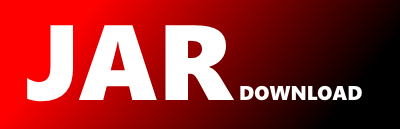
com.google.cloud.servicedirectory.v1beta1.RegistrationServiceClient Maven / Gradle / Ivy
Show all versions of google-cloud-servicedirectory Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.servicedirectory.v1beta1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.servicedirectory.v1beta1.stub.RegistrationServiceStub;
import com.google.cloud.servicedirectory.v1beta1.stub.RegistrationServiceStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Service Directory API for registering services. It defines the following
* resource model:
*
* - The API has a collection of [Namespace][google.cloud.servicedirectory.v1beta1.Namespace]
* resources, named `projects/*/locations/*/namespaces/*`.
*
*
- Each Namespace has a collection of [Service][google.cloud.servicedirectory.v1beta1.Service]
* resources, named `projects/*/locations/*/namespaces/*/services/*`.
*
*
- Each Service has a collection of [Endpoint][google.cloud.servicedirectory.v1beta1.Endpoint]
* resources, named
* `projects/*/locations/*/namespaces/*/services/*/endpoints/*`.
*
*
This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Namespace namespace = Namespace.newBuilder().build();
* String namespaceId = "namespaceId790852566";
* Namespace response =
* registrationServiceClient.createNamespace(parent, namespace, namespaceId);
* }
* }
*
* Note: close() needs to be called on the RegistrationServiceClient object to clean up resources
* such as threads. In the example above, try-with-resources is used, which automatically calls
* close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* CreateNamespace
* Creates a namespace, and returns the new namespace.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createNamespace(CreateNamespaceRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createNamespace(LocationName parent, Namespace namespace, String namespaceId)
*
createNamespace(String parent, Namespace namespace, String namespaceId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createNamespaceCallable()
*
*
*
*
* ListNamespaces
* Lists all namespaces.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listNamespaces(ListNamespacesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listNamespaces(LocationName parent)
*
listNamespaces(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listNamespacesPagedCallable()
*
listNamespacesCallable()
*
*
*
*
* GetNamespace
* Gets a namespace.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getNamespace(GetNamespaceRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getNamespace(NamespaceName name)
*
getNamespace(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getNamespaceCallable()
*
*
*
*
* UpdateNamespace
* Updates a namespace.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateNamespace(UpdateNamespaceRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateNamespace(Namespace namespace, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateNamespaceCallable()
*
*
*
*
* DeleteNamespace
* Deletes a namespace. This also deletes all services and endpoints in the namespace.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteNamespace(DeleteNamespaceRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteNamespace(NamespaceName name)
*
deleteNamespace(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteNamespaceCallable()
*
*
*
*
* CreateService
* Creates a service, and returns the new service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createService(CreateServiceRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createService(NamespaceName parent, Service service, String serviceId)
*
createService(String parent, Service service, String serviceId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createServiceCallable()
*
*
*
*
* ListServices
* Lists all services belonging to a namespace.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listServices(ListServicesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listServices(NamespaceName parent)
*
listServices(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listServicesPagedCallable()
*
listServicesCallable()
*
*
*
*
* GetService
* Gets a service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getService(GetServiceRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getService(ServiceName name)
*
getService(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getServiceCallable()
*
*
*
*
* UpdateService
* Updates a service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateService(UpdateServiceRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateService(Service service, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateServiceCallable()
*
*
*
*
* DeleteService
* Deletes a service. This also deletes all endpoints associated with the service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteService(DeleteServiceRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteService(ServiceName name)
*
deleteService(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteServiceCallable()
*
*
*
*
* CreateEndpoint
* Creates an endpoint, and returns the new endpoint.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createEndpoint(CreateEndpointRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createEndpoint(ServiceName parent, Endpoint endpoint, String endpointId)
*
createEndpoint(String parent, Endpoint endpoint, String endpointId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createEndpointCallable()
*
*
*
*
* ListEndpoints
* Lists all endpoints.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listEndpoints(ListEndpointsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listEndpoints(ServiceName parent)
*
listEndpoints(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listEndpointsPagedCallable()
*
listEndpointsCallable()
*
*
*
*
* GetEndpoint
* Gets an endpoint.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getEndpoint(GetEndpointRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getEndpoint(EndpointName name)
*
getEndpoint(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getEndpointCallable()
*
*
*
*
* UpdateEndpoint
* Updates an endpoint.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateEndpoint(UpdateEndpointRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateEndpoint(Endpoint endpoint, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateEndpointCallable()
*
*
*
*
* DeleteEndpoint
* Deletes an endpoint.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteEndpoint(DeleteEndpointRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteEndpoint(EndpointName name)
*
deleteEndpoint(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteEndpointCallable()
*
*
*
*
* GetIamPolicy
* Gets the IAM Policy for a resource
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getIamPolicy(GetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getIamPolicyCallable()
*
*
*
*
* SetIamPolicy
* Sets the IAM Policy for a resource
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* setIamPolicy(SetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* setIamPolicyCallable()
*
*
*
*
* TestIamPermissions
* Tests IAM permissions for a resource (namespace, service or service workload only).
