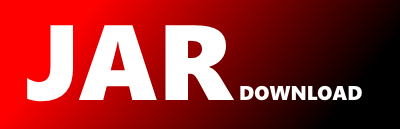
com.google.cloud.logging.v2.ConfigSettings Maven / Gradle / Ivy
Show all versions of google-cloud-logging Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.logging.v2;
import static com.google.cloud.logging.v2.ConfigClient.ListBucketsPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListExclusionsPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListLinksPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListSinksPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListViewsPagedResponse;
import com.google.api.core.ApiFunction;
import com.google.api.gax.core.GoogleCredentialsProvider;
import com.google.api.gax.core.InstantiatingExecutorProvider;
import com.google.api.gax.grpc.InstantiatingGrpcChannelProvider;
import com.google.api.gax.rpc.ApiClientHeaderProvider;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.ClientSettings;
import com.google.api.gax.rpc.OperationCallSettings;
import com.google.api.gax.rpc.PagedCallSettings;
import com.google.api.gax.rpc.TransportChannelProvider;
import com.google.api.gax.rpc.UnaryCallSettings;
import com.google.cloud.logging.v2.stub.ConfigServiceV2StubSettings;
import com.google.logging.v2.BucketMetadata;
import com.google.logging.v2.CmekSettings;
import com.google.logging.v2.CopyLogEntriesMetadata;
import com.google.logging.v2.CopyLogEntriesRequest;
import com.google.logging.v2.CopyLogEntriesResponse;
import com.google.logging.v2.CreateBucketRequest;
import com.google.logging.v2.CreateExclusionRequest;
import com.google.logging.v2.CreateLinkRequest;
import com.google.logging.v2.CreateSinkRequest;
import com.google.logging.v2.CreateViewRequest;
import com.google.logging.v2.DeleteBucketRequest;
import com.google.logging.v2.DeleteExclusionRequest;
import com.google.logging.v2.DeleteLinkRequest;
import com.google.logging.v2.DeleteSinkRequest;
import com.google.logging.v2.DeleteViewRequest;
import com.google.logging.v2.GetBucketRequest;
import com.google.logging.v2.GetCmekSettingsRequest;
import com.google.logging.v2.GetExclusionRequest;
import com.google.logging.v2.GetLinkRequest;
import com.google.logging.v2.GetSettingsRequest;
import com.google.logging.v2.GetSinkRequest;
import com.google.logging.v2.GetViewRequest;
import com.google.logging.v2.Link;
import com.google.logging.v2.LinkMetadata;
import com.google.logging.v2.ListBucketsRequest;
import com.google.logging.v2.ListBucketsResponse;
import com.google.logging.v2.ListExclusionsRequest;
import com.google.logging.v2.ListExclusionsResponse;
import com.google.logging.v2.ListLinksRequest;
import com.google.logging.v2.ListLinksResponse;
import com.google.logging.v2.ListSinksRequest;
import com.google.logging.v2.ListSinksResponse;
import com.google.logging.v2.ListViewsRequest;
import com.google.logging.v2.ListViewsResponse;
import com.google.logging.v2.LogBucket;
import com.google.logging.v2.LogExclusion;
import com.google.logging.v2.LogSink;
import com.google.logging.v2.LogView;
import com.google.logging.v2.Settings;
import com.google.logging.v2.UndeleteBucketRequest;
import com.google.logging.v2.UpdateBucketRequest;
import com.google.logging.v2.UpdateCmekSettingsRequest;
import com.google.logging.v2.UpdateExclusionRequest;
import com.google.logging.v2.UpdateSettingsRequest;
import com.google.logging.v2.UpdateSinkRequest;
import com.google.logging.v2.UpdateViewRequest;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import java.io.IOException;
import java.util.List;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Settings class to configure an instance of {@link ConfigClient}.
*
* The default instance has everything set to sensible defaults:
*
*
* - The default service address (logging.googleapis.com) and default port (443) are used.
*
- Credentials are acquired automatically through Application Default Credentials.
*
- Retries are configured for idempotent methods but not for non-idempotent methods.
*
*
* The builder of this class is recursive, so contained classes are themselves builders. When
* build() is called, the tree of builders is called to create the complete settings object.
