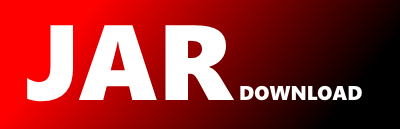
com.google.cloud.video.livestream.v1.LivestreamServiceClient Maven / Gradle / Ivy
Show all versions of google-cloud-live-stream Show documentation
/*
* Copyright 2023 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.video.livestream.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.video.livestream.v1.stub.LivestreamServiceStub;
import com.google.cloud.video.livestream.v1.stub.LivestreamServiceStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Using Live Stream API, you can generate live streams in the various
* renditions and streaming formats. The streaming format include HTTP Live Streaming (HLS) and
* Dynamic Adaptive Streaming over HTTP (DASH). You can send a source stream in the various ways,
* including Real-Time Messaging Protocol (RTMP) and Secure Reliable Transport (SRT).
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ChannelName name = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]");
* Channel response = livestreamServiceClient.getChannel(name);
* }
* }
*
* Note: close() needs to be called on the LivestreamServiceClient object to clean up resources
* such as threads. In the example above, try-with-resources is used, which automatically calls
* close().
*
*
*
* Method
* Description
* Method Variants
*
* CreateChannel
* Creates a channel with the provided unique ID in the specified region.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - createChannelAsync(CreateChannelRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - createChannelAsync(LocationName parent, Channel channel, String channelId)
*
- createChannelAsync(String parent, Channel channel, String channelId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - createChannelOperationCallable()
*
- createChannelCallable()
*
*
*
*
* ListChannels
* Returns a list of all channels in the specified region.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - listChannels(ListChannelsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - listChannels(LocationName parent)
*
- listChannels(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - listChannelsPagedCallable()
*
- listChannelsCallable()
*
*
*
*
* GetChannel
* Returns the specified channel.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - getChannel(GetChannelRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - getChannel(ChannelName name)
*
- getChannel(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - getChannelCallable()
*
*
*
*
* DeleteChannel
* Deletes the specified channel.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - deleteChannelAsync(DeleteChannelRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - deleteChannelAsync(ChannelName name)
*
- deleteChannelAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - deleteChannelOperationCallable()
*
- deleteChannelCallable()
*
*
*
*
* UpdateChannel
* Updates the specified channel.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - updateChannelAsync(UpdateChannelRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - updateChannelAsync(Channel channel, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - updateChannelOperationCallable()
*
- updateChannelCallable()
*
*
*
*
* StartChannel
* Starts the specified channel. Part of the video pipeline will be created only when the StartChannel request is received by the server.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - startChannelAsync(StartChannelRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - startChannelAsync(ChannelName name)
*
- startChannelAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - startChannelOperationCallable()
*
- startChannelCallable()
*
*
*
*
* StopChannel
* Stops the specified channel. Part of the video pipeline will be released when the StopChannel request is received by the server.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - stopChannelAsync(StopChannelRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - stopChannelAsync(ChannelName name)
*
- stopChannelAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - stopChannelOperationCallable()
*
- stopChannelCallable()
*
*
*
*
* CreateInput
* Creates an input with the provided unique ID in the specified region.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - createInputAsync(CreateInputRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - createInputAsync(LocationName parent, Input input, String inputId)
*
- createInputAsync(String parent, Input input, String inputId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - createInputOperationCallable()
*
- createInputCallable()
*
*
*
*
* ListInputs
* Returns a list of all inputs in the specified region.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - listInputs(ListInputsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - listInputs(LocationName parent)
*
- listInputs(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - listInputsPagedCallable()
*
- listInputsCallable()
*
*
*
*
* GetInput
* Returns the specified input.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - getInput(GetInputRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - getInput(InputName name)
*
- getInput(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - getInputCallable()
*
*
*
*
* DeleteInput
* Deletes the specified input.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - deleteInputAsync(DeleteInputRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - deleteInputAsync(InputName name)
*
- deleteInputAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - deleteInputOperationCallable()
*
- deleteInputCallable()
*
*
*
*
* UpdateInput
* Updates the specified input.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - updateInputAsync(UpdateInputRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - updateInputAsync(Input input, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - updateInputOperationCallable()
*
- updateInputCallable()
*
*
*
*
* CreateEvent
* Creates an event with the provided unique ID in the specified channel.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - createEvent(CreateEventRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - createEvent(ChannelName parent, Event event, String eventId)
*
- createEvent(String parent, Event event, String eventId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - createEventCallable()
*
*
*
*
* ListEvents
* Returns a list of all events in the specified channel.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - listEvents(ListEventsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - listEvents(ChannelName parent)
*
- listEvents(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - listEventsPagedCallable()
*
- listEventsCallable()
*
*
*
*
* GetEvent
* Returns the specified event.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - getEvent(GetEventRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - getEvent(EventName name)
*
- getEvent(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - getEventCallable()
*
*
*
*
* DeleteEvent
* Deletes the specified event.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - deleteEvent(DeleteEventRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - deleteEvent(EventName name)
*
- deleteEvent(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - deleteEventCallable()
*
*
*
*
* CreateAsset
* Creates a Asset with the provided unique ID in the specified region.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - createAssetAsync(CreateAssetRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - createAssetAsync(LocationName parent, Asset asset, String assetId)
*
- createAssetAsync(String parent, Asset asset, String assetId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - createAssetOperationCallable()
*
- createAssetCallable()
*
*
*
*
* DeleteAsset
* Deletes the specified asset if it is not used.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - deleteAssetAsync(DeleteAssetRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - deleteAssetAsync(AssetName name)
*
- deleteAssetAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - deleteAssetOperationCallable()
*
- deleteAssetCallable()
*
*
*
*
* GetAsset
* Returns the specified asset.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - getAsset(GetAssetRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - getAsset(AssetName name)
*
- getAsset(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - getAssetCallable()
*
*
*
*
* ListAssets
