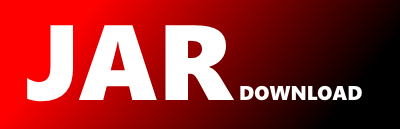
com.google.cloud.notebooks.v1.stub.GrpcManagedNotebookServiceStub Maven / Gradle / Ivy
Show all versions of google-cloud-notebooks Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.notebooks.v1.stub;
import static com.google.cloud.notebooks.v1.ManagedNotebookServiceClient.ListLocationsPagedResponse;
import static com.google.cloud.notebooks.v1.ManagedNotebookServiceClient.ListRuntimesPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.notebooks.v1.CreateRuntimeRequest;
import com.google.cloud.notebooks.v1.DeleteRuntimeRequest;
import com.google.cloud.notebooks.v1.DiagnoseRuntimeRequest;
import com.google.cloud.notebooks.v1.GetRuntimeRequest;
import com.google.cloud.notebooks.v1.ListRuntimesRequest;
import com.google.cloud.notebooks.v1.ListRuntimesResponse;
import com.google.cloud.notebooks.v1.OperationMetadata;
import com.google.cloud.notebooks.v1.RefreshRuntimeTokenInternalRequest;
import com.google.cloud.notebooks.v1.RefreshRuntimeTokenInternalResponse;
import com.google.cloud.notebooks.v1.ReportRuntimeEventRequest;
import com.google.cloud.notebooks.v1.ResetRuntimeRequest;
import com.google.cloud.notebooks.v1.Runtime;
import com.google.cloud.notebooks.v1.StartRuntimeRequest;
import com.google.cloud.notebooks.v1.StopRuntimeRequest;
import com.google.cloud.notebooks.v1.SwitchRuntimeRequest;
import com.google.cloud.notebooks.v1.UpdateRuntimeRequest;
import com.google.cloud.notebooks.v1.UpgradeRuntimeRequest;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import com.google.protobuf.Empty;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the ManagedNotebookService service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public class GrpcManagedNotebookServiceStub extends ManagedNotebookServiceStub {
private static final MethodDescriptor
listRuntimesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/ListRuntimes")
.setRequestMarshaller(ProtoUtils.marshaller(ListRuntimesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListRuntimesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/GetRuntime")
.setRequestMarshaller(ProtoUtils.marshaller(GetRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Runtime.getDefaultInstance()))
.build();
private static final MethodDescriptor
createRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/CreateRuntime")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/UpdateRuntime")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/DeleteRuntime")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
startRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/StartRuntime")
.setRequestMarshaller(ProtoUtils.marshaller(StartRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor stopRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/StopRuntime")
.setRequestMarshaller(ProtoUtils.marshaller(StopRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
switchRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/SwitchRuntime")
.setRequestMarshaller(
ProtoUtils.marshaller(SwitchRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
resetRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/ResetRuntime")
.setRequestMarshaller(ProtoUtils.marshaller(ResetRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
upgradeRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/UpgradeRuntime")
.setRequestMarshaller(
ProtoUtils.marshaller(UpgradeRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
reportRuntimeEventMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.notebooks.v1.ManagedNotebookService/ReportRuntimeEvent")
.setRequestMarshaller(
ProtoUtils.marshaller(ReportRuntimeEventRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor<
RefreshRuntimeTokenInternalRequest, RefreshRuntimeTokenInternalResponse>
refreshRuntimeTokenInternalMethodDescriptor =
MethodDescriptor
.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName(
"google.cloud.notebooks.v1.ManagedNotebookService/RefreshRuntimeTokenInternal")
.setRequestMarshaller(
ProtoUtils.marshaller(RefreshRuntimeTokenInternalRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(RefreshRuntimeTokenInternalResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
diagnoseRuntimeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.notebooks.v1.ManagedNotebookService/DiagnoseRuntime")
.setRequestMarshaller(
ProtoUtils.marshaller(DiagnoseRuntimeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listLocationsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/ListLocations")
.setRequestMarshaller(
ProtoUtils.marshaller(ListLocationsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListLocationsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getLocationMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/GetLocation")
.setRequestMarshaller(ProtoUtils.marshaller(GetLocationRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Location.getDefaultInstance()))
.build();
