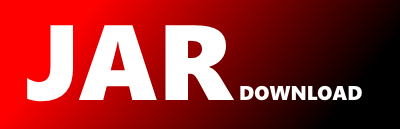
com.google.cloud.gkebackup.v1.BackupForGKEClient Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.gkebackup.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.gkebackup.v1.stub.BackupForGKEStub;
import com.google.cloud.gkebackup.v1.stub.BackupForGKEStubSettings;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: BackupForGKE allows Kubernetes administrators to configure, execute, and
* manage backup and restore operations for their GKE clusters.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupPlanName name = BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]");
* BackupPlan response = backupForGKEClient.getBackupPlan(name);
* }
* }
*
* Note: close() needs to be called on the BackupForGKEClient object to clean up resources such
* as threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* CreateBackupPlan
* Creates a new BackupPlan in a given location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createBackupPlanAsync(CreateBackupPlanRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createBackupPlanAsync(LocationName parent, BackupPlan backupPlan, String backupPlanId)
*
createBackupPlanAsync(String parent, BackupPlan backupPlan, String backupPlanId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createBackupPlanOperationCallable()
*
createBackupPlanCallable()
*
*
*
*
* ListBackupPlans
* Lists BackupPlans in a given location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listBackupPlans(ListBackupPlansRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listBackupPlans(LocationName parent)
*
listBackupPlans(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listBackupPlansPagedCallable()
*
listBackupPlansCallable()
*
*
*
*
* GetBackupPlan
* Retrieve the details of a single BackupPlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getBackupPlan(GetBackupPlanRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getBackupPlan(BackupPlanName name)
*
getBackupPlan(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getBackupPlanCallable()
*
*
*
*
* UpdateBackupPlan
* Update a BackupPlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateBackupPlanAsync(UpdateBackupPlanRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateBackupPlanAsync(BackupPlan backupPlan, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateBackupPlanOperationCallable()
*
updateBackupPlanCallable()
*
*
*
*
* DeleteBackupPlan
* Deletes an existing BackupPlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteBackupPlanAsync(DeleteBackupPlanRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteBackupPlanAsync(BackupPlanName name)
*
deleteBackupPlanAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteBackupPlanOperationCallable()
*
deleteBackupPlanCallable()
*
*
*
*
* CreateBackup
* Creates a Backup for the given BackupPlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createBackupAsync(CreateBackupRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createBackupAsync(BackupPlanName parent, Backup backup, String backupId)
*
createBackupAsync(String parent, Backup backup, String backupId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createBackupOperationCallable()
*
createBackupCallable()
*
*
*
*
* ListBackups
* Lists the Backups for a given BackupPlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listBackups(ListBackupsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listBackups(BackupPlanName parent)
*
listBackups(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listBackupsPagedCallable()
*
listBackupsCallable()
*
*
*
*
* GetBackup
* Retrieve the details of a single Backup.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getBackup(GetBackupRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getBackup(BackupName name)
*
getBackup(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getBackupCallable()
*
*
*
*
* UpdateBackup
* Update a Backup.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateBackupAsync(UpdateBackupRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateBackupAsync(Backup backup, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateBackupOperationCallable()
*
updateBackupCallable()
*
*
*
*
* DeleteBackup
* Deletes an existing Backup.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteBackupAsync(DeleteBackupRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteBackupAsync(BackupName name)
*
deleteBackupAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteBackupOperationCallable()
*
deleteBackupCallable()
*
*
*
*
* ListVolumeBackups
* Lists the VolumeBackups for a given Backup.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listVolumeBackups(ListVolumeBackupsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listVolumeBackups(BackupName parent)
*
listVolumeBackups(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listVolumeBackupsPagedCallable()
*
listVolumeBackupsCallable()
*
*
*
*
* GetVolumeBackup
* Retrieve the details of a single VolumeBackup.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getVolumeBackup(GetVolumeBackupRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getVolumeBackup(VolumeBackupName name)
*
getVolumeBackup(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getVolumeBackupCallable()
*
*
*
*
* CreateRestorePlan
* Creates a new RestorePlan in a given location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createRestorePlanAsync(CreateRestorePlanRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createRestorePlanAsync(LocationName parent, RestorePlan restorePlan, String restorePlanId)
*
createRestorePlanAsync(String parent, RestorePlan restorePlan, String restorePlanId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createRestorePlanOperationCallable()
*
createRestorePlanCallable()
*
*
*
*
* ListRestorePlans
* Lists RestorePlans in a given location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listRestorePlans(ListRestorePlansRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listRestorePlans(LocationName parent)
*
listRestorePlans(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listRestorePlansPagedCallable()
*
listRestorePlansCallable()
*
*
*
*
* GetRestorePlan
* Retrieve the details of a single RestorePlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getRestorePlan(GetRestorePlanRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getRestorePlan(RestorePlanName name)
*
getRestorePlan(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getRestorePlanCallable()
*
*
*
*
* UpdateRestorePlan
* Update a RestorePlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateRestorePlanAsync(UpdateRestorePlanRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateRestorePlanAsync(RestorePlan restorePlan, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateRestorePlanOperationCallable()
*
updateRestorePlanCallable()
*
*
*
*
* DeleteRestorePlan
* Deletes an existing RestorePlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteRestorePlanAsync(DeleteRestorePlanRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteRestorePlanAsync(RestorePlanName name)
*
deleteRestorePlanAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteRestorePlanOperationCallable()
*
deleteRestorePlanCallable()
*
*
*
*
* CreateRestore
* Creates a new Restore for the given RestorePlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createRestoreAsync(CreateRestoreRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createRestoreAsync(RestorePlanName parent, Restore restore, String restoreId)
*
createRestoreAsync(String parent, Restore restore, String restoreId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createRestoreOperationCallable()
*
createRestoreCallable()
*
*
*
*
* ListRestores
* Lists the Restores for a given RestorePlan.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listRestores(ListRestoresRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listRestores(RestorePlanName parent)
*
listRestores(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listRestoresPagedCallable()
*
listRestoresCallable()
*
*
*
*
* GetRestore
* Retrieves the details of a single Restore.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getRestore(GetRestoreRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getRestore(RestoreName name)
*
getRestore(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getRestoreCallable()
*
*
*
*
* UpdateRestore
* Update a Restore.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateRestoreAsync(UpdateRestoreRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateRestoreAsync(Restore restore, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateRestoreOperationCallable()
*
updateRestoreCallable()
*
*
*
*
* DeleteRestore
* Deletes an existing Restore.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteRestoreAsync(DeleteRestoreRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteRestoreAsync(RestoreName name)
*
deleteRestoreAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteRestoreOperationCallable()
*
deleteRestoreCallable()
*
*
*
*
* ListVolumeRestores
* Lists the VolumeRestores for a given Restore.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listVolumeRestores(ListVolumeRestoresRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listVolumeRestores(RestoreName parent)
*
listVolumeRestores(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listVolumeRestoresPagedCallable()
*
listVolumeRestoresCallable()
*
*
*
*
* GetVolumeRestore
* Retrieve the details of a single VolumeRestore.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getVolumeRestore(GetVolumeRestoreRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getVolumeRestore(VolumeRestoreName name)
*
getVolumeRestore(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getVolumeRestoreCallable()
*
*
*
*
* GetBackupIndexDownloadUrl
* Retrieve the link to the backupIndex.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getBackupIndexDownloadUrl(GetBackupIndexDownloadUrlRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getBackupIndexDownloadUrl(BackupName backup)
*
getBackupIndexDownloadUrl(String backup)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getBackupIndexDownloadUrlCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listLocationsPagedCallable()
*
listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getLocationCallable()
*
*
*
*
* SetIamPolicy
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* setIamPolicy(SetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* setIamPolicyCallable()
*
*
*
*
* GetIamPolicy
* Gets the access control policy for a resource. Returns an empty policyif the resource exists and does not have a policy set.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getIamPolicy(GetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getIamPolicyCallable()
*
*
*
*
* TestIamPermissions
* Returns permissions that a caller has on the specified resource. If theresource does not exist, this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
Note: This operation is designed to be used for buildingpermission-aware UIs and command-line tools, not for authorizationchecking. This operation may "fail open" without warning.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* testIamPermissions(TestIamPermissionsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* testIamPermissionsCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of BackupForGKESettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* BackupForGKESettings backupForGKESettings =
* BackupForGKESettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* BackupForGKEClient backupForGKEClient = BackupForGKEClient.create(backupForGKESettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* BackupForGKESettings backupForGKESettings =
* BackupForGKESettings.newBuilder().setEndpoint(myEndpoint).build();
* BackupForGKEClient backupForGKEClient = BackupForGKEClient.create(backupForGKESettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* BackupForGKESettings backupForGKESettings = BackupForGKESettings.newHttpJsonBuilder().build();
* BackupForGKEClient backupForGKEClient = BackupForGKEClient.create(backupForGKESettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class BackupForGKEClient implements BackgroundResource {
private final BackupForGKESettings settings;
private final BackupForGKEStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of BackupForGKEClient with default settings. */
public static final BackupForGKEClient create() throws IOException {
return create(BackupForGKESettings.newBuilder().build());
}
/**
* Constructs an instance of BackupForGKEClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final BackupForGKEClient create(BackupForGKESettings settings) throws IOException {
return new BackupForGKEClient(settings);
}
/**
* Constructs an instance of BackupForGKEClient, using the given stub for making calls. This is
* for advanced usage - prefer using create(BackupForGKESettings).
