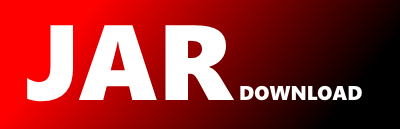
com.google.cloud.logging.Logging Maven / Gradle / Ivy
Show all versions of google-cloud-logging Show documentation
/*
* Copyright 2016 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.logging;
import com.google.api.core.ApiFuture;
import com.google.api.core.BetaApi;
import com.google.api.gax.paging.AsyncPage;
import com.google.api.gax.paging.Page;
import com.google.cloud.MonitoredResource;
import com.google.cloud.MonitoredResourceDescriptor;
import com.google.cloud.Service;
import com.google.common.base.Ascii;
import com.google.common.collect.ImmutableMap;
import java.util.Map;
public interface Logging extends AutoCloseable, Service {
/**
* Class for specifying options for listing sinks, monitored resources and monitored resource
* descriptors.
*/
final class ListOption extends Option {
private static final long serialVersionUID = -6857294816115909271L;
enum OptionType implements Option.OptionType {
PAGE_SIZE,
PAGE_TOKEN;
@SuppressWarnings("unchecked")
T get(Map options) {
return (T) options.get(this);
}
}
private ListOption(ListOption.OptionType option, Object value) {
super(option, value);
}
/** Returns an option to specify the maximum number of resources returned per page. */
public static ListOption pageSize(int pageSize) {
return new ListOption(ListOption.OptionType.PAGE_SIZE, pageSize);
}
/** Returns an option to specify the page token from which to start listing resources. */
public static ListOption pageToken(String pageToken) {
return new ListOption(ListOption.OptionType.PAGE_TOKEN, pageToken);
}
}
/** Class for specifying options for writing log entries. */
final class WriteOption extends Option {
private static final long serialVersionUID = 715900132268584612L;
enum OptionType implements Option.OptionType {
LOG_NAME,
RESOURCE,
LABELS,
LOG_DESTINATION,
AUTO_POPULATE_METADATA,
PARTIAL_SUCCESS;
@SuppressWarnings("unchecked")
T get(Map options) {
return (T) options.get(this);
}
}
private WriteOption(WriteOption.OptionType option, Object value) {
super(option, value);
}
/**
* Returns an option to specify a default log name (see {@link LogEntry#getLogName()}) for those
* log entries that do not specify their own log name. Example: {@code syslog}.
*/
public static WriteOption logName(String logName) {
return new WriteOption(WriteOption.OptionType.LOG_NAME, logName);
}
/**
* Returns an option to specify a default monitored resource (see {@link
* LogEntry#getResource()}) for those log entries that do not specify their own resource.
*/
public static WriteOption resource(MonitoredResource resource) {
return new WriteOption(WriteOption.OptionType.RESOURCE, resource);
}
/**
* Sets an option to specify (key, value) pairs that are added to the {@link
* LogEntry#getLabels()} of each log entry written, except when a log entry already has a value
* associated to the same key.
*/
public static WriteOption labels(Map labels) {
return new WriteOption(WriteOption.OptionType.LABELS, ImmutableMap.copyOf(labels));
}
/**
* Returns an option to specify a log destination resource path (see {@link LogDestinationName}
* for details)
*/
public static WriteOption destination(LogDestinationName destination) {
return new WriteOption(WriteOption.OptionType.LOG_DESTINATION, destination);
}
/**
* Returns an option to opt-out automatic population of log entries metadata fields that are not
* set.
*/
public static WriteOption autoPopulateMetadata(boolean autoPopulateMetadata) {
return new WriteOption(WriteOption.OptionType.AUTO_POPULATE_METADATA, autoPopulateMetadata);
}
/**
* Returns an option to set partialSuccess flag. See the
* API documentation for more details.
*/
public static WriteOption partialSuccess(boolean partialSuccess) {
return new WriteOption(WriteOption.OptionType.PARTIAL_SUCCESS, partialSuccess);
}
}
/** Fields according to which log entries can be sorted. */
enum SortingField {
TIMESTAMP;
String selector() {
return Ascii.toLowerCase(name());
}
}
/** Sorting orders available when listing log entries. */
enum SortingOrder {
DESCENDING("desc"),
ASCENDING("asc");
private final String selector;
SortingOrder(String selector) {
this.selector = selector;
}
String selector() {
return selector;
}
}
/** Class for specifying options for listing log entries. */
final class EntryListOption extends Option {
private static final long serialVersionUID = -1561159676386917050L;
enum OptionType implements Option.OptionType {
ORDER_BY,
FILTER,
ORGANIZATION,
BILLINGACCOUNT,
FOLDER;
@SuppressWarnings("unchecked")
T get(Map options) {
return (T) options.get(this);
}
}
private EntryListOption(Option.OptionType option, Object value) {
super(option, value);
}
/** Returns an option to specify the maximum number of log entries returned per page. */
public static EntryListOption pageSize(int pageSize) {
return new EntryListOption(ListOption.OptionType.PAGE_SIZE, pageSize);
}
/** Returns an option to specify the page token from which to start listing log entries. */
public static EntryListOption pageToken(String pageToken) {
return new EntryListOption(ListOption.OptionType.PAGE_TOKEN, pageToken);
}
/**
* Returns an option to sort log entries. If not specified, log entries are sorted in ascending
* (most-recent last) order with respect to the {@link LogEntry#getTimestamp()} value.
