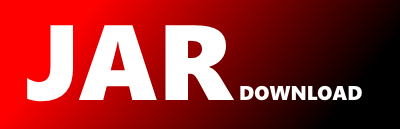
com.google.cloud.retail.v2.ServingConfigServiceClient Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.retail.v2;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.retail.v2.stub.ServingConfigServiceStub;
import com.google.cloud.retail.v2.stub.ServingConfigServiceStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Service for modifying ServingConfig.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* CatalogName parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* ServingConfig servingConfig = ServingConfig.newBuilder().build();
* String servingConfigId = "servingConfigId-831052759";
* ServingConfig response =
* servingConfigServiceClient.createServingConfig(parent, servingConfig, servingConfigId);
* }
* }
*
* Note: close() needs to be called on the ServingConfigServiceClient object to clean up
* resources such as threads. In the example above, try-with-resources is used, which automatically
* calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* CreateServingConfig
* Creates a ServingConfig.
*
A maximum of 100 [ServingConfig][google.cloud.retail.v2.ServingConfig]s are allowed in a [Catalog][google.cloud.retail.v2.Catalog], otherwise a FAILED_PRECONDITION error is returned.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createServingConfig(CreateServingConfigRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createServingConfig(CatalogName parent, ServingConfig servingConfig, String servingConfigId)
*
createServingConfig(String parent, ServingConfig servingConfig, String servingConfigId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createServingConfigCallable()
*
*
*
*
* DeleteServingConfig
* Deletes a ServingConfig.
*
Returns a NotFound error if the ServingConfig does not exist.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteServingConfig(DeleteServingConfigRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteServingConfig(ServingConfigName name)
*
deleteServingConfig(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteServingConfigCallable()
*
*
*
*
* UpdateServingConfig
* Updates a ServingConfig.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateServingConfig(UpdateServingConfigRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateServingConfig(ServingConfig servingConfig, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateServingConfigCallable()
*
*
*
*
* GetServingConfig
* Gets a ServingConfig.
*
Returns a NotFound error if the ServingConfig does not exist.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getServingConfig(GetServingConfigRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getServingConfig(ServingConfigName name)
*
getServingConfig(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getServingConfigCallable()
*
*
*
*
* ListServingConfigs
* Lists all ServingConfigs linked to this catalog.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listServingConfigs(ListServingConfigsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listServingConfigs(CatalogName parent)
*
listServingConfigs(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listServingConfigsPagedCallable()
*
listServingConfigsCallable()
*
*
*
*
* AddControl
* Enables a Control on the specified ServingConfig. The control is added in the last position of the list of controls it belongs to (e.g. if it's a facet spec control it will be applied in the last position of servingConfig.facetSpecIds) Returns a ALREADY_EXISTS error if the control has already been applied. Returns a FAILED_PRECONDITION error if the addition could exceed maximum number of control allowed for that type of control.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* addControl(AddControlRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* addControl(ServingConfigName servingConfig)
*
addControl(String servingConfig)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* addControlCallable()
*
*
*
*
* RemoveControl
* Disables a Control on the specified ServingConfig. The control is removed from the ServingConfig. Returns a NOT_FOUND error if the Control is not enabled for the ServingConfig.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* removeControl(RemoveControlRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* removeControl(ServingConfigName servingConfig)
*
removeControl(String servingConfig)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* removeControlCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of ServingConfigServiceSettings
* to create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ServingConfigServiceSettings servingConfigServiceSettings =
* ServingConfigServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create(servingConfigServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ServingConfigServiceSettings servingConfigServiceSettings =
* ServingConfigServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create(servingConfigServiceSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* ServingConfigServiceSettings servingConfigServiceSettings =
* ServingConfigServiceSettings.newHttpJsonBuilder().build();
* ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create(servingConfigServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class ServingConfigServiceClient implements BackgroundResource {
private final ServingConfigServiceSettings settings;
private final ServingConfigServiceStub stub;
/** Constructs an instance of ServingConfigServiceClient with default settings. */
public static final ServingConfigServiceClient create() throws IOException {
return create(ServingConfigServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of ServingConfigServiceClient, using the given settings. The channels
* are created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final ServingConfigServiceClient create(ServingConfigServiceSettings settings)
throws IOException {
return new ServingConfigServiceClient(settings);
}
/**
* Constructs an instance of ServingConfigServiceClient, using the given stub for making calls.
