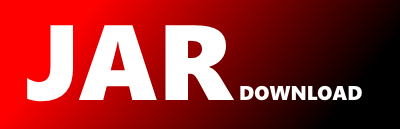
com.google.cloud.logging.v2.stub.GrpcConfigServiceV2Stub Maven / Gradle / Ivy
Show all versions of google-cloud-logging Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.logging.v2.stub;
import static com.google.cloud.logging.v2.ConfigClient.ListBucketsPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListExclusionsPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListLinksPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListSinksPagedResponse;
import static com.google.cloud.logging.v2.ConfigClient.ListViewsPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.logging.v2.BucketMetadata;
import com.google.logging.v2.CmekSettings;
import com.google.logging.v2.CopyLogEntriesMetadata;
import com.google.logging.v2.CopyLogEntriesRequest;
import com.google.logging.v2.CopyLogEntriesResponse;
import com.google.logging.v2.CreateBucketRequest;
import com.google.logging.v2.CreateExclusionRequest;
import com.google.logging.v2.CreateLinkRequest;
import com.google.logging.v2.CreateSinkRequest;
import com.google.logging.v2.CreateViewRequest;
import com.google.logging.v2.DeleteBucketRequest;
import com.google.logging.v2.DeleteExclusionRequest;
import com.google.logging.v2.DeleteLinkRequest;
import com.google.logging.v2.DeleteSinkRequest;
import com.google.logging.v2.DeleteViewRequest;
import com.google.logging.v2.GetBucketRequest;
import com.google.logging.v2.GetCmekSettingsRequest;
import com.google.logging.v2.GetExclusionRequest;
import com.google.logging.v2.GetLinkRequest;
import com.google.logging.v2.GetSettingsRequest;
import com.google.logging.v2.GetSinkRequest;
import com.google.logging.v2.GetViewRequest;
import com.google.logging.v2.Link;
import com.google.logging.v2.LinkMetadata;
import com.google.logging.v2.ListBucketsRequest;
import com.google.logging.v2.ListBucketsResponse;
import com.google.logging.v2.ListExclusionsRequest;
import com.google.logging.v2.ListExclusionsResponse;
import com.google.logging.v2.ListLinksRequest;
import com.google.logging.v2.ListLinksResponse;
import com.google.logging.v2.ListSinksRequest;
import com.google.logging.v2.ListSinksResponse;
import com.google.logging.v2.ListViewsRequest;
import com.google.logging.v2.ListViewsResponse;
import com.google.logging.v2.LogBucket;
import com.google.logging.v2.LogExclusion;
import com.google.logging.v2.LogSink;
import com.google.logging.v2.LogView;
import com.google.logging.v2.Settings;
import com.google.logging.v2.UndeleteBucketRequest;
import com.google.logging.v2.UpdateBucketRequest;
import com.google.logging.v2.UpdateCmekSettingsRequest;
import com.google.logging.v2.UpdateExclusionRequest;
import com.google.logging.v2.UpdateSettingsRequest;
import com.google.logging.v2.UpdateSinkRequest;
import com.google.logging.v2.UpdateViewRequest;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import com.google.protobuf.Empty;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the ConfigServiceV2 service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public class GrpcConfigServiceV2Stub extends ConfigServiceV2Stub {
private static final MethodDescriptor
listBucketsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/ListBuckets")
.setRequestMarshaller(ProtoUtils.marshaller(ListBucketsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListBucketsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getBucketMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/GetBucket")
.setRequestMarshaller(ProtoUtils.marshaller(GetBucketRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogBucket.getDefaultInstance()))
.build();
private static final MethodDescriptor
createBucketAsyncMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/CreateBucketAsync")
.setRequestMarshaller(ProtoUtils.marshaller(CreateBucketRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateBucketAsyncMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/UpdateBucketAsync")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateBucketRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
createBucketMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/CreateBucket")
.setRequestMarshaller(ProtoUtils.marshaller(CreateBucketRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogBucket.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateBucketMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/UpdateBucket")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateBucketRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogBucket.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteBucketMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/DeleteBucket")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteBucketRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
undeleteBucketMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/UndeleteBucket")
.setRequestMarshaller(
ProtoUtils.marshaller(UndeleteBucketRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
listViewsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/ListViews")
.setRequestMarshaller(ProtoUtils.marshaller(ListViewsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListViewsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getViewMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/GetView")
.setRequestMarshaller(ProtoUtils.marshaller(GetViewRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogView.getDefaultInstance()))
.build();
private static final MethodDescriptor createViewMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/CreateView")
.setRequestMarshaller(ProtoUtils.marshaller(CreateViewRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogView.getDefaultInstance()))
.build();
