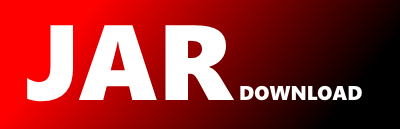
com.google.cloud.gkebackup.v1.stub.GrpcBackupForGKEStub Maven / Gradle / Ivy
Show all versions of google-cloud-gke-backup Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.gkebackup.v1.stub;
import static com.google.cloud.gkebackup.v1.BackupForGKEClient.ListBackupPlansPagedResponse;
import static com.google.cloud.gkebackup.v1.BackupForGKEClient.ListBackupsPagedResponse;
import static com.google.cloud.gkebackup.v1.BackupForGKEClient.ListLocationsPagedResponse;
import static com.google.cloud.gkebackup.v1.BackupForGKEClient.ListRestorePlansPagedResponse;
import static com.google.cloud.gkebackup.v1.BackupForGKEClient.ListRestoresPagedResponse;
import static com.google.cloud.gkebackup.v1.BackupForGKEClient.ListVolumeBackupsPagedResponse;
import static com.google.cloud.gkebackup.v1.BackupForGKEClient.ListVolumeRestoresPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.gkebackup.v1.Backup;
import com.google.cloud.gkebackup.v1.BackupPlan;
import com.google.cloud.gkebackup.v1.CreateBackupPlanRequest;
import com.google.cloud.gkebackup.v1.CreateBackupRequest;
import com.google.cloud.gkebackup.v1.CreateRestorePlanRequest;
import com.google.cloud.gkebackup.v1.CreateRestoreRequest;
import com.google.cloud.gkebackup.v1.DeleteBackupPlanRequest;
import com.google.cloud.gkebackup.v1.DeleteBackupRequest;
import com.google.cloud.gkebackup.v1.DeleteRestorePlanRequest;
import com.google.cloud.gkebackup.v1.DeleteRestoreRequest;
import com.google.cloud.gkebackup.v1.GetBackupIndexDownloadUrlRequest;
import com.google.cloud.gkebackup.v1.GetBackupIndexDownloadUrlResponse;
import com.google.cloud.gkebackup.v1.GetBackupPlanRequest;
import com.google.cloud.gkebackup.v1.GetBackupRequest;
import com.google.cloud.gkebackup.v1.GetRestorePlanRequest;
import com.google.cloud.gkebackup.v1.GetRestoreRequest;
import com.google.cloud.gkebackup.v1.GetVolumeBackupRequest;
import com.google.cloud.gkebackup.v1.GetVolumeRestoreRequest;
import com.google.cloud.gkebackup.v1.ListBackupPlansRequest;
import com.google.cloud.gkebackup.v1.ListBackupPlansResponse;
import com.google.cloud.gkebackup.v1.ListBackupsRequest;
import com.google.cloud.gkebackup.v1.ListBackupsResponse;
import com.google.cloud.gkebackup.v1.ListRestorePlansRequest;
import com.google.cloud.gkebackup.v1.ListRestorePlansResponse;
import com.google.cloud.gkebackup.v1.ListRestoresRequest;
import com.google.cloud.gkebackup.v1.ListRestoresResponse;
import com.google.cloud.gkebackup.v1.ListVolumeBackupsRequest;
import com.google.cloud.gkebackup.v1.ListVolumeBackupsResponse;
import com.google.cloud.gkebackup.v1.ListVolumeRestoresRequest;
import com.google.cloud.gkebackup.v1.ListVolumeRestoresResponse;
import com.google.cloud.gkebackup.v1.OperationMetadata;
import com.google.cloud.gkebackup.v1.Restore;
import com.google.cloud.gkebackup.v1.RestorePlan;
import com.google.cloud.gkebackup.v1.UpdateBackupPlanRequest;
import com.google.cloud.gkebackup.v1.UpdateBackupRequest;
import com.google.cloud.gkebackup.v1.UpdateRestorePlanRequest;
import com.google.cloud.gkebackup.v1.UpdateRestoreRequest;
import com.google.cloud.gkebackup.v1.VolumeBackup;
import com.google.cloud.gkebackup.v1.VolumeRestore;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import com.google.protobuf.Empty;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the BackupForGKE service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public class GrpcBackupForGKEStub extends BackupForGKEStub {
private static final MethodDescriptor
createBackupPlanMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/CreateBackupPlan")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateBackupPlanRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listBackupPlansMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/ListBackupPlans")
.setRequestMarshaller(
ProtoUtils.marshaller(ListBackupPlansRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListBackupPlansResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getBackupPlanMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/GetBackupPlan")
.setRequestMarshaller(
ProtoUtils.marshaller(GetBackupPlanRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(BackupPlan.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateBackupPlanMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/UpdateBackupPlan")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateBackupPlanRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteBackupPlanMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/DeleteBackupPlan")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteBackupPlanRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
createBackupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/CreateBackup")
.setRequestMarshaller(ProtoUtils.marshaller(CreateBackupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listBackupsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/ListBackups")
.setRequestMarshaller(ProtoUtils.marshaller(ListBackupsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListBackupsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getBackupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/GetBackup")
.setRequestMarshaller(ProtoUtils.marshaller(GetBackupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Backup.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateBackupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/UpdateBackup")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateBackupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteBackupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/DeleteBackup")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteBackupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listVolumeBackupsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/ListVolumeBackups")
.setRequestMarshaller(
ProtoUtils.marshaller(ListVolumeBackupsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListVolumeBackupsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getVolumeBackupMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/GetVolumeBackup")
.setRequestMarshaller(
ProtoUtils.marshaller(GetVolumeBackupRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(VolumeBackup.getDefaultInstance()))
.build();
private static final MethodDescriptor
createRestorePlanMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/CreateRestorePlan")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateRestorePlanRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listRestorePlansMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/ListRestorePlans")
.setRequestMarshaller(
ProtoUtils.marshaller(ListRestorePlansRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListRestorePlansResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getRestorePlanMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/GetRestorePlan")
.setRequestMarshaller(
ProtoUtils.marshaller(GetRestorePlanRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(RestorePlan.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateRestorePlanMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/UpdateRestorePlan")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateRestorePlanRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteRestorePlanMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/DeleteRestorePlan")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteRestorePlanRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
createRestoreMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/CreateRestore")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateRestoreRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listRestoresMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/ListRestores")
.setRequestMarshaller(ProtoUtils.marshaller(ListRestoresRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListRestoresResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getRestoreMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/GetRestore")
.setRequestMarshaller(ProtoUtils.marshaller(GetRestoreRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Restore.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateRestoreMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/UpdateRestore")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateRestoreRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteRestoreMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/DeleteRestore")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteRestoreRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listVolumeRestoresMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/ListVolumeRestores")
.setRequestMarshaller(
ProtoUtils.marshaller(ListVolumeRestoresRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListVolumeRestoresResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getVolumeRestoreMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/GetVolumeRestore")
.setRequestMarshaller(
ProtoUtils.marshaller(GetVolumeRestoreRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(VolumeRestore.getDefaultInstance()))
.build();
private static final MethodDescriptor<
GetBackupIndexDownloadUrlRequest, GetBackupIndexDownloadUrlResponse>
getBackupIndexDownloadUrlMethodDescriptor =
