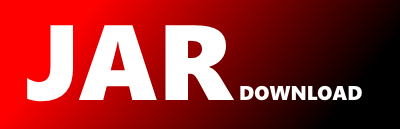
com.google.cloud.networkconnectivity.v1.stub.HubServiceStubSettings Maven / Gradle / Ivy
Show all versions of google-cloud-networkconnectivity Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.networkconnectivity.v1.stub;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListGroupsPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListHubSpokesPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListHubsPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListLocationsPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListRouteTablesPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListRoutesPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.ListSpokesPagedResponse;
import static com.google.cloud.networkconnectivity.v1.HubServiceClient.QueryHubStatusPagedResponse;
import com.google.api.core.ApiFunction;
import com.google.api.core.ApiFuture;
import com.google.api.core.ObsoleteApi;
import com.google.api.gax.core.GaxProperties;
import com.google.api.gax.core.GoogleCredentialsProvider;
import com.google.api.gax.core.InstantiatingExecutorProvider;
import com.google.api.gax.grpc.GaxGrpcProperties;
import com.google.api.gax.grpc.GrpcTransportChannel;
import com.google.api.gax.grpc.InstantiatingGrpcChannelProvider;
import com.google.api.gax.grpc.ProtoOperationTransformers;
import com.google.api.gax.longrunning.OperationSnapshot;
import com.google.api.gax.longrunning.OperationTimedPollAlgorithm;
import com.google.api.gax.retrying.RetrySettings;
import com.google.api.gax.rpc.ApiCallContext;
import com.google.api.gax.rpc.ApiClientHeaderProvider;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallSettings;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.PagedCallSettings;
import com.google.api.gax.rpc.PagedListDescriptor;
import com.google.api.gax.rpc.PagedListResponseFactory;
import com.google.api.gax.rpc.StatusCode;
import com.google.api.gax.rpc.StubSettings;
import com.google.api.gax.rpc.TransportChannelProvider;
import com.google.api.gax.rpc.UnaryCallSettings;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.networkconnectivity.v1.AcceptHubSpokeRequest;
import com.google.cloud.networkconnectivity.v1.AcceptHubSpokeResponse;
import com.google.cloud.networkconnectivity.v1.CreateHubRequest;
import com.google.cloud.networkconnectivity.v1.CreateSpokeRequest;
import com.google.cloud.networkconnectivity.v1.DeleteHubRequest;
import com.google.cloud.networkconnectivity.v1.DeleteSpokeRequest;
import com.google.cloud.networkconnectivity.v1.GetGroupRequest;
import com.google.cloud.networkconnectivity.v1.GetHubRequest;
import com.google.cloud.networkconnectivity.v1.GetRouteRequest;
import com.google.cloud.networkconnectivity.v1.GetRouteTableRequest;
import com.google.cloud.networkconnectivity.v1.GetSpokeRequest;
import com.google.cloud.networkconnectivity.v1.Group;
import com.google.cloud.networkconnectivity.v1.Hub;
import com.google.cloud.networkconnectivity.v1.HubStatusEntry;
import com.google.cloud.networkconnectivity.v1.ListGroupsRequest;
import com.google.cloud.networkconnectivity.v1.ListGroupsResponse;
import com.google.cloud.networkconnectivity.v1.ListHubSpokesRequest;
import com.google.cloud.networkconnectivity.v1.ListHubSpokesResponse;
import com.google.cloud.networkconnectivity.v1.ListHubsRequest;
import com.google.cloud.networkconnectivity.v1.ListHubsResponse;
import com.google.cloud.networkconnectivity.v1.ListRouteTablesRequest;
import com.google.cloud.networkconnectivity.v1.ListRouteTablesResponse;
import com.google.cloud.networkconnectivity.v1.ListRoutesRequest;
import com.google.cloud.networkconnectivity.v1.ListRoutesResponse;
import com.google.cloud.networkconnectivity.v1.ListSpokesRequest;
import com.google.cloud.networkconnectivity.v1.ListSpokesResponse;
import com.google.cloud.networkconnectivity.v1.OperationMetadata;
import com.google.cloud.networkconnectivity.v1.QueryHubStatusRequest;
import com.google.cloud.networkconnectivity.v1.QueryHubStatusResponse;
import com.google.cloud.networkconnectivity.v1.RejectHubSpokeRequest;
import com.google.cloud.networkconnectivity.v1.RejectHubSpokeResponse;
import com.google.cloud.networkconnectivity.v1.Route;
import com.google.cloud.networkconnectivity.v1.RouteTable;
import com.google.cloud.networkconnectivity.v1.Spoke;
import com.google.cloud.networkconnectivity.v1.UpdateGroupRequest;
import com.google.cloud.networkconnectivity.v1.UpdateHubRequest;
import com.google.cloud.networkconnectivity.v1.UpdateSpokeRequest;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.google.common.collect.Lists;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import java.io.IOException;
import java.time.Duration;
import java.util.List;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Settings class to configure an instance of {@link HubServiceStub}.
*
* The default instance has everything set to sensible defaults:
*
*
* - The default service address (networkconnectivity.googleapis.com) and default port (443) are
* used.
*
- Credentials are acquired automatically through Application Default Credentials.
*
- Retries are configured for idempotent methods but not for non-idempotent methods.
*
*
* The builder of this class is recursive, so contained classes are themselves builders. When
* build() is called, the tree of builders is called to create the complete settings object.
*
*
For example, to set the
* [RetrySettings](https://cloud.google.com/java/docs/reference/gax/latest/com.google.api.gax.retrying.RetrySettings)
* of getHub:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* HubServiceStubSettings.Builder hubServiceSettingsBuilder = HubServiceStubSettings.newBuilder();
* hubServiceSettingsBuilder
* .getHubSettings()
* .setRetrySettings(
* hubServiceSettingsBuilder
* .getHubSettings()
* .getRetrySettings()
* .toBuilder()
* .setInitialRetryDelayDuration(Duration.ofSeconds(1))
* .setInitialRpcTimeoutDuration(Duration.ofSeconds(5))
* .setMaxAttempts(5)
* .setMaxRetryDelayDuration(Duration.ofSeconds(30))
* .setMaxRpcTimeoutDuration(Duration.ofSeconds(60))
* .setRetryDelayMultiplier(1.3)
* .setRpcTimeoutMultiplier(1.5)
* .setTotalTimeoutDuration(Duration.ofSeconds(300))
* .build());
* HubServiceStubSettings hubServiceSettings = hubServiceSettingsBuilder.build();
* }
*
* Please refer to the [Client Side Retry
* Guide](https://github.com/googleapis/google-cloud-java/blob/main/docs/client_retries.md) for
* additional support in setting retries.
