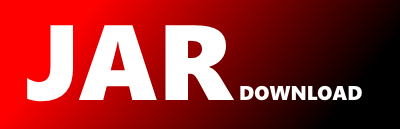
com.google.cloud.telcoautomation.v1alpha1.TelcoAutomationClient Maven / Gradle / Ivy
Show all versions of google-cloud-telcoautomation Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.telcoautomation.v1alpha1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.telcoautomation.v1alpha1.stub.TelcoAutomationStub;
import com.google.cloud.telcoautomation.v1alpha1.stub.TelcoAutomationStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.longrunning.Operation;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: TelcoAutomation Service manages the control plane cluster a.k.a.
* Orchestration Cluster (GKE cluster with config controller) of TNA. It also exposes blueprint APIs
* which manages the lifecycle of blueprints that control the infrastructure setup (e.g GDCE
* clusters) and deployment of network functions.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* OrchestrationClusterName name =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]");
* OrchestrationCluster response = telcoAutomationClient.getOrchestrationCluster(name);
* }
* }
*
* Note: close() needs to be called on the TelcoAutomationClient object to clean up resources
* such as threads. In the example above, try-with-resources is used, which automatically calls
* close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* ListOrchestrationClusters
* Lists OrchestrationClusters in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listOrchestrationClusters(ListOrchestrationClustersRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listOrchestrationClusters(LocationName parent)
*
listOrchestrationClusters(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listOrchestrationClustersPagedCallable()
*
listOrchestrationClustersCallable()
*
*
*
*
* GetOrchestrationCluster
* Gets details of a single OrchestrationCluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getOrchestrationCluster(GetOrchestrationClusterRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getOrchestrationCluster(OrchestrationClusterName name)
*
getOrchestrationCluster(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getOrchestrationClusterCallable()
*
*
*
*
* CreateOrchestrationCluster
* Creates a new OrchestrationCluster in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createOrchestrationClusterAsync(CreateOrchestrationClusterRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createOrchestrationClusterAsync(LocationName parent, OrchestrationCluster orchestrationCluster, String orchestrationClusterId)
*
createOrchestrationClusterAsync(String parent, OrchestrationCluster orchestrationCluster, String orchestrationClusterId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createOrchestrationClusterOperationCallable()
*
createOrchestrationClusterCallable()
*
*
*
*
* DeleteOrchestrationCluster
* Deletes a single OrchestrationCluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteOrchestrationClusterAsync(DeleteOrchestrationClusterRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteOrchestrationClusterAsync(OrchestrationClusterName name)
*
deleteOrchestrationClusterAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteOrchestrationClusterOperationCallable()
*
deleteOrchestrationClusterCallable()
*
*
*
*
* ListEdgeSlms
* Lists EdgeSlms in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listEdgeSlms(ListEdgeSlmsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listEdgeSlms(LocationName parent)
*
listEdgeSlms(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listEdgeSlmsPagedCallable()
*
listEdgeSlmsCallable()
*
*
*
*
* GetEdgeSlm
* Gets details of a single EdgeSlm.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getEdgeSlm(GetEdgeSlmRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getEdgeSlm(EdgeSlmName name)
*
getEdgeSlm(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getEdgeSlmCallable()
*
*
*
*
* CreateEdgeSlm
* Creates a new EdgeSlm in a given project and location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createEdgeSlmAsync(CreateEdgeSlmRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createEdgeSlmAsync(LocationName parent, EdgeSlm edgeSlm, String edgeSlmId)
*
createEdgeSlmAsync(String parent, EdgeSlm edgeSlm, String edgeSlmId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createEdgeSlmOperationCallable()
*
createEdgeSlmCallable()
*
*
*
*
* DeleteEdgeSlm
* Deletes a single EdgeSlm.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteEdgeSlmAsync(DeleteEdgeSlmRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteEdgeSlmAsync(EdgeSlmName name)
*
deleteEdgeSlmAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteEdgeSlmOperationCallable()
*
deleteEdgeSlmCallable()
*
*
*
*
* CreateBlueprint
* Creates a blueprint.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createBlueprint(CreateBlueprintRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createBlueprint(OrchestrationClusterName parent, Blueprint blueprint, String blueprintId)
*
createBlueprint(String parent, Blueprint blueprint, String blueprintId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createBlueprintCallable()
*
*
*
*
* UpdateBlueprint
* Updates a blueprint.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateBlueprint(UpdateBlueprintRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateBlueprint(Blueprint blueprint, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateBlueprintCallable()
*
*
*
*
* GetBlueprint
* Returns the requested blueprint.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getBlueprint(GetBlueprintRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getBlueprint(BlueprintName name)
*
getBlueprint(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getBlueprintCallable()
*
*
*
*
* DeleteBlueprint
* Deletes a blueprint and all its revisions.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteBlueprint(DeleteBlueprintRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteBlueprint(BlueprintName name)
*
deleteBlueprint(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteBlueprintCallable()
*
*
*
*
* ListBlueprints
* List all blueprints.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listBlueprints(ListBlueprintsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listBlueprints(OrchestrationClusterName parent)
*
listBlueprints(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listBlueprintsPagedCallable()
*
listBlueprintsCallable()
*
*
*
*
* ApproveBlueprint
* Approves a blueprint and commits a new revision.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* approveBlueprint(ApproveBlueprintRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* approveBlueprint(BlueprintName name)
*
approveBlueprint(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* approveBlueprintCallable()
*
*
*
*
* ProposeBlueprint
* Proposes a blueprint for approval of changes.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* proposeBlueprint(ProposeBlueprintRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* proposeBlueprint(BlueprintName name)
*
proposeBlueprint(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* proposeBlueprintCallable()
*
*
*
*
* RejectBlueprint
* Rejects a blueprint revision proposal and flips it back to Draft state.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* rejectBlueprint(RejectBlueprintRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* rejectBlueprint(BlueprintName name)
*
rejectBlueprint(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* rejectBlueprintCallable()
*
*
*
*
* ListBlueprintRevisions
* List blueprint revisions of a given blueprint.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listBlueprintRevisions(ListBlueprintRevisionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listBlueprintRevisions(BlueprintName name)
*
listBlueprintRevisions(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listBlueprintRevisionsPagedCallable()
*
listBlueprintRevisionsCallable()
*
*
*
*
* SearchBlueprintRevisions
* Searches across blueprint revisions.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* searchBlueprintRevisions(SearchBlueprintRevisionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* searchBlueprintRevisions(OrchestrationClusterName parent, String query)
*
searchBlueprintRevisions(String parent, String query)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* searchBlueprintRevisionsPagedCallable()
*
searchBlueprintRevisionsCallable()
*
*
*
*
* SearchDeploymentRevisions
* Searches across deployment revisions.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* searchDeploymentRevisions(SearchDeploymentRevisionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* searchDeploymentRevisions(OrchestrationClusterName parent, String query)
*
searchDeploymentRevisions(String parent, String query)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* searchDeploymentRevisionsPagedCallable()
*
searchDeploymentRevisionsCallable()
*
*
*
*
* DiscardBlueprintChanges
* Discards the changes in a blueprint and reverts the blueprint to the last approved blueprint revision. No changes take place if a blueprint does not have revisions.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* discardBlueprintChanges(DiscardBlueprintChangesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* discardBlueprintChanges(BlueprintName name)
*
discardBlueprintChanges(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* discardBlueprintChangesCallable()
*
*
*
*
* ListPublicBlueprints
* Lists the blueprints in TNA's public catalog. Default page size = 20, Max Page Size = 100.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listPublicBlueprints(ListPublicBlueprintsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listPublicBlueprints(LocationName parent)
*
listPublicBlueprints(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listPublicBlueprintsPagedCallable()
*
listPublicBlueprintsCallable()
*
*
*
*
* GetPublicBlueprint
* Returns the requested public blueprint.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getPublicBlueprint(GetPublicBlueprintRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getPublicBlueprint(PublicBlueprintName name)
*
getPublicBlueprint(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getPublicBlueprintCallable()
*
*
*
*
* CreateDeployment
* Creates a deployment.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createDeployment(CreateDeploymentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createDeployment(OrchestrationClusterName parent, Deployment deployment, String deploymentId)
*
createDeployment(String parent, Deployment deployment, String deploymentId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createDeploymentCallable()
*
*
*
*
* UpdateDeployment
* Updates a deployment.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateDeployment(UpdateDeploymentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateDeployment(Deployment deployment, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateDeploymentCallable()
*
*
*
*
* GetDeployment
* Returns the requested deployment.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getDeployment(GetDeploymentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getDeployment(DeploymentName name)
*
getDeployment(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getDeploymentCallable()
*
*
*
*
* RemoveDeployment
* Removes the deployment by marking it as DELETING. Post which deployment and it's revisions gets deleted.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* removeDeployment(RemoveDeploymentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* removeDeployment(DeploymentName name)
*
removeDeployment(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* removeDeploymentCallable()
*
*
*
*
* ListDeployments
* List all deployments.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listDeployments(ListDeploymentsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listDeployments(OrchestrationClusterName parent)
*
listDeployments(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listDeploymentsPagedCallable()
*
listDeploymentsCallable()
*
*
*
*
* ListDeploymentRevisions
* List deployment revisions of a given deployment.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listDeploymentRevisions(ListDeploymentRevisionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listDeploymentRevisions(DeploymentName name)
*
listDeploymentRevisions(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listDeploymentRevisionsPagedCallable()
*
listDeploymentRevisionsCallable()