* This is for advanced usage - prefer using create(ServingConfigServiceSettings).
*/
public static final ServingConfigServiceClient create(ServingConfigServiceStub stub) {
return new ServingConfigServiceClient(stub);
}
/**
* Constructs an instance of ServingConfigServiceClient, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected ServingConfigServiceClient(ServingConfigServiceSettings settings) throws IOException {
this.settings = settings;
this.stub = ((ServingConfigServiceStubSettings) settings.getStubSettings()).createStub();
}
protected ServingConfigServiceClient(ServingConfigServiceStub stub) {
this.settings = null;
this.stub = stub;
}
public final ServingConfigServiceSettings getSettings() {
return settings;
}
public ServingConfigServiceStub getStub() {
return stub;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a ServingConfig.
*
*
A maximum of 100 [ServingConfig][google.cloud.retail.v2.ServingConfig]s are allowed in a
* [Catalog][google.cloud.retail.v2.Catalog], otherwise a FAILED_PRECONDITION error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* CatalogName parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* ServingConfig servingConfig = ServingConfig.newBuilder().build();
* String servingConfigId = "servingConfigId-831052759";
* ServingConfig response =
* servingConfigServiceClient.createServingConfig(parent, servingConfig, servingConfigId);
* }
* }
*
* @param parent Required. Full resource name of parent. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param servingConfig Required. The ServingConfig to create.
* @param servingConfigId Required. The ID to use for the ServingConfig, which will become the
* final component of the ServingConfig's resource name.
* This value should be 4-63 characters, and valid characters are /[a-z][0-9]-_/.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig createServingConfig(
CatalogName parent, ServingConfig servingConfig, String servingConfigId) {
CreateServingConfigRequest request =
CreateServingConfigRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setServingConfig(servingConfig)
.setServingConfigId(servingConfigId)
.build();
return createServingConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a ServingConfig.
*
*
A maximum of 100 [ServingConfig][google.cloud.retail.v2.ServingConfig]s are allowed in a
* [Catalog][google.cloud.retail.v2.Catalog], otherwise a FAILED_PRECONDITION error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* String parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString();
* ServingConfig servingConfig = ServingConfig.newBuilder().build();
* String servingConfigId = "servingConfigId-831052759";
* ServingConfig response =
* servingConfigServiceClient.createServingConfig(parent, servingConfig, servingConfigId);
* }
* }
*
* @param parent Required. Full resource name of parent. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @param servingConfig Required. The ServingConfig to create.
* @param servingConfigId Required. The ID to use for the ServingConfig, which will become the
* final component of the ServingConfig's resource name.
* This value should be 4-63 characters, and valid characters are /[a-z][0-9]-_/.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig createServingConfig(
String parent, ServingConfig servingConfig, String servingConfigId) {
CreateServingConfigRequest request =
CreateServingConfigRequest.newBuilder()
.setParent(parent)
.setServingConfig(servingConfig)
.setServingConfigId(servingConfigId)
.build();
return createServingConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a ServingConfig.
*
*
A maximum of 100 [ServingConfig][google.cloud.retail.v2.ServingConfig]s are allowed in a
* [Catalog][google.cloud.retail.v2.Catalog], otherwise a FAILED_PRECONDITION error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* CreateServingConfigRequest request =
* CreateServingConfigRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setServingConfig(ServingConfig.newBuilder().build())
* .setServingConfigId("servingConfigId-831052759")
* .build();
* ServingConfig response = servingConfigServiceClient.createServingConfig(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig createServingConfig(CreateServingConfigRequest request) {
return createServingConfigCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a ServingConfig.
*
* A maximum of 100 [ServingConfig][google.cloud.retail.v2.ServingConfig]s are allowed in a
* [Catalog][google.cloud.retail.v2.Catalog], otherwise a FAILED_PRECONDITION error is returned.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* CreateServingConfigRequest request =
* CreateServingConfigRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setServingConfig(ServingConfig.newBuilder().build())
* .setServingConfigId("servingConfigId-831052759")
* .build();
* ApiFuture future =
* servingConfigServiceClient.createServingConfigCallable().futureCall(request);
* // Do something.
* ServingConfig response = future.get();
* }
* }
*/
public final UnaryCallable
createServingConfigCallable() {
return stub.createServingConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a ServingConfig.
