with resource name: `projects/{project_id}/databases/{database_id}/collectionGroups/__default__/fields/*`.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateFieldAsync(UpdateFieldRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateFieldAsync(Field field)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateFieldOperationCallable()
*
updateFieldCallable()
*
*
*
*
* ListFields
* Lists the field configuration and metadata for this database.
*
Currently, [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields] only supports listing fields that have been explicitly overridden. To issue this query, call [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields] with the filter set to `indexConfig.usesAncestorConfig:false` or `ttlConfig:*`.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listFields(ListFieldsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listFields(CollectionGroupName parent)
*
listFields(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listFieldsPagedCallable()
*
listFieldsCallable()
*
*
*
*
* ExportDocuments
* Exports a copy of all or a subset of documents from Google Cloud Firestore to another storage system, such as Google Cloud Storage. Recent updates to documents may not be reflected in the export. The export occurs in the background and its progress can be monitored and managed via the Operation resource that is created. The output of an export may only be used once the associated operation is done. If an export operation is cancelled before completion it may leave partial data behind in Google Cloud Storage.
*
For more details on export behavior and output format, refer to: https://cloud.google.com/firestore/docs/manage-data/export-import
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* exportDocumentsAsync(ExportDocumentsRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* exportDocumentsAsync(DatabaseName name)
*
exportDocumentsAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* exportDocumentsOperationCallable()
*
exportDocumentsCallable()
*
*
*
*
* ImportDocuments
* Imports documents into Google Cloud Firestore. Existing documents with the same name are overwritten. The import occurs in the background and its progress can be monitored and managed via the Operation resource that is created. If an ImportDocuments operation is cancelled, it is possible that a subset of the data has already been imported to Cloud Firestore.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* importDocumentsAsync(ImportDocumentsRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* importDocumentsAsync(DatabaseName name)
*
importDocumentsAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* importDocumentsOperationCallable()
*
importDocumentsCallable()
*
*
*
*
* BulkDeleteDocuments
* Bulk deletes a subset of documents from Google Cloud Firestore. Documents created or updated after the underlying system starts to process the request will not be deleted. The bulk delete occurs in the background and its progress can be monitored and managed via the Operation resource that is created.
*
For more details on bulk delete behavior, refer to: https://cloud.google.com/firestore/docs/manage-data/bulk-delete
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* bulkDeleteDocumentsAsync(BulkDeleteDocumentsRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* bulkDeleteDocumentsAsync(DatabaseName name)
*
bulkDeleteDocumentsAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* bulkDeleteDocumentsOperationCallable()
*
bulkDeleteDocumentsCallable()
*
*
*
*
* CreateDatabase
* Create a database.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createDatabaseAsync(CreateDatabaseRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createDatabaseAsync(ProjectName parent, Database database, String databaseId)
*
createDatabaseAsync(String parent, Database database, String databaseId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createDatabaseOperationCallable()
*
createDatabaseCallable()
*
*
*
*
* GetDatabase
* Gets information about a database.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getDatabase(GetDatabaseRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getDatabase(DatabaseName name)
*
getDatabase(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getDatabaseCallable()
*
*
*
*
* ListDatabases
* List all the databases in the project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listDatabases(ListDatabasesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listDatabases(ProjectName parent)
*
listDatabases(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listDatabasesCallable()
*
*
*
*
* UpdateDatabase
* Updates a database.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateDatabaseAsync(UpdateDatabaseRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateDatabaseAsync(Database database, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateDatabaseOperationCallable()
*
updateDatabaseCallable()
*
*
*
*
* DeleteDatabase
* Deletes a database.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteDatabaseAsync(DeleteDatabaseRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteDatabaseAsync(DatabaseName name)
*
deleteDatabaseAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteDatabaseOperationCallable()
*
deleteDatabaseCallable()
*
*
*
*
* GetBackup
* Gets information about a backup.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getBackup(GetBackupRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getBackup(BackupName name)
*
getBackup(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getBackupCallable()
*
*
*
*
* ListBackups
* Lists all the backups.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listBackups(ListBackupsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listBackups(LocationName parent)
*
listBackups(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listBackupsCallable()
*
*
*
*
* DeleteBackup
* Deletes a backup.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteBackup(DeleteBackupRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteBackup(BackupName name)
*
deleteBackup(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteBackupCallable()
*
*
*
*
* RestoreDatabase
* Creates a new database by restoring from an existing backup.
*
The new database must be in the same cloud region or multi-region location as the existing backup. This behaves similar to [FirestoreAdmin.CreateDatabase][google.firestore.admin.v1.FirestoreAdmin.CreateDatabase] except instead of creating a new empty database, a new database is created with the database type, index configuration, and documents from an existing backup.
*
The [long-running operation][google.longrunning.Operation] can be used to track the progress of the restore, with the Operation's [metadata][google.longrunning.Operation.metadata] field type being the [RestoreDatabaseMetadata][google.firestore.admin.v1.RestoreDatabaseMetadata]. The [response][google.longrunning.Operation.response] type is the [Database][google.firestore.admin.v1.Database] if the restore was successful. The new database is not readable or writeable until the LRO has completed.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* restoreDatabaseAsync(RestoreDatabaseRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* restoreDatabaseOperationCallable()
*
restoreDatabaseCallable()
*
*
*
*
* CreateBackupSchedule
* Creates a backup schedule on a database. At most two backup schedules can be configured on a database, one daily backup schedule and one weekly backup schedule.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createBackupSchedule(CreateBackupScheduleRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createBackupSchedule(DatabaseName parent, BackupSchedule backupSchedule)
*
createBackupSchedule(String parent, BackupSchedule backupSchedule)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createBackupScheduleCallable()
*
*
*
*
* GetBackupSchedule
* Gets information about a backup schedule.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getBackupSchedule(GetBackupScheduleRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getBackupSchedule(BackupScheduleName name)
*
getBackupSchedule(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getBackupScheduleCallable()
*
*
*
*
* ListBackupSchedules
* List backup schedules.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listBackupSchedules(ListBackupSchedulesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listBackupSchedules(DatabaseName parent)
*
listBackupSchedules(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listBackupSchedulesCallable()
*
*
*
*
* UpdateBackupSchedule
* Updates a backup schedule.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateBackupSchedule(UpdateBackupScheduleRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateBackupSchedule(BackupSchedule backupSchedule, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateBackupScheduleCallable()
*
*
*
*
* DeleteBackupSchedule
* Deletes a backup schedule.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteBackupSchedule(DeleteBackupScheduleRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteBackupSchedule(BackupScheduleName name)
*
deleteBackupSchedule(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteBackupScheduleCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of FirestoreAdminSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* FirestoreAdminSettings firestoreAdminSettings =
* FirestoreAdminSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create(firestoreAdminSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* FirestoreAdminSettings firestoreAdminSettings =
* FirestoreAdminSettings.newBuilder().setEndpoint(myEndpoint).build();
* FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create(firestoreAdminSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* FirestoreAdminSettings firestoreAdminSettings =
* FirestoreAdminSettings.newHttpJsonBuilder().build();
* FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create(firestoreAdminSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class FirestoreAdminClient implements BackgroundResource {
private final FirestoreAdminSettings settings;
private final FirestoreAdminStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of FirestoreAdminClient with default settings. */
public static final FirestoreAdminClient create() throws IOException {
return create(FirestoreAdminSettings.newBuilder().build());
}
/**
* Constructs an instance of FirestoreAdminClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final FirestoreAdminClient create(FirestoreAdminSettings settings)
throws IOException {
return new FirestoreAdminClient(settings);
}
/**
* Constructs an instance of FirestoreAdminClient, using the given stub for making calls. This is
* for advanced usage - prefer using create(FirestoreAdminSettings).