*
* To configure the RetrySettings of a Long Running Operation method, create an
* OperationTimedPollAlgorithm object and update the RPC's polling algorithm. For example, to
* configure the RetrySettings for createHub:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* HubServiceStubSettings.Builder hubServiceSettingsBuilder = HubServiceStubSettings.newBuilder();
* TimedRetryAlgorithm timedRetryAlgorithm =
* OperationalTimedPollAlgorithm.create(
* RetrySettings.newBuilder()
* .setInitialRetryDelayDuration(Duration.ofMillis(500))
* .setRetryDelayMultiplier(1.5)
* .setMaxRetryDelayDuration(Duration.ofMillis(5000))
* .setTotalTimeoutDuration(Duration.ofHours(24))
* .build());
* hubServiceSettingsBuilder
* .createClusterOperationSettings()
* .setPollingAlgorithm(timedRetryAlgorithm)
* .build();
* }
*/
@Generated("by gapic-generator-java")
public class HubServiceStubSettings extends StubSettings {
/** The default scopes of the service. */
private static final ImmutableList DEFAULT_SERVICE_SCOPES =
ImmutableList.builder().add("https://www.googleapis.com/auth/cloud-platform").build();
private final PagedCallSettings
listHubsSettings;
private final UnaryCallSettings getHubSettings;
private final UnaryCallSettings createHubSettings;
private final OperationCallSettings
createHubOperationSettings;
private final UnaryCallSettings updateHubSettings;
private final OperationCallSettings
updateHubOperationSettings;
private final UnaryCallSettings deleteHubSettings;
private final OperationCallSettings
deleteHubOperationSettings;
private final PagedCallSettings<
ListHubSpokesRequest, ListHubSpokesResponse, ListHubSpokesPagedResponse>
listHubSpokesSettings;
private final PagedCallSettings<
QueryHubStatusRequest, QueryHubStatusResponse, QueryHubStatusPagedResponse>
queryHubStatusSettings;
private final PagedCallSettings
listSpokesSettings;
private final UnaryCallSettings getSpokeSettings;
private final UnaryCallSettings createSpokeSettings;
private final OperationCallSettings
createSpokeOperationSettings;
private final UnaryCallSettings updateSpokeSettings;
private final OperationCallSettings
updateSpokeOperationSettings;
private final UnaryCallSettings rejectHubSpokeSettings;
private final OperationCallSettings<
RejectHubSpokeRequest, RejectHubSpokeResponse, OperationMetadata>
rejectHubSpokeOperationSettings;
private final UnaryCallSettings acceptHubSpokeSettings;
private final OperationCallSettings<
AcceptHubSpokeRequest, AcceptHubSpokeResponse, OperationMetadata>
acceptHubSpokeOperationSettings;
private final UnaryCallSettings deleteSpokeSettings;
private final OperationCallSettings
deleteSpokeOperationSettings;
private final UnaryCallSettings getRouteTableSettings;
private final UnaryCallSettings getRouteSettings;
private final PagedCallSettings
listRoutesSettings;
private final PagedCallSettings<
ListRouteTablesRequest, ListRouteTablesResponse, ListRouteTablesPagedResponse>
listRouteTablesSettings;
private final UnaryCallSettings getGroupSettings;
private final PagedCallSettings
listGroupsSettings;
private final UnaryCallSettings updateGroupSettings;
private final OperationCallSettings
updateGroupOperationSettings;
private final PagedCallSettings<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>
listLocationsSettings;
private final UnaryCallSettings getLocationSettings;
private final UnaryCallSettings setIamPolicySettings;
private final UnaryCallSettings getIamPolicySettings;
private final UnaryCallSettings
testIamPermissionsSettings;
private static final PagedListDescriptor
LIST_HUBS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListHubsRequest injectToken(ListHubsRequest payload, String token) {
return ListHubsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListHubsRequest injectPageSize(ListHubsRequest payload, int pageSize) {
return ListHubsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListHubsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListHubsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListHubsResponse payload) {
return payload.getHubsList();
}
};
private static final PagedListDescriptor
LIST_HUB_SPOKES_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListHubSpokesRequest injectToken(ListHubSpokesRequest payload, String token) {
return ListHubSpokesRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListHubSpokesRequest injectPageSize(ListHubSpokesRequest payload, int pageSize) {
return ListHubSpokesRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListHubSpokesRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListHubSpokesResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListHubSpokesResponse payload) {
return payload.getSpokesList();
}
};
private static final PagedListDescriptor<
QueryHubStatusRequest, QueryHubStatusResponse, HubStatusEntry>
QUERY_HUB_STATUS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public QueryHubStatusRequest injectToken(QueryHubStatusRequest payload, String token) {
return QueryHubStatusRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public QueryHubStatusRequest injectPageSize(
QueryHubStatusRequest payload, int pageSize) {
return QueryHubStatusRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(QueryHubStatusRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(QueryHubStatusResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(QueryHubStatusResponse payload) {
return payload.getHubStatusEntriesList();
}
};
private static final PagedListDescriptor
LIST_SPOKES_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListSpokesRequest injectToken(ListSpokesRequest payload, String token) {
return ListSpokesRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListSpokesRequest injectPageSize(ListSpokesRequest payload, int pageSize) {
return ListSpokesRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListSpokesRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListSpokesResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListSpokesResponse payload) {
return payload.getSpokesList();
}
};
private static final PagedListDescriptor
LIST_ROUTES_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListRoutesRequest injectToken(ListRoutesRequest payload, String token) {
return ListRoutesRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListRoutesRequest injectPageSize(ListRoutesRequest payload, int pageSize) {
return ListRoutesRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListRoutesRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListRoutesResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListRoutesResponse payload) {
return payload.getRoutesList();
}
};
private static final PagedListDescriptor<
ListRouteTablesRequest, ListRouteTablesResponse, RouteTable>
