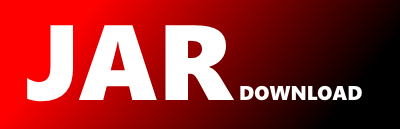
com.google.cloud.notebooks.v1.ManagedNotebookServiceClient Maven / Gradle / Ivy
Show all versions of google-cloud-notebooks Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.notebooks.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.notebooks.v1.stub.ManagedNotebookServiceStub;
import com.google.cloud.notebooks.v1.stub.ManagedNotebookServiceStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.longrunning.OperationsClient;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: API v1 service for Managed Notebooks.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* RuntimeName name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]");
* Runtime response = managedNotebookServiceClient.getRuntime(name);
* }
* }
*
* Note: close() needs to be called on the ManagedNotebookServiceClient object to clean up
* resources such as threads. In the example above, try-with-resources is used, which automatically
* calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* ListRuntimes
* Lists Runtimes in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listRuntimes(ListRuntimesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listRuntimes(LocationName parent)
*
listRuntimes(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listRuntimesPagedCallable()
*
listRuntimesCallable()
*
*
*
*
* GetRuntime
* Gets details of a single Runtime. The location must be a regional endpoint rather than zonal.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getRuntime(GetRuntimeRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getRuntime(RuntimeName name)
*
getRuntime(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getRuntimeCallable()
*
*
*
*
* CreateRuntime
* Creates a new Runtime in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createRuntimeAsync(CreateRuntimeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createRuntimeAsync(RuntimeName parent, String runtimeId, Runtime runtime)
*
createRuntimeAsync(String parent, String runtimeId, Runtime runtime)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createRuntimeOperationCallable()
*
createRuntimeCallable()
*
*
*
*
* UpdateRuntime
* Update Notebook Runtime configuration.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateRuntimeAsync(UpdateRuntimeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateRuntimeAsync(Runtime runtime, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateRuntimeOperationCallable()
*
updateRuntimeCallable()
*
*
*
*
* DeleteRuntime
* Deletes a single Runtime.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteRuntimeAsync(DeleteRuntimeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteRuntimeAsync(RuntimeName name)
*
deleteRuntimeAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteRuntimeOperationCallable()
*
deleteRuntimeCallable()
*
*
*
*
* StartRuntime
* Starts a Managed Notebook Runtime. Perform "Start" on GPU instances; "Resume" on CPU instances See: https://cloud.google.com/compute/docs/instances/stop-start-instance https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* startRuntimeAsync(StartRuntimeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* startRuntimeAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* startRuntimeOperationCallable()
*
startRuntimeCallable()
*
*
*
*
* StopRuntime
* Stops a Managed Notebook Runtime. Perform "Stop" on GPU instances; "Suspend" on CPU instances See: https://cloud.google.com/compute/docs/instances/stop-start-instance https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* stopRuntimeAsync(StopRuntimeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* stopRuntimeAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* stopRuntimeOperationCallable()
*
stopRuntimeCallable()
*
*
*
*
* SwitchRuntime
* Switch a Managed Notebook Runtime.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* switchRuntimeAsync(SwitchRuntimeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* switchRuntimeAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* switchRuntimeOperationCallable()
*
switchRuntimeCallable()
*
*
*
*
* ResetRuntime
* Resets a Managed Notebook Runtime.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* resetRuntimeAsync(ResetRuntimeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* resetRuntimeAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* resetRuntimeOperationCallable()
*
resetRuntimeCallable()
*
*
*
*
* UpgradeRuntime
* Upgrades a Managed Notebook Runtime to the latest version.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* upgradeRuntimeAsync(UpgradeRuntimeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* upgradeRuntimeAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* upgradeRuntimeOperationCallable()
*
upgradeRuntimeCallable()
*
*
*
*
* ReportRuntimeEvent
* Report and process a runtime event.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* reportRuntimeEventAsync(ReportRuntimeEventRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* reportRuntimeEventAsync(RuntimeName name)
*
reportRuntimeEventAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* reportRuntimeEventOperationCallable()
*
reportRuntimeEventCallable()
*
*
*
*
* RefreshRuntimeTokenInternal
* Gets an access token for the consumer service account that the customer attached to the runtime. Only accessible from the tenant instance.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* refreshRuntimeTokenInternal(RefreshRuntimeTokenInternalRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* refreshRuntimeTokenInternal(RuntimeName name, String vmId)
*
refreshRuntimeTokenInternal(String name, String vmId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* refreshRuntimeTokenInternalCallable()
*
*
*
*
* DiagnoseRuntime