*/
public static final BackupForGKEClient create(BackupForGKEStub stub) {
return new BackupForGKEClient(stub);
}
/**
* Constructs an instance of BackupForGKEClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected BackupForGKEClient(BackupForGKESettings settings) throws IOException {
this.settings = settings;
this.stub = ((BackupForGKEStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected BackupForGKEClient(BackupForGKEStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final BackupForGKESettings getSettings() {
return settings;
}
public BackupForGKEStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new BackupPlan in a given location.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* BackupPlan backupPlan = BackupPlan.newBuilder().build();
* String backupPlanId = "backupPlanId-84871546";
* BackupPlan response =
* backupForGKEClient.createBackupPlanAsync(parent, backupPlan, backupPlanId).get();
* }
* }
*
* @param parent Required. The location within which to create the BackupPlan. Format:
* `projects/*/locations/*`
* @param backupPlan Required. The BackupPlan resource object to create.
* @param backupPlanId Required. The client-provided short name for the BackupPlan resource. This
* name must:
* - be between 1 and 63 characters long (inclusive) - consist of only lower-case ASCII
* letters, numbers, and dashes - start with a lower-case letter - end with a lower-case
* letter or number - be unique within the set of BackupPlans in this location
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createBackupPlanAsync(
LocationName parent, BackupPlan backupPlan, String backupPlanId) {
CreateBackupPlanRequest request =
CreateBackupPlanRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setBackupPlan(backupPlan)
.setBackupPlanId(backupPlanId)
.build();
return createBackupPlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new BackupPlan in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* BackupPlan backupPlan = BackupPlan.newBuilder().build();
* String backupPlanId = "backupPlanId-84871546";
* BackupPlan response =
* backupForGKEClient.createBackupPlanAsync(parent, backupPlan, backupPlanId).get();
* }
* }
*
* @param parent Required. The location within which to create the BackupPlan. Format:
* `projects/*/locations/*`
* @param backupPlan Required. The BackupPlan resource object to create.
* @param backupPlanId Required. The client-provided short name for the BackupPlan resource. This
* name must:
* - be between 1 and 63 characters long (inclusive) - consist of only lower-case ASCII
* letters, numbers, and dashes - start with a lower-case letter - end with a lower-case
* letter or number - be unique within the set of BackupPlans in this location
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createBackupPlanAsync(
String parent, BackupPlan backupPlan, String backupPlanId) {
CreateBackupPlanRequest request =
CreateBackupPlanRequest.newBuilder()
.setParent(parent)
.setBackupPlan(backupPlan)
.setBackupPlanId(backupPlanId)
.build();
return createBackupPlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new BackupPlan in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateBackupPlanRequest request =
* CreateBackupPlanRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setBackupPlan(BackupPlan.newBuilder().build())
* .setBackupPlanId("backupPlanId-84871546")
* .build();
* BackupPlan response = backupForGKEClient.createBackupPlanAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createBackupPlanAsync(
CreateBackupPlanRequest request) {
return createBackupPlanOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new BackupPlan in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateBackupPlanRequest request =
* CreateBackupPlanRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setBackupPlan(BackupPlan.newBuilder().build())
* .setBackupPlanId("backupPlanId-84871546")
* .build();
* OperationFuture future =
* backupForGKEClient.createBackupPlanOperationCallable().futureCall(request);
* // Do something.
* BackupPlan response = future.get();
* }
* }
*/
public final OperationCallable
createBackupPlanOperationCallable() {
return stub.createBackupPlanOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new BackupPlan in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateBackupPlanRequest request =
* CreateBackupPlanRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setBackupPlan(BackupPlan.newBuilder().build())
* .setBackupPlanId("backupPlanId-84871546")
* .build();
* ApiFuture future =
* backupForGKEClient.createBackupPlanCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createBackupPlanCallable() {
return stub.createBackupPlanCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists BackupPlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (BackupPlan element : backupForGKEClient.listBackupPlans(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The location that contains the BackupPlans to list. Format:
* `projects/*/locations/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupPlansPagedResponse listBackupPlans(LocationName parent) {
ListBackupPlansRequest request =
ListBackupPlansRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listBackupPlans(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists BackupPlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (BackupPlan element : backupForGKEClient.listBackupPlans(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The location that contains the BackupPlans to list. Format:
* `projects/*/locations/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupPlansPagedResponse listBackupPlans(String parent) {
ListBackupPlansRequest request = ListBackupPlansRequest.newBuilder().setParent(parent).build();
return listBackupPlans(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists BackupPlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListBackupPlansRequest request =
* ListBackupPlansRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (BackupPlan element : backupForGKEClient.listBackupPlans(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupPlansPagedResponse listBackupPlans(ListBackupPlansRequest request) {
return listBackupPlansPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists BackupPlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListBackupPlansRequest request =
* ListBackupPlansRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* backupForGKEClient.listBackupPlansPagedCallable().futureCall(request);
* // Do something.
* for (BackupPlan element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listBackupPlansPagedCallable() {
return stub.listBackupPlansPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists BackupPlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListBackupPlansRequest request =
* ListBackupPlansRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListBackupPlansResponse response =
* backupForGKEClient.listBackupPlansCallable().call(request);
* for (BackupPlan element : response.getBackupPlansList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listBackupPlansCallable() {
return stub.listBackupPlansCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupPlanName name = BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]");
* BackupPlan response = backupForGKEClient.getBackupPlan(name);
* }
* }
*
* @param name Required. Fully qualified BackupPlan name. Format:
* `projects/*/locations/*/backupPlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupPlan getBackupPlan(BackupPlanName name) {
GetBackupPlanRequest request =
GetBackupPlanRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getBackupPlan(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name = BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString();
* BackupPlan response = backupForGKEClient.getBackupPlan(name);
* }
* }
*
* @param name Required. Fully qualified BackupPlan name. Format:
* `projects/*/locations/*/backupPlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupPlan getBackupPlan(String name) {
GetBackupPlanRequest request = GetBackupPlanRequest.newBuilder().setName(name).build();
return getBackupPlan(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetBackupPlanRequest request =
* GetBackupPlanRequest.newBuilder()
* .setName(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .build();
* BackupPlan response = backupForGKEClient.getBackupPlan(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final BackupPlan getBackupPlan(GetBackupPlanRequest request) {
return getBackupPlanCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetBackupPlanRequest request =
* GetBackupPlanRequest.newBuilder()
* .setName(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .build();
* ApiFuture future = backupForGKEClient.getBackupPlanCallable().futureCall(request);
* // Do something.
* BackupPlan response = future.get();
* }
* }
*/
public final UnaryCallable getBackupPlanCallable() {
return stub.getBackupPlanCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupPlan backupPlan = BackupPlan.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* BackupPlan response = backupForGKEClient.updateBackupPlanAsync(backupPlan, updateMask).get();
* }
* }
*
* @param backupPlan Required. A new version of the BackupPlan resource that contains updated
* fields. This may be sparsely populated if an `update_mask` is provided.
