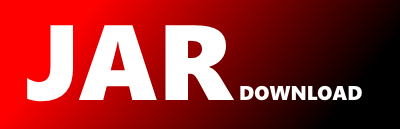
com.google.cloud.oracledatabase.v1.OracleDatabaseClient Maven / Gradle / Ivy
Show all versions of google-cloud-oracledatabase Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.oracledatabase.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.oracledatabase.v1.stub.OracleDatabaseStub;
import com.google.cloud.oracledatabase.v1.stub.OracleDatabaseStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import com.google.protobuf.Timestamp;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Service describing handlers for resources
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CloudExadataInfrastructureName name =
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]");
* CloudExadataInfrastructure response =
* oracleDatabaseClient.getCloudExadataInfrastructure(name);
* }
* }
*
* Note: close() needs to be called on the OracleDatabaseClient object to clean up resources such
* as threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* ListCloudExadataInfrastructures
* Lists Exadata Infrastructures in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listCloudExadataInfrastructures(ListCloudExadataInfrastructuresRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listCloudExadataInfrastructures(LocationName parent)
*
listCloudExadataInfrastructures(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listCloudExadataInfrastructuresPagedCallable()
*
listCloudExadataInfrastructuresCallable()
*
*
*
*
* GetCloudExadataInfrastructure
* Gets details of a single Exadata Infrastructure.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getCloudExadataInfrastructure(GetCloudExadataInfrastructureRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getCloudExadataInfrastructure(CloudExadataInfrastructureName name)
*
getCloudExadataInfrastructure(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getCloudExadataInfrastructureCallable()
*
*
*
*
* CreateCloudExadataInfrastructure
* Creates a new Exadata Infrastructure in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createCloudExadataInfrastructureAsync(CreateCloudExadataInfrastructureRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createCloudExadataInfrastructureAsync(LocationName parent, CloudExadataInfrastructure cloudExadataInfrastructure, String cloudExadataInfrastructureId)
*
createCloudExadataInfrastructureAsync(String parent, CloudExadataInfrastructure cloudExadataInfrastructure, String cloudExadataInfrastructureId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createCloudExadataInfrastructureOperationCallable()
*
createCloudExadataInfrastructureCallable()
*
*
*
*
* DeleteCloudExadataInfrastructure
* Deletes a single Exadata Infrastructure.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteCloudExadataInfrastructureAsync(DeleteCloudExadataInfrastructureRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteCloudExadataInfrastructureAsync(CloudExadataInfrastructureName name)
*
deleteCloudExadataInfrastructureAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteCloudExadataInfrastructureOperationCallable()
*
deleteCloudExadataInfrastructureCallable()
*
*
*
*
* ListCloudVmClusters
* Lists the VM Clusters in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listCloudVmClusters(ListCloudVmClustersRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listCloudVmClusters(LocationName parent)
*
listCloudVmClusters(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listCloudVmClustersPagedCallable()
*
listCloudVmClustersCallable()
*
*
*
*
* GetCloudVmCluster
* Gets details of a single VM Cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getCloudVmCluster(GetCloudVmClusterRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getCloudVmCluster(CloudVmClusterName name)
*
getCloudVmCluster(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getCloudVmClusterCallable()
*
*
*
*
* CreateCloudVmCluster
* Creates a new VM Cluster in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createCloudVmClusterAsync(CreateCloudVmClusterRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createCloudVmClusterAsync(LocationName parent, CloudVmCluster cloudVmCluster, String cloudVmClusterId)
*
createCloudVmClusterAsync(String parent, CloudVmCluster cloudVmCluster, String cloudVmClusterId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createCloudVmClusterOperationCallable()
*
createCloudVmClusterCallable()
*
*
*
*
* DeleteCloudVmCluster
* Deletes a single VM Cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteCloudVmClusterAsync(DeleteCloudVmClusterRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteCloudVmClusterAsync(CloudVmClusterName name)
*
deleteCloudVmClusterAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteCloudVmClusterOperationCallable()
*
deleteCloudVmClusterCallable()
*
*
*
*
* ListEntitlements
* Lists the entitlements in a given project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listEntitlements(ListEntitlementsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listEntitlements(LocationName parent)
*
listEntitlements(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listEntitlementsPagedCallable()
*
listEntitlementsCallable()
*
*
*
*
* ListDbServers
* Lists the database servers of an Exadata Infrastructure instance.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listDbServers(ListDbServersRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listDbServers(CloudExadataInfrastructureName parent)
*
listDbServers(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listDbServersPagedCallable()
*
listDbServersCallable()
*
*
*
*
* ListDbNodes
* Lists the database nodes of a VM Cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listDbNodes(ListDbNodesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listDbNodes(CloudVmClusterName parent)
*
listDbNodes(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listDbNodesPagedCallable()
*
listDbNodesCallable()
*
*
*
*
* ListGiVersions
* Lists all the valid Oracle Grid Infrastructure (GI) versions for the given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listGiVersions(ListGiVersionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listGiVersions(LocationName parent)
*
listGiVersions(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listGiVersionsPagedCallable()
*
listGiVersionsCallable()
*
*
*
*
* ListDbSystemShapes
* Lists the database system shapes available for the project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listDbSystemShapes(ListDbSystemShapesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listDbSystemShapes(LocationName parent)
*
listDbSystemShapes(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listDbSystemShapesPagedCallable()
*
listDbSystemShapesCallable()
*
*
*
*
* ListAutonomousDatabases
* Lists the Autonomous Databases in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listAutonomousDatabases(ListAutonomousDatabasesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listAutonomousDatabases(LocationName parent)
*
listAutonomousDatabases(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listAutonomousDatabasesPagedCallable()
*
listAutonomousDatabasesCallable()
*
*
*
*
* GetAutonomousDatabase
* Gets the details of a single Autonomous Database.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getAutonomousDatabase(GetAutonomousDatabaseRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getAutonomousDatabase(AutonomousDatabaseName name)
*
getAutonomousDatabase(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getAutonomousDatabaseCallable()
*
*
*
*
* CreateAutonomousDatabase
* Creates a new Autonomous Database in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createAutonomousDatabaseAsync(CreateAutonomousDatabaseRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createAutonomousDatabaseAsync(LocationName parent, AutonomousDatabase autonomousDatabase, String autonomousDatabaseId)
*
createAutonomousDatabaseAsync(String parent, AutonomousDatabase autonomousDatabase, String autonomousDatabaseId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createAutonomousDatabaseOperationCallable()
*
createAutonomousDatabaseCallable()
*
*
*
*
* DeleteAutonomousDatabase
* Deletes a single Autonomous Database.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteAutonomousDatabaseAsync(DeleteAutonomousDatabaseRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteAutonomousDatabaseAsync(AutonomousDatabaseName name)
*
deleteAutonomousDatabaseAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteAutonomousDatabaseOperationCallable()
*
deleteAutonomousDatabaseCallable()
*
*
*
*
* RestoreAutonomousDatabase
* Restores a single Autonomous Database.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* restoreAutonomousDatabaseAsync(RestoreAutonomousDatabaseRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* restoreAutonomousDatabaseAsync(AutonomousDatabaseName name, Timestamp restoreTime)
*
restoreAutonomousDatabaseAsync(String name, Timestamp restoreTime)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* restoreAutonomousDatabaseOperationCallable()
*
restoreAutonomousDatabaseCallable()
*
*
*
*
* GenerateAutonomousDatabaseWallet
* Generates a wallet for an Autonomous Database.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* generateAutonomousDatabaseWallet(GenerateAutonomousDatabaseWalletRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* generateAutonomousDatabaseWallet(AutonomousDatabaseName name, GenerateType type, boolean isRegional, String password)
*
generateAutonomousDatabaseWallet(String name, GenerateType type, boolean isRegional, String password)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* generateAutonomousDatabaseWalletCallable()
*
*
*
*
* ListAutonomousDbVersions
* Lists all the available Autonomous Database versions for a project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listAutonomousDbVersions(ListAutonomousDbVersionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listAutonomousDbVersions(LocationName parent)
*
listAutonomousDbVersions(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listAutonomousDbVersionsPagedCallable()
*
listAutonomousDbVersionsCallable()
*
*
*
*
* ListAutonomousDatabaseCharacterSets
* Lists Autonomous Database Character Sets in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listAutonomousDatabaseCharacterSets(ListAutonomousDatabaseCharacterSetsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listAutonomousDatabaseCharacterSets(LocationName parent)
*
listAutonomousDatabaseCharacterSets(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listAutonomousDatabaseCharacterSetsPagedCallable()
*
listAutonomousDatabaseCharacterSetsCallable()
*
*
*
*
* ListAutonomousDatabaseBackups
* Lists the long-term and automatic backups of an Autonomous Database.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listAutonomousDatabaseBackups(ListAutonomousDatabaseBackupsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listAutonomousDatabaseBackups(LocationName parent)
*
listAutonomousDatabaseBackups(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listAutonomousDatabaseBackupsPagedCallable()
*
listAutonomousDatabaseBackupsCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listLocationsPagedCallable()
*
listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getLocationCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of OracleDatabaseSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* OracleDatabaseSettings oracleDatabaseSettings =
* OracleDatabaseSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create(oracleDatabaseSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* OracleDatabaseSettings oracleDatabaseSettings =
* OracleDatabaseSettings.newBuilder().setEndpoint(myEndpoint).build();
* OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create(oracleDatabaseSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class OracleDatabaseClient implements BackgroundResource {
private final OracleDatabaseSettings settings;
private final OracleDatabaseStub stub;
private final OperationsClient httpJsonOperationsClient;
/** Constructs an instance of OracleDatabaseClient with default settings. */
public static final OracleDatabaseClient create() throws IOException {
return create(OracleDatabaseSettings.newBuilder().build());
}
/**
* Constructs an instance of OracleDatabaseClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final OracleDatabaseClient create(OracleDatabaseSettings settings)
throws IOException {
return new OracleDatabaseClient(settings);
}
/**
* Constructs an instance of OracleDatabaseClient, using the given stub for making calls. This is
* for advanced usage - prefer using create(OracleDatabaseSettings).