*
*
*
*
* DiscardDeploymentChanges
* Discards the changes in a deployment and reverts the deployment to the last approved deployment revision. No changes take place if a deployment does not have revisions.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* discardDeploymentChanges(DiscardDeploymentChangesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* discardDeploymentChanges(DeploymentName name)
*
discardDeploymentChanges(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* discardDeploymentChangesCallable()
*
*
*
*
* ApplyDeployment
* Applies the deployment's YAML files to the parent orchestration cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* applyDeployment(ApplyDeploymentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* applyDeployment(DeploymentName name)
*
applyDeployment(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* applyDeploymentCallable()
*
*
*
*
* ComputeDeploymentStatus
* Returns the requested deployment status.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* computeDeploymentStatus(ComputeDeploymentStatusRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* computeDeploymentStatus(DeploymentName name)
*
computeDeploymentStatus(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* computeDeploymentStatusCallable()
*
*
*
*
* RollbackDeployment
* Rollback the active deployment to the given past approved deployment revision.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* rollbackDeployment(RollbackDeploymentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* rollbackDeployment(DeploymentName name, String revisionId)
*
rollbackDeployment(String name, String revisionId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* rollbackDeploymentCallable()
*
*
*
*
* GetHydratedDeployment
* Returns the requested hydrated deployment.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getHydratedDeployment(GetHydratedDeploymentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getHydratedDeployment(HydratedDeploymentName name)
*
getHydratedDeployment(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getHydratedDeploymentCallable()
*
*
*
*
* ListHydratedDeployments
* List all hydrated deployments present under a deployment.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listHydratedDeployments(ListHydratedDeploymentsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listHydratedDeployments(DeploymentName parent)
*
listHydratedDeployments(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listHydratedDeploymentsPagedCallable()
*
listHydratedDeploymentsCallable()
*
*
*
*
* UpdateHydratedDeployment
* Updates a hydrated deployment.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateHydratedDeployment(UpdateHydratedDeploymentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* updateHydratedDeployment(HydratedDeployment hydratedDeployment, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateHydratedDeploymentCallable()
*
*
*
*
* ApplyHydratedDeployment
* Applies a hydrated deployment to a workload cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* applyHydratedDeployment(ApplyHydratedDeploymentRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* applyHydratedDeployment(HydratedDeploymentName name)
*
applyHydratedDeployment(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* applyHydratedDeploymentCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listLocationsPagedCallable()
*
listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getLocationCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of TelcoAutomationSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* TelcoAutomationSettings telcoAutomationSettings =
* TelcoAutomationSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* TelcoAutomationClient telcoAutomationClient =
* TelcoAutomationClient.create(telcoAutomationSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* TelcoAutomationSettings telcoAutomationSettings =
* TelcoAutomationSettings.newBuilder().setEndpoint(myEndpoint).build();
* TelcoAutomationClient telcoAutomationClient =
* TelcoAutomationClient.create(telcoAutomationSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* TelcoAutomationSettings telcoAutomationSettings =
* TelcoAutomationSettings.newHttpJsonBuilder().build();
* TelcoAutomationClient telcoAutomationClient =
* TelcoAutomationClient.create(telcoAutomationSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class TelcoAutomationClient implements BackgroundResource {
private final TelcoAutomationSettings settings;
private final TelcoAutomationStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of TelcoAutomationClient with default settings. */
public static final TelcoAutomationClient create() throws IOException {
return create(TelcoAutomationSettings.newBuilder().build());
}
/**
* Constructs an instance of TelcoAutomationClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final TelcoAutomationClient create(TelcoAutomationSettings settings)
throws IOException {
return new TelcoAutomationClient(settings);
}
/**
* Constructs an instance of TelcoAutomationClient, using the given stub for making calls. This is
* for advanced usage - prefer using create(TelcoAutomationSettings).
*/
public static final TelcoAutomationClient create(TelcoAutomationStub stub) {
return new TelcoAutomationClient(stub);
}
/**
* Constructs an instance of TelcoAutomationClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected TelcoAutomationClient(TelcoAutomationSettings settings) throws IOException {
this.settings = settings;
this.stub = ((TelcoAutomationStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected TelcoAutomationClient(TelcoAutomationStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final TelcoAutomationSettings getSettings() {
return settings;
}
public TelcoAutomationStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists OrchestrationClusters in a given project and location.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (OrchestrationCluster element :
* telcoAutomationClient.listOrchestrationClusters(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListOrchestrationClustersRequest
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListOrchestrationClustersPagedResponse listOrchestrationClusters(
LocationName parent) {
ListOrchestrationClustersRequest request =
ListOrchestrationClustersRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listOrchestrationClusters(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists OrchestrationClusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (OrchestrationCluster element :
* telcoAutomationClient.listOrchestrationClusters(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListOrchestrationClustersRequest
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListOrchestrationClustersPagedResponse listOrchestrationClusters(String parent) {
ListOrchestrationClustersRequest request =
ListOrchestrationClustersRequest.newBuilder().setParent(parent).build();
return listOrchestrationClusters(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists OrchestrationClusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListOrchestrationClustersRequest request =
* ListOrchestrationClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (OrchestrationCluster element :
* telcoAutomationClient.listOrchestrationClusters(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListOrchestrationClustersPagedResponse listOrchestrationClusters(
ListOrchestrationClustersRequest request) {
return listOrchestrationClustersPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists OrchestrationClusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListOrchestrationClustersRequest request =
* ListOrchestrationClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* telcoAutomationClient.listOrchestrationClustersPagedCallable().futureCall(request);
* // Do something.
* for (OrchestrationCluster element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable<
ListOrchestrationClustersRequest, ListOrchestrationClustersPagedResponse>
listOrchestrationClustersPagedCallable() {
return stub.listOrchestrationClustersPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists OrchestrationClusters in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListOrchestrationClustersRequest request =
* ListOrchestrationClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListOrchestrationClustersResponse response =
* telcoAutomationClient.listOrchestrationClustersCallable().call(request);
* for (OrchestrationCluster element : response.getOrchestrationClustersList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listOrchestrationClustersCallable() {
return stub.listOrchestrationClustersCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single OrchestrationCluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* OrchestrationClusterName name =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]");
* OrchestrationCluster response = telcoAutomationClient.getOrchestrationCluster(name);
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OrchestrationCluster getOrchestrationCluster(OrchestrationClusterName name) {
GetOrchestrationClusterRequest request =
GetOrchestrationClusterRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getOrchestrationCluster(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single OrchestrationCluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString();
* OrchestrationCluster response = telcoAutomationClient.getOrchestrationCluster(name);
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OrchestrationCluster getOrchestrationCluster(String name) {
GetOrchestrationClusterRequest request =
GetOrchestrationClusterRequest.newBuilder().setName(name).build();
return getOrchestrationCluster(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single OrchestrationCluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetOrchestrationClusterRequest request =
* GetOrchestrationClusterRequest.newBuilder()
* .setName(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .build();
* OrchestrationCluster response = telcoAutomationClient.getOrchestrationCluster(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OrchestrationCluster getOrchestrationCluster(
GetOrchestrationClusterRequest request) {
return getOrchestrationClusterCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single OrchestrationCluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetOrchestrationClusterRequest request =
* GetOrchestrationClusterRequest.newBuilder()
* .setName(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.getOrchestrationClusterCallable().futureCall(request);
* // Do something.
* OrchestrationCluster response = future.get();
* }
* }
*/
public final UnaryCallable
getOrchestrationClusterCallable() {
return stub.getOrchestrationClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new OrchestrationCluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* OrchestrationCluster orchestrationCluster = OrchestrationCluster.newBuilder().build();
* String orchestrationClusterId = "orchestrationClusterId75469684";
* OrchestrationCluster response =
* telcoAutomationClient
* .createOrchestrationClusterAsync(parent, orchestrationCluster, orchestrationClusterId)
* .get();
* }
* }
*
* @param parent Required. Value for parent.
* @param orchestrationCluster Required. The resource being created
* @param orchestrationClusterId Required. Id of the requesting object If auto-generating Id
* server-side, remove this field and orchestration_cluster_id from the method_signature of
* Create RPC
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
createOrchestrationClusterAsync(
LocationName parent,
OrchestrationCluster orchestrationCluster,
String orchestrationClusterId) {
CreateOrchestrationClusterRequest request =
CreateOrchestrationClusterRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setOrchestrationCluster(orchestrationCluster)
.setOrchestrationClusterId(orchestrationClusterId)
.build();
return createOrchestrationClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new OrchestrationCluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* OrchestrationCluster orchestrationCluster = OrchestrationCluster.newBuilder().build();
* String orchestrationClusterId = "orchestrationClusterId75469684";
* OrchestrationCluster response =
* telcoAutomationClient
* .createOrchestrationClusterAsync(parent, orchestrationCluster, orchestrationClusterId)
* .get();
* }
* }
*
* @param parent Required. Value for parent.