* @param updateMask Optional. This is used to specify the fields to be overwritten in the
* BackupPlan targeted for update. The values for each of these updated fields will be taken
* from the `backup_plan` provided with this request. Field names are relative to the root of
* the resource (e.g., `description`, `backup_config.include_volume_data`, etc.) If no
* `update_mask` is provided, all fields in `backup_plan` will be written to the target
* BackupPlan resource. Note that OUTPUT_ONLY and IMMUTABLE fields in `backup_plan` are
* ignored and are not used to update the target BackupPlan.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateBackupPlanAsync(
BackupPlan backupPlan, FieldMask updateMask) {
UpdateBackupPlanRequest request =
UpdateBackupPlanRequest.newBuilder()
.setBackupPlan(backupPlan)
.setUpdateMask(updateMask)
.build();
return updateBackupPlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateBackupPlanRequest request =
* UpdateBackupPlanRequest.newBuilder()
* .setBackupPlan(BackupPlan.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* BackupPlan response = backupForGKEClient.updateBackupPlanAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateBackupPlanAsync(
UpdateBackupPlanRequest request) {
return updateBackupPlanOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateBackupPlanRequest request =
* UpdateBackupPlanRequest.newBuilder()
* .setBackupPlan(BackupPlan.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* OperationFuture future =
* backupForGKEClient.updateBackupPlanOperationCallable().futureCall(request);
* // Do something.
* BackupPlan response = future.get();
* }
* }
*/
public final OperationCallable
updateBackupPlanOperationCallable() {
return stub.updateBackupPlanOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateBackupPlanRequest request =
* UpdateBackupPlanRequest.newBuilder()
* .setBackupPlan(BackupPlan.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* backupForGKEClient.updateBackupPlanCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateBackupPlanCallable() {
return stub.updateBackupPlanCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupPlanName name = BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]");
* backupForGKEClient.deleteBackupPlanAsync(name).get();
* }
* }
*
* @param name Required. Fully qualified BackupPlan name. Format:
* `projects/*/locations/*/backupPlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteBackupPlanAsync(
BackupPlanName name) {
DeleteBackupPlanRequest request =
DeleteBackupPlanRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteBackupPlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name = BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString();
* backupForGKEClient.deleteBackupPlanAsync(name).get();
* }
* }
*
* @param name Required. Fully qualified BackupPlan name. Format:
* `projects/*/locations/*/backupPlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteBackupPlanAsync(String name) {
DeleteBackupPlanRequest request = DeleteBackupPlanRequest.newBuilder().setName(name).build();
return deleteBackupPlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteBackupPlanRequest request =
* DeleteBackupPlanRequest.newBuilder()
* .setName(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .setEtag("etag3123477")
* .build();
* backupForGKEClient.deleteBackupPlanAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteBackupPlanAsync(
DeleteBackupPlanRequest request) {
return deleteBackupPlanOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteBackupPlanRequest request =
* DeleteBackupPlanRequest.newBuilder()
* .setName(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .setEtag("etag3123477")
* .build();
* OperationFuture future =
* backupForGKEClient.deleteBackupPlanOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteBackupPlanOperationCallable() {
return stub.deleteBackupPlanOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteBackupPlanRequest request =
* DeleteBackupPlanRequest.newBuilder()
* .setName(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .setEtag("etag3123477")
* .build();
* ApiFuture future =
* backupForGKEClient.deleteBackupPlanCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteBackupPlanCallable() {
return stub.deleteBackupPlanCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Backup for the given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupPlanName parent = BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]");
* Backup backup = Backup.newBuilder().build();
* String backupId = "backupId2121930365";
* Backup response = backupForGKEClient.createBackupAsync(parent, backup, backupId).get();
* }
* }
*
* @param parent Required. The BackupPlan within which to create the Backup. Format:
* `projects/*/locations/*/backupPlans/*`
* @param backup Optional. The Backup resource to create.
* @param backupId Optional. The client-provided short name for the Backup resource. This name
* must:
* - be between 1 and 63 characters long (inclusive) - consist of only lower-case ASCII
* letters, numbers, and dashes - start with a lower-case letter - end with a lower-case
* letter or number - be unique within the set of Backups in this BackupPlan
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createBackupAsync(
BackupPlanName parent, Backup backup, String backupId) {
CreateBackupRequest request =
CreateBackupRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setBackup(backup)
.setBackupId(backupId)
.build();
return createBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Backup for the given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent = BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString();
* Backup backup = Backup.newBuilder().build();
* String backupId = "backupId2121930365";
* Backup response = backupForGKEClient.createBackupAsync(parent, backup, backupId).get();
* }
* }
*
* @param parent Required. The BackupPlan within which to create the Backup. Format:
* `projects/*/locations/*/backupPlans/*`
* @param backup Optional. The Backup resource to create.
* @param backupId Optional. The client-provided short name for the Backup resource. This name
* must:
* - be between 1 and 63 characters long (inclusive) - consist of only lower-case ASCII
* letters, numbers, and dashes - start with a lower-case letter - end with a lower-case
* letter or number - be unique within the set of Backups in this BackupPlan
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createBackupAsync(
String parent, Backup backup, String backupId) {
CreateBackupRequest request =
CreateBackupRequest.newBuilder()
.setParent(parent)
.setBackup(backup)
.setBackupId(backupId)
.build();
return createBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Backup for the given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateBackupRequest request =
* CreateBackupRequest.newBuilder()
* .setParent(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .setBackup(Backup.newBuilder().build())
* .setBackupId("backupId2121930365")
* .build();
* Backup response = backupForGKEClient.createBackupAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createBackupAsync(
CreateBackupRequest request) {
return createBackupOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Backup for the given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateBackupRequest request =
* CreateBackupRequest.newBuilder()
* .setParent(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .setBackup(Backup.newBuilder().build())
* .setBackupId("backupId2121930365")
* .build();
* OperationFuture future =
* backupForGKEClient.createBackupOperationCallable().futureCall(request);
* // Do something.
* Backup response = future.get();
* }
* }
*/
public final OperationCallable
createBackupOperationCallable() {
return stub.createBackupOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Backup for the given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateBackupRequest request =
* CreateBackupRequest.newBuilder()
* .setParent(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .setBackup(Backup.newBuilder().build())
* .setBackupId("backupId2121930365")
* .build();
* ApiFuture future = backupForGKEClient.createBackupCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createBackupCallable() {
return stub.createBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Backups for a given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupPlanName parent = BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]");
* for (Backup element : backupForGKEClient.listBackups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The BackupPlan that contains the Backups to list. Format:
* `projects/*/locations/*/backupPlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupsPagedResponse listBackups(BackupPlanName parent) {
ListBackupsRequest request =
ListBackupsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Backups for a given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent = BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString();
* for (Backup element : backupForGKEClient.listBackups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The BackupPlan that contains the Backups to list. Format:
* `projects/*/locations/*/backupPlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupsPagedResponse listBackups(String parent) {
ListBackupsRequest request = ListBackupsRequest.newBuilder().setParent(parent).build();
return listBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Backups for a given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListBackupsRequest request =
* ListBackupsRequest.newBuilder()
* .setParent(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Backup element : backupForGKEClient.listBackups(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBackupsPagedResponse listBackups(ListBackupsRequest request) {
return listBackupsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Backups for a given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListBackupsRequest request =
* ListBackupsRequest.newBuilder()
* .setParent(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future = backupForGKEClient.listBackupsPagedCallable().futureCall(request);
* // Do something.
* for (Backup element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listBackupsPagedCallable() {
return stub.listBackupsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Backups for a given BackupPlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListBackupsRequest request =
* ListBackupsRequest.newBuilder()
* .setParent(BackupPlanName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListBackupsResponse response = backupForGKEClient.listBackupsCallable().call(request);
* for (Backup element : response.getBackupsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listBackupsCallable() {
return stub.listBackupsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupName name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]");
* Backup response = backupForGKEClient.getBackup(name);
* }
* }
*
* @param name Required. Full name of the Backup resource. Format:
* `projects/*/locations/*/backupPlans/*/backups/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Backup getBackup(BackupName name) {
GetBackupRequest request =
GetBackupRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name =
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString();
* Backup response = backupForGKEClient.getBackup(name);
* }
* }
*
* @param name Required. Full name of the Backup resource. Format:
* `projects/*/locations/*/backupPlans/*/backups/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Backup getBackup(String name) {
GetBackupRequest request = GetBackupRequest.newBuilder().setName(name).build();
return getBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetBackupRequest request =
* GetBackupRequest.newBuilder()
* .setName(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .build();
* Backup response = backupForGKEClient.getBackup(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Backup getBackup(GetBackupRequest request) {
return getBackupCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetBackupRequest request =
* GetBackupRequest.newBuilder()
* .setName(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .build();
* ApiFuture future = backupForGKEClient.getBackupCallable().futureCall(request);
* // Do something.