*/
public static final OracleDatabaseClient create(OracleDatabaseStub stub) {
return new OracleDatabaseClient(stub);
}
/**
* Constructs an instance of OracleDatabaseClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected OracleDatabaseClient(OracleDatabaseSettings settings) throws IOException {
this.settings = settings;
this.stub = ((OracleDatabaseStubSettings) settings.getStubSettings()).createStub();
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected OracleDatabaseClient(OracleDatabaseStub stub) {
this.settings = null;
this.stub = stub;
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final OracleDatabaseSettings getSettings() {
return settings;
}
public OracleDatabaseStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Exadata Infrastructures in a given project and location.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (CloudExadataInfrastructure element :
* oracleDatabaseClient.listCloudExadataInfrastructures(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for CloudExadataInfrastructure in the following
* format: projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListCloudExadataInfrastructuresPagedResponse listCloudExadataInfrastructures(
LocationName parent) {
ListCloudExadataInfrastructuresRequest request =
ListCloudExadataInfrastructuresRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listCloudExadataInfrastructures(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Exadata Infrastructures in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (CloudExadataInfrastructure element :
* oracleDatabaseClient.listCloudExadataInfrastructures(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for CloudExadataInfrastructure in the following
* format: projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListCloudExadataInfrastructuresPagedResponse listCloudExadataInfrastructures(
String parent) {
ListCloudExadataInfrastructuresRequest request =
ListCloudExadataInfrastructuresRequest.newBuilder().setParent(parent).build();
return listCloudExadataInfrastructures(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Exadata Infrastructures in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListCloudExadataInfrastructuresRequest request =
* ListCloudExadataInfrastructuresRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (CloudExadataInfrastructure element :
* oracleDatabaseClient.listCloudExadataInfrastructures(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListCloudExadataInfrastructuresPagedResponse listCloudExadataInfrastructures(
ListCloudExadataInfrastructuresRequest request) {
return listCloudExadataInfrastructuresPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Exadata Infrastructures in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListCloudExadataInfrastructuresRequest request =
* ListCloudExadataInfrastructuresRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listCloudExadataInfrastructuresPagedCallable().futureCall(request);
* // Do something.
* for (CloudExadataInfrastructure element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable<
ListCloudExadataInfrastructuresRequest, ListCloudExadataInfrastructuresPagedResponse>
listCloudExadataInfrastructuresPagedCallable() {
return stub.listCloudExadataInfrastructuresPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Exadata Infrastructures in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListCloudExadataInfrastructuresRequest request =
* ListCloudExadataInfrastructuresRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListCloudExadataInfrastructuresResponse response =
* oracleDatabaseClient.listCloudExadataInfrastructuresCallable().call(request);
* for (CloudExadataInfrastructure element : response.getCloudExadataInfrastructuresList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable<
ListCloudExadataInfrastructuresRequest, ListCloudExadataInfrastructuresResponse>
listCloudExadataInfrastructuresCallable() {
return stub.listCloudExadataInfrastructuresCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Exadata Infrastructure.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CloudExadataInfrastructureName name =
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]");
* CloudExadataInfrastructure response =
* oracleDatabaseClient.getCloudExadataInfrastructure(name);
* }
* }
*
* @param name Required. The name of the Cloud Exadata Infrastructure in the following format:
* projects/{project}/locations/{location}/cloudExadataInfrastructures/{cloud_exadata_infrastructure}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CloudExadataInfrastructure getCloudExadataInfrastructure(
CloudExadataInfrastructureName name) {
GetCloudExadataInfrastructureRequest request =
GetCloudExadataInfrastructureRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getCloudExadataInfrastructure(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Exadata Infrastructure.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String name =
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString();
* CloudExadataInfrastructure response =
* oracleDatabaseClient.getCloudExadataInfrastructure(name);
* }
* }
*
* @param name Required. The name of the Cloud Exadata Infrastructure in the following format:
* projects/{project}/locations/{location}/cloudExadataInfrastructures/{cloud_exadata_infrastructure}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CloudExadataInfrastructure getCloudExadataInfrastructure(String name) {
GetCloudExadataInfrastructureRequest request =
GetCloudExadataInfrastructureRequest.newBuilder().setName(name).build();
return getCloudExadataInfrastructure(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Exadata Infrastructure.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GetCloudExadataInfrastructureRequest request =
* GetCloudExadataInfrastructureRequest.newBuilder()
* .setName(
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString())
* .build();
* CloudExadataInfrastructure response =
* oracleDatabaseClient.getCloudExadataInfrastructure(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CloudExadataInfrastructure getCloudExadataInfrastructure(
GetCloudExadataInfrastructureRequest request) {
return getCloudExadataInfrastructureCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single Exadata Infrastructure.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GetCloudExadataInfrastructureRequest request =
* GetCloudExadataInfrastructureRequest.newBuilder()
* .setName(
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString())
* .build();
* ApiFuture future =
* oracleDatabaseClient.getCloudExadataInfrastructureCallable().futureCall(request);
* // Do something.
* CloudExadataInfrastructure response = future.get();
* }
* }
*/
public final UnaryCallable
getCloudExadataInfrastructureCallable() {
return stub.getCloudExadataInfrastructureCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Exadata Infrastructure in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* CloudExadataInfrastructure cloudExadataInfrastructure =
* CloudExadataInfrastructure.newBuilder().build();
* String cloudExadataInfrastructureId = "cloudExadataInfrastructureId975345409";
* CloudExadataInfrastructure response =
* oracleDatabaseClient
* .createCloudExadataInfrastructureAsync(
* parent, cloudExadataInfrastructure, cloudExadataInfrastructureId)
* .get();
* }
* }
*
* @param parent Required. The parent value for CloudExadataInfrastructure in the following
* format: projects/{project}/locations/{location}.
* @param cloudExadataInfrastructure Required. Details of the Exadata Infrastructure instance to
* create.
* @param cloudExadataInfrastructureId Required. The ID of the Exadata Infrastructure to create.
* This value is restricted to (^[a-z]([a-z0-9-]{0,61}[a-z0-9])?$) and must be a maximum of 63
* characters in length. The value must start with a letter and end with a letter or a number.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
createCloudExadataInfrastructureAsync(
LocationName parent,
CloudExadataInfrastructure cloudExadataInfrastructure,
String cloudExadataInfrastructureId) {
CreateCloudExadataInfrastructureRequest request =
CreateCloudExadataInfrastructureRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setCloudExadataInfrastructure(cloudExadataInfrastructure)
.setCloudExadataInfrastructureId(cloudExadataInfrastructureId)
.build();
return createCloudExadataInfrastructureAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Exadata Infrastructure in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* CloudExadataInfrastructure cloudExadataInfrastructure =
* CloudExadataInfrastructure.newBuilder().build();
* String cloudExadataInfrastructureId = "cloudExadataInfrastructureId975345409";
* CloudExadataInfrastructure response =
* oracleDatabaseClient
* .createCloudExadataInfrastructureAsync(
* parent, cloudExadataInfrastructure, cloudExadataInfrastructureId)
* .get();
* }
* }
*
* @param parent Required. The parent value for CloudExadataInfrastructure in the following
* format: projects/{project}/locations/{location}.
* @param cloudExadataInfrastructure Required. Details of the Exadata Infrastructure instance to
* create.
* @param cloudExadataInfrastructureId Required. The ID of the Exadata Infrastructure to create.
* This value is restricted to (^[a-z]([a-z0-9-]{0,61}[a-z0-9])?$) and must be a maximum of 63
* characters in length. The value must start with a letter and end with a letter or a number.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
createCloudExadataInfrastructureAsync(
String parent,
CloudExadataInfrastructure cloudExadataInfrastructure,
String cloudExadataInfrastructureId) {
CreateCloudExadataInfrastructureRequest request =
CreateCloudExadataInfrastructureRequest.newBuilder()
.setParent(parent)
.setCloudExadataInfrastructure(cloudExadataInfrastructure)
.setCloudExadataInfrastructureId(cloudExadataInfrastructureId)
.build();
return createCloudExadataInfrastructureAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Exadata Infrastructure in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CreateCloudExadataInfrastructureRequest request =
* CreateCloudExadataInfrastructureRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setCloudExadataInfrastructureId("cloudExadataInfrastructureId975345409")
* .setCloudExadataInfrastructure(CloudExadataInfrastructure.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* CloudExadataInfrastructure response =
* oracleDatabaseClient.createCloudExadataInfrastructureAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
createCloudExadataInfrastructureAsync(CreateCloudExadataInfrastructureRequest request) {
return createCloudExadataInfrastructureOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Exadata Infrastructure in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CreateCloudExadataInfrastructureRequest request =
* CreateCloudExadataInfrastructureRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setCloudExadataInfrastructureId("cloudExadataInfrastructureId975345409")
* .setCloudExadataInfrastructure(CloudExadataInfrastructure.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* oracleDatabaseClient
* .createCloudExadataInfrastructureOperationCallable()
* .futureCall(request);
* // Do something.