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* testIamPermissions(TestIamPermissionsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* testIamPermissionsCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listLocationsPagedCallable()
*
listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getLocationCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of RegistrationServiceSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* RegistrationServiceSettings registrationServiceSettings =
* RegistrationServiceSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* RegistrationServiceClient registrationServiceClient =
* RegistrationServiceClient.create(registrationServiceSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* RegistrationServiceSettings registrationServiceSettings =
* RegistrationServiceSettings.newBuilder().setEndpoint(myEndpoint).build();
* RegistrationServiceClient registrationServiceClient =
* RegistrationServiceClient.create(registrationServiceSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* RegistrationServiceSettings registrationServiceSettings =
* RegistrationServiceSettings.newHttpJsonBuilder().build();
* RegistrationServiceClient registrationServiceClient =
* RegistrationServiceClient.create(registrationServiceSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class RegistrationServiceClient implements BackgroundResource {
private final RegistrationServiceSettings settings;
private final RegistrationServiceStub stub;
/** Constructs an instance of RegistrationServiceClient with default settings. */
public static final RegistrationServiceClient create() throws IOException {
return create(RegistrationServiceSettings.newBuilder().build());
}
/**
* Constructs an instance of RegistrationServiceClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final RegistrationServiceClient create(RegistrationServiceSettings settings)
throws IOException {
return new RegistrationServiceClient(settings);
}
/**
* Constructs an instance of RegistrationServiceClient, using the given stub for making calls.
* This is for advanced usage - prefer using create(RegistrationServiceSettings).
*/
public static final RegistrationServiceClient create(RegistrationServiceStub stub) {
return new RegistrationServiceClient(stub);
}
/**
* Constructs an instance of RegistrationServiceClient, using the given settings. This is
* protected so that it is easy to make a subclass, but otherwise, the static factory methods
* should be preferred.
*/
protected RegistrationServiceClient(RegistrationServiceSettings settings) throws IOException {
this.settings = settings;
this.stub = ((RegistrationServiceStubSettings) settings.getStubSettings()).createStub();
}
protected RegistrationServiceClient(RegistrationServiceStub stub) {
this.settings = null;
this.stub = stub;
}
public final RegistrationServiceSettings getSettings() {
return settings;
}
public RegistrationServiceStub getStub() {
return stub;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a namespace, and returns the new namespace.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Namespace namespace = Namespace.newBuilder().build();
* String namespaceId = "namespaceId790852566";
* Namespace response =
* registrationServiceClient.createNamespace(parent, namespace, namespaceId);
* }
* }
*
* @param parent Required. The resource name of the project and location the namespace will be
* created in.
* @param namespace Required. A namespace with initial fields set.
* @param namespaceId Required. The Resource ID must be 1-63 characters long, and comply with
* <a href="https://www.ietf.org/rfc/rfc1035.txt" target="_blank">RFC1035</a>.
* Specifically, the name must be 1-63 characters long and match the regular expression
* `[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?` which means the first character must be a lowercase
* letter, and all following characters must be a dash, lowercase letter, or digit, except the
* last character, which cannot be a dash.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Namespace createNamespace(
LocationName parent, Namespace namespace, String namespaceId) {
CreateNamespaceRequest request =
CreateNamespaceRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setNamespace(namespace)
.setNamespaceId(namespaceId)
.build();
return createNamespace(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a namespace, and returns the new namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Namespace namespace = Namespace.newBuilder().build();
* String namespaceId = "namespaceId790852566";
* Namespace response =
* registrationServiceClient.createNamespace(parent, namespace, namespaceId);
* }
* }
*
* @param parent Required. The resource name of the project and location the namespace will be
* created in.
* @param namespace Required. A namespace with initial fields set.
* @param namespaceId Required. The Resource ID must be 1-63 characters long, and comply with
* <a href="https://www.ietf.org/rfc/rfc1035.txt" target="_blank">RFC1035</a>.