*/
public static EntryListOption sortOrder(SortingField field, SortingOrder order) {
return new EntryListOption(
EntryListOption.OptionType.ORDER_BY, field.selector() + ' ' + order.selector());
}
/**
* Returns an option to specify a filter to the log entries to be listed.
*
* @see Advanced Logs
* Filters
*/
public static EntryListOption filter(String filter) {
return new EntryListOption(EntryListOption.OptionType.FILTER, filter);
}
/** Returns an option to specify an organization for the log entries to be listed. */
public static EntryListOption organization(String organization) {
return new EntryListOption(EntryListOption.OptionType.ORGANIZATION, organization);
}
/** Returns an option to specify a billingAccount for the log entries to be listed. */
public static EntryListOption billingAccount(String billingAccount) {
return new EntryListOption(EntryListOption.OptionType.BILLINGACCOUNT, billingAccount);
}
/** Returns an option to specify a folder for the log entries to be listed. */
public static EntryListOption folder(String folder) {
return new EntryListOption(EntryListOption.OptionType.FOLDER, folder);
}
}
/** Class for specifying options for tailing log entries. */
final class TailOption extends Option {
private static final long serialVersionUID = -772271612198662617L;
enum OptionType implements Option.OptionType {
FILTER,
BUFFERWINDOW,
PROJECT,
ORGANIZATION,
BILLINGACCOUNT,
FOLDER;
@SuppressWarnings("unchecked")
T get(Map options) {
return (T) options.get(this);
}
}
private TailOption(Option.OptionType option, Object value) {
super(option, value);
}
/**
* Returns an option to specify a filter to the log entries to be tailed.
*
* @see Advanced Logs
* Filters
*/
public static TailOption filter(String filter) {
return new TailOption(TailOption.OptionType.FILTER, filter);
}
/**
* Returns an option to specify the amount of time to buffer log entries at the server before
* being returned to prevent out of order results due to late arriving log entries. Valid values
* are between 0-60000 ms. Default is 2000 ms.
*
* @see Duration
* format
*/
public static TailOption bufferWindow(String duration) {
return new TailOption(TailOption.OptionType.BUFFERWINDOW, duration);
}
/** Returns an option to specify an organization for the log entries to be tailed. */
public static TailOption organization(String organization) {
return new TailOption(TailOption.OptionType.ORGANIZATION, organization);
}
/** Returns an option to specify a billingAccount for the log entries to be tailed. */
public static TailOption billingAccount(String billingAccount) {
return new TailOption(TailOption.OptionType.BILLINGACCOUNT, billingAccount);
}
/** Returns an option to specify a folder for the log entries to be tailed. */
public static TailOption folder(String folder) {
return new TailOption(TailOption.OptionType.FOLDER, folder);
}
/** Returns an option to specify a project for the log entries to be tailed. */
public static TailOption project(String project) {
return new TailOption(TailOption.OptionType.PROJECT, project);
}
}
/** Sets synchronicity {@link Synchronicity} of logging writes, defaults to asynchronous. */
void setWriteSynchronicity(Synchronicity synchronicity);
/** Retrieves current set synchronicity {@link Synchronicity} of logging writes. */
Synchronicity getWriteSynchronicity();
/**
* Sets flush severity for asynchronous logging writes. It is disabled by default, enabled when
* this method is called with any {@link Severity} value other than {@link Severity#NONE}. Logs
* will be immediately written out for entries at or higher than flush severity.
*
* Enabling this can cause the leaking and hanging threads, see BUG(2796) BUG(3880). However
* you can explicitly call {@link #flush}.
*
*
TODO: Enable this by default once functionality to trigger rpc is available in generated
* code.
*/
void setFlushSeverity(Severity flushSeverity);
/* Retrieves flush severity for asynchronous logging writes. */
Severity getFlushSeverity();
/**
* Creates a new sink.
*
*
Example of creating a sink to export logs to a BigQuery dataset (in the {@link
* LoggingOptions#getProjectId()} project).