* Returns a list of all assets in the specified region.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - listAssets(ListAssetsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - listAssets(LocationName parent)
*
- listAssets(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - listAssetsPagedCallable()
*
- listAssetsCallable()
*
*
*
*
* GetPool
* Returns the specified pool.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - getPool(GetPoolRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* - getPool(PoolName name)
*
- getPool(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - getPoolCallable()
*
*
*
*
* UpdatePool
* Updates the specified pool.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - updatePoolAsync(UpdatePoolRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* - updatePoolAsync(Pool pool, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - updatePoolOperationCallable()
*
- updatePoolCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - listLocationsPagedCallable()
*
- listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* - getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* - getLocationCallable()
*
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of LivestreamServiceSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* LivestreamServiceSettings livestreamServiceSettings =
* LivestreamServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* LivestreamServiceClient livestreamServiceClient =
* LivestreamServiceClient.create(livestreamServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* LivestreamServiceSettings livestreamServiceSettings =
* LivestreamServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* LivestreamServiceClient livestreamServiceClient =
* LivestreamServiceClient.create(livestreamServiceSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* LivestreamServiceSettings livestreamServiceSettings =
* LivestreamServiceSettings.newHttpJsonBuilder().build();
* LivestreamServiceClient livestreamServiceClient =
* LivestreamServiceClient.create(livestreamServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class LivestreamServiceClient implements BackgroundResource {
private final LivestreamServiceSettings settings;
private final LivestreamServiceStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of LivestreamServiceClient with default settings. */
public static final LivestreamServiceClient create() throws IOException {
return create(LivestreamServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of LivestreamServiceClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final LivestreamServiceClient create(LivestreamServiceSettings settings)
throws IOException {
return new LivestreamServiceClient(settings);
}
/**
* Constructs an instance of LivestreamServiceClient, using the given stub for making calls. This
* is for advanced usage - prefer using create(LivestreamServiceSettings).
*/
public static final LivestreamServiceClient create(LivestreamServiceStub stub) {
return new LivestreamServiceClient(stub);
}
/**
* Constructs an instance of LivestreamServiceClient, using the given settings. This is protected
* so that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected LivestreamServiceClient(LivestreamServiceSettings settings) throws IOException {
this.settings = settings;
this.stub = ((LivestreamServiceStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected LivestreamServiceClient(LivestreamServiceStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final LivestreamServiceSettings getSettings() {
return settings;
}
public LivestreamServiceStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a channel with the provided unique ID in the specified region.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Channel channel = Channel.newBuilder().build();
* String channelId = "channelId1461735806";
* Channel response =
* livestreamServiceClient.createChannelAsync(parent, channel, channelId).get();
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @param channel Required. The channel resource to be created.
* @param channelId Required. The ID of the channel resource to be created. This value must be
* 1-63 characters, begin and end with `[a-z0-9]`, could contain dashes (-) in between.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createChannelAsync(
LocationName parent, Channel channel, String channelId) {
CreateChannelRequest request =
CreateChannelRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setChannel(channel)
.setChannelId(channelId)
.build();
return createChannelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a channel with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Channel channel = Channel.newBuilder().build();
* String channelId = "channelId1461735806";
* Channel response =
* livestreamServiceClient.createChannelAsync(parent, channel, channelId).get();
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @param channel Required. The channel resource to be created.
* @param channelId Required. The ID of the channel resource to be created. This value must be
* 1-63 characters, begin and end with `[a-z0-9]`, could contain dashes (-) in between.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createChannelAsync(
String parent, Channel channel, String channelId) {
CreateChannelRequest request =
CreateChannelRequest.newBuilder()
.setParent(parent)
.setChannel(channel)
.setChannelId(channelId)
.build();
return createChannelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a channel with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateChannelRequest request =
* CreateChannelRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setChannel(Channel.newBuilder().build())
* .setChannelId("channelId1461735806")
* .setRequestId("requestId693933066")
* .build();
* Channel response = livestreamServiceClient.createChannelAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createChannelAsync(
CreateChannelRequest request) {
return createChannelOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a channel with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateChannelRequest request =
* CreateChannelRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setChannel(Channel.newBuilder().build())
* .setChannelId("channelId1461735806")
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.createChannelOperationCallable().futureCall(request);
* // Do something.
* Channel response = future.get();
* }
* }
*/
public final OperationCallable
createChannelOperationCallable() {
return stub.createChannelOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a channel with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateChannelRequest request =
* CreateChannelRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setChannel(Channel.newBuilder().build())
* .setChannelId("channelId1461735806")
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.createChannelCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createChannelCallable() {
return stub.createChannelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all channels in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Channel element : livestreamServiceClient.listChannels(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListChannelsPagedResponse listChannels(LocationName parent) {
ListChannelsRequest request =
ListChannelsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listChannels(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all channels in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Channel element : livestreamServiceClient.listChannels(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListChannelsPagedResponse listChannels(String parent) {
ListChannelsRequest request = ListChannelsRequest.newBuilder().setParent(parent).build();
return listChannels(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all channels in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListChannelsRequest request =
* ListChannelsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Channel element : livestreamServiceClient.listChannels(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListChannelsPagedResponse listChannels(ListChannelsRequest request) {
return listChannelsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all channels in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListChannelsRequest request =
* ListChannelsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* livestreamServiceClient.listChannelsPagedCallable().futureCall(request);
* // Do something.