* Creates a Diagnostic File and runs Diagnostic Tool given a Runtime.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* diagnoseRuntimeAsync(DiagnoseRuntimeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* diagnoseRuntimeAsync(RuntimeName name, DiagnosticConfig diagnosticConfig)
*
diagnoseRuntimeAsync(String name, DiagnosticConfig diagnosticConfig)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* diagnoseRuntimeOperationCallable()
*
diagnoseRuntimeCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listLocationsPagedCallable()
*
listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getLocationCallable()
*
*
*
*
* SetIamPolicy
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* setIamPolicy(SetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* setIamPolicyCallable()
*
*
*
*
* GetIamPolicy
* Gets the access control policy for a resource. Returns an empty policyif the resource exists and does not have a policy set.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getIamPolicy(GetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getIamPolicyCallable()
*
*
*
*
* TestIamPermissions
* Returns permissions that a caller has on the specified resource. If theresource does not exist, this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
Note: This operation is designed to be used for buildingpermission-aware UIs and command-line tools, not for authorizationchecking. This operation may "fail open" without warning.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* testIamPermissions(TestIamPermissionsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* testIamPermissionsCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of ManagedNotebookServiceSettings
* to create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ManagedNotebookServiceSettings managedNotebookServiceSettings =
* ManagedNotebookServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create(managedNotebookServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ManagedNotebookServiceSettings managedNotebookServiceSettings =
* ManagedNotebookServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create(managedNotebookServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class ManagedNotebookServiceClient implements BackgroundResource {
private final ManagedNotebookServiceSettings settings;
private final ManagedNotebookServiceStub stub;
private final OperationsClient operationsClient;
/** Constructs an instance of ManagedNotebookServiceClient with default settings. */
public static final ManagedNotebookServiceClient create() throws IOException {
return create(ManagedNotebookServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of ManagedNotebookServiceClient, using the given settings. The channels
* are created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final ManagedNotebookServiceClient create(ManagedNotebookServiceSettings settings)
throws IOException {
return new ManagedNotebookServiceClient(settings);
}
/**
* Constructs an instance of ManagedNotebookServiceClient, using the given stub for making calls.
* This is for advanced usage - prefer using create(ManagedNotebookServiceSettings).
*/
public static final ManagedNotebookServiceClient create(ManagedNotebookServiceStub stub) {
return new ManagedNotebookServiceClient(stub);
}
/**
* Constructs an instance of ManagedNotebookServiceClient, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected ManagedNotebookServiceClient(ManagedNotebookServiceSettings settings)
throws IOException {
this.settings = settings;
this.stub = ((ManagedNotebookServiceStubSettings) settings.getStubSettings()).createStub();
this.operationsClient = OperationsClient.create(this.stub.getOperationsStub());
}
protected ManagedNotebookServiceClient(ManagedNotebookServiceStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient = OperationsClient.create(this.stub.getOperationsStub());
}
public final ManagedNotebookServiceSettings getSettings() {
return settings;
}
public ManagedNotebookServiceStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final OperationsClient getOperationsClient() {
return operationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Runtimes in a given project and location.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Runtime element : managedNotebookServiceClient.listRuntimes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Format: `parent=projects/{project_id}/locations/{location}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRuntimesPagedResponse listRuntimes(LocationName parent) {
ListRuntimesRequest request =
ListRuntimesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listRuntimes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Runtimes in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Runtime element : managedNotebookServiceClient.listRuntimes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Format: `parent=projects/{project_id}/locations/{location}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRuntimesPagedResponse listRuntimes(String parent) {
ListRuntimesRequest request = ListRuntimesRequest.newBuilder().setParent(parent).build();
return listRuntimes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Runtimes in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ListRuntimesRequest request =
* ListRuntimesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Runtime element : managedNotebookServiceClient.listRuntimes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRuntimesPagedResponse listRuntimes(ListRuntimesRequest request) {
return listRuntimesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Runtimes in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ListRuntimesRequest request =
* ListRuntimesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.listRuntimesPagedCallable().futureCall(request);
* // Do something.