*
*
* {
* @code
* String sinkName = "my_sink_name";
* String datasetName = "my_dataset";
* SinkInfo sinkInfo = SinkInfo.of(sinkName, DatasetDestination.of(datasetName));
* Sink sink = logging.create(sinkInfo);
* }
*
*
* @return the created sink
* @throws LoggingException upon failure
*/
Sink create(SinkInfo sink);
/**
* Creates a new metric.
*
* Example of creating a metric for logs with severity higher or equal to ERROR.
*
*
* {
* @code
* String metricName = "my_metric_name";
* MetricInfo metricInfo = MetricInfo.of(metricName, "severity>=ERROR");
* Metric metric = logging.create(metricInfo);
* }
*
*
* @return the created metric
* @throws LoggingException upon failure
*/
Metric create(MetricInfo metric);
/**
* Creates a new exclusion in a specified parent resource. Only log entries belonging to that
* resource can be excluded. You can have up to 10 exclusions in a resource.
*
* Example of creating the exclusion:
*
*
* {
* @code
* String exclusionName = "my_exclusion_name";
* Exclusion exclusion = Exclusion.of(exclusionName,
* "resource.type=gcs_bucket severity<ERROR sample(insertId, 0.99)");
* Exclusion exclusion = logging.create(exclusion);
* }
*
*
* @return the created exclusion
* @throws LoggingException upon failure
*/
Exclusion create(Exclusion exclusion);
/**
* Sends a request for creating a sink. This method returns a {@code ApiFuture} object to consume
* the result. {@link ApiFuture#get()} returns the created sink.
*
* Example of asynchronously creating a sink to export logs to a BigQuery dataset (in the
* {@link LoggingOptions#getProjectId()} project).
*
*
{@code
* String sinkName = "my_sink_name";
* String datasetName = "my_dataset";
* SinkInfo sinkInfo = SinkInfo.of(sinkName, DatasetDestination.of(datasetName));
* ApiFuture future = logging.createAsync(sinkInfo);
* // ...
* Sink sink = future.get();
* }
*/
ApiFuture createAsync(SinkInfo sink);
/**
* Sends a request for creating a metric. This method returns a {@code ApiFuture} object to
* consume the result. {@link ApiFuture#get()} returns the created metric.
*
* Example of asynchronously creating a metric for logs with severity higher or equal to ERROR.
*
*
{@code
* String metricName = "my_metric_name";
* MetricInfo metricInfo = MetricInfo.of(metricName, "severity>=ERROR");
* ApiFuture future = logging.createAsync(metricInfo);
* // ...
* Metric metric = future.get();
* }
*/
ApiFuture createAsync(MetricInfo metric);
/**
* Sends a request to create the exclusion. This method returns an {@code ApiFuture} object to
* consume the result. {@link ApiFuture#get()} returns the created exclusion.
*
* Example of asynchronously creating the exclusion:
*
*
{@code
* String exclusionName = "my_exclusion_name";
* Exclusion exclusion = Exclusion.of(exclusionName,
* "resource.type=gcs_bucket severity future = logging.createAsync(exclusion);
* // ...
* Exclusion exclusion = future.get();
* }
*/
ApiFuture createAsync(Exclusion exclusion);
/**
* Updates a sink or creates one if it does not exist.
*
* Example of updating a sink.
*
*
{@code
* String sinkName = "my_sink_name";
* String datasetName = "my_dataset";
* SinkInfo sinkInfo = SinkInfo.newBuilder(sinkName, DatasetDestination.of(datasetName))
* .setVersionFormat(SinkInfo.VersionFormat.V2).setFilter("severity>=ERROR").build();
* Sink sink = logging.update(sinkInfo);
* }
*
* @return the created sink
* @throws LoggingException upon failure
*/
Sink update(SinkInfo sink);
/**
* Updates a metric or creates one if it does not exist.
*
* Example of updating a metric.
*
*
{@code
* String metricName = "my_metric_name";
* MetricInfo metricInfo = MetricInfo.newBuilder(metricName, "severity>=ERROR").setDescription("new description")
* .build();
* Metric metric = logging.update(metricInfo);
* }
*
* @return the created metric
* @throws LoggingException upon failure
*/
Metric update(MetricInfo metric);
/**
* Updates one or more properties of an existing exclusion.
*
* Example of updating the exclusion:
*
*
{@code
* String exclusionName = "my_exclusion_name";
* Exclusion exclusion = Exclusion
* .newBuilder(exclusionName, "resource.type=gcs_bucket severity
*
* @return the updated exclusion
* @throws LoggingException upon failure
*/
Exclusion update(Exclusion exclusion);
/**
* Sends a request for updating a sink (or creating it, if it does not exist). This method returns
* a {@code ApiFuture} object to consume the result. {@link ApiFuture#get()} returns the
* updated/created sink or {@code null} if not found.