private static final MethodDescriptor updateViewMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/UpdateView")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateViewRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogView.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteViewMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/DeleteView")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteViewRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
listSinksMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/ListSinks")
.setRequestMarshaller(ProtoUtils.marshaller(ListSinksRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListSinksResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getSinkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/GetSink")
.setRequestMarshaller(ProtoUtils.marshaller(GetSinkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogSink.getDefaultInstance()))
.build();
private static final MethodDescriptor createSinkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/CreateSink")
.setRequestMarshaller(ProtoUtils.marshaller(CreateSinkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogSink.getDefaultInstance()))
.build();
private static final MethodDescriptor updateSinkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/UpdateSink")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateSinkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogSink.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteSinkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/DeleteSink")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteSinkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor createLinkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/CreateLink")
.setRequestMarshaller(ProtoUtils.marshaller(CreateLinkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor deleteLinkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/DeleteLink")
.setRequestMarshaller(ProtoUtils.marshaller(DeleteLinkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listLinksMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/ListLinks")
.setRequestMarshaller(ProtoUtils.marshaller(ListLinksRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(ListLinksResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getLinkMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/GetLink")
.setRequestMarshaller(ProtoUtils.marshaller(GetLinkRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Link.getDefaultInstance()))
.build();
private static final MethodDescriptor
listExclusionsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/ListExclusions")
.setRequestMarshaller(
ProtoUtils.marshaller(ListExclusionsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListExclusionsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getExclusionMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/GetExclusion")
.setRequestMarshaller(ProtoUtils.marshaller(GetExclusionRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogExclusion.getDefaultInstance()))
.build();
private static final MethodDescriptor
createExclusionMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/CreateExclusion")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateExclusionRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogExclusion.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateExclusionMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/UpdateExclusion")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateExclusionRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(LogExclusion.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteExclusionMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/DeleteExclusion")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteExclusionRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Empty.getDefaultInstance()))
.build();
private static final MethodDescriptor
getCmekSettingsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/GetCmekSettings")
.setRequestMarshaller(
ProtoUtils.marshaller(GetCmekSettingsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(CmekSettings.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateCmekSettingsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/UpdateCmekSettings")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateCmekSettingsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(CmekSettings.getDefaultInstance()))
.build();
private static final MethodDescriptor getSettingsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/GetSettings")
.setRequestMarshaller(ProtoUtils.marshaller(GetSettingsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Settings.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateSettingsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/UpdateSettings")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateSettingsRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Settings.getDefaultInstance()))
.build();
private static final MethodDescriptor
copyLogEntriesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.logging.v2.ConfigServiceV2/CopyLogEntries")
.setRequestMarshaller(
ProtoUtils.marshaller(CopyLogEntriesRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private final UnaryCallable listBucketsCallable;
private final UnaryCallable
listBucketsPagedCallable;
private final UnaryCallable getBucketCallable;
private final UnaryCallable createBucketAsyncCallable;
private final OperationCallable
createBucketAsyncOperationCallable;
private final UnaryCallable updateBucketAsyncCallable;
private final OperationCallable
updateBucketAsyncOperationCallable;
private final UnaryCallable createBucketCallable;
private final UnaryCallable updateBucketCallable;
private final UnaryCallable deleteBucketCallable;
private final UnaryCallable undeleteBucketCallable;
private final UnaryCallable listViewsCallable;