* for (Runtime element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listRuntimesPagedCallable() {
return stub.listRuntimesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Runtimes in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ListRuntimesRequest request =
* ListRuntimesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListRuntimesResponse response =
* managedNotebookServiceClient.listRuntimesCallable().call(request);
* for (Runtime element : response.getRuntimesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listRuntimesCallable() {
return stub.listRuntimesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Runtime. The location must be a regional endpoint rather than zonal.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* RuntimeName name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]");
* Runtime response = managedNotebookServiceClient.getRuntime(name);
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Runtime getRuntime(RuntimeName name) {
GetRuntimeRequest request =
GetRuntimeRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getRuntime(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Runtime. The location must be a regional endpoint rather than zonal.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString();
* Runtime response = managedNotebookServiceClient.getRuntime(name);
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Runtime getRuntime(String name) {
GetRuntimeRequest request = GetRuntimeRequest.newBuilder().setName(name).build();
return getRuntime(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Runtime. The location must be a regional endpoint rather than zonal.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* GetRuntimeRequest request =
* GetRuntimeRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .build();
* Runtime response = managedNotebookServiceClient.getRuntime(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Runtime getRuntime(GetRuntimeRequest request) {
return getRuntimeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Runtime. The location must be a regional endpoint rather than zonal.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* GetRuntimeRequest request =
* GetRuntimeRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .build();
* ApiFuture future =
* managedNotebookServiceClient.getRuntimeCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final UnaryCallable getRuntimeCallable() {
return stub.getRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Runtime in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* RuntimeName parent = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]");
* String runtimeId = "runtimeId121455379";
* Runtime runtime = Runtime.newBuilder().build();
* Runtime response =
* managedNotebookServiceClient.createRuntimeAsync(parent, runtimeId, runtime).get();
* }
* }
*
* @param parent Required. Format: `parent=projects/{project_id}/locations/{location}`
* @param runtimeId Required. User-defined unique ID of this Runtime.
* @param runtime Required. The Runtime to be created.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createRuntimeAsync(
RuntimeName parent, String runtimeId, Runtime runtime) {
CreateRuntimeRequest request =
CreateRuntimeRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setRuntimeId(runtimeId)
.setRuntime(runtime)
.build();
return createRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Runtime in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String parent = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString();
* String runtimeId = "runtimeId121455379";
* Runtime runtime = Runtime.newBuilder().build();
* Runtime response =
* managedNotebookServiceClient.createRuntimeAsync(parent, runtimeId, runtime).get();
* }
* }
*
* @param parent Required. Format: `parent=projects/{project_id}/locations/{location}`
* @param runtimeId Required. User-defined unique ID of this Runtime.