MethodDescriptor
.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.gkebackup.v1.BackupForGKE/GetBackupIndexDownloadUrl")
.setRequestMarshaller(
ProtoUtils.marshaller(GetBackupIndexDownloadUrlRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(GetBackupIndexDownloadUrlResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
listLocationsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/ListLocations")
.setRequestMarshaller(
ProtoUtils.marshaller(ListLocationsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListLocationsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getLocationMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/GetLocation")
.setRequestMarshaller(ProtoUtils.marshaller(GetLocationRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Location.getDefaultInstance()))
.build();
private static final MethodDescriptor setIamPolicyMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.iam.v1.IAMPolicy/SetIamPolicy")
.setRequestMarshaller(ProtoUtils.marshaller(SetIamPolicyRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Policy.getDefaultInstance()))
.build();
private static final MethodDescriptor getIamPolicyMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.iam.v1.IAMPolicy/GetIamPolicy")
.setRequestMarshaller(ProtoUtils.marshaller(GetIamPolicyRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Policy.getDefaultInstance()))
.build();
private static final MethodDescriptor
testIamPermissionsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.iam.v1.IAMPolicy/TestIamPermissions")
.setRequestMarshaller(
ProtoUtils.marshaller(TestIamPermissionsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(TestIamPermissionsResponse.getDefaultInstance()))
.build();
private final UnaryCallable createBackupPlanCallable;
private final OperationCallable
createBackupPlanOperationCallable;
private final UnaryCallable
listBackupPlansCallable;
private final UnaryCallable
listBackupPlansPagedCallable;
private final UnaryCallable getBackupPlanCallable;
private final UnaryCallable updateBackupPlanCallable;
private final OperationCallable
updateBackupPlanOperationCallable;
private final UnaryCallable deleteBackupPlanCallable;
private final OperationCallable
deleteBackupPlanOperationCallable;
private final UnaryCallable createBackupCallable;
private final OperationCallable
createBackupOperationCallable;
private final UnaryCallable listBackupsCallable;
private final UnaryCallable
listBackupsPagedCallable;
private final UnaryCallable getBackupCallable;
private final UnaryCallable updateBackupCallable;
private final OperationCallable
updateBackupOperationCallable;
private final UnaryCallable deleteBackupCallable;
private final OperationCallable
deleteBackupOperationCallable;
private final UnaryCallable
listVolumeBackupsCallable;
private final UnaryCallable
listVolumeBackupsPagedCallable;
private final UnaryCallable getVolumeBackupCallable;
private final UnaryCallable createRestorePlanCallable;
private final OperationCallable
createRestorePlanOperationCallable;
private final UnaryCallable
listRestorePlansCallable;
private final UnaryCallable
listRestorePlansPagedCallable;
private final UnaryCallable getRestorePlanCallable;
private final UnaryCallable updateRestorePlanCallable;
private final OperationCallable
updateRestorePlanOperationCallable;
private final UnaryCallable deleteRestorePlanCallable;
private final OperationCallable
deleteRestorePlanOperationCallable;
private final UnaryCallable createRestoreCallable;
private final OperationCallable
createRestoreOperationCallable;
private final UnaryCallable listRestoresCallable;
private final UnaryCallable
listRestoresPagedCallable;
private final UnaryCallable getRestoreCallable;
private final UnaryCallable updateRestoreCallable;
private final OperationCallable
updateRestoreOperationCallable;
private final UnaryCallable deleteRestoreCallable;
private final OperationCallable
deleteRestoreOperationCallable;
private final UnaryCallable
listVolumeRestoresCallable;
private final UnaryCallable
listVolumeRestoresPagedCallable;
private final UnaryCallable getVolumeRestoreCallable;
private final UnaryCallable
getBackupIndexDownloadUrlCallable;
private final UnaryCallable listLocationsCallable;
private final UnaryCallable
listLocationsPagedCallable;
private final UnaryCallable getLocationCallable;
private final UnaryCallable setIamPolicyCallable;
private final UnaryCallable getIamPolicyCallable;
private final UnaryCallable
testIamPermissionsCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcBackupForGKEStub create(BackupForGKEStubSettings settings)
throws IOException {
