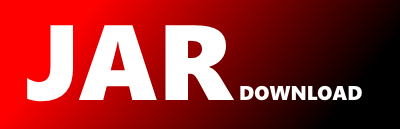
com.google.cloud.speech.v2.stub.GrpcSpeechStub Maven / Gradle / Ivy
Show all versions of google-cloud-speech Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.speech.v2.stub;
import static com.google.cloud.speech.v2.SpeechClient.ListCustomClassesPagedResponse;
import static com.google.cloud.speech.v2.SpeechClient.ListLocationsPagedResponse;
import static com.google.cloud.speech.v2.SpeechClient.ListPhraseSetsPagedResponse;
import static com.google.cloud.speech.v2.SpeechClient.ListRecognizersPagedResponse;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.core.BackgroundResourceAggregation;
import com.google.api.gax.grpc.GrpcCallSettings;
import com.google.api.gax.grpc.GrpcStubCallableFactory;
import com.google.api.gax.rpc.BidiStreamingCallable;
import com.google.api.gax.rpc.ClientContext;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.RequestParamsBuilder;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.speech.v2.BatchRecognizeRequest;
import com.google.cloud.speech.v2.BatchRecognizeResponse;
import com.google.cloud.speech.v2.Config;
import com.google.cloud.speech.v2.CreateCustomClassRequest;
import com.google.cloud.speech.v2.CreatePhraseSetRequest;
import com.google.cloud.speech.v2.CreateRecognizerRequest;
import com.google.cloud.speech.v2.CustomClass;
import com.google.cloud.speech.v2.DeleteCustomClassRequest;
import com.google.cloud.speech.v2.DeletePhraseSetRequest;
import com.google.cloud.speech.v2.DeleteRecognizerRequest;
import com.google.cloud.speech.v2.GetConfigRequest;
import com.google.cloud.speech.v2.GetCustomClassRequest;
import com.google.cloud.speech.v2.GetPhraseSetRequest;
import com.google.cloud.speech.v2.GetRecognizerRequest;
import com.google.cloud.speech.v2.ListCustomClassesRequest;
import com.google.cloud.speech.v2.ListCustomClassesResponse;
import com.google.cloud.speech.v2.ListPhraseSetsRequest;
import com.google.cloud.speech.v2.ListPhraseSetsResponse;
import com.google.cloud.speech.v2.ListRecognizersRequest;
import com.google.cloud.speech.v2.ListRecognizersResponse;
import com.google.cloud.speech.v2.OperationMetadata;
import com.google.cloud.speech.v2.PhraseSet;
import com.google.cloud.speech.v2.RecognizeRequest;
import com.google.cloud.speech.v2.RecognizeResponse;
import com.google.cloud.speech.v2.Recognizer;
import com.google.cloud.speech.v2.StreamingRecognizeRequest;
import com.google.cloud.speech.v2.StreamingRecognizeResponse;
import com.google.cloud.speech.v2.UndeleteCustomClassRequest;
import com.google.cloud.speech.v2.UndeletePhraseSetRequest;
import com.google.cloud.speech.v2.UndeleteRecognizerRequest;
import com.google.cloud.speech.v2.UpdateConfigRequest;
import com.google.cloud.speech.v2.UpdateCustomClassRequest;
import com.google.cloud.speech.v2.UpdatePhraseSetRequest;
import com.google.cloud.speech.v2.UpdateRecognizerRequest;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.GrpcOperationsStub;
import io.grpc.MethodDescriptor;
import io.grpc.protobuf.ProtoUtils;
import java.io.IOException;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* gRPC stub implementation for the Speech service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@Generated("by gapic-generator-java")
public class GrpcSpeechStub extends SpeechStub {
private static final MethodDescriptor
createRecognizerMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/CreateRecognizer")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateRecognizerRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listRecognizersMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/ListRecognizers")
.setRequestMarshaller(
ProtoUtils.marshaller(ListRecognizersRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListRecognizersResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getRecognizerMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/GetRecognizer")
.setRequestMarshaller(
ProtoUtils.marshaller(GetRecognizerRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Recognizer.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateRecognizerMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/UpdateRecognizer")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateRecognizerRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteRecognizerMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/DeleteRecognizer")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteRecognizerRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
undeleteRecognizerMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/UndeleteRecognizer")
.setRequestMarshaller(
ProtoUtils.marshaller(UndeleteRecognizerRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
recognizeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/Recognize")
.setRequestMarshaller(ProtoUtils.marshaller(RecognizeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(RecognizeResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
streamingRecognizeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.BIDI_STREAMING)