* CloudExadataInfrastructure response = future.get();
* }
* }
*/
public final OperationCallable<
CreateCloudExadataInfrastructureRequest, CloudExadataInfrastructure, OperationMetadata>
createCloudExadataInfrastructureOperationCallable() {
return stub.createCloudExadataInfrastructureOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Exadata Infrastructure in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CreateCloudExadataInfrastructureRequest request =
* CreateCloudExadataInfrastructureRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setCloudExadataInfrastructureId("cloudExadataInfrastructureId975345409")
* .setCloudExadataInfrastructure(CloudExadataInfrastructure.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* oracleDatabaseClient.createCloudExadataInfrastructureCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
createCloudExadataInfrastructureCallable() {
return stub.createCloudExadataInfrastructureCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Exadata Infrastructure.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CloudExadataInfrastructureName name =
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]");
* oracleDatabaseClient.deleteCloudExadataInfrastructureAsync(name).get();
* }
* }
*
* @param name Required. The name of the Cloud Exadata Infrastructure in the following format:
* projects/{project}/locations/{location}/cloudExadataInfrastructures/{cloud_exadata_infrastructure}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteCloudExadataInfrastructureAsync(
CloudExadataInfrastructureName name) {
DeleteCloudExadataInfrastructureRequest request =
DeleteCloudExadataInfrastructureRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteCloudExadataInfrastructureAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Exadata Infrastructure.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String name =
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString();
* oracleDatabaseClient.deleteCloudExadataInfrastructureAsync(name).get();
* }
* }
*
* @param name Required. The name of the Cloud Exadata Infrastructure in the following format:
* projects/{project}/locations/{location}/cloudExadataInfrastructures/{cloud_exadata_infrastructure}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteCloudExadataInfrastructureAsync(
String name) {
DeleteCloudExadataInfrastructureRequest request =
DeleteCloudExadataInfrastructureRequest.newBuilder().setName(name).build();
return deleteCloudExadataInfrastructureAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Exadata Infrastructure.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* DeleteCloudExadataInfrastructureRequest request =
* DeleteCloudExadataInfrastructureRequest.newBuilder()
* .setName(
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* oracleDatabaseClient.deleteCloudExadataInfrastructureAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteCloudExadataInfrastructureAsync(
DeleteCloudExadataInfrastructureRequest request) {
return deleteCloudExadataInfrastructureOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Exadata Infrastructure.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* DeleteCloudExadataInfrastructureRequest request =
* DeleteCloudExadataInfrastructureRequest.newBuilder()
* .setName(
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* OperationFuture future =
* oracleDatabaseClient
* .deleteCloudExadataInfrastructureOperationCallable()
* .futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteCloudExadataInfrastructureOperationCallable() {
return stub.deleteCloudExadataInfrastructureOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Exadata Infrastructure.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* DeleteCloudExadataInfrastructureRequest request =
* DeleteCloudExadataInfrastructureRequest.newBuilder()
* .setName(
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* ApiFuture future =
* oracleDatabaseClient.deleteCloudExadataInfrastructureCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable
deleteCloudExadataInfrastructureCallable() {
return stub.deleteCloudExadataInfrastructureCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VM Clusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (CloudVmCluster element : oracleDatabaseClient.listCloudVmClusters(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the parent in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListCloudVmClustersPagedResponse listCloudVmClusters(LocationName parent) {
ListCloudVmClustersRequest request =
ListCloudVmClustersRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listCloudVmClusters(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VM Clusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (CloudVmCluster element : oracleDatabaseClient.listCloudVmClusters(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of the parent in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListCloudVmClustersPagedResponse listCloudVmClusters(String parent) {
ListCloudVmClustersRequest request =
ListCloudVmClustersRequest.newBuilder().setParent(parent).build();
return listCloudVmClusters(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VM Clusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListCloudVmClustersRequest request =
* ListCloudVmClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* for (CloudVmCluster element :
* oracleDatabaseClient.listCloudVmClusters(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListCloudVmClustersPagedResponse listCloudVmClusters(
ListCloudVmClustersRequest request) {
return listCloudVmClustersPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VM Clusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListCloudVmClustersRequest request =
* ListCloudVmClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listCloudVmClustersPagedCallable().futureCall(request);
* // Do something.
* for (CloudVmCluster element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listCloudVmClustersPagedCallable() {
return stub.listCloudVmClustersPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the VM Clusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListCloudVmClustersRequest request =
* ListCloudVmClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* while (true) {
* ListCloudVmClustersResponse response =
* oracleDatabaseClient.listCloudVmClustersCallable().call(request);
* for (CloudVmCluster element : response.getCloudVmClustersList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listCloudVmClustersCallable() {
return stub.listCloudVmClustersCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CloudVmClusterName name =
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]");
* CloudVmCluster response = oracleDatabaseClient.getCloudVmCluster(name);
* }
* }
*
* @param name Required. The name of the Cloud VM Cluster in the following format:
* projects/{project}/locations/{location}/cloudVmClusters/{cloud_vm_cluster}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CloudVmCluster getCloudVmCluster(CloudVmClusterName name) {
GetCloudVmClusterRequest request =
GetCloudVmClusterRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getCloudVmCluster(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String name =
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString();
* CloudVmCluster response = oracleDatabaseClient.getCloudVmCluster(name);
* }
* }
*
* @param name Required. The name of the Cloud VM Cluster in the following format:
* projects/{project}/locations/{location}/cloudVmClusters/{cloud_vm_cluster}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CloudVmCluster getCloudVmCluster(String name) {
GetCloudVmClusterRequest request = GetCloudVmClusterRequest.newBuilder().setName(name).build();
return getCloudVmCluster(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GetCloudVmClusterRequest request =
* GetCloudVmClusterRequest.newBuilder()
* .setName(
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString())
* .build();
* CloudVmCluster response = oracleDatabaseClient.getCloudVmCluster(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CloudVmCluster getCloudVmCluster(GetCloudVmClusterRequest request) {
return getCloudVmClusterCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GetCloudVmClusterRequest request =
* GetCloudVmClusterRequest.newBuilder()
* .setName(
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString())
* .build();
* ApiFuture future =
* oracleDatabaseClient.getCloudVmClusterCallable().futureCall(request);
* // Do something.
* CloudVmCluster response = future.get();
* }
* }
*/
public final UnaryCallable getCloudVmClusterCallable() {
return stub.getCloudVmClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new VM Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* CloudVmCluster cloudVmCluster = CloudVmCluster.newBuilder().build();
* String cloudVmClusterId = "cloudVmClusterId-1217103287";
* CloudVmCluster response =
* oracleDatabaseClient
* .createCloudVmClusterAsync(parent, cloudVmCluster, cloudVmClusterId)
* .get();
* }
* }
*
* @param parent Required. The name of the parent in the following format:
* projects/{project}/locations/{location}.
* @param cloudVmCluster Required. The resource being created
* @param cloudVmClusterId Required. The ID of the VM Cluster to create. This value is restricted
* to (^[a-z]([a-z0-9-]{0,61}[a-z0-9])?$) and must be a maximum of 63 characters in length.
* The value must start with a letter and end with a letter or a number.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createCloudVmClusterAsync(
LocationName parent, CloudVmCluster cloudVmCluster, String cloudVmClusterId) {
CreateCloudVmClusterRequest request =
CreateCloudVmClusterRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setCloudVmCluster(cloudVmCluster)
.setCloudVmClusterId(cloudVmClusterId)
.build();
return createCloudVmClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new VM Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* CloudVmCluster cloudVmCluster = CloudVmCluster.newBuilder().build();
* String cloudVmClusterId = "cloudVmClusterId-1217103287";
* CloudVmCluster response =
* oracleDatabaseClient
* .createCloudVmClusterAsync(parent, cloudVmCluster, cloudVmClusterId)
* .get();
* }
* }
*
* @param parent Required. The name of the parent in the following format:
* projects/{project}/locations/{location}.
* @param cloudVmCluster Required. The resource being created
* @param cloudVmClusterId Required. The ID of the VM Cluster to create. This value is restricted
* to (^[a-z]([a-z0-9-]{0,61}[a-z0-9])?$) and must be a maximum of 63 characters in length.
* The value must start with a letter and end with a letter or a number.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createCloudVmClusterAsync(
String parent, CloudVmCluster cloudVmCluster, String cloudVmClusterId) {
CreateCloudVmClusterRequest request =
CreateCloudVmClusterRequest.newBuilder()
.setParent(parent)
.setCloudVmCluster(cloudVmCluster)
.setCloudVmClusterId(cloudVmClusterId)
.build();
return createCloudVmClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new VM Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CreateCloudVmClusterRequest request =
* CreateCloudVmClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setCloudVmClusterId("cloudVmClusterId-1217103287")
* .setCloudVmCluster(CloudVmCluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* CloudVmCluster response = oracleDatabaseClient.createCloudVmClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createCloudVmClusterAsync(
CreateCloudVmClusterRequest request) {
return createCloudVmClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new VM Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CreateCloudVmClusterRequest request =
* CreateCloudVmClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setCloudVmClusterId("cloudVmClusterId-1217103287")
* .setCloudVmCluster(CloudVmCluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* oracleDatabaseClient.createCloudVmClusterOperationCallable().futureCall(request);
* // Do something.
* CloudVmCluster response = future.get();
* }
* }
*/
public final OperationCallable
createCloudVmClusterOperationCallable() {
return stub.createCloudVmClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new VM Cluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CreateCloudVmClusterRequest request =
* CreateCloudVmClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setCloudVmClusterId("cloudVmClusterId-1217103287")
* .setCloudVmCluster(CloudVmCluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* oracleDatabaseClient.createCloudVmClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
createCloudVmClusterCallable() {
return stub.createCloudVmClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CloudVmClusterName name =
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]");
* oracleDatabaseClient.deleteCloudVmClusterAsync(name).get();
* }
* }
*
* @param name Required. The name of the Cloud VM Cluster in the following format:
* projects/{project}/locations/{location}/cloudVmClusters/{cloud_vm_cluster}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteCloudVmClusterAsync(
CloudVmClusterName name) {
DeleteCloudVmClusterRequest request =
DeleteCloudVmClusterRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteCloudVmClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String name =
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString();
* oracleDatabaseClient.deleteCloudVmClusterAsync(name).get();
* }
* }
*
* @param name Required. The name of the Cloud VM Cluster in the following format:
* projects/{project}/locations/{location}/cloudVmClusters/{cloud_vm_cluster}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteCloudVmClusterAsync(String name) {
DeleteCloudVmClusterRequest request =
DeleteCloudVmClusterRequest.newBuilder().setName(name).build();
return deleteCloudVmClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* DeleteCloudVmClusterRequest request =
* DeleteCloudVmClusterRequest.newBuilder()
* .setName(
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* oracleDatabaseClient.deleteCloudVmClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteCloudVmClusterAsync(
DeleteCloudVmClusterRequest request) {
return deleteCloudVmClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* DeleteCloudVmClusterRequest request =
* DeleteCloudVmClusterRequest.newBuilder()
* .setName(
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* OperationFuture future =
* oracleDatabaseClient.deleteCloudVmClusterOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteCloudVmClusterOperationCallable() {
return stub.deleteCloudVmClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* DeleteCloudVmClusterRequest request =
* DeleteCloudVmClusterRequest.newBuilder()
* .setName(
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* ApiFuture future =
* oracleDatabaseClient.deleteCloudVmClusterCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable
deleteCloudVmClusterCallable() {
return stub.deleteCloudVmClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the entitlements in a given project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Entitlement element : oracleDatabaseClient.listEntitlements(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for the entitlement in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEntitlementsPagedResponse listEntitlements(LocationName parent) {
ListEntitlementsRequest request =
ListEntitlementsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listEntitlements(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the entitlements in a given project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Entitlement element : oracleDatabaseClient.listEntitlements(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for the entitlement in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEntitlementsPagedResponse listEntitlements(String parent) {
ListEntitlementsRequest request =
ListEntitlementsRequest.newBuilder().setParent(parent).build();
return listEntitlements(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the entitlements in a given project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListEntitlementsRequest request =
* ListEntitlementsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Entitlement element : oracleDatabaseClient.listEntitlements(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEntitlementsPagedResponse listEntitlements(ListEntitlementsRequest request) {
return listEntitlementsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the entitlements in a given project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListEntitlementsRequest request =
* ListEntitlementsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listEntitlementsPagedCallable().futureCall(request);
* // Do something.