return new GrpcBackupForGKEStub(settings, ClientContext.create(settings));
}
public static final GrpcBackupForGKEStub create(ClientContext clientContext) throws IOException {
return new GrpcBackupForGKEStub(BackupForGKEStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcBackupForGKEStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcBackupForGKEStub(
BackupForGKEStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcBackupForGKEStub, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcBackupForGKEStub(BackupForGKEStubSettings settings, ClientContext clientContext)
throws IOException {
this(settings, clientContext, new GrpcBackupForGKECallableFactory());
}
/**
* Constructs an instance of GrpcBackupForGKEStub, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcBackupForGKEStub(
BackupForGKEStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings createBackupPlanTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createBackupPlanMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
listBackupPlansTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listBackupPlansMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getBackupPlanTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getBackupPlanMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateBackupPlanTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateBackupPlanMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add(
"backup_plan.name", String.valueOf(request.getBackupPlan().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteBackupPlanTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteBackupPlanMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createBackupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createBackupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings listBackupsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listBackupsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getBackupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getBackupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateBackupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateBackupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("backup.name", String.valueOf(request.getBackup().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteBackupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteBackupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listVolumeBackupsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listVolumeBackupsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getVolumeBackupTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getVolumeBackupMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createRestorePlanTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createRestorePlanMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
listRestorePlansTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listRestorePlansMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getRestorePlanTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getRestorePlanMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateRestorePlanTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateRestorePlanMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add(
"restore_plan.name", String.valueOf(request.getRestorePlan().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteRestorePlanTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteRestorePlanMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createRestoreTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createRestoreMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings listRestoresTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listRestoresMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getRestoreTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getRestoreMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateRestoreTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateRestoreMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("restore.name", String.valueOf(request.getRestore().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteRestoreTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteRestoreMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listVolumeRestoresTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listVolumeRestoresMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getVolumeRestoreTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getVolumeRestoreMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
getBackupIndexDownloadUrlTransportSettings =
GrpcCallSettings
.newBuilder()
