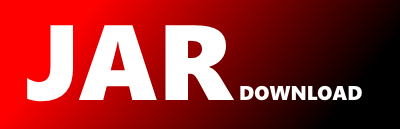
com.google.cloud.retail.v2.CatalogServiceClient Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.retail.v2;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.retail.v2.stub.CatalogServiceStub;
import com.google.cloud.retail.v2.stub.CatalogServiceStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Service for managing catalog configuration.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* Catalog catalog = Catalog.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Catalog response = catalogServiceClient.updateCatalog(catalog, updateMask);
* }
* }
*
* Note: close() needs to be called on the CatalogServiceClient object to clean up resources such
* as threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* ListCatalogs
* Lists all the [Catalog][google.cloud.retail.v2.Catalog]s associated with the project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listCatalogs(ListCatalogsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listCatalogs(LocationName parent)
*
listCatalogs(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listCatalogsPagedCallable()
*
listCatalogsCallable()
*
*
*
*
* UpdateCatalog
* Updates the [Catalog][google.cloud.retail.v2.Catalog]s.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateCatalog(UpdateCatalogRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateCatalog(Catalog catalog, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateCatalogCallable()
*
*
*
*
* SetDefaultBranch
* Set a specified branch id as default branch. API methods such as [SearchService.Search][google.cloud.retail.v2.SearchService.Search], [ProductService.GetProduct][google.cloud.retail.v2.ProductService.GetProduct], [ProductService.ListProducts][google.cloud.retail.v2.ProductService.ListProducts] will treat requests using "default_branch" to the actual branch id set as default.
*
For example, if `projects/*/locations/*/catalogs/*/branches/1` is set as default, setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to `projects/*/locations/*/catalogs/*/branches/default_branch` is equivalent to setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to `projects/*/locations/*/catalogs/*/branches/1`.
*
Using multiple branches can be useful when developers would like to have a staging branch to test and verify for future usage. When it becomes ready, developers switch on the staging branch using this API while keeping using `projects/*/locations/*/catalogs/*/branches/default_branch` as [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to route the traffic to this staging branch.
*
CAUTION: If you have live predict/search traffic, switching the default branch could potentially cause outages if the ID space of the new branch is very different from the old one.
*
More specifically:
*
* - PredictionService will only return product IDs from branch {newBranch}.
*
- SearchService will only return product IDs from branch {newBranch} (if branch is not explicitly set).
*
- UserEventService will only join events with products from branch {newBranch}.
*
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* setDefaultBranch(SetDefaultBranchRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* setDefaultBranch(CatalogName catalog)
*
setDefaultBranch(String catalog)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* setDefaultBranchCallable()
*
*
*
*
* GetDefaultBranch
* Get which branch is currently default branch set by [CatalogService.SetDefaultBranch][google.cloud.retail.v2.CatalogService.SetDefaultBranch] method under a specified parent catalog.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getDefaultBranch(GetDefaultBranchRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getDefaultBranch(CatalogName catalog)
*
getDefaultBranch(String catalog)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getDefaultBranchCallable()
*
*
*
*
* GetCompletionConfig
* Gets a [CompletionConfig][google.cloud.retail.v2.CompletionConfig].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getCompletionConfig(GetCompletionConfigRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getCompletionConfig(CompletionConfigName name)
*
getCompletionConfig(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getCompletionConfigCallable()
*
*
*
*
* UpdateCompletionConfig
* Updates the [CompletionConfig][google.cloud.retail.v2.CompletionConfig]s.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateCompletionConfig(UpdateCompletionConfigRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateCompletionConfig(CompletionConfig completionConfig, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateCompletionConfigCallable()
*
*
*
*
* GetAttributesConfig
* Gets an [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getAttributesConfig(GetAttributesConfigRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getAttributesConfig(AttributesConfigName name)
*
getAttributesConfig(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getAttributesConfigCallable()
*
*
*
*
* UpdateAttributesConfig
* Updates the [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
The catalog attributes in the request will be updated in the catalog, or inserted if they do not exist. Existing catalog attributes not included in the request will remain unchanged. Attributes that are assigned to products, but do not exist at the catalog level, are always included in the response. The product attribute is assigned default values for missing catalog attribute fields, e.g., searchable and dynamic facetable options.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateAttributesConfig(UpdateAttributesConfigRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateAttributesConfig(AttributesConfig attributesConfig, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateAttributesConfigCallable()
*
*
*
*
* AddCatalogAttribute
* Adds the specified [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to the [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
If the [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to add already exists, an ALREADY_EXISTS error is returned.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* addCatalogAttribute(AddCatalogAttributeRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* addCatalogAttributeCallable()
*
*
*
*
* RemoveCatalogAttribute
* Removes the specified [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] from the [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
If the [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to remove does not exist, a NOT_FOUND error is returned.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* removeCatalogAttribute(RemoveCatalogAttributeRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* removeCatalogAttributeCallable()
*
*
*
*
* ReplaceCatalogAttribute
* Replaces the specified [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] in the [AttributesConfig][google.cloud.retail.v2.AttributesConfig] by updating the catalog attribute with the same [CatalogAttribute.key][google.cloud.retail.v2.CatalogAttribute.key].