*
* Example of asynchronously updating a sink.
*
*
{@code
* String sinkName = "my_sink_name";
* String datasetName = "my_dataset";
* SinkInfo sinkInfo = SinkInfo.newBuilder(sinkName, DatasetDestination.of(datasetName))
* .setVersionFormat(SinkInfo.VersionFormat.V2).setFilter("severity>=ERROR").build();
* ApiFuture future = logging.updateAsync(sinkInfo);
* // ...
* Sink sink = future.get();
* }
*/
ApiFuture updateAsync(SinkInfo sink);
/**
* Sends a request for updating a metric (or creating it, if it does not exist). This method
* returns a {@code ApiFuture} object to consume the result. {@link ApiFuture#get()} returns the
* updated/created metric or {@code null} if not found.
*
* Example of asynchronously updating a metric.
*
*
{@code
* String metricName = "my_metric_name";
* MetricInfo metricInfo = MetricInfo.newBuilder(metricName, "severity>=ERROR").setDescription("new description")
* .build();
* ApiFuture future = logging.updateAsync(metricInfo);
* // ...
* Metric metric = future.get();
* }
*/
ApiFuture updateAsync(MetricInfo metric);
/**
* Sends a request to change one or more properties of an existing exclusion. This method returns
* an {@code ApiFuture} object to consume the result. {@link ApiFuture#get()} returns the updated
* exclusion or {@code null} if not found.
*
* Example of asynchronous exclusion update:
*
*
{@code
* String exclusionName = "my_exclusion_name";
* Exclusion exclusion = Exclusion
* .newBuilder(exclusionName, "resource.type=gcs_bucket severity future = logging.updateAsync(exclusion);
* // ...
* Exclusion exclusion = future.get();
* }
*/
ApiFuture updateAsync(Exclusion exclusion);
/**
* Returns the requested sink or {@code null} if not found.
*
* Example of getting a sink.
*
*
{@code
* String sinkName = "my_sink_name";
* Sink sink = logging.getSink(sinkName);
* if (sink == null) {
* // sink was not found
* }
* }
*
* @throws LoggingException upon failure
*/
Sink getSink(String sink);
/**
* Sends a request for getting a sink. This method returns a {@code ApiFuture} object to consume
* the result. {@link ApiFuture#get()} returns the requested sink or {@code null} if not found.
*
* Example of asynchronously getting a sink.
*
*
{@code
* String sinkName = "my_sink_name";
* ApiFuture future = logging.getSinkAsync(sinkName);
* // ...
* Sink sink = future.get();
* if (sink == null) {
* // sink was not found
* }
* }
*
* @throws LoggingException upon failure
*/
ApiFuture getSinkAsync(String sink);
/**
* Lists the sinks. This method returns a {@link Page} object that can be used to consume
* paginated results. Use {@link ListOption} to specify the page size or the page token from which
* to start listing sinks.
*
* Example of listing sinks, specifying the page size.
*
*
{@code
* Page sinks = logging.listSinks(ListOption.pageSize(100));
* Iterator sinkIterator = sinks.iterateAll().iterator();
* while (sinkIterator.hasNext()) {
* Sink sink = sinkIterator.next();
* // do something with the sink
* }
* }
*
* @throws LoggingException upon failure
*/
Page listSinks(ListOption... options);
/**
* Sends a request for listing sinks. This method returns a {@code ApiFuture} object to consume
* the result. {@link ApiFuture#get()} returns an {@link AsyncPage} object that can be used to
* asynchronously handle paginated results. Use {@link ListOption} to specify the page size or the
* page token from which to start listing sinks.
*
* Example of asynchronously listing sinks, specifying the page size.
*
*
{@code
* ApiFuture> future = logging.listSinksAsync(ListOption.pageSize(100));
* // ...
* AsyncPage sinks = future.get();
* Iterator sinkIterator = sinks.iterateAll().iterator();
* while (sinkIterator.hasNext()) {
* Sink sink = sinkIterator.next();
* // do something with the sink
* }
* }
*/
ApiFuture> listSinksAsync(ListOption... options);
/**
* Deletes the requested sink.
*
* Example of deleting a sink.
*
*
{@code
* String sinkName = "my_sink_name";
* boolean deleted = logging.deleteSink(sinkName);
* if (deleted) {
* // the sink was deleted
* } else {
* // the sink was not found
* }
* }
*
* @return {@code true} if the sink was deleted, {@code false} if it was not found
*/
boolean deleteSink(String sink);
/**
* Sends a request for deleting a sink. This method returns a {@code ApiFuture} object to consume
* the result. {@link ApiFuture#get()} returns {@code true} if the sink was deleted, {@code false}
* if it was not found.