* for (Channel element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listChannelsPagedCallable() {
return stub.listChannelsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all channels in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListChannelsRequest request =
* ListChannelsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListChannelsResponse response =
* livestreamServiceClient.listChannelsCallable().call(request);
* for (Channel element : response.getChannelsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listChannelsCallable() {
return stub.listChannelsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ChannelName name = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]");
* Channel response = livestreamServiceClient.getChannel(name);
* }
* }
*
* @param name Required. The name of the channel resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Channel getChannel(ChannelName name) {
GetChannelRequest request =
GetChannelRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getChannel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString();
* Channel response = livestreamServiceClient.getChannel(name);
* }
* }
*
* @param name Required. The name of the channel resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Channel getChannel(String name) {
GetChannelRequest request = GetChannelRequest.newBuilder().setName(name).build();
return getChannel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetChannelRequest request =
* GetChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .build();
* Channel response = livestreamServiceClient.getChannel(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Channel getChannel(GetChannelRequest request) {
return getChannelCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetChannelRequest request =
* GetChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .build();
* ApiFuture future = livestreamServiceClient.getChannelCallable().futureCall(request);
* // Do something.
* Channel response = future.get();
* }
* }
*/
public final UnaryCallable getChannelCallable() {
return stub.getChannelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ChannelName name = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]");
* livestreamServiceClient.deleteChannelAsync(name).get();
* }
* }
*
* @param name Required. The name of the channel resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteChannelAsync(ChannelName name) {
DeleteChannelRequest request =
DeleteChannelRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteChannelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString();
* livestreamServiceClient.deleteChannelAsync(name).get();
* }
* }
*
* @param name Required. The name of the channel resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteChannelAsync(String name) {
DeleteChannelRequest request = DeleteChannelRequest.newBuilder().setName(name).build();
return deleteChannelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteChannelRequest request =
* DeleteChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* livestreamServiceClient.deleteChannelAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteChannelAsync(
DeleteChannelRequest request) {
return deleteChannelOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteChannelRequest request =
* DeleteChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* OperationFuture future =
* livestreamServiceClient.deleteChannelOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteChannelOperationCallable() {
return stub.deleteChannelOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteChannelRequest request =
* DeleteChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* ApiFuture future =
* livestreamServiceClient.deleteChannelCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteChannelCallable() {
return stub.deleteChannelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* Channel channel = Channel.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Channel response = livestreamServiceClient.updateChannelAsync(channel, updateMask).get();
* }
* }
*
* @param channel Required. The channel resource to be updated.
* @param updateMask Field mask is used to specify the fields to be overwritten in the Channel
* resource by the update. You can only update the following fields:
*
* - [`inputAttachments`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#inputattachment)
*
- [`inputConfig`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#inputconfig)
*
- [`output`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#output)
*
- [`elementaryStreams`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#elementarystream)
*
- [`muxStreams`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#muxstream)
*
- [`manifests`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#manifest)
*
- [`spriteSheets`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#spritesheet)
*
- [`logConfig`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#logconfig)
*
- [`timecodeConfig`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#timecodeconfig)
*
- [`encryptions`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.channels#encryption)
*
* The fields specified in the update_mask are relative to the resource, not the full
* request. A field will be overwritten if it is in the mask.
*
If the mask is not present, then each field from the list above is updated if the field
* appears in the request payload. To unset a field, add the field to the update mask and
* remove it from the request payload.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateChannelAsync(
Channel channel, FieldMask updateMask) {
UpdateChannelRequest request =
UpdateChannelRequest.newBuilder().setChannel(channel).setUpdateMask(updateMask).build();
return updateChannelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* UpdateChannelRequest request =
* UpdateChannelRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setChannel(Channel.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Channel response = livestreamServiceClient.updateChannelAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateChannelAsync(
UpdateChannelRequest request) {
return updateChannelOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* UpdateChannelRequest request =
* UpdateChannelRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setChannel(Channel.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.updateChannelOperationCallable().futureCall(request);
* // Do something.
* Channel response = future.get();
* }
* }
*/
public final OperationCallable
updateChannelOperationCallable() {
return stub.updateChannelOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* UpdateChannelRequest request =
* UpdateChannelRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setChannel(Channel.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.updateChannelCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateChannelCallable() {
return stub.updateChannelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts the specified channel. Part of the video pipeline will be created only when the
* StartChannel request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ChannelName name = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]");
* ChannelOperationResponse response = livestreamServiceClient.startChannelAsync(name).get();
* }
* }
*
* @param name Required. The name of the channel resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture startChannelAsync(
ChannelName name) {
StartChannelRequest request =
StartChannelRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return startChannelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts the specified channel. Part of the video pipeline will be created only when the
* StartChannel request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString();
* ChannelOperationResponse response = livestreamServiceClient.startChannelAsync(name).get();
* }
* }
*
* @param name Required. The name of the channel resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture startChannelAsync(
String name) {
StartChannelRequest request = StartChannelRequest.newBuilder().setName(name).build();
return startChannelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts the specified channel. Part of the video pipeline will be created only when the
* StartChannel request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* StartChannelRequest request =
* StartChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setRequestId("requestId693933066")
* .build();
* ChannelOperationResponse response = livestreamServiceClient.startChannelAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture startChannelAsync(
StartChannelRequest request) {
return startChannelOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts the specified channel. Part of the video pipeline will be created only when the
* StartChannel request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* StartChannelRequest request =
* StartChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.startChannelOperationCallable().futureCall(request);
* // Do something.