* for (Entitlement element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listEntitlementsPagedCallable() {
return stub.listEntitlementsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the entitlements in a given project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListEntitlementsRequest request =
* ListEntitlementsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListEntitlementsResponse response =
* oracleDatabaseClient.listEntitlementsCallable().call(request);
* for (Entitlement element : response.getEntitlementsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listEntitlementsCallable() {
return stub.listEntitlementsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database servers of an Exadata Infrastructure instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CloudExadataInfrastructureName parent =
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]");
* for (DbServer element : oracleDatabaseClient.listDbServers(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for database server in the following format:
* projects/{project}/locations/{location}/cloudExadataInfrastructures/{cloudExadataInfrastructure}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDbServersPagedResponse listDbServers(CloudExadataInfrastructureName parent) {
ListDbServersRequest request =
ListDbServersRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listDbServers(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database servers of an Exadata Infrastructure instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent =
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString();
* for (DbServer element : oracleDatabaseClient.listDbServers(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for database server in the following format:
* projects/{project}/locations/{location}/cloudExadataInfrastructures/{cloudExadataInfrastructure}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDbServersPagedResponse listDbServers(String parent) {
ListDbServersRequest request = ListDbServersRequest.newBuilder().setParent(parent).build();
return listDbServers(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database servers of an Exadata Infrastructure instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListDbServersRequest request =
* ListDbServersRequest.newBuilder()
* .setParent(
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (DbServer element : oracleDatabaseClient.listDbServers(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDbServersPagedResponse listDbServers(ListDbServersRequest request) {
return listDbServersPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database servers of an Exadata Infrastructure instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListDbServersRequest request =
* ListDbServersRequest.newBuilder()
* .setParent(
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listDbServersPagedCallable().futureCall(request);
* // Do something.
* for (DbServer element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listDbServersPagedCallable() {
return stub.listDbServersPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database servers of an Exadata Infrastructure instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListDbServersRequest request =
* ListDbServersRequest.newBuilder()
* .setParent(
* CloudExadataInfrastructureName.of(
* "[PROJECT]", "[LOCATION]", "[CLOUD_EXADATA_INFRASTRUCTURE]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListDbServersResponse response = oracleDatabaseClient.listDbServersCallable().call(request);
* for (DbServer element : response.getDbServersList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listDbServersCallable() {
return stub.listDbServersCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database nodes of a VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CloudVmClusterName parent =
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]");
* for (DbNode element : oracleDatabaseClient.listDbNodes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for database node in the following format:
* projects/{project}/locations/{location}/cloudVmClusters/{cloudVmCluster}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDbNodesPagedResponse listDbNodes(CloudVmClusterName parent) {
ListDbNodesRequest request =
ListDbNodesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listDbNodes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database nodes of a VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent =
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString();
* for (DbNode element : oracleDatabaseClient.listDbNodes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for database node in the following format:
* projects/{project}/locations/{location}/cloudVmClusters/{cloudVmCluster}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDbNodesPagedResponse listDbNodes(String parent) {
ListDbNodesRequest request = ListDbNodesRequest.newBuilder().setParent(parent).build();
return listDbNodes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database nodes of a VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListDbNodesRequest request =
* ListDbNodesRequest.newBuilder()
* .setParent(
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (DbNode element : oracleDatabaseClient.listDbNodes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDbNodesPagedResponse listDbNodes(ListDbNodesRequest request) {
return listDbNodesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database nodes of a VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListDbNodesRequest request =
* ListDbNodesRequest.newBuilder()
* .setParent(
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listDbNodesPagedCallable().futureCall(request);
* // Do something.
* for (DbNode element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listDbNodesPagedCallable() {
return stub.listDbNodesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database nodes of a VM Cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListDbNodesRequest request =
* ListDbNodesRequest.newBuilder()
* .setParent(
* CloudVmClusterName.of("[PROJECT]", "[LOCATION]", "[CLOUD_VM_CLUSTER]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListDbNodesResponse response = oracleDatabaseClient.listDbNodesCallable().call(request);
* for (DbNode element : response.getDbNodesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listDbNodesCallable() {
return stub.listDbNodesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the valid Oracle Grid Infrastructure (GI) versions for the given project and
* location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (GiVersion element : oracleDatabaseClient.listGiVersions(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for Grid Infrastructure Version in the following
* format: Format: projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListGiVersionsPagedResponse listGiVersions(LocationName parent) {
ListGiVersionsRequest request =
ListGiVersionsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listGiVersions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the valid Oracle Grid Infrastructure (GI) versions for the given project and
* location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (GiVersion element : oracleDatabaseClient.listGiVersions(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for Grid Infrastructure Version in the following
* format: Format: projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListGiVersionsPagedResponse listGiVersions(String parent) {
ListGiVersionsRequest request = ListGiVersionsRequest.newBuilder().setParent(parent).build();
return listGiVersions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the valid Oracle Grid Infrastructure (GI) versions for the given project and
* location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListGiVersionsRequest request =
* ListGiVersionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (GiVersion element : oracleDatabaseClient.listGiVersions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListGiVersionsPagedResponse listGiVersions(ListGiVersionsRequest request) {
return listGiVersionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the valid Oracle Grid Infrastructure (GI) versions for the given project and
* location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListGiVersionsRequest request =
* ListGiVersionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listGiVersionsPagedCallable().futureCall(request);
* // Do something.
* for (GiVersion element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listGiVersionsPagedCallable() {
return stub.listGiVersionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the valid Oracle Grid Infrastructure (GI) versions for the given project and
* location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListGiVersionsRequest request =
* ListGiVersionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListGiVersionsResponse response =
* oracleDatabaseClient.listGiVersionsCallable().call(request);
* for (GiVersion element : response.getGiVersionsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listGiVersionsCallable() {
return stub.listGiVersionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database system shapes available for the project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (DbSystemShape element : oracleDatabaseClient.listDbSystemShapes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for Database System Shapes in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDbSystemShapesPagedResponse listDbSystemShapes(LocationName parent) {
ListDbSystemShapesRequest request =
ListDbSystemShapesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listDbSystemShapes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database system shapes available for the project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (DbSystemShape element : oracleDatabaseClient.listDbSystemShapes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for Database System Shapes in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDbSystemShapesPagedResponse listDbSystemShapes(String parent) {
ListDbSystemShapesRequest request =
ListDbSystemShapesRequest.newBuilder().setParent(parent).build();
return listDbSystemShapes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database system shapes available for the project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListDbSystemShapesRequest request =
* ListDbSystemShapesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (DbSystemShape element : oracleDatabaseClient.listDbSystemShapes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDbSystemShapesPagedResponse listDbSystemShapes(
ListDbSystemShapesRequest request) {
return listDbSystemShapesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database system shapes available for the project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListDbSystemShapesRequest request =
* ListDbSystemShapesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listDbSystemShapesPagedCallable().futureCall(request);
* // Do something.
* for (DbSystemShape element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listDbSystemShapesPagedCallable() {
return stub.listDbSystemShapesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the database system shapes available for the project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListDbSystemShapesRequest request =
* ListDbSystemShapesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListDbSystemShapesResponse response =
* oracleDatabaseClient.listDbSystemShapesCallable().call(request);
* for (DbSystemShape element : response.getDbSystemShapesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listDbSystemShapesCallable() {
return stub.listDbSystemShapesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Autonomous Databases in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (AutonomousDatabase element :
* oracleDatabaseClient.listAutonomousDatabases(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for the Autonomous Database in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDatabasesPagedResponse listAutonomousDatabases(LocationName parent) {
ListAutonomousDatabasesRequest request =
ListAutonomousDatabasesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listAutonomousDatabases(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Autonomous Databases in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (AutonomousDatabase element :
* oracleDatabaseClient.listAutonomousDatabases(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for the Autonomous Database in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDatabasesPagedResponse listAutonomousDatabases(String parent) {
ListAutonomousDatabasesRequest request =
ListAutonomousDatabasesRequest.newBuilder().setParent(parent).build();
return listAutonomousDatabases(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Autonomous Databases in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDatabasesRequest request =
* ListAutonomousDatabasesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (AutonomousDatabase element :
* oracleDatabaseClient.listAutonomousDatabases(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDatabasesPagedResponse listAutonomousDatabases(
ListAutonomousDatabasesRequest request) {
return listAutonomousDatabasesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Autonomous Databases in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDatabasesRequest request =
* ListAutonomousDatabasesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listAutonomousDatabasesPagedCallable().futureCall(request);
* // Do something.
