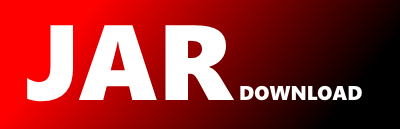
com.google.cloud.video.livestream.v1.stub.LivestreamServiceStubSettings Maven / Gradle / Ivy
Show all versions of google-cloud-live-stream Show documentation
/*
* Copyright 2023 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.video.livestream.v1.stub;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListAssetsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListChannelsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListEventsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListInputsPagedResponse;
import static com.google.cloud.video.livestream.v1.LivestreamServiceClient.ListLocationsPagedResponse;
import com.google.api.core.ApiFunction;
import com.google.api.core.ApiFuture;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.GaxProperties;
import com.google.api.gax.core.GoogleCredentialsProvider;
import com.google.api.gax.core.InstantiatingExecutorProvider;
import com.google.api.gax.grpc.GaxGrpcProperties;
import com.google.api.gax.grpc.GrpcTransportChannel;
import com.google.api.gax.grpc.InstantiatingGrpcChannelProvider;
import com.google.api.gax.grpc.ProtoOperationTransformers;
import com.google.api.gax.httpjson.GaxHttpJsonProperties;
import com.google.api.gax.httpjson.HttpJsonTransportChannel;
import com.google.api.gax.httpjson.InstantiatingHttpJsonChannelProvider;
import com.google.api.gax.longrunning.OperationSnapshot;
import com.google.api.gax.longrunning.OperationTimedPollAlgorithm;
import com.google.api.gax.retrying.RetrySettings;
import com.google.api.gax.rpc.ApiCallContext;
import com.google.api.gax.rpc.ApiClientHeaderProvider;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallSettings;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.PagedCallSettings;
import com.google.api.gax.rpc.PagedListDescriptor;
import com.google.api.gax.rpc.PagedListResponseFactory;
import com.google.api.gax.rpc.StatusCode;
import com.google.api.gax.rpc.StubSettings;
import com.google.api.gax.rpc.TransportChannelProvider;
import com.google.api.gax.rpc.UnaryCallSettings;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.video.livestream.v1.Asset;
import com.google.cloud.video.livestream.v1.Channel;
import com.google.cloud.video.livestream.v1.ChannelOperationResponse;
import com.google.cloud.video.livestream.v1.CreateAssetRequest;
import com.google.cloud.video.livestream.v1.CreateChannelRequest;
import com.google.cloud.video.livestream.v1.CreateEventRequest;
import com.google.cloud.video.livestream.v1.CreateInputRequest;
import com.google.cloud.video.livestream.v1.DeleteAssetRequest;
import com.google.cloud.video.livestream.v1.DeleteChannelRequest;
import com.google.cloud.video.livestream.v1.DeleteEventRequest;
import com.google.cloud.video.livestream.v1.DeleteInputRequest;
import com.google.cloud.video.livestream.v1.Event;
import com.google.cloud.video.livestream.v1.GetAssetRequest;
import com.google.cloud.video.livestream.v1.GetChannelRequest;
import com.google.cloud.video.livestream.v1.GetEventRequest;
import com.google.cloud.video.livestream.v1.GetInputRequest;
import com.google.cloud.video.livestream.v1.GetPoolRequest;
import com.google.cloud.video.livestream.v1.Input;
import com.google.cloud.video.livestream.v1.ListAssetsRequest;
import com.google.cloud.video.livestream.v1.ListAssetsResponse;
import com.google.cloud.video.livestream.v1.ListChannelsRequest;
import com.google.cloud.video.livestream.v1.ListChannelsResponse;
import com.google.cloud.video.livestream.v1.ListEventsRequest;
import com.google.cloud.video.livestream.v1.ListEventsResponse;
import com.google.cloud.video.livestream.v1.ListInputsRequest;
import com.google.cloud.video.livestream.v1.ListInputsResponse;
import com.google.cloud.video.livestream.v1.OperationMetadata;
import com.google.cloud.video.livestream.v1.Pool;
import com.google.cloud.video.livestream.v1.StartChannelRequest;
import com.google.cloud.video.livestream.v1.StopChannelRequest;
import com.google.cloud.video.livestream.v1.UpdateChannelRequest;
import com.google.cloud.video.livestream.v1.UpdateInputRequest;
import com.google.cloud.video.livestream.v1.UpdatePoolRequest;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Lists;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import java.io.IOException;
import java.util.List;
import javax.annotation.Generated;
import org.threeten.bp.Duration;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Settings class to configure an instance of {@link LivestreamServiceStub}.
*
* The default instance has everything set to sensible defaults:
*
*
* - The default service address (livestream.googleapis.com) and default port (443) are used.
*
- Credentials are acquired automatically through Application Default Credentials.
*
- Retries are configured for idempotent methods but not for non-idempotent methods.
*
*
* The builder of this class is recursive, so contained classes are themselves builders. When
* build() is called, the tree of builders is called to create the complete settings object.
