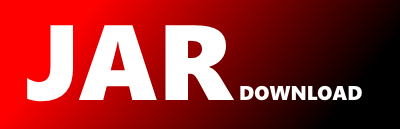
com.google.cloud.video.livestream.v1.stub.GrpcLivestreamServiceStub Maven / Gradle / Ivy
Show all versions of google-cloud-live-stream Show documentation
/*
* Copyright 2023 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.video.livestream.v1.stub;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListAssetsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListChannelsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListEventsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListInputsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListLocationsPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.video.livestream.v1.Asset;
import com.google.cloud.video.livestream.v1.Channel;
import com.google.cloud.video.livestream.v1.ChannelOperationResponse;
import com.google.cloud.video.livestream.v1.CreateAssetRequest;
import com.google.cloud.video.livestream.v1.CreateChannelRequest;
import com.google.cloud.video.livestream.v1.CreateEventRequest;
import com.google.cloud.video.livestream.v1.CreateInputRequest;
import com.google.cloud.video.livestream.v1.DeleteAssetRequest;
import com.google.cloud.video.livestream.v1.DeleteChannelRequest;
import com.google.cloud.video.livestream.v1.DeleteEventRequest;
import com.google.cloud.video.livestream.v1.DeleteInputRequest;
import com.google.cloud.video.livestream.v1.Event;
import com.google.cloud.video.livestream.v1.GetAssetRequest;
import com.google.cloud.video.livestream.v1.GetChannelRequest;
import com.google.cloud.video.livestream.v1.GetEventRequest;
import com.google.cloud.video.livestream.v1.GetInputRequest;
import com.google.cloud.video.livestream.v1.GetPoolRequest;
import com.google.cloud.video.livestream.v1.Input;
import com.google.cloud.video.livestream.v1.ListAssetsRequest;
import com.google.cloud.video.livestream.v1.ListAssetsResponse;
import com.google.cloud.video.livestream.v1.ListChannelsRequest;
import com.google.cloud.video.livestream.v1.ListChannelsResponse;
import com.google.cloud.video.livestream.v1.ListEventsRequest;
import com.google.cloud.video.livestream.v1.ListEventsResponse;
import com.google.cloud.video.livestream.v1.ListInputsRequest;
import com.google.cloud.video.livestream.v1.ListInputsResponse;
import com.google.cloud.video.livestream.v1.OperationMetadata;
import com.google.cloud.video.livestream.v1.Pool;
import com.google.cloud.video.livestream.v1.StartChannelRequest;
import com.google.cloud.video.livestream.v1.StopChannelRequest;
import com.google.cloud.video.livestream.v1.UpdateChannelRequest;
import com.google.cloud.video.livestream.v1.UpdateInputRequest;
import com.google.cloud.video.livestream.v1.UpdatePoolRequest;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import com.google.protobuf.Empty;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the LivestreamService service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public class GrpcLivestreamServiceStub extends LivestreamServiceStub {
private static final MethodDescriptor
createChannelMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/CreateChannel")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateChannelRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listChannelsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/ListChannels")
.setRequestMarshaller(ProtoUtils.marshaller(ListChannelsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListChannelsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getChannelMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/GetChannel")
.setRequestMarshaller(ProtoUtils.marshaller(GetChannelRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Channel.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteChannelMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/DeleteChannel")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteChannelRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateChannelMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/UpdateChannel")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateChannelRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
startChannelMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/StartChannel")
.setRequestMarshaller(ProtoUtils.marshaller(StartChannelRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor stopChannelMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/StopChannel")
.setRequestMarshaller(ProtoUtils.marshaller(StopChannelRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor createInputMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/CreateInput")
.setRequestMarshaller(ProtoUtils.marshaller(CreateInputRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listInputsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/ListInputs")
.setRequestMarshaller(ProtoUtils.marshaller(ListInputsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListInputsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getInputMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/GetInput")
.setRequestMarshaller(ProtoUtils.marshaller(GetInputRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Input.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteInputMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/DeleteInput")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteInputRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor updateInputMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/UpdateInput")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateInputRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor createEventMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/CreateEvent")
.setRequestMarshaller(ProtoUtils.marshaller(CreateEventRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Event.getDefaultInstance()))
.build();
private static final MethodDescriptor
listEventsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/ListEvents")
.setRequestMarshaller(ProtoUtils.marshaller(ListEventsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListEventsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getEventMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/GetEvent")