*
* Returns a NotFound error if the ServingConfig does not exist.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* ServingConfigName name =
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]");
* servingConfigServiceClient.deleteServingConfig(name);
* }
* }
*
* @param name Required. The resource name of the ServingConfig to delete. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteServingConfig(ServingConfigName name) {
DeleteServingConfigRequest request =
DeleteServingConfigRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
deleteServingConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a ServingConfig.
*
* Returns a NotFound error if the ServingConfig does not exist.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* String name =
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString();
* servingConfigServiceClient.deleteServingConfig(name);
* }
* }
*
* @param name Required. The resource name of the ServingConfig to delete. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteServingConfig(String name) {
DeleteServingConfigRequest request =
DeleteServingConfigRequest.newBuilder().setName(name).build();
deleteServingConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a ServingConfig.
*
* Returns a NotFound error if the ServingConfig does not exist.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* DeleteServingConfigRequest request =
* DeleteServingConfigRequest.newBuilder()
* .setName(
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString())
* .build();
* servingConfigServiceClient.deleteServingConfig(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteServingConfig(DeleteServingConfigRequest request) {
deleteServingConfigCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a ServingConfig.
*
* Returns a NotFound error if the ServingConfig does not exist.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* DeleteServingConfigRequest request =
* DeleteServingConfigRequest.newBuilder()
* .setName(
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString())
* .build();
* ApiFuture future =
* servingConfigServiceClient.deleteServingConfigCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteServingConfigCallable() {
return stub.deleteServingConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a ServingConfig.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* ServingConfig servingConfig = ServingConfig.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* ServingConfig response =
* servingConfigServiceClient.updateServingConfig(servingConfig, updateMask);
* }
* }
*
* @param servingConfig Required. The ServingConfig to update.
* @param updateMask Indicates which fields in the provided
* [ServingConfig][google.cloud.retail.v2.ServingConfig] to update. The following are NOT
* supported:
*
* - [ServingConfig.name][google.cloud.retail.v2.ServingConfig.name]
*
* If not set, all supported fields are updated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig updateServingConfig(
ServingConfig servingConfig, FieldMask updateMask) {
UpdateServingConfigRequest request =
UpdateServingConfigRequest.newBuilder()
.setServingConfig(servingConfig)
.setUpdateMask(updateMask)
.build();
return updateServingConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a ServingConfig.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* UpdateServingConfigRequest request =
* UpdateServingConfigRequest.newBuilder()
* .setServingConfig(ServingConfig.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ServingConfig response = servingConfigServiceClient.updateServingConfig(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig updateServingConfig(UpdateServingConfigRequest request) {
return updateServingConfigCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a ServingConfig.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* UpdateServingConfigRequest request =
* UpdateServingConfigRequest.newBuilder()
* .setServingConfig(ServingConfig.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* servingConfigServiceClient.updateServingConfigCallable().futureCall(request);
* // Do something.
* ServingConfig response = future.get();
* }
* }
*/
public final UnaryCallable
updateServingConfigCallable() {
return stub.updateServingConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a ServingConfig.
*
* Returns a NotFound error if the ServingConfig does not exist.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* ServingConfigName name =
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]");
* ServingConfig response = servingConfigServiceClient.getServingConfig(name);
* }
* }
*
* @param name Required. The resource name of the ServingConfig to get. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig getServingConfig(ServingConfigName name) {
GetServingConfigRequest request =
GetServingConfigRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getServingConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a ServingConfig.
*
* Returns a NotFound error if the ServingConfig does not exist.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* String name =
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString();
* ServingConfig response = servingConfigServiceClient.getServingConfig(name);
* }
* }
*
* @param name Required. The resource name of the ServingConfig to get. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig getServingConfig(String name) {
GetServingConfigRequest request = GetServingConfigRequest.newBuilder().setName(name).build();
return getServingConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a ServingConfig.
*
* Returns a NotFound error if the ServingConfig does not exist.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* GetServingConfigRequest request =
* GetServingConfigRequest.newBuilder()
* .setName(
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString())
* .build();
* ServingConfig response = servingConfigServiceClient.getServingConfig(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig getServingConfig(GetServingConfigRequest request) {
return getServingConfigCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a ServingConfig.
*
* Returns a NotFound error if the ServingConfig does not exist.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* GetServingConfigRequest request =
* GetServingConfigRequest.newBuilder()
* .setName(
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString())
* .build();
* ApiFuture future =
* servingConfigServiceClient.getServingConfigCallable().futureCall(request);
* // Do something.
