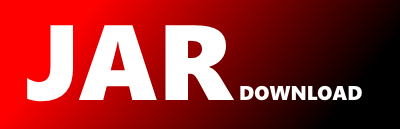
com.google.cloud.networkconnectivity.v1.HubServiceClient Maven / Gradle / Ivy
Show all versions of google-cloud-networkconnectivity Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.networkconnectivity.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.networkconnectivity.v1.stub.HubServiceStub;
import com.google.cloud.networkconnectivity.v1.stub.HubServiceStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.longrunning.OperationsClient;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Network Connectivity Center is a hub-and-spoke abstraction for network
* connectivity management in Google Cloud. It reduces operational complexity through a simple,
* centralized connectivity management model.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName name = HubName.of("[PROJECT]", "[HUB]");
* Hub response = hubServiceClient.getHub(name);
* }
* }
*
* Note: close() needs to be called on the HubServiceClient object to clean up resources such as
* threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* ListHubs
* Lists the Network Connectivity Center hubs associated with a given project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listHubs(ListHubsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listHubs(LocationName parent)
*
listHubs(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listHubsPagedCallable()
*
listHubsCallable()
*
*
*
*
* GetHub
* Gets details about a Network Connectivity Center hub.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getHub(GetHubRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getHub(HubName name)
*
getHub(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getHubCallable()
*
*
*
*
* CreateHub
* Creates a new Network Connectivity Center hub in the specified project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createHubAsync(CreateHubRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createHubAsync(LocationName parent, Hub hub, String hubId)
*
createHubAsync(String parent, Hub hub, String hubId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createHubOperationCallable()
*
createHubCallable()
*
*
*
*
* UpdateHub
* Updates the description and/or labels of a Network Connectivity Center hub.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateHubAsync(UpdateHubRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateHubAsync(Hub hub, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateHubOperationCallable()
*
updateHubCallable()
*
*
*
*
* DeleteHub
* Deletes a Network Connectivity Center hub.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteHubAsync(DeleteHubRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteHubAsync(HubName name)
*
deleteHubAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteHubOperationCallable()
*
deleteHubCallable()
*
*
*
*
* ListHubSpokes
* Lists the Network Connectivity Center spokes associated with a specified hub and location. The list includes both spokes that are attached to the hub and spokes that have been proposed but not yet accepted.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listHubSpokes(ListHubSpokesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listHubSpokes(HubName name)
*
listHubSpokes(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listHubSpokesPagedCallable()
*
listHubSpokesCallable()
*
*
*
*
* QueryHubStatus
* Query the Private Service Connect propagation status of a Network Connectivity Center hub.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* queryHubStatus(QueryHubStatusRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* queryHubStatus(HubName name)
*
queryHubStatus(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* queryHubStatusPagedCallable()
*
queryHubStatusCallable()
*
*
*
*
* ListSpokes
* Lists the Network Connectivity Center spokes in a specified project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listSpokes(ListSpokesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listSpokes(LocationName parent)
*
listSpokes(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listSpokesPagedCallable()
*
listSpokesCallable()
*
*
*
*
* GetSpoke
* Gets details about a Network Connectivity Center spoke.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getSpoke(GetSpokeRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getSpoke(SpokeName name)
*
getSpoke(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getSpokeCallable()
*
*
*
*
* CreateSpoke
* Creates a Network Connectivity Center spoke.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createSpokeAsync(CreateSpokeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createSpokeAsync(LocationName parent, Spoke spoke, String spokeId)
*
createSpokeAsync(String parent, Spoke spoke, String spokeId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createSpokeOperationCallable()
*
createSpokeCallable()
*
*
*
*
* UpdateSpoke
* Updates the parameters of a Network Connectivity Center spoke.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateSpokeAsync(UpdateSpokeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateSpokeAsync(Spoke spoke, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateSpokeOperationCallable()
*
updateSpokeCallable()
*
*
*
*
* RejectHubSpoke
* Rejects a Network Connectivity Center spoke from being attached to a hub. If the spoke was previously in the `ACTIVE` state, it transitions to the `INACTIVE` state and is no longer able to connect to other spokes that are attached to the hub.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* rejectHubSpokeAsync(RejectHubSpokeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* rejectHubSpokeAsync(HubName name, SpokeName spokeUri)
*
rejectHubSpokeAsync(HubName name, String spokeUri)
*
rejectHubSpokeAsync(String name, SpokeName spokeUri)
*
rejectHubSpokeAsync(String name, String spokeUri)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* rejectHubSpokeOperationCallable()
*
rejectHubSpokeCallable()
*
*
*
*
* AcceptHubSpoke
* Accepts a proposal to attach a Network Connectivity Center spoke to a hub.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* acceptHubSpokeAsync(AcceptHubSpokeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* acceptHubSpokeAsync(HubName name, SpokeName spokeUri)
*
acceptHubSpokeAsync(HubName name, String spokeUri)
*
acceptHubSpokeAsync(String name, SpokeName spokeUri)
*
acceptHubSpokeAsync(String name, String spokeUri)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* acceptHubSpokeOperationCallable()
*
acceptHubSpokeCallable()
*
*
*
*
* DeleteSpoke
* Deletes a Network Connectivity Center spoke.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteSpokeAsync(DeleteSpokeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteSpokeAsync(SpokeName name)
*
deleteSpokeAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteSpokeOperationCallable()
*
deleteSpokeCallable()
*
*
*
*
* GetRouteTable
* Gets details about a Network Connectivity Center route table.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getRouteTable(GetRouteTableRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getRouteTable(RouteTableName name)
*
getRouteTable(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getRouteTableCallable()
*
*
*
*
* GetRoute
* Gets details about the specified route.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getRoute(GetRouteRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getRoute(HubRouteName name)
*
getRoute(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getRouteCallable()
*
*
*
*
* ListRoutes
* Lists routes in a given route table.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listRoutes(ListRoutesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listRoutes(RouteTableName parent)
*
listRoutes(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listRoutesPagedCallable()
*
listRoutesCallable()
*
*
*
*
* ListRouteTables
* Lists route tables in a given hub.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listRouteTables(ListRouteTablesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listRouteTables(HubName parent)
*
listRouteTables(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listRouteTablesPagedCallable()
*
listRouteTablesCallable()
*
*
*
*
* GetGroup
* Gets details about a Network Connectivity Center group.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getGroup(GetGroupRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getGroup(GroupName name)
*
getGroup(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getGroupCallable()
*
*
*
*
* ListGroups
* Lists groups in a given hub.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listGroups(ListGroupsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listGroups(HubName parent)
*
listGroups(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listGroupsPagedCallable()
*
listGroupsCallable()
*
*
*
*
* UpdateGroup
* Updates the parameters of a Network Connectivity Center group.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateGroupAsync(UpdateGroupRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateGroupAsync(Group group, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateGroupOperationCallable()
*
updateGroupCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listLocationsPagedCallable()
*
listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getLocationCallable()
*
*
*
*
* SetIamPolicy
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* setIamPolicy(SetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* setIamPolicyCallable()
*
*
*
*
* GetIamPolicy
* Gets the access control policy for a resource. Returns an empty policyif the resource exists and does not have a policy set.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getIamPolicy(GetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getIamPolicyCallable()
*
*
*
*
* TestIamPermissions
* Returns permissions that a caller has on the specified resource. If theresource does not exist, this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
Note: This operation is designed to be used for buildingpermission-aware UIs and command-line tools, not for authorizationchecking. This operation may "fail open" without warning.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* testIamPermissions(TestIamPermissionsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* testIamPermissionsCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of HubServiceSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* HubServiceSettings hubServiceSettings =
* HubServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* HubServiceClient hubServiceClient = HubServiceClient.create(hubServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* HubServiceSettings hubServiceSettings =
* HubServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* HubServiceClient hubServiceClient = HubServiceClient.create(hubServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class HubServiceClient implements BackgroundResource {
private final HubServiceSettings settings;
private final HubServiceStub stub;
private final OperationsClient operationsClient;
/** Constructs an instance of HubServiceClient with default settings. */
public static final HubServiceClient create() throws IOException {
return create(HubServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of HubServiceClient, using the given settings. The channels are created
* based on the settings passed in, or defaults for any settings that are not set.
