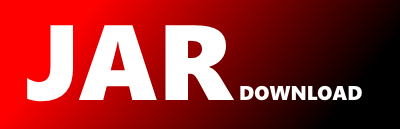
com.google.cloud.recommendationengine.v1beta1.package-info Maven / Gradle / Ivy
Show all versions of google-cloud-recommendations-ai Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
/**
* A client to Recommendations AI
*
* The interfaces provided are listed below, along with usage samples.
*
*
======================= CatalogServiceClient =======================
*
*
Service Description: Service for ingesting catalog information of the customer's website.
*
*
Sample for CatalogServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* CatalogName parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* CatalogItem catalogItem = CatalogItem.newBuilder().build();
* CatalogItem response = catalogServiceClient.createCatalogItem(parent, catalogItem);
* }
* }
*
* ======================= PredictionApiKeyRegistryClient =======================
*
*
Service Description: Service for registering API keys for use with the `predict` method. If
* you use an API key to request predictions, you must first register the API key. Otherwise, your
* prediction request is rejected. If you use OAuth to authenticate your `predict` method call, you
* do not need to register an API key. You can register up to 20 API keys per project.
*
*
Sample for PredictionApiKeyRegistryClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionApiKeyRegistryClient predictionApiKeyRegistryClient =
* PredictionApiKeyRegistryClient.create()) {
* EventStoreName parent =
* EventStoreName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[EVENT_STORE]");
* PredictionApiKeyRegistration predictionApiKeyRegistration =
* PredictionApiKeyRegistration.newBuilder().build();
* PredictionApiKeyRegistration response =
* predictionApiKeyRegistryClient.createPredictionApiKeyRegistration(
* parent, predictionApiKeyRegistration);
* }
* }
*
* ======================= PredictionServiceClient =======================
*
*
Service Description: Service for making recommendation prediction.
*
*
Sample for PredictionServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (PredictionServiceClient predictionServiceClient = PredictionServiceClient.create()) {
* PlacementName name =
* PlacementName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[EVENT_STORE]", "[PLACEMENT]");
* UserEvent userEvent = UserEvent.newBuilder().build();
* for (Map.Entry element :
* predictionServiceClient.predict(name, userEvent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* ======================= UserEventServiceClient =======================
*
*
Service Description: Service for ingesting end user actions on the customer website.
*
*
Sample for UserEventServiceClient:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (UserEventServiceClient userEventServiceClient = UserEventServiceClient.create()) {
* EventStoreName parent =
* EventStoreName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[EVENT_STORE]");
* UserEvent userEvent = UserEvent.newBuilder().build();
* UserEvent response = userEventServiceClient.writeUserEvent(parent, userEvent);
* }
* }
*/
@Generated("by gapic-generator-java")
package com.google.cloud.recommendationengine.v1beta1;
import javax.annotation.Generated;