* ChannelOperationResponse response = future.get();
* }
* }
*/
public final OperationCallable
startChannelOperationCallable() {
return stub.startChannelOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts the specified channel. Part of the video pipeline will be created only when the
* StartChannel request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* StartChannelRequest request =
* StartChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.startChannelCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable startChannelCallable() {
return stub.startChannelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops the specified channel. Part of the video pipeline will be released when the StopChannel
* request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ChannelName name = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]");
* ChannelOperationResponse response = livestreamServiceClient.stopChannelAsync(name).get();
* }
* }
*
* @param name Required. The name of the channel resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture stopChannelAsync(
ChannelName name) {
StopChannelRequest request =
StopChannelRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return stopChannelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops the specified channel. Part of the video pipeline will be released when the StopChannel
* request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString();
* ChannelOperationResponse response = livestreamServiceClient.stopChannelAsync(name).get();
* }
* }
*
* @param name Required. The name of the channel resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture stopChannelAsync(
String name) {
StopChannelRequest request = StopChannelRequest.newBuilder().setName(name).build();
return stopChannelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops the specified channel. Part of the video pipeline will be released when the StopChannel
* request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* StopChannelRequest request =
* StopChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setRequestId("requestId693933066")
* .build();
* ChannelOperationResponse response = livestreamServiceClient.stopChannelAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture stopChannelAsync(
StopChannelRequest request) {
return stopChannelOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops the specified channel. Part of the video pipeline will be released when the StopChannel
* request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* StopChannelRequest request =
* StopChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.stopChannelOperationCallable().futureCall(request);
* // Do something.
* ChannelOperationResponse response = future.get();
* }
* }
*/
public final OperationCallable
stopChannelOperationCallable() {
return stub.stopChannelOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops the specified channel. Part of the video pipeline will be released when the StopChannel
* request is received by the server.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* StopChannelRequest request =
* StopChannelRequest.newBuilder()
* .setName(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.stopChannelCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable stopChannelCallable() {
return stub.stopChannelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an input with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Input input = Input.newBuilder().build();
* String inputId = "inputId1954846341";
* Input response = livestreamServiceClient.createInputAsync(parent, input, inputId).get();
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @param input Required. The input resource to be created.
* @param inputId Required. The ID of the input resource to be created. This value must be 1-63
* characters, begin and end with `[a-z0-9]`, could contain dashes (-) in between.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createInputAsync(
LocationName parent, Input input, String inputId) {
CreateInputRequest request =
CreateInputRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setInput(input)
.setInputId(inputId)
.build();
return createInputAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an input with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Input input = Input.newBuilder().build();
* String inputId = "inputId1954846341";
* Input response = livestreamServiceClient.createInputAsync(parent, input, inputId).get();
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @param input Required. The input resource to be created.
* @param inputId Required. The ID of the input resource to be created. This value must be 1-63
* characters, begin and end with `[a-z0-9]`, could contain dashes (-) in between.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createInputAsync(
String parent, Input input, String inputId) {
CreateInputRequest request =
CreateInputRequest.newBuilder()
.setParent(parent)
.setInput(input)
.setInputId(inputId)
.build();
return createInputAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an input with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateInputRequest request =
* CreateInputRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setInput(Input.newBuilder().build())
* .setInputId("inputId1954846341")
* .setRequestId("requestId693933066")
* .build();
* Input response = livestreamServiceClient.createInputAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createInputAsync(
CreateInputRequest request) {
return createInputOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an input with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateInputRequest request =
* CreateInputRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setInput(Input.newBuilder().build())
* .setInputId("inputId1954846341")
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.createInputOperationCallable().futureCall(request);
* // Do something.
* Input response = future.get();
* }
* }
*/
public final OperationCallable
createInputOperationCallable() {
return stub.createInputOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an input with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateInputRequest request =
* CreateInputRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setInput(Input.newBuilder().build())
* .setInputId("inputId1954846341")
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.createInputCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createInputCallable() {
return stub.createInputCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all inputs in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Input element : livestreamServiceClient.listInputs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInputsPagedResponse listInputs(LocationName parent) {
ListInputsRequest request =
ListInputsRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listInputs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all inputs in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Input element : livestreamServiceClient.listInputs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInputsPagedResponse listInputs(String parent) {
ListInputsRequest request = ListInputsRequest.newBuilder().setParent(parent).build();
return listInputs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all inputs in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListInputsRequest request =
* ListInputsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Input element : livestreamServiceClient.listInputs(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListInputsPagedResponse listInputs(ListInputsRequest request) {
return listInputsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all inputs in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListInputsRequest request =
* ListInputsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* livestreamServiceClient.listInputsPagedCallable().futureCall(request);
* // Do something.
* for (Input element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listInputsPagedCallable() {
return stub.listInputsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all inputs in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListInputsRequest request =
* ListInputsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListInputsResponse response = livestreamServiceClient.listInputsCallable().call(request);
* for (Input element : response.getInputsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listInputsCallable() {
return stub.listInputsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* InputName name = InputName.of("[PROJECT]", "[LOCATION]", "[INPUT]");
* Input response = livestreamServiceClient.getInput(name);
* }
* }
*
* @param name Required. The name of the input resource, in the form of:
* `projects/{project}/locations/{location}/inputs/{inputId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Input getInput(InputName name) {
GetInputRequest request =
GetInputRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getInput(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = InputName.of("[PROJECT]", "[LOCATION]", "[INPUT]").toString();
* Input response = livestreamServiceClient.getInput(name);
* }
* }
*
* @param name Required. The name of the input resource, in the form of:
* `projects/{project}/locations/{location}/inputs/{inputId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Input getInput(String name) {
GetInputRequest request = GetInputRequest.newBuilder().setName(name).build();
return getInput(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetInputRequest request =
* GetInputRequest.newBuilder()
* .setName(InputName.of("[PROJECT]", "[LOCATION]", "[INPUT]").toString())
* .build();
* Input response = livestreamServiceClient.getInput(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Input getInput(GetInputRequest request) {
return getInputCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetInputRequest request =
* GetInputRequest.newBuilder()
* .setName(InputName.of("[PROJECT]", "[LOCATION]", "[INPUT]").toString())
* .build();
* ApiFuture future = livestreamServiceClient.getInputCallable().futureCall(request);
* // Do something.