* @param orchestrationCluster Required. The resource being created
* @param orchestrationClusterId Required. Id of the requesting object If auto-generating Id
* server-side, remove this field and orchestration_cluster_id from the method_signature of
* Create RPC
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
createOrchestrationClusterAsync(
String parent, OrchestrationCluster orchestrationCluster, String orchestrationClusterId) {
CreateOrchestrationClusterRequest request =
CreateOrchestrationClusterRequest.newBuilder()
.setParent(parent)
.setOrchestrationCluster(orchestrationCluster)
.setOrchestrationClusterId(orchestrationClusterId)
.build();
return createOrchestrationClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new OrchestrationCluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateOrchestrationClusterRequest request =
* CreateOrchestrationClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setOrchestrationClusterId("orchestrationClusterId75469684")
* .setOrchestrationCluster(OrchestrationCluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OrchestrationCluster response =
* telcoAutomationClient.createOrchestrationClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture
createOrchestrationClusterAsync(CreateOrchestrationClusterRequest request) {
return createOrchestrationClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new OrchestrationCluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateOrchestrationClusterRequest request =
* CreateOrchestrationClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setOrchestrationClusterId("orchestrationClusterId75469684")
* .setOrchestrationCluster(OrchestrationCluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* telcoAutomationClient.createOrchestrationClusterOperationCallable().futureCall(request);
* // Do something.
* OrchestrationCluster response = future.get();
* }
* }
*/
public final OperationCallable<
CreateOrchestrationClusterRequest, OrchestrationCluster, OperationMetadata>
createOrchestrationClusterOperationCallable() {
return stub.createOrchestrationClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new OrchestrationCluster in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateOrchestrationClusterRequest request =
* CreateOrchestrationClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setOrchestrationClusterId("orchestrationClusterId75469684")
* .setOrchestrationCluster(OrchestrationCluster.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* telcoAutomationClient.createOrchestrationClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
createOrchestrationClusterCallable() {
return stub.createOrchestrationClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single OrchestrationCluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* OrchestrationClusterName name =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]");
* telcoAutomationClient.deleteOrchestrationClusterAsync(name).get();
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteOrchestrationClusterAsync(
OrchestrationClusterName name) {
DeleteOrchestrationClusterRequest request =
DeleteOrchestrationClusterRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteOrchestrationClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single OrchestrationCluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString();
* telcoAutomationClient.deleteOrchestrationClusterAsync(name).get();
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteOrchestrationClusterAsync(
String name) {
DeleteOrchestrationClusterRequest request =
DeleteOrchestrationClusterRequest.newBuilder().setName(name).build();
return deleteOrchestrationClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single OrchestrationCluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeleteOrchestrationClusterRequest request =
* DeleteOrchestrationClusterRequest.newBuilder()
* .setName(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setRequestId("requestId693933066")
* .build();
* telcoAutomationClient.deleteOrchestrationClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteOrchestrationClusterAsync(
DeleteOrchestrationClusterRequest request) {
return deleteOrchestrationClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single OrchestrationCluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeleteOrchestrationClusterRequest request =
* DeleteOrchestrationClusterRequest.newBuilder()
* .setName(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* telcoAutomationClient.deleteOrchestrationClusterOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteOrchestrationClusterOperationCallable() {
return stub.deleteOrchestrationClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single OrchestrationCluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeleteOrchestrationClusterRequest request =
* DeleteOrchestrationClusterRequest.newBuilder()
* .setName(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* telcoAutomationClient.deleteOrchestrationClusterCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable
deleteOrchestrationClusterCallable() {
return stub.deleteOrchestrationClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists EdgeSlms in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (EdgeSlm element : telcoAutomationClient.listEdgeSlms(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListEdgeSlmsRequest
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEdgeSlmsPagedResponse listEdgeSlms(LocationName parent) {
ListEdgeSlmsRequest request =
ListEdgeSlmsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listEdgeSlms(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists EdgeSlms in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (EdgeSlm element : telcoAutomationClient.listEdgeSlms(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value for ListEdgeSlmsRequest
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEdgeSlmsPagedResponse listEdgeSlms(String parent) {
ListEdgeSlmsRequest request = ListEdgeSlmsRequest.newBuilder().setParent(parent).build();
return listEdgeSlms(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists EdgeSlms in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListEdgeSlmsRequest request =
* ListEdgeSlmsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (EdgeSlm element : telcoAutomationClient.listEdgeSlms(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListEdgeSlmsPagedResponse listEdgeSlms(ListEdgeSlmsRequest request) {
return listEdgeSlmsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists EdgeSlms in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListEdgeSlmsRequest request =
* ListEdgeSlmsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* telcoAutomationClient.listEdgeSlmsPagedCallable().futureCall(request);
* // Do something.
* for (EdgeSlm element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listEdgeSlmsPagedCallable() {
return stub.listEdgeSlmsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists EdgeSlms in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListEdgeSlmsRequest request =
* ListEdgeSlmsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListEdgeSlmsResponse response = telcoAutomationClient.listEdgeSlmsCallable().call(request);
* for (EdgeSlm element : response.getEdgeSlmsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listEdgeSlmsCallable() {
return stub.listEdgeSlmsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single EdgeSlm.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* EdgeSlmName name = EdgeSlmName.of("[PROJECT]", "[LOCATION]", "[EDGE_SLM]");
* EdgeSlm response = telcoAutomationClient.getEdgeSlm(name);
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final EdgeSlm getEdgeSlm(EdgeSlmName name) {
GetEdgeSlmRequest request =
GetEdgeSlmRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getEdgeSlm(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single EdgeSlm.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name = EdgeSlmName.of("[PROJECT]", "[LOCATION]", "[EDGE_SLM]").toString();
* EdgeSlm response = telcoAutomationClient.getEdgeSlm(name);
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final EdgeSlm getEdgeSlm(String name) {
GetEdgeSlmRequest request = GetEdgeSlmRequest.newBuilder().setName(name).build();
return getEdgeSlm(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single EdgeSlm.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetEdgeSlmRequest request =
* GetEdgeSlmRequest.newBuilder()
* .setName(EdgeSlmName.of("[PROJECT]", "[LOCATION]", "[EDGE_SLM]").toString())
* .build();
* EdgeSlm response = telcoAutomationClient.getEdgeSlm(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final EdgeSlm getEdgeSlm(GetEdgeSlmRequest request) {
return getEdgeSlmCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a single EdgeSlm.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetEdgeSlmRequest request =
* GetEdgeSlmRequest.newBuilder()
* .setName(EdgeSlmName.of("[PROJECT]", "[LOCATION]", "[EDGE_SLM]").toString())
* .build();
* ApiFuture future = telcoAutomationClient.getEdgeSlmCallable().futureCall(request);
* // Do something.
* EdgeSlm response = future.get();
* }
* }
*/
public final UnaryCallable getEdgeSlmCallable() {
return stub.getEdgeSlmCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new EdgeSlm in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* EdgeSlm edgeSlm = EdgeSlm.newBuilder().build();
* String edgeSlmId = "edgeSlmId213186994";
* EdgeSlm response = telcoAutomationClient.createEdgeSlmAsync(parent, edgeSlm, edgeSlmId).get();
* }
* }
*
* @param parent Required. Value for parent.
* @param edgeSlm Required. The resource being created
* @param edgeSlmId Required. Id of the requesting object If auto-generating Id server-side,
* remove this field and edge_slm_id from the method_signature of Create RPC
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createEdgeSlmAsync(
LocationName parent, EdgeSlm edgeSlm, String edgeSlmId) {
CreateEdgeSlmRequest request =
CreateEdgeSlmRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setEdgeSlm(edgeSlm)
.setEdgeSlmId(edgeSlmId)
.build();
return createEdgeSlmAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new EdgeSlm in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* EdgeSlm edgeSlm = EdgeSlm.newBuilder().build();
* String edgeSlmId = "edgeSlmId213186994";
* EdgeSlm response = telcoAutomationClient.createEdgeSlmAsync(parent, edgeSlm, edgeSlmId).get();
* }
* }
*
* @param parent Required. Value for parent.
* @param edgeSlm Required. The resource being created
* @param edgeSlmId Required. Id of the requesting object If auto-generating Id server-side,
* remove this field and edge_slm_id from the method_signature of Create RPC
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createEdgeSlmAsync(
String parent, EdgeSlm edgeSlm, String edgeSlmId) {
CreateEdgeSlmRequest request =
CreateEdgeSlmRequest.newBuilder()
.setParent(parent)
.setEdgeSlm(edgeSlm)
.setEdgeSlmId(edgeSlmId)
.build();
return createEdgeSlmAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new EdgeSlm in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateEdgeSlmRequest request =
* CreateEdgeSlmRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setEdgeSlmId("edgeSlmId213186994")
* .setEdgeSlm(EdgeSlm.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* EdgeSlm response = telcoAutomationClient.createEdgeSlmAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createEdgeSlmAsync(
CreateEdgeSlmRequest request) {
return createEdgeSlmOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new EdgeSlm in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateEdgeSlmRequest request =
* CreateEdgeSlmRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setEdgeSlmId("edgeSlmId213186994")
* .setEdgeSlm(EdgeSlm.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* telcoAutomationClient.createEdgeSlmOperationCallable().futureCall(request);
* // Do something.