* for (AutonomousDatabase element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listAutonomousDatabasesPagedCallable() {
return stub.listAutonomousDatabasesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the Autonomous Databases in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDatabasesRequest request =
* ListAutonomousDatabasesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListAutonomousDatabasesResponse response =
* oracleDatabaseClient.listAutonomousDatabasesCallable().call(request);
* for (AutonomousDatabase element : response.getAutonomousDatabasesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listAutonomousDatabasesCallable() {
return stub.listAutonomousDatabasesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* AutonomousDatabaseName name =
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]");
* AutonomousDatabase response = oracleDatabaseClient.getAutonomousDatabase(name);
* }
* }
*
* @param name Required. The name of the Autonomous Database in the following format:
* projects/{project}/locations/{location}/autonomousDatabases/{autonomous_database}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AutonomousDatabase getAutonomousDatabase(AutonomousDatabaseName name) {
GetAutonomousDatabaseRequest request =
GetAutonomousDatabaseRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getAutonomousDatabase(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String name =
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]").toString();
* AutonomousDatabase response = oracleDatabaseClient.getAutonomousDatabase(name);
* }
* }
*
* @param name Required. The name of the Autonomous Database in the following format:
* projects/{project}/locations/{location}/autonomousDatabases/{autonomous_database}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AutonomousDatabase getAutonomousDatabase(String name) {
GetAutonomousDatabaseRequest request =
GetAutonomousDatabaseRequest.newBuilder().setName(name).build();
return getAutonomousDatabase(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GetAutonomousDatabaseRequest request =
* GetAutonomousDatabaseRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .build();
* AutonomousDatabase response = oracleDatabaseClient.getAutonomousDatabase(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AutonomousDatabase getAutonomousDatabase(GetAutonomousDatabaseRequest request) {
return getAutonomousDatabaseCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GetAutonomousDatabaseRequest request =
* GetAutonomousDatabaseRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .build();
* ApiFuture future =
* oracleDatabaseClient.getAutonomousDatabaseCallable().futureCall(request);
* // Do something.
* AutonomousDatabase response = future.get();
* }
* }
*/
public final UnaryCallable
getAutonomousDatabaseCallable() {
return stub.getAutonomousDatabaseCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Autonomous Database in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* AutonomousDatabase autonomousDatabase = AutonomousDatabase.newBuilder().build();
* String autonomousDatabaseId = "autonomousDatabaseId-1972693114";
* AutonomousDatabase response =
* oracleDatabaseClient
* .createAutonomousDatabaseAsync(parent, autonomousDatabase, autonomousDatabaseId)
* .get();
* }
* }
*
* @param parent Required. The name of the parent in the following format:
* projects/{project}/locations/{location}.
* @param autonomousDatabase Required. The Autonomous Database being created.
* @param autonomousDatabaseId Required. The ID of the Autonomous Database to create. This value
* is restricted to (^[a-z]([a-z0-9-]{0,61}[a-z0-9])?$) and must be a maximum of 63 characters
* in length. The value must start with a letter and end with a letter or a number.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createAutonomousDatabaseAsync(
LocationName parent, AutonomousDatabase autonomousDatabase, String autonomousDatabaseId) {
CreateAutonomousDatabaseRequest request =
CreateAutonomousDatabaseRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setAutonomousDatabase(autonomousDatabase)
.setAutonomousDatabaseId(autonomousDatabaseId)
.build();
return createAutonomousDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Autonomous Database in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* AutonomousDatabase autonomousDatabase = AutonomousDatabase.newBuilder().build();
* String autonomousDatabaseId = "autonomousDatabaseId-1972693114";
* AutonomousDatabase response =
* oracleDatabaseClient
* .createAutonomousDatabaseAsync(parent, autonomousDatabase, autonomousDatabaseId)
* .get();
* }
* }
*
* @param parent Required. The name of the parent in the following format:
* projects/{project}/locations/{location}.
* @param autonomousDatabase Required. The Autonomous Database being created.
* @param autonomousDatabaseId Required. The ID of the Autonomous Database to create. This value
* is restricted to (^[a-z]([a-z0-9-]{0,61}[a-z0-9])?$) and must be a maximum of 63 characters
* in length. The value must start with a letter and end with a letter or a number.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createAutonomousDatabaseAsync(
String parent, AutonomousDatabase autonomousDatabase, String autonomousDatabaseId) {
CreateAutonomousDatabaseRequest request =
CreateAutonomousDatabaseRequest.newBuilder()
.setParent(parent)
.setAutonomousDatabase(autonomousDatabase)
.setAutonomousDatabaseId(autonomousDatabaseId)
.build();
return createAutonomousDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Autonomous Database in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CreateAutonomousDatabaseRequest request =
* CreateAutonomousDatabaseRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setAutonomousDatabaseId("autonomousDatabaseId-1972693114")
* .setAutonomousDatabase(AutonomousDatabase.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* AutonomousDatabase response =
* oracleDatabaseClient.createAutonomousDatabaseAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createAutonomousDatabaseAsync(
CreateAutonomousDatabaseRequest request) {
return createAutonomousDatabaseOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Autonomous Database in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CreateAutonomousDatabaseRequest request =
* CreateAutonomousDatabaseRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setAutonomousDatabaseId("autonomousDatabaseId-1972693114")
* .setAutonomousDatabase(AutonomousDatabase.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* oracleDatabaseClient.createAutonomousDatabaseOperationCallable().futureCall(request);
* // Do something.
* AutonomousDatabase response = future.get();
* }
* }
*/
public final OperationCallable<
CreateAutonomousDatabaseRequest, AutonomousDatabase, OperationMetadata>
createAutonomousDatabaseOperationCallable() {
return stub.createAutonomousDatabaseOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new Autonomous Database in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* CreateAutonomousDatabaseRequest request =
* CreateAutonomousDatabaseRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setAutonomousDatabaseId("autonomousDatabaseId-1972693114")
* .setAutonomousDatabase(AutonomousDatabase.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* oracleDatabaseClient.createAutonomousDatabaseCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
createAutonomousDatabaseCallable() {
return stub.createAutonomousDatabaseCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* AutonomousDatabaseName name =
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]");
* oracleDatabaseClient.deleteAutonomousDatabaseAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource in the following format:
* projects/{project}/locations/{location}/autonomousDatabases/{autonomous_database}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteAutonomousDatabaseAsync(
AutonomousDatabaseName name) {
DeleteAutonomousDatabaseRequest request =
DeleteAutonomousDatabaseRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteAutonomousDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String name =
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]").toString();
* oracleDatabaseClient.deleteAutonomousDatabaseAsync(name).get();
* }
* }
*
* @param name Required. The name of the resource in the following format:
* projects/{project}/locations/{location}/autonomousDatabases/{autonomous_database}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteAutonomousDatabaseAsync(
String name) {
DeleteAutonomousDatabaseRequest request =
DeleteAutonomousDatabaseRequest.newBuilder().setName(name).build();
return deleteAutonomousDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* DeleteAutonomousDatabaseRequest request =
* DeleteAutonomousDatabaseRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .setRequestId("requestId693933066")
* .build();
* oracleDatabaseClient.deleteAutonomousDatabaseAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteAutonomousDatabaseAsync(
DeleteAutonomousDatabaseRequest request) {
return deleteAutonomousDatabaseOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* DeleteAutonomousDatabaseRequest request =
* DeleteAutonomousDatabaseRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* oracleDatabaseClient.deleteAutonomousDatabaseOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteAutonomousDatabaseOperationCallable() {
return stub.deleteAutonomousDatabaseOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* DeleteAutonomousDatabaseRequest request =
* DeleteAutonomousDatabaseRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* oracleDatabaseClient.deleteAutonomousDatabaseCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable
deleteAutonomousDatabaseCallable() {
return stub.deleteAutonomousDatabaseCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restores a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* AutonomousDatabaseName name =
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]");
* Timestamp restoreTime = Timestamp.newBuilder().build();
* AutonomousDatabase response =
* oracleDatabaseClient.restoreAutonomousDatabaseAsync(name, restoreTime).get();
* }
* }
*
* @param name Required. The name of the Autonomous Database in the following format:
* projects/{project}/locations/{location}/autonomousDatabases/{autonomous_database}.
* @param restoreTime Required. The time and date to restore the database to.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
restoreAutonomousDatabaseAsync(AutonomousDatabaseName name, Timestamp restoreTime) {
RestoreAutonomousDatabaseRequest request =
RestoreAutonomousDatabaseRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setRestoreTime(restoreTime)
.build();
return restoreAutonomousDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restores a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String name =
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]").toString();
* Timestamp restoreTime = Timestamp.newBuilder().build();
* AutonomousDatabase response =
* oracleDatabaseClient.restoreAutonomousDatabaseAsync(name, restoreTime).get();
* }
* }
*
* @param name Required. The name of the Autonomous Database in the following format:
* projects/{project}/locations/{location}/autonomousDatabases/{autonomous_database}.
* @param restoreTime Required. The time and date to restore the database to.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
restoreAutonomousDatabaseAsync(String name, Timestamp restoreTime) {
RestoreAutonomousDatabaseRequest request =
RestoreAutonomousDatabaseRequest.newBuilder()
.setName(name)
.setRestoreTime(restoreTime)
.build();
return restoreAutonomousDatabaseAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restores a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* RestoreAutonomousDatabaseRequest request =
* RestoreAutonomousDatabaseRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .setRestoreTime(Timestamp.newBuilder().build())
* .build();
* AutonomousDatabase response =
* oracleDatabaseClient.restoreAutonomousDatabaseAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
restoreAutonomousDatabaseAsync(RestoreAutonomousDatabaseRequest request) {
return restoreAutonomousDatabaseOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restores a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* RestoreAutonomousDatabaseRequest request =
* RestoreAutonomousDatabaseRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .setRestoreTime(Timestamp.newBuilder().build())
* .build();
* OperationFuture future =
* oracleDatabaseClient.restoreAutonomousDatabaseOperationCallable().futureCall(request);
* // Do something.
* AutonomousDatabase response = future.get();
* }
* }
*/
public final OperationCallable<
RestoreAutonomousDatabaseRequest, AutonomousDatabase, OperationMetadata>
restoreAutonomousDatabaseOperationCallable() {
return stub.restoreAutonomousDatabaseOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restores a single Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* RestoreAutonomousDatabaseRequest request =
* RestoreAutonomousDatabaseRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .setRestoreTime(Timestamp.newBuilder().build())
* .build();
* ApiFuture future =
* oracleDatabaseClient.restoreAutonomousDatabaseCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
restoreAutonomousDatabaseCallable() {
return stub.restoreAutonomousDatabaseCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generates a wallet for an Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* AutonomousDatabaseName name =
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]");
* GenerateType type = GenerateType.forNumber(0);
* boolean isRegional = true;
* String password = "password1216985755";
* GenerateAutonomousDatabaseWalletResponse response =
* oracleDatabaseClient.generateAutonomousDatabaseWallet(name, type, isRegional, password);
* }
* }
*
* @param name Required. The name of the Autonomous Database in the following format:
* projects/{project}/locations/{location}/autonomousDatabases/{autonomous_database}.
* @param type Optional. The type of wallet generation for the Autonomous Database. The default
* value is SINGLE.
