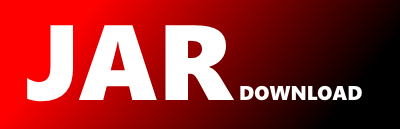
com.google.cloud.networkconnectivity.v1.stub.HubServiceStub Maven / Gradle / Ivy
Show all versions of google-cloud-networkconnectivity Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.networkconnectivity.v1.stub;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListGroupsPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListHubSpokesPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListHubsPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListLocationsPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListRouteTablesPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListRoutesPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListSpokesPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.QueryHubStatusPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.networkconnectivity.v1.AcceptHubSpokeRequest;
import com.google.cloud.networkconnectivity.v1.AcceptHubSpokeResponse;
import com.google.cloud.networkconnectivity.v1.CreateHubRequest;
import com.google.cloud.networkconnectivity.v1.CreateSpokeRequest;
import com.google.cloud.networkconnectivity.v1.DeleteHubRequest;
import com.google.cloud.networkconnectivity.v1.DeleteSpokeRequest;
import com.google.cloud.networkconnectivity.v1.GetGroupRequest;
import com.google.cloud.networkconnectivity.v1.GetHubRequest;
import com.google.cloud.networkconnectivity.v1.GetRouteRequest;
import com.google.cloud.networkconnectivity.v1.GetRouteTableRequest;
import com.google.cloud.networkconnectivity.v1.GetSpokeRequest;
import com.google.cloud.networkconnectivity.v1.Group;
import com.google.cloud.networkconnectivity.v1.Hub;
import com.google.cloud.networkconnectivity.v1.ListGroupsRequest;
import com.google.cloud.networkconnectivity.v1.ListGroupsResponse;
import com.google.cloud.networkconnectivity.v1.ListHubSpokesRequest;
import com.google.cloud.networkconnectivity.v1.ListHubSpokesResponse;
import com.google.cloud.networkconnectivity.v1.ListHubsRequest;
import com.google.cloud.networkconnectivity.v1.ListHubsResponse;
import com.google.cloud.networkconnectivity.v1.ListRouteTablesRequest;
import com.google.cloud.networkconnectivity.v1.ListRouteTablesResponse;
import com.google.cloud.networkconnectivity.v1.ListRoutesRequest;
import com.google.cloud.networkconnectivity.v1.ListRoutesResponse;
import com.google.cloud.networkconnectivity.v1.ListSpokesRequest;
import com.google.cloud.networkconnectivity.v1.ListSpokesResponse;
import com.google.cloud.networkconnectivity.v1.OperationMetadata;
import com.google.cloud.networkconnectivity.v1.QueryHubStatusRequest;
import com.google.cloud.networkconnectivity.v1.QueryHubStatusResponse;
import com.google.cloud.networkconnectivity.v1.RejectHubSpokeRequest;
import com.google.cloud.networkconnectivity.v1.RejectHubSpokeResponse;
import com.google.cloud.networkconnectivity.v1.Route;
import com.google.cloud.networkconnectivity.v1.RouteTable;
import com.google.cloud.networkconnectivity.v1.Spoke;
import com.google.cloud.networkconnectivity.v1.UpdateGroupRequest;
import com.google.cloud.networkconnectivity.v1.UpdateHubRequest;
import com.google.cloud.networkconnectivity.v1.UpdateSpokeRequest;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.OperationsStub;
import com.google.protobuf.Empty;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Base stub class for the HubService service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public abstract class HubServiceStub implements BackgroundResource {
public OperationsStub getOperationsStub() {
throw new UnsupportedOperationException("Not implemented: getOperationsStub()");
}
public UnaryCallable listHubsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listHubsPagedCallable()");
}
public UnaryCallable listHubsCallable() {
throw new UnsupportedOperationException("Not implemented: listHubsCallable()");
}
public UnaryCallable getHubCallable() {
throw new UnsupportedOperationException("Not implemented: getHubCallable()");
}
public OperationCallable createHubOperationCallable() {
throw new UnsupportedOperationException("Not implemented: createHubOperationCallable()");
}
public UnaryCallable createHubCallable() {
throw new UnsupportedOperationException("Not implemented: createHubCallable()");
}
public OperationCallable updateHubOperationCallable() {
throw new UnsupportedOperationException("Not implemented: updateHubOperationCallable()");
}
public UnaryCallable updateHubCallable() {
throw new UnsupportedOperationException("Not implemented: updateHubCallable()");
}
public OperationCallable
deleteHubOperationCallable() {
throw new UnsupportedOperationException("Not implemented: deleteHubOperationCallable()");
}
public UnaryCallable deleteHubCallable() {
throw new UnsupportedOperationException("Not implemented: deleteHubCallable()");
}
public UnaryCallable
listHubSpokesPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listHubSpokesPagedCallable()");
}
public UnaryCallable listHubSpokesCallable() {
throw new UnsupportedOperationException("Not implemented: listHubSpokesCallable()");
}
public UnaryCallable
queryHubStatusPagedCallable() {
throw new UnsupportedOperationException("Not implemented: queryHubStatusPagedCallable()");
}
public UnaryCallable queryHubStatusCallable() {
throw new UnsupportedOperationException("Not implemented: queryHubStatusCallable()");
}
public UnaryCallable listSpokesPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listSpokesPagedCallable()");
}
public UnaryCallable listSpokesCallable() {
throw new UnsupportedOperationException("Not implemented: listSpokesCallable()");
}
public UnaryCallable getSpokeCallable() {
throw new UnsupportedOperationException("Not implemented: getSpokeCallable()");
}
public OperationCallable
createSpokeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: createSpokeOperationCallable()");
}
public UnaryCallable createSpokeCallable() {
throw new UnsupportedOperationException("Not implemented: createSpokeCallable()");
}
public OperationCallable
updateSpokeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: updateSpokeOperationCallable()");
}
public UnaryCallable updateSpokeCallable() {
throw new UnsupportedOperationException("Not implemented: updateSpokeCallable()");
}
public OperationCallable
rejectHubSpokeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: rejectHubSpokeOperationCallable()");
}
public UnaryCallable rejectHubSpokeCallable() {
throw new UnsupportedOperationException("Not implemented: rejectHubSpokeCallable()");
}
public OperationCallable
acceptHubSpokeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: acceptHubSpokeOperationCallable()");
}
public UnaryCallable acceptHubSpokeCallable() {
throw new UnsupportedOperationException("Not implemented: acceptHubSpokeCallable()");
}
public OperationCallable
deleteSpokeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: deleteSpokeOperationCallable()");
}
public UnaryCallable deleteSpokeCallable() {
throw new UnsupportedOperationException("Not implemented: deleteSpokeCallable()");
}
public UnaryCallable getRouteTableCallable() {
throw new UnsupportedOperationException("Not implemented: getRouteTableCallable()");
}
public UnaryCallable getRouteCallable() {
throw new UnsupportedOperationException("Not implemented: getRouteCallable()");
}
public UnaryCallable listRoutesPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listRoutesPagedCallable()");
}
public UnaryCallable listRoutesCallable() {
throw new UnsupportedOperationException("Not implemented: listRoutesCallable()");
}
public UnaryCallable
listRouteTablesPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listRouteTablesPagedCallable()");
}
public UnaryCallable listRouteTablesCallable() {
throw new UnsupportedOperationException("Not implemented: listRouteTablesCallable()");
}
public UnaryCallable getGroupCallable() {
throw new UnsupportedOperationException("Not implemented: getGroupCallable()");
}
public UnaryCallable listGroupsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listGroupsPagedCallable()");
}
public UnaryCallable listGroupsCallable() {
throw new UnsupportedOperationException("Not implemented: listGroupsCallable()");
}
public OperationCallable
updateGroupOperationCallable() {
throw new UnsupportedOperationException("Not implemented: updateGroupOperationCallable()");
}
public UnaryCallable updateGroupCallable() {
throw new UnsupportedOperationException("Not implemented: updateGroupCallable()");
}
public UnaryCallable
listLocationsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listLocationsPagedCallable()");
}
public UnaryCallable listLocationsCallable() {
throw new UnsupportedOperationException("Not implemented: listLocationsCallable()");
}
public UnaryCallable getLocationCallable() {
throw new UnsupportedOperationException("Not implemented: getLocationCallable()");
}
public UnaryCallable setIamPolicyCallable() {
throw new UnsupportedOperationException("Not implemented: setIamPolicyCallable()");
}
public UnaryCallable getIamPolicyCallable() {
throw new UnsupportedOperationException("Not implemented: getIamPolicyCallable()");
}
public UnaryCallable
testIamPermissionsCallable() {
throw new UnsupportedOperationException("Not implemented: testIamPermissionsCallable()");
}
@Override
public abstract void close();
}