*
*
For example, to set the total timeout of getBucket to 30 seconds:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ConfigSettings.Builder configSettingsBuilder = ConfigSettings.newBuilder();
* configSettingsBuilder
* .getBucketSettings()
* .setRetrySettings(
* configSettingsBuilder
* .getBucketSettings()
* .getRetrySettings()
* .toBuilder()
* .setTotalTimeout(Duration.ofSeconds(30))
* .build());
* ConfigSettings configSettings = configSettingsBuilder.build();
* }
*/
@Generated("by gapic-generator-java")
public class ConfigSettings extends ClientSettings {
/** Returns the object with the settings used for calls to listBuckets. */
public PagedCallSettings
listBucketsSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).listBucketsSettings();
}
/** Returns the object with the settings used for calls to getBucket. */
public UnaryCallSettings getBucketSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).getBucketSettings();
}
/** Returns the object with the settings used for calls to createBucketAsync. */
public UnaryCallSettings createBucketAsyncSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).createBucketAsyncSettings();
}
/** Returns the object with the settings used for calls to createBucketAsync. */
public OperationCallSettings
createBucketAsyncOperationSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).createBucketAsyncOperationSettings();
}
/** Returns the object with the settings used for calls to updateBucketAsync. */
public UnaryCallSettings updateBucketAsyncSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).updateBucketAsyncSettings();
}
/** Returns the object with the settings used for calls to updateBucketAsync. */
public OperationCallSettings
updateBucketAsyncOperationSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).updateBucketAsyncOperationSettings();
}
/** Returns the object with the settings used for calls to createBucket. */
public UnaryCallSettings createBucketSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).createBucketSettings();
}
/** Returns the object with the settings used for calls to updateBucket. */
public UnaryCallSettings updateBucketSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).updateBucketSettings();
}
/** Returns the object with the settings used for calls to deleteBucket. */
public UnaryCallSettings deleteBucketSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).deleteBucketSettings();
}
/** Returns the object with the settings used for calls to undeleteBucket. */
public UnaryCallSettings undeleteBucketSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).undeleteBucketSettings();
}
/** Returns the object with the settings used for calls to listViews. */
public PagedCallSettings
listViewsSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).listViewsSettings();
}
/** Returns the object with the settings used for calls to getView. */
public UnaryCallSettings getViewSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).getViewSettings();
}
/** Returns the object with the settings used for calls to createView. */
public UnaryCallSettings createViewSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).createViewSettings();
}
/** Returns the object with the settings used for calls to updateView. */
public UnaryCallSettings updateViewSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).updateViewSettings();
}
/** Returns the object with the settings used for calls to deleteView. */
public UnaryCallSettings deleteViewSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).deleteViewSettings();
}
/** Returns the object with the settings used for calls to listSinks. */
public PagedCallSettings
listSinksSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).listSinksSettings();
}
/** Returns the object with the settings used for calls to getSink. */
public UnaryCallSettings getSinkSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).getSinkSettings();
}
/** Returns the object with the settings used for calls to createSink. */
public UnaryCallSettings createSinkSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).createSinkSettings();
}
/** Returns the object with the settings used for calls to updateSink. */
public UnaryCallSettings updateSinkSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).updateSinkSettings();
}
/** Returns the object with the settings used for calls to deleteSink. */
public UnaryCallSettings deleteSinkSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).deleteSinkSettings();
}
/** Returns the object with the settings used for calls to createLink. */
public UnaryCallSettings createLinkSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).createLinkSettings();
}
/** Returns the object with the settings used for calls to createLink. */
public OperationCallSettings
createLinkOperationSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).createLinkOperationSettings();
}
/** Returns the object with the settings used for calls to deleteLink. */
public UnaryCallSettings deleteLinkSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).deleteLinkSettings();
}
/** Returns the object with the settings used for calls to deleteLink. */
public OperationCallSettings
deleteLinkOperationSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).deleteLinkOperationSettings();
}
/** Returns the object with the settings used for calls to listLinks. */
public PagedCallSettings
listLinksSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).listLinksSettings();
}
/** Returns the object with the settings used for calls to getLink. */
public UnaryCallSettings getLinkSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).getLinkSettings();
}
/** Returns the object with the settings used for calls to listExclusions. */
public PagedCallSettings<
ListExclusionsRequest, ListExclusionsResponse, ListExclusionsPagedResponse>
listExclusionsSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).listExclusionsSettings();
}
/** Returns the object with the settings used for calls to getExclusion. */
public UnaryCallSettings getExclusionSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).getExclusionSettings();
}
/** Returns the object with the settings used for calls to createExclusion. */
public UnaryCallSettings createExclusionSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).createExclusionSettings();
}
/** Returns the object with the settings used for calls to updateExclusion. */
public UnaryCallSettings updateExclusionSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).updateExclusionSettings();
}
/** Returns the object with the settings used for calls to deleteExclusion. */
public UnaryCallSettings deleteExclusionSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).deleteExclusionSettings();
}
/** Returns the object with the settings used for calls to getCmekSettings. */
public UnaryCallSettings getCmekSettingsSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).getCmekSettingsSettings();
}
/** Returns the object with the settings used for calls to updateCmekSettings. */
public UnaryCallSettings updateCmekSettingsSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).updateCmekSettingsSettings();
}
/** Returns the object with the settings used for calls to getSettings. */
public UnaryCallSettings getSettingsSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).getSettingsSettings();
}
/** Returns the object with the settings used for calls to updateSettings. */
public UnaryCallSettings updateSettingsSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).updateSettingsSettings();
}
/** Returns the object with the settings used for calls to copyLogEntries. */
public UnaryCallSettings copyLogEntriesSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).copyLogEntriesSettings();
}
/** Returns the object with the settings used for calls to copyLogEntries. */
public OperationCallSettings<
CopyLogEntriesRequest, CopyLogEntriesResponse, CopyLogEntriesMetadata>
copyLogEntriesOperationSettings() {
return ((ConfigServiceV2StubSettings) getStubSettings()).copyLogEntriesOperationSettings();
}
public static final ConfigSettings create(ConfigServiceV2StubSettings stub) throws IOException {
return new ConfigSettings.Builder(stub.toBuilder()).build();
}
/** Returns a builder for the default ExecutorProvider for this service. */
public static InstantiatingExecutorProvider.Builder defaultExecutorProviderBuilder() {
return ConfigServiceV2StubSettings.defaultExecutorProviderBuilder();
}
/** Returns the default service endpoint. */
public static String getDefaultEndpoint() {
return ConfigServiceV2StubSettings.getDefaultEndpoint();
}
/** Returns the default service scopes. */
public static List getDefaultServiceScopes() {
return ConfigServiceV2StubSettings.getDefaultServiceScopes();
}
/** Returns a builder for the default credentials for this service. */
public static GoogleCredentialsProvider.Builder defaultCredentialsProviderBuilder() {
return ConfigServiceV2StubSettings.defaultCredentialsProviderBuilder();
}
/** Returns a builder for the default ChannelProvider for this service. */
public static InstantiatingGrpcChannelProvider.Builder defaultGrpcTransportProviderBuilder() {
return ConfigServiceV2StubSettings.defaultGrpcTransportProviderBuilder();
}
public static TransportChannelProvider defaultTransportChannelProvider() {
return ConfigServiceV2StubSettings.defaultTransportChannelProvider();
}
public static ApiClientHeaderProvider.Builder defaultApiClientHeaderProviderBuilder() {
return ConfigServiceV2StubSettings.defaultApiClientHeaderProviderBuilder();
}
/** Returns a new builder for this class. */
public static Builder newBuilder() {
return Builder.createDefault();
}
/** Returns a new builder for this class. */
public static Builder newBuilder(ClientContext clientContext) {
return new Builder(clientContext);
}
/** Returns a builder containing all the values of this settings class. */
public Builder toBuilder() {
return new Builder(this);
}
protected ConfigSettings(Builder settingsBuilder) throws IOException {
super(settingsBuilder);
}
/** Builder for ConfigSettings. */
public static class Builder extends ClientSettings.Builder {
protected Builder() throws IOException {
this(((ClientContext) null));
}
protected Builder(ClientContext clientContext) {
super(ConfigServiceV2StubSettings.newBuilder(clientContext));
}
protected Builder(ConfigSettings settings) {
super(settings.getStubSettings().toBuilder());
}
protected Builder(ConfigServiceV2StubSettings.Builder stubSettings) {
super(stubSettings);
}
private static Builder createDefault() {
return new Builder(ConfigServiceV2StubSettings.newBuilder());
}
public ConfigServiceV2StubSettings.Builder getStubSettingsBuilder() {
return ((ConfigServiceV2StubSettings.Builder) getStubSettings());
}
/**
* Applies the given settings updater function to all of the unary API methods in this service.