* Input response = future.get();
* }
* }
*/
public final UnaryCallable getInputCallable() {
return stub.getInputCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* InputName name = InputName.of("[PROJECT]", "[LOCATION]", "[INPUT]");
* livestreamServiceClient.deleteInputAsync(name).get();
* }
* }
*
* @param name Required. The name of the input resource, in the form of:
* `projects/{project}/locations/{location}/inputs/{inputId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteInputAsync(InputName name) {
DeleteInputRequest request =
DeleteInputRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteInputAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = InputName.of("[PROJECT]", "[LOCATION]", "[INPUT]").toString();
* livestreamServiceClient.deleteInputAsync(name).get();
* }
* }
*
* @param name Required. The name of the input resource, in the form of:
* `projects/{project}/locations/{location}/inputs/{inputId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteInputAsync(String name) {
DeleteInputRequest request = DeleteInputRequest.newBuilder().setName(name).build();
return deleteInputAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteInputRequest request =
* DeleteInputRequest.newBuilder()
* .setName(InputName.of("[PROJECT]", "[LOCATION]", "[INPUT]").toString())
* .setRequestId("requestId693933066")
* .build();
* livestreamServiceClient.deleteInputAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteInputAsync(
DeleteInputRequest request) {
return deleteInputOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteInputRequest request =
* DeleteInputRequest.newBuilder()
* .setName(InputName.of("[PROJECT]", "[LOCATION]", "[INPUT]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.deleteInputOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteInputOperationCallable() {
return stub.deleteInputOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteInputRequest request =
* DeleteInputRequest.newBuilder()
* .setName(InputName.of("[PROJECT]", "[LOCATION]", "[INPUT]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.deleteInputCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteInputCallable() {
return stub.deleteInputCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* Input input = Input.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Input response = livestreamServiceClient.updateInputAsync(input, updateMask).get();
* }
* }
*
* @param input Required. The input resource to be updated.
* @param updateMask Field mask is used to specify the fields to be overwritten in the Input
* resource by the update. You can only update the following fields:
*
* - [`preprocessingConfig`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.inputs#PreprocessingConfig)
*
- [`securityRules`](https://cloud.google.com/livestream/docs/reference/rest/v1/projects.locations.inputs#SecurityRule)
*
* The fields specified in the update_mask are relative to the resource, not the full
* request. A field will be overwritten if it is in the mask.
*
If the mask is not present, then each field from the list above is updated if the field
* appears in the request payload. To unset a field, add the field to the update mask and
* remove it from the request payload.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateInputAsync(
Input input, FieldMask updateMask) {
UpdateInputRequest request =
UpdateInputRequest.newBuilder().setInput(input).setUpdateMask(updateMask).build();
return updateInputAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified input.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* UpdateInputRequest request =
* UpdateInputRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setInput(Input.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Input response = livestreamServiceClient.updateInputAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateInputAsync(
UpdateInputRequest request) {
return updateInputOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* UpdateInputRequest request =
* UpdateInputRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setInput(Input.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.updateInputOperationCallable().futureCall(request);
* // Do something.
* Input response = future.get();
* }
* }
*/
public final OperationCallable
updateInputOperationCallable() {
return stub.updateInputOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified input.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* UpdateInputRequest request =
* UpdateInputRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setInput(Input.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.updateInputCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateInputCallable() {
return stub.updateInputCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an event with the provided unique ID in the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ChannelName parent = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]");
* Event event = Event.newBuilder().build();
* String eventId = "eventId-1376502443";
* Event response = livestreamServiceClient.createEvent(parent, event, eventId);
* }
* }
*
* @param parent Required. The parent channel for the resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @param event Required. The event resource to be created.
* @param eventId Required. The ID of the event resource to be created. This value must be 1-63
* characters, begin and end with `[a-z0-9]`, could contain dashes (-) in between.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Event createEvent(ChannelName parent, Event event, String eventId) {
CreateEventRequest request =
CreateEventRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setEvent(event)
.setEventId(eventId)
.build();
return createEvent(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an event with the provided unique ID in the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String parent = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString();
* Event event = Event.newBuilder().build();
* String eventId = "eventId-1376502443";
* Event response = livestreamServiceClient.createEvent(parent, event, eventId);
* }
* }
*
* @param parent Required. The parent channel for the resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @param event Required. The event resource to be created.
* @param eventId Required. The ID of the event resource to be created. This value must be 1-63
* characters, begin and end with `[a-z0-9]`, could contain dashes (-) in between.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Event createEvent(String parent, Event event, String eventId) {
CreateEventRequest request =
CreateEventRequest.newBuilder()
.setParent(parent)
.setEvent(event)
.setEventId(eventId)
.build();
return createEvent(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an event with the provided unique ID in the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateEventRequest request =
* CreateEventRequest.newBuilder()
* .setParent(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setEvent(Event.newBuilder().build())
* .setEventId("eventId-1376502443")
* .setRequestId("requestId693933066")
* .build();
* Event response = livestreamServiceClient.createEvent(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Event createEvent(CreateEventRequest request) {
return createEventCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an event with the provided unique ID in the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateEventRequest request =
* CreateEventRequest.newBuilder()
* .setParent(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setEvent(Event.newBuilder().build())
* .setEventId("eventId-1376502443")
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = livestreamServiceClient.createEventCallable().futureCall(request);
* // Do something.