.setFullMethodName("google.cloud.speech.v2.Speech/StreamingRecognize")
.setRequestMarshaller(
ProtoUtils.marshaller(StreamingRecognizeRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(StreamingRecognizeResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
batchRecognizeMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/BatchRecognize")
.setRequestMarshaller(
ProtoUtils.marshaller(BatchRecognizeRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor getConfigMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/GetConfig")
.setRequestMarshaller(ProtoUtils.marshaller(GetConfigRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Config.getDefaultInstance()))
.build();
private static final MethodDescriptor updateConfigMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/UpdateConfig")
.setRequestMarshaller(ProtoUtils.marshaller(UpdateConfigRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Config.getDefaultInstance()))
.build();
private static final MethodDescriptor
createCustomClassMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/CreateCustomClass")
.setRequestMarshaller(
ProtoUtils.marshaller(CreateCustomClassRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listCustomClassesMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/ListCustomClasses")
.setRequestMarshaller(
ProtoUtils.marshaller(ListCustomClassesRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListCustomClassesResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getCustomClassMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/GetCustomClass")
.setRequestMarshaller(
ProtoUtils.marshaller(GetCustomClassRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(CustomClass.getDefaultInstance()))
.build();
private static final MethodDescriptor
updateCustomClassMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/UpdateCustomClass")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdateCustomClassRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deleteCustomClassMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/DeleteCustomClass")
.setRequestMarshaller(
ProtoUtils.marshaller(DeleteCustomClassRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
undeleteCustomClassMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/UndeleteCustomClass")
.setRequestMarshaller(
ProtoUtils.marshaller(UndeleteCustomClassRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
createPhraseSetMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/CreatePhraseSet")
.setRequestMarshaller(
ProtoUtils.marshaller(CreatePhraseSetRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listPhraseSetsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/ListPhraseSets")
.setRequestMarshaller(
ProtoUtils.marshaller(ListPhraseSetsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListPhraseSetsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor
getPhraseSetMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/GetPhraseSet")
.setRequestMarshaller(ProtoUtils.marshaller(GetPhraseSetRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(PhraseSet.getDefaultInstance()))
.build();
private static final MethodDescriptor
updatePhraseSetMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/UpdatePhraseSet")
.setRequestMarshaller(
ProtoUtils.marshaller(UpdatePhraseSetRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
deletePhraseSetMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/DeletePhraseSet")
.setRequestMarshaller(
ProtoUtils.marshaller(DeletePhraseSetRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
undeletePhraseSetMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.speech.v2.Speech/UndeletePhraseSet")
.setRequestMarshaller(
ProtoUtils.marshaller(UndeletePhraseSetRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Operation.getDefaultInstance()))
.build();
private static final MethodDescriptor
listLocationsMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/ListLocations")
.setRequestMarshaller(
ProtoUtils.marshaller(ListLocationsRequest.getDefaultInstance()))
.setResponseMarshaller(
ProtoUtils.marshaller(ListLocationsResponse.getDefaultInstance()))
.build();
private static final MethodDescriptor getLocationMethodDescriptor =
MethodDescriptor.newBuilder()
.setType(MethodDescriptor.MethodType.UNARY)
.setFullMethodName("google.cloud.location.Locations/GetLocation")
.setRequestMarshaller(ProtoUtils.marshaller(GetLocationRequest.getDefaultInstance()))
.setResponseMarshaller(ProtoUtils.marshaller(Location.getDefaultInstance()))
.build();
private final UnaryCallable createRecognizerCallable;
private final OperationCallable
createRecognizerOperationCallable;
private final UnaryCallable
listRecognizersCallable;
private final UnaryCallable
listRecognizersPagedCallable;
private final UnaryCallable getRecognizerCallable;
private final UnaryCallable updateRecognizerCallable;
private final OperationCallable
updateRecognizerOperationCallable;
private final UnaryCallable deleteRecognizerCallable;
private final OperationCallable