*
* Example of asynchronously deleting a sink.
*
*
{@code
* String sinkName = "my_sink_name";
* ApiFuture future = logging.deleteSinkAsync(sinkName);
* // ...
* boolean deleted = future.get();
* if (deleted) {
* // the sink was deleted
* } else {
* // the sink was not found
* }
* }
*/
ApiFuture deleteSinkAsync(String sink);
/**
* Lists the log names. This method returns a {@link Page} object that can be used to consume
* paginated results. Use {@link ListOption} to specify the page size or the page token from which
* to start listing logs.
*
* Example of listing log names, specifying the page size.
*
*
{@code
* Page logNames = logging.listLogs(ListOption.pageSize(100));
* Iterator logIterator = logNames.iterateAll().iterator();
* while (logIterator.hasNext()) {
* String logName = logIterator.next();
* // do something with the log name
* }
* }
*
* @throws LoggingException upon failure
*/
default Page listLogs(ListOption... options) {
throw new UnsupportedOperationException(
"method listLogs() does not have default implementation");
}
/**
* Sends a request for listing log names. This method returns a {@code ApiFuture} object to
* consume the result. {@link ApiFuture#get()} returns an {@link AsyncPage} object that can be
* used to asynchronously handle paginated results. Use {@link ListOption} to specify the page
* size or the page token from which to start listing log names.
*
* Example of asynchronously listing log names, specifying the page size.
*
*
{@code
* ApiFuture> future = logging.listLogsAsync(ListOption.pageSize(100));
* // ...
* AsyncPage logNames = future.get();
* Iterator logIterator = logNames.iterateAll().iterator();
* while (logIterator.hasNext()) {
* String logName = logIterator.next();
* // do something with the log name
* }
* }
*/
default ApiFuture> listLogsAsync(ListOption... options) {
throw new UnsupportedOperationException(
"method listLogsAsync() does not have default implementation");
}
/**
* Deletes a log and all its log entries. The log will reappear if new entries are written to it.
*
* Example of deleting a log.
*
*
{@code
* String logName = "my_log_name";
* boolean deleted = logging.deleteLog(logName);
* if (deleted) {
* // the log was deleted
* } else {
* // the log was not found
* }
* }
*
* @return {@code true} if the log was deleted, {@code false} if it was not found
*/
boolean deleteLog(String log);
/**
* Deletes a log and all its log entries for given log destination (see 'logName' parameter in
* https://cloud.google.com/logging/docs/reference/v2/rest/v2/LogEntry). The log will reappear if
* new entries are written to it.
*
* Example of deleting a log by folder destination.
*
*
{@code
* String logName = "my_log_name";
* String folder = "my_folder";
* boolean deleted = logging.deleteLog(logName, LogDestinationName.folder(folder));
* if (deleted) {
* // the log was deleted
* } else {
* // the log was not found
* }
* }
*
* @return {@code true} if the log was deleted, {@code false} if it was not found
*/
default boolean deleteLog(String log, LogDestinationName destination) {
throw new UnsupportedOperationException(
"method deleteLog() does not have default implementation");
}
/**
* Sends a request for deleting a log and all its log entries for given log destination (see
* 'logName' parameter in https://cloud.google.com/logging/docs/reference/v2/rest/v2/LogEntry).
* This method returns a {@code ApiFuture} object to consume the result. {@link ApiFuture#get()}
* returns {@code true} if the log was deleted, {@code false} if it was not found.
*
* Example of asynchronously deleting a log by folder destination.
*
*
{@code
* String logName = "my_log_name";
* String folder = "my_folder";
* ApiFuture future = logging.deleteLogAsync(logName, LogDestinationName.folder(folder));
* // ...
* boolean deleted = future.get();
* if (deleted) {
* // the log was deleted
* } else {
* // the log was not found
* }
* }
*/
default ApiFuture deleteLogAsync(String log, LogDestinationName destination) {
throw new UnsupportedOperationException(
"method deleteLogAsync() does not have default implementation");
}
/**
* Sends a request for deleting a log and all its log entries. This method returns a {@code
* ApiFuture} object to consume the result. {@link ApiFuture#get()} returns {@code true} if the
* log was deleted, {@code false} if it was not found.
*
* Example of asynchronously deleting a log.
*
*
{@code
* String logName = "my_log_name";
* ApiFuture future = logging.deleteLogAsync(logName);
* // ...
* boolean deleted = future.get();
* if (deleted) {
* // the log was deleted
* } else {
* // the log was not found
* }
* }
*/
ApiFuture deleteLogAsync(String log);
/**
* Lists the monitored resource descriptors used by Cloud Logging. This method returns a {@link
* Page} object that can be used to consume paginated results. Use {@link ListOption} to specify
* the page size or the page token from which to start listing resource descriptors.