* Specifically, the name must be 1-63 characters long and match the regular expression
* `[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?` which means the first character must be a lowercase
* letter, and all following characters must be a dash, lowercase letter, or digit, except the
* last character, which cannot be a dash.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Namespace createNamespace(String parent, Namespace namespace, String namespaceId) {
CreateNamespaceRequest request =
CreateNamespaceRequest.newBuilder()
.setParent(parent)
.setNamespace(namespace)
.setNamespaceId(namespaceId)
.build();
return createNamespace(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a namespace, and returns the new namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* CreateNamespaceRequest request =
* CreateNamespaceRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setNamespaceId("namespaceId790852566")
* .setNamespace(Namespace.newBuilder().build())
* .build();
* Namespace response = registrationServiceClient.createNamespace(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Namespace createNamespace(CreateNamespaceRequest request) {
return createNamespaceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a namespace, and returns the new namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* CreateNamespaceRequest request =
* CreateNamespaceRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setNamespaceId("namespaceId790852566")
* .setNamespace(Namespace.newBuilder().build())
* .build();
* ApiFuture future =
* registrationServiceClient.createNamespaceCallable().futureCall(request);
* // Do something.
* Namespace response = future.get();
* }
* }
*/
public final UnaryCallable createNamespaceCallable() {
return stub.createNamespaceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all namespaces.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Namespace element : registrationServiceClient.listNamespaces(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The resource name of the project and location whose namespaces you'd
* like to list.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListNamespacesPagedResponse listNamespaces(LocationName parent) {
ListNamespacesRequest request =
ListNamespacesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listNamespaces(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all namespaces.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Namespace element : registrationServiceClient.listNamespaces(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The resource name of the project and location whose namespaces you'd
* like to list.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListNamespacesPagedResponse listNamespaces(String parent) {
ListNamespacesRequest request = ListNamespacesRequest.newBuilder().setParent(parent).build();
return listNamespaces(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all namespaces.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListNamespacesRequest request =
* ListNamespacesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Namespace element : registrationServiceClient.listNamespaces(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListNamespacesPagedResponse listNamespaces(ListNamespacesRequest request) {
return listNamespacesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all namespaces.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListNamespacesRequest request =
* ListNamespacesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* registrationServiceClient.listNamespacesPagedCallable().futureCall(request);
* // Do something.
* for (Namespace element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listNamespacesPagedCallable() {
return stub.listNamespacesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all namespaces.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListNamespacesRequest request =
* ListNamespacesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListNamespacesResponse response =
* registrationServiceClient.listNamespacesCallable().call(request);
* for (Namespace element : response.getNamespacesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listNamespacesCallable() {
return stub.listNamespacesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* NamespaceName name = NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]");
* Namespace response = registrationServiceClient.getNamespace(name);
* }
* }
*
* @param name Required. The name of the namespace to retrieve.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Namespace getNamespace(NamespaceName name) {
GetNamespaceRequest request =
GetNamespaceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getNamespace(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String name = NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString();
* Namespace response = registrationServiceClient.getNamespace(name);
* }
* }
*
* @param name Required. The name of the namespace to retrieve.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Namespace getNamespace(String name) {
GetNamespaceRequest request = GetNamespaceRequest.newBuilder().setName(name).build();
return getNamespace(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetNamespaceRequest request =
* GetNamespaceRequest.newBuilder()
* .setName(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .build();
* Namespace response = registrationServiceClient.getNamespace(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Namespace getNamespace(GetNamespaceRequest request) {
return getNamespaceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetNamespaceRequest request =
* GetNamespaceRequest.newBuilder()
* .setName(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .build();
* ApiFuture future =
* registrationServiceClient.getNamespaceCallable().futureCall(request);
* // Do something.
* Namespace response = future.get();
* }
* }
*/
public final UnaryCallable getNamespaceCallable() {
return stub.getNamespaceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* Namespace namespace = Namespace.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Namespace response = registrationServiceClient.updateNamespace(namespace, updateMask);
* }
* }
*
* @param namespace Required. The updated namespace.