* ServingConfig response = future.get();
* }
* }
*/
public final UnaryCallable getServingConfigCallable() {
return stub.getServingConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all ServingConfigs linked to this catalog.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* CatalogName parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]");
* for (ServingConfig element :
* servingConfigServiceClient.listServingConfigs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServingConfigsPagedResponse listServingConfigs(CatalogName parent) {
ListServingConfigsRequest request =
ListServingConfigsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listServingConfigs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all ServingConfigs linked to this catalog.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* String parent = CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString();
* for (ServingConfig element :
* servingConfigServiceClient.listServingConfigs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The catalog resource name. Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServingConfigsPagedResponse listServingConfigs(String parent) {
ListServingConfigsRequest request =
ListServingConfigsRequest.newBuilder().setParent(parent).build();
return listServingConfigs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all ServingConfigs linked to this catalog.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* ListServingConfigsRequest request =
* ListServingConfigsRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (ServingConfig element :
* servingConfigServiceClient.listServingConfigs(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServingConfigsPagedResponse listServingConfigs(
ListServingConfigsRequest request) {
return listServingConfigsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all ServingConfigs linked to this catalog.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* ListServingConfigsRequest request =
* ListServingConfigsRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* servingConfigServiceClient.listServingConfigsPagedCallable().futureCall(request);
* // Do something.
* for (ServingConfig element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listServingConfigsPagedCallable() {
return stub.listServingConfigsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all ServingConfigs linked to this catalog.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* ListServingConfigsRequest request =
* ListServingConfigsRequest.newBuilder()
* .setParent(CatalogName.of("[PROJECT]", "[LOCATION]", "[CATALOG]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListServingConfigsResponse response =
* servingConfigServiceClient.listServingConfigsCallable().call(request);
* for (ServingConfig element : response.getServingConfigsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listServingConfigsCallable() {
return stub.listServingConfigsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enables a Control on the specified ServingConfig. The control is added in the last position of
* the list of controls it belongs to (e.g. if it's a facet spec control it will be applied in the
* last position of servingConfig.facetSpecIds) Returns a ALREADY_EXISTS error if the control has
* already been applied. Returns a FAILED_PRECONDITION error if the addition could exceed maximum
* number of control allowed for that type of control.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* ServingConfigName servingConfig =
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]");
* ServingConfig response = servingConfigServiceClient.addControl(servingConfig);
* }
* }
*
* @param servingConfig Required. The source ServingConfig resource name . Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig addControl(ServingConfigName servingConfig) {
AddControlRequest request =
AddControlRequest.newBuilder()
.setServingConfig(servingConfig == null ? null : servingConfig.toString())
.build();
return addControl(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enables a Control on the specified ServingConfig. The control is added in the last position of
* the list of controls it belongs to (e.g. if it's a facet spec control it will be applied in the
* last position of servingConfig.facetSpecIds) Returns a ALREADY_EXISTS error if the control has
* already been applied. Returns a FAILED_PRECONDITION error if the addition could exceed maximum
* number of control allowed for that type of control.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* String servingConfig =
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString();
* ServingConfig response = servingConfigServiceClient.addControl(servingConfig);
* }
* }
*
* @param servingConfig Required. The source ServingConfig resource name . Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig addControl(String servingConfig) {
AddControlRequest request =
AddControlRequest.newBuilder().setServingConfig(servingConfig).build();
return addControl(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enables a Control on the specified ServingConfig. The control is added in the last position of
* the list of controls it belongs to (e.g. if it's a facet spec control it will be applied in the
* last position of servingConfig.facetSpecIds) Returns a ALREADY_EXISTS error if the control has
* already been applied. Returns a FAILED_PRECONDITION error if the addition could exceed maximum
* number of control allowed for that type of control.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* AddControlRequest request =
* AddControlRequest.newBuilder()
* .setServingConfig(
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString())
* .setControlId("controlId-395080872")
* .build();
* ServingConfig response = servingConfigServiceClient.addControl(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig addControl(AddControlRequest request) {
return addControlCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enables a Control on the specified ServingConfig. The control is added in the last position of
* the list of controls it belongs to (e.g. if it's a facet spec control it will be applied in the
* last position of servingConfig.facetSpecIds) Returns a ALREADY_EXISTS error if the control has
* already been applied. Returns a FAILED_PRECONDITION error if the addition could exceed maximum
* number of control allowed for that type of control.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* AddControlRequest request =
* AddControlRequest.newBuilder()
* .setServingConfig(
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString())
* .setControlId("controlId-395080872")
* .build();
* ApiFuture future =
* servingConfigServiceClient.addControlCallable().futureCall(request);
* // Do something.