*
* Example of listing monitored resource descriptors, specifying the page size.
*
*
{@code
* Page descriptors = logging
* .listMonitoredResourceDescriptors(ListOption.pageSize(100));
* Iterator descriptorIterator = descriptors.iterateAll().iterator();
* while (descriptorIterator.hasNext()) {
* MonitoredResourceDescriptor descriptor = descriptorIterator.next();
* // do something with the descriptor
* }
* }
*
* @throws LoggingException upon failure
*/
Page listMonitoredResourceDescriptors(ListOption... options);
/**
* Sends a request for listing monitored resource descriptors used by Cloud Logging. This method
* returns a {@code ApiFuture} object to consume the result. {@link ApiFuture#get()} returns an
* {@link AsyncPage} object that can be used to asynchronously handle paginated results. Use
* {@link ListOption} to specify the page size or the page token from which to start listing
* resource descriptors.
*
* Example of asynchronously listing monitored resource descriptors, specifying the page size.
*
*
{@code
* ApiFuture> future = logging
* .listMonitoredResourceDescriptorsAsync(ListOption.pageSize(100));
* // ...
* AsyncPage descriptors = future.get();
* Iterator descriptorIterator = descriptors.iterateAll().iterator();
* while (descriptorIterator.hasNext()) {
* MonitoredResourceDescriptor descriptor = descriptorIterator.next();
* // do something with the descriptor
* }
* }
*/
ApiFuture> listMonitoredResourceDescriptorsAsync(
ListOption... options);
/**
* Returns the requested metric or {@code null} if not found.
*
* Example of getting a metric.
*
*
{@code
* String metricName = "my_metric_name";
* Metric metric = logging.getMetric(metricName);
* if (metric == null) {
* // metric was not found
* }
* }
*
* @throws LoggingException upon failure
*/
Metric getMetric(String metric);
/**
* Sends a request for getting a metric. This method returns a {@code ApiFuture} object to consume
* the result. {@link ApiFuture#get()} returns the requested metric or {@code null} if not found.
*
* Example of asynchronously getting a metric.
*
*
{@code
* String metricName = "my_metric_name";
* ApiFuture future = logging.getMetricAsync(metricName);
* // ...
* Metric metric = future.get();
* if (metric == null) {
* // metric was not found
* }
* }
*
* @throws LoggingException upon failure
*/
ApiFuture getMetricAsync(String metric);
/**
* Lists the metrics. This method returns a {@link Page} object that can be used to consume
* paginated results. Use {@link ListOption} to specify the page size or the page token from which
* to start listing metrics.
*
* Example of listing metrics, specifying the page size.
*
*
{@code
* Page metrics = logging.listMetrics(ListOption.pageSize(100));
* Iterator metricIterator = metrics.iterateAll().iterator();
* while (metricIterator.hasNext()) {
* Metric metric = metricIterator.next();
* // do something with the metric
* }
* }
*
* @throws LoggingException upon failure
*/
Page listMetrics(ListOption... options);
/**
* Sends a request for listing metrics. This method returns a {@code ApiFuture} object to consume
* the result. {@link ApiFuture#get()} returns an {@link AsyncPage} object that can be used to
* asynchronously handle paginated results. Use {@link ListOption} to specify the page size or the
* page token from which to start listing metrics.
*
* Example of asynchronously listing metrics, specifying the page size.
*
*
{@code
* ApiFuture> future = logging.listMetricsAsync(ListOption.pageSize(100));
* // ...
* AsyncPage metrics = future.get();
* Iterator metricIterator = metrics.iterateAll().iterator();
* while (metricIterator.hasNext()) {
* Metric metric = metricIterator.next();
* // do something with the metric
* }
* }
*/
ApiFuture> listMetricsAsync(ListOption... options);
/**
* Deletes the requested metric.
*
* Example of deleting a metric.
*
*
{@code
* String metricName = "my_metric_name";
* boolean deleted = logging.deleteMetric(metricName);
* if (deleted) {
* // the metric was deleted
* } else {
* // the metric was not found
* }
* }
*
* @return {@code true} if the metric was deleted, {@code false} if it was not found
*/
boolean deleteMetric(String metric);
/**
* Sends a request for deleting a metric. This method returns a {@code ApiFuture} object to
* consume the result. {@link ApiFuture#get()} returns {@code true} if the metric was deleted,
* {@code false} if it was not found.
*
* Example of asynchronously deleting a metric.
*
*
{@code
* String metricName = "my_metric_name";
* ApiFuture future = logging.deleteMetricAsync(metricName);
* // ...