* EdgeSlm response = future.get();
* }
* }
*/
public final OperationCallable
createEdgeSlmOperationCallable() {
return stub.createEdgeSlmOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new EdgeSlm in a given project and location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateEdgeSlmRequest request =
* CreateEdgeSlmRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setEdgeSlmId("edgeSlmId213186994")
* .setEdgeSlm(EdgeSlm.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* telcoAutomationClient.createEdgeSlmCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createEdgeSlmCallable() {
return stub.createEdgeSlmCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single EdgeSlm.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* EdgeSlmName name = EdgeSlmName.of("[PROJECT]", "[LOCATION]", "[EDGE_SLM]");
* telcoAutomationClient.deleteEdgeSlmAsync(name).get();
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteEdgeSlmAsync(EdgeSlmName name) {
DeleteEdgeSlmRequest request =
DeleteEdgeSlmRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteEdgeSlmAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single EdgeSlm.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name = EdgeSlmName.of("[PROJECT]", "[LOCATION]", "[EDGE_SLM]").toString();
* telcoAutomationClient.deleteEdgeSlmAsync(name).get();
* }
* }
*
* @param name Required. Name of the resource
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteEdgeSlmAsync(String name) {
DeleteEdgeSlmRequest request = DeleteEdgeSlmRequest.newBuilder().setName(name).build();
return deleteEdgeSlmAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single EdgeSlm.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeleteEdgeSlmRequest request =
* DeleteEdgeSlmRequest.newBuilder()
* .setName(EdgeSlmName.of("[PROJECT]", "[LOCATION]", "[EDGE_SLM]").toString())
* .setRequestId("requestId693933066")
* .build();
* telcoAutomationClient.deleteEdgeSlmAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteEdgeSlmAsync(
DeleteEdgeSlmRequest request) {
return deleteEdgeSlmOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single EdgeSlm.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeleteEdgeSlmRequest request =
* DeleteEdgeSlmRequest.newBuilder()
* .setName(EdgeSlmName.of("[PROJECT]", "[LOCATION]", "[EDGE_SLM]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* telcoAutomationClient.deleteEdgeSlmOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteEdgeSlmOperationCallable() {
return stub.deleteEdgeSlmOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a single EdgeSlm.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeleteEdgeSlmRequest request =
* DeleteEdgeSlmRequest.newBuilder()
* .setName(EdgeSlmName.of("[PROJECT]", "[LOCATION]", "[EDGE_SLM]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future =
* telcoAutomationClient.deleteEdgeSlmCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteEdgeSlmCallable() {
return stub.deleteEdgeSlmCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* OrchestrationClusterName parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]");
* Blueprint blueprint = Blueprint.newBuilder().build();
* String blueprintId = "blueprintId-1159505138";
* Blueprint response = telcoAutomationClient.createBlueprint(parent, blueprint, blueprintId);
* }
* }
*
* @param parent Required. The name of parent resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @param blueprint Required. The `Blueprint` to create.
* @param blueprintId Optional. The name of the blueprint.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint createBlueprint(
OrchestrationClusterName parent, Blueprint blueprint, String blueprintId) {
CreateBlueprintRequest request =
CreateBlueprintRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setBlueprint(blueprint)
.setBlueprintId(blueprintId)
.build();
return createBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString();
* Blueprint blueprint = Blueprint.newBuilder().build();
* String blueprintId = "blueprintId-1159505138";
* Blueprint response = telcoAutomationClient.createBlueprint(parent, blueprint, blueprintId);
* }
* }
*
* @param parent Required. The name of parent resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @param blueprint Required. The `Blueprint` to create.
* @param blueprintId Optional. The name of the blueprint.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint createBlueprint(String parent, Blueprint blueprint, String blueprintId) {
CreateBlueprintRequest request =
CreateBlueprintRequest.newBuilder()
.setParent(parent)
.setBlueprint(blueprint)
.setBlueprintId(blueprintId)
.build();
return createBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateBlueprintRequest request =
* CreateBlueprintRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setBlueprintId("blueprintId-1159505138")
* .setBlueprint(Blueprint.newBuilder().build())
* .build();
* Blueprint response = telcoAutomationClient.createBlueprint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint createBlueprint(CreateBlueprintRequest request) {
return createBlueprintCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateBlueprintRequest request =
* CreateBlueprintRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setBlueprintId("blueprintId-1159505138")
* .setBlueprint(Blueprint.newBuilder().build())
* .build();
* ApiFuture future =
* telcoAutomationClient.createBlueprintCallable().futureCall(request);
* // Do something.
* Blueprint response = future.get();
* }
* }
*/
public final UnaryCallable createBlueprintCallable() {
return stub.createBlueprintCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* Blueprint blueprint = Blueprint.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Blueprint response = telcoAutomationClient.updateBlueprint(blueprint, updateMask);
* }
* }
*
* @param blueprint Required. The `blueprint` to update.
* @param updateMask Required. Update mask is used to specify the fields to be overwritten in the
* `blueprint` resource by the update.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint updateBlueprint(Blueprint blueprint, FieldMask updateMask) {
UpdateBlueprintRequest request =
UpdateBlueprintRequest.newBuilder()
.setBlueprint(blueprint)
.setUpdateMask(updateMask)
.build();
return updateBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* UpdateBlueprintRequest request =
* UpdateBlueprintRequest.newBuilder()
* .setBlueprint(Blueprint.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Blueprint response = telcoAutomationClient.updateBlueprint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint updateBlueprint(UpdateBlueprintRequest request) {
return updateBlueprintCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* UpdateBlueprintRequest request =
* UpdateBlueprintRequest.newBuilder()
* .setBlueprint(Blueprint.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* telcoAutomationClient.updateBlueprintCallable().futureCall(request);
* // Do something.
* Blueprint response = future.get();
* }
* }
*/
public final UnaryCallable updateBlueprintCallable() {
return stub.updateBlueprintCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* BlueprintName name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]");
* Blueprint response = telcoAutomationClient.getBlueprint(name);
* }
* }
*
* @param name Required. The name of the blueprint. Case 1: If the name provided in the request is
* {blueprint_id}{@literal @}{revision_id}, then the revision with revision_id will be
* returned. Case 2: If the name provided in the request is {blueprint}, then the current
* state of the blueprint is returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint getBlueprint(BlueprintName name) {
GetBlueprintRequest request =
GetBlueprintRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString();
* Blueprint response = telcoAutomationClient.getBlueprint(name);
* }
* }
*
* @param name Required. The name of the blueprint. Case 1: If the name provided in the request is
* {blueprint_id}{@literal @}{revision_id}, then the revision with revision_id will be
* returned. Case 2: If the name provided in the request is {blueprint}, then the current
* state of the blueprint is returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint getBlueprint(String name) {
GetBlueprintRequest request = GetBlueprintRequest.newBuilder().setName(name).build();
return getBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetBlueprintRequest request =
* GetBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .setView(BlueprintView.forNumber(0))
* .build();
* Blueprint response = telcoAutomationClient.getBlueprint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint getBlueprint(GetBlueprintRequest request) {
return getBlueprintCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetBlueprintRequest request =
* GetBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .setView(BlueprintView.forNumber(0))
* .build();
* ApiFuture future =
* telcoAutomationClient.getBlueprintCallable().futureCall(request);
* // Do something.
* Blueprint response = future.get();
* }
* }
*/
public final UnaryCallable getBlueprintCallable() {
return stub.getBlueprintCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a blueprint and all its revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* BlueprintName name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]");
* telcoAutomationClient.deleteBlueprint(name);
* }
* }
*
* @param name Required. The name of blueprint to delete. Blueprint name should be in the format
* {blueprint_id}, if {blueprint_id}{@literal @}{revision_id} is passed then the API throws
* invalid argument.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteBlueprint(BlueprintName name) {
DeleteBlueprintRequest request =
DeleteBlueprintRequest.newBuilder().setName(name == null ? null : name.toString()).build();
deleteBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a blueprint and all its revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString();
* telcoAutomationClient.deleteBlueprint(name);
* }
* }
*
* @param name Required. The name of blueprint to delete. Blueprint name should be in the format
* {blueprint_id}, if {blueprint_id}{@literal @}{revision_id} is passed then the API throws
* invalid argument.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteBlueprint(String name) {
DeleteBlueprintRequest request = DeleteBlueprintRequest.newBuilder().setName(name).build();
deleteBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a blueprint and all its revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeleteBlueprintRequest request =
* DeleteBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* telcoAutomationClient.deleteBlueprint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteBlueprint(DeleteBlueprintRequest request) {
deleteBlueprintCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a blueprint and all its revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeleteBlueprintRequest request =
* DeleteBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* ApiFuture future = telcoAutomationClient.deleteBlueprintCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteBlueprintCallable() {
return stub.deleteBlueprintCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all blueprints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* OrchestrationClusterName parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]");
* for (Blueprint element : telcoAutomationClient.listBlueprints(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of parent orchestration cluster resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBlueprintsPagedResponse listBlueprints(OrchestrationClusterName parent) {
ListBlueprintsRequest request =
ListBlueprintsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listBlueprints(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all blueprints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString();
* for (Blueprint element : telcoAutomationClient.listBlueprints(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of parent orchestration cluster resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBlueprintsPagedResponse listBlueprints(String parent) {
ListBlueprintsRequest request = ListBlueprintsRequest.newBuilder().setParent(parent).build();
return listBlueprints(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all blueprints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListBlueprintsRequest request =
* ListBlueprintsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Blueprint element : telcoAutomationClient.listBlueprints(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBlueprintsPagedResponse listBlueprints(ListBlueprintsRequest request) {
return listBlueprintsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all blueprints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListBlueprintsRequest request =
* ListBlueprintsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* telcoAutomationClient.listBlueprintsPagedCallable().futureCall(request);
* // Do something.