* ServingConfig response = future.get();
* }
* }
*/
public final UnaryCallable addControlCallable() {
return stub.addControlCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disables a Control on the specified ServingConfig. The control is removed from the
* ServingConfig. Returns a NOT_FOUND error if the Control is not enabled for the ServingConfig.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* ServingConfigName servingConfig =
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]");
* ServingConfig response = servingConfigServiceClient.removeControl(servingConfig);
* }
* }
*
* @param servingConfig Required. The source ServingConfig resource name . Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig removeControl(ServingConfigName servingConfig) {
RemoveControlRequest request =
RemoveControlRequest.newBuilder()
.setServingConfig(servingConfig == null ? null : servingConfig.toString())
.build();
return removeControl(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disables a Control on the specified ServingConfig. The control is removed from the
* ServingConfig. Returns a NOT_FOUND error if the Control is not enabled for the ServingConfig.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* String servingConfig =
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString();
* ServingConfig response = servingConfigServiceClient.removeControl(servingConfig);
* }
* }
*
* @param servingConfig Required. The source ServingConfig resource name . Format:
* `projects/{project_number}/locations/{location_id}/catalogs/{catalog_id}/servingConfigs/{serving_config_id}`
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig removeControl(String servingConfig) {
RemoveControlRequest request =
RemoveControlRequest.newBuilder().setServingConfig(servingConfig).build();
return removeControl(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disables a Control on the specified ServingConfig. The control is removed from the
* ServingConfig. Returns a NOT_FOUND error if the Control is not enabled for the ServingConfig.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* RemoveControlRequest request =
* RemoveControlRequest.newBuilder()
* .setServingConfig(
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString())
* .setControlId("controlId-395080872")
* .build();
* ServingConfig response = servingConfigServiceClient.removeControl(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServingConfig removeControl(RemoveControlRequest request) {
return removeControlCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disables a Control on the specified ServingConfig. The control is removed from the
* ServingConfig. Returns a NOT_FOUND error if the Control is not enabled for the ServingConfig.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (ServingConfigServiceClient servingConfigServiceClient =
* ServingConfigServiceClient.create()) {
* RemoveControlRequest request =
* RemoveControlRequest.newBuilder()
* .setServingConfig(
* ServingConfigName.of("[PROJECT]", "[LOCATION]", "[CATALOG]", "[SERVING_CONFIG]")
* .toString())
* .setControlId("controlId-395080872")
* .build();
* ApiFuture future =
* servingConfigServiceClient.removeControlCallable().futureCall(request);
* // Do something.
* ServingConfig response = future.get();
* }
* }
*/
public final UnaryCallable removeControlCallable() {
return stub.removeControlCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListServingConfigsPagedResponse
extends AbstractPagedListResponse<
ListServingConfigsRequest,
ListServingConfigsResponse,
ServingConfig,
ListServingConfigsPage,
ListServingConfigsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListServingConfigsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListServingConfigsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListServingConfigsPagedResponse(ListServingConfigsPage page) {
super(page, ListServingConfigsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListServingConfigsPage
extends AbstractPage<
ListServingConfigsRequest,
ListServingConfigsResponse,
ServingConfig,
ListServingConfigsPage> {
private ListServingConfigsPage(
PageContext context,
ListServingConfigsResponse response) {
super(context, response);
}
private static ListServingConfigsPage createEmptyPage() {
return new ListServingConfigsPage(null, null);
}
@Override
protected ListServingConfigsPage createPage(
PageContext context,
ListServingConfigsResponse response) {
return new ListServingConfigsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListServingConfigsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListServingConfigsRequest,
ListServingConfigsResponse,
ServingConfig,
ListServingConfigsPage,
ListServingConfigsFixedSizeCollection> {
private ListServingConfigsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListServingConfigsFixedSizeCollection createEmptyCollection() {
return new ListServingConfigsFixedSizeCollection(null, 0);
}
@Override
protected ListServingConfigsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListServingConfigsFixedSizeCollection(pages, collectionSize);
}
}
}