.setMethodDescriptor(getBackupIndexDownloadUrlMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("backup", String.valueOf(request.getBackup()));
return builder.build();
})
.build();
GrpcCallSettings listLocationsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listLocationsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getLocationTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getLocationMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings setIamPolicyTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(setIamPolicyMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("resource", String.valueOf(request.getResource()));
return builder.build();
})
.build();
GrpcCallSettings getIamPolicyTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getIamPolicyMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("resource", String.valueOf(request.getResource()));
return builder.build();
})
.build();
GrpcCallSettings
testIamPermissionsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(testIamPermissionsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("resource", String.valueOf(request.getResource()));
return builder.build();
})
.build();
this.createBackupPlanCallable =
callableFactory.createUnaryCallable(
createBackupPlanTransportSettings, settings.createBackupPlanSettings(), clientContext);
this.createBackupPlanOperationCallable =
callableFactory.createOperationCallable(
createBackupPlanTransportSettings,
settings.createBackupPlanOperationSettings(),
clientContext,
operationsStub);
this.listBackupPlansCallable =
callableFactory.createUnaryCallable(
listBackupPlansTransportSettings, settings.listBackupPlansSettings(), clientContext);
this.listBackupPlansPagedCallable =
callableFactory.createPagedCallable(
listBackupPlansTransportSettings, settings.listBackupPlansSettings(), clientContext);
this.getBackupPlanCallable =
callableFactory.createUnaryCallable(
getBackupPlanTransportSettings, settings.getBackupPlanSettings(), clientContext);
this.updateBackupPlanCallable =
callableFactory.createUnaryCallable(
updateBackupPlanTransportSettings, settings.updateBackupPlanSettings(), clientContext);
this.updateBackupPlanOperationCallable =
callableFactory.createOperationCallable(
updateBackupPlanTransportSettings,
settings.updateBackupPlanOperationSettings(),
clientContext,
operationsStub);
this.deleteBackupPlanCallable =
callableFactory.createUnaryCallable(
deleteBackupPlanTransportSettings, settings.deleteBackupPlanSettings(), clientContext);
this.deleteBackupPlanOperationCallable =
callableFactory.createOperationCallable(
deleteBackupPlanTransportSettings,
settings.deleteBackupPlanOperationSettings(),
clientContext,
operationsStub);
this.createBackupCallable =
callableFactory.createUnaryCallable(
createBackupTransportSettings, settings.createBackupSettings(), clientContext);
this.createBackupOperationCallable =
callableFactory.createOperationCallable(
createBackupTransportSettings,
settings.createBackupOperationSettings(),
clientContext,
operationsStub);
this.listBackupsCallable =
callableFactory.createUnaryCallable(
listBackupsTransportSettings, settings.listBackupsSettings(), clientContext);
this.listBackupsPagedCallable =
callableFactory.createPagedCallable(
listBackupsTransportSettings, settings.listBackupsSettings(), clientContext);
this.getBackupCallable =
callableFactory.createUnaryCallable(
getBackupTransportSettings, settings.getBackupSettings(), clientContext);
this.updateBackupCallable =
callableFactory.createUnaryCallable(
updateBackupTransportSettings, settings.updateBackupSettings(), clientContext);
this.updateBackupOperationCallable =
callableFactory.createOperationCallable(
updateBackupTransportSettings,
settings.updateBackupOperationSettings(),
clientContext,
operationsStub);
this.deleteBackupCallable =
callableFactory.createUnaryCallable(
deleteBackupTransportSettings, settings.deleteBackupSettings(), clientContext);
this.deleteBackupOperationCallable =
callableFactory.createOperationCallable(
deleteBackupTransportSettings,
settings.deleteBackupOperationSettings(),
clientContext,
operationsStub);
this.listVolumeBackupsCallable =
callableFactory.createUnaryCallable(
listVolumeBackupsTransportSettings,
settings.listVolumeBackupsSettings(),
clientContext);
this.listVolumeBackupsPagedCallable =
callableFactory.createPagedCallable(
listVolumeBackupsTransportSettings,
settings.listVolumeBackupsSettings(),
clientContext);
this.getVolumeBackupCallable =
callableFactory.createUnaryCallable(
getVolumeBackupTransportSettings, settings.getVolumeBackupSettings(), clientContext);
this.createRestorePlanCallable =
callableFactory.createUnaryCallable(
createRestorePlanTransportSettings,