* @param runtime Required. The Runtime to be created.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createRuntimeAsync(
String parent, String runtimeId, Runtime runtime) {
CreateRuntimeRequest request =
CreateRuntimeRequest.newBuilder()
.setParent(parent)
.setRuntimeId(runtimeId)
.setRuntime(runtime)
.build();
return createRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Runtime in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* CreateRuntimeRequest request =
* CreateRuntimeRequest.newBuilder()
* .setParent(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setRuntimeId("runtimeId121455379")
* .setRuntime(Runtime.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Runtime response = managedNotebookServiceClient.createRuntimeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createRuntimeAsync(
CreateRuntimeRequest request) {
return createRuntimeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Runtime in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* CreateRuntimeRequest request =
* CreateRuntimeRequest.newBuilder()
* .setParent(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setRuntimeId("runtimeId121455379")
* .setRuntime(Runtime.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* managedNotebookServiceClient.createRuntimeOperationCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final OperationCallable
createRuntimeOperationCallable() {
return stub.createRuntimeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Runtime in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* CreateRuntimeRequest request =
* CreateRuntimeRequest.newBuilder()
* .setParent(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setRuntimeId("runtimeId121455379")
* .setRuntime(Runtime.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.createRuntimeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createRuntimeCallable() {
return stub.createRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update Notebook Runtime configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* Runtime runtime = Runtime.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Runtime response = managedNotebookServiceClient.updateRuntimeAsync(runtime, updateMask).get();
* }
* }
*
* @param runtime Required. The Runtime to be updated.
* @param updateMask Required. Specifies the path, relative to `Runtime`, of the field to update.
* For example, to change the software configuration kernels, the `update_mask` parameter
* would be specified as `software_config.kernels`, and the `PATCH` request body would specify
* the new value, as follows:
* { "software_config":{ "kernels": [{ 'repository':
* 'gcr.io/deeplearning-platform-release/pytorch-gpu', 'tag': 'latest' }], } }
*
Currently, only the following fields can be updated: - `software_config.kernels` -
* `software_config.post_startup_script` - `software_config.custom_gpu_driver_path` -
* `software_config.idle_shutdown` - `software_config.idle_shutdown_timeout` -
* `software_config.disable_terminal`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateRuntimeAsync(
Runtime runtime, FieldMask updateMask) {
UpdateRuntimeRequest request =
UpdateRuntimeRequest.newBuilder().setRuntime(runtime).setUpdateMask(updateMask).build();
return updateRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update Notebook Runtime configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* UpdateRuntimeRequest request =
* UpdateRuntimeRequest.newBuilder()
* .setRuntime(Runtime.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Runtime response = managedNotebookServiceClient.updateRuntimeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateRuntimeAsync(
UpdateRuntimeRequest request) {
return updateRuntimeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update Notebook Runtime configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* UpdateRuntimeRequest request =
* UpdateRuntimeRequest.newBuilder()
* .setRuntime(Runtime.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* managedNotebookServiceClient.updateRuntimeOperationCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final OperationCallable
updateRuntimeOperationCallable() {
return stub.updateRuntimeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update Notebook Runtime configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* UpdateRuntimeRequest request =
* UpdateRuntimeRequest.newBuilder()
* .setRuntime(Runtime.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.updateRuntimeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateRuntimeCallable() {
return stub.updateRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* RuntimeName name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]");
* managedNotebookServiceClient.deleteRuntimeAsync(name).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteRuntimeAsync(RuntimeName name) {
DeleteRuntimeRequest request =
DeleteRuntimeRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString();
* managedNotebookServiceClient.deleteRuntimeAsync(name).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteRuntimeAsync(String name) {
DeleteRuntimeRequest request = DeleteRuntimeRequest.newBuilder().setName(name).build();
return deleteRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* DeleteRuntimeRequest request =
* DeleteRuntimeRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setRequestId("requestId693933066")
* .build();
* managedNotebookServiceClient.deleteRuntimeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteRuntimeAsync(
DeleteRuntimeRequest request) {
return deleteRuntimeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* DeleteRuntimeRequest request =
* DeleteRuntimeRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* managedNotebookServiceClient.deleteRuntimeOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteRuntimeOperationCallable() {
return stub.deleteRuntimeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* DeleteRuntimeRequest request =
* DeleteRuntimeRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.deleteRuntimeCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteRuntimeCallable() {
return stub.deleteRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts a Managed Notebook Runtime. Perform "Start" on GPU instances; "Resume" on CPU instances
* See: https://cloud.google.com/compute/docs/instances/stop-start-instance