*
* Note: This method does not support applying settings to streaming methods.
*/
public Builder applyToAllUnaryMethods(
ApiFunction, Void> settingsUpdater) {
super.applyToAllUnaryMethods(
getStubSettingsBuilder().unaryMethodSettingsBuilders(), settingsUpdater);
return this;
}
/** Returns the builder for the settings used for calls to listBuckets. */
public PagedCallSettings.Builder<
ListBucketsRequest, ListBucketsResponse, ListBucketsPagedResponse>
listBucketsSettings() {
return getStubSettingsBuilder().listBucketsSettings();
}
/** Returns the builder for the settings used for calls to getBucket. */
public UnaryCallSettings.Builder getBucketSettings() {
return getStubSettingsBuilder().getBucketSettings();
}
/** Returns the builder for the settings used for calls to createBucketAsync. */
public UnaryCallSettings.Builder createBucketAsyncSettings() {
return getStubSettingsBuilder().createBucketAsyncSettings();
}
/** Returns the builder for the settings used for calls to createBucketAsync. */
public OperationCallSettings.Builder
createBucketAsyncOperationSettings() {
return getStubSettingsBuilder().createBucketAsyncOperationSettings();
}
/** Returns the builder for the settings used for calls to updateBucketAsync. */
public UnaryCallSettings.Builder updateBucketAsyncSettings() {
return getStubSettingsBuilder().updateBucketAsyncSettings();
}
/** Returns the builder for the settings used for calls to updateBucketAsync. */
public OperationCallSettings.Builder
updateBucketAsyncOperationSettings() {
return getStubSettingsBuilder().updateBucketAsyncOperationSettings();
}
/** Returns the builder for the settings used for calls to createBucket. */
public UnaryCallSettings.Builder createBucketSettings() {
return getStubSettingsBuilder().createBucketSettings();
}
/** Returns the builder for the settings used for calls to updateBucket. */
public UnaryCallSettings.Builder updateBucketSettings() {
return getStubSettingsBuilder().updateBucketSettings();
}
/** Returns the builder for the settings used for calls to deleteBucket. */
public UnaryCallSettings.Builder deleteBucketSettings() {
return getStubSettingsBuilder().deleteBucketSettings();
}
/** Returns the builder for the settings used for calls to undeleteBucket. */
public UnaryCallSettings.Builder undeleteBucketSettings() {
return getStubSettingsBuilder().undeleteBucketSettings();
}
/** Returns the builder for the settings used for calls to listViews. */
public PagedCallSettings.Builder
listViewsSettings() {
return getStubSettingsBuilder().listViewsSettings();
}
/** Returns the builder for the settings used for calls to getView. */
public UnaryCallSettings.Builder getViewSettings() {
return getStubSettingsBuilder().getViewSettings();
}
/** Returns the builder for the settings used for calls to createView. */
public UnaryCallSettings.Builder createViewSettings() {
return getStubSettingsBuilder().createViewSettings();
}
/** Returns the builder for the settings used for calls to updateView. */
public UnaryCallSettings.Builder updateViewSettings() {
return getStubSettingsBuilder().updateViewSettings();
}
/** Returns the builder for the settings used for calls to deleteView. */
public UnaryCallSettings.Builder deleteViewSettings() {
return getStubSettingsBuilder().deleteViewSettings();
}
/** Returns the builder for the settings used for calls to listSinks. */
public PagedCallSettings.Builder
listSinksSettings() {
return getStubSettingsBuilder().listSinksSettings();
}
/** Returns the builder for the settings used for calls to getSink. */
public UnaryCallSettings.Builder getSinkSettings() {
return getStubSettingsBuilder().getSinkSettings();
}
/** Returns the builder for the settings used for calls to createSink. */
public UnaryCallSettings.Builder createSinkSettings() {
return getStubSettingsBuilder().createSinkSettings();
}
/** Returns the builder for the settings used for calls to updateSink. */