* @param updateMask Required. List of fields to be updated in this request.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Namespace updateNamespace(Namespace namespace, FieldMask updateMask) {
UpdateNamespaceRequest request =
UpdateNamespaceRequest.newBuilder()
.setNamespace(namespace)
.setUpdateMask(updateMask)
.build();
return updateNamespace(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* UpdateNamespaceRequest request =
* UpdateNamespaceRequest.newBuilder()
* .setNamespace(Namespace.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Namespace response = registrationServiceClient.updateNamespace(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Namespace updateNamespace(UpdateNamespaceRequest request) {
return updateNamespaceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* UpdateNamespaceRequest request =
* UpdateNamespaceRequest.newBuilder()
* .setNamespace(Namespace.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* registrationServiceClient.updateNamespaceCallable().futureCall(request);
* // Do something.
* Namespace response = future.get();
* }
* }
*/
public final UnaryCallable updateNamespaceCallable() {
return stub.updateNamespaceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a namespace. This also deletes all services and endpoints in the namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* NamespaceName name = NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]");
* registrationServiceClient.deleteNamespace(name);
* }
* }
*
* @param name Required. The name of the namespace to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteNamespace(NamespaceName name) {
DeleteNamespaceRequest request =
DeleteNamespaceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
deleteNamespace(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a namespace. This also deletes all services and endpoints in the namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String name = NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString();
* registrationServiceClient.deleteNamespace(name);
* }
* }
*
* @param name Required. The name of the namespace to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteNamespace(String name) {
DeleteNamespaceRequest request = DeleteNamespaceRequest.newBuilder().setName(name).build();
deleteNamespace(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a namespace. This also deletes all services and endpoints in the namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* DeleteNamespaceRequest request =
* DeleteNamespaceRequest.newBuilder()
* .setName(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .build();
* registrationServiceClient.deleteNamespace(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteNamespace(DeleteNamespaceRequest request) {
deleteNamespaceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a namespace. This also deletes all services and endpoints in the namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* DeleteNamespaceRequest request =
* DeleteNamespaceRequest.newBuilder()
* .setName(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .build();
* ApiFuture future =
* registrationServiceClient.deleteNamespaceCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteNamespaceCallable() {
return stub.deleteNamespaceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a service, and returns the new service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* NamespaceName parent = NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]");
* Service service = Service.newBuilder().build();
* String serviceId = "serviceId-194185552";
* Service response = registrationServiceClient.createService(parent, service, serviceId);
* }
* }
*
* @param parent Required. The resource name of the namespace this service will belong to.
* @param service Required. A service with initial fields set.
* @param serviceId Required. The Resource ID must be 1-63 characters long, and comply with <a
* href="https://www.ietf.org/rfc/rfc1035.txt" target="_blank">RFC1035</a>.
* Specifically, the name must be 1-63 characters long and match the regular expression
* `[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?` which means the first character must be a lowercase
* letter, and all following characters must be a dash, lowercase letter, or digit, except the
* last character, which cannot be a dash.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Service createService(NamespaceName parent, Service service, String serviceId) {
CreateServiceRequest request =
CreateServiceRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setService(service)
.setServiceId(serviceId)
.build();
return createService(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a service, and returns the new service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String parent = NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString();
* Service service = Service.newBuilder().build();
* String serviceId = "serviceId-194185552";
* Service response = registrationServiceClient.createService(parent, service, serviceId);
* }
* }
*
* @param parent Required. The resource name of the namespace this service will belong to.
* @param service Required. A service with initial fields set.
* @param serviceId Required. The Resource ID must be 1-63 characters long, and comply with <a
* href="https://www.ietf.org/rfc/rfc1035.txt" target="_blank">RFC1035</a>.
* Specifically, the name must be 1-63 characters long and match the regular expression
* `[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?` which means the first character must be a lowercase
* letter, and all following characters must be a dash, lowercase letter, or digit, except the
* last character, which cannot be a dash.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Service createService(String parent, Service service, String serviceId) {
CreateServiceRequest request =
CreateServiceRequest.newBuilder()
.setParent(parent)
.setService(service)
.setServiceId(serviceId)
.build();
return createService(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a service, and returns the new service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* CreateServiceRequest request =
* CreateServiceRequest.newBuilder()
* .setParent(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .setServiceId("serviceId-194185552")
* .setService(Service.newBuilder().build())
* .build();
* Service response = registrationServiceClient.createService(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Service createService(CreateServiceRequest request) {
return createServiceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a service, and returns the new service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* CreateServiceRequest request =
* CreateServiceRequest.newBuilder()
* .setParent(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .setServiceId("serviceId-194185552")
* .setService(Service.newBuilder().build())
* .build();
* ApiFuture future =
* registrationServiceClient.createServiceCallable().futureCall(request);
* // Do something.