*
*
For example, to set the total timeout of getChannel to 30 seconds:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* LivestreamServiceStubSettings.Builder livestreamServiceSettingsBuilder =
* LivestreamServiceStubSettings.newBuilder();
* livestreamServiceSettingsBuilder
* .getChannelSettings()
* .setRetrySettings(
* livestreamServiceSettingsBuilder
* .getChannelSettings()
* .getRetrySettings()
* .toBuilder()
* .setTotalTimeout(Duration.ofSeconds(30))
* .build());
* LivestreamServiceStubSettings livestreamServiceSettings =
* livestreamServiceSettingsBuilder.build();
* }
*/
@Generated("by gapic-generator-java")
public class LivestreamServiceStubSettings extends StubSettings {
/** The default scopes of the service. */
private static final ImmutableList DEFAULT_SERVICE_SCOPES =
ImmutableList.builder().add("https://www.googleapis.com/auth/cloud-platform").build();
private final UnaryCallSettings createChannelSettings;
private final OperationCallSettings
createChannelOperationSettings;
private final PagedCallSettings<
ListChannelsRequest, ListChannelsResponse, ListChannelsPagedResponse>
listChannelsSettings;
private final UnaryCallSettings getChannelSettings;
private final UnaryCallSettings deleteChannelSettings;
private final OperationCallSettings
deleteChannelOperationSettings;
private final UnaryCallSettings updateChannelSettings;
private final OperationCallSettings
updateChannelOperationSettings;
private final UnaryCallSettings startChannelSettings;
private final OperationCallSettings<
StartChannelRequest, ChannelOperationResponse, OperationMetadata>
startChannelOperationSettings;
private final UnaryCallSettings stopChannelSettings;
private final OperationCallSettings<
StopChannelRequest, ChannelOperationResponse, OperationMetadata>
stopChannelOperationSettings;
private final UnaryCallSettings createInputSettings;
private final OperationCallSettings
createInputOperationSettings;
private final PagedCallSettings
listInputsSettings;
private final UnaryCallSettings getInputSettings;
private final UnaryCallSettings deleteInputSettings;
private final OperationCallSettings
deleteInputOperationSettings;
private final UnaryCallSettings updateInputSettings;
private final OperationCallSettings
updateInputOperationSettings;
private final UnaryCallSettings createEventSettings;
private final PagedCallSettings
listEventsSettings;
private final UnaryCallSettings getEventSettings;
private final UnaryCallSettings deleteEventSettings;
private final UnaryCallSettings createAssetSettings;
private final OperationCallSettings
createAssetOperationSettings;
private final UnaryCallSettings deleteAssetSettings;
private final OperationCallSettings
deleteAssetOperationSettings;
private final UnaryCallSettings getAssetSettings;
private final PagedCallSettings
listAssetsSettings;
private final UnaryCallSettings getPoolSettings;
private final UnaryCallSettings updatePoolSettings;
private final OperationCallSettings
updatePoolOperationSettings;
private final PagedCallSettings<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>
listLocationsSettings;
private final UnaryCallSettings getLocationSettings;
private static final PagedListDescriptor
LIST_CHANNELS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListChannelsRequest injectToken(ListChannelsRequest payload, String token) {
return ListChannelsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListChannelsRequest injectPageSize(ListChannelsRequest payload, int pageSize) {
return ListChannelsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListChannelsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListChannelsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListChannelsResponse payload) {
return payload.getChannelsList() == null
? ImmutableList.of()
: payload.getChannelsList();
}
};
private static final PagedListDescriptor
LIST_INPUTS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListInputsRequest injectToken(ListInputsRequest payload, String token) {
return ListInputsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListInputsRequest injectPageSize(ListInputsRequest payload, int pageSize) {
return ListInputsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListInputsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListInputsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListInputsResponse payload) {
return payload.getInputsList() == null
? ImmutableList.of()
: payload.getInputsList();
}
};
private static final PagedListDescriptor
LIST_EVENTS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListEventsRequest injectToken(ListEventsRequest payload, String token) {
return ListEventsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListEventsRequest injectPageSize(ListEventsRequest payload, int pageSize) {
return ListEventsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListEventsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListEventsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListEventsResponse payload) {
return payload.getEventsList() == null
? ImmutableList.of()
: payload.getEventsList();
}
};
private static final PagedListDescriptor
LIST_ASSETS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListAssetsRequest injectToken(ListAssetsRequest payload, String token) {
return ListAssetsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListAssetsRequest injectPageSize(ListAssetsRequest payload, int pageSize) {
return ListAssetsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListAssetsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListAssetsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListAssetsResponse payload) {
return payload.getAssetsList() == null
? ImmutableList.of()
: payload.getAssetsList();
}
};
private static final PagedListDescriptor
LIST_LOCATIONS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListLocationsRequest injectToken(ListLocationsRequest payload, String token) {
return ListLocationsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListLocationsRequest injectPageSize(ListLocationsRequest payload, int pageSize) {
return ListLocationsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListLocationsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListLocationsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListLocationsResponse payload) {
return payload.getLocationsList() == null
? ImmutableList.of()
: payload.getLocationsList();
}
};
private static final PagedListResponseFactory<
ListChannelsRequest, ListChannelsResponse, ListChannelsPagedResponse>
LIST_CHANNELS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListChannelsRequest, ListChannelsResponse, ListChannelsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListChannelsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_CHANNELS_PAGE_STR_DESC, request, context);
return ListChannelsPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListInputsRequest, ListInputsResponse, ListInputsPagedResponse>
LIST_INPUTS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListInputsRequest, ListInputsResponse, ListInputsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListInputsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_INPUTS_PAGE_STR_DESC, request, context);
return ListInputsPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListEventsRequest, ListEventsResponse, ListEventsPagedResponse>
LIST_EVENTS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListEventsRequest, ListEventsResponse, ListEventsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListEventsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_EVENTS_PAGE_STR_DESC, request, context);
return ListEventsPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListAssetsRequest, ListAssetsResponse, ListAssetsPagedResponse>
LIST_ASSETS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListAssetsRequest, ListAssetsResponse, ListAssetsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListAssetsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_ASSETS_PAGE_STR_DESC, request, context);
return ListAssetsPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>
LIST_LOCATIONS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListLocationsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_LOCATIONS_PAGE_STR_DESC, request, context);
return ListLocationsPagedResponse.createAsync(pageContext, futureResponse);
}
};
/** Returns the object with the settings used for calls to createChannel. */
public UnaryCallSettings createChannelSettings() {
return createChannelSettings;
}
/** Returns the object with the settings used for calls to createChannel. */
public OperationCallSettings
createChannelOperationSettings() {
return createChannelOperationSettings;
}
/** Returns the object with the settings used for calls to listChannels. */
public PagedCallSettings
listChannelsSettings() {
return listChannelsSettings;
}
/** Returns the object with the settings used for calls to getChannel. */
public UnaryCallSettings getChannelSettings() {
return getChannelSettings;
}
/** Returns the object with the settings used for calls to deleteChannel. */
public UnaryCallSettings deleteChannelSettings() {
return deleteChannelSettings;
}
/** Returns the object with the settings used for calls to deleteChannel. */
public OperationCallSettings
deleteChannelOperationSettings() {
return deleteChannelOperationSettings;
}
/** Returns the object with the settings used for calls to updateChannel. */
public UnaryCallSettings updateChannelSettings() {
return updateChannelSettings;
}
/** Returns the object with the settings used for calls to updateChannel. */
public OperationCallSettings
updateChannelOperationSettings() {
return updateChannelOperationSettings;
}
/** Returns the object with the settings used for calls to startChannel. */
public UnaryCallSettings startChannelSettings() {
return startChannelSettings;
}
/** Returns the object with the settings used for calls to startChannel. */
public OperationCallSettings
startChannelOperationSettings() {
return startChannelOperationSettings;
}
/** Returns the object with the settings used for calls to stopChannel. */
public UnaryCallSettings stopChannelSettings() {
return stopChannelSettings;
}
/** Returns the object with the settings used for calls to stopChannel. */
public OperationCallSettings
stopChannelOperationSettings() {
return stopChannelOperationSettings;
}
/** Returns the object with the settings used for calls to createInput. */
public UnaryCallSettings createInputSettings() {
return createInputSettings;
}
/** Returns the object with the settings used for calls to createInput. */
public OperationCallSettings
createInputOperationSettings() {
return createInputOperationSettings;
}
/** Returns the object with the settings used for calls to listInputs. */
public PagedCallSettings
listInputsSettings() {
return listInputsSettings;
}
/** Returns the object with the settings used for calls to getInput. */
public UnaryCallSettings getInputSettings() {
return getInputSettings;
}
/** Returns the object with the settings used for calls to deleteInput. */
public UnaryCallSettings deleteInputSettings() {
return deleteInputSettings;
}
/** Returns the object with the settings used for calls to deleteInput. */
public OperationCallSettings
deleteInputOperationSettings() {
return deleteInputOperationSettings;
}
/** Returns the object with the settings used for calls to updateInput. */
public UnaryCallSettings updateInputSettings() {
return updateInputSettings;
}
/** Returns the object with the settings used for calls to updateInput. */
public OperationCallSettings
updateInputOperationSettings() {
return updateInputOperationSettings;
}
/** Returns the object with the settings used for calls to createEvent. */
public UnaryCallSettings createEventSettings() {
return createEventSettings;
}
/** Returns the object with the settings used for calls to listEvents. */