* Backup response = future.get();
* }
* }
*/
public final UnaryCallable getBackupCallable() {
return stub.getBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* Backup backup = Backup.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Backup response = backupForGKEClient.updateBackupAsync(backup, updateMask).get();
* }
* }
*
* @param backup Required. A new version of the Backup resource that contains updated fields. This
* may be sparsely populated if an `update_mask` is provided.
* @param updateMask Optional. This is used to specify the fields to be overwritten in the Backup
* targeted for update. The values for each of these updated fields will be taken from the
* `backup_plan` provided with this request. Field names are relative to the root of the
* resource. If no `update_mask` is provided, all fields in `backup` will be written to the
* target Backup resource. Note that OUTPUT_ONLY and IMMUTABLE fields in `backup` are ignored
* and are not used to update the target Backup.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateBackupAsync(
Backup backup, FieldMask updateMask) {
UpdateBackupRequest request =
UpdateBackupRequest.newBuilder().setBackup(backup).setUpdateMask(updateMask).build();
return updateBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateBackupRequest request =
* UpdateBackupRequest.newBuilder()
* .setBackup(Backup.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Backup response = backupForGKEClient.updateBackupAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateBackupAsync(
UpdateBackupRequest request) {
return updateBackupOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateBackupRequest request =
* UpdateBackupRequest.newBuilder()
* .setBackup(Backup.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* OperationFuture future =
* backupForGKEClient.updateBackupOperationCallable().futureCall(request);
* // Do something.
* Backup response = future.get();
* }
* }
*/
public final OperationCallable
updateBackupOperationCallable() {
return stub.updateBackupOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateBackupRequest request =
* UpdateBackupRequest.newBuilder()
* .setBackup(Backup.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = backupForGKEClient.updateBackupCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateBackupCallable() {
return stub.updateBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupName name = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]");
* backupForGKEClient.deleteBackupAsync(name).get();
* }
* }
*
* @param name Required. Name of the Backup resource. Format:
* `projects/*/locations/*/backupPlans/*/backups/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteBackupAsync(BackupName name) {
DeleteBackupRequest request =
DeleteBackupRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name =
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString();
* backupForGKEClient.deleteBackupAsync(name).get();
* }
* }
*
* @param name Required. Name of the Backup resource. Format:
* `projects/*/locations/*/backupPlans/*/backups/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteBackupAsync(String name) {
DeleteBackupRequest request = DeleteBackupRequest.newBuilder().setName(name).build();
return deleteBackupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteBackupRequest request =
* DeleteBackupRequest.newBuilder()
* .setName(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* backupForGKEClient.deleteBackupAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteBackupAsync(
DeleteBackupRequest request) {
return deleteBackupOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteBackupRequest request =
* DeleteBackupRequest.newBuilder()
* .setName(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* OperationFuture future =
* backupForGKEClient.deleteBackupOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteBackupOperationCallable() {
return stub.deleteBackupOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteBackupRequest request =
* DeleteBackupRequest.newBuilder()
* .setName(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* ApiFuture future = backupForGKEClient.deleteBackupCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteBackupCallable() {
return stub.deleteBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeBackups for a given Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupName parent = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]");
* for (VolumeBackup element : backupForGKEClient.listVolumeBackups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The Backup that contains the VolumeBackups to list. Format:
* `projects/*/locations/*/backupPlans/*/backups/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumeBackupsPagedResponse listVolumeBackups(BackupName parent) {
ListVolumeBackupsRequest request =
ListVolumeBackupsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listVolumeBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeBackups for a given Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent =
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString();
* for (VolumeBackup element : backupForGKEClient.listVolumeBackups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The Backup that contains the VolumeBackups to list. Format:
* `projects/*/locations/*/backupPlans/*/backups/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumeBackupsPagedResponse listVolumeBackups(String parent) {
ListVolumeBackupsRequest request =
ListVolumeBackupsRequest.newBuilder().setParent(parent).build();
return listVolumeBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeBackups for a given Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListVolumeBackupsRequest request =
* ListVolumeBackupsRequest.newBuilder()
* .setParent(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (VolumeBackup element : backupForGKEClient.listVolumeBackups(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumeBackupsPagedResponse listVolumeBackups(ListVolumeBackupsRequest request) {
return listVolumeBackupsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeBackups for a given Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListVolumeBackupsRequest request =
* ListVolumeBackupsRequest.newBuilder()
* .setParent(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* backupForGKEClient.listVolumeBackupsPagedCallable().futureCall(request);
* // Do something.
* for (VolumeBackup element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listVolumeBackupsPagedCallable() {
return stub.listVolumeBackupsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeBackups for a given Backup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListVolumeBackupsRequest request =
* ListVolumeBackupsRequest.newBuilder()
* .setParent(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListVolumeBackupsResponse response =
* backupForGKEClient.listVolumeBackupsCallable().call(request);
* for (VolumeBackup element : response.getVolumeBackupsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listVolumeBackupsCallable() {
return stub.listVolumeBackupsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single VolumeBackup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* VolumeBackupName name =
* VolumeBackupName.of(
* "[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]", "[VOLUME_BACKUP]");
* VolumeBackup response = backupForGKEClient.getVolumeBackup(name);
* }
* }
*
* @param name Required. Full name of the VolumeBackup resource. Format:
* `projects/*/locations/*/backupPlans/*/backups/*/volumeBackups/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeBackup getVolumeBackup(VolumeBackupName name) {
GetVolumeBackupRequest request =
GetVolumeBackupRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getVolumeBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single VolumeBackup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name =
* VolumeBackupName.of(
* "[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]", "[VOLUME_BACKUP]")
* .toString();
* VolumeBackup response = backupForGKEClient.getVolumeBackup(name);
* }
* }
*
* @param name Required. Full name of the VolumeBackup resource. Format:
* `projects/*/locations/*/backupPlans/*/backups/*/volumeBackups/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeBackup getVolumeBackup(String name) {
GetVolumeBackupRequest request = GetVolumeBackupRequest.newBuilder().setName(name).build();
return getVolumeBackup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single VolumeBackup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetVolumeBackupRequest request =
* GetVolumeBackupRequest.newBuilder()
* .setName(
* VolumeBackupName.of(
* "[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]", "[VOLUME_BACKUP]")
* .toString())
* .build();
* VolumeBackup response = backupForGKEClient.getVolumeBackup(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeBackup getVolumeBackup(GetVolumeBackupRequest request) {
return getVolumeBackupCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single VolumeBackup.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetVolumeBackupRequest request =
* GetVolumeBackupRequest.newBuilder()
* .setName(
* VolumeBackupName.of(
* "[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]", "[VOLUME_BACKUP]")
* .toString())
* .build();
* ApiFuture future =
* backupForGKEClient.getVolumeBackupCallable().futureCall(request);
* // Do something.
* VolumeBackup response = future.get();
* }
* }
*/
public final UnaryCallable getVolumeBackupCallable() {
return stub.getVolumeBackupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new RestorePlan in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* RestorePlan restorePlan = RestorePlan.newBuilder().build();
* String restorePlanId = "restorePlanId-857896366";
* RestorePlan response =
* backupForGKEClient.createRestorePlanAsync(parent, restorePlan, restorePlanId).get();
* }
* }
*
* @param parent Required. The location within which to create the RestorePlan. Format:
* `projects/*/locations/*`
* @param restorePlan Required. The RestorePlan resource object to create.
* @param restorePlanId Required. The client-provided short name for the RestorePlan resource.
* This name must:
* - be between 1 and 63 characters long (inclusive) - consist of only lower-case ASCII
* letters, numbers, and dashes - start with a lower-case letter - end with a lower-case
* letter or number - be unique within the set of RestorePlans in this location
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createRestorePlanAsync(
LocationName parent, RestorePlan restorePlan, String restorePlanId) {
CreateRestorePlanRequest request =
CreateRestorePlanRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setRestorePlan(restorePlan)
.setRestorePlanId(restorePlanId)
.build();
return createRestorePlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new RestorePlan in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* RestorePlan restorePlan = RestorePlan.newBuilder().build();
* String restorePlanId = "restorePlanId-857896366";
* RestorePlan response =
* backupForGKEClient.createRestorePlanAsync(parent, restorePlan, restorePlanId).get();
* }
* }
*
* @param parent Required. The location within which to create the RestorePlan. Format:
* `projects/*/locations/*`
* @param restorePlan Required. The RestorePlan resource object to create.