private static final MethodDescriptor setIamPolicyMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.iam.v1.IAMPolicy/SetIamPolicy")
.setRequestMarshaller(ProtoUtils.marshaller(SetIamPolicyRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Policy.getDefaultInstance()))
.build();
private static final MethodDescriptor getIamPolicyMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.iam.v1.IAMPolicy/GetIamPolicy")
.setRequestMarshaller(ProtoUtils.marshaller(GetIamPolicyRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Policy.getDefaultInstance()))
.build();
private static final MethodDescriptor
testIamPermissionsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.iam.v1.IAMPolicy/TestIamPermissions")
.setRequestMarshaller(
ProtoUtils.marshaller(TestIamPermissionsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(TestIamPermissionsResponse.getDefaultInstance()))
.build();
private final UnaryCallable listRuntimesCallable;
private final UnaryCallable
listRuntimesPagedCallable;
private final UnaryCallable getRuntimeCallable;
private final UnaryCallable createRuntimeCallable;
private final OperationCallable
createRuntimeOperationCallable;
private final UnaryCallable updateRuntimeCallable;
private final OperationCallable
updateRuntimeOperationCallable;
private final UnaryCallable deleteRuntimeCallable;
private final OperationCallable
deleteRuntimeOperationCallable;
private final UnaryCallable startRuntimeCallable;
private final OperationCallable
startRuntimeOperationCallable;
private final UnaryCallable stopRuntimeCallable;
private final OperationCallable
stopRuntimeOperationCallable;
private final UnaryCallable switchRuntimeCallable;
private final OperationCallable
switchRuntimeOperationCallable;
private final UnaryCallable resetRuntimeCallable;
private final OperationCallable
resetRuntimeOperationCallable;
private final UnaryCallable upgradeRuntimeCallable;
private final OperationCallable
upgradeRuntimeOperationCallable;
private final UnaryCallable reportRuntimeEventCallable;
private final OperationCallable
reportRuntimeEventOperationCallable;
private final UnaryCallable<
RefreshRuntimeTokenInternalRequest, RefreshRuntimeTokenInternalResponse>
refreshRuntimeTokenInternalCallable;
private final UnaryCallable diagnoseRuntimeCallable;
private final OperationCallable
diagnoseRuntimeOperationCallable;
private final UnaryCallable listLocationsCallable;
private final UnaryCallable
listLocationsPagedCallable;
private final UnaryCallable getLocationCallable;
private final UnaryCallable setIamPolicyCallable;
private final UnaryCallable getIamPolicyCallable;
private final UnaryCallable
testIamPermissionsCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcManagedNotebookServiceStub create(
ManagedNotebookServiceStubSettings settings) throws IOException {
return new GrpcManagedNotebookServiceStub(settings, ClientContext.create(settings));
}
public static final GrpcManagedNotebookServiceStub create(ClientContext clientContext)
throws IOException {
return new GrpcManagedNotebookServiceStub(
ManagedNotebookServiceStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcManagedNotebookServiceStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcManagedNotebookServiceStub(
ManagedNotebookServiceStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcManagedNotebookServiceStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcManagedNotebookServiceStub(
ManagedNotebookServiceStubSettings settings, ClientContext clientContext) throws IOException {
this(settings, clientContext, new GrpcManagedNotebookServiceCallableFactory());
}
/**
* Constructs an instance of GrpcManagedNotebookServiceStub, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected GrpcManagedNotebookServiceStub(
ManagedNotebookServiceStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings listRuntimesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listRuntimesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings updateRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("runtime.name", String.valueOf(request.getRuntime().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings startRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(startRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings stopRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(stopRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings switchRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(switchRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings resetRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(resetRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings upgradeRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(upgradeRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings reportRuntimeEventTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(reportRuntimeEventMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings
refreshRuntimeTokenInternalTransportSettings =
GrpcCallSettings
.