* Event response = future.get();
* }
* }
*/
public final UnaryCallable createEventCallable() {
return stub.createEventCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all events in the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ChannelName parent = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]");
* for (Event element : livestreamServiceClient.listEvents(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent channel for the resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEventsPagedResponse listEvents(ChannelName parent) {
ListEventsRequest request =
ListEventsRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listEvents(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all events in the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String parent = ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString();
* for (Event element : livestreamServiceClient.listEvents(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent channel for the resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEventsPagedResponse listEvents(String parent) {
ListEventsRequest request = ListEventsRequest.newBuilder().setParent(parent).build();
return listEvents(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all events in the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListEventsRequest request =
* ListEventsRequest.newBuilder()
* .setParent(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Event element : livestreamServiceClient.listEvents(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEventsPagedResponse listEvents(ListEventsRequest request) {
return listEventsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all events in the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListEventsRequest request =
* ListEventsRequest.newBuilder()
* .setParent(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* livestreamServiceClient.listEventsPagedCallable().futureCall(request);
* // Do something.
* for (Event element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listEventsPagedCallable() {
return stub.listEventsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all events in the specified channel.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListEventsRequest request =
* ListEventsRequest.newBuilder()
* .setParent(ChannelName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListEventsResponse response = livestreamServiceClient.listEventsCallable().call(request);
* for (Event element : response.getEventsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listEventsCallable() {
return stub.listEventsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* EventName name = EventName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]", "[EVENT]");
* Event response = livestreamServiceClient.getEvent(name);
* }
* }
*
* @param name Required. The name of the event resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}/events/{eventId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Event getEvent(EventName name) {
GetEventRequest request =
GetEventRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getEvent(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = EventName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]", "[EVENT]").toString();
* Event response = livestreamServiceClient.getEvent(name);
* }
* }
*
* @param name Required. The name of the event resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}/events/{eventId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Event getEvent(String name) {
GetEventRequest request = GetEventRequest.newBuilder().setName(name).build();
return getEvent(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetEventRequest request =
* GetEventRequest.newBuilder()
* .setName(EventName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]", "[EVENT]").toString())
* .build();
* Event response = livestreamServiceClient.getEvent(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Event getEvent(GetEventRequest request) {
return getEventCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetEventRequest request =
* GetEventRequest.newBuilder()
* .setName(EventName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]", "[EVENT]").toString())
* .build();
* ApiFuture future = livestreamServiceClient.getEventCallable().futureCall(request);
* // Do something.
* Event response = future.get();
* }
* }
*/
public final UnaryCallable getEventCallable() {
return stub.getEventCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* EventName name = EventName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]", "[EVENT]");
* livestreamServiceClient.deleteEvent(name);
* }
* }
*
* @param name Required. The name of the event resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}/events/{eventId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteEvent(EventName name) {
DeleteEventRequest request =
DeleteEventRequest.newBuilder().setName(name == null ? null : name.toString()).build();
deleteEvent(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = EventName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]", "[EVENT]").toString();
* livestreamServiceClient.deleteEvent(name);
* }
* }
*
* @param name Required. The name of the event resource, in the form of:
* `projects/{project}/locations/{location}/channels/{channelId}/events/{eventId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteEvent(String name) {
DeleteEventRequest request = DeleteEventRequest.newBuilder().setName(name).build();
deleteEvent(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteEventRequest request =
* DeleteEventRequest.newBuilder()
* .setName(EventName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]", "[EVENT]").toString())
* .setRequestId("requestId693933066")
* .build();
* livestreamServiceClient.deleteEvent(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteEvent(DeleteEventRequest request) {
deleteEventCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteEventRequest request =
* DeleteEventRequest.newBuilder()
* .setName(EventName.of("[PROJECT]", "[LOCATION]", "[CHANNEL]", "[EVENT]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = livestreamServiceClient.deleteEventCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteEventCallable() {
return stub.deleteEventCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Asset with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Asset asset = Asset.newBuilder().build();
* String assetId = "assetId-704776149";
* Asset response = livestreamServiceClient.createAssetAsync(parent, asset, assetId).get();
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @param asset Required. The asset resource to be created.
* @param assetId Required. The ID of the asset resource to be created. This value must be 1-63
* characters, begin and end with `[a-z0-9]`, could contain dashes (-) in between.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createAssetAsync(
LocationName parent, Asset asset, String assetId) {
CreateAssetRequest request =
CreateAssetRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setAsset(asset)
.setAssetId(assetId)
.build();
return createAssetAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Asset with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Asset asset = Asset.newBuilder().build();
* String assetId = "assetId-704776149";
* Asset response = livestreamServiceClient.createAssetAsync(parent, asset, assetId).get();
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @param asset Required. The asset resource to be created.
* @param assetId Required. The ID of the asset resource to be created. This value must be 1-63
* characters, begin and end with `[a-z0-9]`, could contain dashes (-) in between.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createAssetAsync(
String parent, Asset asset, String assetId) {
CreateAssetRequest request =
CreateAssetRequest.newBuilder()
.setParent(parent)
.setAsset(asset)
.setAssetId(assetId)
.build();
return createAssetAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Asset with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateAssetRequest request =
* CreateAssetRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setAsset(Asset.newBuilder().build())
* .setAssetId("assetId-704776149")
* .setRequestId("requestId693933066")
* .build();
* Asset response = livestreamServiceClient.createAssetAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createAssetAsync(
CreateAssetRequest request) {
return createAssetOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Asset with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateAssetRequest request =
* CreateAssetRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setAsset(Asset.newBuilder().build())
* .setAssetId("assetId-704776149")
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.createAssetOperationCallable().futureCall(request);
* // Do something.