*
If the [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to replace does not exist, a NOT_FOUND error is returned.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* replaceCatalogAttribute(ReplaceCatalogAttributeRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* replaceCatalogAttributeCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of CatalogServiceSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* CatalogServiceSettings catalogServiceSettings =
* CatalogServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* CatalogServiceClient catalogServiceClient = CatalogServiceClient.create(catalogServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* CatalogServiceSettings catalogServiceSettings =
* CatalogServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* CatalogServiceClient catalogServiceClient = CatalogServiceClient.create(catalogServiceSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* CatalogServiceSettings catalogServiceSettings =
* CatalogServiceSettings.newHttpJsonBuilder().build();
* CatalogServiceClient catalogServiceClient = CatalogServiceClient.create(catalogServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class CatalogServiceClient implements BackgroundResource {
private final CatalogServiceSettings settings;
private final CatalogServiceStub stub;
/** Constructs an instance of CatalogServiceClient with default settings. */
public static final CatalogServiceClient create() throws IOException {
return create(CatalogServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of CatalogServiceClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final CatalogServiceClient create(CatalogServiceSettings settings)
throws IOException {
return new CatalogServiceClient(settings);
}
/**
* Constructs an instance of CatalogServiceClient, using the given stub for making calls. This is
* for advanced usage - prefer using create(CatalogServiceSettings).
*/
public static final CatalogServiceClient create(CatalogServiceStub stub) {
return new CatalogServiceClient(stub);
}
/**
* Constructs an instance of CatalogServiceClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected CatalogServiceClient(CatalogServiceSettings settings) throws IOException {
this.settings = settings;
this.stub = ((CatalogServiceStubSettings) settings.getStubSettings()).createStub();
}
protected CatalogServiceClient(CatalogServiceStub stub) {
this.settings = null;
this.stub = stub;
}
public final CatalogServiceSettings getSettings() {
return settings;
}
public CatalogServiceStub getStub() {
return stub;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the [Catalog][google.cloud.retail.v2.Catalog]s associated with the project.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Catalog element : catalogServiceClient.listCatalogs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The account resource name with an associated location.
* If the caller does not have permission to list
* [Catalog][google.cloud.retail.v2.Catalog]s under this location, regardless of whether or
* not this location exists, a PERMISSION_DENIED error is returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListCatalogsPagedResponse listCatalogs(LocationName parent) {
ListCatalogsRequest request =
ListCatalogsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listCatalogs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the [Catalog][google.cloud.retail.v2.Catalog]s associated with the project.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Catalog element : catalogServiceClient.listCatalogs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The account resource name with an associated location.
* If the caller does not have permission to list
* [Catalog][google.cloud.retail.v2.Catalog]s under this location, regardless of whether or
* not this location exists, a PERMISSION_DENIED error is returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListCatalogsPagedResponse listCatalogs(String parent) {
ListCatalogsRequest request = ListCatalogsRequest.newBuilder().setParent(parent).build();
return listCatalogs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the [Catalog][google.cloud.retail.v2.Catalog]s associated with the project.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* ListCatalogsRequest request =
* ListCatalogsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Catalog element : catalogServiceClient.listCatalogs(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListCatalogsPagedResponse listCatalogs(ListCatalogsRequest request) {
return listCatalogsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the [Catalog][google.cloud.retail.v2.Catalog]s associated with the project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* ListCatalogsRequest request =
* ListCatalogsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* catalogServiceClient.listCatalogsPagedCallable().futureCall(request);
* // Do something.