newBuilder()
.setMethodDescriptor(refreshRuntimeTokenInternalMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings diagnoseRuntimeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(diagnoseRuntimeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listLocationsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listLocationsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getLocationTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getLocationMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings setIamPolicyTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(setIamPolicyMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("resource", String.valueOf(request.getResource()));
return builder.build();
})
.build();
GrpcCallSettings getIamPolicyTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getIamPolicyMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("resource", String.valueOf(request.getResource()));
return builder.build();
})
.build();
GrpcCallSettings
testIamPermissionsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(testIamPermissionsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("resource", String.valueOf(request.getResource()));
return builder.build();
})
.build();
this.listRuntimesCallable =
callableFactory.createUnaryCallable(
listRuntimesTransportSettings, settings.listRuntimesSettings(), clientContext);
this.listRuntimesPagedCallable =
callableFactory.createPagedCallable(
listRuntimesTransportSettings, settings.listRuntimesSettings(), clientContext);
this.getRuntimeCallable =
callableFactory.createUnaryCallable(
getRuntimeTransportSettings, settings.getRuntimeSettings(), clientContext);
this.createRuntimeCallable =
callableFactory.createUnaryCallable(
createRuntimeTransportSettings, settings.createRuntimeSettings(), clientContext);
this.createRuntimeOperationCallable =
callableFactory.createOperationCallable(
createRuntimeTransportSettings,
settings.createRuntimeOperationSettings(),
clientContext,
operationsStub);
this.updateRuntimeCallable =
callableFactory.createUnaryCallable(
updateRuntimeTransportSettings, settings.updateRuntimeSettings(), clientContext);
this.updateRuntimeOperationCallable =
callableFactory.createOperationCallable(
updateRuntimeTransportSettings,
settings.updateRuntimeOperationSettings(),
clientContext,
operationsStub);
this.deleteRuntimeCallable =
callableFactory.createUnaryCallable(
deleteRuntimeTransportSettings, settings.deleteRuntimeSettings(), clientContext);
this.deleteRuntimeOperationCallable =
callableFactory.createOperationCallable(
deleteRuntimeTransportSettings,
settings.deleteRuntimeOperationSettings(),
clientContext,
operationsStub);
this.startRuntimeCallable =
callableFactory.createUnaryCallable(
startRuntimeTransportSettings, settings.startRuntimeSettings(), clientContext);
this.startRuntimeOperationCallable =
callableFactory.createOperationCallable(
startRuntimeTransportSettings,
settings.startRuntimeOperationSettings(),
clientContext,
operationsStub);
this.stopRuntimeCallable =
callableFactory.createUnaryCallable(
stopRuntimeTransportSettings, settings.stopRuntimeSettings(), clientContext);
this.stopRuntimeOperationCallable =
callableFactory.createOperationCallable(
stopRuntimeTransportSettings,
settings.stopRuntimeOperationSettings(),
clientContext,
operationsStub);
this.switchRuntimeCallable =
callableFactory.createUnaryCallable(
switchRuntimeTransportSettings, settings.switchRuntimeSettings(), clientContext);
this.switchRuntimeOperationCallable =
callableFactory.createOperationCallable(
switchRuntimeTransportSettings,
settings.switchRuntimeOperationSettings(),
clientContext,
operationsStub);
this.resetRuntimeCallable =
callableFactory.createUnaryCallable(
resetRuntimeTransportSettings, settings.resetRuntimeSettings(), clientContext);
this.resetRuntimeOperationCallable =
callableFactory.createOperationCallable(
resetRuntimeTransportSettings,
settings.resetRuntimeOperationSettings(),
clientContext,
operationsStub);
this.upgradeRuntimeCallable =
callableFactory.createUnaryCallable(
upgradeRuntimeTransportSettings, settings.upgradeRuntimeSettings(), clientContext);
this.upgradeRuntimeOperationCallable =
callableFactory.createOperationCallable(
upgradeRuntimeTransportSettings,
settings.upgradeRuntimeOperationSettings(),
clientContext,
operationsStub);
this.reportRuntimeEventCallable =
callableFactory.createUnaryCallable(
reportRuntimeEventTransportSettings,
settings.reportRuntimeEventSettings(),
clientContext);
this.reportRuntimeEventOperationCallable =