LIST_ROUTE_TABLES_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListRouteTablesRequest injectToken(
ListRouteTablesRequest payload, String token) {
return ListRouteTablesRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListRouteTablesRequest injectPageSize(
ListRouteTablesRequest payload, int pageSize) {
return ListRouteTablesRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListRouteTablesRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListRouteTablesResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListRouteTablesResponse payload) {
return payload.getRouteTablesList();
}
};
private static final PagedListDescriptor
LIST_GROUPS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListGroupsRequest injectToken(ListGroupsRequest payload, String token) {
return ListGroupsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListGroupsRequest injectPageSize(ListGroupsRequest payload, int pageSize) {
return ListGroupsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListGroupsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListGroupsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListGroupsResponse payload) {
return payload.getGroupsList();
}
};
private static final PagedListDescriptor
LIST_LOCATIONS_PAGE_STR_DESC =
new PagedListDescriptor() {
@Override
public String emptyToken() {
return "";
}
@Override
public ListLocationsRequest injectToken(ListLocationsRequest payload, String token) {
return ListLocationsRequest.newBuilder(payload).setPageToken(token).build();
}
@Override
public ListLocationsRequest injectPageSize(ListLocationsRequest payload, int pageSize) {
return ListLocationsRequest.newBuilder(payload).setPageSize(pageSize).build();
}
@Override
public Integer extractPageSize(ListLocationsRequest payload) {
return payload.getPageSize();
}
@Override
public String extractNextToken(ListLocationsResponse payload) {
return payload.getNextPageToken();
}
@Override
public Iterable extractResources(ListLocationsResponse payload) {
return payload.getLocationsList();
}
};
private static final PagedListResponseFactory<
ListHubsRequest, ListHubsResponse, ListHubsPagedResponse>
LIST_HUBS_PAGE_STR_FACT =
new PagedListResponseFactory() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListHubsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_HUBS_PAGE_STR_DESC, request, context);
return ListHubsPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListHubSpokesRequest, ListHubSpokesResponse, ListHubSpokesPagedResponse>
LIST_HUB_SPOKES_PAGE_STR_FACT =
new PagedListResponseFactory<
ListHubSpokesRequest, ListHubSpokesResponse, ListHubSpokesPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListHubSpokesRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_HUB_SPOKES_PAGE_STR_DESC, request, context);
return ListHubSpokesPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
QueryHubStatusRequest, QueryHubStatusResponse, QueryHubStatusPagedResponse>
QUERY_HUB_STATUS_PAGE_STR_FACT =
new PagedListResponseFactory<
QueryHubStatusRequest, QueryHubStatusResponse, QueryHubStatusPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
QueryHubStatusRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext
pageContext =
PageContext.create(
callable, QUERY_HUB_STATUS_PAGE_STR_DESC, request, context);
return QueryHubStatusPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListSpokesRequest, ListSpokesResponse, ListSpokesPagedResponse>
LIST_SPOKES_PAGE_STR_FACT =
new PagedListResponseFactory<
ListSpokesRequest, ListSpokesResponse, ListSpokesPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListSpokesRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_SPOKES_PAGE_STR_DESC, request, context);
return ListSpokesPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListRoutesRequest, ListRoutesResponse, ListRoutesPagedResponse>
LIST_ROUTES_PAGE_STR_FACT =
new PagedListResponseFactory<
ListRoutesRequest, ListRoutesResponse, ListRoutesPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListRoutesRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_ROUTES_PAGE_STR_DESC, request, context);
return ListRoutesPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListRouteTablesRequest, ListRouteTablesResponse, ListRouteTablesPagedResponse>
LIST_ROUTE_TABLES_PAGE_STR_FACT =
new PagedListResponseFactory<
ListRouteTablesRequest, ListRouteTablesResponse, ListRouteTablesPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListRouteTablesRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_ROUTE_TABLES_PAGE_STR_DESC, request, context);
return ListRouteTablesPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListGroupsRequest, ListGroupsResponse, ListGroupsPagedResponse>
LIST_GROUPS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListGroupsRequest, ListGroupsResponse, ListGroupsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListGroupsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_GROUPS_PAGE_STR_DESC, request, context);
return ListGroupsPagedResponse.createAsync(pageContext, futureResponse);
}
};
private static final PagedListResponseFactory<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>
LIST_LOCATIONS_PAGE_STR_FACT =
new PagedListResponseFactory<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>() {
@Override
public ApiFuture getFuturePagedResponse(
UnaryCallable callable,
ListLocationsRequest request,
ApiCallContext context,
ApiFuture futureResponse) {
PageContext pageContext =
PageContext.create(callable, LIST_LOCATIONS_PAGE_STR_DESC, request, context);
return ListLocationsPagedResponse.createAsync(pageContext, futureResponse);
}
};
/** Returns the object with the settings used for calls to listHubs. */
public PagedCallSettings
listHubsSettings() {
return listHubsSettings;
}
/** Returns the object with the settings used for calls to getHub. */
public UnaryCallSettings getHubSettings() {
return getHubSettings;
}
/** Returns the object with the settings used for calls to createHub. */
public UnaryCallSettings createHubSettings() {
return createHubSettings;
}
/** Returns the object with the settings used for calls to createHub. */
public OperationCallSettings
createHubOperationSettings() {
return createHubOperationSettings;
}
/** Returns the object with the settings used for calls to updateHub. */
public UnaryCallSettings updateHubSettings() {
return updateHubSettings;
}
/** Returns the object with the settings used for calls to updateHub. */
public OperationCallSettings
updateHubOperationSettings() {
return updateHubOperationSettings;
}
/** Returns the object with the settings used for calls to deleteHub. */
public UnaryCallSettings deleteHubSettings() {
return deleteHubSettings;
}
/** Returns the object with the settings used for calls to deleteHub. */