* Service response = future.get();
* }
* }
*/
public final UnaryCallable createServiceCallable() {
return stub.createServiceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all services belonging to a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* NamespaceName parent = NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]");
* for (Service element : registrationServiceClient.listServices(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The resource name of the namespace whose services you'd like to list.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServicesPagedResponse listServices(NamespaceName parent) {
ListServicesRequest request =
ListServicesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listServices(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all services belonging to a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String parent = NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString();
* for (Service element : registrationServiceClient.listServices(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The resource name of the namespace whose services you'd like to list.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServicesPagedResponse listServices(String parent) {
ListServicesRequest request = ListServicesRequest.newBuilder().setParent(parent).build();
return listServices(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all services belonging to a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListServicesRequest request =
* ListServicesRequest.newBuilder()
* .setParent(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Service element : registrationServiceClient.listServices(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServicesPagedResponse listServices(ListServicesRequest request) {
return listServicesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all services belonging to a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListServicesRequest request =
* ListServicesRequest.newBuilder()
* .setParent(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* registrationServiceClient.listServicesPagedCallable().futureCall(request);
* // Do something.
* for (Service element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listServicesPagedCallable() {
return stub.listServicesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all services belonging to a namespace.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListServicesRequest request =
* ListServicesRequest.newBuilder()
* .setParent(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListServicesResponse response =
* registrationServiceClient.listServicesCallable().call(request);
* for (Service element : response.getServicesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listServicesCallable() {
return stub.listServicesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ServiceName name = ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]");
* Service response = registrationServiceClient.getService(name);
* }
* }
*
* @param name Required. The name of the service to get.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Service getService(ServiceName name) {
GetServiceRequest request =
GetServiceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getService(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String name =
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString();
* Service response = registrationServiceClient.getService(name);
* }
* }
*
* @param name Required. The name of the service to get.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Service getService(String name) {
GetServiceRequest request = GetServiceRequest.newBuilder().setName(name).build();
return getService(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetServiceRequest request =
* GetServiceRequest.newBuilder()
* .setName(
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString())
* .build();
* Service response = registrationServiceClient.getService(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Service getService(GetServiceRequest request) {
return getServiceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetServiceRequest request =
* GetServiceRequest.newBuilder()
* .setName(
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString())
* .build();
* ApiFuture future =
* registrationServiceClient.getServiceCallable().futureCall(request);
* // Do something.
* Service response = future.get();
* }
* }
*/
public final UnaryCallable getServiceCallable() {
return stub.getServiceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* Service service = Service.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Service response = registrationServiceClient.updateService(service, updateMask);
* }
* }
*
* @param service Required. The updated service.
* @param updateMask Required. List of fields to be updated in this request.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Service updateService(Service service, FieldMask updateMask) {
UpdateServiceRequest request =
UpdateServiceRequest.newBuilder().setService(service).setUpdateMask(updateMask).build();
return updateService(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* UpdateServiceRequest request =
* UpdateServiceRequest.newBuilder()
* .setService(Service.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Service response = registrationServiceClient.updateService(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Service updateService(UpdateServiceRequest request) {
return updateServiceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* UpdateServiceRequest request =
* UpdateServiceRequest.newBuilder()
* .setService(Service.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* registrationServiceClient.updateServiceCallable().futureCall(request);
* // Do something.
* Service response = future.get();
* }
* }
*/
public final UnaryCallable updateServiceCallable() {
return stub.updateServiceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a service. This also deletes all endpoints associated with the service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ServiceName name = ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]");
* registrationServiceClient.deleteService(name);
* }
* }
*
* @param name Required. The name of the service to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteService(ServiceName name) {
DeleteServiceRequest request =
DeleteServiceRequest.newBuilder().setName(name == null ? null : name.toString()).build();
deleteService(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a service. This also deletes all endpoints associated with the service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String name =
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString();
* registrationServiceClient.deleteService(name);
* }
* }
*
* @param name Required. The name of the service to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteService(String name) {
DeleteServiceRequest request = DeleteServiceRequest.newBuilder().setName(name).build();
deleteService(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a service. This also deletes all endpoints associated with the service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* DeleteServiceRequest request =
* DeleteServiceRequest.newBuilder()
* .setName(
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString())
* .build();
* registrationServiceClient.deleteService(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteService(DeleteServiceRequest request) {
deleteServiceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a service. This also deletes all endpoints associated with the service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* DeleteServiceRequest request =
* DeleteServiceRequest.newBuilder()
* .setName(
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString())
* .build();
* ApiFuture future =
* registrationServiceClient.deleteServiceCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteServiceCallable() {
return stub.deleteServiceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an endpoint, and returns the new endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ServiceName parent = ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]");
* Endpoint endpoint = Endpoint.newBuilder().build();
* String endpointId = "endpointId-1837754992";
* Endpoint response = registrationServiceClient.createEndpoint(parent, endpoint, endpointId);
* }
* }
*
* @param parent Required. The resource name of the service that this endpoint provides.