* boolean deleted = future.get();
* if (deleted) {
* // the metric was deleted
* } else {
* // the metric was not found
* }
* }
*/
ApiFuture deleteMetricAsync(String metric);
/**
* Gets the description of an exclusion or {@code null} if not found.
*
* Example of getting the description of an exclusion:
*
*
{@code
* String exclusionName = "my_exclusion_name";
* Exclusion exclusion = logging.getExclusion(exclusionName);
* if (exclusion == null) {
* // exclusion was not found
* }
* }
*
* @throws LoggingException upon failure
*/
Exclusion getExclusion(String exclusion);
/**
* Sends a request to get the description of an exclusion . This method returns an {@code
* ApiFuture} object to consume the result. {@link ApiFuture#get()} returns the requested
* exclusion or {@code null} if not found.
*
* Example of asynchronously getting the exclusion:
*
*
{@code
* String exclusionName = "my_exclusion_name";
* ApiFuture future = logging.getExclusionAsync(exclusionName);
* // ...
* Exclusion exclusion = future.get();
* if (exclusion == null) {
* // exclusion was not found
* }
* }
*
* @throws LoggingException upon failure
*/
ApiFuture getExclusionAsync(String exclusion);
/**
* Deletes the requested exclusion.
*
* Example of deleting the exclusion:
*
*
{@code
* String exclusionName = "my_exclusion_name";
* boolean deleted = logging.deleteExclusion(exclusionName);
* if (deleted) {
* // the exclusion was deleted
* } else {
* // the exclusion was not found
* }
* }
*
* @return {@code true} if the exclusion was deleted, {@code false} if it was not found
*/
boolean deleteExclusion(String exclusion);
/**
* Sends a request to delete an exclusion. This method returns an {@code ApiFuture} object to
* consume the result. {@link ApiFuture#get()} returns {@code true} if the exclusion was deleted,
* {@code false} if it was not found.
*
* Example of asynchronously deleting the exclusion:
*
*
{@code
* String exclusionName = "my_exclusion_name";
* ApiFuture future = logging.deleteExclusionAsync(metricName);
* // ...
* boolean deleted = future.get();
* if (deleted) {
* // the exclusion was deleted
* } else {
* // the exclusion was not found
* }
* }
*/
ApiFuture deleteExclusionAsync(String exclusion);
/**
* Lists the exclusion. This method returns a {@link Page} object that can be used to consume
* paginated results. Use {@link ListOption} to specify the page size or the page token from which
* to start listing exclusion.
*
* Example of listing exclusions, specifying the page size:
*
*
{@code
* Page exclusions = logging.listMetrics(ListOption.pageSize(100));
* Iterator exclusionIterator = exclusions.iterateAll().iterator();
* while (exclusionIterator.hasNext()) {
* Exclusion exclusion = exclusionIterator.next();
* // do something with the exclusion
* }
* }
*
* @throws LoggingException upon failure
*/
Page listExclusions(ListOption... options);
/**
* Sends a request for listing exclusions. This method returns an {@code ApiFuture} object to
* consume the result. {@link ApiFuture#get()} returns an {@link AsyncPage} object that can be
* used to asynchronously handle paginated results. Use {@link ListOption} to specify the page
* size or the page token from which to start listing exclusions.
*
* Example of asynchronously listing exclusions, specifying the page size:
*
*
{@code
* ApiFuture> future = logging.listExclusionsAsync(ListOption.pageSize(100));
* // ...
* AsyncPage exclusions = future.get();
* Iterator exclusionIterator = exclusions.iterateAll().iterator();
* while (exclusionIterator.hasNext()) {
* Exclusion exclusion = exclusionIterator.next();
* // do something with the exclusion
* }
* }
*/
ApiFuture> listExclusionsAsync(ListOption... options);
/**
* Flushes any pending asynchronous logging writes. Logs are automatically flushed based on time
* and message count that be configured via {@link com.google.api.gax.batching.BatchingSettings},
* Logs are also flushed if enabled, at or above flush severity, see {@link #setFlushSeverity}.
* Logging frameworks require support for an explicit flush. See usage in the java.util.logging
* handler{@link LoggingHandler}.
*/
void flush();
/**
* Sends a request to log entries to Cloud Logging. Use {@link WriteOption#logName(String)} to
* provide a log name for those entries that do not specify one. Use {@link
* WriteOption#resource(MonitoredResource)} to provide a monitored resource for those entries that
* do not specify one. Use {@link WriteOption#labels(Map)} to provide some labels to be added to
* every entry in {@code logEntries}.
*
* Example of writing log entries and providing a default log name and monitored resource.
