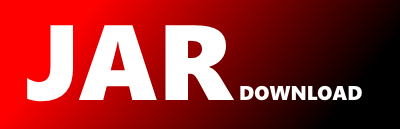
com.google.cloud.oracledatabase.v1.stub.OracleDatabaseStub Maven / Gradle / Ivy
Show all versions of google-cloud-oracledatabase Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.oracledatabase.v1.stub;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListAutonomousDatabaseBackupsPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListAutonomousDatabaseCharacterSetsPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListAutonomousDatabasesPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListAutonomousDbVersionsPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListCloudExadataInfrastructuresPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListCloudVmClustersPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListDbNodesPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListDbServersPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListDbSystemShapesPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListEntitlementsPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListGiVersionsPagedResponse;
import static com.google.cloud.oracledatabase.v1.OracleDatabaseClient.ListLocationsPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.stub.OperationsStub;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.oracledatabase.v1.AutonomousDatabase;
import com.google.cloud.oracledatabase.v1.CloudExadataInfrastructure;
import com.google.cloud.oracledatabase.v1.CloudVmCluster;
import com.google.cloud.oracledatabase.v1.CreateAutonomousDatabaseRequest;
import com.google.cloud.oracledatabase.v1.CreateCloudExadataInfrastructureRequest;
import com.google.cloud.oracledatabase.v1.CreateCloudVmClusterRequest;
import com.google.cloud.oracledatabase.v1.DeleteAutonomousDatabaseRequest;
import com.google.cloud.oracledatabase.v1.DeleteCloudExadataInfrastructureRequest;
import com.google.cloud.oracledatabase.v1.DeleteCloudVmClusterRequest;
import com.google.cloud.oracledatabase.v1.GenerateAutonomousDatabaseWalletRequest;
import com.google.cloud.oracledatabase.v1.GenerateAutonomousDatabaseWalletResponse;
import com.google.cloud.oracledatabase.v1.GetAutonomousDatabaseRequest;
import com.google.cloud.oracledatabase.v1.GetCloudExadataInfrastructureRequest;
import com.google.cloud.oracledatabase.v1.GetCloudVmClusterRequest;
import com.google.cloud.oracledatabase.v1.ListAutonomousDatabaseBackupsRequest;
import com.google.cloud.oracledatabase.v1.ListAutonomousDatabaseBackupsResponse;
import com.google.cloud.oracledatabase.v1.ListAutonomousDatabaseCharacterSetsRequest;
import com.google.cloud.oracledatabase.v1.ListAutonomousDatabaseCharacterSetsResponse;
import com.google.cloud.oracledatabase.v1.ListAutonomousDatabasesRequest;
import com.google.cloud.oracledatabase.v1.ListAutonomousDatabasesResponse;
import com.google.cloud.oracledatabase.v1.ListAutonomousDbVersionsRequest;
import com.google.cloud.oracledatabase.v1.ListAutonomousDbVersionsResponse;
import com.google.cloud.oracledatabase.v1.ListCloudExadataInfrastructuresRequest;
import com.google.cloud.oracledatabase.v1.ListCloudExadataInfrastructuresResponse;
import com.google.cloud.oracledatabase.v1.ListCloudVmClustersRequest;
import com.google.cloud.oracledatabase.v1.ListCloudVmClustersResponse;
import com.google.cloud.oracledatabase.v1.ListDbNodesRequest;
import com.google.cloud.oracledatabase.v1.ListDbNodesResponse;
import com.google.cloud.oracledatabase.v1.ListDbServersRequest;
import com.google.cloud.oracledatabase.v1.ListDbServersResponse;
import com.google.cloud.oracledatabase.v1.ListDbSystemShapesRequest;
import com.google.cloud.oracledatabase.v1.ListDbSystemShapesResponse;
import com.google.cloud.oracledatabase.v1.ListEntitlementsRequest;
import com.google.cloud.oracledatabase.v1.ListEntitlementsResponse;
import com.google.cloud.oracledatabase.v1.ListGiVersionsRequest;
import com.google.cloud.oracledatabase.v1.ListGiVersionsResponse;
import com.google.cloud.oracledatabase.v1.OperationMetadata;
import com.google.cloud.oracledatabase.v1.RestoreAutonomousDatabaseRequest;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Base stub class for the OracleDatabase service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public abstract class OracleDatabaseStub implements BackgroundResource {
public OperationsStub getHttpJsonOperationsStub() {
throw new UnsupportedOperationException("Not implemented: getHttpJsonOperationsStub()");
}
public UnaryCallable<
ListCloudExadataInfrastructuresRequest, ListCloudExadataInfrastructuresPagedResponse>
listCloudExadataInfrastructuresPagedCallable() {
throw new UnsupportedOperationException(
"Not implemented: listCloudExadataInfrastructuresPagedCallable()");
}
public UnaryCallable<
ListCloudExadataInfrastructuresRequest, ListCloudExadataInfrastructuresResponse>
listCloudExadataInfrastructuresCallable() {
throw new UnsupportedOperationException(
"Not implemented: listCloudExadataInfrastructuresCallable()");
}
public UnaryCallable
getCloudExadataInfrastructureCallable() {
throw new UnsupportedOperationException(
"Not implemented: getCloudExadataInfrastructureCallable()");
}
public OperationCallable<
CreateCloudExadataInfrastructureRequest, CloudExadataInfrastructure, OperationMetadata>
createCloudExadataInfrastructureOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: createCloudExadataInfrastructureOperationCallable()");
}
public UnaryCallable
createCloudExadataInfrastructureCallable() {
throw new UnsupportedOperationException(
"Not implemented: createCloudExadataInfrastructureCallable()");
}
public OperationCallable
deleteCloudExadataInfrastructureOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: deleteCloudExadataInfrastructureOperationCallable()");
}
public UnaryCallable
deleteCloudExadataInfrastructureCallable() {
throw new UnsupportedOperationException(
"Not implemented: deleteCloudExadataInfrastructureCallable()");
}
public UnaryCallable
listCloudVmClustersPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listCloudVmClustersPagedCallable()");
}
public UnaryCallable
listCloudVmClustersCallable() {
throw new UnsupportedOperationException("Not implemented: listCloudVmClustersCallable()");
}
public UnaryCallable getCloudVmClusterCallable() {
throw new UnsupportedOperationException("Not implemented: getCloudVmClusterCallable()");
}
public OperationCallable
createCloudVmClusterOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: createCloudVmClusterOperationCallable()");
}
public UnaryCallable createCloudVmClusterCallable() {
throw new UnsupportedOperationException("Not implemented: createCloudVmClusterCallable()");
}
public OperationCallable
deleteCloudVmClusterOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: deleteCloudVmClusterOperationCallable()");
}
public UnaryCallable deleteCloudVmClusterCallable() {
throw new UnsupportedOperationException("Not implemented: deleteCloudVmClusterCallable()");
}
public UnaryCallable
listEntitlementsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listEntitlementsPagedCallable()");
}
public UnaryCallable
listEntitlementsCallable() {
throw new UnsupportedOperationException("Not implemented: listEntitlementsCallable()");
}
public UnaryCallable
listDbServersPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listDbServersPagedCallable()");
}
public UnaryCallable listDbServersCallable() {
throw new UnsupportedOperationException("Not implemented: listDbServersCallable()");
}
public UnaryCallable listDbNodesPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listDbNodesPagedCallable()");
}
public UnaryCallable listDbNodesCallable() {
throw new UnsupportedOperationException("Not implemented: listDbNodesCallable()");
}
public UnaryCallable
listGiVersionsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listGiVersionsPagedCallable()");
}
public UnaryCallable listGiVersionsCallable() {
throw new UnsupportedOperationException("Not implemented: listGiVersionsCallable()");
}
public UnaryCallable
listDbSystemShapesPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listDbSystemShapesPagedCallable()");
}
public UnaryCallable
listDbSystemShapesCallable() {
throw new UnsupportedOperationException("Not implemented: listDbSystemShapesCallable()");
}
public UnaryCallable
listAutonomousDatabasesPagedCallable() {
throw new UnsupportedOperationException(
"Not implemented: listAutonomousDatabasesPagedCallable()");
}
public UnaryCallable
listAutonomousDatabasesCallable() {
throw new UnsupportedOperationException("Not implemented: listAutonomousDatabasesCallable()");
}
public UnaryCallable
getAutonomousDatabaseCallable() {
throw new UnsupportedOperationException("Not implemented: getAutonomousDatabaseCallable()");
}
public OperationCallable
createAutonomousDatabaseOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: createAutonomousDatabaseOperationCallable()");
}
public UnaryCallable
createAutonomousDatabaseCallable() {
throw new UnsupportedOperationException("Not implemented: createAutonomousDatabaseCallable()");
}
public OperationCallable
deleteAutonomousDatabaseOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: deleteAutonomousDatabaseOperationCallable()");
}
public UnaryCallable
deleteAutonomousDatabaseCallable() {
throw new UnsupportedOperationException("Not implemented: deleteAutonomousDatabaseCallable()");
}
public OperationCallable
restoreAutonomousDatabaseOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: restoreAutonomousDatabaseOperationCallable()");
}
public UnaryCallable
restoreAutonomousDatabaseCallable() {
throw new UnsupportedOperationException("Not implemented: restoreAutonomousDatabaseCallable()");
}
public UnaryCallable<
GenerateAutonomousDatabaseWalletRequest, GenerateAutonomousDatabaseWalletResponse>
generateAutonomousDatabaseWalletCallable() {
throw new UnsupportedOperationException(
"Not implemented: generateAutonomousDatabaseWalletCallable()");
}
public UnaryCallable
listAutonomousDbVersionsPagedCallable() {
throw new UnsupportedOperationException(
"Not implemented: listAutonomousDbVersionsPagedCallable()");
}
public UnaryCallable
listAutonomousDbVersionsCallable() {
throw new UnsupportedOperationException("Not implemented: listAutonomousDbVersionsCallable()");
}
public UnaryCallable<
ListAutonomousDatabaseCharacterSetsRequest,
ListAutonomousDatabaseCharacterSetsPagedResponse>
listAutonomousDatabaseCharacterSetsPagedCallable() {
throw new UnsupportedOperationException(
"Not implemented: listAutonomousDatabaseCharacterSetsPagedCallable()");
}
public UnaryCallable<
ListAutonomousDatabaseCharacterSetsRequest, ListAutonomousDatabaseCharacterSetsResponse>
listAutonomousDatabaseCharacterSetsCallable() {
throw new UnsupportedOperationException(
"Not implemented: listAutonomousDatabaseCharacterSetsCallable()");
}
public UnaryCallable<
ListAutonomousDatabaseBackupsRequest, ListAutonomousDatabaseBackupsPagedResponse>
listAutonomousDatabaseBackupsPagedCallable() {
throw new UnsupportedOperationException(
"Not implemented: listAutonomousDatabaseBackupsPagedCallable()");
}
public UnaryCallable
listAutonomousDatabaseBackupsCallable() {
throw new UnsupportedOperationException(
"Not implemented: listAutonomousDatabaseBackupsCallable()");
}
public UnaryCallable
listLocationsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listLocationsPagedCallable()");
}
public UnaryCallable listLocationsCallable() {
throw new UnsupportedOperationException("Not implemented: listLocationsCallable()");
}
public UnaryCallable getLocationCallable() {
throw new UnsupportedOperationException("Not implemented: getLocationCallable()");
}
@Override
public abstract void close();
}