* for (Blueprint element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listBlueprintsPagedCallable() {
return stub.listBlueprintsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all blueprints.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListBlueprintsRequest request =
* ListBlueprintsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListBlueprintsResponse response =
* telcoAutomationClient.listBlueprintsCallable().call(request);
* for (Blueprint element : response.getBlueprintsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listBlueprintsCallable() {
return stub.listBlueprintsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Approves a blueprint and commits a new revision.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* BlueprintName name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]");
* Blueprint response = telcoAutomationClient.approveBlueprint(name);
* }
* }
*
* @param name Required. The name of the blueprint to approve. The blueprint must be in Proposed
* state. A new revision is committed on approval.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint approveBlueprint(BlueprintName name) {
ApproveBlueprintRequest request =
ApproveBlueprintRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return approveBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Approves a blueprint and commits a new revision.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString();
* Blueprint response = telcoAutomationClient.approveBlueprint(name);
* }
* }
*
* @param name Required. The name of the blueprint to approve. The blueprint must be in Proposed
* state. A new revision is committed on approval.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint approveBlueprint(String name) {
ApproveBlueprintRequest request = ApproveBlueprintRequest.newBuilder().setName(name).build();
return approveBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Approves a blueprint and commits a new revision.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ApproveBlueprintRequest request =
* ApproveBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* Blueprint response = telcoAutomationClient.approveBlueprint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint approveBlueprint(ApproveBlueprintRequest request) {
return approveBlueprintCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Approves a blueprint and commits a new revision.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ApproveBlueprintRequest request =
* ApproveBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.approveBlueprintCallable().futureCall(request);
* // Do something.
* Blueprint response = future.get();
* }
* }
*/
public final UnaryCallable approveBlueprintCallable() {
return stub.approveBlueprintCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Proposes a blueprint for approval of changes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* BlueprintName name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]");
* Blueprint response = telcoAutomationClient.proposeBlueprint(name);
* }
* }
*
* @param name Required. The name of the blueprint being proposed.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint proposeBlueprint(BlueprintName name) {
ProposeBlueprintRequest request =
ProposeBlueprintRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return proposeBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Proposes a blueprint for approval of changes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString();
* Blueprint response = telcoAutomationClient.proposeBlueprint(name);
* }
* }
*
* @param name Required. The name of the blueprint being proposed.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint proposeBlueprint(String name) {
ProposeBlueprintRequest request = ProposeBlueprintRequest.newBuilder().setName(name).build();
return proposeBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Proposes a blueprint for approval of changes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ProposeBlueprintRequest request =
* ProposeBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* Blueprint response = telcoAutomationClient.proposeBlueprint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint proposeBlueprint(ProposeBlueprintRequest request) {
return proposeBlueprintCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Proposes a blueprint for approval of changes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ProposeBlueprintRequest request =
* ProposeBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.proposeBlueprintCallable().futureCall(request);
* // Do something.
* Blueprint response = future.get();
* }
* }
*/
public final UnaryCallable proposeBlueprintCallable() {
return stub.proposeBlueprintCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a blueprint revision proposal and flips it back to Draft state.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* BlueprintName name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]");
* Blueprint response = telcoAutomationClient.rejectBlueprint(name);
* }
* }
*
* @param name Required. The name of the blueprint being rejected.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint rejectBlueprint(BlueprintName name) {
RejectBlueprintRequest request =
RejectBlueprintRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return rejectBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a blueprint revision proposal and flips it back to Draft state.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString();
* Blueprint response = telcoAutomationClient.rejectBlueprint(name);
* }
* }
*
* @param name Required. The name of the blueprint being rejected.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint rejectBlueprint(String name) {
RejectBlueprintRequest request = RejectBlueprintRequest.newBuilder().setName(name).build();
return rejectBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a blueprint revision proposal and flips it back to Draft state.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* RejectBlueprintRequest request =
* RejectBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* Blueprint response = telcoAutomationClient.rejectBlueprint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Blueprint rejectBlueprint(RejectBlueprintRequest request) {
return rejectBlueprintCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rejects a blueprint revision proposal and flips it back to Draft state.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* RejectBlueprintRequest request =
* RejectBlueprintRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.rejectBlueprintCallable().futureCall(request);
* // Do something.
* Blueprint response = future.get();
* }
* }
*/
public final UnaryCallable rejectBlueprintCallable() {
return stub.rejectBlueprintCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List blueprint revisions of a given blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* BlueprintName name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]");
* for (Blueprint element : telcoAutomationClient.listBlueprintRevisions(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The name of the blueprint to list revisions for.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBlueprintRevisionsPagedResponse listBlueprintRevisions(BlueprintName name) {
ListBlueprintRevisionsRequest request =
ListBlueprintRevisionsRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return listBlueprintRevisions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List blueprint revisions of a given blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString();
* for (Blueprint element : telcoAutomationClient.listBlueprintRevisions(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The name of the blueprint to list revisions for.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBlueprintRevisionsPagedResponse listBlueprintRevisions(String name) {
ListBlueprintRevisionsRequest request =
ListBlueprintRevisionsRequest.newBuilder().setName(name).build();
return listBlueprintRevisions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List blueprint revisions of a given blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListBlueprintRevisionsRequest request =
* ListBlueprintRevisionsRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Blueprint element : telcoAutomationClient.listBlueprintRevisions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListBlueprintRevisionsPagedResponse listBlueprintRevisions(
ListBlueprintRevisionsRequest request) {
return listBlueprintRevisionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List blueprint revisions of a given blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListBlueprintRevisionsRequest request =
* ListBlueprintRevisionsRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* telcoAutomationClient.listBlueprintRevisionsPagedCallable().futureCall(request);
* // Do something.
* for (Blueprint element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listBlueprintRevisionsPagedCallable() {
return stub.listBlueprintRevisionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List blueprint revisions of a given blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListBlueprintRevisionsRequest request =
* ListBlueprintRevisionsRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListBlueprintRevisionsResponse response =
* telcoAutomationClient.listBlueprintRevisionsCallable().call(request);
* for (Blueprint element : response.getBlueprintsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listBlueprintRevisionsCallable() {
return stub.listBlueprintRevisionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across blueprint revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* OrchestrationClusterName parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]");
* String query = "query107944136";
* for (Blueprint element :
* telcoAutomationClient.searchBlueprintRevisions(parent, query).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of parent orchestration cluster resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @param query Required. Supported queries: 1. "" : Lists all revisions across all blueprints. 2.
* "latest=true" : Lists latest revisions across all blueprints. 3. "name={name}" : Lists all
* revisions of blueprint with name {name}. 4. "name={name} latest=true": Lists latest
* revision of blueprint with name {name}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SearchBlueprintRevisionsPagedResponse searchBlueprintRevisions(
OrchestrationClusterName parent, String query) {
SearchBlueprintRevisionsRequest request =
SearchBlueprintRevisionsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setQuery(query)
.build();
return searchBlueprintRevisions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across blueprint revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString();
* String query = "query107944136";
* for (Blueprint element :
* telcoAutomationClient.searchBlueprintRevisions(parent, query).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of parent orchestration cluster resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @param query Required. Supported queries: 1. "" : Lists all revisions across all blueprints. 2.
* "latest=true" : Lists latest revisions across all blueprints. 3. "name={name}" : Lists all
* revisions of blueprint with name {name}. 4. "name={name} latest=true": Lists latest
* revision of blueprint with name {name}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SearchBlueprintRevisionsPagedResponse searchBlueprintRevisions(
String parent, String query) {
SearchBlueprintRevisionsRequest request =
SearchBlueprintRevisionsRequest.newBuilder().setParent(parent).setQuery(query).build();
return searchBlueprintRevisions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across blueprint revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* SearchBlueprintRevisionsRequest request =
* SearchBlueprintRevisionsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setQuery("query107944136")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Blueprint element :
* telcoAutomationClient.searchBlueprintRevisions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SearchBlueprintRevisionsPagedResponse searchBlueprintRevisions(
SearchBlueprintRevisionsRequest request) {
return searchBlueprintRevisionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across blueprint revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* SearchBlueprintRevisionsRequest request =
* SearchBlueprintRevisionsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setQuery("query107944136")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* telcoAutomationClient.searchBlueprintRevisionsPagedCallable().futureCall(request);
* // Do something.