*
*
{@code
* String logName = "my_log_name";
* List entries = new ArrayList<>();
* entries.add(LogEntry.of(StringPayload.of("Entry payload")));
* Map jsonMap = new HashMap<>();
* jsonMap.put("key", "value");
* entries.add(LogEntry.of(JsonPayload.of(jsonMap)));
* logging.write(entries, WriteOption.logName(logName),
* WriteOption.resource(MonitoredResource.newBuilder("global").build()));
* }
*/
void write(Iterable logEntries, WriteOption... options);
/**
* Lists log entries. This method returns a {@link Page} object that can be used to consume
* paginated results. Use {@link EntryListOption#pageSize(int)} to specify the page size. Use
* {@link EntryListOption#pageToken(String)} to specify the page token from which to start listing
* entries. Use {@link EntryListOption#sortOrder(SortingField, SortingOrder)} to sort log entries
* according to your preferred order (default is most-recent last). Use {@link
* EntryListOption#filter(String)} to filter listed log entries. By default a 24 hour filter is
* applied.
*
* Example of listing log entries for a specific log.
*
*
{@code
* String filter = "logName=projects/my_project_id/logs/my_log_name";
* Page entries = logging.listLogEntries(EntryListOption.filter(filter));
* Iterator entryIterator = entries.iterateAll().iterator();
* while (entryIterator.hasNext()) {
* LogEntry entry = entryIterator.next();
* // do something with the entry
* }
* }
*
* @throws LoggingException upon failure
*/
Page listLogEntries(EntryListOption... options);
/**
* Sends a request for listing log entries. This method returns a {@code ApiFuture} object to
* consume the result. {@link ApiFuture#get()} returns an {@link AsyncPage} object that can be
* used to asynchronously handle paginated results. Use {@link EntryListOption#pageSize(int)} to
* specify the page size. Use {@link EntryListOption#pageToken(String)} to specify the page token
* from which to start listing entries. Use {@link EntryListOption#sortOrder(SortingField,
* SortingOrder)} to sort log entries according to your preferred order (default is most-recent
* last). Use {@link EntryListOption#filter(String)} to filter listed log entries. By default a 24
* hour filter is applied.
*
* Example of asynchronously listing log entries for a specific log.
*
*
{@code
* String filter = "logName=projects/my_project_id/logs/my_log_name";
* ApiFuture> future = logging.listLogEntriesAsync(EntryListOption.filter(filter));
* // ...
* AsyncPage entries = future.get();
* Iterator entryIterator = entries.iterateAll().iterator();
* while (entryIterator.hasNext()) {
* LogEntry entry = entryIterator.next();
* // do something with the entry
* }
* }
*
* @throws LoggingException upon failure
*/
ApiFuture> listLogEntriesAsync(EntryListOption... options);
/**
* Sends a request to stream fresh log entries. The method returns a {@code LogEntryServerStream}
* object to iterate through the returned stream of the log entries. Use
* EntryListOption#bufferWindow(String)} to specify amount of time to buffer log entries at the
* server before being returned. entries. Use {@link TailOption#filter(String)} to filter tailed
* log entries.
*
* Example of streaming log entries for a specific project.
*
*
{@code
* LogEntryServerStream stream = logging.tailLogEntries(TailOption.project("my_project_id"));
* Iterator it = stream.iterator();
* while (it.hasNext()) {
* // do something with entry
* // call stream.cancel(); to stop streaming
* }
* }
*/
@BetaApi("The surface for the tail streaming is not stable yet and may change in the future.")
LogEntryServerStream tailLogEntries(TailOption... options);
/**
* Populates metadata fields of the immutable collection of {@link LogEntry} items. Only empty
* fields are populated. The {@link SourceLocation} is populated only for items with the severity
* set to {@link Severity#DEBUG}. The information about {@link HttpRequest}, trace and span Id is
* retrieved using {@link ContextHandler}.
*
* @param logEntries an immutable collection of {@link LogEntry} items.
* @param customResource a customized instance of the {@link MonitoredResource}. If this parameter
* is {@code null} then the new instance will be generated using {@link
* MonitoredResourceUtil#getResource(String, String)}.
* @param exclusionClassPaths a list of exclussion class path prefixes. If left empty then {@link
* SourceLocation} instance is built based on the caller's stack trace information. Otherwise,
* the information from the first {@link StackTraceElement} along the call stack which class
* name does not start with any not {@code null} exclusion class paths is used.
* @return A collection of {@link LogEntry} items composed from the {@code logEntries} parameter
* with populated metadata fields.
*/
default Iterable populateMetadata(
Iterable logEntries,
MonitoredResource customResource,
String... exclusionClassPaths) {
throw new UnsupportedOperationException(
"method populateMetadata() does not have default implementation");
}
}