public OperationCallSettings
deleteHubOperationSettings() {
return deleteHubOperationSettings;
}
/** Returns the object with the settings used for calls to listHubSpokes. */
public PagedCallSettings
listHubSpokesSettings() {
return listHubSpokesSettings;
}
/** Returns the object with the settings used for calls to queryHubStatus. */
public PagedCallSettings<
QueryHubStatusRequest, QueryHubStatusResponse, QueryHubStatusPagedResponse>
queryHubStatusSettings() {
return queryHubStatusSettings;
}
/** Returns the object with the settings used for calls to listSpokes. */
public PagedCallSettings
listSpokesSettings() {
return listSpokesSettings;
}
/** Returns the object with the settings used for calls to getSpoke. */
public UnaryCallSettings getSpokeSettings() {
return getSpokeSettings;
}
/** Returns the object with the settings used for calls to createSpoke. */
public UnaryCallSettings createSpokeSettings() {
return createSpokeSettings;
}
/** Returns the object with the settings used for calls to createSpoke. */
public OperationCallSettings
createSpokeOperationSettings() {
return createSpokeOperationSettings;
}
/** Returns the object with the settings used for calls to updateSpoke. */
public UnaryCallSettings updateSpokeSettings() {
return updateSpokeSettings;
}
/** Returns the object with the settings used for calls to updateSpoke. */
public OperationCallSettings
updateSpokeOperationSettings() {
return updateSpokeOperationSettings;
}
/** Returns the object with the settings used for calls to rejectHubSpoke. */
public UnaryCallSettings rejectHubSpokeSettings() {
return rejectHubSpokeSettings;
}
/** Returns the object with the settings used for calls to rejectHubSpoke. */
public OperationCallSettings
rejectHubSpokeOperationSettings() {
return rejectHubSpokeOperationSettings;
}
/** Returns the object with the settings used for calls to acceptHubSpoke. */
public UnaryCallSettings acceptHubSpokeSettings() {
return acceptHubSpokeSettings;
}
/** Returns the object with the settings used for calls to acceptHubSpoke. */
public OperationCallSettings
acceptHubSpokeOperationSettings() {
return acceptHubSpokeOperationSettings;
}
/** Returns the object with the settings used for calls to deleteSpoke. */
public UnaryCallSettings deleteSpokeSettings() {
return deleteSpokeSettings;
}
/** Returns the object with the settings used for calls to deleteSpoke. */
public OperationCallSettings
deleteSpokeOperationSettings() {
return deleteSpokeOperationSettings;
}
/** Returns the object with the settings used for calls to getRouteTable. */
public UnaryCallSettings getRouteTableSettings() {
return getRouteTableSettings;
}
/** Returns the object with the settings used for calls to getRoute. */
public UnaryCallSettings getRouteSettings() {
return getRouteSettings;
}
/** Returns the object with the settings used for calls to listRoutes. */
public PagedCallSettings
listRoutesSettings() {
return listRoutesSettings;
}
/** Returns the object with the settings used for calls to listRouteTables. */
public PagedCallSettings<
ListRouteTablesRequest, ListRouteTablesResponse, ListRouteTablesPagedResponse>
listRouteTablesSettings() {
return listRouteTablesSettings;
}
/** Returns the object with the settings used for calls to getGroup. */
public UnaryCallSettings getGroupSettings() {
return getGroupSettings;
}
/** Returns the object with the settings used for calls to listGroups. */
public PagedCallSettings
listGroupsSettings() {
return listGroupsSettings;
}
/** Returns the object with the settings used for calls to updateGroup. */
public UnaryCallSettings updateGroupSettings() {
return updateGroupSettings;
}
/** Returns the object with the settings used for calls to updateGroup. */
public OperationCallSettings
updateGroupOperationSettings() {
return updateGroupOperationSettings;
}
/** Returns the object with the settings used for calls to listLocations. */
public PagedCallSettings
listLocationsSettings() {
return listLocationsSettings;
}
/** Returns the object with the settings used for calls to getLocation. */
public UnaryCallSettings getLocationSettings() {
return getLocationSettings;
}
/** Returns the object with the settings used for calls to setIamPolicy. */
public UnaryCallSettings setIamPolicySettings() {
return setIamPolicySettings;
}
/** Returns the object with the settings used for calls to getIamPolicy. */
public UnaryCallSettings getIamPolicySettings() {
return getIamPolicySettings;
}
/** Returns the object with the settings used for calls to testIamPermissions. */
public UnaryCallSettings
testIamPermissionsSettings() {
return testIamPermissionsSettings;
}
public HubServiceStub createStub() throws IOException {
if (getTransportChannelProvider()
.getTransportName()
.equals(GrpcTransportChannel.getGrpcTransportName())) {
return GrpcHubServiceStub.create(this);
}
throw new UnsupportedOperationException(
String.format(
"Transport not supported: %s", getTransportChannelProvider().getTransportName()));
}
/** Returns the default service name. */
@Override
public String getServiceName() {
return "networkconnectivity";
}
/** Returns a builder for the default ExecutorProvider for this service. */
public static InstantiatingExecutorProvider.Builder defaultExecutorProviderBuilder() {
return InstantiatingExecutorProvider.newBuilder();
}
/** Returns the default service endpoint. */
@ObsoleteApi("Use getEndpoint() instead")
public static String getDefaultEndpoint() {
return "networkconnectivity.googleapis.com:443";
}
/** Returns the default mTLS service endpoint. */
public static String getDefaultMtlsEndpoint() {
return "networkconnectivity.mtls.googleapis.com:443";
}
/** Returns the default service scopes. */
public static List getDefaultServiceScopes() {
return DEFAULT_SERVICE_SCOPES;
}
/** Returns a builder for the default credentials for this service. */
public static GoogleCredentialsProvider.Builder defaultCredentialsProviderBuilder() {
return GoogleCredentialsProvider.newBuilder()
.setScopesToApply(DEFAULT_SERVICE_SCOPES)
.setUseJwtAccessWithScope(true);
}
/** Returns a builder for the default ChannelProvider for this service. */
public static InstantiatingGrpcChannelProvider.Builder defaultGrpcTransportProviderBuilder() {
return InstantiatingGrpcChannelProvider.newBuilder()
.setMaxInboundMessageSize(Integer.MAX_VALUE);
}
public static TransportChannelProvider defaultTransportChannelProvider() {
return defaultGrpcTransportProviderBuilder().build();
}
public static ApiClientHeaderProvider.Builder defaultApiClientHeaderProviderBuilder() {
return ApiClientHeaderProvider.newBuilder()
.setGeneratedLibToken(
"gapic", GaxProperties.getLibraryVersion(HubServiceStubSettings.class))
.setTransportToken(
GaxGrpcProperties.getGrpcTokenName(), GaxGrpcProperties.getGrpcVersion());
}
/** Returns a new builder for this class. */
public static Builder newBuilder() {
return Builder.createDefault();
}
/** Returns a new builder for this class. */
public static Builder newBuilder(ClientContext clientContext) {
return new Builder(clientContext);
}
/** Returns a builder containing all the values of this settings class. */
public Builder toBuilder() {
return new Builder(this);
}
protected HubServiceStubSettings(Builder settingsBuilder) throws IOException {
super(settingsBuilder);
listHubsSettings = settingsBuilder.listHubsSettings().build();
getHubSettings = settingsBuilder.getHubSettings().build();
createHubSettings = settingsBuilder.createHubSettings().build();
createHubOperationSettings = settingsBuilder.createHubOperationSettings().build();
updateHubSettings = settingsBuilder.updateHubSettings().build();
updateHubOperationSettings = settingsBuilder.updateHubOperationSettings().build();
deleteHubSettings = settingsBuilder.deleteHubSettings().build();
deleteHubOperationSettings = settingsBuilder.deleteHubOperationSettings().build();
listHubSpokesSettings = settingsBuilder.listHubSpokesSettings().build();
queryHubStatusSettings = settingsBuilder.queryHubStatusSettings().build();
listSpokesSettings = settingsBuilder.listSpokesSettings().build();
getSpokeSettings = settingsBuilder.getSpokeSettings().build();
createSpokeSettings = settingsBuilder.createSpokeSettings().build();
createSpokeOperationSettings = settingsBuilder.createSpokeOperationSettings().build();
updateSpokeSettings = settingsBuilder.updateSpokeSettings().build();
updateSpokeOperationSettings = settingsBuilder.updateSpokeOperationSettings().build();
rejectHubSpokeSettings = settingsBuilder.rejectHubSpokeSettings().build();
rejectHubSpokeOperationSettings = settingsBuilder.rejectHubSpokeOperationSettings().build();
acceptHubSpokeSettings = settingsBuilder.acceptHubSpokeSettings().build();
acceptHubSpokeOperationSettings = settingsBuilder.acceptHubSpokeOperationSettings().build();
deleteSpokeSettings = settingsBuilder.deleteSpokeSettings().build();
deleteSpokeOperationSettings = settingsBuilder.deleteSpokeOperationSettings().build();
getRouteTableSettings = settingsBuilder.getRouteTableSettings().build();
getRouteSettings = settingsBuilder.getRouteSettings().build();
listRoutesSettings = settingsBuilder.listRoutesSettings().build();
listRouteTablesSettings = settingsBuilder.listRouteTablesSettings().build();
getGroupSettings = settingsBuilder.getGroupSettings().build();
listGroupsSettings = settingsBuilder.listGroupsSettings().build();
updateGroupSettings = settingsBuilder.updateGroupSettings().build();
updateGroupOperationSettings = settingsBuilder.updateGroupOperationSettings().build();
listLocationsSettings = settingsBuilder.listLocationsSettings().build();
getLocationSettings = settingsBuilder.getLocationSettings().build();
setIamPolicySettings = settingsBuilder.setIamPolicySettings().build();
getIamPolicySettings = settingsBuilder.getIamPolicySettings().build();
testIamPermissionsSettings = settingsBuilder.testIamPermissionsSettings().build();
}
/** Builder for HubServiceStubSettings. */
public static class Builder extends StubSettings.Builder {
private final ImmutableList> unaryMethodSettingsBuilders;
private final PagedCallSettings.Builder<
ListHubsRequest, ListHubsResponse, ListHubsPagedResponse>
listHubsSettings;
private final UnaryCallSettings.Builder getHubSettings;
private final UnaryCallSettings.Builder createHubSettings;
private final OperationCallSettings.Builder
createHubOperationSettings;
private final UnaryCallSettings.Builder updateHubSettings;
private final OperationCallSettings.Builder
updateHubOperationSettings;
private final UnaryCallSettings.Builder deleteHubSettings;
private final OperationCallSettings.Builder
deleteHubOperationSettings;
private final PagedCallSettings.Builder<
ListHubSpokesRequest, ListHubSpokesResponse, ListHubSpokesPagedResponse>
listHubSpokesSettings;
private final PagedCallSettings.Builder<
QueryHubStatusRequest, QueryHubStatusResponse, QueryHubStatusPagedResponse>
queryHubStatusSettings;
private final PagedCallSettings.Builder<
ListSpokesRequest, ListSpokesResponse, ListSpokesPagedResponse>
listSpokesSettings;
private final UnaryCallSettings.Builder getSpokeSettings;
private final UnaryCallSettings.Builder createSpokeSettings;
private final OperationCallSettings.Builder
createSpokeOperationSettings;
private final UnaryCallSettings.Builder updateSpokeSettings;
private final OperationCallSettings.Builder
updateSpokeOperationSettings;
private final UnaryCallSettings.Builder
rejectHubSpokeSettings;
private final OperationCallSettings.Builder<
RejectHubSpokeRequest, RejectHubSpokeResponse, OperationMetadata>
rejectHubSpokeOperationSettings;
private final UnaryCallSettings.Builder
acceptHubSpokeSettings;
private final OperationCallSettings.Builder<
AcceptHubSpokeRequest, AcceptHubSpokeResponse, OperationMetadata>
acceptHubSpokeOperationSettings;
private final UnaryCallSettings.Builder deleteSpokeSettings;
private final OperationCallSettings.Builder
deleteSpokeOperationSettings;
private final UnaryCallSettings.Builder getRouteTableSettings;
private final UnaryCallSettings.Builder getRouteSettings;
private final PagedCallSettings.Builder<
ListRoutesRequest, ListRoutesResponse, ListRoutesPagedResponse>
listRoutesSettings;
private final PagedCallSettings.Builder<
ListRouteTablesRequest, ListRouteTablesResponse, ListRouteTablesPagedResponse>
listRouteTablesSettings;
private final UnaryCallSettings.Builder getGroupSettings;
private final PagedCallSettings.Builder<
ListGroupsRequest, ListGroupsResponse, ListGroupsPagedResponse>
listGroupsSettings;
private final UnaryCallSettings.Builder updateGroupSettings;
private final OperationCallSettings.Builder
updateGroupOperationSettings;
private final PagedCallSettings.Builder<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>
listLocationsSettings;
private final UnaryCallSettings.Builder getLocationSettings;
private final UnaryCallSettings.Builder setIamPolicySettings;
private final UnaryCallSettings.Builder getIamPolicySettings;
private final UnaryCallSettings.Builder
testIamPermissionsSettings;
private static final ImmutableMap>
RETRYABLE_CODE_DEFINITIONS;
static {
ImmutableMap.Builder> definitions =
ImmutableMap.builder();
definitions.put(
"retry_policy_0_codes",
ImmutableSet.copyOf(Lists.newArrayList(StatusCode.Code.UNAVAILABLE)));
definitions.put(
"no_retry_1_codes", ImmutableSet.copyOf(Lists.newArrayList()));
RETRYABLE_CODE_DEFINITIONS = definitions.build();
}
private static final ImmutableMap RETRY_PARAM_DEFINITIONS;
static {
ImmutableMap.Builder definitions = ImmutableMap.builder();
RetrySettings settings = null;
settings =
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(1000L))
.setRetryDelayMultiplier(1.3)
.setMaxRetryDelayDuration(Duration.ofMillis(10000L))
.setInitialRpcTimeoutDuration(Duration.ofMillis(60000L))
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ofMillis(60000L))
.setTotalTimeoutDuration(Duration.ofMillis(60000L))
.build();
definitions.put("retry_policy_0_params", settings);
settings =
RetrySettings.newBuilder()
.setInitialRpcTimeoutDuration(Duration.ofMillis(60000L))
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ofMillis(60000L))
.setTotalTimeoutDuration(Duration.ofMillis(60000L))
.build();
definitions.put("no_retry_1_params", settings);
RETRY_PARAM_DEFINITIONS = definitions.build();
}
protected Builder() {
this(((ClientContext) null));
}
protected Builder(ClientContext clientContext) {
super(clientContext);
listHubsSettings = PagedCallSettings.newBuilder(LIST_HUBS_PAGE_STR_FACT);
getHubSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createHubSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createHubOperationSettings = OperationCallSettings.newBuilder();
updateHubSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateHubOperationSettings = OperationCallSettings.newBuilder();
deleteHubSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteHubOperationSettings = OperationCallSettings.newBuilder();
listHubSpokesSettings = PagedCallSettings.newBuilder(LIST_HUB_SPOKES_PAGE_STR_FACT);
queryHubStatusSettings = PagedCallSettings.newBuilder(QUERY_HUB_STATUS_PAGE_STR_FACT);
listSpokesSettings = PagedCallSettings.newBuilder(LIST_SPOKES_PAGE_STR_FACT);
getSpokeSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createSpokeSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
createSpokeOperationSettings = OperationCallSettings.newBuilder();
updateSpokeSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateSpokeOperationSettings = OperationCallSettings.newBuilder();
rejectHubSpokeSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
rejectHubSpokeOperationSettings = OperationCallSettings.newBuilder();
acceptHubSpokeSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
acceptHubSpokeOperationSettings = OperationCallSettings.newBuilder();
deleteSpokeSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
deleteSpokeOperationSettings = OperationCallSettings.newBuilder();
getRouteTableSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
getRouteSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
listRoutesSettings = PagedCallSettings.newBuilder(LIST_ROUTES_PAGE_STR_FACT);
listRouteTablesSettings = PagedCallSettings.newBuilder(LIST_ROUTE_TABLES_PAGE_STR_FACT);
getGroupSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
listGroupsSettings = PagedCallSettings.newBuilder(LIST_GROUPS_PAGE_STR_FACT);
updateGroupSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
updateGroupOperationSettings = OperationCallSettings.newBuilder();
listLocationsSettings = PagedCallSettings.newBuilder(LIST_LOCATIONS_PAGE_STR_FACT);
getLocationSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
setIamPolicySettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
getIamPolicySettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
testIamPermissionsSettings = UnaryCallSettings.newUnaryCallSettingsBuilder();
unaryMethodSettingsBuilders =
ImmutableList.>of(
listHubsSettings,
getHubSettings,
createHubSettings,
updateHubSettings,
deleteHubSettings,
listHubSpokesSettings,
queryHubStatusSettings,
listSpokesSettings,
getSpokeSettings,
createSpokeSettings,
updateSpokeSettings,
rejectHubSpokeSettings,
acceptHubSpokeSettings,
deleteSpokeSettings,
getRouteTableSettings,
getRouteSettings,
listRoutesSettings,
listRouteTablesSettings,
getGroupSettings,
listGroupsSettings,
updateGroupSettings,
listLocationsSettings,
getLocationSettings,
setIamPolicySettings,
getIamPolicySettings,
testIamPermissionsSettings);
initDefaults(this);
}
protected Builder(HubServiceStubSettings settings) {
super(settings);
listHubsSettings = settings.listHubsSettings.toBuilder();
getHubSettings = settings.getHubSettings.toBuilder();
createHubSettings = settings.createHubSettings.toBuilder();
createHubOperationSettings = settings.createHubOperationSettings.toBuilder();
updateHubSettings = settings.updateHubSettings.toBuilder();
updateHubOperationSettings = settings.updateHubOperationSettings.toBuilder();
deleteHubSettings = settings.deleteHubSettings.toBuilder();
deleteHubOperationSettings = settings.deleteHubOperationSettings.toBuilder();
listHubSpokesSettings = settings.listHubSpokesSettings.toBuilder();
queryHubStatusSettings = settings.queryHubStatusSettings.toBuilder();
listSpokesSettings = settings.listSpokesSettings.toBuilder();
getSpokeSettings = settings.getSpokeSettings.toBuilder();
createSpokeSettings = settings.createSpokeSettings.toBuilder();
createSpokeOperationSettings = settings.createSpokeOperationSettings.toBuilder();
updateSpokeSettings = settings.updateSpokeSettings.toBuilder();
updateSpokeOperationSettings = settings.updateSpokeOperationSettings.toBuilder();
rejectHubSpokeSettings = settings.rejectHubSpokeSettings.toBuilder();
rejectHubSpokeOperationSettings = settings.rejectHubSpokeOperationSettings.toBuilder();
acceptHubSpokeSettings = settings.acceptHubSpokeSettings.toBuilder();
acceptHubSpokeOperationSettings = settings.acceptHubSpokeOperationSettings.toBuilder();
deleteSpokeSettings = settings.deleteSpokeSettings.toBuilder();
deleteSpokeOperationSettings = settings.deleteSpokeOperationSettings.toBuilder();
getRouteTableSettings = settings.getRouteTableSettings.toBuilder();
getRouteSettings = settings.getRouteSettings.toBuilder();
listRoutesSettings = settings.listRoutesSettings.toBuilder();
listRouteTablesSettings = settings.listRouteTablesSettings.toBuilder();
getGroupSettings = settings.getGroupSettings.toBuilder();
listGroupsSettings = settings.listGroupsSettings.toBuilder();
updateGroupSettings = settings.updateGroupSettings.toBuilder();
updateGroupOperationSettings = settings.updateGroupOperationSettings.toBuilder();
listLocationsSettings = settings.listLocationsSettings.toBuilder();
getLocationSettings = settings.getLocationSettings.toBuilder();
setIamPolicySettings = settings.setIamPolicySettings.toBuilder();
getIamPolicySettings = settings.getIamPolicySettings.toBuilder();
testIamPermissionsSettings = settings.testIamPermissionsSettings.toBuilder();
unaryMethodSettingsBuilders =
ImmutableList.>of(
listHubsSettings,
getHubSettings,
createHubSettings,
updateHubSettings,
deleteHubSettings,
listHubSpokesSettings,
queryHubStatusSettings,
listSpokesSettings,
getSpokeSettings,
createSpokeSettings,
updateSpokeSettings,
rejectHubSpokeSettings,
acceptHubSpokeSettings,
deleteSpokeSettings,
getRouteTableSettings,
getRouteSettings,
listRoutesSettings,
listRouteTablesSettings,
getGroupSettings,
listGroupsSettings,
updateGroupSettings,
listLocationsSettings,
getLocationSettings,
setIamPolicySettings,
getIamPolicySettings,
testIamPermissionsSettings);
}
private static Builder createDefault() {
Builder builder = new Builder(((ClientContext) null));
builder.setTransportChannelProvider(defaultTransportChannelProvider());
builder.setCredentialsProvider(defaultCredentialsProviderBuilder().build());
builder.setInternalHeaderProvider(defaultApiClientHeaderProviderBuilder().build());
builder.setMtlsEndpoint(getDefaultMtlsEndpoint());
builder.setSwitchToMtlsEndpointAllowed(true);
return initDefaults(builder);
}
private static Builder initDefaults(Builder builder) {
builder
.listHubsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.getHubSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.createHubSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.updateHubSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.deleteHubSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.listHubSpokesSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.queryHubStatusSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.listSpokesSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.getSpokeSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.createSpokeSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.updateSpokeSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.rejectHubSpokeSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.acceptHubSpokeSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.deleteSpokeSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"));
builder
.getRouteTableSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.getRouteSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.listRoutesSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.listRouteTablesSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.getGroupSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.listGroupsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.updateGroupSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.listLocationsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.getLocationSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.setIamPolicySettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.getIamPolicySettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.testIamPermissionsSettings()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"));
builder
.createHubOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(ProtoOperationTransformers.ResponseTransformer.create(Hub.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelayDuration(Duration.ofMillis(45000L))
.setInitialRpcTimeoutDuration(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ZERO)
.setTotalTimeoutDuration(Duration.ofMillis(300000L))
.build()));
builder
.updateHubOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(ProtoOperationTransformers.ResponseTransformer.create(Hub.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelayDuration(Duration.ofMillis(45000L))
.setInitialRpcTimeoutDuration(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ZERO)
.setTotalTimeoutDuration(Duration.ofMillis(300000L))
.build()));
builder
.deleteHubOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Empty.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelayDuration(Duration.ofMillis(45000L))
.setInitialRpcTimeoutDuration(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ZERO)
.setTotalTimeoutDuration(Duration.ofMillis(300000L))
.build()));
builder
.createSpokeOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Spoke.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelayDuration(Duration.ofMillis(45000L))
.setInitialRpcTimeoutDuration(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ZERO)
.setTotalTimeoutDuration(Duration.ofMillis(300000L))
.build()));
builder
.updateSpokeOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Spoke.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelayDuration(Duration.ofMillis(45000L))
.setInitialRpcTimeoutDuration(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ZERO)
.setTotalTimeoutDuration(Duration.ofMillis(300000L))
.build()));
builder
.rejectHubSpokeOperationSettings()
.setInitialCallSettings(
UnaryCallSettings
.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(RejectHubSpokeResponse.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelayDuration(Duration.ofMillis(45000L))
.setInitialRpcTimeoutDuration(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ZERO)
.setTotalTimeoutDuration(Duration.ofMillis(300000L))
.build()));
builder
.acceptHubSpokeOperationSettings()
.setInitialCallSettings(
UnaryCallSettings
.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(AcceptHubSpokeResponse.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelayDuration(Duration.ofMillis(45000L))
.setInitialRpcTimeoutDuration(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ZERO)
.setTotalTimeoutDuration(Duration.ofMillis(300000L))
.build()));
builder
.deleteSpokeOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("no_retry_1_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("no_retry_1_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Empty.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelayDuration(Duration.ofMillis(45000L))
.setInitialRpcTimeoutDuration(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ZERO)
.setTotalTimeoutDuration(Duration.ofMillis(300000L))
.build()));
builder
.updateGroupOperationSettings()
.setInitialCallSettings(
UnaryCallSettings.newUnaryCallSettingsBuilder()
.setRetryableCodes(RETRYABLE_CODE_DEFINITIONS.get("retry_policy_0_codes"))
.setRetrySettings(RETRY_PARAM_DEFINITIONS.get("retry_policy_0_params"))
.build())
.setResponseTransformer(
ProtoOperationTransformers.ResponseTransformer.create(Group.class))
.setMetadataTransformer(
ProtoOperationTransformers.MetadataTransformer.create(OperationMetadata.class))
.setPollingAlgorithm(
OperationTimedPollAlgorithm.create(
RetrySettings.newBuilder()
.setInitialRetryDelayDuration(Duration.ofMillis(5000L))
.setRetryDelayMultiplier(1.5)
.setMaxRetryDelayDuration(Duration.ofMillis(45000L))
.setInitialRpcTimeoutDuration(Duration.ZERO)
.setRpcTimeoutMultiplier(1.0)
.setMaxRpcTimeoutDuration(Duration.ZERO)
.setTotalTimeoutDuration(Duration.ofMillis(300000L))
.build()));
return builder;
}
/**
* Applies the given settings updater function to all of the unary API methods in this service.
*
* Note: This method does not support applying settings to streaming methods.