private final UnaryCallable listViewsPagedCallable;
private final UnaryCallable getViewCallable;
private final UnaryCallable createViewCallable;
private final UnaryCallable updateViewCallable;
private final UnaryCallable deleteViewCallable;
private final UnaryCallable listSinksCallable;
private final UnaryCallable listSinksPagedCallable;
private final UnaryCallable getSinkCallable;
private final UnaryCallable createSinkCallable;
private final UnaryCallable updateSinkCallable;
private final UnaryCallable deleteSinkCallable;
private final UnaryCallable createLinkCallable;
private final OperationCallable
createLinkOperationCallable;
private final UnaryCallable deleteLinkCallable;
private final OperationCallable
deleteLinkOperationCallable;
private final UnaryCallable listLinksCallable;
private final UnaryCallable listLinksPagedCallable;
private final UnaryCallable getLinkCallable;
private final UnaryCallable listExclusionsCallable;
private final UnaryCallable
listExclusionsPagedCallable;
private final UnaryCallable getExclusionCallable;
private final UnaryCallable createExclusionCallable;
private final UnaryCallable updateExclusionCallable;
private final UnaryCallable deleteExclusionCallable;
private final UnaryCallable getCmekSettingsCallable;
private final UnaryCallable updateCmekSettingsCallable;
private final UnaryCallable getSettingsCallable;
private final UnaryCallable updateSettingsCallable;
private final UnaryCallable copyLogEntriesCallable;
private final OperationCallable<
CopyLogEntriesRequest, CopyLogEntriesResponse, CopyLogEntriesMetadata>
copyLogEntriesOperationCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcConfigServiceV2Stub create(ConfigServiceV2StubSettings settings)
throws IOException {
return new GrpcConfigServiceV2Stub(settings, ClientContext.create(settings));
}
public static final GrpcConfigServiceV2Stub create(ClientContext clientContext)
throws IOException {
return new GrpcConfigServiceV2Stub(
ConfigServiceV2StubSettings.newBuilder().build(), clientContext);
}
public static final GrpcConfigServiceV2Stub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcConfigServiceV2Stub(
ConfigServiceV2StubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcConfigServiceV2Stub, using the given settings. This is protected
* so that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcConfigServiceV2Stub(
ConfigServiceV2StubSettings settings, ClientContext clientContext) throws IOException {
this(settings, clientContext, new GrpcConfigServiceV2CallableFactory());
}
/**
* Constructs an instance of GrpcConfigServiceV2Stub, using the given settings. This is protected
* so that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected GrpcConfigServiceV2Stub(
ConfigServiceV2StubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings listBucketsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listBucketsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getBucketTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getBucketMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createBucketAsyncTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createBucketAsyncMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateBucketAsyncTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateBucketAsyncMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createBucketTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createBucketMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateBucketTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateBucketMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteBucketTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteBucketMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings undeleteBucketTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(undeleteBucketMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listViewsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listViewsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getViewTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getViewMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createViewTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createViewMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateViewTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateViewMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteViewTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteViewMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listSinksTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listSinksMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getSinkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getSinkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("sink_name", String.valueOf(request.getSinkName()));
return builder.build();
})
.build();
GrpcCallSettings createSinkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createSinkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateSinkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateSinkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("sink_name", String.valueOf(request.getSinkName()));
return builder.build();
})
.build();
GrpcCallSettings deleteSinkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteSinkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("sink_name", String.valueOf(request.getSinkName()));
return builder.build();
})
.build();
GrpcCallSettings createLinkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createLinkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings deleteLinkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteLinkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listLinksTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listLinksMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getLinkTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getLinkMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
listExclusionsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listExclusionsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getExclusionTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getExclusionMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createExclusionTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createExclusionMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateExclusionTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateExclusionMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteExclusionTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteExclusionMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getCmekSettingsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getCmekSettingsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateCmekSettingsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateCmekSettingsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getSettingsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getSettingsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateSettingsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateSettingsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings copyLogEntriesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(copyLogEntriesMethodDescriptor)
.build();
this.listBucketsCallable =
callableFactory.createUnaryCallable(
listBucketsTransportSettings, settings.listBucketsSettings(), clientContext);
this.listBucketsPagedCallable =
callableFactory.createPagedCallable(
listBucketsTransportSettings, settings.listBucketsSettings(), clientContext);
this.getBucketCallable =
callableFactory.createUnaryCallable(
getBucketTransportSettings, settings.getBucketSettings(), clientContext);
this.createBucketAsyncCallable =
callableFactory.createUnaryCallable(
createBucketAsyncTransportSettings,
settings.createBucketAsyncSettings(),
clientContext);
this.createBucketAsyncOperationCallable =
callableFactory.createOperationCallable(
createBucketAsyncTransportSettings,
settings.createBucketAsyncOperationSettings(),
clientContext,
operationsStub);
this.updateBucketAsyncCallable =
callableFactory.createUnaryCallable(
updateBucketAsyncTransportSettings,
settings.updateBucketAsyncSettings(),
clientContext);
this.updateBucketAsyncOperationCallable =
callableFactory.createOperationCallable(
updateBucketAsyncTransportSettings,
settings.updateBucketAsyncOperationSettings(),
clientContext,
operationsStub);
this.createBucketCallable =
callableFactory.createUnaryCallable(
createBucketTransportSettings, settings.createBucketSettings(), clientContext);
this.updateBucketCallable =
callableFactory.createUnaryCallable(
updateBucketTransportSettings, settings.updateBucketSettings(), clientContext);
this.deleteBucketCallable =
callableFactory.createUnaryCallable(
deleteBucketTransportSettings, settings.deleteBucketSettings(), clientContext);
this.undeleteBucketCallable =
callableFactory.createUnaryCallable(
undeleteBucketTransportSettings, settings.undeleteBucketSettings(), clientContext);
this.listViewsCallable =
callableFactory.createUnaryCallable(
listViewsTransportSettings, settings.listViewsSettings(), clientContext);
this.listViewsPagedCallable =
callableFactory.createPagedCallable(
listViewsTransportSettings, settings.listViewsSettings(), clientContext);
this.getViewCallable =
callableFactory.createUnaryCallable(
getViewTransportSettings, settings.getViewSettings(), clientContext);
this.createViewCallable =
callableFactory.createUnaryCallable(
createViewTransportSettings, settings.createViewSettings(), clientContext);
this.updateViewCallable =
callableFactory.createUnaryCallable(
updateViewTransportSettings, settings.updateViewSettings(), clientContext);
this.deleteViewCallable =
callableFactory.createUnaryCallable(
deleteViewTransportSettings, settings.deleteViewSettings(), clientContext);
this.listSinksCallable =
callableFactory.createUnaryCallable(
listSinksTransportSettings, settings.listSinksSettings(), clientContext);
this.listSinksPagedCallable =
callableFactory.createPagedCallable(
listSinksTransportSettings, settings.listSinksSettings(), clientContext);
this.getSinkCallable =
callableFactory.createUnaryCallable(
getSinkTransportSettings, settings.getSinkSettings(), clientContext);
this.createSinkCallable =
callableFactory.createUnaryCallable(
createSinkTransportSettings, settings.createSinkSettings(), clientContext);
this.updateSinkCallable =
callableFactory.createUnaryCallable(
updateSinkTransportSettings, settings.updateSinkSettings(), clientContext);
this.deleteSinkCallable =
callableFactory.createUnaryCallable(
deleteSinkTransportSettings, settings.deleteSinkSettings(), clientContext);
this.createLinkCallable =
callableFactory.createUnaryCallable(
createLinkTransportSettings, settings.createLinkSettings(), clientContext);
this.createLinkOperationCallable =
callableFactory.createOperationCallable(
createLinkTransportSettings,
settings.createLinkOperationSettings(),
clientContext,
operationsStub);
this.deleteLinkCallable =
callableFactory.createUnaryCallable(
deleteLinkTransportSettings, settings.deleteLinkSettings(), clientContext);
this.deleteLinkOperationCallable =
callableFactory.createOperationCallable(
deleteLinkTransportSettings,
settings.deleteLinkOperationSettings(),
clientContext,
operationsStub);
this.listLinksCallable =
callableFactory.createUnaryCallable(
listLinksTransportSettings, settings.listLinksSettings(), clientContext);
this.listLinksPagedCallable =
callableFactory.createPagedCallable(
listLinksTransportSettings, settings.listLinksSettings(), clientContext);
this.getLinkCallable =
callableFactory.createUnaryCallable(
getLinkTransportSettings, settings.getLinkSettings(), clientContext);
this.listExclusionsCallable =
callableFactory.createUnaryCallable(
listExclusionsTransportSettings, settings.listExclusionsSettings(), clientContext);
this.listExclusionsPagedCallable =
callableFactory.createPagedCallable(
listExclusionsTransportSettings, settings.listExclusionsSettings(), clientContext);
this.getExclusionCallable =
callableFactory.createUnaryCallable(
getExclusionTransportSettings, settings.getExclusionSettings(), clientContext);
this.createExclusionCallable =
callableFactory.createUnaryCallable(
createExclusionTransportSettings, settings.createExclusionSettings(), clientContext);
this.updateExclusionCallable =
callableFactory.createUnaryCallable(
updateExclusionTransportSettings, settings.updateExclusionSettings(), clientContext);
this.deleteExclusionCallable =
callableFactory.createUnaryCallable(
deleteExclusionTransportSettings, settings.deleteExclusionSettings(), clientContext);
this.getCmekSettingsCallable =
callableFactory.createUnaryCallable(
getCmekSettingsTransportSettings, settings.getCmekSettingsSettings(), clientContext);
this.updateCmekSettingsCallable =
callableFactory.createUnaryCallable(
updateCmekSettingsTransportSettings,
settings.updateCmekSettingsSettings(),
clientContext);
this.getSettingsCallable =
callableFactory.createUnaryCallable(
getSettingsTransportSettings, settings.getSettingsSettings(), clientContext);
this.updateSettingsCallable =
callableFactory.createUnaryCallable(
updateSettingsTransportSettings, settings.updateSettingsSettings(), clientContext);
this.copyLogEntriesCallable =
callableFactory.createUnaryCallable(
copyLogEntriesTransportSettings, settings.copyLogEntriesSettings(), clientContext);
this.copyLogEntriesOperationCallable =
callableFactory.createOperationCallable(
copyLogEntriesTransportSettings,
settings.copyLogEntriesOperationSettings(),
clientContext,
operationsStub);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable listBucketsCallable() {
return listBucketsCallable;
}
@Override
public UnaryCallable listBucketsPagedCallable() {
return listBucketsPagedCallable;
}
@Override
public UnaryCallable getBucketCallable() {
return getBucketCallable;
}
@Override
public UnaryCallable createBucketAsyncCallable() {
return createBucketAsyncCallable;
}
@Override
public OperationCallable
createBucketAsyncOperationCallable() {
return createBucketAsyncOperationCallable;
}
@Override
public UnaryCallable updateBucketAsyncCallable() {
return updateBucketAsyncCallable;
}
@Override
public OperationCallable
updateBucketAsyncOperationCallable() {
return updateBucketAsyncOperationCallable;
}
@Override
public UnaryCallable createBucketCallable() {
return createBucketCallable;
}
@Override
public UnaryCallable updateBucketCallable() {
return updateBucketCallable;
}
@Override
public UnaryCallable deleteBucketCallable() {
return deleteBucketCallable;
}
@Override
public UnaryCallable undeleteBucketCallable() {
return undeleteBucketCallable;
}
@Override
public UnaryCallable listViewsCallable() {
return listViewsCallable;
}
@Override
public UnaryCallable listViewsPagedCallable() {
return listViewsPagedCallable;
}
@Override
public UnaryCallable getViewCallable() {
return getViewCallable;
}
@Override
public UnaryCallable createViewCallable() {
return createViewCallable;
}
@Override
public UnaryCallable updateViewCallable() {
return updateViewCallable;
}
@Override
public UnaryCallable deleteViewCallable() {
return deleteViewCallable;
}
@Override
public UnaryCallable listSinksCallable() {
return listSinksCallable;
}
@Override
public UnaryCallable listSinksPagedCallable() {
return listSinksPagedCallable;
}
@Override
public UnaryCallable getSinkCallable() {
return getSinkCallable;
}
@Override
public UnaryCallable createSinkCallable() {
return createSinkCallable;
}
@Override
public UnaryCallable updateSinkCallable() {
return updateSinkCallable;
}
@Override
public UnaryCallable deleteSinkCallable() {
return deleteSinkCallable;
}
@Override
public UnaryCallable createLinkCallable() {
return createLinkCallable;
}
@Override
public OperationCallable createLinkOperationCallable() {
return createLinkOperationCallable;
}
@Override
public UnaryCallable deleteLinkCallable() {
return deleteLinkCallable;
}
@Override
public OperationCallable deleteLinkOperationCallable() {
return deleteLinkOperationCallable;
}
@Override
public UnaryCallable listLinksCallable() {
return listLinksCallable;
}
@Override
public UnaryCallable listLinksPagedCallable() {
return listLinksPagedCallable;
}
@Override
public UnaryCallable getLinkCallable() {
return getLinkCallable;
}
@Override
public UnaryCallable listExclusionsCallable() {
return listExclusionsCallable;
}
@Override
public UnaryCallable
listExclusionsPagedCallable() {
return listExclusionsPagedCallable;
}
@Override
public UnaryCallable getExclusionCallable() {
return getExclusionCallable;
}
@Override
public UnaryCallable createExclusionCallable() {
return createExclusionCallable;
}
@Override
public UnaryCallable updateExclusionCallable() {
return updateExclusionCallable;
}
@Override
public UnaryCallable deleteExclusionCallable() {
return deleteExclusionCallable;
}
@Override
public UnaryCallable getCmekSettingsCallable() {
return getCmekSettingsCallable;
}
@Override
public UnaryCallable updateCmekSettingsCallable() {
return updateCmekSettingsCallable;
}
@Override
public UnaryCallable getSettingsCallable() {
return getSettingsCallable;
}
@Override
public UnaryCallable updateSettingsCallable() {
return updateSettingsCallable;
}
@Override
public UnaryCallable copyLogEntriesCallable() {
return copyLogEntriesCallable;
}
@Override
public OperationCallable
copyLogEntriesOperationCallable() {
return copyLogEntriesOperationCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}