* @param endpoint Required. A endpoint with initial fields set.
* @param endpointId Required. The Resource ID must be 1-63 characters long, and comply with <a
* href="https://www.ietf.org/rfc/rfc1035.txt" target="_blank">RFC1035</a>.
* Specifically, the name must be 1-63 characters long and match the regular expression
* `[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?` which means the first character must be a lowercase
* letter, and all following characters must be a dash, lowercase letter, or digit, except the
* last character, which cannot be a dash.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Endpoint createEndpoint(ServiceName parent, Endpoint endpoint, String endpointId) {
CreateEndpointRequest request =
CreateEndpointRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setEndpoint(endpoint)
.setEndpointId(endpointId)
.build();
return createEndpoint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an endpoint, and returns the new endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String parent =
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString();
* Endpoint endpoint = Endpoint.newBuilder().build();
* String endpointId = "endpointId-1837754992";
* Endpoint response = registrationServiceClient.createEndpoint(parent, endpoint, endpointId);
* }
* }
*
* @param parent Required. The resource name of the service that this endpoint provides.
* @param endpoint Required. A endpoint with initial fields set.
* @param endpointId Required. The Resource ID must be 1-63 characters long, and comply with <a
* href="https://www.ietf.org/rfc/rfc1035.txt" target="_blank">RFC1035</a>.
* Specifically, the name must be 1-63 characters long and match the regular expression
* `[a-z](?:[-a-z0-9]{0,61}[a-z0-9])?` which means the first character must be a lowercase
* letter, and all following characters must be a dash, lowercase letter, or digit, except the
* last character, which cannot be a dash.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Endpoint createEndpoint(String parent, Endpoint endpoint, String endpointId) {
CreateEndpointRequest request =
CreateEndpointRequest.newBuilder()
.setParent(parent)
.setEndpoint(endpoint)
.setEndpointId(endpointId)
.build();
return createEndpoint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an endpoint, and returns the new endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* CreateEndpointRequest request =
* CreateEndpointRequest.newBuilder()
* .setParent(
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString())
* .setEndpointId("endpointId-1837754992")
* .setEndpoint(Endpoint.newBuilder().build())
* .build();
* Endpoint response = registrationServiceClient.createEndpoint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Endpoint createEndpoint(CreateEndpointRequest request) {
return createEndpointCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates an endpoint, and returns the new endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* CreateEndpointRequest request =
* CreateEndpointRequest.newBuilder()
* .setParent(
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString())
* .setEndpointId("endpointId-1837754992")
* .setEndpoint(Endpoint.newBuilder().build())
* .build();
* ApiFuture future =
* registrationServiceClient.createEndpointCallable().futureCall(request);
* // Do something.
* Endpoint response = future.get();
* }
* }
*/
public final UnaryCallable createEndpointCallable() {
return stub.createEndpointCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all endpoints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ServiceName parent = ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]");
* for (Endpoint element : registrationServiceClient.listEndpoints(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The resource name of the service whose endpoints you'd like to list.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEndpointsPagedResponse listEndpoints(ServiceName parent) {
ListEndpointsRequest request =
ListEndpointsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listEndpoints(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all endpoints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String parent =
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString();
* for (Endpoint element : registrationServiceClient.listEndpoints(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The resource name of the service whose endpoints you'd like to list.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEndpointsPagedResponse listEndpoints(String parent) {
ListEndpointsRequest request = ListEndpointsRequest.newBuilder().setParent(parent).build();
return listEndpoints(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all endpoints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListEndpointsRequest request =
* ListEndpointsRequest.newBuilder()
* .setParent(
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (Endpoint element : registrationServiceClient.listEndpoints(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEndpointsPagedResponse listEndpoints(ListEndpointsRequest request) {
return listEndpointsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all endpoints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListEndpointsRequest request =
* ListEndpointsRequest.newBuilder()
* .setParent(
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* registrationServiceClient.listEndpointsPagedCallable().futureCall(request);
* // Do something.