public PagedCallSettings
listEventsSettings() {
return listEventsSettings;
}
/** Returns the object with the settings used for calls to getEvent. */
public UnaryCallSettings getEventSettings() {
return getEventSettings;
}
/** Returns the object with the settings used for calls to deleteEvent. */
public UnaryCallSettings deleteEventSettings() {
return deleteEventSettings;
}
/** Returns the object with the settings used for calls to createAsset. */
public UnaryCallSettings createAssetSettings() {
return createAssetSettings;
}
/** Returns the object with the settings used for calls to createAsset. */
public OperationCallSettings
createAssetOperationSettings() {
return createAssetOperationSettings;
}
/** Returns the object with the settings used for calls to deleteAsset. */
public UnaryCallSettings deleteAssetSettings() {
return deleteAssetSettings;
}
/** Returns the object with the settings used for calls to deleteAsset. */
public OperationCallSettings
deleteAssetOperationSettings() {
return deleteAssetOperationSettings;
}
/** Returns the object with the settings used for calls to getAsset. */
public UnaryCallSettings getAssetSettings() {
return getAssetSettings;
}
/** Returns the object with the settings used for calls to listAssets. */
public PagedCallSettings
listAssetsSettings() {
return listAssetsSettings;
}
/** Returns the object with the settings used for calls to getPool. */
public UnaryCallSettings getPoolSettings() {
return getPoolSettings;
}
/** Returns the object with the settings used for calls to updatePool. */
public UnaryCallSettings updatePoolSettings() {
return updatePoolSettings;
}
/** Returns the object with the settings used for calls to updatePool. */
public OperationCallSettings
updatePoolOperationSettings() {
return updatePoolOperationSettings;
}
/** Returns the object with the settings used for calls to listLocations. */
public PagedCallSettings
listLocationsSettings() {
return listLocationsSettings;
}
/** Returns the object with the settings used for calls to getLocation. */
public UnaryCallSettings getLocationSettings() {
return getLocationSettings;
}
public LivestreamServiceStub createStub() throws IOException {
if (getTransportChannelProvider()
.getTransportName()
.equals(GrpcTransportChannel.getGrpcTransportName())) {
return GrpcLivestreamServiceStub.create(this);
}
if (getTransportChannelProvider()
.getTransportName()
.equals(HttpJsonTransportChannel.getHttpJsonTransportName())) {
return HttpJsonLivestreamServiceStub.create(this);
}
throw new UnsupportedOperationException(
String.format(
"Transport not supported: %s", getTransportChannelProvider().getTransportName()));
}
/** Returns the default service name. */
@Override
public String getServiceName() {
return "livestream";
}
/** Returns a builder for the default ExecutorProvider for this service. */
public static InstantiatingExecutorProvider.Builder defaultExecutorProviderBuilder() {
return InstantiatingExecutorProvider.newBuilder();
}
/** Returns the default service endpoint. */
public static String getDefaultEndpoint() {
return "livestream.googleapis.com:443";
}
/** Returns the default mTLS service endpoint. */
public static String getDefaultMtlsEndpoint() {
return "livestream.mtls.googleapis.com:443";
}
/** Returns the default service scopes. */
public static List getDefaultServiceScopes() {
return DEFAULT_SERVICE_SCOPES;
}
/** Returns a builder for the default credentials for this service. */
public static GoogleCredentialsProvider.Builder defaultCredentialsProviderBuilder() {
return GoogleCredentialsProvider.newBuilder()
.setScopesToApply(DEFAULT_SERVICE_SCOPES)
.setUseJwtAccessWithScope(true);
}
/** Returns a builder for the default gRPC ChannelProvider for this service. */
public static InstantiatingGrpcChannelProvider.Builder defaultGrpcTransportProviderBuilder() {
return InstantiatingGrpcChannelProvider.newBuilder()
.setMaxInboundMessageSize(Integer.MAX_VALUE);
}
/** Returns a builder for the default REST ChannelProvider for this service. */
@BetaApi
public static InstantiatingHttpJsonChannelProvider.Builder
defaultHttpJsonTransportProviderBuilder() {
return InstantiatingHttpJsonChannelProvider.newBuilder();
}
public static TransportChannelProvider defaultTransportChannelProvider() {
return defaultGrpcTransportProviderBuilder().build();
}
@BetaApi("The surface for customizing headers is not stable yet and may change in the future.")
public static ApiClientHeaderProvider.Builder defaultGrpcApiClientHeaderProviderBuilder() {
return ApiClientHeaderProvider.newBuilder()
.setGeneratedLibToken(
"gapic", GaxProperties.getLibraryVersion(LivestreamServiceStubSettings.class))
.setTransportToken(
GaxGrpcProperties.getGrpcTokenName(), GaxGrpcProperties.getGrpcVersion());
}
@BetaApi("The surface for customizing headers is not stable yet and may change in the future.")
public static ApiClientHeaderProvider.Builder defaultHttpJsonApiClientHeaderProviderBuilder() {
return ApiClientHeaderProvider.newBuilder()
.setGeneratedLibToken(
"gapic", GaxProperties.getLibraryVersion(LivestreamServiceStubSettings.class))
.setTransportToken(
GaxHttpJsonProperties.getHttpJsonTokenName(),
GaxHttpJsonProperties.getHttpJsonVersion());
}
public static ApiClientHeaderProvider.Builder defaultApiClientHeaderProviderBuilder() {
return LivestreamServiceStubSettings.defaultGrpcApiClientHeaderProviderBuilder();
}
/** Returns a new gRPC builder for this class. */
public static Builder newBuilder() {
return Builder.createDefault();
}
/** Returns a new REST builder for this class. */
public static Builder newHttpJsonBuilder() {
return Builder.createHttpJsonDefault();
}
/** Returns a new builder for this class. */
public static Builder newBuilder(ClientContext clientContext) {
return new Builder(clientContext);
}
/** Returns a builder containing all the values of this settings class. */
public Builder toBuilder() {
return new Builder(this);
}
protected LivestreamServiceStubSettings(Builder settingsBuilder) throws IOException {
super(settingsBuilder);
createChannelSettings = settingsBuilder.createChannelSettings().build();
createChannelOperationSettings = settingsBuilder.createChannelOperationSettings().build();
listChannelsSettings = settingsBuilder.listChannelsSettings().build();
getChannelSettings = settingsBuilder.getChannelSettings().build();
deleteChannelSettings = settingsBuilder.deleteChannelSettings().build();
deleteChannelOperationSettings = settingsBuilder.deleteChannelOperationSettings().build();
updateChannelSettings = settingsBuilder.updateChannelSettings().build();
updateChannelOperationSettings = settingsBuilder.updateChannelOperationSettings().build();
startChannelSettings = settingsBuilder.startChannelSettings().build();
startChannelOperationSettings = settingsBuilder.startChannelOperationSettings().build();
stopChannelSettings = settingsBuilder.stopChannelSettings().build();
stopChannelOperationSettings = settingsBuilder.stopChannelOperationSettings().build();
createInputSettings = settingsBuilder.createInputSettings().build();
createInputOperationSettings = settingsBuilder.createInputOperationSettings().build();
listInputsSettings = settingsBuilder.listInputsSettings().build();
getInputSettings = settingsBuilder.getInputSettings().build();
deleteInputSettings = settingsBuilder.deleteInputSettings().build();
deleteInputOperationSettings = settingsBuilder.deleteInputOperationSettings().build();
updateInputSettings = settingsBuilder.updateInputSettings().build();
updateInputOperationSettings = settingsBuilder.updateInputOperationSettings().build();
createEventSettings = settingsBuilder.createEventSettings().build();
listEventsSettings = settingsBuilder.listEventsSettings().build();
getEventSettings = settingsBuilder.getEventSettings().build();
deleteEventSettings = settingsBuilder.deleteEventSettings().build();
createAssetSettings = settingsBuilder.createAssetSettings().build();
createAssetOperationSettings = settingsBuilder.createAssetOperationSettings().build();
deleteAssetSettings = settingsBuilder.deleteAssetSettings().build();
deleteAssetOperationSettings = settingsBuilder.deleteAssetOperationSettings().build();
getAssetSettings = settingsBuilder.getAssetSettings().build();
listAssetsSettings = settingsBuilder.listAssetsSettings().build();
getPoolSettings = settingsBuilder.getPoolSettings().build();
updatePoolSettings = settingsBuilder.updatePoolSettings().build();
updatePoolOperationSettings = settingsBuilder.updatePoolOperationSettings().build();
listLocationsSettings = settingsBuilder.listLocationsSettings().build();
getLocationSettings = settingsBuilder.getLocationSettings().build();
}
/** Builder for LivestreamServiceStubSettings. */
public static class Builder extends StubSettings.Builder {
private final ImmutableList> unaryMethodSettingsBuilders;
private final UnaryCallSettings.Builder createChannelSettings;
private final OperationCallSettings.Builder
createChannelOperationSettings;
private final PagedCallSettings.Builder<
ListChannelsRequest, ListChannelsResponse, ListChannelsPagedResponse>
listChannelsSettings;
private final UnaryCallSettings.Builder getChannelSettings;
private final UnaryCallSettings.Builder deleteChannelSettings;
private final OperationCallSettings.Builder
deleteChannelOperationSettings;
private final UnaryCallSettings.Builder updateChannelSettings;
private final OperationCallSettings.Builder
updateChannelOperationSettings;
private final UnaryCallSettings.Builder startChannelSettings;
private final OperationCallSettings.Builder<
StartChannelRequest, ChannelOperationResponse, OperationMetadata>
startChannelOperationSettings;
private final UnaryCallSettings.Builder stopChannelSettings;
private final OperationCallSettings.Builder<
StopChannelRequest, ChannelOperationResponse, OperationMetadata>
stopChannelOperationSettings;
private final UnaryCallSettings.Builder createInputSettings;
private final OperationCallSettings.Builder
createInputOperationSettings;
private final PagedCallSettings.Builder<
ListInputsRequest, ListInputsResponse, ListInputsPagedResponse>
listInputsSettings;
private final UnaryCallSettings.Builder getInputSettings;
private final UnaryCallSettings.Builder deleteInputSettings;
private final OperationCallSettings.Builder
deleteInputOperationSettings;
private final UnaryCallSettings.Builder updateInputSettings;
private final OperationCallSettings.Builder
updateInputOperationSettings;
private final UnaryCallSettings.Builder createEventSettings;
private final PagedCallSettings.Builder<
ListEventsRequest, ListEventsResponse, ListEventsPagedResponse>
listEventsSettings;
private final UnaryCallSettings.Builder getEventSettings;
private final UnaryCallSettings.Builder deleteEventSettings;
private final UnaryCallSettings.Builder createAssetSettings;
private final OperationCallSettings.Builder
createAssetOperationSettings;
private final UnaryCallSettings.Builder deleteAssetSettings;
private final OperationCallSettings.Builder
deleteAssetOperationSettings;
private final UnaryCallSettings.Builder getAssetSettings;
private final PagedCallSettings.Builder<
ListAssetsRequest, ListAssetsResponse, ListAssetsPagedResponse>
listAssetsSettings;
private final UnaryCallSettings.Builder getPoolSettings;
private final UnaryCallSettings.Builder updatePoolSettings;
private final OperationCallSettings.Builder
updatePoolOperationSettings;
private final PagedCallSettings.Builder<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>
listLocationsSettings;
private final UnaryCallSettings.Builder getLocationSettings;
private static final ImmutableMap>
RETRYABLE_CODE_DEFINITIONS;
static {
ImmutableMap.Builder> definitions =
ImmutableMap.builder();
definitions.put(
"no_retry_1_codes", ImmutableSet.copyOf(Lists.newArrayList()));
definitions.put(
"retry_policy_0_codes",
ImmutableSet.copyOf(Lists.newArrayList(StatusCode.Code.UNAVAILABLE)));
definitions.put("no_retry_codes", ImmutableSet.copyOf(Lists.newArrayList()));
RETRYABLE_CODE_DEFINITIONS = definitions.build();
}
private static final ImmutableMap RETRY_PARAM_DEFINITIONS;
static {
ImmutableMap.Builder definitions = ImmutableMap.builder();
RetrySettings settings = null;
settings =
RetrySettings.newBuilder()
.setInitialRpcTimeout(Duration.ofMillis(60000L))
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ofMillis(60000L))
.setTotalTimeout(Duration.ofMillis(60000L))
.build();
definitions.put("no_retry_1_params", settings);
settings =
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(1000L))
.setRetryDelayMultiplier(1.3)
.setMaxRetryDelay(Duration.ofMillis(10000L))
.setInitialRpcTimeout(Duration.ofMillis(60000L))
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ofMillis(60000L))
.setTotalTimeout(Duration.ofMillis(60000L))
.build();
definitions.put("retry_policy_0_params", settings);
settings = RetrySettings.newBuilder().setRpcTimeoutMultiplier(1.0).build();
definitions.put("no_retry_params", settings);
RETRY_PARAM_DEFINITIONS = definitions.build();
}
protected Builder() {
this(((ClientContext) null));
}
protected Builder(ClientContext clientContext) {
super(clientContext);
createChannelSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createChannelOperationSettings = OperationCallSettings.newBuilder();
listChannelsSettings = PagedCallSettings.newBuilder(LIST_CHANNELS_PAGE_STR_FACT);
getChannelSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteChannelSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteChannelOperationSettings = OperationCallSettings.newBuilder();
updateChannelSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateChannelOperationSettings = OperationCallSettings.newBuilder();
startChannelSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
startChannelOperationSettings = OperationCallSettings.newBuilder();
stopChannelSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
stopChannelOperationSettings = OperationCallSettings.newBuilder();
createInputSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createInputOperationSettings = OperationCallSettings.newBuilder();
listInputsSettings = PagedCallSettings.newBuilder(LIST_INPUTS_PAGE_STR_FACT);
getInputSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteInputSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteInputOperationSettings = OperationCallSettings.newBuilder();
updateInputSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateInputOperationSettings = OperationCallSettings.newBuilder();
createEventSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
listEventsSettings = PagedCallSettings.newBuilder(LIST_EVENTS_PAGE_STR_FACT);
getEventSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteEventSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createAssetSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createAssetOperationSettings = OperationCallSettings.newBuilder();
deleteAssetSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteAssetOperationSettings = OperationCallSettings.newBuilder();
getAssetSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
listAssetsSettings = PagedCallSettings.newBuilder(LIST_ASSETS_PAGE_STR_FACT);
getPoolSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updatePoolSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updatePoolOperationSettings = OperationCallSettings.newBuilder();
listLocationsSettings = PagedCallSettings.newBuilder(LIST_LOCATIONS_PAGE_STR_FACT);
getLocationSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
unaryMethodSettingsBuilders =
ImmutableList.>of(
createChannelSettings,
listChannelsSettings,
getChannelSettings,
deleteChannelSettings,
updateChannelSettings,
startChannelSettings,
stopChannelSettings,
createInputSettings,
listInputsSettings,
getInputSettings,
deleteInputSettings,
updateInputSettings,
createEventSettings,
listEventsSettings,
getEventSettings,
deleteEventSettings,
createAssetSettings,
deleteAssetSettings,
getAssetSettings,
listAssetsSettings,
getPoolSettings,
updatePoolSettings,
listLocationsSettings,
getLocationSettings);
initDefaults(this);
}
protected Builder(LivestreamServiceStubSettings settings) {
super(settings);
createChannelSettings = settings.createChannelSettings.toBuilder();
createChannelOperationSettings = settings.createChannelOperationSettings.toBuilder();
listChannelsSettings = settings.listChannelsSettings.toBuilder();
getChannelSettings = settings.getChannelSettings.toBuilder();
deleteChannelSettings = settings.deleteChannelSettings.toBuilder();
deleteChannelOperationSettings = settings.deleteChannelOperationSettings.toBuilder();
updateChannelSettings = settings.updateChannelSettings.toBuilder();
updateChannelOperationSettings = settings.updateChannelOperationSettings.toBuilder();
startChannelSettings = settings.startChannelSettings.toBuilder();
startChannelOperationSettings = settings.startChannelOperationSettings.toBuilder();
stopChannelSettings = settings.stopChannelSettings.toBuilder();
stopChannelOperationSettings = settings.stopChannelOperationSettings.toBuilder();
createInputSettings = settings.createInputSettings.toBuilder();
createInputOperationSettings = settings.createInputOperationSettings.toBuilder();
listInputsSettings = settings.listInputsSettings.toBuilder();
getInputSettings = settings.getInputSettings.toBuilder();
deleteInputSettings = settings.deleteInputSettings.toBuilder();
deleteInputOperationSettings = settings.deleteInputOperationSettings.toBuilder();
updateInputSettings = settings.updateInputSettings.toBuilder();
updateInputOperationSettings = settings.updateInputOperationSettings.toBuilder();
createEventSettings = settings.createEventSettings.toBuilder();
listEventsSettings = settings.listEventsSettings.toBuilder();
getEventSettings = settings.getEventSettings.toBuilder();
deleteEventSettings = settings.deleteEventSettings.toBuilder();
createAssetSettings = settings.createAssetSettings.toBuilder();
createAssetOperationSettings = settings.createAssetOperationSettings.toBuilder();
deleteAssetSettings = settings.deleteAssetSettings.toBuilder();
deleteAssetOperationSettings = settings.deleteAssetOperationSettings.toBuilder();
getAssetSettings = settings.getAssetSettings.toBuilder();
listAssetsSettings = settings.listAssetsSettings.toBuilder();
getPoolSettings = settings.getPoolSettings.toBuilder();
updatePoolSettings = settings.updatePoolSettings.toBuilder();
updatePoolOperationSettings = settings.updatePoolOperationSettings.toBuilder();
listLocationsSettings = settings.listLocationsSettings.toBuilder();
getLocationSettings = settings.getLocationSettings.toBuilder();
unaryMethodSettingsBuilders =
ImmutableList.>of(
createChannelSettings,
listChannelsSettings,
getChannelSettings,
deleteChannelSettings,
updateChannelSettings,
startChannelSettings,
stopChannelSettings,
createInputSettings,
listInputsSettings,
getInputSettings,
deleteInputSettings,
updateInputSettings,
createEventSettings,
listEventsSettings,
getEventSettings,
deleteEventSettings,
createAssetSettings,
deleteAssetSettings,
getAssetSettings,
listAssetsSettings,
getPoolSettings,
updatePoolSettings,
listLocationsSettings,
getLocationSettings);
}
private static Builder createDefault() {
Builder builder = new Builder(((ClientContext) null));
builder.setTransportChannelProvider(defaultTransportChannelProvider());
builder.setCredentialsProvider(defaultCredentialsProviderBuilder().build());
builder.setInternalHeaderProvider(defaultApiClientHeaderProviderBuilder().build());
builder.setEndpoint(getDefaultEndpoint());
builder.setMtlsEndpoint(getDefaultMtlsEndpoint());
builder.setSwitchToMtlsEndpointAllowed(true);
return initDefaults(builder);
}
private static Builder createHttpJsonDefault() {
Builder builder = new Builder(((ClientContext) null));
builder.setTransportChannelProvider(defaultHttpJsonTransportProviderBuilder().build());
builder.setCredentialsProvider(defaultCredentialsProviderBuilder().build());
builder.setInternalHeaderProvider(defaultHttpJsonApiClientHeaderProviderBuilder().build());
builder.setEndpoint(getDefaultEndpoint());
builder.setMtlsEndpoint(getDefaultMtlsEndpoint());
builder.setSwitchToMtlsEndpointAllowed(true);
return initDefaults(builder);
}
private static Builder initDefaults(Builder builder) {
builder
.createChannelSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.listChannelsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.getChannelSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.deleteChannelSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.updateChannelSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.startChannelSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.stopChannelSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.createInputSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.listInputsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.getInputSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.deleteInputSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.updateInputSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.createEventSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.listEventsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.getEventSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.deleteEventSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.createAssetSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.deleteAssetSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.getAssetSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.listAssetsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.getPoolSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.updatePoolSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.listLocationsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.getLocationSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.createChannelOperationSettings()
.setInitialCallSettings(
UnaryCallSettings
.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Channel.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.deleteChannelOperationSettings()
.setInitialCallSettings(
UnaryCallSettings
.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Empty.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.updateChannelOperationSettings()
.setInitialCallSettings(
UnaryCallSettings
.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Channel.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.startChannelOperationSettings()
.setInitialCallSettings(
UnaryCallSettings
.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(ChannelOperationResponse.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.stopChannelOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(ChannelOperationResponse.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.createInputOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Input.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.deleteInputOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Empty.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.updateInputOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Input.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.createAssetOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Asset.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.deleteAssetOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Empty.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.updatePoolOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"))
.build())
.setResponseTransformer(ProtoOperationTransformers.ResponseTransformer.create(Pool.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
return builder;
}
/**
* Applies the given settings updater function to all of the unary API methods in this service.
*
* Note: This method does not support applying settings to streaming methods.
*/
public Builder applyToAllUnaryMethods(
ApiFunction, Void> settingsUpdater) {
super.applyToAllUnaryMethods(unaryMethodSettingsBuilders, settingsUpdater);
return this;
}
public ImmutableList> unaryMethodSettingsBuilders() {
return unaryMethodSettingsBuilders;
}
/** Returns the builder for the settings used for calls to createChannel. */
public UnaryCallSettings.Builder createChannelSettings() {
return createChannelSettings;
}
/** Returns the builder for the settings used for calls to createChannel. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder
createChannelOperationSettings() {
return createChannelOperationSettings;
}
/** Returns the builder for the settings used for calls to listChannels. */
public PagedCallSettings.Builder<
ListChannelsRequest, ListChannelsResponse, ListChannelsPagedResponse>
listChannelsSettings() {
return listChannelsSettings;
}
/** Returns the builder for the settings used for calls to getChannel. */
public UnaryCallSettings.Builder getChannelSettings() {
return getChannelSettings;
}
/** Returns the builder for the settings used for calls to deleteChannel. */
public UnaryCallSettings.Builder deleteChannelSettings() {
return deleteChannelSettings;
}
/** Returns the builder for the settings used for calls to deleteChannel. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder
deleteChannelOperationSettings() {
return deleteChannelOperationSettings;
}
/** Returns the builder for the settings used for calls to updateChannel. */
public UnaryCallSettings.Builder updateChannelSettings() {
return updateChannelSettings;
}
/** Returns the builder for the settings used for calls to updateChannel. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder
updateChannelOperationSettings() {
return updateChannelOperationSettings;
}
/** Returns the builder for the settings used for calls to startChannel. */
public UnaryCallSettings.Builder startChannelSettings() {
return startChannelSettings;
}
/** Returns the builder for the settings used for calls to startChannel. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder<
StartChannelRequest, ChannelOperationResponse, OperationMetadata>
startChannelOperationSettings() {
return startChannelOperationSettings;
}
/** Returns the builder for the settings used for calls to stopChannel. */
public UnaryCallSettings.Builder stopChannelSettings() {
return stopChannelSettings;
}
/** Returns the builder for the settings used for calls to stopChannel. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder<
StopChannelRequest, ChannelOperationResponse, OperationMetadata>
stopChannelOperationSettings() {
return stopChannelOperationSettings;
}
/** Returns the builder for the settings used for calls to createInput. */
public UnaryCallSettings.Builder createInputSettings() {
return createInputSettings;
}
/** Returns the builder for the settings used for calls to createInput. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder
createInputOperationSettings() {
return createInputOperationSettings;
}
/** Returns the builder for the settings used for calls to listInputs. */
public PagedCallSettings.Builder
listInputsSettings() {
return listInputsSettings;
}
/** Returns the builder for the settings used for calls to getInput. */
public UnaryCallSettings.Builder getInputSettings() {
return getInputSettings;
}
/** Returns the builder for the settings used for calls to deleteInput. */
public UnaryCallSettings.Builder deleteInputSettings() {
return deleteInputSettings;
}
/** Returns the builder for the settings used for calls to deleteInput. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder
deleteInputOperationSettings() {
return deleteInputOperationSettings;
}
/** Returns the builder for the settings used for calls to updateInput. */
public UnaryCallSettings.Builder updateInputSettings() {
return updateInputSettings;
}
/** Returns the builder for the settings used for calls to updateInput. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder
updateInputOperationSettings() {
return updateInputOperationSettings;
}
/** Returns the builder for the settings used for calls to createEvent. */
public UnaryCallSettings.Builder createEventSettings() {
return createEventSettings;
}
/** Returns the builder for the settings used for calls to listEvents. */
public PagedCallSettings.Builder
listEventsSettings() {
return listEventsSettings;
}
/** Returns the builder for the settings used for calls to getEvent. */
public UnaryCallSettings.Builder getEventSettings() {
return getEventSettings;
}
/** Returns the builder for the settings used for calls to deleteEvent. */
public UnaryCallSettings.Builder deleteEventSettings() {
return deleteEventSettings;
}
/** Returns the builder for the settings used for calls to createAsset. */
public UnaryCallSettings.Builder createAssetSettings() {
return createAssetSettings;
}
/** Returns the builder for the settings used for calls to createAsset. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder
createAssetOperationSettings() {
return createAssetOperationSettings;
}
/** Returns the builder for the settings used for calls to deleteAsset. */
public UnaryCallSettings.Builder deleteAssetSettings() {
return deleteAssetSettings;
}
/** Returns the builder for the settings used for calls to deleteAsset. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder
deleteAssetOperationSettings() {
return deleteAssetOperationSettings;
}
/** Returns the builder for the settings used for calls to getAsset. */
public UnaryCallSettings.Builder getAssetSettings() {
return getAssetSettings;
}
/** Returns the builder for the settings used for calls to listAssets. */
public PagedCallSettings.Builder
listAssetsSettings() {
return listAssetsSettings;
}
/** Returns the builder for the settings used for calls to getPool. */
public UnaryCallSettings.Builder getPoolSettings() {
return getPoolSettings;
}
/** Returns the builder for the settings used for calls to updatePool. */
public UnaryCallSettings.Builder updatePoolSettings() {
return updatePoolSettings;
}
/** Returns the builder for the settings used for calls to updatePool. */
@BetaApi(
"The surface for use by generated code is not stable yet and may change in the future.")
public OperationCallSettings.Builder
updatePoolOperationSettings() {
return updatePoolOperationSettings;
}
/** Returns the builder for the settings used for calls to listLocations. */
public PagedCallSettings.Builder<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>
listLocationsSettings() {
return listLocationsSettings;
}
/** Returns the builder for the settings used for calls to getLocation. */
public UnaryCallSettings.Builder getLocationSettings() {
return getLocationSettings;
}
@Override
public LivestreamServiceStubSettings build() throws IOException {
return new LivestreamServiceStubSettings(this);
}
}
}