callableFactory.createOperationCallable(
reportRuntimeEventTransportSettings,
settings.reportRuntimeEventOperationSettings(),
clientContext,
operationsStub);
this.refreshRuntimeTokenInternalCallable =
callableFactory.createUnaryCallable(
refreshRuntimeTokenInternalTransportSettings,
settings.refreshRuntimeTokenInternalSettings(),
clientContext);
this.diagnoseRuntimeCallable =
callableFactory.createUnaryCallable(
diagnoseRuntimeTransportSettings, settings.diagnoseRuntimeSettings(), clientContext);
this.diagnoseRuntimeOperationCallable =
callableFactory.createOperationCallable(
diagnoseRuntimeTransportSettings,
settings.diagnoseRuntimeOperationSettings(),
clientContext,
operationsStub);
this.listLocationsCallable =
callableFactory.createUnaryCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.listLocationsPagedCallable =
callableFactory.createPagedCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.getLocationCallable =
callableFactory.createUnaryCallable(
getLocationTransportSettings, settings.getLocationSettings(), clientContext);
this.setIamPolicyCallable =
callableFactory.createUnaryCallable(
setIamPolicyTransportSettings, settings.setIamPolicySettings(), clientContext);
this.getIamPolicyCallable =
callableFactory.createUnaryCallable(
getIamPolicyTransportSettings, settings.getIamPolicySettings(), clientContext);
this.testIamPermissionsCallable =
callableFactory.createUnaryCallable(
testIamPermissionsTransportSettings,
settings.testIamPermissionsSettings(),
clientContext);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable listRuntimesCallable() {
return listRuntimesCallable;
}
@Override
public UnaryCallable listRuntimesPagedCallable() {
return listRuntimesPagedCallable;
}
@Override
public UnaryCallable getRuntimeCallable() {
return getRuntimeCallable;
}
@Override
public UnaryCallable createRuntimeCallable() {
return createRuntimeCallable;
}
@Override
public OperationCallable
createRuntimeOperationCallable() {
return createRuntimeOperationCallable;
}
@Override
public UnaryCallable updateRuntimeCallable() {
return updateRuntimeCallable;
}
@Override
public OperationCallable
updateRuntimeOperationCallable() {
return updateRuntimeOperationCallable;
}
@Override
public UnaryCallable deleteRuntimeCallable() {
return deleteRuntimeCallable;
}
@Override
public OperationCallable
deleteRuntimeOperationCallable() {
return deleteRuntimeOperationCallable;
}
@Override
public UnaryCallable startRuntimeCallable() {
return startRuntimeCallable;
}
@Override
public OperationCallable
startRuntimeOperationCallable() {
return startRuntimeOperationCallable;
}
@Override
public UnaryCallable stopRuntimeCallable() {
return stopRuntimeCallable;
}
@Override
public OperationCallable
stopRuntimeOperationCallable() {
return stopRuntimeOperationCallable;
}
@Override
public UnaryCallable switchRuntimeCallable() {
return switchRuntimeCallable;
}
@Override
public OperationCallable
switchRuntimeOperationCallable() {
return switchRuntimeOperationCallable;
}
@Override
public UnaryCallable resetRuntimeCallable() {
return resetRuntimeCallable;
}
@Override
public OperationCallable
resetRuntimeOperationCallable() {
return resetRuntimeOperationCallable;
}
@Override
public UnaryCallable upgradeRuntimeCallable() {
return upgradeRuntimeCallable;
}
@Override
public OperationCallable
upgradeRuntimeOperationCallable() {
return upgradeRuntimeOperationCallable;
}
@Override
public UnaryCallable reportRuntimeEventCallable() {
return reportRuntimeEventCallable;
}
@Override
public OperationCallable
reportRuntimeEventOperationCallable() {
return reportRuntimeEventOperationCallable;
}
@Override
public UnaryCallable
refreshRuntimeTokenInternalCallable() {
return refreshRuntimeTokenInternalCallable;
}
@Override
public UnaryCallable diagnoseRuntimeCallable() {
return diagnoseRuntimeCallable;
}
@Override
public OperationCallable
diagnoseRuntimeOperationCallable() {
return diagnoseRuntimeOperationCallable;
}
@Override
public UnaryCallable listLocationsCallable() {
return listLocationsCallable;
}
@Override
public UnaryCallable
listLocationsPagedCallable() {
return listLocationsPagedCallable;
}
@Override
public UnaryCallable getLocationCallable() {
return getLocationCallable;
}
@Override
public UnaryCallable setIamPolicyCallable() {
return setIamPolicyCallable;
}
@Override
public UnaryCallable getIamPolicyCallable() {
return getIamPolicyCallable;
}
@Override
public UnaryCallable
testIamPermissionsCallable() {
return testIamPermissionsCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}