* https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = "name3373707";
* Runtime response = managedNotebookServiceClient.startRuntimeAsync(name).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture startRuntimeAsync(String name) {
StartRuntimeRequest request = StartRuntimeRequest.newBuilder().setName(name).build();
return startRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts a Managed Notebook Runtime. Perform "Start" on GPU instances; "Resume" on CPU instances
* See: https://cloud.google.com/compute/docs/instances/stop-start-instance
* https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* StartRuntimeRequest request =
* StartRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* Runtime response = managedNotebookServiceClient.startRuntimeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture startRuntimeAsync(
StartRuntimeRequest request) {
return startRuntimeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts a Managed Notebook Runtime. Perform "Start" on GPU instances; "Resume" on CPU instances
* See: https://cloud.google.com/compute/docs/instances/stop-start-instance
* https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* StartRuntimeRequest request =
* StartRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* managedNotebookServiceClient.startRuntimeOperationCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final OperationCallable
startRuntimeOperationCallable() {
return stub.startRuntimeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts a Managed Notebook Runtime. Perform "Start" on GPU instances; "Resume" on CPU instances
* See: https://cloud.google.com/compute/docs/instances/stop-start-instance
* https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* StartRuntimeRequest request =
* StartRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.startRuntimeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable startRuntimeCallable() {
return stub.startRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops a Managed Notebook Runtime. Perform "Stop" on GPU instances; "Suspend" on CPU instances
* See: https://cloud.google.com/compute/docs/instances/stop-start-instance
* https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = "name3373707";
* Runtime response = managedNotebookServiceClient.stopRuntimeAsync(name).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture stopRuntimeAsync(String name) {
StopRuntimeRequest request = StopRuntimeRequest.newBuilder().setName(name).build();
return stopRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops a Managed Notebook Runtime. Perform "Stop" on GPU instances; "Suspend" on CPU instances
* See: https://cloud.google.com/compute/docs/instances/stop-start-instance
* https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* StopRuntimeRequest request =
* StopRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* Runtime response = managedNotebookServiceClient.stopRuntimeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture stopRuntimeAsync(
StopRuntimeRequest request) {
return stopRuntimeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops a Managed Notebook Runtime. Perform "Stop" on GPU instances; "Suspend" on CPU instances
* See: https://cloud.google.com/compute/docs/instances/stop-start-instance
* https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* StopRuntimeRequest request =
* StopRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* managedNotebookServiceClient.stopRuntimeOperationCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final OperationCallable
stopRuntimeOperationCallable() {
return stub.stopRuntimeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops a Managed Notebook Runtime. Perform "Stop" on GPU instances; "Suspend" on CPU instances
* See: https://cloud.google.com/compute/docs/instances/stop-start-instance
* https://cloud.google.com/compute/docs/instances/suspend-resume-instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* StopRuntimeRequest request =
* StopRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.stopRuntimeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable stopRuntimeCallable() {
return stub.stopRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Switch a Managed Notebook Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = "name3373707";
* Runtime response = managedNotebookServiceClient.switchRuntimeAsync(name).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture switchRuntimeAsync(String name) {
SwitchRuntimeRequest request = SwitchRuntimeRequest.newBuilder().setName(name).build();
return switchRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Switch a Managed Notebook Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* SwitchRuntimeRequest request =
* SwitchRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setMachineType("machineType-218117087")
* .setAcceleratorConfig(RuntimeAcceleratorConfig.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Runtime response = managedNotebookServiceClient.switchRuntimeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture switchRuntimeAsync(
SwitchRuntimeRequest request) {
return switchRuntimeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Switch a Managed Notebook Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* SwitchRuntimeRequest request =
* SwitchRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setMachineType("machineType-218117087")
* .setAcceleratorConfig(RuntimeAcceleratorConfig.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* managedNotebookServiceClient.switchRuntimeOperationCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final OperationCallable
switchRuntimeOperationCallable() {
return stub.switchRuntimeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Switch a Managed Notebook Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* SwitchRuntimeRequest request =
* SwitchRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setMachineType("machineType-218117087")
* .setAcceleratorConfig(RuntimeAcceleratorConfig.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.switchRuntimeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable switchRuntimeCallable() {
return stub.switchRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resets a Managed Notebook Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = "name3373707";