settings.createRestorePlanSettings(),
clientContext);
this.createRestorePlanOperationCallable =
callableFactory.createOperationCallable(
createRestorePlanTransportSettings,
settings.createRestorePlanOperationSettings(),
clientContext,
operationsStub);
this.listRestorePlansCallable =
callableFactory.createUnaryCallable(
listRestorePlansTransportSettings, settings.listRestorePlansSettings(), clientContext);
this.listRestorePlansPagedCallable =
callableFactory.createPagedCallable(
listRestorePlansTransportSettings, settings.listRestorePlansSettings(), clientContext);
this.getRestorePlanCallable =
callableFactory.createUnaryCallable(
getRestorePlanTransportSettings, settings.getRestorePlanSettings(), clientContext);
this.updateRestorePlanCallable =
callableFactory.createUnaryCallable(
updateRestorePlanTransportSettings,
settings.updateRestorePlanSettings(),
clientContext);
this.updateRestorePlanOperationCallable =
callableFactory.createOperationCallable(
updateRestorePlanTransportSettings,
settings.updateRestorePlanOperationSettings(),
clientContext,
operationsStub);
this.deleteRestorePlanCallable =
callableFactory.createUnaryCallable(
deleteRestorePlanTransportSettings,
settings.deleteRestorePlanSettings(),
clientContext);
this.deleteRestorePlanOperationCallable =
callableFactory.createOperationCallable(
deleteRestorePlanTransportSettings,
settings.deleteRestorePlanOperationSettings(),
clientContext,
operationsStub);
this.createRestoreCallable =
callableFactory.createUnaryCallable(
createRestoreTransportSettings, settings.createRestoreSettings(), clientContext);
this.createRestoreOperationCallable =
callableFactory.createOperationCallable(
createRestoreTransportSettings,
settings.createRestoreOperationSettings(),
clientContext,
operationsStub);
this.listRestoresCallable =
callableFactory.createUnaryCallable(
listRestoresTransportSettings, settings.listRestoresSettings(), clientContext);
this.listRestoresPagedCallable =
callableFactory.createPagedCallable(
listRestoresTransportSettings, settings.listRestoresSettings(), clientContext);
this.getRestoreCallable =
callableFactory.createUnaryCallable(
getRestoreTransportSettings, settings.getRestoreSettings(), clientContext);
this.updateRestoreCallable =
callableFactory.createUnaryCallable(
updateRestoreTransportSettings, settings.updateRestoreSettings(), clientContext);
this.updateRestoreOperationCallable =
callableFactory.createOperationCallable(
updateRestoreTransportSettings,
settings.updateRestoreOperationSettings(),
clientContext,
operationsStub);
this.deleteRestoreCallable =
callableFactory.createUnaryCallable(
deleteRestoreTransportSettings, settings.deleteRestoreSettings(), clientContext);
this.deleteRestoreOperationCallable =
callableFactory.createOperationCallable(
deleteRestoreTransportSettings,
settings.deleteRestoreOperationSettings(),
clientContext,
operationsStub);
this.listVolumeRestoresCallable =
callableFactory.createUnaryCallable(
listVolumeRestoresTransportSettings,
settings.listVolumeRestoresSettings(),
clientContext);
this.listVolumeRestoresPagedCallable =
callableFactory.createPagedCallable(
listVolumeRestoresTransportSettings,
settings.listVolumeRestoresSettings(),
clientContext);
this.getVolumeRestoreCallable =
callableFactory.createUnaryCallable(
getVolumeRestoreTransportSettings, settings.getVolumeRestoreSettings(), clientContext);
this.getBackupIndexDownloadUrlCallable =
callableFactory.createUnaryCallable(
getBackupIndexDownloadUrlTransportSettings,
settings.getBackupIndexDownloadUrlSettings(),
clientContext);
this.listLocationsCallable =
callableFactory.createUnaryCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.listLocationsPagedCallable =
callableFactory.createPagedCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.getLocationCallable =
callableFactory.createUnaryCallable(
getLocationTransportSettings, settings.getLocationSettings(), clientContext);
this.setIamPolicyCallable =
callableFactory.createUnaryCallable(
setIamPolicyTransportSettings, settings.setIamPolicySettings(), clientContext);
this.getIamPolicyCallable =
callableFactory.createUnaryCallable(
getIamPolicyTransportSettings, settings.getIamPolicySettings(), clientContext);
this.testIamPermissionsCallable =
callableFactory.createUnaryCallable(
testIamPermissionsTransportSettings,
settings.testIamPermissionsSettings(),
clientContext);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable createBackupPlanCallable() {
return createBackupPlanCallable;
}
@Override
public OperationCallable
createBackupPlanOperationCallable() {
return createBackupPlanOperationCallable;
}
@Override