*/
public static final FirestoreAdminClient create(FirestoreAdminStub stub) {
return new FirestoreAdminClient(stub);
}
/**
* Constructs an instance of FirestoreAdminClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected FirestoreAdminClient(FirestoreAdminSettings settings) throws IOException {
this.settings = settings;
this.stub = ((FirestoreAdminStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected FirestoreAdminClient(FirestoreAdminStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final FirestoreAdminSettings getSettings() {
return settings;
}
public FirestoreAdminStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a composite index. This returns a
* [google.longrunning.Operation][google.longrunning.Operation] which may be used to track the
* status of the creation. The metadata for the operation will be the type
* [IndexOperationMetadata][google.firestore.admin.v1.IndexOperationMetadata].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CollectionGroupName parent =
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]");
* Index index = Index.newBuilder().build();
* Index response = firestoreAdminClient.createIndexAsync(parent, index).get();
* }
* }
*
* @param parent Required. A parent name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}`
* @param index Required. The composite index to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createIndexAsync(
CollectionGroupName parent, Index index) {
CreateIndexRequest request =
CreateIndexRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setIndex(index)
.build();
return createIndexAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a composite index. This returns a
* [google.longrunning.Operation][google.longrunning.Operation] which may be used to track the
* status of the creation. The metadata for the operation will be the type
* [IndexOperationMetadata][google.firestore.admin.v1.IndexOperationMetadata].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String parent = CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString();
* Index index = Index.newBuilder().build();
* Index response = firestoreAdminClient.createIndexAsync(parent, index).get();
* }
* }
*
* @param parent Required. A parent name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}`
* @param index Required. The composite index to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createIndexAsync(
String parent, Index index) {
CreateIndexRequest request =
CreateIndexRequest.newBuilder().setParent(parent).setIndex(index).build();
return createIndexAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a composite index. This returns a
* [google.longrunning.Operation][google.longrunning.Operation] which may be used to track the
* status of the creation. The metadata for the operation will be the type
* [IndexOperationMetadata][google.firestore.admin.v1.IndexOperationMetadata].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CreateIndexRequest request =
* CreateIndexRequest.newBuilder()
* .setParent(
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString())
* .setIndex(Index.newBuilder().build())
* .build();
* Index response = firestoreAdminClient.createIndexAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createIndexAsync(
CreateIndexRequest request) {
return createIndexOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a composite index. This returns a
* [google.longrunning.Operation][google.longrunning.Operation] which may be used to track the
* status of the creation. The metadata for the operation will be the type
* [IndexOperationMetadata][google.firestore.admin.v1.IndexOperationMetadata].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CreateIndexRequest request =
* CreateIndexRequest.newBuilder()
* .setParent(
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString())
* .setIndex(Index.newBuilder().build())
* .build();
* OperationFuture future =
* firestoreAdminClient.createIndexOperationCallable().futureCall(request);
* // Do something.
* Index response = future.get();
* }
* }
*/
public final OperationCallable
createIndexOperationCallable() {
return stub.createIndexOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a composite index. This returns a
* [google.longrunning.Operation][google.longrunning.Operation] which may be used to track the
* status of the creation. The metadata for the operation will be the type
* [IndexOperationMetadata][google.firestore.admin.v1.IndexOperationMetadata].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CreateIndexRequest request =
* CreateIndexRequest.newBuilder()
* .setParent(
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString())
* .setIndex(Index.newBuilder().build())
* .build();
* ApiFuture future = firestoreAdminClient.createIndexCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createIndexCallable() {
return stub.createIndexCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists composite indexes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CollectionGroupName parent =
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]");
* for (Index element : firestoreAdminClient.listIndexes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. A parent name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListIndexesPagedResponse listIndexes(CollectionGroupName parent) {
ListIndexesRequest request =
ListIndexesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listIndexes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists composite indexes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String parent = CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString();
* for (Index element : firestoreAdminClient.listIndexes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. A parent name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListIndexesPagedResponse listIndexes(String parent) {
ListIndexesRequest request = ListIndexesRequest.newBuilder().setParent(parent).build();
return listIndexes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists composite indexes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListIndexesRequest request =
* ListIndexesRequest.newBuilder()
* .setParent(
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Index element : firestoreAdminClient.listIndexes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListIndexesPagedResponse listIndexes(ListIndexesRequest request) {
return listIndexesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists composite indexes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListIndexesRequest request =
* ListIndexesRequest.newBuilder()
* .setParent(
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future = firestoreAdminClient.listIndexesPagedCallable().futureCall(request);
* // Do something.
* for (Index element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listIndexesPagedCallable() {
return stub.listIndexesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists composite indexes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListIndexesRequest request =
* ListIndexesRequest.newBuilder()
* .setParent(
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListIndexesResponse response = firestoreAdminClient.listIndexesCallable().call(request);
* for (Index element : response.getIndexesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listIndexesCallable() {
return stub.listIndexesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a composite index.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* IndexName name = IndexName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[INDEX]");
* Index response = firestoreAdminClient.getIndex(name);
* }
* }
*
* @param name Required. A name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}/indexes/{index_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Index getIndex(IndexName name) {
GetIndexRequest request =
GetIndexRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getIndex(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a composite index.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = IndexName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[INDEX]").toString();
* Index response = firestoreAdminClient.getIndex(name);
* }
* }
*
* @param name Required. A name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}/indexes/{index_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Index getIndex(String name) {
GetIndexRequest request = GetIndexRequest.newBuilder().setName(name).build();
return getIndex(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a composite index.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetIndexRequest request =
* GetIndexRequest.newBuilder()
* .setName(
* IndexName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[INDEX]").toString())
* .build();
* Index response = firestoreAdminClient.getIndex(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Index getIndex(GetIndexRequest request) {
return getIndexCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a composite index.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetIndexRequest request =
* GetIndexRequest.newBuilder()
* .setName(
* IndexName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[INDEX]").toString())
* .build();
* ApiFuture future = firestoreAdminClient.getIndexCallable().futureCall(request);
* // Do something.
* Index response = future.get();
* }
* }
*/
public final UnaryCallable getIndexCallable() {
return stub.getIndexCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a composite index.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* IndexName name = IndexName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[INDEX]");
* firestoreAdminClient.deleteIndex(name);
* }
* }
*
* @param name Required. A name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}/indexes/{index_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteIndex(IndexName name) {
DeleteIndexRequest request =
DeleteIndexRequest.newBuilder().setName(name == null ? null : name.toString()).build();
deleteIndex(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a composite index.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = IndexName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[INDEX]").toString();
* firestoreAdminClient.deleteIndex(name);
* }
* }
*
* @param name Required. A name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}/indexes/{index_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteIndex(String name) {
DeleteIndexRequest request = DeleteIndexRequest.newBuilder().setName(name).build();
deleteIndex(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a composite index.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DeleteIndexRequest request =
* DeleteIndexRequest.newBuilder()
* .setName(
* IndexName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[INDEX]").toString())
* .build();
* firestoreAdminClient.deleteIndex(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteIndex(DeleteIndexRequest request) {
deleteIndexCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a composite index.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DeleteIndexRequest request =
* DeleteIndexRequest.newBuilder()
* .setName(
* IndexName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[INDEX]").toString())
* .build();
* ApiFuture future = firestoreAdminClient.deleteIndexCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteIndexCallable() {
return stub.deleteIndexCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the metadata and configuration for a Field.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* FieldName name = FieldName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[FIELD]");
* Field response = firestoreAdminClient.getField(name);
* }
* }
*
* @param name Required. A name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}/fields/{field_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Field getField(FieldName name) {
GetFieldRequest request =
GetFieldRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getField(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the metadata and configuration for a Field.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = FieldName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[FIELD]").toString();
* Field response = firestoreAdminClient.getField(name);
* }
* }
*
* @param name Required. A name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}/fields/{field_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Field getField(String name) {
GetFieldRequest request = GetFieldRequest.newBuilder().setName(name).build();
return getField(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the metadata and configuration for a Field.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetFieldRequest request =
* GetFieldRequest.newBuilder()
* .setName(
* FieldName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[FIELD]").toString())
* .build();
* Field response = firestoreAdminClient.getField(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Field getField(GetFieldRequest request) {
return getFieldCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the metadata and configuration for a Field.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetFieldRequest request =
* GetFieldRequest.newBuilder()
* .setName(
* FieldName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]", "[FIELD]").toString())
* .build();
* ApiFuture future = firestoreAdminClient.getFieldCallable().futureCall(request);
* // Do something.