* @param restorePlanId Required. The client-provided short name for the RestorePlan resource.
* This name must:
* - be between 1 and 63 characters long (inclusive) - consist of only lower-case ASCII
* letters, numbers, and dashes - start with a lower-case letter - end with a lower-case
* letter or number - be unique within the set of RestorePlans in this location
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createRestorePlanAsync(
String parent, RestorePlan restorePlan, String restorePlanId) {
CreateRestorePlanRequest request =
CreateRestorePlanRequest.newBuilder()
.setParent(parent)
.setRestorePlan(restorePlan)
.setRestorePlanId(restorePlanId)
.build();
return createRestorePlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new RestorePlan in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateRestorePlanRequest request =
* CreateRestorePlanRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setRestorePlan(RestorePlan.newBuilder().build())
* .setRestorePlanId("restorePlanId-857896366")
* .build();
* RestorePlan response = backupForGKEClient.createRestorePlanAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createRestorePlanAsync(
CreateRestorePlanRequest request) {
return createRestorePlanOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new RestorePlan in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateRestorePlanRequest request =
* CreateRestorePlanRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setRestorePlan(RestorePlan.newBuilder().build())
* .setRestorePlanId("restorePlanId-857896366")
* .build();
* OperationFuture future =
* backupForGKEClient.createRestorePlanOperationCallable().futureCall(request);
* // Do something.
* RestorePlan response = future.get();
* }
* }
*/
public final OperationCallable
createRestorePlanOperationCallable() {
return stub.createRestorePlanOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new RestorePlan in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateRestorePlanRequest request =
* CreateRestorePlanRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setRestorePlan(RestorePlan.newBuilder().build())
* .setRestorePlanId("restorePlanId-857896366")
* .build();
* ApiFuture future =
* backupForGKEClient.createRestorePlanCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createRestorePlanCallable() {
return stub.createRestorePlanCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists RestorePlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (RestorePlan element : backupForGKEClient.listRestorePlans(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The location that contains the RestorePlans to list. Format:
* `projects/*/locations/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRestorePlansPagedResponse listRestorePlans(LocationName parent) {
ListRestorePlansRequest request =
ListRestorePlansRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listRestorePlans(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists RestorePlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (RestorePlan element : backupForGKEClient.listRestorePlans(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The location that contains the RestorePlans to list. Format:
* `projects/*/locations/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRestorePlansPagedResponse listRestorePlans(String parent) {
ListRestorePlansRequest request =
ListRestorePlansRequest.newBuilder().setParent(parent).build();
return listRestorePlans(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists RestorePlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListRestorePlansRequest request =
* ListRestorePlansRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (RestorePlan element : backupForGKEClient.listRestorePlans(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRestorePlansPagedResponse listRestorePlans(ListRestorePlansRequest request) {
return listRestorePlansPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists RestorePlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListRestorePlansRequest request =
* ListRestorePlansRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* backupForGKEClient.listRestorePlansPagedCallable().futureCall(request);
* // Do something.
* for (RestorePlan element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listRestorePlansPagedCallable() {
return stub.listRestorePlansPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists RestorePlans in a given location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListRestorePlansRequest request =
* ListRestorePlansRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListRestorePlansResponse response =
* backupForGKEClient.listRestorePlansCallable().call(request);
* for (RestorePlan element : response.getRestorePlansList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listRestorePlansCallable() {
return stub.listRestorePlansCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* RestorePlanName name = RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]");
* RestorePlan response = backupForGKEClient.getRestorePlan(name);
* }
* }
*
* @param name Required. Fully qualified RestorePlan name. Format:
* `projects/*/locations/*/restorePlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RestorePlan getRestorePlan(RestorePlanName name) {
GetRestorePlanRequest request =
GetRestorePlanRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getRestorePlan(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name = RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString();
* RestorePlan response = backupForGKEClient.getRestorePlan(name);
* }
* }
*
* @param name Required. Fully qualified RestorePlan name. Format:
* `projects/*/locations/*/restorePlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RestorePlan getRestorePlan(String name) {
GetRestorePlanRequest request = GetRestorePlanRequest.newBuilder().setName(name).build();
return getRestorePlan(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetRestorePlanRequest request =
* GetRestorePlanRequest.newBuilder()
* .setName(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .build();
* RestorePlan response = backupForGKEClient.getRestorePlan(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RestorePlan getRestorePlan(GetRestorePlanRequest request) {
return getRestorePlanCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetRestorePlanRequest request =
* GetRestorePlanRequest.newBuilder()
* .setName(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .build();
* ApiFuture future =
* backupForGKEClient.getRestorePlanCallable().futureCall(request);
* // Do something.
* RestorePlan response = future.get();
* }
* }
*/
public final UnaryCallable getRestorePlanCallable() {
return stub.getRestorePlanCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* RestorePlan restorePlan = RestorePlan.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* RestorePlan response =
* backupForGKEClient.updateRestorePlanAsync(restorePlan, updateMask).get();
* }
* }
*
* @param restorePlan Required. A new version of the RestorePlan resource that contains updated
* fields. This may be sparsely populated if an `update_mask` is provided.
* @param updateMask Optional. This is used to specify the fields to be overwritten in the
* RestorePlan targeted for update. The values for each of these updated fields will be taken
* from the `restore_plan` provided with this request. Field names are relative to the root of
* the resource. If no `update_mask` is provided, all fields in `restore_plan` will be written
* to the target RestorePlan resource. Note that OUTPUT_ONLY and IMMUTABLE fields in
* `restore_plan` are ignored and are not used to update the target RestorePlan.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateRestorePlanAsync(
RestorePlan restorePlan, FieldMask updateMask) {
UpdateRestorePlanRequest request =
UpdateRestorePlanRequest.newBuilder()
.setRestorePlan(restorePlan)
.setUpdateMask(updateMask)
.build();
return updateRestorePlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateRestorePlanRequest request =
* UpdateRestorePlanRequest.newBuilder()
* .setRestorePlan(RestorePlan.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* RestorePlan response = backupForGKEClient.updateRestorePlanAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateRestorePlanAsync(
UpdateRestorePlanRequest request) {
return updateRestorePlanOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateRestorePlanRequest request =
* UpdateRestorePlanRequest.newBuilder()
* .setRestorePlan(RestorePlan.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* OperationFuture future =
* backupForGKEClient.updateRestorePlanOperationCallable().futureCall(request);
* // Do something.
* RestorePlan response = future.get();
* }
* }
*/
public final OperationCallable
updateRestorePlanOperationCallable() {
return stub.updateRestorePlanOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateRestorePlanRequest request =
* UpdateRestorePlanRequest.newBuilder()
* .setRestorePlan(RestorePlan.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* backupForGKEClient.updateRestorePlanCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateRestorePlanCallable() {
return stub.updateRestorePlanCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* RestorePlanName name = RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]");
* backupForGKEClient.deleteRestorePlanAsync(name).get();
* }
* }
*
* @param name Required. Fully qualified RestorePlan name. Format:
* `projects/*/locations/*/restorePlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteRestorePlanAsync(
RestorePlanName name) {
DeleteRestorePlanRequest request =
DeleteRestorePlanRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteRestorePlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name = RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString();
* backupForGKEClient.deleteRestorePlanAsync(name).get();
* }
* }
*
* @param name Required. Fully qualified RestorePlan name. Format:
* `projects/*/locations/*/restorePlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteRestorePlanAsync(String name) {
DeleteRestorePlanRequest request = DeleteRestorePlanRequest.newBuilder().setName(name).build();
return deleteRestorePlanAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteRestorePlanRequest request =
* DeleteRestorePlanRequest.newBuilder()
* .setName(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* backupForGKEClient.deleteRestorePlanAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteRestorePlanAsync(
DeleteRestorePlanRequest request) {
return deleteRestorePlanOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteRestorePlanRequest request =
* DeleteRestorePlanRequest.newBuilder()
* .setName(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* OperationFuture future =
* backupForGKEClient.deleteRestorePlanOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteRestorePlanOperationCallable() {
return stub.deleteRestorePlanOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteRestorePlanRequest request =
* DeleteRestorePlanRequest.newBuilder()
* .setName(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* ApiFuture future =
* backupForGKEClient.deleteRestorePlanCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteRestorePlanCallable() {
return stub.deleteRestorePlanCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Restore for the given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* RestorePlanName parent = RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]");
* Restore restore = Restore.newBuilder().build();
* String restoreId = "restoreId-1845465015";
* Restore response = backupForGKEClient.createRestoreAsync(parent, restore, restoreId).get();
* }
* }
*
* @param parent Required. The RestorePlan within which to create the Restore. Format:
* `projects/*/locations/*/restorePlans/*`
* @param restore Required. The restore resource to create.