.setRequestMarshaller(ProtoUtils.marshaller(GetEventRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Event.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteEventMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/DeleteEvent")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteEventRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor createAssetMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/CreateAsset")
.setRequestMarshaller(ProtoUtils.marshaller(CreateAssetRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteAssetMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/DeleteAsset")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteAssetRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor getAssetMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/GetAsset")
.setRequestMarshaller(ProtoUtils.marshaller(GetAssetRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Asset.getDefaultInstance()))
.build();
private static final MethodDescriptor
listAssetsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/ListAssets")
.setRequestMarshaller(ProtoUtils.marshaller(ListAssetsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListAssetsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getPoolMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/GetPool")
.setRequestMarshaller(ProtoUtils.marshaller(GetPoolRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Pool.getDefaultInstance()))
.build();
private static final MethodDescriptor updatePoolMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.video.livestream.v1.LivestreamService/UpdatePool")
.setRequestMarshaller(ProtoUtils.marshaller(UpdatePoolRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listLocationsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/ListLocations")
.setRequestMarshaller(
ProtoUtils.marshaller(ListLocationsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListLocationsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getLocationMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/GetLocation")
.setRequestMarshaller(ProtoUtils.marshaller(GetLocationRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Location.getDefaultInstance()))
.build();
private final UnaryCallable createChannelCallable;
private final OperationCallable
createChannelOperationCallable;
private final UnaryCallable listChannelsCallable;
private final UnaryCallable
listChannelsPagedCallable;
private final UnaryCallable getChannelCallable;
private final UnaryCallable deleteChannelCallable;
private final OperationCallable
deleteChannelOperationCallable;
private final UnaryCallable updateChannelCallable;
private final OperationCallable
updateChannelOperationCallable;
private final UnaryCallable startChannelCallable;
private final OperationCallable
startChannelOperationCallable;
private final UnaryCallable stopChannelCallable;
private final OperationCallable
stopChannelOperationCallable;
private final UnaryCallable createInputCallable;
private final OperationCallable
createInputOperationCallable;
private final UnaryCallable listInputsCallable;
private final UnaryCallable listInputsPagedCallable;
private final UnaryCallable getInputCallable;
private final UnaryCallable deleteInputCallable;
private final OperationCallable
deleteInputOperationCallable;
private final UnaryCallable updateInputCallable;
private final OperationCallable
updateInputOperationCallable;
private final UnaryCallable createEventCallable;
private final UnaryCallable listEventsCallable;
private final UnaryCallable listEventsPagedCallable;
private final UnaryCallable getEventCallable;
private final UnaryCallable deleteEventCallable;
private final UnaryCallable createAssetCallable;
private final OperationCallable
createAssetOperationCallable;
private final UnaryCallable deleteAssetCallable;
private final OperationCallable
deleteAssetOperationCallable;
private final UnaryCallable getAssetCallable;
private final UnaryCallable listAssetsCallable;
private final UnaryCallable listAssetsPagedCallable;
private final UnaryCallable getPoolCallable;
private final UnaryCallable updatePoolCallable;
private final OperationCallable
updatePoolOperationCallable;
private final UnaryCallable listLocationsCallable;
private final UnaryCallable
listLocationsPagedCallable;
private final UnaryCallable getLocationCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcLivestreamServiceStub create(LivestreamServiceStubSettings settings)
throws IOException {
return new GrpcLivestreamServiceStub(settings, ClientContext.create(settings));
}
public static final GrpcLivestreamServiceStub create(ClientContext clientContext)
throws IOException {
return new GrpcLivestreamServiceStub(
LivestreamServiceStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcLivestreamServiceStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcLivestreamServiceStub(
LivestreamServiceStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcLivestreamServiceStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcLivestreamServiceStub(
LivestreamServiceStubSettings settings, ClientContext clientContext) throws IOException {
this(settings, clientContext, new GrpcLivestreamServiceCallableFactory());
}
/**
* Constructs an instance of GrpcLivestreamServiceStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcLivestreamServiceStub(
LivestreamServiceStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings createChannelTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createChannelMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings listChannelsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listChannelsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getChannelTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getChannelMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteChannelTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteChannelMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateChannelTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateChannelMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("channel.name", String.valueOf(request.getChannel().getName()));
return builder.build();
})
.build();