deleteRecognizerOperationCallable;
private final UnaryCallable undeleteRecognizerCallable;
private final OperationCallable
undeleteRecognizerOperationCallable;
private final UnaryCallable recognizeCallable;
private final BidiStreamingCallable
streamingRecognizeCallable;
private final UnaryCallable batchRecognizeCallable;
private final OperationCallable
batchRecognizeOperationCallable;
private final UnaryCallable getConfigCallable;
private final UnaryCallable updateConfigCallable;
private final UnaryCallable createCustomClassCallable;
private final OperationCallable
createCustomClassOperationCallable;
private final UnaryCallable
listCustomClassesCallable;
private final UnaryCallable
listCustomClassesPagedCallable;
private final UnaryCallable getCustomClassCallable;
private final UnaryCallable updateCustomClassCallable;
private final OperationCallable
updateCustomClassOperationCallable;
private final UnaryCallable deleteCustomClassCallable;
private final OperationCallable
deleteCustomClassOperationCallable;
private final UnaryCallable undeleteCustomClassCallable;
private final OperationCallable
undeleteCustomClassOperationCallable;
private final UnaryCallable createPhraseSetCallable;
private final OperationCallable
createPhraseSetOperationCallable;
private final UnaryCallable listPhraseSetsCallable;
private final UnaryCallable
listPhraseSetsPagedCallable;
private final UnaryCallable getPhraseSetCallable;
private final UnaryCallable updatePhraseSetCallable;
private final OperationCallable
updatePhraseSetOperationCallable;
private final UnaryCallable deletePhraseSetCallable;
private final OperationCallable
deletePhraseSetOperationCallable;
private final UnaryCallable undeletePhraseSetCallable;
private final OperationCallable
undeletePhraseSetOperationCallable;
private final UnaryCallable listLocationsCallable;
private final UnaryCallable
listLocationsPagedCallable;
private final UnaryCallable getLocationCallable;
private final BackgroundResource backgroundResources;
private final GrpcOperationsStub operationsStub;
private final GrpcStubCallableFactory callableFactory;
public static final GrpcSpeechStub create(SpeechStubSettings settings) throws IOException {
return new GrpcSpeechStub(settings, ClientContext.create(settings));
}
public static final GrpcSpeechStub create(ClientContext clientContext) throws IOException {
return new GrpcSpeechStub(SpeechStubSettings.newBuilder().build(), clientContext);
}
public static final GrpcSpeechStub create(
ClientContext clientContext, GrpcStubCallableFactory callableFactory) throws IOException {
return new GrpcSpeechStub(
SpeechStubSettings.newBuilder().build(), clientContext, callableFactory);
}
/**
* Constructs an instance of GrpcSpeechStub, using the given settings. This is protected so that
* it is easy to make a subclass, but otherwise, the static factory methods should be preferred.
*/
protected GrpcSpeechStub(SpeechStubSettings settings, ClientContext clientContext)
throws IOException {
this(settings, clientContext, new GrpcSpeechCallableFactory());
}
/**
* Constructs an instance of GrpcSpeechStub, using the given settings. This is protected so that
* it is easy to make a subclass, but otherwise, the static factory methods should be preferred.
*/
protected GrpcSpeechStub(
SpeechStubSettings settings,
ClientContext clientContext,
GrpcStubCallableFactory callableFactory)
throws IOException {
this.callableFactory = callableFactory;
this.operationsStub = GrpcOperationsStub.create(clientContext, callableFactory);
GrpcCallSettings createRecognizerTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createRecognizerMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
listRecognizersTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listRecognizersMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getRecognizerTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getRecognizerMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateRecognizerTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateRecognizerMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("recognizer.name", String.valueOf(request.getRecognizer().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteRecognizerTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteRecognizerMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings undeleteRecognizerTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(undeleteRecognizerMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings recognizeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(recognizeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("recognizer", String.valueOf(request.getRecognizer()));
return builder.build();
})
.build();
GrpcCallSettings
streamingRecognizeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(streamingRecognizeMethodDescriptor)
.build();
GrpcCallSettings batchRecognizeTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(batchRecognizeMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("recognizer", String.valueOf(request.getRecognizer()));