* for (Catalog element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listCatalogsPagedCallable() {
return stub.listCatalogsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the [Catalog][google.cloud.retail.v2.Catalog]s associated with the project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* ListCatalogsRequest request =
* ListCatalogsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListCatalogsResponse response = catalogServiceClient.listCatalogsCallable().call(request);
* for (Catalog element : response.getCatalogsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listCatalogsCallable() {
return stub.listCatalogsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the [Catalog][google.cloud.retail.v2.Catalog]s.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* Catalog catalog = Catalog.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Catalog response = catalogServiceClient.updateCatalog(catalog, updateMask);
* }
* }
*
* @param catalog Required. The [Catalog][google.cloud.retail.v2.Catalog] to update.
* If the caller does not have permission to update the
* [Catalog][google.cloud.retail.v2.Catalog], regardless of whether or not it exists, a
* PERMISSION_DENIED error is returned.
*
If the [Catalog][google.cloud.retail.v2.Catalog] to update does not exist, a NOT_FOUND
* error is returned.
* @param updateMask Indicates which fields in the provided
* [Catalog][google.cloud.retail.v2.Catalog] to update.
*
If an unsupported or unknown field is provided, an INVALID_ARGUMENT error is returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Catalog updateCatalog(Catalog catalog, FieldMask updateMask) {
UpdateCatalogRequest request =
UpdateCatalogRequest.newBuilder().setCatalog(catalog).setUpdateMask(updateMask).build();
return updateCatalog(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the [Catalog][google.cloud.retail.v2.Catalog]s.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* UpdateCatalogRequest request =
* UpdateCatalogRequest.newBuilder()
* .setCatalog(Catalog.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Catalog response = catalogServiceClient.updateCatalog(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Catalog updateCatalog(UpdateCatalogRequest request) {
return updateCatalogCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the [Catalog][google.cloud.retail.v2.Catalog]s.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* UpdateCatalogRequest request =
* UpdateCatalogRequest.newBuilder()
* .setCatalog(Catalog.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = catalogServiceClient.updateCatalogCallable().futureCall(request);
* // Do something.
* Catalog response = future.get();
* }
* }
*/
public final UnaryCallable updateCatalogCallable() {
return stub.updateCatalogCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Set a specified branch id as default branch. API methods such as
* [SearchService.Search][google.cloud.retail.v2.SearchService.Search],
* [ProductService.GetProduct][google.cloud.retail.v2.ProductService.GetProduct],
* [ProductService.ListProducts][google.cloud.retail.v2.ProductService.ListProducts] will treat
* requests using "default_branch" to the actual branch id set as default.
*
* For example, if `projects/*/locations/*/catalogs/*/branches/1` is set as
* default, setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to
* `projects/*/locations/*/catalogs/*/branches/default_branch` is equivalent to
* setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to
* `projects/*/locations/*/catalogs/*/branches/1`.
*
*
Using multiple branches can be useful when developers would like to have a staging branch to
* test and verify for future usage. When it becomes ready, developers switch on the staging
* branch using this API while keeping using
* `projects/*/locations/*/catalogs/*/branches/default_branch` as
* [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to route the traffic to
* this staging branch.
*
*
CAUTION: If you have live predict/search traffic, switching the default branch could
* potentially cause outages if the ID space of the new branch is very different from the old one.
*
*
More specifically:
*
*
* - PredictionService will only return product IDs from branch {newBranch}.
*
- SearchService will only return product IDs from branch {newBranch} (if branch is not
* explicitly set).
*
- UserEventService will only join events with products from branch {newBranch}.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* CatalogName catalog = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* catalogServiceClient.setDefaultBranch(catalog);
* }
* }
*
* @param catalog Full resource name of the catalog, such as
* `projects/*/locations/global/catalogs/default_catalog`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void setDefaultBranch(CatalogName catalog) {
SetDefaultBranchRequest request =
SetDefaultBranchRequest.newBuilder()
.setCatalog(catalog == null ? null : catalog.toString())
.build();
setDefaultBranch(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Set a specified branch id as default branch. API methods such as
* [SearchService.Search][google.cloud.retail.v2.SearchService.Search],
* [ProductService.GetProduct][google.cloud.retail.v2.ProductService.GetProduct],
* [ProductService.ListProducts][google.cloud.retail.v2.ProductService.ListProducts] will treat
* requests using "default_branch" to the actual branch id set as default.
*
* For example, if `projects/*/locations/*/catalogs/*/branches/1` is set as
* default, setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to
* `projects/*/locations/*/catalogs/*/branches/default_branch` is equivalent to
* setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to
* `projects/*/locations/*/catalogs/*/branches/1`.
*
*
Using multiple branches can be useful when developers would like to have a staging branch to
* test and verify for future usage. When it becomes ready, developers switch on the staging
* branch using this API while keeping using
* `projects/*/locations/*/catalogs/*/branches/default_branch` as
* [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to route the traffic to
* this staging branch.
*
*
CAUTION: If you have live predict/search traffic, switching the default branch could
* potentially cause outages if the ID space of the new branch is very different from the old one.
*
*
More specifically:
*
*
* - PredictionService will only return product IDs from branch {newBranch}.
*
- SearchService will only return product IDs from branch {newBranch} (if branch is not
* explicitly set).
*
- UserEventService will only join events with products from branch {newBranch}.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* String catalog = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString();
* catalogServiceClient.setDefaultBranch(catalog);
* }
* }
*
* @param catalog Full resource name of the catalog, such as
* `projects/*/locations/global/catalogs/default_catalog`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void setDefaultBranch(String catalog) {
SetDefaultBranchRequest request =
SetDefaultBranchRequest.newBuilder().setCatalog(catalog).build();
setDefaultBranch(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Set a specified branch id as default branch. API methods such as
* [SearchService.Search][google.cloud.retail.v2.SearchService.Search],
* [ProductService.GetProduct][google.cloud.retail.v2.ProductService.GetProduct],
* [ProductService.ListProducts][google.cloud.retail.v2.ProductService.ListProducts] will treat
* requests using "default_branch" to the actual branch id set as default.
*
* For example, if `projects/*/locations/*/catalogs/*/branches/1` is set as
* default, setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to
* `projects/*/locations/*/catalogs/*/branches/default_branch` is equivalent to
* setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to
* `projects/*/locations/*/catalogs/*/branches/1`.
*
*
Using multiple branches can be useful when developers would like to have a staging branch to
* test and verify for future usage. When it becomes ready, developers switch on the staging
* branch using this API while keeping using
* `projects/*/locations/*/catalogs/*/branches/default_branch` as
* [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to route the traffic to
* this staging branch.
*
*
CAUTION: If you have live predict/search traffic, switching the default branch could
* potentially cause outages if the ID space of the new branch is very different from the old one.
*
*
More specifically:
*
*
* - PredictionService will only return product IDs from branch {newBranch}.
*
- SearchService will only return product IDs from branch {newBranch} (if branch is not
* explicitly set).
*
- UserEventService will only join events with products from branch {newBranch}.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* SetDefaultBranchRequest request =
* SetDefaultBranchRequest.newBuilder()
* .setCatalog(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setBranchId(
* BranchName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[BRANCH]").toString())
* .setNote("note3387378")
* .setForce(true)
* .build();
* catalogServiceClient.setDefaultBranch(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void setDefaultBranch(SetDefaultBranchRequest request) {
setDefaultBranchCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Set a specified branch id as default branch. API methods such as
* [SearchService.Search][google.cloud.retail.v2.SearchService.Search],
* [ProductService.GetProduct][google.cloud.retail.v2.ProductService.GetProduct],
* [ProductService.ListProducts][google.cloud.retail.v2.ProductService.ListProducts] will treat
* requests using "default_branch" to the actual branch id set as default.
*
* For example, if `projects/*/locations/*/catalogs/*/branches/1` is set as
* default, setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to
* `projects/*/locations/*/catalogs/*/branches/default_branch` is equivalent to
* setting [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to
* `projects/*/locations/*/catalogs/*/branches/1`.
*
*
Using multiple branches can be useful when developers would like to have a staging branch to
* test and verify for future usage. When it becomes ready, developers switch on the staging
* branch using this API while keeping using
* `projects/*/locations/*/catalogs/*/branches/default_branch` as
* [SearchRequest.branch][google.cloud.retail.v2.SearchRequest.branch] to route the traffic to
* this staging branch.
*
*
CAUTION: If you have live predict/search traffic, switching the default branch could
* potentially cause outages if the ID space of the new branch is very different from the old one.
*
*
More specifically:
*
*
* - PredictionService will only return product IDs from branch {newBranch}.
*
- SearchService will only return product IDs from branch {newBranch} (if branch is not
* explicitly set).
*
- UserEventService will only join events with products from branch {newBranch}.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* SetDefaultBranchRequest request =
* SetDefaultBranchRequest.newBuilder()
* .setCatalog(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setBranchId(
* BranchName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[BRANCH]").toString())
* .setNote("note3387378")
* .setForce(true)
* .build();
* ApiFuture future = catalogServiceClient.setDefaultBranchCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable setDefaultBranchCallable() {
return stub.setDefaultBranchCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get which branch is currently default branch set by
* [CatalogService.SetDefaultBranch][google.cloud.retail.v2.CatalogService.SetDefaultBranch]
* method under a specified parent catalog.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* CatalogName catalog = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* GetDefaultBranchResponse response = catalogServiceClient.getDefaultBranch(catalog);
* }
* }
*
* @param catalog The parent catalog resource name, such as
* `projects/*/locations/global/catalogs/default_catalog`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetDefaultBranchResponse getDefaultBranch(CatalogName catalog) {
GetDefaultBranchRequest request =
GetDefaultBranchRequest.newBuilder()
.setCatalog(catalog == null ? null : catalog.toString())
.build();
return getDefaultBranch(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get which branch is currently default branch set by
* [CatalogService.SetDefaultBranch][google.cloud.retail.v2.CatalogService.SetDefaultBranch]
* method under a specified parent catalog.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* String catalog = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString();
* GetDefaultBranchResponse response = catalogServiceClient.getDefaultBranch(catalog);
* }
* }
*
* @param catalog The parent catalog resource name, such as
* `projects/*/locations/global/catalogs/default_catalog`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetDefaultBranchResponse getDefaultBranch(String catalog) {
GetDefaultBranchRequest request =
GetDefaultBranchRequest.newBuilder().setCatalog(catalog).build();
return getDefaultBranch(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get which branch is currently default branch set by
* [CatalogService.SetDefaultBranch][google.cloud.retail.v2.CatalogService.SetDefaultBranch]
* method under a specified parent catalog.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* GetDefaultBranchRequest request =
* GetDefaultBranchRequest.newBuilder()
* .setCatalog(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .build();
* GetDefaultBranchResponse response = catalogServiceClient.getDefaultBranch(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetDefaultBranchResponse getDefaultBranch(GetDefaultBranchRequest request) {
return getDefaultBranchCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Get which branch is currently default branch set by
* [CatalogService.SetDefaultBranch][google.cloud.retail.v2.CatalogService.SetDefaultBranch]
* method under a specified parent catalog.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* GetDefaultBranchRequest request =
* GetDefaultBranchRequest.newBuilder()
* .setCatalog(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .build();
* ApiFuture future =
* catalogServiceClient.getDefaultBranchCallable().futureCall(request);
* // Do something.
* GetDefaultBranchResponse response = future.get();
* }
* }
*/
public final UnaryCallable
getDefaultBranchCallable() {
return stub.getDefaultBranchCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [CompletionConfig][google.cloud.retail.v2.CompletionConfig].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* CompletionConfigName name = CompletionConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* CompletionConfig response = catalogServiceClient.getCompletionConfig(name);
* }
* }
*
* @param name Required. Full CompletionConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/completionConfig`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CompletionConfig getCompletionConfig(CompletionConfigName name) {
GetCompletionConfigRequest request =
GetCompletionConfigRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getCompletionConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [CompletionConfig][google.cloud.retail.v2.CompletionConfig].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* String name = CompletionConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString();
* CompletionConfig response = catalogServiceClient.getCompletionConfig(name);
* }
* }
*
* @param name Required. Full CompletionConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/completionConfig`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CompletionConfig getCompletionConfig(String name) {
GetCompletionConfigRequest request =
GetCompletionConfigRequest.newBuilder().setName(name).build();
return getCompletionConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [CompletionConfig][google.cloud.retail.v2.CompletionConfig].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* GetCompletionConfigRequest request =
* GetCompletionConfigRequest.newBuilder()
* .setName(CompletionConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .build();
* CompletionConfig response = catalogServiceClient.getCompletionConfig(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CompletionConfig getCompletionConfig(GetCompletionConfigRequest request) {
return getCompletionConfigCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [CompletionConfig][google.cloud.retail.v2.CompletionConfig].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* GetCompletionConfigRequest request =
* GetCompletionConfigRequest.newBuilder()
* .setName(CompletionConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .build();
* ApiFuture future =
* catalogServiceClient.getCompletionConfigCallable().futureCall(request);
* // Do something.
* CompletionConfig response = future.get();
* }
* }
*/
public final UnaryCallable
getCompletionConfigCallable() {
return stub.getCompletionConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the [CompletionConfig][google.cloud.retail.v2.CompletionConfig]s.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* CompletionConfig completionConfig = CompletionConfig.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* CompletionConfig response =
* catalogServiceClient.updateCompletionConfig(completionConfig, updateMask);
* }
* }
*
* @param completionConfig Required. The
* [CompletionConfig][google.cloud.retail.v2.CompletionConfig] to update.
* If the caller does not have permission to update the
* [CompletionConfig][google.cloud.retail.v2.CompletionConfig], then a PERMISSION_DENIED error
* is returned.
*
If the [CompletionConfig][google.cloud.retail.v2.CompletionConfig] to update does not
* exist, a NOT_FOUND error is returned.
* @param updateMask Indicates which fields in the provided
* [CompletionConfig][google.cloud.retail.v2.CompletionConfig] to update. The following are
* the only supported fields:
*
* - [CompletionConfig.matching_order][google.cloud.retail.v2.CompletionConfig.matching_order]
*
- [CompletionConfig.max_suggestions][google.cloud.retail.v2.CompletionConfig.max_suggestions]
*
- [CompletionConfig.min_prefix_length][google.cloud.retail.v2.CompletionConfig.min_prefix_length]
*
- [CompletionConfig.auto_learning][google.cloud.retail.v2.CompletionConfig.auto_learning]
*
* If not set, all supported fields are updated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CompletionConfig updateCompletionConfig(
CompletionConfig completionConfig, FieldMask updateMask) {
UpdateCompletionConfigRequest request =
UpdateCompletionConfigRequest.newBuilder()
.setCompletionConfig(completionConfig)
.setUpdateMask(updateMask)
.build();
return updateCompletionConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the [CompletionConfig][google.cloud.retail.v2.CompletionConfig]s.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* UpdateCompletionConfigRequest request =
* UpdateCompletionConfigRequest.newBuilder()
* .setCompletionConfig(CompletionConfig.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* CompletionConfig response = catalogServiceClient.updateCompletionConfig(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final CompletionConfig updateCompletionConfig(UpdateCompletionConfigRequest request) {
return updateCompletionConfigCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the [CompletionConfig][google.cloud.retail.v2.CompletionConfig]s.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* UpdateCompletionConfigRequest request =
* UpdateCompletionConfigRequest.newBuilder()
* .setCompletionConfig(CompletionConfig.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* catalogServiceClient.updateCompletionConfigCallable().futureCall(request);
* // Do something.
* CompletionConfig response = future.get();
* }
* }
*/
public final UnaryCallable
updateCompletionConfigCallable() {
return stub.updateCompletionConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* AttributesConfigName name = AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* AttributesConfig response = catalogServiceClient.getAttributesConfig(name);
* }
* }
*
* @param name Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AttributesConfig getAttributesConfig(AttributesConfigName name) {
GetAttributesConfigRequest request =
GetAttributesConfigRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getAttributesConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* String name = AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString();
* AttributesConfig response = catalogServiceClient.getAttributesConfig(name);
* }
* }
*
* @param name Required. Full AttributesConfig resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/attributesConfig`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AttributesConfig getAttributesConfig(String name) {
GetAttributesConfigRequest request =
GetAttributesConfigRequest.newBuilder().setName(name).build();
return getAttributesConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* GetAttributesConfigRequest request =
* GetAttributesConfigRequest.newBuilder()
* .setName(AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .build();
* AttributesConfig response = catalogServiceClient.getAttributesConfig(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AttributesConfig getAttributesConfig(GetAttributesConfigRequest request) {
return getAttributesConfigCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* GetAttributesConfigRequest request =
* GetAttributesConfigRequest.newBuilder()
* .setName(AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .build();
* ApiFuture future =
* catalogServiceClient.getAttributesConfigCallable().futureCall(request);
* // Do something.
* AttributesConfig response = future.get();
* }
* }
*/
public final UnaryCallable
getAttributesConfigCallable() {
return stub.getAttributesConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* The catalog attributes in the request will be updated in the catalog, or inserted if they do
* not exist. Existing catalog attributes not included in the request will remain unchanged.
* Attributes that are assigned to products, but do not exist at the catalog level, are always
* included in the response. The product attribute is assigned default values for missing catalog
* attribute fields, e.g., searchable and dynamic facetable options.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* AttributesConfig attributesConfig = AttributesConfig.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* AttributesConfig response =
* catalogServiceClient.updateAttributesConfig(attributesConfig, updateMask);
* }
* }
*
* @param attributesConfig Required. The
* [AttributesConfig][google.cloud.retail.v2.AttributesConfig] to update.
* @param updateMask Indicates which fields in the provided
* [AttributesConfig][google.cloud.retail.v2.AttributesConfig] to update. The following is the
* only supported field:
*
* - [AttributesConfig.catalog_attributes][google.cloud.retail.v2.AttributesConfig.catalog_attributes]
*
* If not set, all supported fields are updated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AttributesConfig updateAttributesConfig(
AttributesConfig attributesConfig, FieldMask updateMask) {
UpdateAttributesConfigRequest request =
UpdateAttributesConfigRequest.newBuilder()
.setAttributesConfig(attributesConfig)
.setUpdateMask(updateMask)
.build();
return updateAttributesConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
*
The catalog attributes in the request will be updated in the catalog, or inserted if they do
* not exist. Existing catalog attributes not included in the request will remain unchanged.
* Attributes that are assigned to products, but do not exist at the catalog level, are always
* included in the response. The product attribute is assigned default values for missing catalog
* attribute fields, e.g., searchable and dynamic facetable options.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* UpdateAttributesConfigRequest request =
* UpdateAttributesConfigRequest.newBuilder()
* .setAttributesConfig(AttributesConfig.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* AttributesConfig response = catalogServiceClient.updateAttributesConfig(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AttributesConfig updateAttributesConfig(UpdateAttributesConfigRequest request) {
return updateAttributesConfigCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* The catalog attributes in the request will be updated in the catalog, or inserted if they do
* not exist. Existing catalog attributes not included in the request will remain unchanged.
* Attributes that are assigned to products, but do not exist at the catalog level, are always
* included in the response. The product attribute is assigned default values for missing catalog
* attribute fields, e.g., searchable and dynamic facetable options.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* UpdateAttributesConfigRequest request =
* UpdateAttributesConfigRequest.newBuilder()
* .setAttributesConfig(AttributesConfig.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* catalogServiceClient.updateAttributesConfigCallable().futureCall(request);
* // Do something.
* AttributesConfig response = future.get();
* }
* }
*/
public final UnaryCallable
updateAttributesConfigCallable() {
return stub.updateAttributesConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Adds the specified [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to the
* [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* If the [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to add already exists, an
* ALREADY_EXISTS error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* AddCatalogAttributeRequest request =
* AddCatalogAttributeRequest.newBuilder()
* .setAttributesConfig(
* AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setCatalogAttribute(CatalogAttribute.newBuilder().build())
* .build();
* AttributesConfig response = catalogServiceClient.addCatalogAttribute(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AttributesConfig addCatalogAttribute(AddCatalogAttributeRequest request) {
return addCatalogAttributeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Adds the specified [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to the
* [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* If the [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to add already exists, an
* ALREADY_EXISTS error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* AddCatalogAttributeRequest request =
* AddCatalogAttributeRequest.newBuilder()
* .setAttributesConfig(
* AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setCatalogAttribute(CatalogAttribute.newBuilder().build())
* .build();
* ApiFuture future =
* catalogServiceClient.addCatalogAttributeCallable().futureCall(request);
* // Do something.
* AttributesConfig response = future.get();
* }
* }
*/
public final UnaryCallable
addCatalogAttributeCallable() {
return stub.addCatalogAttributeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Removes the specified [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] from the
* [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* If the [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to remove does not exist,
* a NOT_FOUND error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* RemoveCatalogAttributeRequest request =
* RemoveCatalogAttributeRequest.newBuilder()
* .setAttributesConfig(
* AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setKey("key106079")
* .build();
* AttributesConfig response = catalogServiceClient.removeCatalogAttribute(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AttributesConfig removeCatalogAttribute(RemoveCatalogAttributeRequest request) {
return removeCatalogAttributeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Removes the specified [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] from the
* [AttributesConfig][google.cloud.retail.v2.AttributesConfig].
*
* If the [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to remove does not exist,
* a NOT_FOUND error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* RemoveCatalogAttributeRequest request =
* RemoveCatalogAttributeRequest.newBuilder()
* .setAttributesConfig(
* AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setKey("key106079")
* .build();
* ApiFuture future =
* catalogServiceClient.removeCatalogAttributeCallable().futureCall(request);
* // Do something.
* AttributesConfig response = future.get();
* }
* }
*/
public final UnaryCallable
removeCatalogAttributeCallable() {
return stub.removeCatalogAttributeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Replaces the specified [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] in the
* [AttributesConfig][google.cloud.retail.v2.AttributesConfig] by updating the catalog attribute
* with the same [CatalogAttribute.key][google.cloud.retail.v2.CatalogAttribute.key].
*
* If the [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to replace does not
* exist, a NOT_FOUND error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* ReplaceCatalogAttributeRequest request =
* ReplaceCatalogAttributeRequest.newBuilder()
* .setAttributesConfig(
* AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setCatalogAttribute(CatalogAttribute.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* AttributesConfig response = catalogServiceClient.replaceCatalogAttribute(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AttributesConfig replaceCatalogAttribute(ReplaceCatalogAttributeRequest request) {
return replaceCatalogAttributeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Replaces the specified [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] in the
* [AttributesConfig][google.cloud.retail.v2.AttributesConfig] by updating the catalog attribute
* with the same [CatalogAttribute.key][google.cloud.retail.v2.CatalogAttribute.key].
*
* If the [CatalogAttribute][google.cloud.retail.v2.CatalogAttribute] to replace does not
* exist, a NOT_FOUND error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (CatalogServiceClient catalogServiceClient = CatalogServiceClient.create()) {
* ReplaceCatalogAttributeRequest request =
* ReplaceCatalogAttributeRequest.newBuilder()
* .setAttributesConfig(
* AttributesConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setCatalogAttribute(CatalogAttribute.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* catalogServiceClient.replaceCatalogAttributeCallable().futureCall(request);
* // Do something.
* AttributesConfig response = future.get();
* }
* }
*/
public final UnaryCallable
replaceCatalogAttributeCallable() {
return stub.replaceCatalogAttributeCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListCatalogsPagedResponse
extends AbstractPagedListResponse<
ListCatalogsRequest,
ListCatalogsResponse,
Catalog,
ListCatalogsPage,
ListCatalogsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListCatalogsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListCatalogsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListCatalogsPagedResponse(ListCatalogsPage page) {
super(page, ListCatalogsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListCatalogsPage
extends AbstractPage {
private ListCatalogsPage(
PageContext context,
ListCatalogsResponse response) {
super(context, response);
}
private static ListCatalogsPage createEmptyPage() {
return new ListCatalogsPage(null, null);
}
@Override
protected ListCatalogsPage createPage(
PageContext context,
ListCatalogsResponse response) {
return new ListCatalogsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListCatalogsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListCatalogsRequest,
ListCatalogsResponse,
Catalog,
ListCatalogsPage,
ListCatalogsFixedSizeCollection> {
private ListCatalogsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListCatalogsFixedSizeCollection createEmptyCollection() {
return new ListCatalogsFixedSizeCollection(null, 0);
}
@Override
protected ListCatalogsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListCatalogsFixedSizeCollection(pages, collectionSize);
}
}
}