*/
public static final HubServiceClient create(HubServiceSettings settings) throws IOException {
return new HubServiceClient(settings);
}
/**
* Constructs an instance of HubServiceClient, using the given stub for making calls. This is for
* advanced usage - prefer using create(HubServiceSettings).
*/
public static final HubServiceClient create(HubServiceStub stub) {
return new HubServiceClient(stub);
}
/**
* Constructs an instance of HubServiceClient, using the given settings. This is protected so that
* it is easy to make a subclass, but otherwise, the static factory methods should be preferred.
*/
protected HubServiceClient(HubServiceSettings settings) throws IOException {
this.settings = settings;
this.stub = ((HubServiceStubSettings) settings.getStubSettings()).createStub();
this.operationsClient = OperationsClient.create(this.stub.getOperationsStub());
}
protected HubServiceClient(HubServiceStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient = OperationsClient.create(this.stub.getOperationsStub());
}
public final HubServiceSettings getSettings() {
return settings;
}
public HubServiceStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final OperationsClient getOperationsClient() {
return operationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center hubs associated with a given project.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Hub element : hubServiceClient.listHubs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource's name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListHubsPagedResponse listHubs(LocationName parent) {
ListHubsRequest request =
ListHubsRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listHubs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center hubs associated with a given project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Hub element : hubServiceClient.listHubs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource's name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListHubsPagedResponse listHubs(String parent) {
ListHubsRequest request = ListHubsRequest.newBuilder().setParent(parent).build();
return listHubs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center hubs associated with a given project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListHubsRequest request =
* ListHubsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Hub element : hubServiceClient.listHubs(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListHubsPagedResponse listHubs(ListHubsRequest request) {
return listHubsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center hubs associated with a given project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListHubsRequest request =
* ListHubsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future = hubServiceClient.listHubsPagedCallable().futureCall(request);
* // Do something.
* for (Hub element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listHubsPagedCallable() {
return stub.listHubsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center hubs associated with a given project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListHubsRequest request =
* ListHubsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListHubsResponse response = hubServiceClient.listHubsCallable().call(request);
* for (Hub element : response.getHubsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listHubsCallable() {
return stub.listHubsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName name = HubName.of("[PROJECT]", "[HUB]");
* Hub response = hubServiceClient.getHub(name);
* }
* }
*
* @param name Required. The name of the hub resource to get.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Hub getHub(HubName name) {
GetHubRequest request =
GetHubRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getHub(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = HubName.of("[PROJECT]", "[HUB]").toString();
* Hub response = hubServiceClient.getHub(name);
* }
* }
*
* @param name Required. The name of the hub resource to get.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Hub getHub(String name) {
GetHubRequest request = GetHubRequest.newBuilder().setName(name).build();
return getHub(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetHubRequest request =
* GetHubRequest.newBuilder().setName(HubName.of("[PROJECT]", "[HUB]").toString()).build();
* Hub response = hubServiceClient.getHub(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Hub getHub(GetHubRequest request) {
return getHubCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetHubRequest request =
* GetHubRequest.newBuilder().setName(HubName.of("[PROJECT]", "[HUB]").toString()).build();
* ApiFuture future = hubServiceClient.getHubCallable().futureCall(request);
* // Do something.
* Hub response = future.get();
* }
* }
*/
public final UnaryCallable getHubCallable() {
return stub.getHubCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Network Connectivity Center hub in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Hub hub = Hub.newBuilder().build();
* String hubId = "hubId99628272";
* Hub response = hubServiceClient.createHubAsync(parent, hub, hubId).get();
* }
* }
*
* @param parent Required. The parent resource.
* @param hub Required. The initial values for a new hub.
* @param hubId Required. A unique identifier for the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createHubAsync(
LocationName parent, Hub hub, String hubId) {
CreateHubRequest request =
CreateHubRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setHub(hub)
.setHubId(hubId)
.build();
return createHubAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Network Connectivity Center hub in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Hub hub = Hub.newBuilder().build();
* String hubId = "hubId99628272";
* Hub response = hubServiceClient.createHubAsync(parent, hub, hubId).get();
* }
* }
*
* @param parent Required. The parent resource.
* @param hub Required. The initial values for a new hub.
* @param hubId Required. A unique identifier for the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createHubAsync(
String parent, Hub hub, String hubId) {
CreateHubRequest request =
CreateHubRequest.newBuilder().setParent(parent).setHub(hub).setHubId(hubId).build();
return createHubAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Network Connectivity Center hub in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* CreateHubRequest request =
* CreateHubRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setHubId("hubId99628272")
* .setHub(Hub.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Hub response = hubServiceClient.createHubAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createHubAsync(CreateHubRequest request) {
return createHubOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Network Connectivity Center hub in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* CreateHubRequest request =
* CreateHubRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setHubId("hubId99628272")
* .setHub(Hub.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* hubServiceClient.createHubOperationCallable().futureCall(request);
* // Do something.
* Hub response = future.get();
* }
* }
*/
public final OperationCallable
createHubOperationCallable() {
return stub.createHubOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Network Connectivity Center hub in the specified project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* CreateHubRequest request =
* CreateHubRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setHubId("hubId99628272")
* .setHub(Hub.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = hubServiceClient.createHubCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createHubCallable() {
return stub.createHubCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the description and/or labels of a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* Hub hub = Hub.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Hub response = hubServiceClient.updateHubAsync(hub, updateMask).get();
* }
* }
*
* @param hub Required. The state that the hub should be in after the update.