* @param isRegional Optional. True when requesting regional connection strings in PDB connect
* info, applicable to cross-region Data Guard only.
* @param password Required. The password used to encrypt the keys inside the wallet. The password
* must be a minimum of 8 characters.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAutonomousDatabaseWalletResponse generateAutonomousDatabaseWallet(
AutonomousDatabaseName name, GenerateType type, boolean isRegional, String password) {
GenerateAutonomousDatabaseWalletRequest request =
GenerateAutonomousDatabaseWalletRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setType(type)
.setIsRegional(isRegional)
.setPassword(password)
.build();
return generateAutonomousDatabaseWallet(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generates a wallet for an Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String name =
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]").toString();
* GenerateType type = GenerateType.forNumber(0);
* boolean isRegional = true;
* String password = "password1216985755";
* GenerateAutonomousDatabaseWalletResponse response =
* oracleDatabaseClient.generateAutonomousDatabaseWallet(name, type, isRegional, password);
* }
* }
*
* @param name Required. The name of the Autonomous Database in the following format:
* projects/{project}/locations/{location}/autonomousDatabases/{autonomous_database}.
* @param type Optional. The type of wallet generation for the Autonomous Database. The default
* value is SINGLE.
* @param isRegional Optional. True when requesting regional connection strings in PDB connect
* info, applicable to cross-region Data Guard only.
* @param password Required. The password used to encrypt the keys inside the wallet. The password
* must be a minimum of 8 characters.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAutonomousDatabaseWalletResponse generateAutonomousDatabaseWallet(
String name, GenerateType type, boolean isRegional, String password) {
GenerateAutonomousDatabaseWalletRequest request =
GenerateAutonomousDatabaseWalletRequest.newBuilder()
.setName(name)
.setType(type)
.setIsRegional(isRegional)
.setPassword(password)
.build();
return generateAutonomousDatabaseWallet(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generates a wallet for an Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GenerateAutonomousDatabaseWalletRequest request =
* GenerateAutonomousDatabaseWalletRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .setType(GenerateType.forNumber(0))
* .setIsRegional(true)
* .setPassword("password1216985755")
* .build();
* GenerateAutonomousDatabaseWalletResponse response =
* oracleDatabaseClient.generateAutonomousDatabaseWallet(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAutonomousDatabaseWalletResponse generateAutonomousDatabaseWallet(
GenerateAutonomousDatabaseWalletRequest request) {
return generateAutonomousDatabaseWalletCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generates a wallet for an Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GenerateAutonomousDatabaseWalletRequest request =
* GenerateAutonomousDatabaseWalletRequest.newBuilder()
* .setName(
* AutonomousDatabaseName.of("[PROJECT]", "[LOCATION]", "[AUTONOMOUS_DATABASE]")
* .toString())
* .setType(GenerateType.forNumber(0))
* .setIsRegional(true)
* .setPassword("password1216985755")
* .build();
* ApiFuture future =
* oracleDatabaseClient.generateAutonomousDatabaseWalletCallable().futureCall(request);
* // Do something.
* GenerateAutonomousDatabaseWalletResponse response = future.get();
* }
* }
*/
public final UnaryCallable<
GenerateAutonomousDatabaseWalletRequest, GenerateAutonomousDatabaseWalletResponse>
generateAutonomousDatabaseWalletCallable() {
return stub.generateAutonomousDatabaseWalletCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the available Autonomous Database versions for a project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (AutonomousDbVersion element :
* oracleDatabaseClient.listAutonomousDbVersions(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for the Autonomous Database in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDbVersionsPagedResponse listAutonomousDbVersions(LocationName parent) {
ListAutonomousDbVersionsRequest request =
ListAutonomousDbVersionsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listAutonomousDbVersions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the available Autonomous Database versions for a project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (AutonomousDbVersion element :
* oracleDatabaseClient.listAutonomousDbVersions(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for the Autonomous Database in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDbVersionsPagedResponse listAutonomousDbVersions(String parent) {
ListAutonomousDbVersionsRequest request =
ListAutonomousDbVersionsRequest.newBuilder().setParent(parent).build();
return listAutonomousDbVersions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the available Autonomous Database versions for a project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDbVersionsRequest request =
* ListAutonomousDbVersionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (AutonomousDbVersion element :
* oracleDatabaseClient.listAutonomousDbVersions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDbVersionsPagedResponse listAutonomousDbVersions(
ListAutonomousDbVersionsRequest request) {
return listAutonomousDbVersionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the available Autonomous Database versions for a project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDbVersionsRequest request =
* ListAutonomousDbVersionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listAutonomousDbVersionsPagedCallable().futureCall(request);
* // Do something.
* for (AutonomousDbVersion element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listAutonomousDbVersionsPagedCallable() {
return stub.listAutonomousDbVersionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the available Autonomous Database versions for a project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDbVersionsRequest request =
* ListAutonomousDbVersionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListAutonomousDbVersionsResponse response =
* oracleDatabaseClient.listAutonomousDbVersionsCallable().call(request);
* for (AutonomousDbVersion element : response.getAutonomousDbVersionsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listAutonomousDbVersionsCallable() {
return stub.listAutonomousDbVersionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Autonomous Database Character Sets in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (AutonomousDatabaseCharacterSet element :
* oracleDatabaseClient.listAutonomousDatabaseCharacterSets(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for the Autonomous Database in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDatabaseCharacterSetsPagedResponse listAutonomousDatabaseCharacterSets(
LocationName parent) {
ListAutonomousDatabaseCharacterSetsRequest request =
ListAutonomousDatabaseCharacterSetsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listAutonomousDatabaseCharacterSets(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Autonomous Database Character Sets in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (AutonomousDatabaseCharacterSet element :
* oracleDatabaseClient.listAutonomousDatabaseCharacterSets(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for the Autonomous Database in the following format:
* projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDatabaseCharacterSetsPagedResponse listAutonomousDatabaseCharacterSets(
String parent) {
ListAutonomousDatabaseCharacterSetsRequest request =
ListAutonomousDatabaseCharacterSetsRequest.newBuilder().setParent(parent).build();
return listAutonomousDatabaseCharacterSets(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Autonomous Database Character Sets in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDatabaseCharacterSetsRequest request =
* ListAutonomousDatabaseCharacterSetsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* for (AutonomousDatabaseCharacterSet element :
* oracleDatabaseClient.listAutonomousDatabaseCharacterSets(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDatabaseCharacterSetsPagedResponse listAutonomousDatabaseCharacterSets(
ListAutonomousDatabaseCharacterSetsRequest request) {
return listAutonomousDatabaseCharacterSetsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Autonomous Database Character Sets in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDatabaseCharacterSetsRequest request =
* ListAutonomousDatabaseCharacterSetsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* ApiFuture future =
* oracleDatabaseClient
* .listAutonomousDatabaseCharacterSetsPagedCallable()
* .futureCall(request);
* // Do something.
* for (AutonomousDatabaseCharacterSet element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable<
ListAutonomousDatabaseCharacterSetsRequest,
ListAutonomousDatabaseCharacterSetsPagedResponse>
listAutonomousDatabaseCharacterSetsPagedCallable() {
return stub.listAutonomousDatabaseCharacterSetsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists Autonomous Database Character Sets in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDatabaseCharacterSetsRequest request =
* ListAutonomousDatabaseCharacterSetsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .build();
* while (true) {
* ListAutonomousDatabaseCharacterSetsResponse response =
* oracleDatabaseClient.listAutonomousDatabaseCharacterSetsCallable().call(request);
* for (AutonomousDatabaseCharacterSet element :
* response.getAutonomousDatabaseCharacterSetsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable<
ListAutonomousDatabaseCharacterSetsRequest, ListAutonomousDatabaseCharacterSetsResponse>
listAutonomousDatabaseCharacterSetsCallable() {
return stub.listAutonomousDatabaseCharacterSetsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the long-term and automatic backups of an Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (AutonomousDatabaseBackup element :
* oracleDatabaseClient.listAutonomousDatabaseBackups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for ListAutonomousDatabaseBackups in the following
* format: projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDatabaseBackupsPagedResponse listAutonomousDatabaseBackups(
LocationName parent) {
ListAutonomousDatabaseBackupsRequest request =
ListAutonomousDatabaseBackupsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listAutonomousDatabaseBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the long-term and automatic backups of an Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (AutonomousDatabaseBackup element :
* oracleDatabaseClient.listAutonomousDatabaseBackups(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent value for ListAutonomousDatabaseBackups in the following
* format: projects/{project}/locations/{location}.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDatabaseBackupsPagedResponse listAutonomousDatabaseBackups(
String parent) {
ListAutonomousDatabaseBackupsRequest request =
ListAutonomousDatabaseBackupsRequest.newBuilder().setParent(parent).build();
return listAutonomousDatabaseBackups(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the long-term and automatic backups of an Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDatabaseBackupsRequest request =
* ListAutonomousDatabaseBackupsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (AutonomousDatabaseBackup element :
* oracleDatabaseClient.listAutonomousDatabaseBackups(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAutonomousDatabaseBackupsPagedResponse listAutonomousDatabaseBackups(
ListAutonomousDatabaseBackupsRequest request) {
return listAutonomousDatabaseBackupsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the long-term and automatic backups of an Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDatabaseBackupsRequest request =
* ListAutonomousDatabaseBackupsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listAutonomousDatabaseBackupsPagedCallable().futureCall(request);
* // Do something.