* Field response = future.get();
* }
* }
*/
public final UnaryCallable getFieldCallable() {
return stub.getFieldCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a field configuration. Currently, field updates apply only to single field index
* configuration. However, calls to
* [FirestoreAdmin.UpdateField][google.firestore.admin.v1.FirestoreAdmin.UpdateField] should
* provide a field mask to avoid changing any configuration that the caller isn't aware of. The
* field mask should be specified as: `{ paths: "index_config" }`.
*
* This call returns a [google.longrunning.Operation][google.longrunning.Operation] which may
* be used to track the status of the field update. The metadata for the operation will be the
* type [FieldOperationMetadata][google.firestore.admin.v1.FieldOperationMetadata].
*
*
To configure the default field settings for the database, use the special `Field` with
* resource name:
* `projects/{project_id}/databases/{database_id}/collectionGroups/__default__/fields/*`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* Field field = Field.newBuilder().build();
* Field response = firestoreAdminClient.updateFieldAsync(field).get();
* }
* }
*
* @param field Required. The field to be updated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateFieldAsync(Field field) {
UpdateFieldRequest request = UpdateFieldRequest.newBuilder().setField(field).build();
return updateFieldAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a field configuration. Currently, field updates apply only to single field index
* configuration. However, calls to
* [FirestoreAdmin.UpdateField][google.firestore.admin.v1.FirestoreAdmin.UpdateField] should
* provide a field mask to avoid changing any configuration that the caller isn't aware of. The
* field mask should be specified as: `{ paths: "index_config" }`.
*
* This call returns a [google.longrunning.Operation][google.longrunning.Operation] which may
* be used to track the status of the field update. The metadata for the operation will be the
* type [FieldOperationMetadata][google.firestore.admin.v1.FieldOperationMetadata].
*
*
To configure the default field settings for the database, use the special `Field` with
* resource name:
* `projects/{project_id}/databases/{database_id}/collectionGroups/__default__/fields/*`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* UpdateFieldRequest request =
* UpdateFieldRequest.newBuilder()
* .setField(Field.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Field response = firestoreAdminClient.updateFieldAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateFieldAsync(
UpdateFieldRequest request) {
return updateFieldOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a field configuration. Currently, field updates apply only to single field index
* configuration. However, calls to
* [FirestoreAdmin.UpdateField][google.firestore.admin.v1.FirestoreAdmin.UpdateField] should
* provide a field mask to avoid changing any configuration that the caller isn't aware of. The
* field mask should be specified as: `{ paths: "index_config" }`.
*
* This call returns a [google.longrunning.Operation][google.longrunning.Operation] which may
* be used to track the status of the field update. The metadata for the operation will be the
* type [FieldOperationMetadata][google.firestore.admin.v1.FieldOperationMetadata].
*
*
To configure the default field settings for the database, use the special `Field` with
* resource name:
* `projects/{project_id}/databases/{database_id}/collectionGroups/__default__/fields/*`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* UpdateFieldRequest request =
* UpdateFieldRequest.newBuilder()
* .setField(Field.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* OperationFuture future =
* firestoreAdminClient.updateFieldOperationCallable().futureCall(request);
* // Do something.
* Field response = future.get();
* }
* }
*/
public final OperationCallable
updateFieldOperationCallable() {
return stub.updateFieldOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a field configuration. Currently, field updates apply only to single field index
* configuration. However, calls to
* [FirestoreAdmin.UpdateField][google.firestore.admin.v1.FirestoreAdmin.UpdateField] should
* provide a field mask to avoid changing any configuration that the caller isn't aware of. The
* field mask should be specified as: `{ paths: "index_config" }`.
*
* This call returns a [google.longrunning.Operation][google.longrunning.Operation] which may
* be used to track the status of the field update. The metadata for the operation will be the
* type [FieldOperationMetadata][google.firestore.admin.v1.FieldOperationMetadata].
*
*
To configure the default field settings for the database, use the special `Field` with
* resource name:
* `projects/{project_id}/databases/{database_id}/collectionGroups/__default__/fields/*`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* UpdateFieldRequest request =
* UpdateFieldRequest.newBuilder()
* .setField(Field.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = firestoreAdminClient.updateFieldCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateFieldCallable() {
return stub.updateFieldCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the field configuration and metadata for this database.
*
* Currently, [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields]
* only supports listing fields that have been explicitly overridden. To issue this query, call
* [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields] with the
* filter set to `indexConfig.usesAncestorConfig:false` or `ttlConfig:*`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CollectionGroupName parent =
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]");
* for (Field element : firestoreAdminClient.listFields(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. A parent name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListFieldsPagedResponse listFields(CollectionGroupName parent) {
ListFieldsRequest request =
ListFieldsRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listFields(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the field configuration and metadata for this database.
*
* Currently, [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields]
* only supports listing fields that have been explicitly overridden. To issue this query, call
* [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields] with the
* filter set to `indexConfig.usesAncestorConfig:false` or `ttlConfig:*`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String parent = CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString();
* for (Field element : firestoreAdminClient.listFields(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. A parent name of the form
* `projects/{project_id}/databases/{database_id}/collectionGroups/{collection_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListFieldsPagedResponse listFields(String parent) {
ListFieldsRequest request = ListFieldsRequest.newBuilder().setParent(parent).build();
return listFields(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the field configuration and metadata for this database.
*
* Currently, [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields]
* only supports listing fields that have been explicitly overridden. To issue this query, call
* [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields] with the
* filter set to `indexConfig.usesAncestorConfig:false` or `ttlConfig:*`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListFieldsRequest request =
* ListFieldsRequest.newBuilder()
* .setParent(
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Field element : firestoreAdminClient.listFields(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListFieldsPagedResponse listFields(ListFieldsRequest request) {
return listFieldsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the field configuration and metadata for this database.
*
* Currently, [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields]
* only supports listing fields that have been explicitly overridden. To issue this query, call
* [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields] with the
* filter set to `indexConfig.usesAncestorConfig:false` or `ttlConfig:*`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListFieldsRequest request =
* ListFieldsRequest.newBuilder()
* .setParent(
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future = firestoreAdminClient.listFieldsPagedCallable().futureCall(request);
* // Do something.
* for (Field element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listFieldsPagedCallable() {
return stub.listFieldsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the field configuration and metadata for this database.
*
* Currently, [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields]
* only supports listing fields that have been explicitly overridden. To issue this query, call
* [FirestoreAdmin.ListFields][google.firestore.admin.v1.FirestoreAdmin.ListFields] with the
* filter set to `indexConfig.usesAncestorConfig:false` or `ttlConfig:*`.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListFieldsRequest request =
* ListFieldsRequest.newBuilder()
* .setParent(
* CollectionGroupName.of("[PROJECT]", "[DATABASE]", "[COLLECTION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListFieldsResponse response = firestoreAdminClient.listFieldsCallable().call(request);
* for (Field element : response.getFieldsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listFieldsCallable() {
return stub.listFieldsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Exports a copy of all or a subset of documents from Google Cloud Firestore to another storage
* system, such as Google Cloud Storage. Recent updates to documents may not be reflected in the
* export. The export occurs in the background and its progress can be monitored and managed via
* the Operation resource that is created. The output of an export may only be used once the
* associated operation is done. If an export operation is cancelled before completion it may
* leave partial data behind in Google Cloud Storage.
*
* For more details on export behavior and output format, refer to:
* https://cloud.google.com/firestore/docs/manage-data/export-import
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DatabaseName name = DatabaseName.of("[PROJECT]", "[DATABASE]");
* ExportDocumentsResponse response = firestoreAdminClient.exportDocumentsAsync(name).get();
* }
* }
*
* @param name Required. Database to export. Should be of the form:
* `projects/{project_id}/databases/{database_id}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
exportDocumentsAsync(DatabaseName name) {
ExportDocumentsRequest request =
ExportDocumentsRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return exportDocumentsAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Exports a copy of all or a subset of documents from Google Cloud Firestore to another storage
* system, such as Google Cloud Storage. Recent updates to documents may not be reflected in the
* export. The export occurs in the background and its progress can be monitored and managed via
* the Operation resource that is created. The output of an export may only be used once the
* associated operation is done. If an export operation is cancelled before completion it may
* leave partial data behind in Google Cloud Storage.