public UnaryCallable listBackupPlansCallable() {
return listBackupPlansCallable;
}
@Override
public UnaryCallable
listBackupPlansPagedCallable() {
return listBackupPlansPagedCallable;
}
@Override
public UnaryCallable getBackupPlanCallable() {
return getBackupPlanCallable;
}
@Override
public UnaryCallable updateBackupPlanCallable() {
return updateBackupPlanCallable;
}
@Override
public OperationCallable
updateBackupPlanOperationCallable() {
return updateBackupPlanOperationCallable;
}
@Override
public UnaryCallable deleteBackupPlanCallable() {
return deleteBackupPlanCallable;
}
@Override
public OperationCallable
deleteBackupPlanOperationCallable() {
return deleteBackupPlanOperationCallable;
}
@Override
public UnaryCallable createBackupCallable() {
return createBackupCallable;
}
@Override
public OperationCallable
createBackupOperationCallable() {
return createBackupOperationCallable;
}
@Override
public UnaryCallable listBackupsCallable() {
return listBackupsCallable;
}
@Override
public UnaryCallable listBackupsPagedCallable() {
return listBackupsPagedCallable;
}
@Override
public UnaryCallable getBackupCallable() {
return getBackupCallable;
}
@Override
public UnaryCallable updateBackupCallable() {
return updateBackupCallable;
}
@Override
public OperationCallable
updateBackupOperationCallable() {
return updateBackupOperationCallable;
}
@Override
public UnaryCallable deleteBackupCallable() {
return deleteBackupCallable;
}
@Override
public OperationCallable
deleteBackupOperationCallable() {
return deleteBackupOperationCallable;
}
@Override
public UnaryCallable
listVolumeBackupsCallable() {
return listVolumeBackupsCallable;
}
@Override
public UnaryCallable
listVolumeBackupsPagedCallable() {
return listVolumeBackupsPagedCallable;
}
@Override
public UnaryCallable getVolumeBackupCallable() {
return getVolumeBackupCallable;
}
@Override
public UnaryCallable createRestorePlanCallable() {
return createRestorePlanCallable;
}
@Override
public OperationCallable
createRestorePlanOperationCallable() {
return createRestorePlanOperationCallable;
}
@Override
public UnaryCallable
listRestorePlansCallable() {
return listRestorePlansCallable;
}
@Override
public UnaryCallable
listRestorePlansPagedCallable() {
return listRestorePlansPagedCallable;
}
@Override
public UnaryCallable getRestorePlanCallable() {
return getRestorePlanCallable;
}
@Override
public UnaryCallable updateRestorePlanCallable() {
return updateRestorePlanCallable;
}
@Override
public OperationCallable
updateRestorePlanOperationCallable() {
return updateRestorePlanOperationCallable;
}
@Override
public UnaryCallable deleteRestorePlanCallable() {
return deleteRestorePlanCallable;
}
@Override
public OperationCallable
deleteRestorePlanOperationCallable() {
return deleteRestorePlanOperationCallable;
}
@Override
public UnaryCallable createRestoreCallable() {
return createRestoreCallable;
}
@Override
public OperationCallable
createRestoreOperationCallable() {
return createRestoreOperationCallable;
}
@Override
public UnaryCallable listRestoresCallable() {
return listRestoresCallable;
}
@Override
public UnaryCallable listRestoresPagedCallable() {
return listRestoresPagedCallable;
}
@Override
public UnaryCallable getRestoreCallable() {
return getRestoreCallable;
}
@Override
public UnaryCallable updateRestoreCallable() {
return updateRestoreCallable;
}
@Override
public OperationCallable
updateRestoreOperationCallable() {
return updateRestoreOperationCallable;
}
@Override
public UnaryCallable deleteRestoreCallable() {
return deleteRestoreCallable;
}
@Override
public OperationCallable
deleteRestoreOperationCallable() {
return deleteRestoreOperationCallable;
}
@Override
public UnaryCallable
listVolumeRestoresCallable() {
return listVolumeRestoresCallable;
}
@Override
public UnaryCallable
listVolumeRestoresPagedCallable() {
return listVolumeRestoresPagedCallable;
}
@Override
public UnaryCallable getVolumeRestoreCallable() {
return getVolumeRestoreCallable;
}
@Override
public UnaryCallable
getBackupIndexDownloadUrlCallable() {
return getBackupIndexDownloadUrlCallable;
}
@Override
public UnaryCallable listLocationsCallable() {
return listLocationsCallable;
}
@Override
public UnaryCallable
listLocationsPagedCallable() {
return listLocationsPagedCallable;
}
@Override
public UnaryCallable getLocationCallable() {
return getLocationCallable;
}
@Override
public UnaryCallable setIamPolicyCallable() {
return setIamPolicyCallable;
}
@Override
public UnaryCallable getIamPolicyCallable() {
return getIamPolicyCallable;
}
@Override
public UnaryCallable
testIamPermissionsCallable() {
return testIamPermissionsCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}