return builder.build();
})
.build();
GrpcCallSettings getConfigTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getConfigMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateConfigTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateConfigMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("config.name", String.valueOf(request.getConfig().getName()));
return builder.build();
})
.build();
GrpcCallSettings createCustomClassTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createCustomClassMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
listCustomClassesTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listCustomClassesMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getCustomClassTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getCustomClassMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updateCustomClassTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updateCustomClassMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add(
"custom_class.name", String.valueOf(request.getCustomClass().getName()));
return builder.build();
})
.build();
GrpcCallSettings deleteCustomClassTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deleteCustomClassMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings undeleteCustomClassTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(undeleteCustomClassMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings createPhraseSetTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(createPhraseSetMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings
listPhraseSetsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listPhraseSetsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("parent", String.valueOf(request.getParent()));
return builder.build();
})
.build();
GrpcCallSettings getPhraseSetTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getPhraseSetMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings updatePhraseSetTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(updatePhraseSetMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("phrase_set.name", String.valueOf(request.getPhraseSet().getName()));
return builder.build();
})
.build();
GrpcCallSettings deletePhraseSetTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(deletePhraseSetMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings undeletePhraseSetTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(undeletePhraseSetMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings listLocationsTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(listLocationsMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
GrpcCallSettings getLocationTransportSettings =
GrpcCallSettings.newBuilder()
.setMethodDescriptor(getLocationMethodDescriptor)
.setParamsExtractor(
request -> {
RequestParamsBuilder builder = RequestParamsBuilder.create();
builder.add("name", String.valueOf(request.getName()));
return builder.build();
})
.build();
this.createRecognizerCallable =
callableFactory.createUnaryCallable(
createRecognizerTransportSettings, settings.createRecognizerSettings(), clientContext);
this.createRecognizerOperationCallable =
callableFactory.createOperationCallable(
createRecognizerTransportSettings,
settings.createRecognizerOperationSettings(),
clientContext,
operationsStub);
this.listRecognizersCallable =
callableFactory.createUnaryCallable(
listRecognizersTransportSettings, settings.listRecognizersSettings(), clientContext);
this.listRecognizersPagedCallable =
callableFactory.createPagedCallable(
listRecognizersTransportSettings, settings.listRecognizersSettings(), clientContext);
this.getRecognizerCallable =
callableFactory.createUnaryCallable(
getRecognizerTransportSettings, settings.getRecognizerSettings(), clientContext);
this.updateRecognizerCallable =
callableFactory.createUnaryCallable(
updateRecognizerTransportSettings, settings.updateRecognizerSettings(), clientContext);
this.updateRecognizerOperationCallable =
callableFactory.createOperationCallable(
updateRecognizerTransportSettings,
settings.updateRecognizerOperationSettings(),
clientContext,
operationsStub);
this.deleteRecognizerCallable =
callableFactory.createUnaryCallable(
deleteRecognizerTransportSettings, settings.deleteRecognizerSettings(), clientContext);
this.deleteRecognizerOperationCallable =
callableFactory.createOperationCallable(
deleteRecognizerTransportSettings,
settings.deleteRecognizerOperationSettings(),
clientContext,
operationsStub);
this.undeleteRecognizerCallable =
callableFactory.createUnaryCallable(
undeleteRecognizerTransportSettings,
settings.undeleteRecognizerSettings(),
clientContext);
this.undeleteRecognizerOperationCallable =
callableFactory.createOperationCallable(
undeleteRecognizerTransportSettings,
settings.undeleteRecognizerOperationSettings(),
clientContext,
operationsStub);
this.recognizeCallable =
callableFactory.createUnaryCallable(
recognizeTransportSettings, settings.recognizeSettings(), clientContext);
