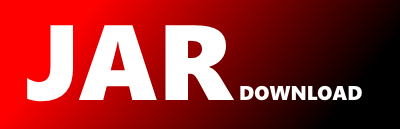
com.google.cloud.firestore.AutoValue_Query_QueryOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of google-cloud-firestore Show documentation
Show all versions of google-cloud-firestore Show documentation
Java idiomatic client for Google Cloud Firestore.
package com.google.cloud.firestore;
import com.google.common.collect.ImmutableList;
import com.google.firestore.v1.Cursor;
import com.google.firestore.v1.StructuredQuery;
import javax.annotation.Generated;
import javax.annotation.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_Query_QueryOptions extends Query.QueryOptions {
private final ResourcePath parentPath;
private final String collectionId;
private final boolean allDescendants;
@Nullable
private final Integer limit;
private final Query.LimitType limitType;
@Nullable
private final Integer offset;
@Nullable
private final Cursor startCursor;
@Nullable
private final Cursor endCursor;
private final ImmutableList filters;
private final ImmutableList fieldOrders;
private final ImmutableList fieldProjections;
private final boolean kindless;
private final boolean requireConsistency;
private AutoValue_Query_QueryOptions(
ResourcePath parentPath,
String collectionId,
boolean allDescendants,
@Nullable Integer limit,
Query.LimitType limitType,
@Nullable Integer offset,
@Nullable Cursor startCursor,
@Nullable Cursor endCursor,
ImmutableList filters,
ImmutableList fieldOrders,
ImmutableList fieldProjections,
boolean kindless,
boolean requireConsistency) {
this.parentPath = parentPath;
this.collectionId = collectionId;
this.allDescendants = allDescendants;
this.limit = limit;
this.limitType = limitType;
this.offset = offset;
this.startCursor = startCursor;
this.endCursor = endCursor;
this.filters = filters;
this.fieldOrders = fieldOrders;
this.fieldProjections = fieldProjections;
this.kindless = kindless;
this.requireConsistency = requireConsistency;
}
@Override
ResourcePath getParentPath() {
return parentPath;
}
@Override
String getCollectionId() {
return collectionId;
}
@Override
boolean getAllDescendants() {
return allDescendants;
}
@Nullable
@Override
Integer getLimit() {
return limit;
}
@Override
Query.LimitType getLimitType() {
return limitType;
}
@Nullable
@Override
Integer getOffset() {
return offset;
}
@Nullable
@Override
Cursor getStartCursor() {
return startCursor;
}
@Nullable
@Override
Cursor getEndCursor() {
return endCursor;
}
@Override
ImmutableList getFilters() {
return filters;
}
@Override
ImmutableList getFieldOrders() {
return fieldOrders;
}
@Override
ImmutableList getFieldProjections() {
return fieldProjections;
}
@Override
boolean isKindless() {
return kindless;
}
@Override
boolean getRequireConsistency() {
return requireConsistency;
}
@Override
public String toString() {
return "QueryOptions{"
+ "parentPath=" + parentPath + ", "
+ "collectionId=" + collectionId + ", "
+ "allDescendants=" + allDescendants + ", "
+ "limit=" + limit + ", "
+ "limitType=" + limitType + ", "
+ "offset=" + offset + ", "
+ "startCursor=" + startCursor + ", "
+ "endCursor=" + endCursor + ", "
+ "filters=" + filters + ", "
+ "fieldOrders=" + fieldOrders + ", "
+ "fieldProjections=" + fieldProjections + ", "
+ "kindless=" + kindless + ", "
+ "requireConsistency=" + requireConsistency
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof Query.QueryOptions) {
Query.QueryOptions that = (Query.QueryOptions) o;
return this.parentPath.equals(that.getParentPath())
&& this.collectionId.equals(that.getCollectionId())
&& this.allDescendants == that.getAllDescendants()
&& (this.limit == null ? that.getLimit() == null : this.limit.equals(that.getLimit()))
&& this.limitType.equals(that.getLimitType())
&& (this.offset == null ? that.getOffset() == null : this.offset.equals(that.getOffset()))
&& (this.startCursor == null ? that.getStartCursor() == null : this.startCursor.equals(that.getStartCursor()))
&& (this.endCursor == null ? that.getEndCursor() == null : this.endCursor.equals(that.getEndCursor()))
&& this.filters.equals(that.getFilters())
&& this.fieldOrders.equals(that.getFieldOrders())
&& this.fieldProjections.equals(that.getFieldProjections())
&& this.kindless == that.isKindless()
&& this.requireConsistency == that.getRequireConsistency();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= parentPath.hashCode();
h$ *= 1000003;
h$ ^= collectionId.hashCode();
h$ *= 1000003;
h$ ^= allDescendants ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (limit == null) ? 0 : limit.hashCode();
h$ *= 1000003;
h$ ^= limitType.hashCode();
h$ *= 1000003;
h$ ^= (offset == null) ? 0 : offset.hashCode();
h$ *= 1000003;
h$ ^= (startCursor == null) ? 0 : startCursor.hashCode();
h$ *= 1000003;
h$ ^= (endCursor == null) ? 0 : endCursor.hashCode();
h$ *= 1000003;
h$ ^= filters.hashCode();