*
* For more details on export behavior and output format, refer to:
* https://cloud.google.com/firestore/docs/manage-data/export-import
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = DatabaseName.of("[PROJECT]", "[DATABASE]").toString();
* ExportDocumentsResponse response = firestoreAdminClient.exportDocumentsAsync(name).get();
* }
* }
*
* @param name Required. Database to export. Should be of the form:
* `projects/{project_id}/databases/{database_id}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
exportDocumentsAsync(String name) {
ExportDocumentsRequest request = ExportDocumentsRequest.newBuilder().setName(name).build();
return exportDocumentsAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Exports a copy of all or a subset of documents from Google Cloud Firestore to another storage
* system, such as Google Cloud Storage. Recent updates to documents may not be reflected in the
* export. The export occurs in the background and its progress can be monitored and managed via
* the Operation resource that is created. The output of an export may only be used once the
* associated operation is done. If an export operation is cancelled before completion it may
* leave partial data behind in Google Cloud Storage.
*
* For more details on export behavior and output format, refer to:
* https://cloud.google.com/firestore/docs/manage-data/export-import
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ExportDocumentsRequest request =
* ExportDocumentsRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .addAllCollectionIds(new ArrayList())
* .setOutputUriPrefix("outputUriPrefix499858205")
* .addAllNamespaceIds(new ArrayList())
* .setSnapshotTime(Timestamp.newBuilder().build())
* .build();
* ExportDocumentsResponse response = firestoreAdminClient.exportDocumentsAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
exportDocumentsAsync(ExportDocumentsRequest request) {
return exportDocumentsOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Exports a copy of all or a subset of documents from Google Cloud Firestore to another storage
* system, such as Google Cloud Storage. Recent updates to documents may not be reflected in the
* export. The export occurs in the background and its progress can be monitored and managed via
* the Operation resource that is created. The output of an export may only be used once the
* associated operation is done. If an export operation is cancelled before completion it may
* leave partial data behind in Google Cloud Storage.
*
* For more details on export behavior and output format, refer to:
* https://cloud.google.com/firestore/docs/manage-data/export-import
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ExportDocumentsRequest request =
* ExportDocumentsRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .addAllCollectionIds(new ArrayList())
* .setOutputUriPrefix("outputUriPrefix499858205")
* .addAllNamespaceIds(new ArrayList())
* .setSnapshotTime(Timestamp.newBuilder().build())
* .build();
* OperationFuture future =
* firestoreAdminClient.exportDocumentsOperationCallable().futureCall(request);
* // Do something.
* ExportDocumentsResponse response = future.get();
* }
* }
*/
public final OperationCallable<
ExportDocumentsRequest, ExportDocumentsResponse, ExportDocumentsMetadata>
exportDocumentsOperationCallable() {
return stub.exportDocumentsOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Exports a copy of all or a subset of documents from Google Cloud Firestore to another storage
* system, such as Google Cloud Storage. Recent updates to documents may not be reflected in the
* export. The export occurs in the background and its progress can be monitored and managed via
* the Operation resource that is created. The output of an export may only be used once the
* associated operation is done. If an export operation is cancelled before completion it may
* leave partial data behind in Google Cloud Storage.
*
* For more details on export behavior and output format, refer to:
* https://cloud.google.com/firestore/docs/manage-data/export-import
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ExportDocumentsRequest request =
* ExportDocumentsRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .addAllCollectionIds(new ArrayList())
* .setOutputUriPrefix("outputUriPrefix499858205")
* .addAllNamespaceIds(new ArrayList())
* .setSnapshotTime(Timestamp.newBuilder().build())
* .build();
* ApiFuture future =
* firestoreAdminClient.exportDocumentsCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable exportDocumentsCallable() {
return stub.exportDocumentsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Imports documents into Google Cloud Firestore. Existing documents with the same name are
* overwritten. The import occurs in the background and its progress can be monitored and managed
* via the Operation resource that is created. If an ImportDocuments operation is cancelled, it is
* possible that a subset of the data has already been imported to Cloud Firestore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DatabaseName name = DatabaseName.of("[PROJECT]", "[DATABASE]");
* firestoreAdminClient.importDocumentsAsync(name).get();
* }
* }
*
* @param name Required. Database to import into. Should be of the form:
* `projects/{project_id}/databases/{database_id}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture importDocumentsAsync(
DatabaseName name) {
ImportDocumentsRequest request =
ImportDocumentsRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return importDocumentsAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Imports documents into Google Cloud Firestore. Existing documents with the same name are
* overwritten. The import occurs in the background and its progress can be monitored and managed
* via the Operation resource that is created. If an ImportDocuments operation is cancelled, it is
* possible that a subset of the data has already been imported to Cloud Firestore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = DatabaseName.of("[PROJECT]", "[DATABASE]").toString();
* firestoreAdminClient.importDocumentsAsync(name).get();
* }
* }
*
* @param name Required. Database to import into. Should be of the form:
* `projects/{project_id}/databases/{database_id}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture importDocumentsAsync(String name) {
ImportDocumentsRequest request = ImportDocumentsRequest.newBuilder().setName(name).build();
return importDocumentsAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Imports documents into Google Cloud Firestore. Existing documents with the same name are
* overwritten. The import occurs in the background and its progress can be monitored and managed
* via the Operation resource that is created. If an ImportDocuments operation is cancelled, it is
* possible that a subset of the data has already been imported to Cloud Firestore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ImportDocumentsRequest request =
* ImportDocumentsRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .addAllCollectionIds(new ArrayList())
* .setInputUriPrefix("inputUriPrefix-97481100")
* .addAllNamespaceIds(new ArrayList())
* .build();
* firestoreAdminClient.importDocumentsAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture importDocumentsAsync(
ImportDocumentsRequest request) {
return importDocumentsOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Imports documents into Google Cloud Firestore. Existing documents with the same name are
* overwritten. The import occurs in the background and its progress can be monitored and managed
* via the Operation resource that is created. If an ImportDocuments operation is cancelled, it is
* possible that a subset of the data has already been imported to Cloud Firestore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ImportDocumentsRequest request =
* ImportDocumentsRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .addAllCollectionIds(new ArrayList())
* .setInputUriPrefix("inputUriPrefix-97481100")
* .addAllNamespaceIds(new ArrayList())
* .build();
* OperationFuture future =
* firestoreAdminClient.importDocumentsOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
importDocumentsOperationCallable() {
return stub.importDocumentsOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Imports documents into Google Cloud Firestore. Existing documents with the same name are
* overwritten. The import occurs in the background and its progress can be monitored and managed
* via the Operation resource that is created. If an ImportDocuments operation is cancelled, it is
* possible that a subset of the data has already been imported to Cloud Firestore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ImportDocumentsRequest request =
* ImportDocumentsRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .addAllCollectionIds(new ArrayList())
* .setInputUriPrefix("inputUriPrefix-97481100")
* .addAllNamespaceIds(new ArrayList())
* .build();
* ApiFuture future =
* firestoreAdminClient.importDocumentsCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable importDocumentsCallable() {
return stub.importDocumentsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Bulk deletes a subset of documents from Google Cloud Firestore. Documents created or updated
* after the underlying system starts to process the request will not be deleted. The bulk delete
* occurs in the background and its progress can be monitored and managed via the Operation
* resource that is created.