* for (Endpoint element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listEndpointsPagedCallable() {
return stub.listEndpointsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists all endpoints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListEndpointsRequest request =
* ListEndpointsRequest.newBuilder()
* .setParent(
* ServiceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListEndpointsResponse response =
* registrationServiceClient.listEndpointsCallable().call(request);
* for (Endpoint element : response.getEndpointsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listEndpointsCallable() {
return stub.listEndpointsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* EndpointName name =
* EndpointName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]", "[ENDPOINT]");
* Endpoint response = registrationServiceClient.getEndpoint(name);
* }
* }
*
* @param name Required. The name of the endpoint to get.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Endpoint getEndpoint(EndpointName name) {
GetEndpointRequest request =
GetEndpointRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getEndpoint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String name =
* EndpointName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]", "[ENDPOINT]")
* .toString();
* Endpoint response = registrationServiceClient.getEndpoint(name);
* }
* }
*
* @param name Required. The name of the endpoint to get.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Endpoint getEndpoint(String name) {
GetEndpointRequest request = GetEndpointRequest.newBuilder().setName(name).build();
return getEndpoint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetEndpointRequest request =
* GetEndpointRequest.newBuilder()
* .setName(
* EndpointName.of(
* "[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]", "[ENDPOINT]")
* .toString())
* .build();
* Endpoint response = registrationServiceClient.getEndpoint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Endpoint getEndpoint(GetEndpointRequest request) {
return getEndpointCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetEndpointRequest request =
* GetEndpointRequest.newBuilder()
* .setName(
* EndpointName.of(
* "[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]", "[ENDPOINT]")
* .toString())
* .build();
* ApiFuture future =
* registrationServiceClient.getEndpointCallable().futureCall(request);
* // Do something.
* Endpoint response = future.get();
* }
* }
*/
public final UnaryCallable getEndpointCallable() {
return stub.getEndpointCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* Endpoint endpoint = Endpoint.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Endpoint response = registrationServiceClient.updateEndpoint(endpoint, updateMask);
* }
* }
*
* @param endpoint Required. The updated endpoint.
* @param updateMask Required. List of fields to be updated in this request.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Endpoint updateEndpoint(Endpoint endpoint, FieldMask updateMask) {
UpdateEndpointRequest request =
UpdateEndpointRequest.newBuilder().setEndpoint(endpoint).setUpdateMask(updateMask).build();
return updateEndpoint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* UpdateEndpointRequest request =
* UpdateEndpointRequest.newBuilder()
* .setEndpoint(Endpoint.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Endpoint response = registrationServiceClient.updateEndpoint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Endpoint updateEndpoint(UpdateEndpointRequest request) {
return updateEndpointCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* UpdateEndpointRequest request =
* UpdateEndpointRequest.newBuilder()
* .setEndpoint(Endpoint.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* registrationServiceClient.updateEndpointCallable().futureCall(request);
* // Do something.
* Endpoint response = future.get();
* }
* }
*/
public final UnaryCallable updateEndpointCallable() {
return stub.updateEndpointCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* EndpointName name =
* EndpointName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]", "[ENDPOINT]");
* registrationServiceClient.deleteEndpoint(name);
* }
* }
*
* @param name Required. The name of the endpoint to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteEndpoint(EndpointName name) {
DeleteEndpointRequest request =
DeleteEndpointRequest.newBuilder().setName(name == null ? null : name.toString()).build();
deleteEndpoint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* String name =
* EndpointName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]", "[ENDPOINT]")
* .toString();
* registrationServiceClient.deleteEndpoint(name);
* }
* }
*
* @param name Required. The name of the endpoint to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteEndpoint(String name) {
DeleteEndpointRequest request = DeleteEndpointRequest.newBuilder().setName(name).build();
deleteEndpoint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* DeleteEndpointRequest request =
* DeleteEndpointRequest.newBuilder()
* .setName(
* EndpointName.of(
* "[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]", "[ENDPOINT]")
* .toString())
* .build();
* registrationServiceClient.deleteEndpoint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteEndpoint(DeleteEndpointRequest request) {
deleteEndpointCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes an endpoint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* DeleteEndpointRequest request =
* DeleteEndpointRequest.newBuilder()
* .setName(
* EndpointName.of(
* "[PROJECT]", "[LOCATION]", "[NAMESPACE]", "[SERVICE]", "[ENDPOINT]")
* .toString())
* .build();
* ApiFuture future =
* registrationServiceClient.deleteEndpointCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteEndpointCallable() {
return stub.deleteEndpointCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the IAM Policy for a resource
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* Policy response = registrationServiceClient.getIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(GetIamPolicyRequest request) {
return getIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the IAM Policy for a resource
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* ApiFuture future =
* registrationServiceClient.getIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable getIamPolicyCallable() {
return stub.getIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the IAM Policy for a resource
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Policy response = registrationServiceClient.setIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(SetIamPolicyRequest request) {
return setIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the IAM Policy for a resource
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* registrationServiceClient.setIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable setIamPolicyCallable() {
return stub.setIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tests IAM permissions for a resource (namespace, service or service workload only).