* @param restoreId Required. The client-provided short name for the Restore resource. This name
* must:
* - be between 1 and 63 characters long (inclusive) - consist of only lower-case ASCII
* letters, numbers, and dashes - start with a lower-case letter - end with a lower-case
* letter or number - be unique within the set of Restores in this RestorePlan.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createRestoreAsync(
RestorePlanName parent, Restore restore, String restoreId) {
CreateRestoreRequest request =
CreateRestoreRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setRestore(restore)
.setRestoreId(restoreId)
.build();
return createRestoreAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Restore for the given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent = RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString();
* Restore restore = Restore.newBuilder().build();
* String restoreId = "restoreId-1845465015";
* Restore response = backupForGKEClient.createRestoreAsync(parent, restore, restoreId).get();
* }
* }
*
* @param parent Required. The RestorePlan within which to create the Restore. Format:
* `projects/*/locations/*/restorePlans/*`
* @param restore Required. The restore resource to create.
* @param restoreId Required. The client-provided short name for the Restore resource. This name
* must:
* - be between 1 and 63 characters long (inclusive) - consist of only lower-case ASCII
* letters, numbers, and dashes - start with a lower-case letter - end with a lower-case
* letter or number - be unique within the set of Restores in this RestorePlan.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createRestoreAsync(
String parent, Restore restore, String restoreId) {
CreateRestoreRequest request =
CreateRestoreRequest.newBuilder()
.setParent(parent)
.setRestore(restore)
.setRestoreId(restoreId)
.build();
return createRestoreAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Restore for the given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateRestoreRequest request =
* CreateRestoreRequest.newBuilder()
* .setParent(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .setRestore(Restore.newBuilder().build())
* .setRestoreId("restoreId-1845465015")
* .build();
* Restore response = backupForGKEClient.createRestoreAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createRestoreAsync(
CreateRestoreRequest request) {
return createRestoreOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Restore for the given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateRestoreRequest request =
* CreateRestoreRequest.newBuilder()
* .setParent(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .setRestore(Restore.newBuilder().build())
* .setRestoreId("restoreId-1845465015")
* .build();
* OperationFuture future =
* backupForGKEClient.createRestoreOperationCallable().futureCall(request);
* // Do something.
* Restore response = future.get();
* }
* }
*/
public final OperationCallable
createRestoreOperationCallable() {
return stub.createRestoreOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Restore for the given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* CreateRestoreRequest request =
* CreateRestoreRequest.newBuilder()
* .setParent(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .setRestore(Restore.newBuilder().build())
* .setRestoreId("restoreId-1845465015")
* .build();
* ApiFuture future = backupForGKEClient.createRestoreCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createRestoreCallable() {
return stub.createRestoreCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Restores for a given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* RestorePlanName parent = RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]");
* for (Restore element : backupForGKEClient.listRestores(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The RestorePlan that contains the Restores to list. Format:
* `projects/*/locations/*/restorePlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRestoresPagedResponse listRestores(RestorePlanName parent) {
ListRestoresRequest request =
ListRestoresRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listRestores(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Restores for a given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent = RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString();
* for (Restore element : backupForGKEClient.listRestores(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The RestorePlan that contains the Restores to list. Format:
* `projects/*/locations/*/restorePlans/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRestoresPagedResponse listRestores(String parent) {
ListRestoresRequest request = ListRestoresRequest.newBuilder().setParent(parent).build();
return listRestores(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Restores for a given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListRestoresRequest request =
* ListRestoresRequest.newBuilder()
* .setParent(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Restore element : backupForGKEClient.listRestores(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRestoresPagedResponse listRestores(ListRestoresRequest request) {
return listRestoresPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Restores for a given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListRestoresRequest request =
* ListRestoresRequest.newBuilder()
* .setParent(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* backupForGKEClient.listRestoresPagedCallable().futureCall(request);
* // Do something.
* for (Restore element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listRestoresPagedCallable() {
return stub.listRestoresPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Restores for a given RestorePlan.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListRestoresRequest request =
* ListRestoresRequest.newBuilder()
* .setParent(RestorePlanName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListRestoresResponse response = backupForGKEClient.listRestoresCallable().call(request);
* for (Restore element : response.getRestoresList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listRestoresCallable() {
return stub.listRestoresCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the details of a single Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* RestoreName name = RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]");
* Restore response = backupForGKEClient.getRestore(name);
* }
* }
*
* @param name Required. Name of the restore resource. Format:
* `projects/*/locations/*/restorePlans/*/restores/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Restore getRestore(RestoreName name) {
GetRestoreRequest request =
GetRestoreRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getRestore(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the details of a single Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name =
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]").toString();
* Restore response = backupForGKEClient.getRestore(name);
* }
* }
*
* @param name Required. Name of the restore resource. Format:
* `projects/*/locations/*/restorePlans/*/restores/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Restore getRestore(String name) {
GetRestoreRequest request = GetRestoreRequest.newBuilder().setName(name).build();
return getRestore(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the details of a single Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetRestoreRequest request =
* GetRestoreRequest.newBuilder()
* .setName(
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]")
* .toString())
* .build();
* Restore response = backupForGKEClient.getRestore(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Restore getRestore(GetRestoreRequest request) {
return getRestoreCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the details of a single Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetRestoreRequest request =
* GetRestoreRequest.newBuilder()
* .setName(
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]")
* .toString())
* .build();
* ApiFuture future = backupForGKEClient.getRestoreCallable().futureCall(request);
* // Do something.
* Restore response = future.get();
* }
* }
*/
public final UnaryCallable getRestoreCallable() {
return stub.getRestoreCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* Restore restore = Restore.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Restore response = backupForGKEClient.updateRestoreAsync(restore, updateMask).get();
* }
* }
*
* @param restore Required. A new version of the Restore resource that contains updated fields.
* This may be sparsely populated if an `update_mask` is provided.
* @param updateMask Optional. This is used to specify the fields to be overwritten in the Restore
* targeted for update. The values for each of these updated fields will be taken from the
* `restore` provided with this request. Field names are relative to the root of the resource.
* If no `update_mask` is provided, all fields in `restore` will be written to the target
* Restore resource. Note that OUTPUT_ONLY and IMMUTABLE fields in `restore` are ignored and
* are not used to update the target Restore.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateRestoreAsync(
Restore restore, FieldMask updateMask) {
UpdateRestoreRequest request =
UpdateRestoreRequest.newBuilder().setRestore(restore).setUpdateMask(updateMask).build();
return updateRestoreAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateRestoreRequest request =
* UpdateRestoreRequest.newBuilder()
* .setRestore(Restore.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Restore response = backupForGKEClient.updateRestoreAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateRestoreAsync(
UpdateRestoreRequest request) {
return updateRestoreOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateRestoreRequest request =
* UpdateRestoreRequest.newBuilder()
* .setRestore(Restore.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* OperationFuture future =
* backupForGKEClient.updateRestoreOperationCallable().futureCall(request);
* // Do something.