* @param updateMask Optional. In the case of an update to an existing hub, field mask is used to
* specify the fields to be overwritten. The fields specified in the update_mask are relative
* to the resource, not the full request. A field is overwritten if it is in the mask. If the
* user does not provide a mask, then all fields are overwritten.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateHubAsync(
Hub hub, FieldMask updateMask) {
UpdateHubRequest request =
UpdateHubRequest.newBuilder().setHub(hub).setUpdateMask(updateMask).build();
return updateHubAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the description and/or labels of a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* UpdateHubRequest request =
* UpdateHubRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setHub(Hub.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Hub response = hubServiceClient.updateHubAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateHubAsync(UpdateHubRequest request) {
return updateHubOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the description and/or labels of a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* UpdateHubRequest request =
* UpdateHubRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setHub(Hub.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* hubServiceClient.updateHubOperationCallable().futureCall(request);
* // Do something.
* Hub response = future.get();
* }
* }
*/
public final OperationCallable
updateHubOperationCallable() {
return stub.updateHubOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the description and/or labels of a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* UpdateHubRequest request =
* UpdateHubRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setHub(Hub.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = hubServiceClient.updateHubCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateHubCallable() {
return stub.updateHubCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName name = HubName.of("[PROJECT]", "[HUB]");
* hubServiceClient.deleteHubAsync(name).get();
* }
* }
*
* @param name Required. The name of the hub to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteHubAsync(HubName name) {
DeleteHubRequest request =
DeleteHubRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteHubAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = HubName.of("[PROJECT]", "[HUB]").toString();
* hubServiceClient.deleteHubAsync(name).get();
* }
* }
*
* @param name Required. The name of the hub to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteHubAsync(String name) {
DeleteHubRequest request = DeleteHubRequest.newBuilder().setName(name).build();
return deleteHubAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* DeleteHubRequest request =
* DeleteHubRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setRequestId("requestId693933066")
* .build();
* hubServiceClient.deleteHubAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteHubAsync(DeleteHubRequest request) {
return deleteHubOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* DeleteHubRequest request =
* DeleteHubRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* hubServiceClient.deleteHubOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteHubOperationCallable() {
return stub.deleteHubOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* DeleteHubRequest request =
* DeleteHubRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = hubServiceClient.deleteHubCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteHubCallable() {
return stub.deleteHubCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes associated with a specified hub and location. The
* list includes both spokes that are attached to the hub and spokes that have been proposed but
* not yet accepted.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName name = HubName.of("[PROJECT]", "[HUB]");
* for (Spoke element : hubServiceClient.listHubSpokes(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The name of the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListHubSpokesPagedResponse listHubSpokes(HubName name) {
ListHubSpokesRequest request =
ListHubSpokesRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return listHubSpokes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes associated with a specified hub and location. The
* list includes both spokes that are attached to the hub and spokes that have been proposed but
* not yet accepted.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = HubName.of("[PROJECT]", "[HUB]").toString();
* for (Spoke element : hubServiceClient.listHubSpokes(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The name of the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListHubSpokesPagedResponse listHubSpokes(String name) {
ListHubSpokesRequest request = ListHubSpokesRequest.newBuilder().setName(name).build();
return listHubSpokes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes associated with a specified hub and location. The
* list includes both spokes that are attached to the hub and spokes that have been proposed but
* not yet accepted.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListHubSpokesRequest request =
* ListHubSpokesRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .addAllSpokeLocations(new ArrayList())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Spoke element : hubServiceClient.listHubSpokes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListHubSpokesPagedResponse listHubSpokes(ListHubSpokesRequest request) {
return listHubSpokesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes associated with a specified hub and location. The
* list includes both spokes that are attached to the hub and spokes that have been proposed but
* not yet accepted.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListHubSpokesRequest request =
* ListHubSpokesRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .addAllSpokeLocations(new ArrayList())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future = hubServiceClient.listHubSpokesPagedCallable().futureCall(request);
* // Do something.
* for (Spoke element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listHubSpokesPagedCallable() {
return stub.listHubSpokesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes associated with a specified hub and location. The
* list includes both spokes that are attached to the hub and spokes that have been proposed but
* not yet accepted.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListHubSpokesRequest request =
* ListHubSpokesRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .addAllSpokeLocations(new ArrayList())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListHubSpokesResponse response = hubServiceClient.listHubSpokesCallable().call(request);
* for (Spoke element : response.getSpokesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listHubSpokesCallable() {
return stub.listHubSpokesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Query the Private Service Connect propagation status of a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName name = HubName.of("[PROJECT]", "[HUB]");
* for (HubStatusEntry element : hubServiceClient.queryHubStatus(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The name of the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueryHubStatusPagedResponse queryHubStatus(HubName name) {
QueryHubStatusRequest request =
QueryHubStatusRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return queryHubStatus(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Query the Private Service Connect propagation status of a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = HubName.of("[PROJECT]", "[HUB]").toString();
* for (HubStatusEntry element : hubServiceClient.queryHubStatus(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The name of the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueryHubStatusPagedResponse queryHubStatus(String name) {
QueryHubStatusRequest request = QueryHubStatusRequest.newBuilder().setName(name).build();
return queryHubStatus(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Query the Private Service Connect propagation status of a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* QueryHubStatusRequest request =
* QueryHubStatusRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .setGroupBy("groupBy293428022")
* .build();
* for (HubStatusEntry element : hubServiceClient.queryHubStatus(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueryHubStatusPagedResponse queryHubStatus(QueryHubStatusRequest request) {
return queryHubStatusPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Query the Private Service Connect propagation status of a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* QueryHubStatusRequest request =
* QueryHubStatusRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .setGroupBy("groupBy293428022")
* .build();
* ApiFuture future =
* hubServiceClient.queryHubStatusPagedCallable().futureCall(request);
* // Do something.
* for (HubStatusEntry element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
queryHubStatusPagedCallable() {
return stub.queryHubStatusPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Query the Private Service Connect propagation status of a Network Connectivity Center hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* QueryHubStatusRequest request =
* QueryHubStatusRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .setGroupBy("groupBy293428022")
* .build();
* while (true) {
* QueryHubStatusResponse response = hubServiceClient.queryHubStatusCallable().call(request);
* for (HubStatusEntry element : response.getHubStatusEntriesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
queryHubStatusCallable() {
return stub.queryHubStatusCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes in a specified project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Spoke element : hubServiceClient.listSpokes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSpokesPagedResponse listSpokes(LocationName parent) {
ListSpokesRequest request =
ListSpokesRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listSpokes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes in a specified project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Spoke element : hubServiceClient.listSpokes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSpokesPagedResponse listSpokes(String parent) {
ListSpokesRequest request = ListSpokesRequest.newBuilder().setParent(parent).build();
return listSpokes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes in a specified project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListSpokesRequest request =
* ListSpokesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Spoke element : hubServiceClient.listSpokes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListSpokesPagedResponse listSpokes(ListSpokesRequest request) {
return listSpokesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes in a specified project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListSpokesRequest request =
* ListSpokesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future = hubServiceClient.listSpokesPagedCallable().futureCall(request);
* // Do something.