* Asset response = future.get();
* }
* }
*/
public final OperationCallable
createAssetOperationCallable() {
return stub.createAssetOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Asset with the provided unique ID in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* CreateAssetRequest request =
* CreateAssetRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setAsset(Asset.newBuilder().build())
* .setAssetId("assetId-704776149")
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.createAssetCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createAssetCallable() {
return stub.createAssetCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified asset if it is not used.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* AssetName name = AssetName.of("[PROJECT]", "[LOCATION]", "[ASSET]");
* livestreamServiceClient.deleteAssetAsync(name).get();
* }
* }
*
* @param name Required. The name of the asset resource, in the form of:
* `projects/{project}/locations/{location}/assets/{assetId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteAssetAsync(AssetName name) {
DeleteAssetRequest request =
DeleteAssetRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteAssetAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified asset if it is not used.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = AssetName.of("[PROJECT]", "[LOCATION]", "[ASSET]").toString();
* livestreamServiceClient.deleteAssetAsync(name).get();
* }
* }
*
* @param name Required. The name of the asset resource, in the form of:
* `projects/{project}/locations/{location}/assets/{assetId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteAssetAsync(String name) {
DeleteAssetRequest request = DeleteAssetRequest.newBuilder().setName(name).build();
return deleteAssetAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified asset if it is not used.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteAssetRequest request =
* DeleteAssetRequest.newBuilder()
* .setName(AssetName.of("[PROJECT]", "[LOCATION]", "[ASSET]").toString())
* .setRequestId("requestId693933066")
* .build();
* livestreamServiceClient.deleteAssetAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteAssetAsync(
DeleteAssetRequest request) {
return deleteAssetOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified asset if it is not used.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteAssetRequest request =
* DeleteAssetRequest.newBuilder()
* .setName(AssetName.of("[PROJECT]", "[LOCATION]", "[ASSET]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.deleteAssetOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteAssetOperationCallable() {
return stub.deleteAssetOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified asset if it is not used.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* DeleteAssetRequest request =
* DeleteAssetRequest.newBuilder()
* .setName(AssetName.of("[PROJECT]", "[LOCATION]", "[ASSET]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.deleteAssetCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteAssetCallable() {
return stub.deleteAssetCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified asset.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* AssetName name = AssetName.of("[PROJECT]", "[LOCATION]", "[ASSET]");
* Asset response = livestreamServiceClient.getAsset(name);
* }
* }
*
* @param name Required. Name of the resource, in the following form:
* `projects/{project}/locations/{location}/assets/{asset}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Asset getAsset(AssetName name) {
GetAssetRequest request =
GetAssetRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getAsset(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified asset.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = AssetName.of("[PROJECT]", "[LOCATION]", "[ASSET]").toString();
* Asset response = livestreamServiceClient.getAsset(name);
* }
* }
*
* @param name Required. Name of the resource, in the following form:
* `projects/{project}/locations/{location}/assets/{asset}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Asset getAsset(String name) {
GetAssetRequest request = GetAssetRequest.newBuilder().setName(name).build();
return getAsset(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified asset.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetAssetRequest request =
* GetAssetRequest.newBuilder()
* .setName(AssetName.of("[PROJECT]", "[LOCATION]", "[ASSET]").toString())
* .build();
* Asset response = livestreamServiceClient.getAsset(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Asset getAsset(GetAssetRequest request) {
return getAssetCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified asset.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetAssetRequest request =
* GetAssetRequest.newBuilder()
* .setName(AssetName.of("[PROJECT]", "[LOCATION]", "[ASSET]").toString())
* .build();
* ApiFuture future = livestreamServiceClient.getAssetCallable().futureCall(request);
* // Do something.
* Asset response = future.get();
* }
* }
*/
public final UnaryCallable getAssetCallable() {
return stub.getAssetCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all assets in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Asset element : livestreamServiceClient.listAssets(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAssetsPagedResponse listAssets(LocationName parent) {
ListAssetsRequest request =
ListAssetsRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listAssets(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all assets in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Asset element : livestreamServiceClient.listAssets(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent location for the resource, in the form of:
* `projects/{project}/locations/{location}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAssetsPagedResponse listAssets(String parent) {
ListAssetsRequest request = ListAssetsRequest.newBuilder().setParent(parent).build();
return listAssets(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all assets in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListAssetsRequest request =
* ListAssetsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Asset element : livestreamServiceClient.listAssets(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAssetsPagedResponse listAssets(ListAssetsRequest request) {
return listAssetsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all assets in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListAssetsRequest request =
* ListAssetsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* livestreamServiceClient.listAssetsPagedCallable().futureCall(request);
* // Do something.
* for (Asset element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listAssetsPagedCallable() {
return stub.listAssetsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of all assets in the specified region.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListAssetsRequest request =
* ListAssetsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListAssetsResponse response = livestreamServiceClient.listAssetsCallable().call(request);
* for (Asset element : response.getAssetsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listAssetsCallable() {
return stub.listAssetsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified pool.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* PoolName name = PoolName.of("[PROJECT]", "[LOCATION]", "[POOL]");
* Pool response = livestreamServiceClient.getPool(name);
* }
* }
*
* @param name Required. The name of the pool resource, in the form of:
* `projects/{project}/locations/{location}/pools/{poolId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Pool getPool(PoolName name) {
GetPoolRequest request =
GetPoolRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getPool(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified pool.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* String name = PoolName.of("[PROJECT]", "[LOCATION]", "[POOL]").toString();
* Pool response = livestreamServiceClient.getPool(name);
* }
* }
*
* @param name Required. The name of the pool resource, in the form of:
* `projects/{project}/locations/{location}/pools/{poolId}`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Pool getPool(String name) {
GetPoolRequest request = GetPoolRequest.newBuilder().setName(name).build();
return getPool(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified pool.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetPoolRequest request =
* GetPoolRequest.newBuilder()
* .setName(PoolName.of("[PROJECT]", "[LOCATION]", "[POOL]").toString())
* .build();
* Pool response = livestreamServiceClient.getPool(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Pool getPool(GetPoolRequest request) {
return getPoolCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the specified pool.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetPoolRequest request =
* GetPoolRequest.newBuilder()
* .setName(PoolName.of("[PROJECT]", "[LOCATION]", "[POOL]").toString())
* .build();
* ApiFuture future = livestreamServiceClient.getPoolCallable().futureCall(request);
* // Do something.