h$ *= 1000003;
h$ ^= fieldOrders.hashCode();
h$ *= 1000003;
h$ ^= fieldProjections.hashCode();
h$ *= 1000003;
h$ ^= kindless ? 1231 : 1237;
h$ *= 1000003;
h$ ^= requireConsistency ? 1231 : 1237;
return h$;
}
@Override
Query.QueryOptions.Builder toBuilder() {
return new AutoValue_Query_QueryOptions.Builder(this);
}
static final class Builder extends Query.QueryOptions.Builder {
private ResourcePath parentPath;
private String collectionId;
private boolean allDescendants;
private Integer limit;
private Query.LimitType limitType;
private Integer offset;
private Cursor startCursor;
private Cursor endCursor;
private ImmutableList filters;
private ImmutableList fieldOrders;
private ImmutableList fieldProjections;
private boolean kindless;
private boolean requireConsistency;
private byte set$0;
Builder() {
}
Builder(Query.QueryOptions source) {
this.parentPath = source.getParentPath();
this.collectionId = source.getCollectionId();
this.allDescendants = source.getAllDescendants();
this.limit = source.getLimit();
this.limitType = source.getLimitType();
this.offset = source.getOffset();
this.startCursor = source.getStartCursor();
this.endCursor = source.getEndCursor();
this.filters = source.getFilters();
this.fieldOrders = source.getFieldOrders();
this.fieldProjections = source.getFieldProjections();
this.kindless = source.isKindless();
this.requireConsistency = source.getRequireConsistency();
set$0 = (byte) 7;
}
@Override
Query.QueryOptions.Builder setParentPath(ResourcePath parentPath) {
if (parentPath == null) {
throw new NullPointerException("Null parentPath");
}
this.parentPath = parentPath;
return this;
}
@Override
Query.QueryOptions.Builder setCollectionId(String collectionId) {
if (collectionId == null) {
throw new NullPointerException("Null collectionId");
}
this.collectionId = collectionId;
return this;
}
@Override
Query.QueryOptions.Builder setAllDescendants(boolean allDescendants) {
this.allDescendants = allDescendants;
set$0 |= (byte) 1;
return this;
}
@Override
Query.QueryOptions.Builder setLimit(Integer limit) {
this.limit = limit;
return this;
}
@Override
Query.QueryOptions.Builder setLimitType(Query.LimitType limitType) {
if (limitType == null) {
throw new NullPointerException("Null limitType");
}
this.limitType = limitType;
return this;
}
@Override
Query.QueryOptions.Builder setOffset(Integer offset) {
this.offset = offset;
return this;
}
@Override
Query.QueryOptions.Builder setStartCursor(@Nullable Cursor startCursor) {
this.startCursor = startCursor;
return this;
}
@Override
Query.QueryOptions.Builder setEndCursor(@Nullable Cursor endCursor) {
this.endCursor = endCursor;
return this;
}
@Override
Query.QueryOptions.Builder setFilters(ImmutableList filters) {
if (filters == null) {
throw new NullPointerException("Null filters");
}
this.filters = filters;
return this;
}
@Override
Query.QueryOptions.Builder setFieldOrders(ImmutableList fieldOrders) {
if (fieldOrders == null) {
throw new NullPointerException("Null fieldOrders");
}
this.fieldOrders = fieldOrders;
return this;
}
@Override
Query.QueryOptions.Builder setFieldProjections(ImmutableList fieldProjections) {
if (fieldProjections == null) {
throw new NullPointerException("Null fieldProjections");
}
this.fieldProjections = fieldProjections;
return this;
}
@Override
Query.QueryOptions.Builder setKindless(boolean kindless) {
this.kindless = kindless;
set$0 |= (byte) 2;
return this;
}
@Override
Query.QueryOptions.Builder setRequireConsistency(boolean requireConsistency) {
this.requireConsistency = requireConsistency;
set$0 |= (byte) 4;
return this;
}
@Override
Query.QueryOptions build() {
if (set$0 != 7
|| this.parentPath == null
|| this.collectionId == null
|| this.limitType == null
|| this.filters == null
|| this.fieldOrders == null
|| this.fieldProjections == null) {
StringBuilder missing = new StringBuilder();
if (this.parentPath == null) {
missing.append(" parentPath");
}
if (this.collectionId == null) {
missing.append(" collectionId");
}
if ((set$0 & 1) == 0) {
missing.append(" allDescendants");
}
if (this.limitType == null) {
missing.append(" limitType");
}
if (this.filters == null) {
missing.append(" filters");
}
if (this.fieldOrders == null) {
missing.append(" fieldOrders");
}
if (this.fieldProjections == null) {
missing.append(" fieldProjections");
}
if ((set$0 & 2) == 0) {
missing.append(" kindless");
}
if ((set$0 & 4) == 0) {
missing.append(" requireConsistency");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_Query_QueryOptions(
this.parentPath,
this.collectionId,
this.allDescendants,
this.limit,
this.limitType,
this.offset,
this.startCursor,
this.endCursor,
this.filters,
this.fieldOrders,
this.fieldProjections,
this.kindless,
this.requireConsistency);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy