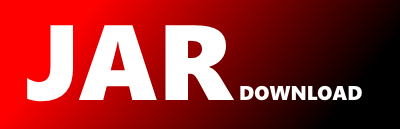
com.google.cloud.retail.v2.ModelServiceClient Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.retail.v2;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.retail.v2.stub.ModelServiceStub;
import com.google.cloud.retail.v2.stub.ModelServiceStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Service for performing CRUD operations on models. Recommendation models
* contain all the metadata necessary to generate a set of models for the `Predict()` API. A model
* is queried indirectly via a ServingConfig, which associates a model with a given Placement (e.g.
* Frequently Bought Together on Home Page).
*
* This service allows you to do the following:
*
*
* - Initiate training of a model.
*
- Pause training of an existing model.
*
- List all the available models along with their metadata.
*
- Control their tuning schedule.
*
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ModelName name = ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]");
* Model response = modelServiceClient.getModel(name);
* }
* }
*
* Note: close() needs to be called on the ModelServiceClient object to clean up resources such
* as threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* CreateModel
* Creates a new model.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createModelAsync(CreateModelRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createModelAsync(CatalogName parent, Model model)
*
createModelAsync(String parent, Model model)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createModelOperationCallable()
*
createModelCallable()
*
*
*
*
* GetModel
* Gets a model.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getModel(GetModelRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getModel(ModelName name)
*
getModel(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getModelCallable()
*
*
*
*
* PauseModel
* Pauses the training of an existing model.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* pauseModel(PauseModelRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* pauseModel(ModelName name)
*
pauseModel(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* pauseModelCallable()
*
*
*
*
* ResumeModel
* Resumes the training of an existing model.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* resumeModel(ResumeModelRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* resumeModel(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* resumeModelCallable()
*
*
*
*
* DeleteModel
* Deletes an existing model.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteModel(DeleteModelRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteModel(ModelName name)
*
deleteModel(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteModelCallable()
*
*
*
*
* ListModels
* Lists all the models linked to this event store.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listModels(ListModelsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listModels(CatalogName parent)
*
listModels(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listModelsPagedCallable()
*
listModelsCallable()
*
*
*
*
* UpdateModel
* Update of model metadata. Only fields that currently can be updated are: `filtering_option` and `periodic_tuning_state`. If other values are provided, this API method ignores them.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateModel(UpdateModelRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateModel(Model model, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateModelCallable()
*
*
*
*
* TuneModel
* Tunes an existing model.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* tuneModelAsync(TuneModelRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* tuneModelAsync(ModelName name)
*
tuneModelAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* tuneModelOperationCallable()
*
tuneModelCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of ModelServiceSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ModelServiceSettings modelServiceSettings =
* ModelServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* ModelServiceClient modelServiceClient = ModelServiceClient.create(modelServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ModelServiceSettings modelServiceSettings =
* ModelServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* ModelServiceClient modelServiceClient = ModelServiceClient.create(modelServiceSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ModelServiceSettings modelServiceSettings = ModelServiceSettings.newHttpJsonBuilder().build();
* ModelServiceClient modelServiceClient = ModelServiceClient.create(modelServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class ModelServiceClient implements BackgroundResource {
private final ModelServiceSettings settings;
private final ModelServiceStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of ModelServiceClient with default settings. */
public static final ModelServiceClient create() throws IOException {
return create(ModelServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of ModelServiceClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final ModelServiceClient create(ModelServiceSettings settings) throws IOException {
return new ModelServiceClient(settings);
}
/**
* Constructs an instance of ModelServiceClient, using the given stub for making calls. This is
* for advanced usage - prefer using create(ModelServiceSettings).
*/
public static final ModelServiceClient create(ModelServiceStub stub) {
return new ModelServiceClient(stub);
}
/**
* Constructs an instance of ModelServiceClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected ModelServiceClient(ModelServiceSettings settings) throws IOException {
this.settings = settings;
this.stub = ((ModelServiceStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected ModelServiceClient(ModelServiceStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final ModelServiceSettings getSettings() {
return settings;
}
public ModelServiceStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new model.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* CatalogName parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* Model model = Model.newBuilder().build();
* Model response = modelServiceClient.createModelAsync(parent, model).get();
* }
* }
*
* @param parent Required. The parent resource under which to create the model. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param model Required. The payload of the [Model][google.cloud.retail.v2.Model] to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createModelAsync(
CatalogName parent, Model model) {
CreateModelRequest request =
CreateModelRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setModel(model)
.build();
return createModelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* String parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString();
* Model model = Model.newBuilder().build();
* Model response = modelServiceClient.createModelAsync(parent, model).get();
* }
* }
*
* @param parent Required. The parent resource under which to create the model. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param model Required. The payload of the [Model][google.cloud.retail.v2.Model] to create.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createModelAsync(
String parent, Model model) {
CreateModelRequest request =
CreateModelRequest.newBuilder().setParent(parent).setModel(model).build();
return createModelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* CreateModelRequest request =
* CreateModelRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setModel(Model.newBuilder().build())
* .setDryRun(true)
* .build();
* Model response = modelServiceClient.createModelAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createModelAsync(
CreateModelRequest request) {
return createModelOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* CreateModelRequest request =
* CreateModelRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setModel(Model.newBuilder().build())
* .setDryRun(true)
* .build();
* OperationFuture future =
* modelServiceClient.createModelOperationCallable().futureCall(request);
* // Do something.
* Model response = future.get();
* }
* }
*/
public final OperationCallable
createModelOperationCallable() {
return stub.createModelOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* CreateModelRequest request =
* CreateModelRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setModel(Model.newBuilder().build())
* .setDryRun(true)
* .build();
* ApiFuture future = modelServiceClient.createModelCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createModelCallable() {
return stub.createModelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ModelName name = ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]");
* Model response = modelServiceClient.getModel(name);
* }
* }
*
* @param name Required. The resource name of the [Model][google.cloud.retail.v2.Model] to get.
* Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog}/models/{model_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model getModel(ModelName name) {
GetModelRequest request =
GetModelRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getModel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* String name = ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString();
* Model response = modelServiceClient.getModel(name);
* }
* }
*
* @param name Required. The resource name of the [Model][google.cloud.retail.v2.Model] to get.
* Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog}/models/{model_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model getModel(String name) {
GetModelRequest request = GetModelRequest.newBuilder().setName(name).build();
return getModel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* GetModelRequest request =
* GetModelRequest.newBuilder()
* .setName(ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString())
* .build();
* Model response = modelServiceClient.getModel(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model getModel(GetModelRequest request) {
return getModelCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* GetModelRequest request =
* GetModelRequest.newBuilder()
* .setName(ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString())
* .build();
* ApiFuture future = modelServiceClient.getModelCallable().futureCall(request);
* // Do something.
* Model response = future.get();
* }
* }
*/
public final UnaryCallable getModelCallable() {
return stub.getModelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Pauses the training of an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ModelName name = ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]");
* Model response = modelServiceClient.pauseModel(name);
* }
* }
*
* @param name Required. The name of the model to pause. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model pauseModel(ModelName name) {
PauseModelRequest request =
PauseModelRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return pauseModel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Pauses the training of an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* String name = ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString();
* Model response = modelServiceClient.pauseModel(name);
* }
* }
*
* @param name Required. The name of the model to pause. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model pauseModel(String name) {
PauseModelRequest request = PauseModelRequest.newBuilder().setName(name).build();
return pauseModel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Pauses the training of an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* PauseModelRequest request =
* PauseModelRequest.newBuilder()
* .setName(ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString())
* .build();
* Model response = modelServiceClient.pauseModel(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model pauseModel(PauseModelRequest request) {
return pauseModelCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Pauses the training of an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* PauseModelRequest request =
* PauseModelRequest.newBuilder()
* .setName(ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString())
* .build();
* ApiFuture future = modelServiceClient.pauseModelCallable().futureCall(request);
* // Do something.
* Model response = future.get();
* }
* }
*/
public final UnaryCallable pauseModelCallable() {
return stub.pauseModelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resumes the training of an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* String name = "name3373707";
* Model response = modelServiceClient.resumeModel(name);
* }
* }
*
* @param name Required. The name of the model to resume. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model resumeModel(String name) {
ResumeModelRequest request = ResumeModelRequest.newBuilder().setName(name).build();
return resumeModel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resumes the training of an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ResumeModelRequest request = ResumeModelRequest.newBuilder().setName("name3373707").build();
* Model response = modelServiceClient.resumeModel(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model resumeModel(ResumeModelRequest request) {
return resumeModelCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resumes the training of an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ResumeModelRequest request = ResumeModelRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = modelServiceClient.resumeModelCallable().futureCall(request);
* // Do something.
* Model response = future.get();
* }
* }
*/
public final UnaryCallable resumeModelCallable() {
return stub.resumeModelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ModelName name = ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]");
* modelServiceClient.deleteModel(name);
* }
* }
*
* @param name Required. The resource name of the [Model][google.cloud.retail.v2.Model] to delete.
* Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteModel(ModelName name) {
DeleteModelRequest request =
DeleteModelRequest.newBuilder().setName(name == null ? null : name.toString()).build();
deleteModel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* String name = ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString();
* modelServiceClient.deleteModel(name);
* }
* }
*
* @param name Required. The resource name of the [Model][google.cloud.retail.v2.Model] to delete.
* Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteModel(String name) {
DeleteModelRequest request = DeleteModelRequest.newBuilder().setName(name).build();
deleteModel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* DeleteModelRequest request =
* DeleteModelRequest.newBuilder()
* .setName(ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString())
* .build();
* modelServiceClient.deleteModel(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteModel(DeleteModelRequest request) {
deleteModelCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* DeleteModelRequest request =
* DeleteModelRequest.newBuilder()
* .setName(ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString())
* .build();
* ApiFuture future = modelServiceClient.deleteModelCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteModelCallable() {
return stub.deleteModelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the models linked to this event store.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* CatalogName parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* for (Model element : modelServiceClient.listModels(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent for which to list models. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListModelsPagedResponse listModels(CatalogName parent) {
ListModelsRequest request =
ListModelsRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listModels(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the models linked to this event store.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* String parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString();
* for (Model element : modelServiceClient.listModels(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent for which to list models. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListModelsPagedResponse listModels(String parent) {
ListModelsRequest request = ListModelsRequest.newBuilder().setParent(parent).build();
return listModels(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the models linked to this event store.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ListModelsRequest request =
* ListModelsRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Model element : modelServiceClient.listModels(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListModelsPagedResponse listModels(ListModelsRequest request) {
return listModelsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the models linked to this event store.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ListModelsRequest request =
* ListModelsRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future = modelServiceClient.listModelsPagedCallable().futureCall(request);
* // Do something.
* for (Model element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listModelsPagedCallable() {
return stub.listModelsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all the models linked to this event store.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ListModelsRequest request =
* ListModelsRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListModelsResponse response = modelServiceClient.listModelsCallable().call(request);
* for (Model element : response.getModelsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listModelsCallable() {
return stub.listModelsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update of model metadata. Only fields that currently can be updated are: `filtering_option` and
* `periodic_tuning_state`. If other values are provided, this API method ignores them.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* Model model = Model.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Model response = modelServiceClient.updateModel(model, updateMask);
* }
* }
*
* @param model Required. The body of the updated [Model][google.cloud.retail.v2.Model].
* @param updateMask Optional. Indicates which fields in the provided 'model' to update. If not
* set, by default updates all fields.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model updateModel(Model model, FieldMask updateMask) {
UpdateModelRequest request =
UpdateModelRequest.newBuilder().setModel(model).setUpdateMask(updateMask).build();
return updateModel(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update of model metadata. Only fields that currently can be updated are: `filtering_option` and
* `periodic_tuning_state`. If other values are provided, this API method ignores them.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* UpdateModelRequest request =
* UpdateModelRequest.newBuilder()
* .setModel(Model.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Model response = modelServiceClient.updateModel(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Model updateModel(UpdateModelRequest request) {
return updateModelCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Update of model metadata. Only fields that currently can be updated are: `filtering_option` and
* `periodic_tuning_state`. If other values are provided, this API method ignores them.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* UpdateModelRequest request =
* UpdateModelRequest.newBuilder()
* .setModel(Model.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = modelServiceClient.updateModelCallable().futureCall(request);
* // Do something.
* Model response = future.get();
* }
* }
*/
public final UnaryCallable updateModelCallable() {
return stub.updateModelCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tunes an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* ModelName name = ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]");
* TuneModelResponse response = modelServiceClient.tuneModelAsync(name).get();
* }
* }
*
* @param name Required. The resource name of the model to tune. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture tuneModelAsync(
ModelName name) {
TuneModelRequest request =
TuneModelRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return tuneModelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tunes an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* String name = ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString();
* TuneModelResponse response = modelServiceClient.tuneModelAsync(name).get();
* }
* }
*
* @param name Required. The resource name of the model to tune. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/models/{model_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture tuneModelAsync(String name) {
TuneModelRequest request = TuneModelRequest.newBuilder().setName(name).build();
return tuneModelAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tunes an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* TuneModelRequest request =
* TuneModelRequest.newBuilder()
* .setName(ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString())
* .build();
* TuneModelResponse response = modelServiceClient.tuneModelAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture tuneModelAsync(
TuneModelRequest request) {
return tuneModelOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tunes an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* TuneModelRequest request =
* TuneModelRequest.newBuilder()
* .setName(ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString())
* .build();
* OperationFuture future =
* modelServiceClient.tuneModelOperationCallable().futureCall(request);
* // Do something.
* TuneModelResponse response = future.get();
* }
* }
*/
public final OperationCallable
tuneModelOperationCallable() {
return stub.tuneModelOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tunes an existing model.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ModelServiceClient modelServiceClient = ModelServiceClient.create()) {
* TuneModelRequest request =
* TuneModelRequest.newBuilder()
* .setName(ModelName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[MODEL]").toString())
* .build();
* ApiFuture future = modelServiceClient.tuneModelCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable tuneModelCallable() {
return stub.tuneModelCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListModelsPagedResponse
extends AbstractPagedListResponse<
ListModelsRequest,
ListModelsResponse,
Model,
ListModelsPage,
ListModelsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListModelsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListModelsPagedResponse(input), MoreExecutors.directExecutor());
}
private ListModelsPagedResponse(ListModelsPage page) {
super(page, ListModelsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListModelsPage
extends AbstractPage {
private ListModelsPage(
PageContext context,
ListModelsResponse response) {
super(context, response);
}
private static ListModelsPage createEmptyPage() {
return new ListModelsPage(null, null);
}
@Override
protected ListModelsPage createPage(
PageContext context,
ListModelsResponse response) {
return new ListModelsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListModelsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListModelsRequest,
ListModelsResponse,
Model,
ListModelsPage,
ListModelsFixedSizeCollection> {
private ListModelsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListModelsFixedSizeCollection createEmptyCollection() {
return new ListModelsFixedSizeCollection(null, 0);
}
@Override
protected ListModelsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListModelsFixedSizeCollection(pages, collectionSize);
}
}
}