* for (Spoke element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listSpokesPagedCallable() {
return stub.listSpokesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Network Connectivity Center spokes in a specified project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListSpokesRequest request =
* ListSpokesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListSpokesResponse response = hubServiceClient.listSpokesCallable().call(request);
* for (Spoke element : response.getSpokesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listSpokesCallable() {
return stub.listSpokesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* SpokeName name = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]");
* Spoke response = hubServiceClient.getSpoke(name);
* }
* }
*
* @param name Required. The name of the spoke resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Spoke getSpoke(SpokeName name) {
GetSpokeRequest request =
GetSpokeRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getSpoke(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString();
* Spoke response = hubServiceClient.getSpoke(name);
* }
* }
*
* @param name Required. The name of the spoke resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Spoke getSpoke(String name) {
GetSpokeRequest request = GetSpokeRequest.newBuilder().setName(name).build();
return getSpoke(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetSpokeRequest request =
* GetSpokeRequest.newBuilder()
* .setName(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .build();
* Spoke response = hubServiceClient.getSpoke(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Spoke getSpoke(GetSpokeRequest request) {
return getSpokeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetSpokeRequest request =
* GetSpokeRequest.newBuilder()
* .setName(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .build();
* ApiFuture future = hubServiceClient.getSpokeCallable().futureCall(request);
* // Do something.
* Spoke response = future.get();
* }
* }
*/
public final UnaryCallable getSpokeCallable() {
return stub.getSpokeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Spoke spoke = Spoke.newBuilder().build();
* String spokeId = "spokeId-1998996281";
* Spoke response = hubServiceClient.createSpokeAsync(parent, spoke, spokeId).get();
* }
* }
*
* @param parent Required. The parent resource.
* @param spoke Required. The initial values for a new spoke.
* @param spokeId Required. Unique id for the spoke to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createSpokeAsync(
LocationName parent, Spoke spoke, String spokeId) {
CreateSpokeRequest request =
CreateSpokeRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setSpoke(spoke)
.setSpokeId(spokeId)
.build();
return createSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Spoke spoke = Spoke.newBuilder().build();
* String spokeId = "spokeId-1998996281";
* Spoke response = hubServiceClient.createSpokeAsync(parent, spoke, spokeId).get();
* }
* }
*
* @param parent Required. The parent resource.
* @param spoke Required. The initial values for a new spoke.
* @param spokeId Required. Unique id for the spoke to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createSpokeAsync(
String parent, Spoke spoke, String spokeId) {
CreateSpokeRequest request =
CreateSpokeRequest.newBuilder()
.setParent(parent)
.setSpoke(spoke)
.setSpokeId(spokeId)
.build();
return createSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* CreateSpokeRequest request =
* CreateSpokeRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setSpokeId("spokeId-1998996281")
* .setSpoke(Spoke.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Spoke response = hubServiceClient.createSpokeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createSpokeAsync(
CreateSpokeRequest request) {
return createSpokeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* CreateSpokeRequest request =
* CreateSpokeRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setSpokeId("spokeId-1998996281")
* .setSpoke(Spoke.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* hubServiceClient.createSpokeOperationCallable().futureCall(request);
* // Do something.
* Spoke response = future.get();
* }
* }
*/
public final OperationCallable
createSpokeOperationCallable() {
return stub.createSpokeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* CreateSpokeRequest request =
* CreateSpokeRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setSpokeId("spokeId-1998996281")
* .setSpoke(Spoke.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = hubServiceClient.createSpokeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createSpokeCallable() {
return stub.createSpokeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* Spoke spoke = Spoke.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Spoke response = hubServiceClient.updateSpokeAsync(spoke, updateMask).get();
* }
* }
*
* @param spoke Required. The state that the spoke should be in after the update.
* @param updateMask Optional. In the case of an update to an existing spoke, field mask is used
* to specify the fields to be overwritten. The fields specified in the update_mask are
* relative to the resource, not the full request. A field is overwritten if it is in the
* mask. If the user does not provide a mask, then all fields are overwritten.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateSpokeAsync(
Spoke spoke, FieldMask updateMask) {
UpdateSpokeRequest request =
UpdateSpokeRequest.newBuilder().setSpoke(spoke).setUpdateMask(updateMask).build();
return updateSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* UpdateSpokeRequest request =
* UpdateSpokeRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setSpoke(Spoke.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Spoke response = hubServiceClient.updateSpokeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateSpokeAsync(
UpdateSpokeRequest request) {
return updateSpokeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* UpdateSpokeRequest request =
* UpdateSpokeRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setSpoke(Spoke.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* hubServiceClient.updateSpokeOperationCallable().futureCall(request);
* // Do something.
* Spoke response = future.get();
* }
* }
*/
public final OperationCallable
updateSpokeOperationCallable() {
return stub.updateSpokeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* UpdateSpokeRequest request =
* UpdateSpokeRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setSpoke(Spoke.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = hubServiceClient.updateSpokeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateSpokeCallable() {
return stub.updateSpokeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a Network Connectivity Center spoke from being attached to a hub. If the spoke was
* previously in the `ACTIVE` state, it transitions to the `INACTIVE` state and is no longer able
* to connect to other spokes that are attached to the hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName name = HubName.of("[PROJECT]", "[HUB]");
* SpokeName spokeUri = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]");
* RejectHubSpokeResponse response = hubServiceClient.rejectHubSpokeAsync(name, spokeUri).get();
* }
* }
*
* @param name Required. The name of the hub from which to reject the spoke.
* @param spokeUri Required. The URI of the spoke to reject from the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture rejectHubSpokeAsync(
HubName name, SpokeName spokeUri) {
RejectHubSpokeRequest request =
RejectHubSpokeRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setSpokeUri(spokeUri == null ? null : spokeUri.toString())
.build();
return rejectHubSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a Network Connectivity Center spoke from being attached to a hub. If the spoke was
* previously in the `ACTIVE` state, it transitions to the `INACTIVE` state and is no longer able
* to connect to other spokes that are attached to the hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName name = HubName.of("[PROJECT]", "[HUB]");
* String spokeUri = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString();
* RejectHubSpokeResponse response = hubServiceClient.rejectHubSpokeAsync(name, spokeUri).get();
* }
* }
*
* @param name Required. The name of the hub from which to reject the spoke.
* @param spokeUri Required. The URI of the spoke to reject from the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture rejectHubSpokeAsync(
HubName name, String spokeUri) {
RejectHubSpokeRequest request =
RejectHubSpokeRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setSpokeUri(spokeUri)
.build();
return rejectHubSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a Network Connectivity Center spoke from being attached to a hub. If the spoke was
* previously in the `ACTIVE` state, it transitions to the `INACTIVE` state and is no longer able
* to connect to other spokes that are attached to the hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = HubName.of("[PROJECT]", "[HUB]").toString();
* SpokeName spokeUri = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]");
* RejectHubSpokeResponse response = hubServiceClient.rejectHubSpokeAsync(name, spokeUri).get();
* }
* }
*
* @param name Required. The name of the hub from which to reject the spoke.