*
* For more details on bulk delete behavior, refer to:
* https://cloud.google.com/firestore/docs/manage-data/bulk-delete
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DatabaseName name = DatabaseName.of("[PROJECT]", "[DATABASE]");
* BulkDeleteDocumentsResponse response =
* firestoreAdminClient.bulkDeleteDocumentsAsync(name).get();
* }
* }
*
* @param name Required. Database to operate. Should be of the form:
* `projects/{project_id}/databases/{database_id}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
bulkDeleteDocumentsAsync(DatabaseName name) {
BulkDeleteDocumentsRequest request =
BulkDeleteDocumentsRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return bulkDeleteDocumentsAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Bulk deletes a subset of documents from Google Cloud Firestore. Documents created or updated
* after the underlying system starts to process the request will not be deleted. The bulk delete
* occurs in the background and its progress can be monitored and managed via the Operation
* resource that is created.
*
* For more details on bulk delete behavior, refer to:
* https://cloud.google.com/firestore/docs/manage-data/bulk-delete
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = DatabaseName.of("[PROJECT]", "[DATABASE]").toString();
* BulkDeleteDocumentsResponse response =
* firestoreAdminClient.bulkDeleteDocumentsAsync(name).get();
* }
* }
*
* @param name Required. Database to operate. Should be of the form:
* `projects/{project_id}/databases/{database_id}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
bulkDeleteDocumentsAsync(String name) {
BulkDeleteDocumentsRequest request =
BulkDeleteDocumentsRequest.newBuilder().setName(name).build();
return bulkDeleteDocumentsAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Bulk deletes a subset of documents from Google Cloud Firestore. Documents created or updated
* after the underlying system starts to process the request will not be deleted. The bulk delete
* occurs in the background and its progress can be monitored and managed via the Operation
* resource that is created.
*
* For more details on bulk delete behavior, refer to:
* https://cloud.google.com/firestore/docs/manage-data/bulk-delete
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* BulkDeleteDocumentsRequest request =
* BulkDeleteDocumentsRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .addAllCollectionIds(new ArrayList())
* .addAllNamespaceIds(new ArrayList())
* .build();
* BulkDeleteDocumentsResponse response =
* firestoreAdminClient.bulkDeleteDocumentsAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
bulkDeleteDocumentsAsync(BulkDeleteDocumentsRequest request) {
return bulkDeleteDocumentsOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Bulk deletes a subset of documents from Google Cloud Firestore. Documents created or updated
* after the underlying system starts to process the request will not be deleted. The bulk delete
* occurs in the background and its progress can be monitored and managed via the Operation
* resource that is created.
*
* For more details on bulk delete behavior, refer to:
* https://cloud.google.com/firestore/docs/manage-data/bulk-delete
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* BulkDeleteDocumentsRequest request =
* BulkDeleteDocumentsRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .addAllCollectionIds(new ArrayList())
* .addAllNamespaceIds(new ArrayList())
* .build();
* OperationFuture future =
* firestoreAdminClient.bulkDeleteDocumentsOperationCallable().futureCall(request);
* // Do something.
* BulkDeleteDocumentsResponse response = future.get();
* }
* }
*/
public final OperationCallable<
BulkDeleteDocumentsRequest, BulkDeleteDocumentsResponse, BulkDeleteDocumentsMetadata>
bulkDeleteDocumentsOperationCallable() {
return stub.bulkDeleteDocumentsOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Bulk deletes a subset of documents from Google Cloud Firestore. Documents created or updated
* after the underlying system starts to process the request will not be deleted. The bulk delete
* occurs in the background and its progress can be monitored and managed via the Operation
* resource that is created.
*
* For more details on bulk delete behavior, refer to:
* https://cloud.google.com/firestore/docs/manage-data/bulk-delete
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* BulkDeleteDocumentsRequest request =
* BulkDeleteDocumentsRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .addAllCollectionIds(new ArrayList())
* .addAllNamespaceIds(new ArrayList())
* .build();
* ApiFuture future =
* firestoreAdminClient.bulkDeleteDocumentsCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable bulkDeleteDocumentsCallable() {
return stub.bulkDeleteDocumentsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ProjectName parent = ProjectName.of("[PROJECT]");
* Database database = Database.newBuilder().build();
* String databaseId = "databaseId1688905718";
* Database response =
* firestoreAdminClient.createDatabaseAsync(parent, database, databaseId).get();
* }
* }
*
* @param parent Required. A parent name of the form `projects/{project_id}`
* @param database Required. The Database to create.
* @param databaseId Required. The ID to use for the database, which will become the final
* component of the database's resource name.
* This value should be 4-63 characters. Valid characters are /[a-z][0-9]-/ with first
* character a letter and the last a letter or a number. Must not be UUID-like
* /[0-9a-f]{8}(-[0-9a-f]{4}){3}-[0-9a-f]{12}/.
*
"(default)" database ID is also valid.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createDatabaseAsync(
ProjectName parent, Database database, String databaseId) {
CreateDatabaseRequest request =
CreateDatabaseRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setDatabase(database)
.setDatabaseId(databaseId)
.build();
return createDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String parent = ProjectName.of("[PROJECT]").toString();
* Database database = Database.newBuilder().build();
* String databaseId = "databaseId1688905718";
* Database response =
* firestoreAdminClient.createDatabaseAsync(parent, database, databaseId).get();
* }
* }
*
* @param parent Required. A parent name of the form `projects/{project_id}`
* @param database Required. The Database to create.
* @param databaseId Required. The ID to use for the database, which will become the final
* component of the database's resource name.
* This value should be 4-63 characters. Valid characters are /[a-z][0-9]-/ with first
* character a letter and the last a letter or a number. Must not be UUID-like
* /[0-9a-f]{8}(-[0-9a-f]{4}){3}-[0-9a-f]{12}/.
*
"(default)" database ID is also valid.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createDatabaseAsync(
String parent, Database database, String databaseId) {
CreateDatabaseRequest request =
CreateDatabaseRequest.newBuilder()
.setParent(parent)
.setDatabase(database)
.setDatabaseId(databaseId)
.build();
return createDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CreateDatabaseRequest request =
* CreateDatabaseRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setDatabase(Database.newBuilder().build())
* .setDatabaseId("databaseId1688905718")
* .build();
* Database response = firestoreAdminClient.createDatabaseAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createDatabaseAsync(
CreateDatabaseRequest request) {
return createDatabaseOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CreateDatabaseRequest request =
* CreateDatabaseRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setDatabase(Database.newBuilder().build())
* .setDatabaseId("databaseId1688905718")
* .build();
* OperationFuture future =
* firestoreAdminClient.createDatabaseOperationCallable().futureCall(request);
* // Do something.
* Database response = future.get();
* }
* }
*/
public final OperationCallable
createDatabaseOperationCallable() {
return stub.createDatabaseOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Create a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CreateDatabaseRequest request =
* CreateDatabaseRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setDatabase(Database.newBuilder().build())
* .setDatabaseId("databaseId1688905718")
* .build();
* ApiFuture future =
* firestoreAdminClient.createDatabaseCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createDatabaseCallable() {
return stub.createDatabaseCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DatabaseName name = DatabaseName.of("[PROJECT]", "[DATABASE]");
* Database response = firestoreAdminClient.getDatabase(name);
* }
* }
*
* @param name Required. A name of the form `projects/{project_id}/databases/{database_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Database getDatabase(DatabaseName name) {
GetDatabaseRequest request =
GetDatabaseRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getDatabase(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = DatabaseName.of("[PROJECT]", "[DATABASE]").toString();
* Database response = firestoreAdminClient.getDatabase(name);
* }
* }
*
* @param name Required. A name of the form `projects/{project_id}/databases/{database_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Database getDatabase(String name) {
GetDatabaseRequest request = GetDatabaseRequest.newBuilder().setName(name).build();
return getDatabase(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetDatabaseRequest request =
* GetDatabaseRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .build();
* Database response = firestoreAdminClient.getDatabase(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Database getDatabase(GetDatabaseRequest request) {
return getDatabaseCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetDatabaseRequest request =
* GetDatabaseRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .build();
* ApiFuture future = firestoreAdminClient.getDatabaseCallable().futureCall(request);
* // Do something.