* Pool response = future.get();
* }
* }
*/
public final UnaryCallable getPoolCallable() {
return stub.getPoolCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified pool.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* Pool pool = Pool.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Pool response = livestreamServiceClient.updatePoolAsync(pool, updateMask).get();
* }
* }
*
* @param pool Required. The pool resource to be updated.
* @param updateMask Field mask is used to specify the fields to be overwritten in the Pool
* resource by the update. You can only update the following fields:
*
* - `networkConfig`
*
* The fields specified in the update_mask are relative to the resource, not the full
* request. A field will be overwritten if it is in the mask.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updatePoolAsync(
Pool pool, FieldMask updateMask) {
UpdatePoolRequest request =
UpdatePoolRequest.newBuilder().setPool(pool).setUpdateMask(updateMask).build();
return updatePoolAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified pool.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* UpdatePoolRequest request =
* UpdatePoolRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setPool(Pool.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Pool response = livestreamServiceClient.updatePoolAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updatePoolAsync(UpdatePoolRequest request) {
return updatePoolOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified pool.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* UpdatePoolRequest request =
* UpdatePoolRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setPool(Pool.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* livestreamServiceClient.updatePoolOperationCallable().futureCall(request);
* // Do something.
* Pool response = future.get();
* }
* }
*/
public final OperationCallable
updatePoolOperationCallable() {
return stub.updatePoolOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the specified pool.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* UpdatePoolRequest request =
* UpdatePoolRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setPool(Pool.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* livestreamServiceClient.updatePoolCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updatePoolCallable() {
return stub.updatePoolCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : livestreamServiceClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* livestreamServiceClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response =
* livestreamServiceClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = livestreamServiceClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (LivestreamServiceClient livestreamServiceClient = LivestreamServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future =
* livestreamServiceClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListChannelsPagedResponse
extends AbstractPagedListResponse<
ListChannelsRequest,
ListChannelsResponse,
Channel,
ListChannelsPage,
ListChannelsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListChannelsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListChannelsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListChannelsPagedResponse(ListChannelsPage page) {
super(page, ListChannelsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListChannelsPage
extends AbstractPage {
private ListChannelsPage(
PageContext context,
ListChannelsResponse response) {
super(context, response);
}
private static ListChannelsPage createEmptyPage() {
return new ListChannelsPage(null, null);
}
@Override
protected ListChannelsPage createPage(
PageContext context,
ListChannelsResponse response) {
return new ListChannelsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListChannelsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListChannelsRequest,
ListChannelsResponse,
Channel,
ListChannelsPage,
ListChannelsFixedSizeCollection> {
private ListChannelsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListChannelsFixedSizeCollection createEmptyCollection() {
return new ListChannelsFixedSizeCollection(null, 0);
}
@Override
protected ListChannelsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListChannelsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListInputsPagedResponse
extends AbstractPagedListResponse<
ListInputsRequest,
ListInputsResponse,
Input,
ListInputsPage,
ListInputsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListInputsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListInputsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListInputsPagedResponse(ListInputsPage page) {
super(page, ListInputsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListInputsPage
extends AbstractPage {
private ListInputsPage(
PageContext context,
ListInputsResponse response) {
super(context, response);
}
private static ListInputsPage createEmptyPage() {
return new ListInputsPage(null, null);
}
@Override
protected ListInputsPage createPage(
PageContext context,
ListInputsResponse response) {
return new ListInputsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListInputsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListInputsRequest,
ListInputsResponse,
Input,
ListInputsPage,
ListInputsFixedSizeCollection> {
private ListInputsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListInputsFixedSizeCollection createEmptyCollection() {
return new ListInputsFixedSizeCollection(null, 0);
}
@Override
protected ListInputsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListInputsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListEventsPagedResponse
extends AbstractPagedListResponse<
ListEventsRequest,
ListEventsResponse,
Event,
ListEventsPage,
ListEventsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListEventsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListEventsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListEventsPagedResponse(ListEventsPage page) {
super(page, ListEventsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListEventsPage
extends AbstractPage {
private ListEventsPage(
PageContext context,
ListEventsResponse response) {
super(context, response);
}
private static ListEventsPage createEmptyPage() {
return new ListEventsPage(null, null);
}
@Override
protected ListEventsPage createPage(
PageContext context,
ListEventsResponse response) {
return new ListEventsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListEventsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListEventsRequest,
ListEventsResponse,
Event,
ListEventsPage,
ListEventsFixedSizeCollection> {
private ListEventsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListEventsFixedSizeCollection createEmptyCollection() {
return new ListEventsFixedSizeCollection(null, 0);
}
@Override
protected ListEventsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListEventsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListAssetsPagedResponse
extends AbstractPagedListResponse<
ListAssetsRequest,
ListAssetsResponse,
Asset,
ListAssetsPage,
ListAssetsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListAssetsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListAssetsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListAssetsPagedResponse(ListAssetsPage page) {
super(page, ListAssetsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListAssetsPage
extends AbstractPage {
private ListAssetsPage(
PageContext context,
ListAssetsResponse response) {
super(context, response);
}
private static ListAssetsPage createEmptyPage() {
return new ListAssetsPage(null, null);
}
@Override
protected ListAssetsPage createPage(
PageContext context,
ListAssetsResponse response) {
return new ListAssetsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListAssetsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListAssetsRequest,
ListAssetsResponse,
Asset,
ListAssetsPage,
ListAssetsFixedSizeCollection> {
private ListAssetsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListAssetsFixedSizeCollection createEmptyCollection() {
return new ListAssetsFixedSizeCollection(null, 0);
}
@Override
protected ListAssetsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListAssetsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}