* @param spokeUri Required. The URI of the spoke to reject from the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture rejectHubSpokeAsync(
String name, SpokeName spokeUri) {
RejectHubSpokeRequest request =
RejectHubSpokeRequest.newBuilder()
.setName(name)
.setSpokeUri(spokeUri == null ? null : spokeUri.toString())
.build();
return rejectHubSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a Network Connectivity Center spoke from being attached to a hub. If the spoke was
* previously in the `ACTIVE` state, it transitions to the `INACTIVE` state and is no longer able
* to connect to other spokes that are attached to the hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = HubName.of("[PROJECT]", "[HUB]").toString();
* String spokeUri = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString();
* RejectHubSpokeResponse response = hubServiceClient.rejectHubSpokeAsync(name, spokeUri).get();
* }
* }
*
* @param name Required. The name of the hub from which to reject the spoke.
* @param spokeUri Required. The URI of the spoke to reject from the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture rejectHubSpokeAsync(
String name, String spokeUri) {
RejectHubSpokeRequest request =
RejectHubSpokeRequest.newBuilder().setName(name).setSpokeUri(spokeUri).build();
return rejectHubSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a Network Connectivity Center spoke from being attached to a hub. If the spoke was
* previously in the `ACTIVE` state, it transitions to the `INACTIVE` state and is no longer able
* to connect to other spokes that are attached to the hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* RejectHubSpokeRequest request =
* RejectHubSpokeRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setSpokeUri(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .setRequestId("requestId693933066")
* .setDetails("details1557721666")
* .build();
* RejectHubSpokeResponse response = hubServiceClient.rejectHubSpokeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture rejectHubSpokeAsync(
RejectHubSpokeRequest request) {
return rejectHubSpokeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a Network Connectivity Center spoke from being attached to a hub. If the spoke was
* previously in the `ACTIVE` state, it transitions to the `INACTIVE` state and is no longer able
* to connect to other spokes that are attached to the hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* RejectHubSpokeRequest request =
* RejectHubSpokeRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setSpokeUri(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .setRequestId("requestId693933066")
* .setDetails("details1557721666")
* .build();
* OperationFuture future =
* hubServiceClient.rejectHubSpokeOperationCallable().futureCall(request);
* // Do something.
* RejectHubSpokeResponse response = future.get();
* }
* }
*/
public final OperationCallable
rejectHubSpokeOperationCallable() {
return stub.rejectHubSpokeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a Network Connectivity Center spoke from being attached to a hub. If the spoke was
* previously in the `ACTIVE` state, it transitions to the `INACTIVE` state and is no longer able
* to connect to other spokes that are attached to the hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* RejectHubSpokeRequest request =
* RejectHubSpokeRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setSpokeUri(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .setRequestId("requestId693933066")
* .setDetails("details1557721666")
* .build();
* ApiFuture future = hubServiceClient.rejectHubSpokeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable rejectHubSpokeCallable() {
return stub.rejectHubSpokeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Accepts a proposal to attach a Network Connectivity Center spoke to a hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName name = HubName.of("[PROJECT]", "[HUB]");
* SpokeName spokeUri = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]");
* AcceptHubSpokeResponse response = hubServiceClient.acceptHubSpokeAsync(name, spokeUri).get();
* }
* }
*
* @param name Required. The name of the hub into which to accept the spoke.
* @param spokeUri Required. The URI of the spoke to accept into the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture acceptHubSpokeAsync(
HubName name, SpokeName spokeUri) {
AcceptHubSpokeRequest request =
AcceptHubSpokeRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setSpokeUri(spokeUri == null ? null : spokeUri.toString())
.build();
return acceptHubSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Accepts a proposal to attach a Network Connectivity Center spoke to a hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName name = HubName.of("[PROJECT]", "[HUB]");
* String spokeUri = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString();
* AcceptHubSpokeResponse response = hubServiceClient.acceptHubSpokeAsync(name, spokeUri).get();
* }
* }
*
* @param name Required. The name of the hub into which to accept the spoke.
* @param spokeUri Required. The URI of the spoke to accept into the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture acceptHubSpokeAsync(
HubName name, String spokeUri) {
AcceptHubSpokeRequest request =
AcceptHubSpokeRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setSpokeUri(spokeUri)
.build();
return acceptHubSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Accepts a proposal to attach a Network Connectivity Center spoke to a hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = HubName.of("[PROJECT]", "[HUB]").toString();
* SpokeName spokeUri = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]");
* AcceptHubSpokeResponse response = hubServiceClient.acceptHubSpokeAsync(name, spokeUri).get();
* }
* }
*
* @param name Required. The name of the hub into which to accept the spoke.
* @param spokeUri Required. The URI of the spoke to accept into the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture acceptHubSpokeAsync(
String name, SpokeName spokeUri) {
AcceptHubSpokeRequest request =
AcceptHubSpokeRequest.newBuilder()
.setName(name)
.setSpokeUri(spokeUri == null ? null : spokeUri.toString())
.build();
return acceptHubSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Accepts a proposal to attach a Network Connectivity Center spoke to a hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = HubName.of("[PROJECT]", "[HUB]").toString();
* String spokeUri = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString();
* AcceptHubSpokeResponse response = hubServiceClient.acceptHubSpokeAsync(name, spokeUri).get();
* }
* }
*
* @param name Required. The name of the hub into which to accept the spoke.
* @param spokeUri Required. The URI of the spoke to accept into the hub.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture acceptHubSpokeAsync(
String name, String spokeUri) {
AcceptHubSpokeRequest request =
AcceptHubSpokeRequest.newBuilder().setName(name).setSpokeUri(spokeUri).build();
return acceptHubSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Accepts a proposal to attach a Network Connectivity Center spoke to a hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* AcceptHubSpokeRequest request =
* AcceptHubSpokeRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setSpokeUri(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .setRequestId("requestId693933066")
* .build();
* AcceptHubSpokeResponse response = hubServiceClient.acceptHubSpokeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture acceptHubSpokeAsync(
AcceptHubSpokeRequest request) {
return acceptHubSpokeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Accepts a proposal to attach a Network Connectivity Center spoke to a hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* AcceptHubSpokeRequest request =
* AcceptHubSpokeRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setSpokeUri(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* hubServiceClient.acceptHubSpokeOperationCallable().futureCall(request);
* // Do something.
* AcceptHubSpokeResponse response = future.get();
* }
* }
*/
public final OperationCallable
acceptHubSpokeOperationCallable() {
return stub.acceptHubSpokeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Accepts a proposal to attach a Network Connectivity Center spoke to a hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* AcceptHubSpokeRequest request =
* AcceptHubSpokeRequest.newBuilder()
* .setName(HubName.of("[PROJECT]", "[HUB]").toString())
* .setSpokeUri(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = hubServiceClient.acceptHubSpokeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable acceptHubSpokeCallable() {
return stub.acceptHubSpokeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* SpokeName name = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]");
* hubServiceClient.deleteSpokeAsync(name).get();
* }
* }
*
* @param name Required. The name of the spoke to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteSpokeAsync(SpokeName name) {
DeleteSpokeRequest request =
DeleteSpokeRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString();
* hubServiceClient.deleteSpokeAsync(name).get();
* }
* }
*
* @param name Required. The name of the spoke to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteSpokeAsync(String name) {
DeleteSpokeRequest request = DeleteSpokeRequest.newBuilder().setName(name).build();
return deleteSpokeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* DeleteSpokeRequest request =
* DeleteSpokeRequest.newBuilder()
* .setName(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .setRequestId("requestId693933066")
* .build();
* hubServiceClient.deleteSpokeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteSpokeAsync(
DeleteSpokeRequest request) {
return deleteSpokeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* DeleteSpokeRequest request =
* DeleteSpokeRequest.newBuilder()
* .setName(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* hubServiceClient.deleteSpokeOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteSpokeOperationCallable() {
return stub.deleteSpokeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a Network Connectivity Center spoke.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* DeleteSpokeRequest request =
* DeleteSpokeRequest.newBuilder()
* .setName(SpokeName.of("[PROJECT]", "[LOCATION]", "[SPOKE]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = hubServiceClient.deleteSpokeCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteSpokeCallable() {
return stub.deleteSpokeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center route table.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* RouteTableName name = RouteTableName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]");
* RouteTable response = hubServiceClient.getRouteTable(name);
* }
* }
*
* @param name Required. The name of the route table resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RouteTable getRouteTable(RouteTableName name) {
GetRouteTableRequest request =
GetRouteTableRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getRouteTable(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center route table.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = RouteTableName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]").toString();
* RouteTable response = hubServiceClient.getRouteTable(name);
* }
* }
*
* @param name Required. The name of the route table resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RouteTable getRouteTable(String name) {
GetRouteTableRequest request = GetRouteTableRequest.newBuilder().setName(name).build();
return getRouteTable(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center route table.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetRouteTableRequest request =
* GetRouteTableRequest.newBuilder()
* .setName(RouteTableName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]").toString())
* .build();
* RouteTable response = hubServiceClient.getRouteTable(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RouteTable getRouteTable(GetRouteTableRequest request) {
return getRouteTableCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center route table.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetRouteTableRequest request =
* GetRouteTableRequest.newBuilder()
* .setName(RouteTableName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]").toString())
* .build();
* ApiFuture future = hubServiceClient.getRouteTableCallable().futureCall(request);
* // Do something.
* RouteTable response = future.get();
* }
* }
*/
public final UnaryCallable getRouteTableCallable() {
return stub.getRouteTableCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about the specified route.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubRouteName name = HubRouteName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]", "[ROUTE]");
* Route response = hubServiceClient.getRoute(name);
* }
* }
*
* @param name Required. The name of the route resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Route getRoute(HubRouteName name) {
GetRouteRequest request =
GetRouteRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getRoute(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about the specified route.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = HubRouteName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]", "[ROUTE]").toString();
* Route response = hubServiceClient.getRoute(name);
* }
* }
*
* @param name Required. The name of the route resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Route getRoute(String name) {
GetRouteRequest request = GetRouteRequest.newBuilder().setName(name).build();
return getRoute(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about the specified route.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetRouteRequest request =
* GetRouteRequest.newBuilder()
* .setName(HubRouteName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]", "[ROUTE]").toString())
* .build();
* Route response = hubServiceClient.getRoute(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Route getRoute(GetRouteRequest request) {
return getRouteCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about the specified route.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetRouteRequest request =
* GetRouteRequest.newBuilder()
* .setName(HubRouteName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]", "[ROUTE]").toString())
* .build();
* ApiFuture future = hubServiceClient.getRouteCallable().futureCall(request);
* // Do something.
* Route response = future.get();
* }
* }
*/
public final UnaryCallable getRouteCallable() {
return stub.getRouteCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists routes in a given route table.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* RouteTableName parent = RouteTableName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]");
* for (Route element : hubServiceClient.listRoutes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource's name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRoutesPagedResponse listRoutes(RouteTableName parent) {
ListRoutesRequest request =
ListRoutesRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listRoutes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists routes in a given route table.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String parent = RouteTableName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]").toString();
* for (Route element : hubServiceClient.listRoutes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource's name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRoutesPagedResponse listRoutes(String parent) {
ListRoutesRequest request = ListRoutesRequest.newBuilder().setParent(parent).build();
return listRoutes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists routes in a given route table.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListRoutesRequest request =
* ListRoutesRequest.newBuilder()
* .setParent(RouteTableName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Route element : hubServiceClient.listRoutes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRoutesPagedResponse listRoutes(ListRoutesRequest request) {
return listRoutesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists routes in a given route table.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListRoutesRequest request =
* ListRoutesRequest.newBuilder()
* .setParent(RouteTableName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future = hubServiceClient.listRoutesPagedCallable().futureCall(request);
* // Do something.
* for (Route element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listRoutesPagedCallable() {
return stub.listRoutesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists routes in a given route table.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListRoutesRequest request =
* ListRoutesRequest.newBuilder()
* .setParent(RouteTableName.of("[PROJECT]", "[HUB]", "[ROUTE_TABLE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListRoutesResponse response = hubServiceClient.listRoutesCallable().call(request);
* for (Route element : response.getRoutesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listRoutesCallable() {
return stub.listRoutesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists route tables in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName parent = HubName.of("[PROJECT]", "[HUB]");
* for (RouteTable element : hubServiceClient.listRouteTables(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource's name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRouteTablesPagedResponse listRouteTables(HubName parent) {
ListRouteTablesRequest request =
ListRouteTablesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listRouteTables(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists route tables in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String parent = HubName.of("[PROJECT]", "[HUB]").toString();
* for (RouteTable element : hubServiceClient.listRouteTables(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource's name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRouteTablesPagedResponse listRouteTables(String parent) {
ListRouteTablesRequest request = ListRouteTablesRequest.newBuilder().setParent(parent).build();
return listRouteTables(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists route tables in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListRouteTablesRequest request =
* ListRouteTablesRequest.newBuilder()
* .setParent(HubName.of("[PROJECT]", "[HUB]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (RouteTable element : hubServiceClient.listRouteTables(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRouteTablesPagedResponse listRouteTables(ListRouteTablesRequest request) {
return listRouteTablesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists route tables in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListRouteTablesRequest request =
* ListRouteTablesRequest.newBuilder()
* .setParent(HubName.of("[PROJECT]", "[HUB]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* hubServiceClient.listRouteTablesPagedCallable().futureCall(request);
* // Do something.
* for (RouteTable element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listRouteTablesPagedCallable() {
return stub.listRouteTablesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists route tables in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListRouteTablesRequest request =
* ListRouteTablesRequest.newBuilder()
* .setParent(HubName.of("[PROJECT]", "[HUB]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListRouteTablesResponse response = hubServiceClient.listRouteTablesCallable().call(request);
* for (RouteTable element : response.getRouteTablesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listRouteTablesCallable() {
return stub.listRouteTablesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center group.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GroupName name = GroupName.of("[PROJECT]", "[HUB]", "[GROUP]");
* Group response = hubServiceClient.getGroup(name);
* }
* }
*
* @param name Required. The name of the route table resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Group getGroup(GroupName name) {
GetGroupRequest request =
GetGroupRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getGroup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center group.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String name = GroupName.of("[PROJECT]", "[HUB]", "[GROUP]").toString();
* Group response = hubServiceClient.getGroup(name);
* }
* }
*
* @param name Required. The name of the route table resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Group getGroup(String name) {
GetGroupRequest request = GetGroupRequest.newBuilder().setName(name).build();
return getGroup(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center group.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetGroupRequest request =
* GetGroupRequest.newBuilder()
* .setName(GroupName.of("[PROJECT]", "[HUB]", "[GROUP]").toString())
* .build();
* Group response = hubServiceClient.getGroup(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Group getGroup(GetGroupRequest request) {
return getGroupCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details about a Network Connectivity Center group.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetGroupRequest request =
* GetGroupRequest.newBuilder()
* .setName(GroupName.of("[PROJECT]", "[HUB]", "[GROUP]").toString())
* .build();
* ApiFuture future = hubServiceClient.getGroupCallable().futureCall(request);
* // Do something.
* Group response = future.get();
* }
* }
*/
public final UnaryCallable getGroupCallable() {
return stub.getGroupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists groups in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* HubName parent = HubName.of("[PROJECT]", "[HUB]");
* for (Group element : hubServiceClient.listGroups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource's name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListGroupsPagedResponse listGroups(HubName parent) {
ListGroupsRequest request =
ListGroupsRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listGroups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists groups in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* String parent = HubName.of("[PROJECT]", "[HUB]").toString();
* for (Group element : hubServiceClient.listGroups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource's name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListGroupsPagedResponse listGroups(String parent) {
ListGroupsRequest request = ListGroupsRequest.newBuilder().setParent(parent).build();
return listGroups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists groups in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListGroupsRequest request =
* ListGroupsRequest.newBuilder()
* .setParent(HubName.of("[PROJECT]", "[HUB]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Group element : hubServiceClient.listGroups(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListGroupsPagedResponse listGroups(ListGroupsRequest request) {
return listGroupsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists groups in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListGroupsRequest request =
* ListGroupsRequest.newBuilder()
* .setParent(HubName.of("[PROJECT]", "[HUB]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future = hubServiceClient.listGroupsPagedCallable().futureCall(request);
* // Do something.
* for (Group element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listGroupsPagedCallable() {
return stub.listGroupsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists groups in a given hub.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListGroupsRequest request =
* ListGroupsRequest.newBuilder()
* .setParent(HubName.of("[PROJECT]", "[HUB]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListGroupsResponse response = hubServiceClient.listGroupsCallable().call(request);
* for (Group element : response.getGroupsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listGroupsCallable() {
return stub.listGroupsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a Network Connectivity Center group.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* Group group = Group.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Group response = hubServiceClient.updateGroupAsync(group, updateMask).get();
* }
* }
*
* @param group Required. The state that the group should be in after the update.
* @param updateMask Optional. In the case of an update to an existing group, field mask is used
* to specify the fields to be overwritten. The fields specified in the update_mask are
* relative to the resource, not the full request. A field is overwritten if it is in the
* mask. If the user does not provide a mask, then all fields are overwritten.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateGroupAsync(
Group group, FieldMask updateMask) {
UpdateGroupRequest request =
UpdateGroupRequest.newBuilder().setGroup(group).setUpdateMask(updateMask).build();
return updateGroupAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a Network Connectivity Center group.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* UpdateGroupRequest request =
* UpdateGroupRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setGroup(Group.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Group response = hubServiceClient.updateGroupAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateGroupAsync(
UpdateGroupRequest request) {
return updateGroupOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a Network Connectivity Center group.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* UpdateGroupRequest request =
* UpdateGroupRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setGroup(Group.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* hubServiceClient.updateGroupOperationCallable().futureCall(request);
* // Do something.
* Group response = future.get();
* }
* }
*/
public final OperationCallable
updateGroupOperationCallable() {
return stub.updateGroupOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the parameters of a Network Connectivity Center group.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* UpdateGroupRequest request =
* UpdateGroupRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setGroup(Group.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = hubServiceClient.updateGroupCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateGroupCallable() {
return stub.updateGroupCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : hubServiceClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* hubServiceClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response = hubServiceClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = hubServiceClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = hubServiceClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(GroupName.of("[PROJECT]", "[HUB]", "[GROUP]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Policy response = hubServiceClient.setIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(SetIamPolicyRequest request) {
return setIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(GroupName.of("[PROJECT]", "[HUB]", "[GROUP]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = hubServiceClient.setIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable setIamPolicyCallable() {
return stub.setIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(GroupName.of("[PROJECT]", "[HUB]", "[GROUP]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* Policy response = hubServiceClient.getIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(GetIamPolicyRequest request) {
return getIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(GroupName.of("[PROJECT]", "[HUB]", "[GROUP]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* ApiFuture future = hubServiceClient.getIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable getIamPolicyCallable() {
return stub.getIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(GroupName.of("[PROJECT]", "[HUB]", "[GROUP]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* TestIamPermissionsResponse response = hubServiceClient.testIamPermissions(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(TestIamPermissionsRequest request) {
return testIamPermissionsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (HubServiceClient hubServiceClient = HubServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(GroupName.of("[PROJECT]", "[HUB]", "[GROUP]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* ApiFuture future =
* hubServiceClient.testIamPermissionsCallable().futureCall(request);
* // Do something.