* for (Blueprint element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
searchBlueprintRevisionsPagedCallable() {
return stub.searchBlueprintRevisionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across blueprint revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* SearchBlueprintRevisionsRequest request =
* SearchBlueprintRevisionsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setQuery("query107944136")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* SearchBlueprintRevisionsResponse response =
* telcoAutomationClient.searchBlueprintRevisionsCallable().call(request);
* for (Blueprint element : response.getBlueprintsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
searchBlueprintRevisionsCallable() {
return stub.searchBlueprintRevisionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across deployment revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* OrchestrationClusterName parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]");
* String query = "query107944136";
* for (Deployment element :
* telcoAutomationClient.searchDeploymentRevisions(parent, query).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of parent orchestration cluster resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @param query Required. Supported queries: 1. "" : Lists all revisions across all deployments.
* 2. "latest=true" : Lists latest revisions across all deployments. 3. "name={name}" : Lists
* all revisions of deployment with name {name}. 4. "name={name} latest=true": Lists latest
* revision of deployment with name {name}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SearchDeploymentRevisionsPagedResponse searchDeploymentRevisions(
OrchestrationClusterName parent, String query) {
SearchDeploymentRevisionsRequest request =
SearchDeploymentRevisionsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setQuery(query)
.build();
return searchDeploymentRevisions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across deployment revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString();
* String query = "query107944136";
* for (Deployment element :
* telcoAutomationClient.searchDeploymentRevisions(parent, query).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of parent orchestration cluster resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @param query Required. Supported queries: 1. "" : Lists all revisions across all deployments.
* 2. "latest=true" : Lists latest revisions across all deployments. 3. "name={name}" : Lists
* all revisions of deployment with name {name}. 4. "name={name} latest=true": Lists latest
* revision of deployment with name {name}
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SearchDeploymentRevisionsPagedResponse searchDeploymentRevisions(
String parent, String query) {
SearchDeploymentRevisionsRequest request =
SearchDeploymentRevisionsRequest.newBuilder().setParent(parent).setQuery(query).build();
return searchDeploymentRevisions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across deployment revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* SearchDeploymentRevisionsRequest request =
* SearchDeploymentRevisionsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setQuery("query107944136")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Deployment element :
* telcoAutomationClient.searchDeploymentRevisions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final SearchDeploymentRevisionsPagedResponse searchDeploymentRevisions(
SearchDeploymentRevisionsRequest request) {
return searchDeploymentRevisionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across deployment revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* SearchDeploymentRevisionsRequest request =
* SearchDeploymentRevisionsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setQuery("query107944136")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* telcoAutomationClient.searchDeploymentRevisionsPagedCallable().futureCall(request);
* // Do something.
* for (Deployment element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable<
SearchDeploymentRevisionsRequest, SearchDeploymentRevisionsPagedResponse>
searchDeploymentRevisionsPagedCallable() {
return stub.searchDeploymentRevisionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Searches across deployment revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* SearchDeploymentRevisionsRequest request =
* SearchDeploymentRevisionsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setQuery("query107944136")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* SearchDeploymentRevisionsResponse response =
* telcoAutomationClient.searchDeploymentRevisionsCallable().call(request);
* for (Deployment element : response.getDeploymentsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
searchDeploymentRevisionsCallable() {
return stub.searchDeploymentRevisionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Discards the changes in a blueprint and reverts the blueprint to the last approved blueprint
* revision. No changes take place if a blueprint does not have revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* BlueprintName name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]");
* DiscardBlueprintChangesResponse response =
* telcoAutomationClient.discardBlueprintChanges(name);
* }
* }
*
* @param name Required. The name of the blueprint of which changes are being discarded.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DiscardBlueprintChangesResponse discardBlueprintChanges(BlueprintName name) {
DiscardBlueprintChangesRequest request =
DiscardBlueprintChangesRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return discardBlueprintChanges(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Discards the changes in a blueprint and reverts the blueprint to the last approved blueprint
* revision. No changes take place if a blueprint does not have revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* BlueprintName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString();
* DiscardBlueprintChangesResponse response =
* telcoAutomationClient.discardBlueprintChanges(name);
* }
* }
*
* @param name Required. The name of the blueprint of which changes are being discarded.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DiscardBlueprintChangesResponse discardBlueprintChanges(String name) {
DiscardBlueprintChangesRequest request =
DiscardBlueprintChangesRequest.newBuilder().setName(name).build();
return discardBlueprintChanges(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Discards the changes in a blueprint and reverts the blueprint to the last approved blueprint
* revision. No changes take place if a blueprint does not have revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DiscardBlueprintChangesRequest request =
* DiscardBlueprintChangesRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* DiscardBlueprintChangesResponse response =
* telcoAutomationClient.discardBlueprintChanges(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DiscardBlueprintChangesResponse discardBlueprintChanges(
DiscardBlueprintChangesRequest request) {
return discardBlueprintChangesCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Discards the changes in a blueprint and reverts the blueprint to the last approved blueprint
* revision. No changes take place if a blueprint does not have revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DiscardBlueprintChangesRequest request =
* DiscardBlueprintChangesRequest.newBuilder()
* .setName(
* BlueprintName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[BLUEPRINT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.discardBlueprintChangesCallable().futureCall(request);
* // Do something.
* DiscardBlueprintChangesResponse response = future.get();
* }
* }
*/
public final UnaryCallable
discardBlueprintChangesCallable() {
return stub.discardBlueprintChangesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the blueprints in TNA's public catalog. Default page size = 20, Max Page Size = 100.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (PublicBlueprint element :
* telcoAutomationClient.listPublicBlueprints(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value of public blueprint. Format should be -
* "projects/{project_id}/locations/{location_name}".
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListPublicBlueprintsPagedResponse listPublicBlueprints(LocationName parent) {
ListPublicBlueprintsRequest request =
ListPublicBlueprintsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listPublicBlueprints(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the blueprints in TNA's public catalog. Default page size = 20, Max Page Size = 100.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (PublicBlueprint element :
* telcoAutomationClient.listPublicBlueprints(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent value of public blueprint. Format should be -
* "projects/{project_id}/locations/{location_name}".
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListPublicBlueprintsPagedResponse listPublicBlueprints(String parent) {
ListPublicBlueprintsRequest request =
ListPublicBlueprintsRequest.newBuilder().setParent(parent).build();
return listPublicBlueprints(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the blueprints in TNA's public catalog. Default page size = 20, Max Page Size = 100.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListPublicBlueprintsRequest request =
* ListPublicBlueprintsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (PublicBlueprint element :
* telcoAutomationClient.listPublicBlueprints(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListPublicBlueprintsPagedResponse listPublicBlueprints(
ListPublicBlueprintsRequest request) {
return listPublicBlueprintsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the blueprints in TNA's public catalog. Default page size = 20, Max Page Size = 100.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListPublicBlueprintsRequest request =
* ListPublicBlueprintsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* telcoAutomationClient.listPublicBlueprintsPagedCallable().futureCall(request);
* // Do something.
* for (PublicBlueprint element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listPublicBlueprintsPagedCallable() {
return stub.listPublicBlueprintsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists the blueprints in TNA's public catalog. Default page size = 20, Max Page Size = 100.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListPublicBlueprintsRequest request =
* ListPublicBlueprintsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListPublicBlueprintsResponse response =
* telcoAutomationClient.listPublicBlueprintsCallable().call(request);
* for (PublicBlueprint element : response.getPublicBlueprintsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listPublicBlueprintsCallable() {
return stub.listPublicBlueprintsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested public blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* PublicBlueprintName name =
* PublicBlueprintName.of("[PROJECT]", "[LOCATION]", "[PUBLIC_LUEPRINT]");
* PublicBlueprint response = telcoAutomationClient.getPublicBlueprint(name);
* }
* }
*
* @param name Required. The name of the public blueprint.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final PublicBlueprint getPublicBlueprint(PublicBlueprintName name) {
GetPublicBlueprintRequest request =
GetPublicBlueprintRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getPublicBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested public blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* PublicBlueprintName.of("[PROJECT]", "[LOCATION]", "[PUBLIC_LUEPRINT]").toString();
* PublicBlueprint response = telcoAutomationClient.getPublicBlueprint(name);
* }
* }
*
* @param name Required. The name of the public blueprint.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final PublicBlueprint getPublicBlueprint(String name) {
GetPublicBlueprintRequest request =
GetPublicBlueprintRequest.newBuilder().setName(name).build();
return getPublicBlueprint(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested public blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetPublicBlueprintRequest request =
* GetPublicBlueprintRequest.newBuilder()
* .setName(
* PublicBlueprintName.of("[PROJECT]", "[LOCATION]", "[PUBLIC_LUEPRINT]").toString())
* .build();
* PublicBlueprint response = telcoAutomationClient.getPublicBlueprint(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final PublicBlueprint getPublicBlueprint(GetPublicBlueprintRequest request) {
return getPublicBlueprintCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested public blueprint.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetPublicBlueprintRequest request =
* GetPublicBlueprintRequest.newBuilder()
* .setName(
* PublicBlueprintName.of("[PROJECT]", "[LOCATION]", "[PUBLIC_LUEPRINT]").toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.getPublicBlueprintCallable().futureCall(request);
* // Do something.