* Database response = future.get();
* }
* }
*/
public final UnaryCallable getDatabaseCallable() {
return stub.getDatabaseCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all the databases in the project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ProjectName parent = ProjectName.of("[PROJECT]");
* ListDatabasesResponse response = firestoreAdminClient.listDatabases(parent);
* }
* }
*
* @param parent Required. A parent name of the form `projects/{project_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDatabasesResponse listDatabases(ProjectName parent) {
ListDatabasesRequest request =
ListDatabasesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listDatabases(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all the databases in the project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String parent = ProjectName.of("[PROJECT]").toString();
* ListDatabasesResponse response = firestoreAdminClient.listDatabases(parent);
* }
* }
*
* @param parent Required. A parent name of the form `projects/{project_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDatabasesResponse listDatabases(String parent) {
ListDatabasesRequest request = ListDatabasesRequest.newBuilder().setParent(parent).build();
return listDatabases(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all the databases in the project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListDatabasesRequest request =
* ListDatabasesRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setShowDeleted(true)
* .build();
* ListDatabasesResponse response = firestoreAdminClient.listDatabases(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDatabasesResponse listDatabases(ListDatabasesRequest request) {
return listDatabasesCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all the databases in the project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListDatabasesRequest request =
* ListDatabasesRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setShowDeleted(true)
* .build();
* ApiFuture future =
* firestoreAdminClient.listDatabasesCallable().futureCall(request);
* // Do something.
* ListDatabasesResponse response = future.get();
* }
* }
*/
public final UnaryCallable listDatabasesCallable() {
return stub.listDatabasesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* Database database = Database.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Database response = firestoreAdminClient.updateDatabaseAsync(database, updateMask).get();
* }
* }
*
* @param database Required. The database to update.
* @param updateMask The list of fields to be updated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateDatabaseAsync(
Database database, FieldMask updateMask) {
UpdateDatabaseRequest request =
UpdateDatabaseRequest.newBuilder().setDatabase(database).setUpdateMask(updateMask).build();
return updateDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* UpdateDatabaseRequest request =
* UpdateDatabaseRequest.newBuilder()
* .setDatabase(Database.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Database response = firestoreAdminClient.updateDatabaseAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateDatabaseAsync(
UpdateDatabaseRequest request) {
return updateDatabaseOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* UpdateDatabaseRequest request =
* UpdateDatabaseRequest.newBuilder()
* .setDatabase(Database.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* OperationFuture future =
* firestoreAdminClient.updateDatabaseOperationCallable().futureCall(request);
* // Do something.
* Database response = future.get();
* }
* }
*/
public final OperationCallable
updateDatabaseOperationCallable() {
return stub.updateDatabaseOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* UpdateDatabaseRequest request =
* UpdateDatabaseRequest.newBuilder()
* .setDatabase(Database.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* firestoreAdminClient.updateDatabaseCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateDatabaseCallable() {
return stub.updateDatabaseCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DatabaseName name = DatabaseName.of("[PROJECT]", "[DATABASE]");
* Database response = firestoreAdminClient.deleteDatabaseAsync(name).get();
* }
* }
*
* @param name Required. A name of the form `projects/{project_id}/databases/{database_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteDatabaseAsync(
DatabaseName name) {
DeleteDatabaseRequest request =
DeleteDatabaseRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = DatabaseName.of("[PROJECT]", "[DATABASE]").toString();
* Database response = firestoreAdminClient.deleteDatabaseAsync(name).get();
* }
* }
*
* @param name Required. A name of the form `projects/{project_id}/databases/{database_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteDatabaseAsync(String name) {
DeleteDatabaseRequest request = DeleteDatabaseRequest.newBuilder().setName(name).build();
return deleteDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DeleteDatabaseRequest request =
* DeleteDatabaseRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .setEtag("etag3123477")
* .build();
* Database response = firestoreAdminClient.deleteDatabaseAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteDatabaseAsync(
DeleteDatabaseRequest request) {
return deleteDatabaseOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DeleteDatabaseRequest request =
* DeleteDatabaseRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .setEtag("etag3123477")
* .build();
* OperationFuture future =
* firestoreAdminClient.deleteDatabaseOperationCallable().futureCall(request);
* // Do something.
* Database response = future.get();
* }
* }
*/
public final OperationCallable
deleteDatabaseOperationCallable() {
return stub.deleteDatabaseOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DeleteDatabaseRequest request =
* DeleteDatabaseRequest.newBuilder()
* .setName(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .setEtag("etag3123477")
* .build();
* ApiFuture future =
* firestoreAdminClient.deleteDatabaseCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable deleteDatabaseCallable() {
return stub.deleteDatabaseCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* BackupName name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]");
* Backup response = firestoreAdminClient.getBackup(name);
* }
* }
*
* @param name Required. Name of the backup to fetch.
* Format is `projects/{project}/locations/{location}/backups/{backup}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Backup getBackup(BackupName name) {
GetBackupRequest request =
GetBackupRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a backup.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString();
* Backup response = firestoreAdminClient.getBackup(name);
* }
* }
*
* @param name Required. Name of the backup to fetch.
* Format is `projects/{project}/locations/{location}/backups/{backup}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Backup getBackup(String name) {
GetBackupRequest request = GetBackupRequest.newBuilder().setName(name).build();
return getBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a backup.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetBackupRequest request =
* GetBackupRequest.newBuilder()
* .setName(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .build();
* Backup response = firestoreAdminClient.getBackup(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Backup getBackup(GetBackupRequest request) {
return getBackupCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetBackupRequest request =
* GetBackupRequest.newBuilder()
* .setName(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .build();
* ApiFuture future = firestoreAdminClient.getBackupCallable().futureCall(request);
* // Do something.
* Backup response = future.get();
* }
* }
*/
public final UnaryCallable getBackupCallable() {
return stub.getBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the backups.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* ListBackupsResponse response = firestoreAdminClient.listBackups(parent);
* }
* }
*
* @param parent Required. The location to list backups from.
* Format is `projects/{project}/locations/{location}`. Use `{location} = '-'` to list
* backups from all locations for the given project. This allows listing backups from a single
* location or from all locations.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupsResponse listBackups(LocationName parent) {
ListBackupsRequest request =
ListBackupsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the backups.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* ListBackupsResponse response = firestoreAdminClient.listBackups(parent);
* }
* }
*
* @param parent Required. The location to list backups from.
* Format is `projects/{project}/locations/{location}`. Use `{location} = '-'` to list
* backups from all locations for the given project. This allows listing backups from a single
* location or from all locations.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupsResponse listBackups(String parent) {
ListBackupsRequest request = ListBackupsRequest.newBuilder().setParent(parent).build();
return listBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the backups.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListBackupsRequest request =
* ListBackupsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .build();
* ListBackupsResponse response = firestoreAdminClient.listBackups(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupsResponse listBackups(ListBackupsRequest request) {
return listBackupsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the backups.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListBackupsRequest request =
* ListBackupsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .build();
* ApiFuture future =
* firestoreAdminClient.listBackupsCallable().futureCall(request);
* // Do something.
* ListBackupsResponse response = future.get();
* }
* }
*/
public final UnaryCallable listBackupsCallable() {
return stub.listBackupsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* BackupName name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]");
* firestoreAdminClient.deleteBackup(name);
* }
* }
*
* @param name Required. Name of the backup to delete.
* format is `projects/{project}/locations/{location}/backups/{backup}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteBackup(BackupName name) {
DeleteBackupRequest request =
DeleteBackupRequest.newBuilder().setName(name == null ? null : name.toString()).build();
deleteBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a backup.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString();
* firestoreAdminClient.deleteBackup(name);
* }
* }
*
* @param name Required. Name of the backup to delete.
* format is `projects/{project}/locations/{location}/backups/{backup}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteBackup(String name) {
DeleteBackupRequest request = DeleteBackupRequest.newBuilder().setName(name).build();
deleteBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a backup.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DeleteBackupRequest request =
* DeleteBackupRequest.newBuilder()
* .setName(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .build();
* firestoreAdminClient.deleteBackup(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteBackup(DeleteBackupRequest request) {
deleteBackupCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DeleteBackupRequest request =
* DeleteBackupRequest.newBuilder()
* .setName(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .build();
* ApiFuture future = firestoreAdminClient.deleteBackupCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteBackupCallable() {
return stub.deleteBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new database by restoring from an existing backup.
