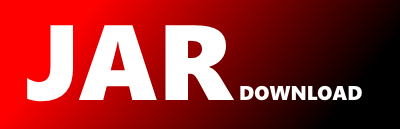
com.google.cloud.firestore.AutoValue_BulkWriterOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-cloud-firestore Show documentation
Show all versions of google-cloud-firestore Show documentation
Java idiomatic client for Google Cloud Firestore.
package com.google.cloud.firestore;
import java.util.concurrent.ScheduledExecutorService;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_BulkWriterOptions extends BulkWriterOptions {
private final boolean throttlingEnabled;
@Nullable
private final Double initialOpsPerSecond;
@Nullable
private final Double maxOpsPerSecond;
@Nullable
private final ScheduledExecutorService executor;
private AutoValue_BulkWriterOptions(
boolean throttlingEnabled,
@Nullable Double initialOpsPerSecond,
@Nullable Double maxOpsPerSecond,
@Nullable ScheduledExecutorService executor) {
this.throttlingEnabled = throttlingEnabled;
this.initialOpsPerSecond = initialOpsPerSecond;
this.maxOpsPerSecond = maxOpsPerSecond;
this.executor = executor;
}
@Override
public boolean getThrottlingEnabled() {
return throttlingEnabled;
}
@Nullable
@Override
public Double getInitialOpsPerSecond() {
return initialOpsPerSecond;
}
@Nullable
@Override
public Double getMaxOpsPerSecond() {
return maxOpsPerSecond;
}
@Nullable
@Override
public ScheduledExecutorService getExecutor() {
return executor;
}
@Override
public String toString() {
return "BulkWriterOptions{"
+ "throttlingEnabled=" + throttlingEnabled + ", "
+ "initialOpsPerSecond=" + initialOpsPerSecond + ", "
+ "maxOpsPerSecond=" + maxOpsPerSecond + ", "
+ "executor=" + executor
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof BulkWriterOptions) {
BulkWriterOptions that = (BulkWriterOptions) o;
return this.throttlingEnabled == that.getThrottlingEnabled()
&& (this.initialOpsPerSecond == null ? that.getInitialOpsPerSecond() == null : this.initialOpsPerSecond.equals(that.getInitialOpsPerSecond()))
&& (this.maxOpsPerSecond == null ? that.getMaxOpsPerSecond() == null : this.maxOpsPerSecond.equals(that.getMaxOpsPerSecond()))
&& (this.executor == null ? that.getExecutor() == null : this.executor.equals(that.getExecutor()));
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= throttlingEnabled ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (initialOpsPerSecond == null) ? 0 : initialOpsPerSecond.hashCode();
h$ *= 1000003;
h$ ^= (maxOpsPerSecond == null) ? 0 : maxOpsPerSecond.hashCode();
h$ *= 1000003;
h$ ^= (executor == null) ? 0 : executor.hashCode();
return h$;
}
@Override
public BulkWriterOptions.Builder toBuilder() {
return new AutoValue_BulkWriterOptions.Builder(this);
}
static final class Builder extends BulkWriterOptions.Builder {
private boolean throttlingEnabled;
private Double initialOpsPerSecond;
private Double maxOpsPerSecond;
private ScheduledExecutorService executor;
private byte set$0;
Builder() {
}
Builder(BulkWriterOptions source) {
this.throttlingEnabled = source.getThrottlingEnabled();
this.initialOpsPerSecond = source.getInitialOpsPerSecond();
this.maxOpsPerSecond = source.getMaxOpsPerSecond();
this.executor = source.getExecutor();
set$0 = (byte) 1;
}
@Override
public BulkWriterOptions.Builder setThrottlingEnabled(boolean throttlingEnabled) {
this.throttlingEnabled = throttlingEnabled;
set$0 |= (byte) 1;
return this;
}
@Override
BulkWriterOptions.Builder setInitialOpsPerSecond(@Nullable Double initialOpsPerSecond) {
this.initialOpsPerSecond = initialOpsPerSecond;
return this;
}
@Override
BulkWriterOptions.Builder setMaxOpsPerSecond(@Nullable Double maxOpsPerSecond) {
this.maxOpsPerSecond = maxOpsPerSecond;
return this;
}
@Override
public BulkWriterOptions.Builder setExecutor(@Nullable ScheduledExecutorService executor) {
this.executor = executor;
return this;
}
@Override
public BulkWriterOptions autoBuild() {
if (set$0 != 1) {
String missing = " throttlingEnabled";
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_BulkWriterOptions(
this.throttlingEnabled,
this.initialOpsPerSecond,
this.maxOpsPerSecond,
this.executor);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy