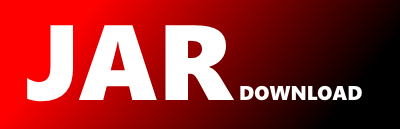
com.google.cloud.logging.v2.stub.ConfigServiceV2StubSettings Maven / Gradle / Ivy
Show all versions of google-cloud-logging Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.logging.v2.stub;
import static com.google.cloud.logging.v2.ConfigClient.ListBucketsPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListExclusionsPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListLinksPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListSinksPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListViewsPagedResponse;
import com.google.api.core.ApiFunction;
import com.google.api.core.ApiFuture;
import com.google.api.gax.core.GaxProperties;
import com.google.api.gax.core.GoogleCredentialsProvider;
import com.google.api.gax.core.InstantiatingExecutorProvider;
import com.google.api.gax.grpc.GaxGrpcProperties;
import com.google.api.gax.grpc.GrpcTransportChannel;
import com.google.api.gax.grpc.InstantiatingGrpcChannelProvider;
import com.google.api.gax.grpc.ProtoOperationTransformers;
import com.google.api.gax.longrunning.OperationSnapshot;
import com.google.api.gax.longrunning.OperationTimedPollAlgorithm;
import com.google.api.gax.retrying.RetrySettings;
import com.google.api.gax.rpc.ApiCallContext;
import com.google.api.gax.rpc.ApiClientHeaderProvider;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallSettings;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.PagedCallSettings;
import com.google.api.gax.rpc.PagedListDescriptor;
import com.google.api.gax.rpc.PagedListResponseFactory;
import com.google.api.gax.rpc.StatusCode;
import com.google.api.gax.rpc.StubSettings;
import com.google.api.gax.rpc.TransportChannelProvider;
import com.google.api.gax.rpc.UnaryCallSettings;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Lists;
import com.google.logging.v2.BucketMetadata;
import com.google.logging.v2.CmekSettings;
import com.google.logging.v2.CopyLogEntriesMetadata;
import com.google.logging.v2.CopyLogEntriesRequest;
import com.google.logging.v2.CopyLogEntriesResponse;
import com.google.logging.v2.CreateBucketRequest;
import com.google.logging.v2.CreateExclusionRequest;
import com.google.logging.v2.CreateLinkRequest;
import com.google.logging.v2.CreateSinkRequest;
import com.google.logging.v2.CreateViewRequest;
import com.google.logging.v2.DeleteBucketRequest;
import com.google.logging.v2.DeleteExclusionRequest;
import com.google.logging.v2.DeleteLinkRequest;
import com.google.logging.v2.DeleteSinkRequest;
import com.google.logging.v2.DeleteViewRequest;
import com.google.logging.v2.GetBucketRequest;
import com.google.logging.v2.GetCmekSettingsRequest;
import com.google.logging.v2.GetExclusionRequest;
import com.google.logging.v2.GetLinkRequest;
import com.google.logging.v2.GetSettingsRequest;
import com.google.logging.v2.GetSinkRequest;
import com.google.logging.v2.GetViewRequest;
import com.google.logging.v2.Link;
import com.google.logging.v2.LinkMetadata;
import com.google.logging.v2.ListBucketsRequest;
import com.google.logging.v2.ListBucketsResponse;
import com.google.logging.v2.ListExclusionsRequest;
import com.google.logging.v2.ListExclusionsResponse;
import com.google.logging.v2.ListLinksRequest;
import com.google.logging.v2.ListLinksResponse;
import com.google.logging.v2.ListSinksRequest;
import com.google.logging.v2.ListSinksResponse;
import com.google.logging.v2.ListViewsRequest;
import com.google.logging.v2.ListViewsResponse;
import com.google.logging.v2.LogBucket;
import com.google.logging.v2.LogExclusion;
import com.google.logging.v2.LogSink;
import com.google.logging.v2.LogView;
import com.google.logging.v2.Settings;
import com.google.logging.v2.UndeleteBucketRequest;
import com.google.logging.v2.UpdateBucketRequest;
import com.google.logging.v2.UpdateCmekSettingsRequest;
import com.google.logging.v2.UpdateExclusionRequest;
import com.google.logging.v2.UpdateSettingsRequest;
import com.google.logging.v2.UpdateSinkRequest;
import com.google.logging.v2.UpdateViewRequest;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import java.io.IOException;
import java.util.List;
import javax.annotation.Generated;
import org.threeten.bp.Duration;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Settings class to configure an instance of {@link ConfigServiceV2Stub}.
*
* The default instance has everything set to sensible defaults:
*
*
* - The default service address (logging.googleapis.com) and default port (443) are used.
*
- Credentials are acquired automatically through Application Default Credentials.
*
- Retries are configured for idempotent methods but not for non-idempotent methods.
*
*
* The builder of this class is recursive, so contained classes are themselves builders. When
* build() is called, the tree of builders is called to create the complete settings object.
*
*
For example, to set the total timeout of getBucket to 30 seconds:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ConfigServiceV2StubSettings.Builder configSettingsBuilder =
* ConfigServiceV2StubSettings.newBuilder();
* configSettingsBuilder
* .getBucketSettings()
* .setRetrySettings(
* configSettingsBuilder
* .getBucketSettings()
* .getRetrySettings()
* .toBuilder()
* .setTotalTimeout(Duration.ofSeconds(30))
* .build());
* ConfigServiceV2StubSettings configSettings = configSettingsBuilder.build();
* }
*/
@Generated("by gapic-generator-java")
public class ConfigServiceV2StubSettings extends StubSettings {
/** The default scopes of the service. */
private static final ImmutableList DEFAULT_SERVICE_SCOPES =
ImmutableList.builder()
.add("https://www.googleapis.com/auth/cloud-platform")
.add("https://www.googleapis.com/auth/cloud-platform.read-only")
.add("https://www.googleapis.com/auth/logging.admin")
.add("https://www.googleapis.com/auth/logging.read")
.build();
private final PagedCallSettings
listBucketsSettings;
private final UnaryCallSettings getBucketSettings;
private final UnaryCallSettings createBucketAsyncSettings;
private final OperationCallSettings
createBucketAsyncOperationSettings;
private final UnaryCallSettings updateBucketAsyncSettings;
private final OperationCallSettings
updateBucketAsyncOperationSettings;
private final UnaryCallSettings createBucketSettings;
private final UnaryCallSettings updateBucketSettings;
private final UnaryCallSettings deleteBucketSettings;
private final UnaryCallSettings undeleteBucketSettings;
private final PagedCallSettings
listViewsSettings;
private final UnaryCallSettings getViewSettings;
private final UnaryCallSettings createViewSettings;
private final UnaryCallSettings updateViewSettings;
private final UnaryCallSettings deleteViewSettings;
private final PagedCallSettings
listSinksSettings;
private final UnaryCallSettings getSinkSettings;
private final UnaryCallSettings createSinkSettings;
private final UnaryCallSettings updateSinkSettings;
private final UnaryCallSettings deleteSinkSettings;
private final UnaryCallSettings createLinkSettings;
private final OperationCallSettings
createLinkOperationSettings;
private final UnaryCallSettings deleteLinkSettings;
private final OperationCallSettings
deleteLinkOperationSettings;
private final PagedCallSettings
listLinksSettings;
private final UnaryCallSettings getLinkSettings;
private final PagedCallSettings<
ListExclusionsRequest, ListExclusionsResponse, ListExclusionsPagedResponse>
listExclusionsSettings;
private final UnaryCallSettings getExclusionSettings;
private final UnaryCallSettings createExclusionSettings;
private final UnaryCallSettings updateExclusionSettings;
private final UnaryCallSettings deleteExclusionSettings;
private final UnaryCallSettings getCmekSettingsSettings;
private final UnaryCallSettings
updateCmekSettingsSettings;
private final UnaryCallSettings getSettingsSettings;
private final UnaryCallSettings updateSettingsSettings;
private final UnaryCallSettings copyLogEntriesSettings;
private final OperationCallSettings<
CopyLogEntriesRequest, CopyLogEntriesResponse, CopyLogEntriesMetadata>
copyLogEntriesOperationSettings;
private static final PagedListDescriptor
LIST_BUCKETS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListBucketsRequest injectToken(ListBucketsRequest payload, String token) {
return ListBucketsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListBucketsRequest injectPageSize(ListBucketsRequest payload, int pageSize) {
return ListBucketsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListBucketsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListBucketsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListBucketsResponse payload) {
return payload.getBucketsList() == null
? ImmutableList.of()
: payload.getBucketsList();
}
};
private static final PagedListDescriptor
LIST_VIEWS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListViewsRequest injectToken(ListViewsRequest payload, String token) {
return ListViewsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListViewsRequest injectPageSize(ListViewsRequest payload, int pageSize) {
return ListViewsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListViewsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListViewsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListViewsResponse payload) {
return payload.getViewsList() == null
? ImmutableList.of()
: payload.getViewsList();
}
};
private static final PagedListDescriptor
LIST_SINKS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListSinksRequest injectToken(ListSinksRequest payload, String token) {
return ListSinksRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListSinksRequest injectPageSize(ListSinksRequest payload, int pageSize) {
return ListSinksRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListSinksRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListSinksResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListSinksResponse payload) {
return payload.getSinksList() == null
? ImmutableList.of()
: payload.getSinksList();
}
};
private static final PagedListDescriptor
LIST_LINKS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListLinksRequest injectToken(ListLinksRequest payload, String token) {
return ListLinksRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListLinksRequest injectPageSize(ListLinksRequest payload, int pageSize) {
return ListLinksRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListLinksRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListLinksResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListLinksResponse payload) {
return payload.getLinksList() == null
? ImmutableList.of()
: payload.getLinksList();
}
};
private static final PagedListDescriptor<
ListExclusionsRequest, ListExclusionsResponse, LogExclusion>
LIST_EXCLUSIONS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListExclusionsRequest injectToken(ListExclusionsRequest payload, String token) {
return ListExclusionsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListExclusionsRequest injectPageSize(
ListExclusionsRequest payload, int pageSize) {
return ListExclusionsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListExclusionsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListExclusionsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListExclusionsResponse payload) {
return payload.getExclusionsList() == null
? ImmutableList.of()
: payload.getExclusionsList();
}
};
private static final PagedListResponseFactory<
ListBucketsRequest, ListBucketsResponse, ListBucketsPagedResponse>
LIST_BUCKETS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListBucketsRequest, ListBucketsResponse, ListBucketsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListBucketsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_BUCKETS_PAGE_STR_DESC, request, context);
return ListBucketsPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListViewsRequest, ListViewsResponse, ListViewsPagedResponse>
LIST_VIEWS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListViewsRequest, ListViewsResponse, ListViewsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListViewsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_VIEWS_PAGE_STR_DESC, request, context);
return ListViewsPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListSinksRequest, ListSinksResponse, ListSinksPagedResponse>
LIST_SINKS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListSinksRequest, ListSinksResponse, ListSinksPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListSinksRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_SINKS_PAGE_STR_DESC, request, context);
return ListSinksPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListLinksRequest, ListLinksResponse, ListLinksPagedResponse>
LIST_LINKS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListLinksRequest, ListLinksResponse, ListLinksPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListLinksRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_LINKS_PAGE_STR_DESC, request, context);
return ListLinksPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListExclusionsRequest, ListExclusionsResponse, ListExclusionsPagedResponse>
LIST_EXCLUSIONS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListExclusionsRequest, ListExclusionsResponse, ListExclusionsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListExclusionsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_EXCLUSIONS_PAGE_STR_DESC, request, context);
return ListExclusionsPagedResponse.createAsync(pageContext, futureResponse);
}
};
/** Returns the object with the settings used for calls to listBuckets. */
public PagedCallSettings
listBucketsSettings() {
return listBucketsSettings;
}
/** Returns the object with the settings used for calls to getBucket. */
public UnaryCallSettings getBucketSettings() {
return getBucketSettings;
}
/** Returns the object with the settings used for calls to createBucketAsync. */
public UnaryCallSettings createBucketAsyncSettings() {
return createBucketAsyncSettings;
}
/** Returns the object with the settings used for calls to createBucketAsync. */
public OperationCallSettings
createBucketAsyncOperationSettings() {
return createBucketAsyncOperationSettings;
}
/** Returns the object with the settings used for calls to updateBucketAsync. */
public UnaryCallSettings updateBucketAsyncSettings() {
return updateBucketAsyncSettings;
}
/** Returns the object with the settings used for calls to updateBucketAsync. */
public OperationCallSettings
updateBucketAsyncOperationSettings() {
return updateBucketAsyncOperationSettings;
}
/** Returns the object with the settings used for calls to createBucket. */
public UnaryCallSettings createBucketSettings() {
return createBucketSettings;
}
/** Returns the object with the settings used for calls to updateBucket. */
public UnaryCallSettings updateBucketSettings() {
return updateBucketSettings;
}
/** Returns the object with the settings used for calls to deleteBucket. */
public UnaryCallSettings deleteBucketSettings() {
return deleteBucketSettings;
}
/** Returns the object with the settings used for calls to undeleteBucket. */
public UnaryCallSettings undeleteBucketSettings() {
return undeleteBucketSettings;
}
/** Returns the object with the settings used for calls to listViews. */
public PagedCallSettings
listViewsSettings() {
return listViewsSettings;
}
/** Returns the object with the settings used for calls to getView. */
public UnaryCallSettings getViewSettings() {
return getViewSettings;
}
/** Returns the object with the settings used for calls to createView. */
public UnaryCallSettings createViewSettings() {
return createViewSettings;
}
/** Returns the object with the settings used for calls to updateView. */
public UnaryCallSettings updateViewSettings() {
return updateViewSettings;
}
/** Returns the object with the settings used for calls to deleteView. */
public UnaryCallSettings deleteViewSettings() {
return deleteViewSettings;
}
/** Returns the object with the settings used for calls to listSinks. */
public PagedCallSettings
listSinksSettings() {
return listSinksSettings;
}
/** Returns the object with the settings used for calls to getSink. */
public UnaryCallSettings getSinkSettings() {
return getSinkSettings;
}
/** Returns the object with the settings used for calls to createSink. */
public UnaryCallSettings createSinkSettings() {
return createSinkSettings;
}
/** Returns the object with the settings used for calls to updateSink. */
public UnaryCallSettings updateSinkSettings() {
return updateSinkSettings;
}
/** Returns the object with the settings used for calls to deleteSink. */
public UnaryCallSettings deleteSinkSettings() {
return deleteSinkSettings;
}
/** Returns the object with the settings used for calls to createLink. */
public UnaryCallSettings createLinkSettings() {
return createLinkSettings;
}
/** Returns the object with the settings used for calls to createLink. */
public OperationCallSettings
createLinkOperationSettings() {
return createLinkOperationSettings;
}
/** Returns the object with the settings used for calls to deleteLink. */
public UnaryCallSettings deleteLinkSettings() {
return deleteLinkSettings;
}
/** Returns the object with the settings used for calls to deleteLink. */
public OperationCallSettings
deleteLinkOperationSettings() {
return deleteLinkOperationSettings;
}
/** Returns the object with the settings used for calls to listLinks. */
public PagedCallSettings
listLinksSettings() {
return listLinksSettings;
}
/** Returns the object with the settings used for calls to getLink. */
public UnaryCallSettings getLinkSettings() {
return getLinkSettings;
}
/** Returns the object with the settings used for calls to listExclusions. */
public PagedCallSettings<
ListExclusionsRequest, ListExclusionsResponse, ListExclusionsPagedResponse>
listExclusionsSettings() {
return listExclusionsSettings;
}
/** Returns the object with the settings used for calls to getExclusion. */
public UnaryCallSettings getExclusionSettings() {
return getExclusionSettings;
}
/** Returns the object with the settings used for calls to createExclusion. */
public UnaryCallSettings createExclusionSettings() {
return createExclusionSettings;
}
/** Returns the object with the settings used for calls to updateExclusion. */
public UnaryCallSettings updateExclusionSettings() {
return updateExclusionSettings;
}
/** Returns the object with the settings used for calls to deleteExclusion. */
public UnaryCallSettings deleteExclusionSettings() {
return deleteExclusionSettings;
}
/** Returns the object with the settings used for calls to getCmekSettings. */
public UnaryCallSettings getCmekSettingsSettings() {
return getCmekSettingsSettings;
}
/** Returns the object with the settings used for calls to updateCmekSettings. */
public UnaryCallSettings updateCmekSettingsSettings() {
return updateCmekSettingsSettings;
}
/** Returns the object with the settings used for calls to getSettings. */
public UnaryCallSettings getSettingsSettings() {
return getSettingsSettings;
}
/** Returns the object with the settings used for calls to updateSettings. */
public UnaryCallSettings updateSettingsSettings() {
return updateSettingsSettings;
}
/** Returns the object with the settings used for calls to copyLogEntries. */
public UnaryCallSettings copyLogEntriesSettings() {
return copyLogEntriesSettings;
}
/** Returns the object with the settings used for calls to copyLogEntries. */
public OperationCallSettings<
CopyLogEntriesRequest, CopyLogEntriesResponse, CopyLogEntriesMetadata>
copyLogEntriesOperationSettings() {
return copyLogEntriesOperationSettings;
}
public ConfigServiceV2Stub createStub() throws IOException {
if (getTransportChannelProvider()
.getTransportName()
.equals(GrpcTransportChannel.getGrpcTransportName())) {
return GrpcConfigServiceV2Stub.create(this);
}
throw new UnsupportedOperationException(
String.format(
"Transport not supported: %s", getTransportChannelProvider().getTransportName()));
}
/** Returns the endpoint set by the user or the the service's default endpoint. */
@Override
public String getEndpoint() {
if (super.getEndpoint() != null) {
return super.getEndpoint();
}
return getDefaultEndpoint();
}
/** Returns the default service name. */
@Override
public String getServiceName() {
return "logging";
}
/** Returns a builder for the default ExecutorProvider for this service. */
public static InstantiatingExecutorProvider.Builder defaultExecutorProviderBuilder() {
return InstantiatingExecutorProvider.newBuilder();
}
/** Returns the default service endpoint. */
public static String getDefaultEndpoint() {
return "logging.googleapis.com:443";
}
/** Returns the default mTLS service endpoint. */
public static String getDefaultMtlsEndpoint() {
return "logging.mtls.googleapis.com:443";
}
/** Returns the default service scopes. */
public static List getDefaultServiceScopes() {
return DEFAULT_SERVICE_SCOPES;
}
/** Returns a builder for the default credentials for this service. */
public static GoogleCredentialsProvider.Builder defaultCredentialsProviderBuilder() {
return GoogleCredentialsProvider.newBuilder()
.setScopesToApply(DEFAULT_SERVICE_SCOPES)
.setUseJwtAccessWithScope(true);
}
/** Returns a builder for the default ChannelProvider for this service. */
public static InstantiatingGrpcChannelProvider.Builder defaultGrpcTransportProviderBuilder() {
return InstantiatingGrpcChannelProvider.newBuilder()
.setMaxInboundMessageSize(Integer.MAX_VALUE);
}
public static TransportChannelProvider defaultTransportChannelProvider() {
return defaultGrpcTransportProviderBuilder().build();
}
public static ApiClientHeaderProvider.Builder defaultApiClientHeaderProviderBuilder() {
return ApiClientHeaderProvider.newBuilder()
.setGeneratedLibToken(
"gapic", GaxProperties.getLibraryVersion(ConfigServiceV2StubSettings.class))
.setTransportToken(
GaxGrpcProperties.getGrpcTokenName(), GaxGrpcProperties.getGrpcVersion());
}
/** Returns a new builder for this class. */
public static Builder newBuilder() {
return Builder.createDefault();
}
/** Returns a new builder for this class. */
public static Builder newBuilder(ClientContext clientContext) {
return new Builder(clientContext);
}
/** Returns a builder containing all the values of this settings class. */
public Builder toBuilder() {
return new Builder(this);
}
protected ConfigServiceV2StubSettings(Builder settingsBuilder) throws IOException {
super(settingsBuilder);
listBucketsSettings = settingsBuilder.listBucketsSettings().build();
getBucketSettings = settingsBuilder.getBucketSettings().build();
createBucketAsyncSettings = settingsBuilder.createBucketAsyncSettings().build();
createBucketAsyncOperationSettings =
settingsBuilder.createBucketAsyncOperationSettings().build();
updateBucketAsyncSettings = settingsBuilder.updateBucketAsyncSettings().build();
updateBucketAsyncOperationSettings =
settingsBuilder.updateBucketAsyncOperationSettings().build();
createBucketSettings = settingsBuilder.createBucketSettings().build();
updateBucketSettings = settingsBuilder.updateBucketSettings().build();
deleteBucketSettings = settingsBuilder.deleteBucketSettings().build();
undeleteBucketSettings = settingsBuilder.undeleteBucketSettings().build();
listViewsSettings = settingsBuilder.listViewsSettings().build();
getViewSettings = settingsBuilder.getViewSettings().build();
createViewSettings = settingsBuilder.createViewSettings().build();
updateViewSettings = settingsBuilder.updateViewSettings().build();
deleteViewSettings = settingsBuilder.deleteViewSettings().build();
listSinksSettings = settingsBuilder.listSinksSettings().build();
getSinkSettings = settingsBuilder.getSinkSettings().build();
createSinkSettings = settingsBuilder.createSinkSettings().build();
updateSinkSettings = settingsBuilder.updateSinkSettings().build();
deleteSinkSettings = settingsBuilder.deleteSinkSettings().build();
createLinkSettings = settingsBuilder.createLinkSettings().build();
createLinkOperationSettings = settingsBuilder.createLinkOperationSettings().build();
deleteLinkSettings = settingsBuilder.deleteLinkSettings().build();
deleteLinkOperationSettings = settingsBuilder.deleteLinkOperationSettings().build();
listLinksSettings = settingsBuilder.listLinksSettings().build();
getLinkSettings = settingsBuilder.getLinkSettings().build();
listExclusionsSettings = settingsBuilder.listExclusionsSettings().build();
getExclusionSettings = settingsBuilder.getExclusionSettings().build();
createExclusionSettings = settingsBuilder.createExclusionSettings().build();
updateExclusionSettings = settingsBuilder.updateExclusionSettings().build();
deleteExclusionSettings = settingsBuilder.deleteExclusionSettings().build();
getCmekSettingsSettings = settingsBuilder.getCmekSettingsSettings().build();
updateCmekSettingsSettings = settingsBuilder.updateCmekSettingsSettings().build();
getSettingsSettings = settingsBuilder.getSettingsSettings().build();
updateSettingsSettings = settingsBuilder.updateSettingsSettings().build();
copyLogEntriesSettings = settingsBuilder.copyLogEntriesSettings().build();
copyLogEntriesOperationSettings = settingsBuilder.copyLogEntriesOperationSettings().build();
}
/** Builder for ConfigServiceV2StubSettings. */
public static class Builder extends StubSettings.Builder {
private final ImmutableList> unaryMethodSettingsBuilders;
private final PagedCallSettings.Builder<
ListBucketsRequest, ListBucketsResponse, ListBucketsPagedResponse>
listBucketsSettings;
private final UnaryCallSettings.Builder getBucketSettings;
private final UnaryCallSettings.Builder
createBucketAsyncSettings;
private final OperationCallSettings.Builder
createBucketAsyncOperationSettings;
private final UnaryCallSettings.Builder
updateBucketAsyncSettings;
private final OperationCallSettings.Builder
updateBucketAsyncOperationSettings;
private final UnaryCallSettings.Builder createBucketSettings;
private final UnaryCallSettings.Builder updateBucketSettings;
private final UnaryCallSettings.Builder deleteBucketSettings;
private final UnaryCallSettings.Builder undeleteBucketSettings;
private final PagedCallSettings.Builder<
ListViewsRequest, ListViewsResponse, ListViewsPagedResponse>
listViewsSettings;
private final UnaryCallSettings.Builder getViewSettings;
private final UnaryCallSettings.Builder createViewSettings;
private final UnaryCallSettings.Builder updateViewSettings;
private final UnaryCallSettings.Builder deleteViewSettings;
private final PagedCallSettings.Builder<
ListSinksRequest, ListSinksResponse, ListSinksPagedResponse>
listSinksSettings;
private final UnaryCallSettings.Builder getSinkSettings;
private final UnaryCallSettings.Builder createSinkSettings;
private final UnaryCallSettings.Builder updateSinkSettings;
private final UnaryCallSettings.Builder deleteSinkSettings;
private final UnaryCallSettings.Builder createLinkSettings;
private final OperationCallSettings.Builder
createLinkOperationSettings;
private final UnaryCallSettings.Builder deleteLinkSettings;
private final OperationCallSettings.Builder
deleteLinkOperationSettings;
private final PagedCallSettings.Builder<
ListLinksRequest, ListLinksResponse, ListLinksPagedResponse>
listLinksSettings;
private final UnaryCallSettings.Builder getLinkSettings;
private final PagedCallSettings.Builder<
ListExclusionsRequest, ListExclusionsResponse, ListExclusionsPagedResponse>
listExclusionsSettings;
private final UnaryCallSettings.Builder getExclusionSettings;
private final UnaryCallSettings.Builder
createExclusionSettings;
private final UnaryCallSettings.Builder
updateExclusionSettings;
private final UnaryCallSettings.Builder deleteExclusionSettings;
private final UnaryCallSettings.Builder
getCmekSettingsSettings;
private final UnaryCallSettings.Builder
updateCmekSettingsSettings;
private final UnaryCallSettings.Builder getSettingsSettings;
private final UnaryCallSettings.Builder updateSettingsSettings;
private final UnaryCallSettings.Builder
copyLogEntriesSettings;
private final OperationCallSettings.Builder<
CopyLogEntriesRequest, CopyLogEntriesResponse, CopyLogEntriesMetadata>
copyLogEntriesOperationSettings;
private static final ImmutableMap>
RETRYABLE_CODE_DEFINITIONS;
static {
ImmutableMap.Builder> definitions =
ImmutableMap.builder();
definitions.put("no_retry_codes", ImmutableSet.copyOf(Lists.newArrayList()));
definitions.put(
"retry_policy_3_codes",
ImmutableSet.copyOf(
Lists.newArrayList(
StatusCode.Code.DEADLINE_EXCEEDED,
StatusCode.Code.INTERNAL,
StatusCode.Code.UNAVAILABLE)));
definitions.put(
"no_retry_4_codes", ImmutableSet.copyOf(Lists.newArrayList()));
RETRYABLE_CODE_DEFINITIONS = definitions.build();
}
private static final ImmutableMap RETRY_PARAM_DEFINITIONS;
static {
ImmutableMap.Builder definitions = ImmutableMap.builder();
RetrySettings settings = null;
settings = RetrySettings.newBuilder().setRpcTimeoutMultiplier(1.0).build();
definitions.put("no_retry_params", settings);
settings =
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(100L))
.setRetryDelayMultiplier(1.3)
.setMaxRetryDelay(Duration.ofMillis(60000L))
.setInitialRpcTimeout(Duration.ofMillis(60000L))
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ofMillis(60000L))
.setTotalTimeout(Duration.ofMillis(60000L))
.build();
definitions.put("retry_policy_3_params", settings);
settings =
RetrySettings.newBuilder()
.setInitialRpcTimeout(Duration.ofMillis(120000L))
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ofMillis(120000L))
.setTotalTimeout(Duration.ofMillis(120000L))
.build();
definitions.put("no_retry_4_params", settings);
RETRY_PARAM_DEFINITIONS = definitions.build();
}
protected Builder() {
this(((ClientContext) null));
}
protected Builder(ClientContext clientContext) {
super(clientContext);
listBucketsSettings = PagedCallSettings.newBuilder(LIST_BUCKETS_PAGE_STR_FACT);
getBucketSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createBucketAsyncSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createBucketAsyncOperationSettings = OperationCallSettings.newBuilder();
updateBucketAsyncSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateBucketAsyncOperationSettings = OperationCallSettings.newBuilder();
createBucketSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateBucketSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteBucketSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
undeleteBucketSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
listViewsSettings = PagedCallSettings.newBuilder(LIST_VIEWS_PAGE_STR_FACT);
getViewSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createViewSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateViewSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteViewSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
listSinksSettings = PagedCallSettings.newBuilder(LIST_SINKS_PAGE_STR_FACT);
getSinkSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createSinkSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateSinkSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteSinkSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createLinkSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createLinkOperationSettings = OperationCallSettings.newBuilder();
deleteLinkSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteLinkOperationSettings = OperationCallSettings.newBuilder();
listLinksSettings = PagedCallSettings.newBuilder(LIST_LINKS_PAGE_STR_FACT);
getLinkSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
listExclusionsSettings = PagedCallSettings.newBuilder(LIST_EXCLUSIONS_PAGE_STR_FACT);
getExclusionSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createExclusionSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateExclusionSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteExclusionSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
getCmekSettingsSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateCmekSettingsSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
getSettingsSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateSettingsSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
copyLogEntriesSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
copyLogEntriesOperationSettings = OperationCallSettings.newBuilder();
unaryMethodSettingsBuilders =
ImmutableList.>of(
listBucketsSettings,
getBucketSettings,
createBucketAsyncSettings,
updateBucketAsyncSettings,
createBucketSettings,
updateBucketSettings,
deleteBucketSettings,
undeleteBucketSettings,
listViewsSettings,
getViewSettings,
createViewSettings,
updateViewSettings,
deleteViewSettings,
listSinksSettings,
getSinkSettings,
createSinkSettings,
updateSinkSettings,
deleteSinkSettings,
createLinkSettings,
deleteLinkSettings,
listLinksSettings,
getLinkSettings,
listExclusionsSettings,
getExclusionSettings,
createExclusionSettings,
updateExclusionSettings,
deleteExclusionSettings,
getCmekSettingsSettings,
updateCmekSettingsSettings,
getSettingsSettings,
updateSettingsSettings,
copyLogEntriesSettings);
initDefaults(this);
}
protected Builder(ConfigServiceV2StubSettings settings) {
super(settings);
listBucketsSettings = settings.listBucketsSettings.toBuilder();
getBucketSettings = settings.getBucketSettings.toBuilder();
createBucketAsyncSettings = settings.createBucketAsyncSettings.toBuilder();
createBucketAsyncOperationSettings = settings.createBucketAsyncOperationSettings.toBuilder();
updateBucketAsyncSettings = settings.updateBucketAsyncSettings.toBuilder();
updateBucketAsyncOperationSettings = settings.updateBucketAsyncOperationSettings.toBuilder();
createBucketSettings = settings.createBucketSettings.toBuilder();
updateBucketSettings = settings.updateBucketSettings.toBuilder();
deleteBucketSettings = settings.deleteBucketSettings.toBuilder();
undeleteBucketSettings = settings.undeleteBucketSettings.toBuilder();
listViewsSettings = settings.listViewsSettings.toBuilder();
getViewSettings = settings.getViewSettings.toBuilder();
createViewSettings = settings.createViewSettings.toBuilder();
updateViewSettings = settings.updateViewSettings.toBuilder();
deleteViewSettings = settings.deleteViewSettings.toBuilder();
listSinksSettings = settings.listSinksSettings.toBuilder();
getSinkSettings = settings.getSinkSettings.toBuilder();
createSinkSettings = settings.createSinkSettings.toBuilder();
updateSinkSettings = settings.updateSinkSettings.toBuilder();
deleteSinkSettings = settings.deleteSinkSettings.toBuilder();
createLinkSettings = settings.createLinkSettings.toBuilder();
createLinkOperationSettings = settings.createLinkOperationSettings.toBuilder();
deleteLinkSettings = settings.deleteLinkSettings.toBuilder();
deleteLinkOperationSettings = settings.deleteLinkOperationSettings.toBuilder();
listLinksSettings = settings.listLinksSettings.toBuilder();
getLinkSettings = settings.getLinkSettings.toBuilder();
listExclusionsSettings = settings.listExclusionsSettings.toBuilder();
getExclusionSettings = settings.getExclusionSettings.toBuilder();
createExclusionSettings = settings.createExclusionSettings.toBuilder();
updateExclusionSettings = settings.updateExclusionSettings.toBuilder();
deleteExclusionSettings = settings.deleteExclusionSettings.toBuilder();
getCmekSettingsSettings = settings.getCmekSettingsSettings.toBuilder();
updateCmekSettingsSettings = settings.updateCmekSettingsSettings.toBuilder();
getSettingsSettings = settings.getSettingsSettings.toBuilder();
updateSettingsSettings = settings.updateSettingsSettings.toBuilder();
copyLogEntriesSettings = settings.copyLogEntriesSettings.toBuilder();
copyLogEntriesOperationSettings = settings.copyLogEntriesOperationSettings.toBuilder();
unaryMethodSettingsBuilders =
ImmutableList.>of(
listBucketsSettings,
getBucketSettings,
createBucketAsyncSettings,
updateBucketAsyncSettings,
createBucketSettings,
updateBucketSettings,
deleteBucketSettings,
undeleteBucketSettings,
listViewsSettings,
getViewSettings,
createViewSettings,
updateViewSettings,
deleteViewSettings,
listSinksSettings,
getSinkSettings,
createSinkSettings,
updateSinkSettings,
deleteSinkSettings,
createLinkSettings,
deleteLinkSettings,
listLinksSettings,
getLinkSettings,
listExclusionsSettings,
getExclusionSettings,
createExclusionSettings,
updateExclusionSettings,
deleteExclusionSettings,
getCmekSettingsSettings,
updateCmekSettingsSettings,
getSettingsSettings,
updateSettingsSettings,
copyLogEntriesSettings);
}
private static Builder createDefault() {
Builder builder = new Builder(((ClientContext) null));
builder.setTransportChannelProvider(defaultTransportChannelProvider());
builder.setCredentialsProvider(defaultCredentialsProviderBuilder().build());
builder.setInternalHeaderProvider(defaultApiClientHeaderProviderBuilder().build());
builder.setMtlsEndpoint(getDefaultMtlsEndpoint());
builder.setSwitchToMtlsEndpointAllowed(true);
return initDefaults(builder);
}
private static Builder initDefaults(Builder builder) {
builder
.listBucketsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.getBucketSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.createBucketAsyncSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.updateBucketAsyncSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.createBucketSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.updateBucketSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.deleteBucketSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.undeleteBucketSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.listViewsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.getViewSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.createViewSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.updateViewSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.deleteViewSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.listSinksSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_3_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_3_params"));
builder
.getSinkSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_3_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_3_params"));
builder
.createSinkSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_4_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_4_params"));
builder
.updateSinkSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_3_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_3_params"));
builder
.deleteSinkSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_3_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_3_params"));
builder
.createLinkSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.deleteLinkSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.listLinksSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.getLinkSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.listExclusionsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_3_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_3_params"));
builder
.getExclusionSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_3_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_3_params"));
builder
.createExclusionSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_4_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_4_params"));
builder
.updateExclusionSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_4_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_4_params"));
builder
.deleteExclusionSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_3_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_3_params"));
builder
.getCmekSettingsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.updateCmekSettingsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.getSettingsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.updateSettingsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.copyLogEntriesSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"));
builder
.createBucketAsyncOperationSettings()
.setInitialCallSettings(
UnaryCallSettings
.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(LogBucket.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(BucketMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.updateBucketAsyncOperationSettings()
.setInitialCallSettings(
UnaryCallSettings
.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(LogBucket.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(BucketMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.createLinkOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"))
.build())
.setResponseTransformer(ProtoOperationTransformers.ResponseTransformer.create(Link.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(LinkMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.deleteLinkOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Empty.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(LinkMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
builder
.copyLogEntriesOperationSettings()
.setInitialCallSettings(
UnaryCallSettings
.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(CopyLogEntriesResponse.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(CopyLogEntriesMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelay(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelay(Duration.ofMillis(45000L))
.setInitialRpcTimeout(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeout(Duration.ZERO)
.setTotalTimeout(Duration.ofMillis(300000L))
.build()));
return builder;
}
/**
* Applies the given settings updater function to all of the unary API methods in this service.
*
* Note: This method does not support applying settings to streaming methods.
*/
public Builder applyToAllUnaryMethods(
ApiFunction, Void> settingsUpdater) {
super.applyToAllUnaryMethods(unaryMethodSettingsBuilders, settingsUpdater);
return this;
}
public ImmutableList> unaryMethodSettingsBuilders() {
return unaryMethodSettingsBuilders;
}
/** Returns the builder for the settings used for calls to listBuckets. */
public PagedCallSettings.Builder<
ListBucketsRequest, ListBucketsResponse, ListBucketsPagedResponse>
listBucketsSettings() {
return listBucketsSettings;
}
/** Returns the builder for the settings used for calls to getBucket. */
public UnaryCallSettings.Builder getBucketSettings() {
return getBucketSettings;
}
/** Returns the builder for the settings used for calls to createBucketAsync. */
public UnaryCallSettings.Builder createBucketAsyncSettings() {
return createBucketAsyncSettings;
}
/** Returns the builder for the settings used for calls to createBucketAsync. */
public OperationCallSettings.Builder
createBucketAsyncOperationSettings() {
return createBucketAsyncOperationSettings;
}
/** Returns the builder for the settings used for calls to updateBucketAsync. */
public UnaryCallSettings.Builder updateBucketAsyncSettings() {
return updateBucketAsyncSettings;
}
/** Returns the builder for the settings used for calls to updateBucketAsync. */
public OperationCallSettings.Builder
updateBucketAsyncOperationSettings() {
return updateBucketAsyncOperationSettings;
}
/** Returns the builder for the settings used for calls to createBucket. */
public UnaryCallSettings.Builder createBucketSettings() {
return createBucketSettings;
}
/** Returns the builder for the settings used for calls to updateBucket. */
public UnaryCallSettings.Builder updateBucketSettings() {
return updateBucketSettings;
}
/** Returns the builder for the settings used for calls to deleteBucket. */
public UnaryCallSettings.Builder deleteBucketSettings() {
return deleteBucketSettings;
}
/** Returns the builder for the settings used for calls to undeleteBucket. */
public UnaryCallSettings.Builder undeleteBucketSettings() {
return undeleteBucketSettings;
}
/** Returns the builder for the settings used for calls to listViews. */
public PagedCallSettings.Builder
listViewsSettings() {
return listViewsSettings;
}
/** Returns the builder for the settings used for calls to getView. */
public UnaryCallSettings.Builder getViewSettings() {
return getViewSettings;
}
/** Returns the builder for the settings used for calls to createView. */
public UnaryCallSettings.Builder createViewSettings() {
return createViewSettings;
}
/** Returns the builder for the settings used for calls to updateView. */
public UnaryCallSettings.Builder updateViewSettings() {
return updateViewSettings;
}
/** Returns the builder for the settings used for calls to deleteView. */
public UnaryCallSettings.Builder deleteViewSettings() {
return deleteViewSettings;
}
/** Returns the builder for the settings used for calls to listSinks. */
public PagedCallSettings.Builder
listSinksSettings() {
return listSinksSettings;
}
/** Returns the builder for the settings used for calls to getSink. */
public UnaryCallSettings.Builder getSinkSettings() {
return getSinkSettings;
}
/** Returns the builder for the settings used for calls to createSink. */
public UnaryCallSettings.Builder createSinkSettings() {
return createSinkSettings;
}
/** Returns the builder for the settings used for calls to updateSink. */
public UnaryCallSettings.Builder updateSinkSettings() {
return updateSinkSettings;
}
/** Returns the builder for the settings used for calls to deleteSink. */
public UnaryCallSettings.Builder deleteSinkSettings() {
return deleteSinkSettings;
}
/** Returns the builder for the settings used for calls to createLink. */
public UnaryCallSettings.Builder createLinkSettings() {
return createLinkSettings;
}
/** Returns the builder for the settings used for calls to createLink. */
public OperationCallSettings.Builder
createLinkOperationSettings() {
return createLinkOperationSettings;
}
/** Returns the builder for the settings used for calls to deleteLink. */
public UnaryCallSettings.Builder deleteLinkSettings() {
return deleteLinkSettings;
}
/** Returns the builder for the settings used for calls to deleteLink. */
public OperationCallSettings.Builder
deleteLinkOperationSettings() {
return deleteLinkOperationSettings;
}
/** Returns the builder for the settings used for calls to listLinks. */
public PagedCallSettings.Builder
listLinksSettings() {
return listLinksSettings;
}
/** Returns the builder for the settings used for calls to getLink. */
public UnaryCallSettings.Builder getLinkSettings() {
return getLinkSettings;
}
/** Returns the builder for the settings used for calls to listExclusions. */
public PagedCallSettings.Builder<
ListExclusionsRequest, ListExclusionsResponse, ListExclusionsPagedResponse>
listExclusionsSettings() {
return listExclusionsSettings;
}
/** Returns the builder for the settings used for calls to getExclusion. */
public UnaryCallSettings.Builder getExclusionSettings() {
return getExclusionSettings;
}
/** Returns the builder for the settings used for calls to createExclusion. */
public UnaryCallSettings.Builder
createExclusionSettings() {
return createExclusionSettings;
}
/** Returns the builder for the settings used for calls to updateExclusion. */
public UnaryCallSettings.Builder
updateExclusionSettings() {
return updateExclusionSettings;
}
/** Returns the builder for the settings used for calls to deleteExclusion. */
public UnaryCallSettings.Builder deleteExclusionSettings() {
return deleteExclusionSettings;
}
/** Returns the builder for the settings used for calls to getCmekSettings. */
public UnaryCallSettings.Builder
getCmekSettingsSettings() {
return getCmekSettingsSettings;
}
/** Returns the builder for the settings used for calls to updateCmekSettings. */
public UnaryCallSettings.Builder
updateCmekSettingsSettings() {
return updateCmekSettingsSettings;
}
/** Returns the builder for the settings used for calls to getSettings. */
public UnaryCallSettings.Builder getSettingsSettings() {
return getSettingsSettings;
}
/** Returns the builder for the settings used for calls to updateSettings. */
public UnaryCallSettings.Builder updateSettingsSettings() {
return updateSettingsSettings;
}
/** Returns the builder for the settings used for calls to copyLogEntries. */
public UnaryCallSettings.Builder copyLogEntriesSettings() {
return copyLogEntriesSettings;
}
/** Returns the builder for the settings used for calls to copyLogEntries. */
public OperationCallSettings.Builder<
CopyLogEntriesRequest, CopyLogEntriesResponse, CopyLogEntriesMetadata>
copyLogEntriesOperationSettings() {
return copyLogEntriesOperationSettings;
}
/** Returns the endpoint set by the user or the the service's default endpoint. */
@Override
public String getEndpoint() {
if (super.getEndpoint() != null) {
return super.getEndpoint();
}
return getDefaultEndpoint();
}
@Override
public ConfigServiceV2StubSettings build() throws IOException {
return new ConfigServiceV2StubSettings(this);
}
}
}