* Restore response = future.get();
* }
* }
*/
public final OperationCallable
updateRestoreOperationCallable() {
return stub.updateRestoreOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update a Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* UpdateRestoreRequest request =
* UpdateRestoreRequest.newBuilder()
* .setRestore(Restore.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = backupForGKEClient.updateRestoreCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateRestoreCallable() {
return stub.updateRestoreCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* RestoreName name = RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]");
* backupForGKEClient.deleteRestoreAsync(name).get();
* }
* }
*
* @param name Required. Full name of the Restore Format:
* `projects/*/locations/*/restorePlans/*/restores/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteRestoreAsync(RestoreName name) {
DeleteRestoreRequest request =
DeleteRestoreRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteRestoreAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name =
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]").toString();
* backupForGKEClient.deleteRestoreAsync(name).get();
* }
* }
*
* @param name Required. Full name of the Restore Format:
* `projects/*/locations/*/restorePlans/*/restores/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteRestoreAsync(String name) {
DeleteRestoreRequest request = DeleteRestoreRequest.newBuilder().setName(name).build();
return deleteRestoreAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteRestoreRequest request =
* DeleteRestoreRequest.newBuilder()
* .setName(
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]")
* .toString())
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* backupForGKEClient.deleteRestoreAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteRestoreAsync(
DeleteRestoreRequest request) {
return deleteRestoreOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteRestoreRequest request =
* DeleteRestoreRequest.newBuilder()
* .setName(
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]")
* .toString())
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* OperationFuture future =
* backupForGKEClient.deleteRestoreOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteRestoreOperationCallable() {
return stub.deleteRestoreOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* DeleteRestoreRequest request =
* DeleteRestoreRequest.newBuilder()
* .setName(
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]")
* .toString())
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* ApiFuture future = backupForGKEClient.deleteRestoreCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteRestoreCallable() {
return stub.deleteRestoreCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeRestores for a given Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* RestoreName parent = RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]");
* for (VolumeRestore element : backupForGKEClient.listVolumeRestores(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The Restore that contains the VolumeRestores to list. Format:
* `projects/*/locations/*/restorePlans/*/restores/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumeRestoresPagedResponse listVolumeRestores(RestoreName parent) {
ListVolumeRestoresRequest request =
ListVolumeRestoresRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listVolumeRestores(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeRestores for a given Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String parent =
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]").toString();
* for (VolumeRestore element : backupForGKEClient.listVolumeRestores(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The Restore that contains the VolumeRestores to list. Format:
* `projects/*/locations/*/restorePlans/*/restores/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumeRestoresPagedResponse listVolumeRestores(String parent) {
ListVolumeRestoresRequest request =
ListVolumeRestoresRequest.newBuilder().setParent(parent).build();
return listVolumeRestores(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeRestores for a given Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListVolumeRestoresRequest request =
* ListVolumeRestoresRequest.newBuilder()
* .setParent(
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (VolumeRestore element : backupForGKEClient.listVolumeRestores(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListVolumeRestoresPagedResponse listVolumeRestores(
ListVolumeRestoresRequest request) {
return listVolumeRestoresPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeRestores for a given Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListVolumeRestoresRequest request =
* ListVolumeRestoresRequest.newBuilder()
* .setParent(
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* backupForGKEClient.listVolumeRestoresPagedCallable().futureCall(request);
* // Do something.
* for (VolumeRestore element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listVolumeRestoresPagedCallable() {
return stub.listVolumeRestoresPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VolumeRestores for a given Restore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListVolumeRestoresRequest request =
* ListVolumeRestoresRequest.newBuilder()
* .setParent(
* RestoreName.of("[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListVolumeRestoresResponse response =
* backupForGKEClient.listVolumeRestoresCallable().call(request);
* for (VolumeRestore element : response.getVolumeRestoresList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listVolumeRestoresCallable() {
return stub.listVolumeRestoresCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single VolumeRestore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* VolumeRestoreName name =
* VolumeRestoreName.of(
* "[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]", "[VOLUME_RESTORE]");
* VolumeRestore response = backupForGKEClient.getVolumeRestore(name);
* }
* }
*
* @param name Required. Full name of the VolumeRestore resource. Format:
* `projects/*/locations/*/restorePlans/*/restores/*/volumeRestores/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeRestore getVolumeRestore(VolumeRestoreName name) {
GetVolumeRestoreRequest request =
GetVolumeRestoreRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getVolumeRestore(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single VolumeRestore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String name =
* VolumeRestoreName.of(
* "[PROJECT]", "[LOCATION]", "[RESTORE_PLAN]", "[RESTORE]", "[VOLUME_RESTORE]")
* .toString();
* VolumeRestore response = backupForGKEClient.getVolumeRestore(name);
* }
* }
*
* @param name Required. Full name of the VolumeRestore resource. Format:
* `projects/*/locations/*/restorePlans/*/restores/*/volumeRestores/*`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeRestore getVolumeRestore(String name) {
GetVolumeRestoreRequest request = GetVolumeRestoreRequest.newBuilder().setName(name).build();
return getVolumeRestore(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single VolumeRestore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetVolumeRestoreRequest request =
* GetVolumeRestoreRequest.newBuilder()
* .setName(
* VolumeRestoreName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[RESTORE_PLAN]",
* "[RESTORE]",
* "[VOLUME_RESTORE]")
* .toString())
* .build();
* VolumeRestore response = backupForGKEClient.getVolumeRestore(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final VolumeRestore getVolumeRestore(GetVolumeRestoreRequest request) {
return getVolumeRestoreCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the details of a single VolumeRestore.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetVolumeRestoreRequest request =
* GetVolumeRestoreRequest.newBuilder()
* .setName(
* VolumeRestoreName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[RESTORE_PLAN]",
* "[RESTORE]",
* "[VOLUME_RESTORE]")
* .toString())
* .build();
* ApiFuture future =
* backupForGKEClient.getVolumeRestoreCallable().futureCall(request);
* // Do something.
* VolumeRestore response = future.get();
* }
* }
*/
public final UnaryCallable getVolumeRestoreCallable() {
return stub.getVolumeRestoreCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the link to the backupIndex.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* BackupName backup = BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]");
* GetBackupIndexDownloadUrlResponse response =
* backupForGKEClient.getBackupIndexDownloadUrl(backup);
* }
* }
*
* @param backup Required. Full name of Backup resource. Format:
* projects/{project}/locations/{location}/backupPlans/{backup_plan}/backups/{backup}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetBackupIndexDownloadUrlResponse getBackupIndexDownloadUrl(BackupName backup) {
GetBackupIndexDownloadUrlRequest request =
GetBackupIndexDownloadUrlRequest.newBuilder()
.setBackup(backup == null ? null : backup.toString())
.build();
return getBackupIndexDownloadUrl(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the link to the backupIndex.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* String backup =
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString();
* GetBackupIndexDownloadUrlResponse response =
* backupForGKEClient.getBackupIndexDownloadUrl(backup);
* }
* }
*
* @param backup Required. Full name of Backup resource. Format:
* projects/{project}/locations/{location}/backupPlans/{backup_plan}/backups/{backup}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetBackupIndexDownloadUrlResponse getBackupIndexDownloadUrl(String backup) {
GetBackupIndexDownloadUrlRequest request =
GetBackupIndexDownloadUrlRequest.newBuilder().setBackup(backup).build();
return getBackupIndexDownloadUrl(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the link to the backupIndex.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetBackupIndexDownloadUrlRequest request =
* GetBackupIndexDownloadUrlRequest.newBuilder()
* .setBackup(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .build();
* GetBackupIndexDownloadUrlResponse response =
* backupForGKEClient.getBackupIndexDownloadUrl(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetBackupIndexDownloadUrlResponse getBackupIndexDownloadUrl(
GetBackupIndexDownloadUrlRequest request) {
return getBackupIndexDownloadUrlCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieve the link to the backupIndex.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetBackupIndexDownloadUrlRequest request =
* GetBackupIndexDownloadUrlRequest.newBuilder()
* .setBackup(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .build();
* ApiFuture future =
* backupForGKEClient.getBackupIndexDownloadUrlCallable().futureCall(request);
* // Do something.
* GetBackupIndexDownloadUrlResponse response = future.get();
* }
* }
*/
public final UnaryCallable
getBackupIndexDownloadUrlCallable() {
return stub.getBackupIndexDownloadUrlCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : backupForGKEClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* backupForGKEClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response = backupForGKEClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = backupForGKEClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = backupForGKEClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Policy response = backupForGKEClient.setIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(SetIamPolicyRequest request) {
return setIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = backupForGKEClient.setIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable setIamPolicyCallable() {
return stub.setIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* Policy response = backupForGKEClient.getIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(GetIamPolicyRequest request) {
return getIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* ApiFuture future = backupForGKEClient.getIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable getIamPolicyCallable() {
return stub.getIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* TestIamPermissionsResponse response = backupForGKEClient.testIamPermissions(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(TestIamPermissionsRequest request) {
return testIamPermissionsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (BackupForGKEClient backupForGKEClient = BackupForGKEClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(
* BackupName.of("[PROJECT]", "[LOCATION]", "[BACKUP_PLAN]", "[BACKUP]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* ApiFuture future =
* backupForGKEClient.testIamPermissionsCallable().futureCall(request);
* // Do something.