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* TestIamPermissionsResponse response = registrationServiceClient.testIamPermissions(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(TestIamPermissionsRequest request) {
return testIamPermissionsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tests IAM permissions for a resource (namespace, service or service workload only).
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(NamespaceName.of("[PROJECT]", "[LOCATION]", "[NAMESPACE]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* ApiFuture future =
* registrationServiceClient.testIamPermissionsCallable().futureCall(request);
* // Do something.
* TestIamPermissionsResponse response = future.get();
* }
* }
*/
public final UnaryCallable
testIamPermissionsCallable() {
return stub.testIamPermissionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : registrationServiceClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* registrationServiceClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response =
* registrationServiceClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = registrationServiceClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (RegistrationServiceClient registrationServiceClient = RegistrationServiceClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future =
* registrationServiceClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListNamespacesPagedResponse
extends AbstractPagedListResponse<
ListNamespacesRequest,
ListNamespacesResponse,
Namespace,
ListNamespacesPage,
ListNamespacesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListNamespacesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListNamespacesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListNamespacesPagedResponse(ListNamespacesPage page) {
super(page, ListNamespacesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListNamespacesPage
extends AbstractPage<
ListNamespacesRequest, ListNamespacesResponse, Namespace, ListNamespacesPage> {
private ListNamespacesPage(
PageContext context,
ListNamespacesResponse response) {
super(context, response);
}
private static ListNamespacesPage createEmptyPage() {
return new ListNamespacesPage(null, null);
}
@Override
protected ListNamespacesPage createPage(
PageContext context,
ListNamespacesResponse response) {
return new ListNamespacesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListNamespacesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListNamespacesRequest,
ListNamespacesResponse,
Namespace,
ListNamespacesPage,
ListNamespacesFixedSizeCollection> {
private ListNamespacesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListNamespacesFixedSizeCollection createEmptyCollection() {
return new ListNamespacesFixedSizeCollection(null, 0);
}
@Override
protected ListNamespacesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListNamespacesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListServicesPagedResponse
extends AbstractPagedListResponse<
ListServicesRequest,
ListServicesResponse,
Service,
ListServicesPage,
ListServicesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListServicesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListServicesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListServicesPagedResponse(ListServicesPage page) {
super(page, ListServicesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListServicesPage
extends AbstractPage {
private ListServicesPage(
PageContext context,
ListServicesResponse response) {
super(context, response);
}
private static ListServicesPage createEmptyPage() {
return new ListServicesPage(null, null);
}
@Override
protected ListServicesPage createPage(
PageContext context,
ListServicesResponse response) {
return new ListServicesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListServicesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListServicesRequest,
ListServicesResponse,
Service,
ListServicesPage,
ListServicesFixedSizeCollection> {
private ListServicesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListServicesFixedSizeCollection createEmptyCollection() {
return new ListServicesFixedSizeCollection(null, 0);
}
@Override
protected ListServicesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListServicesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListEndpointsPagedResponse
extends AbstractPagedListResponse<
ListEndpointsRequest,
ListEndpointsResponse,
Endpoint,
ListEndpointsPage,
ListEndpointsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListEndpointsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListEndpointsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListEndpointsPagedResponse(ListEndpointsPage page) {
super(page, ListEndpointsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListEndpointsPage
extends AbstractPage<
ListEndpointsRequest, ListEndpointsResponse, Endpoint, ListEndpointsPage> {
private ListEndpointsPage(
PageContext context,
ListEndpointsResponse response) {
super(context, response);
}
private static ListEndpointsPage createEmptyPage() {
return new ListEndpointsPage(null, null);
}
@Override
protected ListEndpointsPage createPage(
PageContext context,
ListEndpointsResponse response) {
return new ListEndpointsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListEndpointsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListEndpointsRequest,
ListEndpointsResponse,
Endpoint,
ListEndpointsPage,
ListEndpointsFixedSizeCollection> {
private ListEndpointsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListEndpointsFixedSizeCollection createEmptyCollection() {
return new ListEndpointsFixedSizeCollection(null, 0);
}
@Override
protected ListEndpointsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListEndpointsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}