*
* The new database must be in the same cloud region or multi-region location as the existing
* backup. This behaves similar to
* [FirestoreAdmin.CreateDatabase][google.firestore.admin.v1.FirestoreAdmin.CreateDatabase] except
* instead of creating a new empty database, a new database is created with the database type,
* index configuration, and documents from an existing backup.
*
*
The [long-running operation][google.longrunning.Operation] can be used to track the progress
* of the restore, with the Operation's [metadata][google.longrunning.Operation.metadata] field
* type being the [RestoreDatabaseMetadata][google.firestore.admin.v1.RestoreDatabaseMetadata].
* The [response][google.longrunning.Operation.response] type is the
* [Database][google.firestore.admin.v1.Database] if the restore was successful. The new database
* is not readable or writeable until the LRO has completed.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* RestoreDatabaseRequest request =
* RestoreDatabaseRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setDatabaseId("databaseId1688905718")
* .setBackup(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .setEncryptionConfig(Database.EncryptionConfig.newBuilder().build())
* .build();
* Database response = firestoreAdminClient.restoreDatabaseAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture restoreDatabaseAsync(
RestoreDatabaseRequest request) {
return restoreDatabaseOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new database by restoring from an existing backup.
*
* The new database must be in the same cloud region or multi-region location as the existing
* backup. This behaves similar to
* [FirestoreAdmin.CreateDatabase][google.firestore.admin.v1.FirestoreAdmin.CreateDatabase] except
* instead of creating a new empty database, a new database is created with the database type,
* index configuration, and documents from an existing backup.
*
*
The [long-running operation][google.longrunning.Operation] can be used to track the progress
* of the restore, with the Operation's [metadata][google.longrunning.Operation.metadata] field
* type being the [RestoreDatabaseMetadata][google.firestore.admin.v1.RestoreDatabaseMetadata].
* The [response][google.longrunning.Operation.response] type is the
* [Database][google.firestore.admin.v1.Database] if the restore was successful. The new database
* is not readable or writeable until the LRO has completed.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* RestoreDatabaseRequest request =
* RestoreDatabaseRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setDatabaseId("databaseId1688905718")
* .setBackup(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .setEncryptionConfig(Database.EncryptionConfig.newBuilder().build())
* .build();
* OperationFuture future =
* firestoreAdminClient.restoreDatabaseOperationCallable().futureCall(request);
* // Do something.
* Database response = future.get();
* }
* }
*/
public final OperationCallable
restoreDatabaseOperationCallable() {
return stub.restoreDatabaseOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new database by restoring from an existing backup.
*
* The new database must be in the same cloud region or multi-region location as the existing
* backup. This behaves similar to
* [FirestoreAdmin.CreateDatabase][google.firestore.admin.v1.FirestoreAdmin.CreateDatabase] except
* instead of creating a new empty database, a new database is created with the database type,
* index configuration, and documents from an existing backup.
*
*
The [long-running operation][google.longrunning.Operation] can be used to track the progress
* of the restore, with the Operation's [metadata][google.longrunning.Operation.metadata] field
* type being the [RestoreDatabaseMetadata][google.firestore.admin.v1.RestoreDatabaseMetadata].
* The [response][google.longrunning.Operation.response] type is the
* [Database][google.firestore.admin.v1.Database] if the restore was successful. The new database
* is not readable or writeable until the LRO has completed.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* RestoreDatabaseRequest request =
* RestoreDatabaseRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setDatabaseId("databaseId1688905718")
* .setBackup(BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP]").toString())
* .setEncryptionConfig(Database.EncryptionConfig.newBuilder().build())
* .build();
* ApiFuture future =
* firestoreAdminClient.restoreDatabaseCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable restoreDatabaseCallable() {
return stub.restoreDatabaseCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a backup schedule on a database. At most two backup schedules can be configured on a
* database, one daily backup schedule and one weekly backup schedule.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DatabaseName parent = DatabaseName.of("[PROJECT]", "[DATABASE]");
* BackupSchedule backupSchedule = BackupSchedule.newBuilder().build();
* BackupSchedule response = firestoreAdminClient.createBackupSchedule(parent, backupSchedule);
* }
* }
*
* @param parent Required. The parent database.
* Format `projects/{project}/databases/{database}`
* @param backupSchedule Required. The backup schedule to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupSchedule createBackupSchedule(
DatabaseName parent, BackupSchedule backupSchedule) {
CreateBackupScheduleRequest request =
CreateBackupScheduleRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setBackupSchedule(backupSchedule)
.build();
return createBackupSchedule(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a backup schedule on a database. At most two backup schedules can be configured on a
* database, one daily backup schedule and one weekly backup schedule.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String parent = DatabaseName.of("[PROJECT]", "[DATABASE]").toString();
* BackupSchedule backupSchedule = BackupSchedule.newBuilder().build();
* BackupSchedule response = firestoreAdminClient.createBackupSchedule(parent, backupSchedule);
* }
* }
*
* @param parent Required. The parent database.
* Format `projects/{project}/databases/{database}`
* @param backupSchedule Required. The backup schedule to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupSchedule createBackupSchedule(String parent, BackupSchedule backupSchedule) {
CreateBackupScheduleRequest request =
CreateBackupScheduleRequest.newBuilder()
.setParent(parent)
.setBackupSchedule(backupSchedule)
.build();
return createBackupSchedule(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a backup schedule on a database. At most two backup schedules can be configured on a
* database, one daily backup schedule and one weekly backup schedule.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CreateBackupScheduleRequest request =
* CreateBackupScheduleRequest.newBuilder()
* .setParent(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .setBackupSchedule(BackupSchedule.newBuilder().build())
* .build();
* BackupSchedule response = firestoreAdminClient.createBackupSchedule(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupSchedule createBackupSchedule(CreateBackupScheduleRequest request) {
return createBackupScheduleCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a backup schedule on a database. At most two backup schedules can be configured on a
* database, one daily backup schedule and one weekly backup schedule.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* CreateBackupScheduleRequest request =
* CreateBackupScheduleRequest.newBuilder()
* .setParent(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .setBackupSchedule(BackupSchedule.newBuilder().build())
* .build();
* ApiFuture future =
* firestoreAdminClient.createBackupScheduleCallable().futureCall(request);
* // Do something.
* BackupSchedule response = future.get();
* }
* }
*/
public final UnaryCallable
createBackupScheduleCallable() {
return stub.createBackupScheduleCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a backup schedule.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* BackupScheduleName name =
* BackupScheduleName.of("[PROJECT]", "[DATABASE]", "[BACKUP_SCHEDULE]");
* BackupSchedule response = firestoreAdminClient.getBackupSchedule(name);
* }
* }
*
* @param name Required. The name of the backup schedule.
* Format `projects/{project}/databases/{database}/backupSchedules/{backup_schedule}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupSchedule getBackupSchedule(BackupScheduleName name) {
GetBackupScheduleRequest request =
GetBackupScheduleRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getBackupSchedule(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a backup schedule.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name =
* BackupScheduleName.of("[PROJECT]", "[DATABASE]", "[BACKUP_SCHEDULE]").toString();
* BackupSchedule response = firestoreAdminClient.getBackupSchedule(name);
* }
* }
*
* @param name Required. The name of the backup schedule.
* Format `projects/{project}/databases/{database}/backupSchedules/{backup_schedule}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupSchedule getBackupSchedule(String name) {
GetBackupScheduleRequest request = GetBackupScheduleRequest.newBuilder().setName(name).build();
return getBackupSchedule(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a backup schedule.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetBackupScheduleRequest request =
* GetBackupScheduleRequest.newBuilder()
* .setName(
* BackupScheduleName.of("[PROJECT]", "[DATABASE]", "[BACKUP_SCHEDULE]").toString())
* .build();
* BackupSchedule response = firestoreAdminClient.getBackupSchedule(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupSchedule getBackupSchedule(GetBackupScheduleRequest request) {
return getBackupScheduleCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a backup schedule.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* GetBackupScheduleRequest request =
* GetBackupScheduleRequest.newBuilder()
* .setName(
* BackupScheduleName.of("[PROJECT]", "[DATABASE]", "[BACKUP_SCHEDULE]").toString())
* .build();
* ApiFuture future =
* firestoreAdminClient.getBackupScheduleCallable().futureCall(request);
* // Do something.