* for (AutonomousDatabaseBackup element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable<
ListAutonomousDatabaseBackupsRequest, ListAutonomousDatabaseBackupsPagedResponse>
listAutonomousDatabaseBackupsPagedCallable() {
return stub.listAutonomousDatabaseBackupsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the long-term and automatic backups of an Autonomous Database.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListAutonomousDatabaseBackupsRequest request =
* ListAutonomousDatabaseBackupsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListAutonomousDatabaseBackupsResponse response =
* oracleDatabaseClient.listAutonomousDatabaseBackupsCallable().call(request);
* for (AutonomousDatabaseBackup element : response.getAutonomousDatabaseBackupsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable<
ListAutonomousDatabaseBackupsRequest, ListAutonomousDatabaseBackupsResponse>
listAutonomousDatabaseBackupsCallable() {
return stub.listAutonomousDatabaseBackupsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : oracleDatabaseClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* oracleDatabaseClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response = oracleDatabaseClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = oracleDatabaseClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (OracleDatabaseClient oracleDatabaseClient = OracleDatabaseClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = oracleDatabaseClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListCloudExadataInfrastructuresPagedResponse
extends AbstractPagedListResponse<
ListCloudExadataInfrastructuresRequest,
ListCloudExadataInfrastructuresResponse,
CloudExadataInfrastructure,
ListCloudExadataInfrastructuresPage,
ListCloudExadataInfrastructuresFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListCloudExadataInfrastructuresRequest,
ListCloudExadataInfrastructuresResponse,
CloudExadataInfrastructure>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListCloudExadataInfrastructuresPage.createEmptyPage()
.createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListCloudExadataInfrastructuresPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListCloudExadataInfrastructuresPagedResponse(ListCloudExadataInfrastructuresPage page) {
super(page, ListCloudExadataInfrastructuresFixedSizeCollection.createEmptyCollection());
}
}
public static class ListCloudExadataInfrastructuresPage
extends AbstractPage<
ListCloudExadataInfrastructuresRequest,
ListCloudExadataInfrastructuresResponse,
CloudExadataInfrastructure,
ListCloudExadataInfrastructuresPage> {
private ListCloudExadataInfrastructuresPage(
PageContext<
ListCloudExadataInfrastructuresRequest,
ListCloudExadataInfrastructuresResponse,
CloudExadataInfrastructure>
context,
ListCloudExadataInfrastructuresResponse response) {
super(context, response);
}
private static ListCloudExadataInfrastructuresPage createEmptyPage() {
return new ListCloudExadataInfrastructuresPage(null, null);
}
@Override
protected ListCloudExadataInfrastructuresPage createPage(
PageContext<
ListCloudExadataInfrastructuresRequest,
ListCloudExadataInfrastructuresResponse,
CloudExadataInfrastructure>
context,
ListCloudExadataInfrastructuresResponse response) {
return new ListCloudExadataInfrastructuresPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListCloudExadataInfrastructuresRequest,
ListCloudExadataInfrastructuresResponse,
CloudExadataInfrastructure>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListCloudExadataInfrastructuresFixedSizeCollection
extends AbstractFixedSizeCollection<
ListCloudExadataInfrastructuresRequest,
ListCloudExadataInfrastructuresResponse,
CloudExadataInfrastructure,
ListCloudExadataInfrastructuresPage,
ListCloudExadataInfrastructuresFixedSizeCollection> {
private ListCloudExadataInfrastructuresFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListCloudExadataInfrastructuresFixedSizeCollection createEmptyCollection() {
return new ListCloudExadataInfrastructuresFixedSizeCollection(null, 0);
}
@Override
protected ListCloudExadataInfrastructuresFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListCloudExadataInfrastructuresFixedSizeCollection(pages, collectionSize);
}
}
public static class ListCloudVmClustersPagedResponse
extends AbstractPagedListResponse<
ListCloudVmClustersRequest,
ListCloudVmClustersResponse,
CloudVmCluster,
ListCloudVmClustersPage,
ListCloudVmClustersFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListCloudVmClustersPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListCloudVmClustersPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListCloudVmClustersPagedResponse(ListCloudVmClustersPage page) {
super(page, ListCloudVmClustersFixedSizeCollection.createEmptyCollection());
}
}
public static class ListCloudVmClustersPage
extends AbstractPage<
ListCloudVmClustersRequest,
ListCloudVmClustersResponse,
CloudVmCluster,
ListCloudVmClustersPage> {
private ListCloudVmClustersPage(
PageContext
context,
ListCloudVmClustersResponse response) {
super(context, response);
}
private static ListCloudVmClustersPage createEmptyPage() {
return new ListCloudVmClustersPage(null, null);
}
@Override
protected ListCloudVmClustersPage createPage(
PageContext
context,
ListCloudVmClustersResponse response) {
return new ListCloudVmClustersPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListCloudVmClustersFixedSizeCollection
extends AbstractFixedSizeCollection<
ListCloudVmClustersRequest,
ListCloudVmClustersResponse,
CloudVmCluster,
ListCloudVmClustersPage,
ListCloudVmClustersFixedSizeCollection> {
private ListCloudVmClustersFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListCloudVmClustersFixedSizeCollection createEmptyCollection() {
return new ListCloudVmClustersFixedSizeCollection(null, 0);
}
@Override
protected ListCloudVmClustersFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListCloudVmClustersFixedSizeCollection(pages, collectionSize);
}
}
public static class ListEntitlementsPagedResponse
extends AbstractPagedListResponse<
ListEntitlementsRequest,
ListEntitlementsResponse,
Entitlement,
ListEntitlementsPage,
ListEntitlementsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListEntitlementsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListEntitlementsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListEntitlementsPagedResponse(ListEntitlementsPage page) {
super(page, ListEntitlementsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListEntitlementsPage
extends AbstractPage<
ListEntitlementsRequest, ListEntitlementsResponse, Entitlement, ListEntitlementsPage> {
private ListEntitlementsPage(
PageContext context,
ListEntitlementsResponse response) {
super(context, response);
}
private static ListEntitlementsPage createEmptyPage() {
return new ListEntitlementsPage(null, null);
}
@Override
protected ListEntitlementsPage createPage(
PageContext context,
ListEntitlementsResponse response) {
return new ListEntitlementsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListEntitlementsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListEntitlementsRequest,
ListEntitlementsResponse,
Entitlement,
ListEntitlementsPage,
ListEntitlementsFixedSizeCollection> {
private ListEntitlementsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListEntitlementsFixedSizeCollection createEmptyCollection() {
return new ListEntitlementsFixedSizeCollection(null, 0);
}
@Override
protected ListEntitlementsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListEntitlementsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListDbServersPagedResponse
extends AbstractPagedListResponse<
ListDbServersRequest,
ListDbServersResponse,
DbServer,
ListDbServersPage,
ListDbServersFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListDbServersPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListDbServersPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListDbServersPagedResponse(ListDbServersPage page) {
super(page, ListDbServersFixedSizeCollection.createEmptyCollection());
}
}
public static class ListDbServersPage
extends AbstractPage<
ListDbServersRequest, ListDbServersResponse, DbServer, ListDbServersPage> {
private ListDbServersPage(
PageContext context,
ListDbServersResponse response) {
super(context, response);
}
private static ListDbServersPage createEmptyPage() {
return new ListDbServersPage(null, null);
}
@Override
protected ListDbServersPage createPage(
PageContext context,
ListDbServersResponse response) {
return new ListDbServersPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListDbServersFixedSizeCollection
extends AbstractFixedSizeCollection<
ListDbServersRequest,
ListDbServersResponse,
DbServer,
ListDbServersPage,
ListDbServersFixedSizeCollection> {
private ListDbServersFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListDbServersFixedSizeCollection createEmptyCollection() {
return new ListDbServersFixedSizeCollection(null, 0);
}
@Override
protected ListDbServersFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListDbServersFixedSizeCollection(pages, collectionSize);
}
}
public static class ListDbNodesPagedResponse
extends AbstractPagedListResponse<
ListDbNodesRequest,
ListDbNodesResponse,
DbNode,
ListDbNodesPage,
ListDbNodesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListDbNodesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListDbNodesPagedResponse(input), MoreExecutors.directExecutor());
}
private ListDbNodesPagedResponse(ListDbNodesPage page) {
super(page, ListDbNodesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListDbNodesPage
extends AbstractPage {
private ListDbNodesPage(
PageContext context,
ListDbNodesResponse response) {
super(context, response);
}
private static ListDbNodesPage createEmptyPage() {
return new ListDbNodesPage(null, null);
}
@Override
protected ListDbNodesPage createPage(
PageContext context,
ListDbNodesResponse response) {
return new ListDbNodesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListDbNodesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListDbNodesRequest,
ListDbNodesResponse,
DbNode,
ListDbNodesPage,
ListDbNodesFixedSizeCollection> {
private ListDbNodesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListDbNodesFixedSizeCollection createEmptyCollection() {
return new ListDbNodesFixedSizeCollection(null, 0);
}
@Override
protected ListDbNodesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListDbNodesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListGiVersionsPagedResponse
extends AbstractPagedListResponse<
ListGiVersionsRequest,
ListGiVersionsResponse,
GiVersion,
ListGiVersionsPage,
ListGiVersionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListGiVersionsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListGiVersionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListGiVersionsPagedResponse(ListGiVersionsPage page) {
super(page, ListGiVersionsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListGiVersionsPage
extends AbstractPage<
ListGiVersionsRequest, ListGiVersionsResponse, GiVersion, ListGiVersionsPage> {
private ListGiVersionsPage(
PageContext context,
ListGiVersionsResponse response) {
super(context, response);
}
private static ListGiVersionsPage createEmptyPage() {
return new ListGiVersionsPage(null, null);
}
@Override
protected ListGiVersionsPage createPage(
PageContext context,
ListGiVersionsResponse response) {
return new ListGiVersionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListGiVersionsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListGiVersionsRequest,
ListGiVersionsResponse,
GiVersion,
ListGiVersionsPage,
ListGiVersionsFixedSizeCollection> {
private ListGiVersionsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListGiVersionsFixedSizeCollection createEmptyCollection() {
return new ListGiVersionsFixedSizeCollection(null, 0);
}
@Override
protected ListGiVersionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListGiVersionsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListDbSystemShapesPagedResponse
extends AbstractPagedListResponse<
ListDbSystemShapesRequest,
ListDbSystemShapesResponse,
DbSystemShape,
ListDbSystemShapesPage,
ListDbSystemShapesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListDbSystemShapesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListDbSystemShapesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListDbSystemShapesPagedResponse(ListDbSystemShapesPage page) {
super(page, ListDbSystemShapesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListDbSystemShapesPage
extends AbstractPage<
ListDbSystemShapesRequest,
ListDbSystemShapesResponse,
DbSystemShape,
ListDbSystemShapesPage> {
private ListDbSystemShapesPage(
PageContext context,
ListDbSystemShapesResponse response) {
super(context, response);
}
private static ListDbSystemShapesPage createEmptyPage() {
return new ListDbSystemShapesPage(null, null);
}
@Override
protected ListDbSystemShapesPage createPage(
PageContext context,
ListDbSystemShapesResponse response) {
return new ListDbSystemShapesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListDbSystemShapesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListDbSystemShapesRequest,
ListDbSystemShapesResponse,
DbSystemShape,
ListDbSystemShapesPage,
ListDbSystemShapesFixedSizeCollection> {
private ListDbSystemShapesFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListDbSystemShapesFixedSizeCollection createEmptyCollection() {
return new ListDbSystemShapesFixedSizeCollection(null, 0);
}
@Override
protected ListDbSystemShapesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListDbSystemShapesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListAutonomousDatabasesPagedResponse
extends AbstractPagedListResponse<
ListAutonomousDatabasesRequest,
ListAutonomousDatabasesResponse,
AutonomousDatabase,
ListAutonomousDatabasesPage,
ListAutonomousDatabasesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListAutonomousDatabasesRequest, ListAutonomousDatabasesResponse, AutonomousDatabase>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListAutonomousDatabasesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListAutonomousDatabasesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListAutonomousDatabasesPagedResponse(ListAutonomousDatabasesPage page) {
super(page, ListAutonomousDatabasesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListAutonomousDatabasesPage
extends AbstractPage<
ListAutonomousDatabasesRequest,
ListAutonomousDatabasesResponse,
AutonomousDatabase,
ListAutonomousDatabasesPage> {
private ListAutonomousDatabasesPage(
PageContext<
ListAutonomousDatabasesRequest, ListAutonomousDatabasesResponse, AutonomousDatabase>
context,
ListAutonomousDatabasesResponse response) {
super(context, response);
}
private static ListAutonomousDatabasesPage createEmptyPage() {
return new ListAutonomousDatabasesPage(null, null);
}
@Override
protected ListAutonomousDatabasesPage createPage(
PageContext<
ListAutonomousDatabasesRequest, ListAutonomousDatabasesResponse, AutonomousDatabase>
context,
ListAutonomousDatabasesResponse response) {
return new ListAutonomousDatabasesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListAutonomousDatabasesRequest, ListAutonomousDatabasesResponse, AutonomousDatabase>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListAutonomousDatabasesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListAutonomousDatabasesRequest,
ListAutonomousDatabasesResponse,
AutonomousDatabase,
ListAutonomousDatabasesPage,
ListAutonomousDatabasesFixedSizeCollection> {
private ListAutonomousDatabasesFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListAutonomousDatabasesFixedSizeCollection createEmptyCollection() {
return new ListAutonomousDatabasesFixedSizeCollection(null, 0);
}
@Override
protected ListAutonomousDatabasesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListAutonomousDatabasesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListAutonomousDbVersionsPagedResponse
extends AbstractPagedListResponse<
ListAutonomousDbVersionsRequest,
ListAutonomousDbVersionsResponse,
AutonomousDbVersion,
ListAutonomousDbVersionsPage,
ListAutonomousDbVersionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListAutonomousDbVersionsRequest,
ListAutonomousDbVersionsResponse,
AutonomousDbVersion>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListAutonomousDbVersionsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListAutonomousDbVersionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListAutonomousDbVersionsPagedResponse(ListAutonomousDbVersionsPage page) {
super(page, ListAutonomousDbVersionsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListAutonomousDbVersionsPage
extends AbstractPage<
ListAutonomousDbVersionsRequest,
ListAutonomousDbVersionsResponse,
AutonomousDbVersion,
ListAutonomousDbVersionsPage> {
private ListAutonomousDbVersionsPage(
PageContext<
ListAutonomousDbVersionsRequest,
ListAutonomousDbVersionsResponse,
AutonomousDbVersion>
context,
ListAutonomousDbVersionsResponse response) {
super(context, response);
}
private static ListAutonomousDbVersionsPage createEmptyPage() {
return new ListAutonomousDbVersionsPage(null, null);
}
@Override
protected ListAutonomousDbVersionsPage createPage(
PageContext<
ListAutonomousDbVersionsRequest,
ListAutonomousDbVersionsResponse,
AutonomousDbVersion>
context,
ListAutonomousDbVersionsResponse response) {
return new ListAutonomousDbVersionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListAutonomousDbVersionsRequest,
ListAutonomousDbVersionsResponse,
AutonomousDbVersion>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListAutonomousDbVersionsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListAutonomousDbVersionsRequest,
ListAutonomousDbVersionsResponse,
AutonomousDbVersion,
ListAutonomousDbVersionsPage,
ListAutonomousDbVersionsFixedSizeCollection> {
private ListAutonomousDbVersionsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListAutonomousDbVersionsFixedSizeCollection createEmptyCollection() {
return new ListAutonomousDbVersionsFixedSizeCollection(null, 0);
}
@Override
protected ListAutonomousDbVersionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListAutonomousDbVersionsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListAutonomousDatabaseCharacterSetsPagedResponse
extends AbstractPagedListResponse<
ListAutonomousDatabaseCharacterSetsRequest,
ListAutonomousDatabaseCharacterSetsResponse,
AutonomousDatabaseCharacterSet,
ListAutonomousDatabaseCharacterSetsPage,
ListAutonomousDatabaseCharacterSetsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListAutonomousDatabaseCharacterSetsRequest,
ListAutonomousDatabaseCharacterSetsResponse,
AutonomousDatabaseCharacterSet>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListAutonomousDatabaseCharacterSetsPage.createEmptyPage()
.createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListAutonomousDatabaseCharacterSetsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListAutonomousDatabaseCharacterSetsPagedResponse(
ListAutonomousDatabaseCharacterSetsPage page) {
super(page, ListAutonomousDatabaseCharacterSetsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListAutonomousDatabaseCharacterSetsPage
extends AbstractPage<
ListAutonomousDatabaseCharacterSetsRequest,
ListAutonomousDatabaseCharacterSetsResponse,
AutonomousDatabaseCharacterSet,
ListAutonomousDatabaseCharacterSetsPage> {
private ListAutonomousDatabaseCharacterSetsPage(
PageContext<
ListAutonomousDatabaseCharacterSetsRequest,
ListAutonomousDatabaseCharacterSetsResponse,
AutonomousDatabaseCharacterSet>
context,
ListAutonomousDatabaseCharacterSetsResponse response) {
super(context, response);
}
private static ListAutonomousDatabaseCharacterSetsPage createEmptyPage() {
return new ListAutonomousDatabaseCharacterSetsPage(null, null);
}
@Override
protected ListAutonomousDatabaseCharacterSetsPage createPage(
PageContext<
ListAutonomousDatabaseCharacterSetsRequest,
ListAutonomousDatabaseCharacterSetsResponse,
AutonomousDatabaseCharacterSet>
context,
ListAutonomousDatabaseCharacterSetsResponse response) {
return new ListAutonomousDatabaseCharacterSetsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListAutonomousDatabaseCharacterSetsRequest,
ListAutonomousDatabaseCharacterSetsResponse,
AutonomousDatabaseCharacterSet>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListAutonomousDatabaseCharacterSetsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListAutonomousDatabaseCharacterSetsRequest,
ListAutonomousDatabaseCharacterSetsResponse,
AutonomousDatabaseCharacterSet,
ListAutonomousDatabaseCharacterSetsPage,
ListAutonomousDatabaseCharacterSetsFixedSizeCollection> {
private ListAutonomousDatabaseCharacterSetsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListAutonomousDatabaseCharacterSetsFixedSizeCollection createEmptyCollection() {
return new ListAutonomousDatabaseCharacterSetsFixedSizeCollection(null, 0);
}
@Override
protected ListAutonomousDatabaseCharacterSetsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListAutonomousDatabaseCharacterSetsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListAutonomousDatabaseBackupsPagedResponse
extends AbstractPagedListResponse<
ListAutonomousDatabaseBackupsRequest,
ListAutonomousDatabaseBackupsResponse,
AutonomousDatabaseBackup,
ListAutonomousDatabaseBackupsPage,
ListAutonomousDatabaseBackupsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListAutonomousDatabaseBackupsRequest,
ListAutonomousDatabaseBackupsResponse,
AutonomousDatabaseBackup>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListAutonomousDatabaseBackupsPage.createEmptyPage()
.createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListAutonomousDatabaseBackupsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListAutonomousDatabaseBackupsPagedResponse(ListAutonomousDatabaseBackupsPage page) {
super(page, ListAutonomousDatabaseBackupsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListAutonomousDatabaseBackupsPage
extends AbstractPage<
ListAutonomousDatabaseBackupsRequest,
ListAutonomousDatabaseBackupsResponse,
AutonomousDatabaseBackup,
ListAutonomousDatabaseBackupsPage> {
private ListAutonomousDatabaseBackupsPage(
PageContext<
ListAutonomousDatabaseBackupsRequest,
ListAutonomousDatabaseBackupsResponse,
AutonomousDatabaseBackup>
context,
ListAutonomousDatabaseBackupsResponse response) {
super(context, response);
}
private static ListAutonomousDatabaseBackupsPage createEmptyPage() {
return new ListAutonomousDatabaseBackupsPage(null, null);
}
@Override
protected ListAutonomousDatabaseBackupsPage createPage(
PageContext<
ListAutonomousDatabaseBackupsRequest,
ListAutonomousDatabaseBackupsResponse,
AutonomousDatabaseBackup>
context,
ListAutonomousDatabaseBackupsResponse response) {
return new ListAutonomousDatabaseBackupsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListAutonomousDatabaseBackupsRequest,
ListAutonomousDatabaseBackupsResponse,
AutonomousDatabaseBackup>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListAutonomousDatabaseBackupsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListAutonomousDatabaseBackupsRequest,
ListAutonomousDatabaseBackupsResponse,
AutonomousDatabaseBackup,
ListAutonomousDatabaseBackupsPage,
ListAutonomousDatabaseBackupsFixedSizeCollection> {
private ListAutonomousDatabaseBackupsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListAutonomousDatabaseBackupsFixedSizeCollection createEmptyCollection() {
return new ListAutonomousDatabaseBackupsFixedSizeCollection(null, 0);
}
@Override
protected ListAutonomousDatabaseBackupsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListAutonomousDatabaseBackupsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}