* Runtime response = managedNotebookServiceClient.resetRuntimeAsync(name).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture resetRuntimeAsync(String name) {
ResetRuntimeRequest request = ResetRuntimeRequest.newBuilder().setName(name).build();
return resetRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resets a Managed Notebook Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ResetRuntimeRequest request =
* ResetRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* Runtime response = managedNotebookServiceClient.resetRuntimeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture resetRuntimeAsync(
ResetRuntimeRequest request) {
return resetRuntimeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resets a Managed Notebook Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ResetRuntimeRequest request =
* ResetRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* managedNotebookServiceClient.resetRuntimeOperationCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final OperationCallable
resetRuntimeOperationCallable() {
return stub.resetRuntimeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resets a Managed Notebook Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ResetRuntimeRequest request =
* ResetRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.resetRuntimeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable resetRuntimeCallable() {
return stub.resetRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Upgrades a Managed Notebook Runtime to the latest version.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = "name3373707";
* Runtime response = managedNotebookServiceClient.upgradeRuntimeAsync(name).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture upgradeRuntimeAsync(String name) {
UpgradeRuntimeRequest request = UpgradeRuntimeRequest.newBuilder().setName(name).build();
return upgradeRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Upgrades a Managed Notebook Runtime to the latest version.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* UpgradeRuntimeRequest request =
* UpgradeRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* Runtime response = managedNotebookServiceClient.upgradeRuntimeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture upgradeRuntimeAsync(
UpgradeRuntimeRequest request) {
return upgradeRuntimeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Upgrades a Managed Notebook Runtime to the latest version.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* UpgradeRuntimeRequest request =
* UpgradeRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* managedNotebookServiceClient.upgradeRuntimeOperationCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final OperationCallable
upgradeRuntimeOperationCallable() {
return stub.upgradeRuntimeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Upgrades a Managed Notebook Runtime to the latest version.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* UpgradeRuntimeRequest request =
* UpgradeRuntimeRequest.newBuilder()
* .setName("name3373707")
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.upgradeRuntimeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable upgradeRuntimeCallable() {
return stub.upgradeRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Report and process a runtime event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* RuntimeName name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]");
* Runtime response = managedNotebookServiceClient.reportRuntimeEventAsync(name).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture reportRuntimeEventAsync(
RuntimeName name) {
ReportRuntimeEventRequest request =
ReportRuntimeEventRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return reportRuntimeEventAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Report and process a runtime event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString();
* Runtime response = managedNotebookServiceClient.reportRuntimeEventAsync(name).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture reportRuntimeEventAsync(String name) {
ReportRuntimeEventRequest request =
ReportRuntimeEventRequest.newBuilder().setName(name).build();
return reportRuntimeEventAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Report and process a runtime event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ReportRuntimeEventRequest request =
* ReportRuntimeEventRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setVmId("vmId3622450")
* .setEvent(Event.newBuilder().build())
* .build();
* Runtime response = managedNotebookServiceClient.reportRuntimeEventAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture reportRuntimeEventAsync(
ReportRuntimeEventRequest request) {
return reportRuntimeEventOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Report and process a runtime event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ReportRuntimeEventRequest request =
* ReportRuntimeEventRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setVmId("vmId3622450")
* .setEvent(Event.newBuilder().build())
* .build();
* OperationFuture future =
* managedNotebookServiceClient.reportRuntimeEventOperationCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final OperationCallable
reportRuntimeEventOperationCallable() {
return stub.reportRuntimeEventOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Report and process a runtime event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ReportRuntimeEventRequest request =
* ReportRuntimeEventRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setVmId("vmId3622450")
* .setEvent(Event.newBuilder().build())
* .build();
* ApiFuture future =
* managedNotebookServiceClient.reportRuntimeEventCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable reportRuntimeEventCallable() {
return stub.reportRuntimeEventCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an access token for the consumer service account that the customer attached to the
* runtime. Only accessible from the tenant instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* RuntimeName name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]");
* String vmId = "vmId3622450";
* RefreshRuntimeTokenInternalResponse response =
* managedNotebookServiceClient.refreshRuntimeTokenInternal(name, vmId);
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @param vmId Required. The VM hardware token for authenticating the VM.