GrpcCallSettings startChannelTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(startChannelMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings stopChannelTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(stopChannelMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createInputTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createInputMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings listInputsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listInputsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getInputTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getInputMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteInputTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteInputMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateInputTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateInputMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("input.name", String.valueOf(request.getInput().getName()));
return builder.build();
})
.build();
GrpcCallSettings createEventTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createEventMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings listEventsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listEventsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getEventTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getEventMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteEventTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteEventMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createAssetTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createAssetMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings deleteAssetTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteAssetMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getAssetTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getAssetMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listAssetsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listAssetsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getPoolTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getPoolMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updatePoolTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updatePoolMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("pool.name", String.valueOf(request.getPool().getName()));
return builder.build();
})
.build();
GrpcCallSettings listLocationsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listLocationsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getLocationTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getLocationMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
this.createChannelCallable =
callableFactory.createUnaryCallable(
createChannelTransportSettings, settings.createChannelSettings(), clientContext);
this.createChannelOperationCallable =
callableFactory.createOperationCallable(
createChannelTransportSettings,
settings.createChannelOperationSettings(),
clientContext,
operationsStub);
this.listChannelsCallable =
callableFactory.createUnaryCallable(
listChannelsTransportSettings, settings.listChannelsSettings(), clientContext);
this.listChannelsPagedCallable =
callableFactory.createPagedCallable(
listChannelsTransportSettings, settings.listChannelsSettings(), clientContext);
this.getChannelCallable =
callableFactory.createUnaryCallable(
getChannelTransportSettings, settings.getChannelSettings(), clientContext);
this.deleteChannelCallable =
callableFactory.createUnaryCallable(
deleteChannelTransportSettings, settings.deleteChannelSettings(), clientContext);
this.deleteChannelOperationCallable =
callableFactory.createOperationCallable(
deleteChannelTransportSettings,
settings.deleteChannelOperationSettings(),
clientContext,
operationsStub);
this.updateChannelCallable =
callableFactory.createUnaryCallable(
updateChannelTransportSettings, settings.updateChannelSettings(), clientContext);
this.updateChannelOperationCallable =
callableFactory.createOperationCallable(
updateChannelTransportSettings,
settings.updateChannelOperationSettings(),
clientContext,
operationsStub);
this.startChannelCallable =
callableFactory.createUnaryCallable(
startChannelTransportSettings, settings.startChannelSettings(), clientContext);
this.startChannelOperationCallable =
callableFactory.createOperationCallable(
startChannelTransportSettings,
settings.startChannelOperationSettings(),
clientContext,
operationsStub);
this.stopChannelCallable =
callableFactory.createUnaryCallable(
stopChannelTransportSettings, settings.stopChannelSettings(), clientContext);
this.stopChannelOperationCallable =
callableFactory.createOperationCallable(
stopChannelTransportSettings,
settings.stopChannelOperationSettings(),
clientContext,
operationsStub);
this.createInputCallable =
callableFactory.createUnaryCallable(
createInputTransportSettings, settings.createInputSettings(), clientContext);
this.createInputOperationCallable =
callableFactory.createOperationCallable(
createInputTransportSettings,
settings.createInputOperationSettings(),
clientContext,
operationsStub);
this.listInputsCallable =
callableFactory.createUnaryCallable(
listInputsTransportSettings, settings.listInputsSettings(), clientContext);
this.listInputsPagedCallable =
callableFactory.createPagedCallable(
listInputsTransportSettings, settings.listInputsSettings(), clientContext);
this.getInputCallable =
callableFactory.createUnaryCallable(
getInputTransportSettings, settings.getInputSettings(), clientContext);
this.deleteInputCallable =
callableFactory.createUnaryCallable(
deleteInputTransportSettings, settings.deleteInputSettings(), clientContext);
this.deleteInputOperationCallable =
callableFactory.createOperationCallable(
deleteInputTransportSettings,
settings.deleteInputOperationSettings(),
clientContext,
operationsStub);
this.updateInputCallable =
callableFactory.createUnaryCallable(
updateInputTransportSettings, settings.updateInputSettings(), clientContext);
this.updateInputOperationCallable =
callableFactory.createOperationCallable(
updateInputTransportSettings,
settings.updateInputOperationSettings(),
clientContext,
operationsStub);
this.createEventCallable =
callableFactory.createUnaryCallable(
createEventTransportSettings, settings.createEventSettings(), clientContext);