this.streamingRecognizeCallable =
callableFactory.createBidiStreamingCallable(
streamingRecognizeTransportSettings,
settings.streamingRecognizeSettings(),
clientContext);
this.batchRecognizeCallable =
callableFactory.createUnaryCallable(
batchRecognizeTransportSettings, settings.batchRecognizeSettings(), clientContext);
this.batchRecognizeOperationCallable =
callableFactory.createOperationCallable(
batchRecognizeTransportSettings,
settings.batchRecognizeOperationSettings(),
clientContext,
operationsStub);
this.getConfigCallable =
callableFactory.createUnaryCallable(
getConfigTransportSettings, settings.getConfigSettings(), clientContext);
this.updateConfigCallable =
callableFactory.createUnaryCallable(
updateConfigTransportSettings, settings.updateConfigSettings(), clientContext);
this.createCustomClassCallable =
callableFactory.createUnaryCallable(
createCustomClassTransportSettings,
settings.createCustomClassSettings(),
clientContext);
this.createCustomClassOperationCallable =
callableFactory.createOperationCallable(
createCustomClassTransportSettings,
settings.createCustomClassOperationSettings(),
clientContext,
operationsStub);
this.listCustomClassesCallable =
callableFactory.createUnaryCallable(
listCustomClassesTransportSettings,
settings.listCustomClassesSettings(),
clientContext);
this.listCustomClassesPagedCallable =
callableFactory.createPagedCallable(
listCustomClassesTransportSettings,
settings.listCustomClassesSettings(),
clientContext);
this.getCustomClassCallable =
callableFactory.createUnaryCallable(
getCustomClassTransportSettings, settings.getCustomClassSettings(), clientContext);
this.updateCustomClassCallable =
callableFactory.createUnaryCallable(
updateCustomClassTransportSettings,
settings.updateCustomClassSettings(),
clientContext);
this.updateCustomClassOperationCallable =
callableFactory.createOperationCallable(
updateCustomClassTransportSettings,
settings.updateCustomClassOperationSettings(),
clientContext,
operationsStub);
this.deleteCustomClassCallable =
callableFactory.createUnaryCallable(
deleteCustomClassTransportSettings,
settings.deleteCustomClassSettings(),
clientContext);
this.deleteCustomClassOperationCallable =
callableFactory.createOperationCallable(
deleteCustomClassTransportSettings,
settings.deleteCustomClassOperationSettings(),
clientContext,
operationsStub);
this.undeleteCustomClassCallable =
callableFactory.createUnaryCallable(
undeleteCustomClassTransportSettings,
settings.undeleteCustomClassSettings(),
clientContext);
this.undeleteCustomClassOperationCallable =
callableFactory.createOperationCallable(
undeleteCustomClassTransportSettings,
settings.undeleteCustomClassOperationSettings(),
clientContext,
operationsStub);
this.createPhraseSetCallable =
callableFactory.createUnaryCallable(
createPhraseSetTransportSettings, settings.createPhraseSetSettings(), clientContext);
this.createPhraseSetOperationCallable =
callableFactory.createOperationCallable(
createPhraseSetTransportSettings,
settings.createPhraseSetOperationSettings(),
clientContext,
operationsStub);
this.listPhraseSetsCallable =
callableFactory.createUnaryCallable(
listPhraseSetsTransportSettings, settings.listPhraseSetsSettings(), clientContext);
this.listPhraseSetsPagedCallable =
callableFactory.createPagedCallable(
listPhraseSetsTransportSettings, settings.listPhraseSetsSettings(), clientContext);
this.getPhraseSetCallable =
callableFactory.createUnaryCallable(
getPhraseSetTransportSettings, settings.getPhraseSetSettings(), clientContext);
this.updatePhraseSetCallable =
callableFactory.createUnaryCallable(
updatePhraseSetTransportSettings, settings.updatePhraseSetSettings(), clientContext);
this.updatePhraseSetOperationCallable =
callableFactory.createOperationCallable(
updatePhraseSetTransportSettings,
settings.updatePhraseSetOperationSettings(),
clientContext,
operationsStub);
this.deletePhraseSetCallable =
callableFactory.createUnaryCallable(
deletePhraseSetTransportSettings, settings.deletePhraseSetSettings(), clientContext);
this.deletePhraseSetOperationCallable =
callableFactory.createOperationCallable(
deletePhraseSetTransportSettings,
settings.deletePhraseSetOperationSettings(),
clientContext,
operationsStub);
this.undeletePhraseSetCallable =
callableFactory.createUnaryCallable(
undeletePhraseSetTransportSettings,
settings.undeletePhraseSetSettings(),
clientContext);
this.undeletePhraseSetOperationCallable =
callableFactory.createOperationCallable(
undeletePhraseSetTransportSettings,
settings.undeletePhraseSetOperationSettings(),
clientContext,
operationsStub);
this.listLocationsCallable =
callableFactory.createUnaryCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.listLocationsPagedCallable =
callableFactory.createPagedCallable(
listLocationsTransportSettings, settings.listLocationsSettings(), clientContext);
this.getLocationCallable =
callableFactory.createUnaryCallable(
getLocationTransportSettings, settings.getLocationSettings(), clientContext);
this.backgroundResources =