public UnaryCallSettings.Builder updateSinkSettings() {
return getStubSettingsBuilder().updateSinkSettings();
}
/** Returns the builder for the settings used for calls to deleteSink. */
public UnaryCallSettings.Builder deleteSinkSettings() {
return getStubSettingsBuilder().deleteSinkSettings();
}
/** Returns the builder for the settings used for calls to createLink. */
public UnaryCallSettings.Builder createLinkSettings() {
return getStubSettingsBuilder().createLinkSettings();
}
/** Returns the builder for the settings used for calls to createLink. */
public OperationCallSettings.Builder
createLinkOperationSettings() {
return getStubSettingsBuilder().createLinkOperationSettings();
}
/** Returns the builder for the settings used for calls to deleteLink. */
public UnaryCallSettings.Builder deleteLinkSettings() {
return getStubSettingsBuilder().deleteLinkSettings();
}
/** Returns the builder for the settings used for calls to deleteLink. */
public OperationCallSettings.Builder
deleteLinkOperationSettings() {
return getStubSettingsBuilder().deleteLinkOperationSettings();
}
/** Returns the builder for the settings used for calls to listLinks. */
public PagedCallSettings.Builder
listLinksSettings() {
return getStubSettingsBuilder().listLinksSettings();
}
/** Returns the builder for the settings used for calls to getLink. */
public UnaryCallSettings.Builder getLinkSettings() {
return getStubSettingsBuilder().getLinkSettings();
}
/** Returns the builder for the settings used for calls to listExclusions. */
public PagedCallSettings.Builder<
ListExclusionsRequest, ListExclusionsResponse, ListExclusionsPagedResponse>
listExclusionsSettings() {
return getStubSettingsBuilder().listExclusionsSettings();
}
/** Returns the builder for the settings used for calls to getExclusion. */
public UnaryCallSettings.Builder getExclusionSettings() {
return getStubSettingsBuilder().getExclusionSettings();
}
/** Returns the builder for the settings used for calls to createExclusion. */
public UnaryCallSettings.Builder
createExclusionSettings() {
return getStubSettingsBuilder().createExclusionSettings();
}
/** Returns the builder for the settings used for calls to updateExclusion. */
public UnaryCallSettings.Builder
updateExclusionSettings() {
return getStubSettingsBuilder().updateExclusionSettings();
}
/** Returns the builder for the settings used for calls to deleteExclusion. */
public UnaryCallSettings.Builder deleteExclusionSettings() {
return getStubSettingsBuilder().deleteExclusionSettings();
}
/** Returns the builder for the settings used for calls to getCmekSettings. */
public UnaryCallSettings.Builder
getCmekSettingsSettings() {
return getStubSettingsBuilder().getCmekSettingsSettings();
}
/** Returns the builder for the settings used for calls to updateCmekSettings. */
public UnaryCallSettings.Builder
updateCmekSettingsSettings() {
return getStubSettingsBuilder().updateCmekSettingsSettings();
}
/** Returns the builder for the settings used for calls to getSettings. */
public UnaryCallSettings.Builder getSettingsSettings() {
return getStubSettingsBuilder().getSettingsSettings();
}
/** Returns the builder for the settings used for calls to updateSettings. */
public UnaryCallSettings.Builder updateSettingsSettings() {
return getStubSettingsBuilder().updateSettingsSettings();
}
/** Returns the builder for the settings used for calls to copyLogEntries. */
public UnaryCallSettings.Builder copyLogEntriesSettings() {
return getStubSettingsBuilder().copyLogEntriesSettings();
}
/** Returns the builder for the settings used for calls to copyLogEntries. */
public OperationCallSettings.Builder<
CopyLogEntriesRequest, CopyLogEntriesResponse, CopyLogEntriesMetadata>
copyLogEntriesOperationSettings() {
return getStubSettingsBuilder().copyLogEntriesOperationSettings();
}
@Override
public ConfigSettings build() throws IOException {
return new ConfigSettings(this);
}
}
}