* https://cloud.google.com/compute/docs/instances/verifying-instance-identity
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RefreshRuntimeTokenInternalResponse refreshRuntimeTokenInternal(
RuntimeName name, String vmId) {
RefreshRuntimeTokenInternalRequest request =
RefreshRuntimeTokenInternalRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setVmId(vmId)
.build();
return refreshRuntimeTokenInternal(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an access token for the consumer service account that the customer attached to the
* runtime. Only accessible from the tenant instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString();
* String vmId = "vmId3622450";
* RefreshRuntimeTokenInternalResponse response =
* managedNotebookServiceClient.refreshRuntimeTokenInternal(name, vmId);
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtime_id}`
* @param vmId Required. The VM hardware token for authenticating the VM.
* https://cloud.google.com/compute/docs/instances/verifying-instance-identity
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RefreshRuntimeTokenInternalResponse refreshRuntimeTokenInternal(
String name, String vmId) {
RefreshRuntimeTokenInternalRequest request =
RefreshRuntimeTokenInternalRequest.newBuilder().setName(name).setVmId(vmId).build();
return refreshRuntimeTokenInternal(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an access token for the consumer service account that the customer attached to the
* runtime. Only accessible from the tenant instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* RefreshRuntimeTokenInternalRequest request =
* RefreshRuntimeTokenInternalRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setVmId("vmId3622450")
* .build();
* RefreshRuntimeTokenInternalResponse response =
* managedNotebookServiceClient.refreshRuntimeTokenInternal(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RefreshRuntimeTokenInternalResponse refreshRuntimeTokenInternal(
RefreshRuntimeTokenInternalRequest request) {
return refreshRuntimeTokenInternalCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an access token for the consumer service account that the customer attached to the
* runtime. Only accessible from the tenant instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* RefreshRuntimeTokenInternalRequest request =
* RefreshRuntimeTokenInternalRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setVmId("vmId3622450")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.refreshRuntimeTokenInternalCallable().futureCall(request);
* // Do something.
* RefreshRuntimeTokenInternalResponse response = future.get();
* }
* }
*/
public final UnaryCallable<
RefreshRuntimeTokenInternalRequest, RefreshRuntimeTokenInternalResponse>
refreshRuntimeTokenInternalCallable() {
return stub.refreshRuntimeTokenInternalCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Diagnostic File and runs Diagnostic Tool given a Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* RuntimeName name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]");
* DiagnosticConfig diagnosticConfig = DiagnosticConfig.newBuilder().build();
* Runtime response =
* managedNotebookServiceClient.diagnoseRuntimeAsync(name, diagnosticConfig).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtimes_id}`
* @param diagnosticConfig Required. Defines flags that are used to run the diagnostic tool
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture diagnoseRuntimeAsync(
RuntimeName name, DiagnosticConfig diagnosticConfig) {
DiagnoseRuntimeRequest request =
DiagnoseRuntimeRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setDiagnosticConfig(diagnosticConfig)
.build();
return diagnoseRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Diagnostic File and runs Diagnostic Tool given a Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* String name = RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString();
* DiagnosticConfig diagnosticConfig = DiagnosticConfig.newBuilder().build();
* Runtime response =
* managedNotebookServiceClient.diagnoseRuntimeAsync(name, diagnosticConfig).get();
* }
* }
*
* @param name Required. Format:
* `projects/{project_id}/locations/{location}/runtimes/{runtimes_id}`
* @param diagnosticConfig Required. Defines flags that are used to run the diagnostic tool
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture diagnoseRuntimeAsync(
String name, DiagnosticConfig diagnosticConfig) {
DiagnoseRuntimeRequest request =
DiagnoseRuntimeRequest.newBuilder()
.setName(name)
.setDiagnosticConfig(diagnosticConfig)
.build();
return diagnoseRuntimeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Diagnostic File and runs Diagnostic Tool given a Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* DiagnoseRuntimeRequest request =
* DiagnoseRuntimeRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setDiagnosticConfig(DiagnosticConfig.newBuilder().build())
* .build();
* Runtime response = managedNotebookServiceClient.diagnoseRuntimeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture diagnoseRuntimeAsync(
DiagnoseRuntimeRequest request) {
return diagnoseRuntimeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Diagnostic File and runs Diagnostic Tool given a Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* DiagnoseRuntimeRequest request =
* DiagnoseRuntimeRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setDiagnosticConfig(DiagnosticConfig.newBuilder().build())
* .build();
* OperationFuture future =
* managedNotebookServiceClient.diagnoseRuntimeOperationCallable().futureCall(request);
* // Do something.