* BackupSchedule response = future.get();
* }
* }
*/
public final UnaryCallable getBackupScheduleCallable() {
return stub.getBackupScheduleCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List backup schedules.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DatabaseName parent = DatabaseName.of("[PROJECT]", "[DATABASE]");
* ListBackupSchedulesResponse response = firestoreAdminClient.listBackupSchedules(parent);
* }
* }
*
* @param parent Required. The parent database.
* Format is `projects/{project}/databases/{database}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupSchedulesResponse listBackupSchedules(DatabaseName parent) {
ListBackupSchedulesRequest request =
ListBackupSchedulesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listBackupSchedules(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List backup schedules.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String parent = DatabaseName.of("[PROJECT]", "[DATABASE]").toString();
* ListBackupSchedulesResponse response = firestoreAdminClient.listBackupSchedules(parent);
* }
* }
*
* @param parent Required. The parent database.
* Format is `projects/{project}/databases/{database}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupSchedulesResponse listBackupSchedules(String parent) {
ListBackupSchedulesRequest request =
ListBackupSchedulesRequest.newBuilder().setParent(parent).build();
return listBackupSchedules(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List backup schedules.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListBackupSchedulesRequest request =
* ListBackupSchedulesRequest.newBuilder()
* .setParent(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .build();
* ListBackupSchedulesResponse response = firestoreAdminClient.listBackupSchedules(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupSchedulesResponse listBackupSchedules(ListBackupSchedulesRequest request) {
return listBackupSchedulesCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List backup schedules.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* ListBackupSchedulesRequest request =
* ListBackupSchedulesRequest.newBuilder()
* .setParent(DatabaseName.of("[PROJECT]", "[DATABASE]").toString())
* .build();
* ApiFuture future =
* firestoreAdminClient.listBackupSchedulesCallable().futureCall(request);
* // Do something.
* ListBackupSchedulesResponse response = future.get();
* }
* }
*/
public final UnaryCallable
listBackupSchedulesCallable() {
return stub.listBackupSchedulesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a backup schedule.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* BackupSchedule backupSchedule = BackupSchedule.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* BackupSchedule response =
* firestoreAdminClient.updateBackupSchedule(backupSchedule, updateMask);
* }
* }
*
* @param backupSchedule Required. The backup schedule to update.
* @param updateMask The list of fields to be updated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupSchedule updateBackupSchedule(
BackupSchedule backupSchedule, FieldMask updateMask) {
UpdateBackupScheduleRequest request =
UpdateBackupScheduleRequest.newBuilder()
.setBackupSchedule(backupSchedule)
.setUpdateMask(updateMask)
.build();
return updateBackupSchedule(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a backup schedule.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* UpdateBackupScheduleRequest request =
* UpdateBackupScheduleRequest.newBuilder()
* .setBackupSchedule(BackupSchedule.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* BackupSchedule response = firestoreAdminClient.updateBackupSchedule(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupSchedule updateBackupSchedule(UpdateBackupScheduleRequest request) {
return updateBackupScheduleCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a backup schedule.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* UpdateBackupScheduleRequest request =
* UpdateBackupScheduleRequest.newBuilder()
* .setBackupSchedule(BackupSchedule.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* firestoreAdminClient.updateBackupScheduleCallable().futureCall(request);
* // Do something.
* BackupSchedule response = future.get();
* }
* }
*/
public final UnaryCallable
updateBackupScheduleCallable() {
return stub.updateBackupScheduleCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a backup schedule.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* BackupScheduleName name =
* BackupScheduleName.of("[PROJECT]", "[DATABASE]", "[BACKUP_SCHEDULE]");
* firestoreAdminClient.deleteBackupSchedule(name);
* }
* }
*
* @param name Required. The name of the backup schedule.
* Format `projects/{project}/databases/{database}/backupSchedules/{backup_schedule}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteBackupSchedule(BackupScheduleName name) {
DeleteBackupScheduleRequest request =
DeleteBackupScheduleRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
deleteBackupSchedule(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a backup schedule.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* String name =
* BackupScheduleName.of("[PROJECT]", "[DATABASE]", "[BACKUP_SCHEDULE]").toString();
* firestoreAdminClient.deleteBackupSchedule(name);
* }
* }
*
* @param name Required. The name of the backup schedule.
* Format `projects/{project}/databases/{database}/backupSchedules/{backup_schedule}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteBackupSchedule(String name) {
DeleteBackupScheduleRequest request =
DeleteBackupScheduleRequest.newBuilder().setName(name).build();
deleteBackupSchedule(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a backup schedule.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DeleteBackupScheduleRequest request =
* DeleteBackupScheduleRequest.newBuilder()
* .setName(
* BackupScheduleName.of("[PROJECT]", "[DATABASE]", "[BACKUP_SCHEDULE]").toString())
* .build();
* firestoreAdminClient.deleteBackupSchedule(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteBackupSchedule(DeleteBackupScheduleRequest request) {
deleteBackupScheduleCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a backup schedule.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (FirestoreAdminClient firestoreAdminClient = FirestoreAdminClient.create()) {
* DeleteBackupScheduleRequest request =
* DeleteBackupScheduleRequest.newBuilder()
* .setName(
* BackupScheduleName.of("[PROJECT]", "[DATABASE]", "[BACKUP_SCHEDULE]").toString())
* .build();
* ApiFuture future =
* firestoreAdminClient.deleteBackupScheduleCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteBackupScheduleCallable() {
return stub.deleteBackupScheduleCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListIndexesPagedResponse
extends AbstractPagedListResponse<
ListIndexesRequest,
ListIndexesResponse,
Index,
ListIndexesPage,
ListIndexesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListIndexesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListIndexesPagedResponse(input), MoreExecutors.directExecutor());
}
private ListIndexesPagedResponse(ListIndexesPage page) {
super(page, ListIndexesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListIndexesPage
extends AbstractPage {
private ListIndexesPage(
PageContext context,
ListIndexesResponse response) {
super(context, response);
}
private static ListIndexesPage createEmptyPage() {
return new ListIndexesPage(null, null);
}
@Override
protected ListIndexesPage createPage(
PageContext context,
ListIndexesResponse response) {
return new ListIndexesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListIndexesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListIndexesRequest,
ListIndexesResponse,
Index,
ListIndexesPage,
ListIndexesFixedSizeCollection> {
private ListIndexesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListIndexesFixedSizeCollection createEmptyCollection() {
return new ListIndexesFixedSizeCollection(null, 0);
}
@Override
protected ListIndexesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListIndexesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListFieldsPagedResponse
extends AbstractPagedListResponse<
ListFieldsRequest,
ListFieldsResponse,
Field,
ListFieldsPage,
ListFieldsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListFieldsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListFieldsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListFieldsPagedResponse(ListFieldsPage page) {
super(page, ListFieldsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListFieldsPage
extends AbstractPage {
private ListFieldsPage(
PageContext context,
ListFieldsResponse response) {
super(context, response);
}
private static ListFieldsPage createEmptyPage() {
return new ListFieldsPage(null, null);
}
@Override
protected ListFieldsPage createPage(
PageContext context,
ListFieldsResponse response) {
return new ListFieldsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListFieldsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListFieldsRequest,
ListFieldsResponse,
Field,
ListFieldsPage,
ListFieldsFixedSizeCollection> {
private ListFieldsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListFieldsFixedSizeCollection createEmptyCollection() {
return new ListFieldsFixedSizeCollection(null, 0);
}
@Override
protected ListFieldsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListFieldsFixedSizeCollection(pages, collectionSize);
}
}
}