* TestIamPermissionsResponse response = future.get();
* }
* }
*/
public final UnaryCallable
testIamPermissionsCallable() {
return stub.testIamPermissionsCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListBackupPlansPagedResponse
extends AbstractPagedListResponse<
ListBackupPlansRequest,
ListBackupPlansResponse,
BackupPlan,
ListBackupPlansPage,
ListBackupPlansFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListBackupPlansPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListBackupPlansPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListBackupPlansPagedResponse(ListBackupPlansPage page) {
super(page, ListBackupPlansFixedSizeCollection.createEmptyCollection());
}
}
public static class ListBackupPlansPage
extends AbstractPage<
ListBackupPlansRequest, ListBackupPlansResponse, BackupPlan, ListBackupPlansPage> {
private ListBackupPlansPage(
PageContext context,
ListBackupPlansResponse response) {
super(context, response);
}
private static ListBackupPlansPage createEmptyPage() {
return new ListBackupPlansPage(null, null);
}
@Override
protected ListBackupPlansPage createPage(
PageContext context,
ListBackupPlansResponse response) {
return new ListBackupPlansPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListBackupPlansFixedSizeCollection
extends AbstractFixedSizeCollection<
ListBackupPlansRequest,
ListBackupPlansResponse,
BackupPlan,
ListBackupPlansPage,
ListBackupPlansFixedSizeCollection> {
private ListBackupPlansFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListBackupPlansFixedSizeCollection createEmptyCollection() {
return new ListBackupPlansFixedSizeCollection(null, 0);
}
@Override
protected ListBackupPlansFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListBackupPlansFixedSizeCollection(pages, collectionSize);
}
}
public static class ListBackupsPagedResponse
extends AbstractPagedListResponse<
ListBackupsRequest,
ListBackupsResponse,
Backup,
ListBackupsPage,
ListBackupsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListBackupsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListBackupsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListBackupsPagedResponse(ListBackupsPage page) {
super(page, ListBackupsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListBackupsPage
extends AbstractPage {
private ListBackupsPage(
PageContext context,
ListBackupsResponse response) {
super(context, response);
}
private static ListBackupsPage createEmptyPage() {
return new ListBackupsPage(null, null);
}
@Override
protected ListBackupsPage createPage(
PageContext context,
ListBackupsResponse response) {
return new ListBackupsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListBackupsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListBackupsRequest,
ListBackupsResponse,
Backup,
ListBackupsPage,
ListBackupsFixedSizeCollection> {
private ListBackupsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListBackupsFixedSizeCollection createEmptyCollection() {
return new ListBackupsFixedSizeCollection(null, 0);
}
@Override
protected ListBackupsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListBackupsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListVolumeBackupsPagedResponse
extends AbstractPagedListResponse<
ListVolumeBackupsRequest,
ListVolumeBackupsResponse,
VolumeBackup,
ListVolumeBackupsPage,
ListVolumeBackupsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListVolumeBackupsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListVolumeBackupsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListVolumeBackupsPagedResponse(ListVolumeBackupsPage page) {
super(page, ListVolumeBackupsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListVolumeBackupsPage
extends AbstractPage<
ListVolumeBackupsRequest,
ListVolumeBackupsResponse,
VolumeBackup,
ListVolumeBackupsPage> {
private ListVolumeBackupsPage(
PageContext context,
ListVolumeBackupsResponse response) {
super(context, response);
}
private static ListVolumeBackupsPage createEmptyPage() {
return new ListVolumeBackupsPage(null, null);
}
@Override
protected ListVolumeBackupsPage createPage(
PageContext context,
ListVolumeBackupsResponse response) {
return new ListVolumeBackupsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListVolumeBackupsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListVolumeBackupsRequest,
ListVolumeBackupsResponse,
VolumeBackup,
ListVolumeBackupsPage,
ListVolumeBackupsFixedSizeCollection> {
private ListVolumeBackupsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListVolumeBackupsFixedSizeCollection createEmptyCollection() {
return new ListVolumeBackupsFixedSizeCollection(null, 0);
}
@Override
protected ListVolumeBackupsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListVolumeBackupsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListRestorePlansPagedResponse
extends AbstractPagedListResponse<
ListRestorePlansRequest,
ListRestorePlansResponse,
RestorePlan,
ListRestorePlansPage,
ListRestorePlansFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListRestorePlansPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListRestorePlansPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListRestorePlansPagedResponse(ListRestorePlansPage page) {
super(page, ListRestorePlansFixedSizeCollection.createEmptyCollection());
}
}
public static class ListRestorePlansPage
extends AbstractPage<
ListRestorePlansRequest, ListRestorePlansResponse, RestorePlan, ListRestorePlansPage> {
private ListRestorePlansPage(
PageContext context,
ListRestorePlansResponse response) {
super(context, response);
}
private static ListRestorePlansPage createEmptyPage() {
return new ListRestorePlansPage(null, null);
}
@Override
protected ListRestorePlansPage createPage(
PageContext context,
ListRestorePlansResponse response) {
return new ListRestorePlansPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListRestorePlansFixedSizeCollection
extends AbstractFixedSizeCollection<
ListRestorePlansRequest,
ListRestorePlansResponse,
RestorePlan,
ListRestorePlansPage,
ListRestorePlansFixedSizeCollection> {
private ListRestorePlansFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListRestorePlansFixedSizeCollection createEmptyCollection() {
return new ListRestorePlansFixedSizeCollection(null, 0);
}
@Override
protected ListRestorePlansFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListRestorePlansFixedSizeCollection(pages, collectionSize);
}
}
public static class ListRestoresPagedResponse
extends AbstractPagedListResponse<
ListRestoresRequest,
ListRestoresResponse,
Restore,
ListRestoresPage,
ListRestoresFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListRestoresPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListRestoresPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListRestoresPagedResponse(ListRestoresPage page) {
super(page, ListRestoresFixedSizeCollection.createEmptyCollection());
}
}
public static class ListRestoresPage
extends AbstractPage {
private ListRestoresPage(
PageContext context,
ListRestoresResponse response) {
super(context, response);
}
private static ListRestoresPage createEmptyPage() {
return new ListRestoresPage(null, null);
}
@Override
protected ListRestoresPage createPage(
PageContext context,
ListRestoresResponse response) {
return new ListRestoresPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListRestoresFixedSizeCollection
extends AbstractFixedSizeCollection<
ListRestoresRequest,
ListRestoresResponse,
Restore,
ListRestoresPage,
ListRestoresFixedSizeCollection> {
private ListRestoresFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListRestoresFixedSizeCollection createEmptyCollection() {
return new ListRestoresFixedSizeCollection(null, 0);
}
@Override
protected ListRestoresFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListRestoresFixedSizeCollection(pages, collectionSize);
}
}
public static class ListVolumeRestoresPagedResponse
extends AbstractPagedListResponse<
ListVolumeRestoresRequest,
ListVolumeRestoresResponse,
VolumeRestore,
ListVolumeRestoresPage,
ListVolumeRestoresFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListVolumeRestoresPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListVolumeRestoresPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListVolumeRestoresPagedResponse(ListVolumeRestoresPage page) {
super(page, ListVolumeRestoresFixedSizeCollection.createEmptyCollection());
}
}
public static class ListVolumeRestoresPage
extends AbstractPage<
ListVolumeRestoresRequest,
ListVolumeRestoresResponse,
VolumeRestore,
ListVolumeRestoresPage> {
private ListVolumeRestoresPage(
PageContext context,
ListVolumeRestoresResponse response) {
super(context, response);
}
private static ListVolumeRestoresPage createEmptyPage() {
return new ListVolumeRestoresPage(null, null);
}
@Override
protected ListVolumeRestoresPage createPage(
PageContext context,
ListVolumeRestoresResponse response) {
return new ListVolumeRestoresPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListVolumeRestoresFixedSizeCollection
extends AbstractFixedSizeCollection<
ListVolumeRestoresRequest,
ListVolumeRestoresResponse,
VolumeRestore,
ListVolumeRestoresPage,
ListVolumeRestoresFixedSizeCollection> {
private ListVolumeRestoresFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListVolumeRestoresFixedSizeCollection createEmptyCollection() {
return new ListVolumeRestoresFixedSizeCollection(null, 0);
}
@Override
protected ListVolumeRestoresFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListVolumeRestoresFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}