* PublicBlueprint response = future.get();
* }
* }
*/
public final UnaryCallable
getPublicBlueprintCallable() {
return stub.getPublicBlueprintCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* OrchestrationClusterName parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]");
* Deployment deployment = Deployment.newBuilder().build();
* String deploymentId = "deploymentId-136894784";
* Deployment response =
* telcoAutomationClient.createDeployment(parent, deployment, deploymentId);
* }
* }
*
* @param parent Required. The name of parent resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @param deployment Required. The `Deployment` to create.
* @param deploymentId Optional. The name of the deployment.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment createDeployment(
OrchestrationClusterName parent, Deployment deployment, String deploymentId) {
CreateDeploymentRequest request =
CreateDeploymentRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setDeployment(deployment)
.setDeploymentId(deploymentId)
.build();
return createDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString();
* Deployment deployment = Deployment.newBuilder().build();
* String deploymentId = "deploymentId-136894784";
* Deployment response =
* telcoAutomationClient.createDeployment(parent, deployment, deploymentId);
* }
* }
*
* @param parent Required. The name of parent resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @param deployment Required. The `Deployment` to create.
* @param deploymentId Optional. The name of the deployment.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment createDeployment(
String parent, Deployment deployment, String deploymentId) {
CreateDeploymentRequest request =
CreateDeploymentRequest.newBuilder()
.setParent(parent)
.setDeployment(deployment)
.setDeploymentId(deploymentId)
.build();
return createDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateDeploymentRequest request =
* CreateDeploymentRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setDeploymentId("deploymentId-136894784")
* .setDeployment(Deployment.newBuilder().build())
* .build();
* Deployment response = telcoAutomationClient.createDeployment(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment createDeployment(CreateDeploymentRequest request) {
return createDeploymentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* CreateDeploymentRequest request =
* CreateDeploymentRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setDeploymentId("deploymentId-136894784")
* .setDeployment(Deployment.newBuilder().build())
* .build();
* ApiFuture future =
* telcoAutomationClient.createDeploymentCallable().futureCall(request);
* // Do something.
* Deployment response = future.get();
* }
* }
*/
public final UnaryCallable createDeploymentCallable() {
return stub.createDeploymentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* Deployment deployment = Deployment.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Deployment response = telcoAutomationClient.updateDeployment(deployment, updateMask);
* }
* }
*
* @param deployment Required. The `deployment` to update.
* @param updateMask Required. Update mask is used to specify the fields to be overwritten in the
* `deployment` resource by the update.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment updateDeployment(Deployment deployment, FieldMask updateMask) {
UpdateDeploymentRequest request =
UpdateDeploymentRequest.newBuilder()
.setDeployment(deployment)
.setUpdateMask(updateMask)
.build();
return updateDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* UpdateDeploymentRequest request =
* UpdateDeploymentRequest.newBuilder()
* .setDeployment(Deployment.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Deployment response = telcoAutomationClient.updateDeployment(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment updateDeployment(UpdateDeploymentRequest request) {
return updateDeploymentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* UpdateDeploymentRequest request =
* UpdateDeploymentRequest.newBuilder()
* .setDeployment(Deployment.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* telcoAutomationClient.updateDeploymentCallable().futureCall(request);
* // Do something.
* Deployment response = future.get();
* }
* }
*/
public final UnaryCallable updateDeploymentCallable() {
return stub.updateDeploymentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeploymentName name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]");
* Deployment response = telcoAutomationClient.getDeployment(name);
* }
* }
*
* @param name Required. The name of the deployment. Case 1: If the name provided in the request
* is {deployment_id}{@literal @}{revision_id}, then the revision with revision_id will be
* returned. Case 2: If the name provided in the request is {deployment}, then the current
* state of the deployment is returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment getDeployment(DeploymentName name) {
GetDeploymentRequest request =
GetDeploymentRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString();
* Deployment response = telcoAutomationClient.getDeployment(name);
* }
* }
*
* @param name Required. The name of the deployment. Case 1: If the name provided in the request
* is {deployment_id}{@literal @}{revision_id}, then the revision with revision_id will be
* returned. Case 2: If the name provided in the request is {deployment}, then the current
* state of the deployment is returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment getDeployment(String name) {
GetDeploymentRequest request = GetDeploymentRequest.newBuilder().setName(name).build();
return getDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetDeploymentRequest request =
* GetDeploymentRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setView(DeploymentView.forNumber(0))
* .build();
* Deployment response = telcoAutomationClient.getDeployment(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment getDeployment(GetDeploymentRequest request) {
return getDeploymentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetDeploymentRequest request =
* GetDeploymentRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setView(DeploymentView.forNumber(0))
* .build();
* ApiFuture future =
* telcoAutomationClient.getDeploymentCallable().futureCall(request);
* // Do something.
* Deployment response = future.get();
* }
* }
*/
public final UnaryCallable getDeploymentCallable() {
return stub.getDeploymentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Removes the deployment by marking it as DELETING. Post which deployment and it's revisions gets
* deleted.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeploymentName name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]");
* telcoAutomationClient.removeDeployment(name);
* }
* }
*
* @param name Required. The name of deployment to initiate delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void removeDeployment(DeploymentName name) {
RemoveDeploymentRequest request =
RemoveDeploymentRequest.newBuilder().setName(name == null ? null : name.toString()).build();
removeDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Removes the deployment by marking it as DELETING. Post which deployment and it's revisions gets
* deleted.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString();
* telcoAutomationClient.removeDeployment(name);
* }
* }
*
* @param name Required. The name of deployment to initiate delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void removeDeployment(String name) {
RemoveDeploymentRequest request = RemoveDeploymentRequest.newBuilder().setName(name).build();
removeDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Removes the deployment by marking it as DELETING. Post which deployment and it's revisions gets
* deleted.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* RemoveDeploymentRequest request =
* RemoveDeploymentRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .build();
* telcoAutomationClient.removeDeployment(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void removeDeployment(RemoveDeploymentRequest request) {
removeDeploymentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Removes the deployment by marking it as DELETING. Post which deployment and it's revisions gets
* deleted.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* RemoveDeploymentRequest request =
* RemoveDeploymentRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.removeDeploymentCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable removeDeploymentCallable() {
return stub.removeDeploymentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all deployments.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* OrchestrationClusterName parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]");
* for (Deployment element : telcoAutomationClient.listDeployments(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of parent orchestration cluster resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDeploymentsPagedResponse listDeployments(OrchestrationClusterName parent) {
ListDeploymentsRequest request =
ListDeploymentsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listDeployments(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all deployments.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent =
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString();
* for (Deployment element : telcoAutomationClient.listDeployments(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The name of parent orchestration cluster resource. Format should be -
* "projects/{project_id}/locations/{location_name}/orchestrationClusters/{orchestration_cluster}".
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDeploymentsPagedResponse listDeployments(String parent) {
ListDeploymentsRequest request = ListDeploymentsRequest.newBuilder().setParent(parent).build();
return listDeployments(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all deployments.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListDeploymentsRequest request =
* ListDeploymentsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Deployment element : telcoAutomationClient.listDeployments(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDeploymentsPagedResponse listDeployments(ListDeploymentsRequest request) {
return listDeploymentsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all deployments.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListDeploymentsRequest request =
* ListDeploymentsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* telcoAutomationClient.listDeploymentsPagedCallable().futureCall(request);
* // Do something.
* for (Deployment element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listDeploymentsPagedCallable() {
return stub.listDeploymentsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all deployments.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListDeploymentsRequest request =
* ListDeploymentsRequest.newBuilder()
* .setParent(
* OrchestrationClusterName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]")
* .toString())
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListDeploymentsResponse response =
* telcoAutomationClient.listDeploymentsCallable().call(request);
* for (Deployment element : response.getDeploymentsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listDeploymentsCallable() {
return stub.listDeploymentsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List deployment revisions of a given deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeploymentName name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]");
* for (Deployment element : telcoAutomationClient.listDeploymentRevisions(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The name of the deployment to list revisions for.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDeploymentRevisionsPagedResponse listDeploymentRevisions(DeploymentName name) {
ListDeploymentRevisionsRequest request =
ListDeploymentRevisionsRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return listDeploymentRevisions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List deployment revisions of a given deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString();
* for (Deployment element : telcoAutomationClient.listDeploymentRevisions(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The name of the deployment to list revisions for.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDeploymentRevisionsPagedResponse listDeploymentRevisions(String name) {
ListDeploymentRevisionsRequest request =
ListDeploymentRevisionsRequest.newBuilder().setName(name).build();
return listDeploymentRevisions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List deployment revisions of a given deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListDeploymentRevisionsRequest request =
* ListDeploymentRevisionsRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Deployment element :
* telcoAutomationClient.listDeploymentRevisions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListDeploymentRevisionsPagedResponse listDeploymentRevisions(
ListDeploymentRevisionsRequest request) {
return listDeploymentRevisionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List deployment revisions of a given deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListDeploymentRevisionsRequest request =
* ListDeploymentRevisionsRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* telcoAutomationClient.listDeploymentRevisionsPagedCallable().futureCall(request);
* // Do something.