* TestIamPermissionsResponse response = future.get();
* }
* }
*/
public final UnaryCallable
testIamPermissionsCallable() {
return stub.testIamPermissionsCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListHubsPagedResponse
extends AbstractPagedListResponse<
ListHubsRequest, ListHubsResponse, Hub, ListHubsPage, ListHubsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListHubsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListHubsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListHubsPagedResponse(ListHubsPage page) {
super(page, ListHubsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListHubsPage
extends AbstractPage {
private ListHubsPage(
PageContext context, ListHubsResponse response) {
super(context, response);
}
private static ListHubsPage createEmptyPage() {
return new ListHubsPage(null, null);
}
@Override
protected ListHubsPage createPage(
PageContext context, ListHubsResponse response) {
return new ListHubsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListHubsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListHubsRequest, ListHubsResponse, Hub, ListHubsPage, ListHubsFixedSizeCollection> {
private ListHubsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListHubsFixedSizeCollection createEmptyCollection() {
return new ListHubsFixedSizeCollection(null, 0);
}
@Override
protected ListHubsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListHubsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListHubSpokesPagedResponse
extends AbstractPagedListResponse<
ListHubSpokesRequest,
ListHubSpokesResponse,
Spoke,
ListHubSpokesPage,
ListHubSpokesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListHubSpokesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListHubSpokesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListHubSpokesPagedResponse(ListHubSpokesPage page) {
super(page, ListHubSpokesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListHubSpokesPage
extends AbstractPage {
private ListHubSpokesPage(
PageContext context,
ListHubSpokesResponse response) {
super(context, response);
}
private static ListHubSpokesPage createEmptyPage() {
return new ListHubSpokesPage(null, null);
}
@Override
protected ListHubSpokesPage createPage(
PageContext context,
ListHubSpokesResponse response) {
return new ListHubSpokesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListHubSpokesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListHubSpokesRequest,
ListHubSpokesResponse,
Spoke,
ListHubSpokesPage,
ListHubSpokesFixedSizeCollection> {
private ListHubSpokesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListHubSpokesFixedSizeCollection createEmptyCollection() {
return new ListHubSpokesFixedSizeCollection(null, 0);
}
@Override
protected ListHubSpokesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListHubSpokesFixedSizeCollection(pages, collectionSize);
}
}
public static class QueryHubStatusPagedResponse
extends AbstractPagedListResponse<
QueryHubStatusRequest,
QueryHubStatusResponse,
HubStatusEntry,
QueryHubStatusPage,
QueryHubStatusFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
QueryHubStatusPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new QueryHubStatusPagedResponse(input),
MoreExecutors.directExecutor());
}
private QueryHubStatusPagedResponse(QueryHubStatusPage page) {
super(page, QueryHubStatusFixedSizeCollection.createEmptyCollection());
}
}
public static class QueryHubStatusPage
extends AbstractPage<
QueryHubStatusRequest, QueryHubStatusResponse, HubStatusEntry, QueryHubStatusPage> {
private QueryHubStatusPage(
PageContext context,
QueryHubStatusResponse response) {
super(context, response);
}
private static QueryHubStatusPage createEmptyPage() {
return new QueryHubStatusPage(null, null);
}
@Override
protected QueryHubStatusPage createPage(
PageContext context,
QueryHubStatusResponse response) {
return new QueryHubStatusPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class QueryHubStatusFixedSizeCollection
extends AbstractFixedSizeCollection<
QueryHubStatusRequest,
QueryHubStatusResponse,
HubStatusEntry,
QueryHubStatusPage,
QueryHubStatusFixedSizeCollection> {
private QueryHubStatusFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static QueryHubStatusFixedSizeCollection createEmptyCollection() {
return new QueryHubStatusFixedSizeCollection(null, 0);
}
@Override
protected QueryHubStatusFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new QueryHubStatusFixedSizeCollection(pages, collectionSize);
}
}
public static class ListSpokesPagedResponse
extends AbstractPagedListResponse<
ListSpokesRequest,
ListSpokesResponse,
Spoke,
ListSpokesPage,
ListSpokesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListSpokesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListSpokesPagedResponse(input), MoreExecutors.directExecutor());
}
private ListSpokesPagedResponse(ListSpokesPage page) {
super(page, ListSpokesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListSpokesPage
extends AbstractPage {
private ListSpokesPage(
PageContext context,
ListSpokesResponse response) {
super(context, response);
}
private static ListSpokesPage createEmptyPage() {
return new ListSpokesPage(null, null);
}
@Override
protected ListSpokesPage createPage(
PageContext context,
ListSpokesResponse response) {
return new ListSpokesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListSpokesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListSpokesRequest,
ListSpokesResponse,
Spoke,
ListSpokesPage,
ListSpokesFixedSizeCollection> {
private ListSpokesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListSpokesFixedSizeCollection createEmptyCollection() {
return new ListSpokesFixedSizeCollection(null, 0);
}
@Override
protected ListSpokesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListSpokesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListRoutesPagedResponse
extends AbstractPagedListResponse<
ListRoutesRequest,
ListRoutesResponse,
Route,
ListRoutesPage,
ListRoutesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListRoutesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListRoutesPagedResponse(input), MoreExecutors.directExecutor());
}
private ListRoutesPagedResponse(ListRoutesPage page) {
super(page, ListRoutesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListRoutesPage
extends AbstractPage {
private ListRoutesPage(
PageContext context,
ListRoutesResponse response) {
super(context, response);
}
private static ListRoutesPage createEmptyPage() {
return new ListRoutesPage(null, null);
}
@Override
protected ListRoutesPage createPage(
PageContext context,
ListRoutesResponse response) {
return new ListRoutesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListRoutesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListRoutesRequest,
ListRoutesResponse,
Route,
ListRoutesPage,
ListRoutesFixedSizeCollection> {
private ListRoutesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListRoutesFixedSizeCollection createEmptyCollection() {
return new ListRoutesFixedSizeCollection(null, 0);
}
@Override
protected ListRoutesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListRoutesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListRouteTablesPagedResponse
extends AbstractPagedListResponse<
ListRouteTablesRequest,
ListRouteTablesResponse,
RouteTable,
ListRouteTablesPage,
ListRouteTablesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListRouteTablesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListRouteTablesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListRouteTablesPagedResponse(ListRouteTablesPage page) {
super(page, ListRouteTablesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListRouteTablesPage
extends AbstractPage<
ListRouteTablesRequest, ListRouteTablesResponse, RouteTable, ListRouteTablesPage> {
private ListRouteTablesPage(
PageContext context,
ListRouteTablesResponse response) {
super(context, response);
}
private static ListRouteTablesPage createEmptyPage() {
return new ListRouteTablesPage(null, null);
}
@Override
protected ListRouteTablesPage createPage(
PageContext context,
ListRouteTablesResponse response) {
return new ListRouteTablesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListRouteTablesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListRouteTablesRequest,
ListRouteTablesResponse,
RouteTable,
ListRouteTablesPage,
ListRouteTablesFixedSizeCollection> {
private ListRouteTablesFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListRouteTablesFixedSizeCollection createEmptyCollection() {
return new ListRouteTablesFixedSizeCollection(null, 0);
}
@Override
protected ListRouteTablesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListRouteTablesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListGroupsPagedResponse
extends AbstractPagedListResponse<
ListGroupsRequest,
ListGroupsResponse,
Group,
ListGroupsPage,
ListGroupsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListGroupsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListGroupsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListGroupsPagedResponse(ListGroupsPage page) {
super(page, ListGroupsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListGroupsPage
extends AbstractPage {
private ListGroupsPage(
PageContext context,
ListGroupsResponse response) {
super(context, response);
}
private static ListGroupsPage createEmptyPage() {
return new ListGroupsPage(null, null);
}
@Override
protected ListGroupsPage createPage(
PageContext context,
ListGroupsResponse response) {
return new ListGroupsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListGroupsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListGroupsRequest,
ListGroupsResponse,
Group,
ListGroupsPage,
ListGroupsFixedSizeCollection> {
private ListGroupsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListGroupsFixedSizeCollection createEmptyCollection() {
return new ListGroupsFixedSizeCollection(null, 0);
}
@Override
protected ListGroupsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListGroupsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}