this.listEventsCallable =
callableFactory.createUnaryCallable(
listEventsTransportSettings, settings.listEventsSettings(), clientContext);
this.listEventsPagedCallable =
callableFactory.createPagedCallable(
listEventsTransportSettings, settings.listEventsSettings(), clientContext);
this.getEventCallable =
callableFactory.createUnaryCallable(
getEventTransportSettings, settings.getEventSettings(), clientContext);
this.deleteEventCallable =
callableFactory.createUnaryCallable(
deleteEventTransportSettings, settings.deleteEventSettings(), clientContext);
this.createAssetCallable =
callableFactory.createUnaryCallable(
createAssetTransportSettings, settings.createAssetSettings(), clientContext);
this.createAssetOperationCallable =
callableFactory.createOperationCallable(
createAssetTransportSettings,
settings.createAssetOperationSettings(),
clientContext,
operationsStub);
this.deleteAssetCallable =
callableFactory.createUnaryCallable(
deleteAssetTransportSettings, settings.deleteAssetSettings(), clientContext);
this.deleteAssetOperationCallable =
callableFactory.createOperationCallable(
deleteAssetTransportSettings,
settings.deleteAssetOperationSettings(),
clientContext,
operationsStub);
this.getAssetCallable =
callableFactory.createUnaryCallable(
getAssetTransportSettings, settings.getAssetSettings(), clientContext);
this.listAssetsCallable =
callableFactory.createUnaryCallable(
listAssetsTransportSettings, settings.listAssetsSettings(), clientContext);
this.listAssetsPagedCallable =
callableFactory.createPagedCallable(
listAssetsTransportSettings, settings.listAssetsSettings(), clientContext);
this.getPoolCallable =
callableFactory.createUnaryCallable(
getPoolTransportSettings, settings.getPoolSettings(), clientContext);
this.updatePoolCallable =
callableFactory.createUnaryCallable(
updatePoolTransportSettings, settings.updatePoolSettings(), clientContext);
this.updatePoolOperationCallable =
callableFactory.createOperationCallable(
updatePoolTransportSettings,
settings.updatePoolOperationSettings(),
clientContext,
operationsStub);
this.listLocationsCallable =
callableFactory.createUnaryCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.listLocationsPagedCallable =
callableFactory.createPagedCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.getLocationCallable =
callableFactory.createUnaryCallable(
getLocationTransportSettings, settings.getLocationSettings(), clientContext);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable createChannelCallable() {
return createChannelCallable;
}
@Override
public OperationCallable
createChannelOperationCallable() {
return createChannelOperationCallable;
}
@Override
public UnaryCallable listChannelsCallable() {
return listChannelsCallable;
}
@Override
public UnaryCallable listChannelsPagedCallable() {
return listChannelsPagedCallable;
}
@Override
public UnaryCallable getChannelCallable() {
return getChannelCallable;
}
@Override
public UnaryCallable deleteChannelCallable() {
return deleteChannelCallable;
}
@Override
public OperationCallable
deleteChannelOperationCallable() {
return deleteChannelOperationCallable;
}
@Override
public UnaryCallable updateChannelCallable() {
return updateChannelCallable;
}
@Override
public OperationCallable
updateChannelOperationCallable() {
return updateChannelOperationCallable;
}
@Override
public UnaryCallable startChannelCallable() {
return startChannelCallable;
}
@Override
public OperationCallable
startChannelOperationCallable() {
return startChannelOperationCallable;
}
@Override
public UnaryCallable stopChannelCallable() {
return stopChannelCallable;
}
@Override
public OperationCallable
stopChannelOperationCallable() {
return stopChannelOperationCallable;
}
@Override
public UnaryCallable createInputCallable() {
return createInputCallable;
}
@Override
public OperationCallable
createInputOperationCallable() {
return createInputOperationCallable;
}
@Override
public UnaryCallable listInputsCallable() {
return listInputsCallable;
}
@Override
public UnaryCallable listInputsPagedCallable() {
return listInputsPagedCallable;
}
@Override
public UnaryCallable getInputCallable() {
return getInputCallable;
}
@Override
public UnaryCallable deleteInputCallable() {
return deleteInputCallable;
}
@Override
public OperationCallable
deleteInputOperationCallable() {
return deleteInputOperationCallable;
}
@Override
public UnaryCallable updateInputCallable() {
return updateInputCallable;
}
@Override
public OperationCallable
updateInputOperationCallable() {
return updateInputOperationCallable;
}
@Override
public UnaryCallable createEventCallable() {
return createEventCallable;
}
@Override
public UnaryCallable listEventsCallable() {
return listEventsCallable;
}
@Override
public UnaryCallable listEventsPagedCallable() {
return listEventsPagedCallable;
}
@Override
public UnaryCallable getEventCallable() {
return getEventCallable;
}
@Override
public UnaryCallable deleteEventCallable() {
return deleteEventCallable;
}
@Override
public UnaryCallable createAssetCallable() {
return createAssetCallable;
}
@Override
public OperationCallable
createAssetOperationCallable() {
return createAssetOperationCallable;
}
@Override
public UnaryCallable deleteAssetCallable() {
return deleteAssetCallable;
}
@Override
public OperationCallable
deleteAssetOperationCallable() {
return deleteAssetOperationCallable;
}
@Override
public UnaryCallable getAssetCallable() {
return getAssetCallable;
}
@Override
public UnaryCallable listAssetsCallable() {
return listAssetsCallable;
}
@Override
public UnaryCallable listAssetsPagedCallable() {
return listAssetsPagedCallable;
}
@Override
public UnaryCallable getPoolCallable() {
return getPoolCallable;
}
@Override
public UnaryCallable updatePoolCallable() {
return updatePoolCallable;
}
@Override
public OperationCallable
updatePoolOperationCallable() {
return updatePoolOperationCallable;
}
@Override
public UnaryCallable listLocationsCallable() {
return listLocationsCallable;
}
@Override
public UnaryCallable
listLocationsPagedCallable() {
return listLocationsPagedCallable;
}
@Override
public UnaryCallable getLocationCallable() {
return getLocationCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}