*/
public Builder applyToAllUnaryMethods(
ApiFunction, Void> settingsUpdater) {
super.applyToAllUnaryMethods(unaryMethodSettingsBuilders, settingsUpdater);
return this;
}
public ImmutableList> unaryMethodSettingsBuilders() {
return unaryMethodSettingsBuilders;
}
/** Returns the builder for the settings used for calls to listHubs. */
public PagedCallSettings.Builder
listHubsSettings() {
return listHubsSettings;
}
/** Returns the builder for the settings used for calls to getHub. */
public UnaryCallSettings.Builder getHubSettings() {
return getHubSettings;
}
/** Returns the builder for the settings used for calls to createHub. */
public UnaryCallSettings.Builder createHubSettings() {
return createHubSettings;
}
/** Returns the builder for the settings used for calls to createHub. */
public OperationCallSettings.Builder
createHubOperationSettings() {
return createHubOperationSettings;
}
/** Returns the builder for the settings used for calls to updateHub. */
public UnaryCallSettings.Builder updateHubSettings() {
return updateHubSettings;
}
/** Returns the builder for the settings used for calls to updateHub. */
public OperationCallSettings.Builder
updateHubOperationSettings() {
return updateHubOperationSettings;
}
/** Returns the builder for the settings used for calls to deleteHub. */
public UnaryCallSettings.Builder deleteHubSettings() {
return deleteHubSettings;
}
/** Returns the builder for the settings used for calls to deleteHub. */
public OperationCallSettings.Builder
deleteHubOperationSettings() {
return deleteHubOperationSettings;
}
/** Returns the builder for the settings used for calls to listHubSpokes. */
public PagedCallSettings.Builder<
ListHubSpokesRequest, ListHubSpokesResponse, ListHubSpokesPagedResponse>
listHubSpokesSettings() {
return listHubSpokesSettings;
}
/** Returns the builder for the settings used for calls to queryHubStatus. */
public PagedCallSettings.Builder<
QueryHubStatusRequest, QueryHubStatusResponse, QueryHubStatusPagedResponse>
queryHubStatusSettings() {
return queryHubStatusSettings;
}
/** Returns the builder for the settings used for calls to listSpokes. */
public PagedCallSettings.Builder
listSpokesSettings() {
return listSpokesSettings;
}
/** Returns the builder for the settings used for calls to getSpoke. */
public UnaryCallSettings.Builder getSpokeSettings() {
return getSpokeSettings;
}
/** Returns the builder for the settings used for calls to createSpoke. */
public UnaryCallSettings.Builder createSpokeSettings() {
return createSpokeSettings;
}
/** Returns the builder for the settings used for calls to createSpoke. */
public OperationCallSettings.Builder
createSpokeOperationSettings() {
return createSpokeOperationSettings;
}
/** Returns the builder for the settings used for calls to updateSpoke. */
public UnaryCallSettings.Builder updateSpokeSettings() {
return updateSpokeSettings;
}
/** Returns the builder for the settings used for calls to updateSpoke. */
public OperationCallSettings.Builder
updateSpokeOperationSettings() {
return updateSpokeOperationSettings;
}
/** Returns the builder for the settings used for calls to rejectHubSpoke. */
public UnaryCallSettings.Builder rejectHubSpokeSettings() {
return rejectHubSpokeSettings;
}
/** Returns the builder for the settings used for calls to rejectHubSpoke. */
public OperationCallSettings.Builder<
RejectHubSpokeRequest, RejectHubSpokeResponse, OperationMetadata>
rejectHubSpokeOperationSettings() {
return rejectHubSpokeOperationSettings;
}
/** Returns the builder for the settings used for calls to acceptHubSpoke. */
public UnaryCallSettings.Builder acceptHubSpokeSettings() {
return acceptHubSpokeSettings;
}
/** Returns the builder for the settings used for calls to acceptHubSpoke. */
public OperationCallSettings.Builder<
AcceptHubSpokeRequest, AcceptHubSpokeResponse, OperationMetadata>
acceptHubSpokeOperationSettings() {
return acceptHubSpokeOperationSettings;
}
/** Returns the builder for the settings used for calls to deleteSpoke. */
public UnaryCallSettings.Builder deleteSpokeSettings() {
return deleteSpokeSettings;
}
/** Returns the builder for the settings used for calls to deleteSpoke. */
public OperationCallSettings.Builder
deleteSpokeOperationSettings() {
return deleteSpokeOperationSettings;
}
/** Returns the builder for the settings used for calls to getRouteTable. */
public UnaryCallSettings.Builder getRouteTableSettings() {
return getRouteTableSettings;
}
/** Returns the builder for the settings used for calls to getRoute. */
public UnaryCallSettings.Builder getRouteSettings() {
return getRouteSettings;
}
/** Returns the builder for the settings used for calls to listRoutes. */
public PagedCallSettings.Builder
listRoutesSettings() {
return listRoutesSettings;
}
/** Returns the builder for the settings used for calls to listRouteTables. */
public PagedCallSettings.Builder<
ListRouteTablesRequest, ListRouteTablesResponse, ListRouteTablesPagedResponse>
listRouteTablesSettings() {
return listRouteTablesSettings;
}
/** Returns the builder for the settings used for calls to getGroup. */
public UnaryCallSettings.Builder getGroupSettings() {
return getGroupSettings;
}
/** Returns the builder for the settings used for calls to listGroups. */
public PagedCallSettings.Builder
listGroupsSettings() {
return listGroupsSettings;
}
/** Returns the builder for the settings used for calls to updateGroup. */
public UnaryCallSettings.Builder updateGroupSettings() {
return updateGroupSettings;
}
/** Returns the builder for the settings used for calls to updateGroup. */
public OperationCallSettings.Builder
updateGroupOperationSettings() {
return updateGroupOperationSettings;
}
/** Returns the builder for the settings used for calls to listLocations. */
public PagedCallSettings.Builder<
ListLocationsRequest, ListLocationsResponse, ListLocationsPagedResponse>
listLocationsSettings() {
return listLocationsSettings;
}
/** Returns the builder for the settings used for calls to getLocation. */
public UnaryCallSettings.Builder getLocationSettings() {
return getLocationSettings;
}
/** Returns the builder for the settings used for calls to setIamPolicy. */
public UnaryCallSettings.Builder setIamPolicySettings() {
return setIamPolicySettings;
}
/** Returns the builder for the settings used for calls to getIamPolicy. */
public UnaryCallSettings.Builder getIamPolicySettings() {
return getIamPolicySettings;
}
/** Returns the builder for the settings used for calls to testIamPermissions. */
public UnaryCallSettings.Builder
testIamPermissionsSettings() {
return testIamPermissionsSettings;
}
@Override
public HubServiceStubSettings build() throws IOException {
return new HubServiceStubSettings(this);
}
}
}