* Runtime response = future.get();
* }
* }
*/
public final OperationCallable
diagnoseRuntimeOperationCallable() {
return stub.diagnoseRuntimeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a Diagnostic File and runs Diagnostic Tool given a Runtime.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* DiagnoseRuntimeRequest request =
* DiagnoseRuntimeRequest.newBuilder()
* .setName(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setDiagnosticConfig(DiagnosticConfig.newBuilder().build())
* .build();
* ApiFuture future =
* managedNotebookServiceClient.diagnoseRuntimeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable diagnoseRuntimeCallable() {
return stub.diagnoseRuntimeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : managedNotebookServiceClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* managedNotebookServiceClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response =
* managedNotebookServiceClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = managedNotebookServiceClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future =
* managedNotebookServiceClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Policy response = managedNotebookServiceClient.setIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(SetIamPolicyRequest request) {
return setIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* managedNotebookServiceClient.setIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable setIamPolicyCallable() {
return stub.setIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* Policy response = managedNotebookServiceClient.getIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(GetIamPolicyRequest request) {
return getIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* ApiFuture future =
* managedNotebookServiceClient.getIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable getIamPolicyCallable() {
return stub.getIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* TestIamPermissionsResponse response =
* managedNotebookServiceClient.testIamPermissions(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(TestIamPermissionsRequest request) {
return testIamPermissionsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ManagedNotebookServiceClient managedNotebookServiceClient =
* ManagedNotebookServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(RuntimeName.of("[PROJECT]", "[LOCATION]", "[RUNTIME]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* ApiFuture future =
* managedNotebookServiceClient.testIamPermissionsCallable().futureCall(request);
* // Do something.
* TestIamPermissionsResponse response = future.get();
* }
* }
*/
public final UnaryCallable
testIamPermissionsCallable() {
return stub.testIamPermissionsCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListRuntimesPagedResponse
extends AbstractPagedListResponse<
ListRuntimesRequest,
ListRuntimesResponse,
Runtime,
ListRuntimesPage,
ListRuntimesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListRuntimesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListRuntimesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListRuntimesPagedResponse(ListRuntimesPage page) {
super(page, ListRuntimesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListRuntimesPage
extends AbstractPage {
private ListRuntimesPage(
PageContext context,
ListRuntimesResponse response) {
super(context, response);
}
private static ListRuntimesPage createEmptyPage() {
return new ListRuntimesPage(null, null);
}
@Override
protected ListRuntimesPage createPage(
PageContext context,
ListRuntimesResponse response) {
return new ListRuntimesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListRuntimesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListRuntimesRequest,
ListRuntimesResponse,
Runtime,
ListRuntimesPage,
ListRuntimesFixedSizeCollection> {
private ListRuntimesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListRuntimesFixedSizeCollection createEmptyCollection() {
return new ListRuntimesFixedSizeCollection(null, 0);
}
@Override
protected ListRuntimesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListRuntimesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}