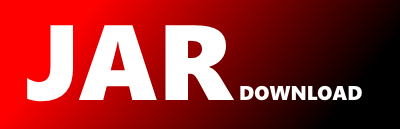
com.google.cloud.video.livestream.v1.stub.LivestreamServiceStub Maven / Gradle / Ivy
Show all versions of google-cloud-live-stream Show documentation
/*
* Copyright 2023 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.video.livestream.v1.stub;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListAssetsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListChannelsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListEventsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListInputsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListLocationsPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.video.livestream.v1.Asset;
import com.google.cloud.video.livestream.v1.Channel;
import com.google.cloud.video.livestream.v1.ChannelOperationResponse;
import com.google.cloud.video.livestream.v1.CreateAssetRequest;
import com.google.cloud.video.livestream.v1.CreateChannelRequest;
import com.google.cloud.video.livestream.v1.CreateEventRequest;
import com.google.cloud.video.livestream.v1.CreateInputRequest;
import com.google.cloud.video.livestream.v1.DeleteAssetRequest;
import com.google.cloud.video.livestream.v1.DeleteChannelRequest;
import com.google.cloud.video.livestream.v1.DeleteEventRequest;
import com.google.cloud.video.livestream.v1.DeleteInputRequest;
import com.google.cloud.video.livestream.v1.Event;
import com.google.cloud.video.livestream.v1.GetAssetRequest;
import com.google.cloud.video.livestream.v1.GetChannelRequest;
import com.google.cloud.video.livestream.v1.GetEventRequest;
import com.google.cloud.video.livestream.v1.GetInputRequest;
import com.google.cloud.video.livestream.v1.GetPoolRequest;
import com.google.cloud.video.livestream.v1.Input;
import com.google.cloud.video.livestream.v1.ListAssetsRequest;
import com.google.cloud.video.livestream.v1.ListAssetsResponse;
import com.google.cloud.video.livestream.v1.ListChannelsRequest;
import com.google.cloud.video.livestream.v1.ListChannelsResponse;
import com.google.cloud.video.livestream.v1.ListEventsRequest;
import com.google.cloud.video.livestream.v1.ListEventsResponse;
import com.google.cloud.video.livestream.v1.ListInputsRequest;
import com.google.cloud.video.livestream.v1.ListInputsResponse;
import com.google.cloud.video.livestream.v1.OperationMetadata;
import com.google.cloud.video.livestream.v1.Pool;
import com.google.cloud.video.livestream.v1.StartChannelRequest;
import com.google.cloud.video.livestream.v1.StopChannelRequest;
import com.google.cloud.video.livestream.v1.UpdateChannelRequest;
import com.google.cloud.video.livestream.v1.UpdateInputRequest;
import com.google.cloud.video.livestream.v1.UpdatePoolRequest;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.OperationsStub;
import com.google.protobuf.Empty;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Base stub class for the LivestreamService service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public abstract class LivestreamServiceStub implements BackgroundResource {
public OperationsStub getOperationsStub() {
return null;
}
public com.google.api.gax.httpjson.longrunning.stub.OperationsStub getHttpJsonOperationsStub() {
return null;
}
public OperationCallable
createChannelOperationCallable() {
throw new UnsupportedOperationException("Not implemented: createChannelOperationCallable()");
}
public UnaryCallable createChannelCallable() {
throw new UnsupportedOperationException("Not implemented: createChannelCallable()");
}
public UnaryCallable listChannelsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listChannelsPagedCallable()");
}
public UnaryCallable listChannelsCallable() {
throw new UnsupportedOperationException("Not implemented: listChannelsCallable()");
}
public UnaryCallable getChannelCallable() {
throw new UnsupportedOperationException("Not implemented: getChannelCallable()");
}
public OperationCallable
deleteChannelOperationCallable() {
throw new UnsupportedOperationException("Not implemented: deleteChannelOperationCallable()");
}
public UnaryCallable deleteChannelCallable() {
throw new UnsupportedOperationException("Not implemented: deleteChannelCallable()");
}
public OperationCallable
updateChannelOperationCallable() {
throw new UnsupportedOperationException("Not implemented: updateChannelOperationCallable()");
}
public UnaryCallable updateChannelCallable() {
throw new UnsupportedOperationException("Not implemented: updateChannelCallable()");
}
public OperationCallable
startChannelOperationCallable() {
throw new UnsupportedOperationException("Not implemented: startChannelOperationCallable()");
}
public UnaryCallable startChannelCallable() {
throw new UnsupportedOperationException("Not implemented: startChannelCallable()");
}
public OperationCallable
stopChannelOperationCallable() {
throw new UnsupportedOperationException("Not implemented: stopChannelOperationCallable()");
}
public UnaryCallable stopChannelCallable() {
throw new UnsupportedOperationException("Not implemented: stopChannelCallable()");
}
public OperationCallable
createInputOperationCallable() {
throw new UnsupportedOperationException("Not implemented: createInputOperationCallable()");
}
public UnaryCallable createInputCallable() {
throw new UnsupportedOperationException("Not implemented: createInputCallable()");
}
public UnaryCallable listInputsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listInputsPagedCallable()");
}
public UnaryCallable listInputsCallable() {
throw new UnsupportedOperationException("Not implemented: listInputsCallable()");
}
public UnaryCallable getInputCallable() {
throw new UnsupportedOperationException("Not implemented: getInputCallable()");
}
public OperationCallable
deleteInputOperationCallable() {
throw new UnsupportedOperationException("Not implemented: deleteInputOperationCallable()");
}
public UnaryCallable deleteInputCallable() {
throw new UnsupportedOperationException("Not implemented: deleteInputCallable()");
}
public OperationCallable
updateInputOperationCallable() {
throw new UnsupportedOperationException("Not implemented: updateInputOperationCallable()");
}
public UnaryCallable updateInputCallable() {
throw new UnsupportedOperationException("Not implemented: updateInputCallable()");
}
public UnaryCallable createEventCallable() {
throw new UnsupportedOperationException("Not implemented: createEventCallable()");
}
public UnaryCallable listEventsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listEventsPagedCallable()");
}
public UnaryCallable listEventsCallable() {
throw new UnsupportedOperationException("Not implemented: listEventsCallable()");
}
public UnaryCallable getEventCallable() {
throw new UnsupportedOperationException("Not implemented: getEventCallable()");
}
public UnaryCallable deleteEventCallable() {
throw new UnsupportedOperationException("Not implemented: deleteEventCallable()");
}
public OperationCallable
createAssetOperationCallable() {
throw new UnsupportedOperationException("Not implemented: createAssetOperationCallable()");
}
public UnaryCallable createAssetCallable() {
throw new UnsupportedOperationException("Not implemented: createAssetCallable()");
}
public OperationCallable
deleteAssetOperationCallable() {
throw new UnsupportedOperationException("Not implemented: deleteAssetOperationCallable()");
}
public UnaryCallable deleteAssetCallable() {
throw new UnsupportedOperationException("Not implemented: deleteAssetCallable()");
}
public UnaryCallable getAssetCallable() {
throw new UnsupportedOperationException("Not implemented: getAssetCallable()");
}
public UnaryCallable listAssetsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listAssetsPagedCallable()");
}
public UnaryCallable listAssetsCallable() {
throw new UnsupportedOperationException("Not implemented: listAssetsCallable()");
}
public UnaryCallable getPoolCallable() {
throw new UnsupportedOperationException("Not implemented: getPoolCallable()");
}
public OperationCallable
updatePoolOperationCallable() {
throw new UnsupportedOperationException("Not implemented: updatePoolOperationCallable()");
}
public UnaryCallable updatePoolCallable() {
throw new UnsupportedOperationException("Not implemented: updatePoolCallable()");
}
public UnaryCallable
listLocationsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listLocationsPagedCallable()");
}
public UnaryCallable listLocationsCallable() {
throw new UnsupportedOperationException("Not implemented: listLocationsCallable()");
}
public UnaryCallable getLocationCallable() {
throw new UnsupportedOperationException("Not implemented: getLocationCallable()");
}
@Override
public abstract void close();
}