new BackgroundResourceAggregation(clientContext.getBackgroundResources());
}
public GrpcOperationsStub getOperationsStub() {
return operationsStub;
}
@Override
public UnaryCallable createRecognizerCallable() {
return createRecognizerCallable;
}
@Override
public OperationCallable
createRecognizerOperationCallable() {
return createRecognizerOperationCallable;
}
@Override
public UnaryCallable listRecognizersCallable() {
return listRecognizersCallable;
}
@Override
public UnaryCallable
listRecognizersPagedCallable() {
return listRecognizersPagedCallable;
}
@Override
public UnaryCallable getRecognizerCallable() {
return getRecognizerCallable;
}
@Override
public UnaryCallable updateRecognizerCallable() {
return updateRecognizerCallable;
}
@Override
public OperationCallable
updateRecognizerOperationCallable() {
return updateRecognizerOperationCallable;
}
@Override
public UnaryCallable deleteRecognizerCallable() {
return deleteRecognizerCallable;
}
@Override
public OperationCallable
deleteRecognizerOperationCallable() {
return deleteRecognizerOperationCallable;
}
@Override
public UnaryCallable undeleteRecognizerCallable() {
return undeleteRecognizerCallable;
}
@Override
public OperationCallable
undeleteRecognizerOperationCallable() {
return undeleteRecognizerOperationCallable;
}
@Override
public UnaryCallable recognizeCallable() {
return recognizeCallable;
}
@Override
public BidiStreamingCallable
streamingRecognizeCallable() {
return streamingRecognizeCallable;
}
@Override
public UnaryCallable batchRecognizeCallable() {
return batchRecognizeCallable;
}
@Override
public OperationCallable
batchRecognizeOperationCallable() {
return batchRecognizeOperationCallable;
}
@Override
public UnaryCallable getConfigCallable() {
return getConfigCallable;
}
@Override
public UnaryCallable updateConfigCallable() {
return updateConfigCallable;
}
@Override
public UnaryCallable createCustomClassCallable() {
return createCustomClassCallable;
}
@Override
public OperationCallable
createCustomClassOperationCallable() {
return createCustomClassOperationCallable;
}
@Override
public UnaryCallable
listCustomClassesCallable() {
return listCustomClassesCallable;
}
@Override
public UnaryCallable
listCustomClassesPagedCallable() {
return listCustomClassesPagedCallable;
}
@Override
public UnaryCallable getCustomClassCallable() {
return getCustomClassCallable;
}
@Override
public UnaryCallable updateCustomClassCallable() {
return updateCustomClassCallable;
}
@Override
public OperationCallable
updateCustomClassOperationCallable() {
return updateCustomClassOperationCallable;
}
@Override
public UnaryCallable deleteCustomClassCallable() {
return deleteCustomClassCallable;
}
@Override
public OperationCallable
deleteCustomClassOperationCallable() {
return deleteCustomClassOperationCallable;
}
@Override
public UnaryCallable undeleteCustomClassCallable() {
return undeleteCustomClassCallable;
}
@Override
public OperationCallable
undeleteCustomClassOperationCallable() {
return undeleteCustomClassOperationCallable;
}
@Override
public UnaryCallable createPhraseSetCallable() {
return createPhraseSetCallable;
}
@Override
public OperationCallable
createPhraseSetOperationCallable() {
return createPhraseSetOperationCallable;
}
@Override
public UnaryCallable listPhraseSetsCallable() {
return listPhraseSetsCallable;
}
@Override
public UnaryCallable
listPhraseSetsPagedCallable() {
return listPhraseSetsPagedCallable;
}
@Override
public UnaryCallable getPhraseSetCallable() {
return getPhraseSetCallable;
}
@Override
public UnaryCallable updatePhraseSetCallable() {
return updatePhraseSetCallable;
}
@Override
public OperationCallable
updatePhraseSetOperationCallable() {
return updatePhraseSetOperationCallable;
}
@Override
public UnaryCallable deletePhraseSetCallable() {
return deletePhraseSetCallable;
}
@Override
public OperationCallable
deletePhraseSetOperationCallable() {
return deletePhraseSetOperationCallable;
}
@Override
public UnaryCallable undeletePhraseSetCallable() {
return undeletePhraseSetCallable;
}
@Override
public OperationCallable
undeletePhraseSetOperationCallable() {
return undeletePhraseSetOperationCallable;
}
@Override
public UnaryCallable listLocationsCallable() {
return listLocationsCallable;
}
@Override
public UnaryCallable
listLocationsPagedCallable() {
return listLocationsPagedCallable;
}
@Override
public UnaryCallable getLocationCallable() {
return getLocationCallable;
}
@Override
public final void close() {
try {
backgroundResources.close();
} catch (RuntimeException e) {
throw e;
} catch (Exception e) {
throw new IllegalStateException("Failed to close resource", e);
}
}
@Override
public void shutdown() {
backgroundResources.shutdown();
}
@Override
public boolean isShutdown() {
return backgroundResources.isShutdown();
}
@Override
public boolean isTerminated() {
return backgroundResources.isTerminated();
}
@Override
public void shutdownNow() {
backgroundResources.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return backgroundResources.awaitTermination(duration, unit);
}
}