* for (Deployment element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listDeploymentRevisionsPagedCallable() {
return stub.listDeploymentRevisionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List deployment revisions of a given deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListDeploymentRevisionsRequest request =
* ListDeploymentRevisionsRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListDeploymentRevisionsResponse response =
* telcoAutomationClient.listDeploymentRevisionsCallable().call(request);
* for (Deployment element : response.getDeploymentsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listDeploymentRevisionsCallable() {
return stub.listDeploymentRevisionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Discards the changes in a deployment and reverts the deployment to the last approved deployment
* revision. No changes take place if a deployment does not have revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeploymentName name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]");
* DiscardDeploymentChangesResponse response =
* telcoAutomationClient.discardDeploymentChanges(name);
* }
* }
*
* @param name Required. The name of the deployment of which changes are being discarded.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DiscardDeploymentChangesResponse discardDeploymentChanges(DeploymentName name) {
DiscardDeploymentChangesRequest request =
DiscardDeploymentChangesRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return discardDeploymentChanges(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Discards the changes in a deployment and reverts the deployment to the last approved deployment
* revision. No changes take place if a deployment does not have revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString();
* DiscardDeploymentChangesResponse response =
* telcoAutomationClient.discardDeploymentChanges(name);
* }
* }
*
* @param name Required. The name of the deployment of which changes are being discarded.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DiscardDeploymentChangesResponse discardDeploymentChanges(String name) {
DiscardDeploymentChangesRequest request =
DiscardDeploymentChangesRequest.newBuilder().setName(name).build();
return discardDeploymentChanges(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Discards the changes in a deployment and reverts the deployment to the last approved deployment
* revision. No changes take place if a deployment does not have revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DiscardDeploymentChangesRequest request =
* DiscardDeploymentChangesRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .build();
* DiscardDeploymentChangesResponse response =
* telcoAutomationClient.discardDeploymentChanges(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final DiscardDeploymentChangesResponse discardDeploymentChanges(
DiscardDeploymentChangesRequest request) {
return discardDeploymentChangesCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Discards the changes in a deployment and reverts the deployment to the last approved deployment
* revision. No changes take place if a deployment does not have revisions.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DiscardDeploymentChangesRequest request =
* DiscardDeploymentChangesRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.discardDeploymentChangesCallable().futureCall(request);
* // Do something.
* DiscardDeploymentChangesResponse response = future.get();
* }
* }
*/
public final UnaryCallable
discardDeploymentChangesCallable() {
return stub.discardDeploymentChangesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Applies the deployment's YAML files to the parent orchestration cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeploymentName name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]");
* Deployment response = telcoAutomationClient.applyDeployment(name);
* }
* }
*
* @param name Required. The name of the deployment to apply to orchestration cluster.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment applyDeployment(DeploymentName name) {
ApplyDeploymentRequest request =
ApplyDeploymentRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return applyDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Applies the deployment's YAML files to the parent orchestration cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString();
* Deployment response = telcoAutomationClient.applyDeployment(name);
* }
* }
*
* @param name Required. The name of the deployment to apply to orchestration cluster.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment applyDeployment(String name) {
ApplyDeploymentRequest request = ApplyDeploymentRequest.newBuilder().setName(name).build();
return applyDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Applies the deployment's YAML files to the parent orchestration cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ApplyDeploymentRequest request =
* ApplyDeploymentRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .build();
* Deployment response = telcoAutomationClient.applyDeployment(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment applyDeployment(ApplyDeploymentRequest request) {
return applyDeploymentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Applies the deployment's YAML files to the parent orchestration cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ApplyDeploymentRequest request =
* ApplyDeploymentRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.applyDeploymentCallable().futureCall(request);
* // Do something.
* Deployment response = future.get();
* }
* }
*/
public final UnaryCallable applyDeploymentCallable() {
return stub.applyDeploymentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested deployment status.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeploymentName name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]");
* ComputeDeploymentStatusResponse response =
* telcoAutomationClient.computeDeploymentStatus(name);
* }
* }
*
* @param name Required. The name of the deployment without revisionID.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ComputeDeploymentStatusResponse computeDeploymentStatus(DeploymentName name) {
ComputeDeploymentStatusRequest request =
ComputeDeploymentStatusRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return computeDeploymentStatus(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested deployment status.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString();
* ComputeDeploymentStatusResponse response =
* telcoAutomationClient.computeDeploymentStatus(name);
* }
* }
*
* @param name Required. The name of the deployment without revisionID.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ComputeDeploymentStatusResponse computeDeploymentStatus(String name) {
ComputeDeploymentStatusRequest request =
ComputeDeploymentStatusRequest.newBuilder().setName(name).build();
return computeDeploymentStatus(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested deployment status.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ComputeDeploymentStatusRequest request =
* ComputeDeploymentStatusRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .build();
* ComputeDeploymentStatusResponse response =
* telcoAutomationClient.computeDeploymentStatus(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ComputeDeploymentStatusResponse computeDeploymentStatus(
ComputeDeploymentStatusRequest request) {
return computeDeploymentStatusCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested deployment status.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ComputeDeploymentStatusRequest request =
* ComputeDeploymentStatusRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.computeDeploymentStatusCallable().futureCall(request);
* // Do something.
* ComputeDeploymentStatusResponse response = future.get();
* }
* }
*/
public final UnaryCallable
computeDeploymentStatusCallable() {
return stub.computeDeploymentStatusCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rollback the active deployment to the given past approved deployment revision.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeploymentName name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]");
* String revisionId = "revisionId-1507445162";
* Deployment response = telcoAutomationClient.rollbackDeployment(name, revisionId);
* }
* }
*
* @param name Required. Name of the deployment.
* @param revisionId Required. The revision id of deployment to roll back to.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment rollbackDeployment(DeploymentName name, String revisionId) {
RollbackDeploymentRequest request =
RollbackDeploymentRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setRevisionId(revisionId)
.build();
return rollbackDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rollback the active deployment to the given past approved deployment revision.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString();
* String revisionId = "revisionId-1507445162";
* Deployment response = telcoAutomationClient.rollbackDeployment(name, revisionId);
* }
* }
*
* @param name Required. Name of the deployment.
* @param revisionId Required. The revision id of deployment to roll back to.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment rollbackDeployment(String name, String revisionId) {
RollbackDeploymentRequest request =
RollbackDeploymentRequest.newBuilder().setName(name).setRevisionId(revisionId).build();
return rollbackDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rollback the active deployment to the given past approved deployment revision.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* RollbackDeploymentRequest request =
* RollbackDeploymentRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setRevisionId("revisionId-1507445162")
* .build();
* Deployment response = telcoAutomationClient.rollbackDeployment(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Deployment rollbackDeployment(RollbackDeploymentRequest request) {
return rollbackDeploymentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Rollback the active deployment to the given past approved deployment revision.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* RollbackDeploymentRequest request =
* RollbackDeploymentRequest.newBuilder()
* .setName(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setRevisionId("revisionId-1507445162")
* .build();
* ApiFuture future =
* telcoAutomationClient.rollbackDeploymentCallable().futureCall(request);
* // Do something.
* Deployment response = future.get();
* }
* }
*/
public final UnaryCallable rollbackDeploymentCallable() {
return stub.rollbackDeploymentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested hydrated deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* HydratedDeploymentName name =
* HydratedDeploymentName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[ORCHESTRATION_CLUSTER]",
* "[DEPLOYMENT]",
* "[HYDRATED_DEPLOYMENT]");
* HydratedDeployment response = telcoAutomationClient.getHydratedDeployment(name);
* }
* }
*
* @param name Required. Name of the hydrated deployment.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HydratedDeployment getHydratedDeployment(HydratedDeploymentName name) {
GetHydratedDeploymentRequest request =
GetHydratedDeploymentRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getHydratedDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested hydrated deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* HydratedDeploymentName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[ORCHESTRATION_CLUSTER]",
* "[DEPLOYMENT]",
* "[HYDRATED_DEPLOYMENT]")
* .toString();
* HydratedDeployment response = telcoAutomationClient.getHydratedDeployment(name);
* }
* }
*
* @param name Required. Name of the hydrated deployment.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HydratedDeployment getHydratedDeployment(String name) {
GetHydratedDeploymentRequest request =
GetHydratedDeploymentRequest.newBuilder().setName(name).build();
return getHydratedDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested hydrated deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetHydratedDeploymentRequest request =
* GetHydratedDeploymentRequest.newBuilder()
* .setName(
* HydratedDeploymentName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[ORCHESTRATION_CLUSTER]",
* "[DEPLOYMENT]",
* "[HYDRATED_DEPLOYMENT]")
* .toString())
* .build();
* HydratedDeployment response = telcoAutomationClient.getHydratedDeployment(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HydratedDeployment getHydratedDeployment(GetHydratedDeploymentRequest request) {
return getHydratedDeploymentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested hydrated deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetHydratedDeploymentRequest request =
* GetHydratedDeploymentRequest.newBuilder()
* .setName(
* HydratedDeploymentName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[ORCHESTRATION_CLUSTER]",
* "[DEPLOYMENT]",
* "[HYDRATED_DEPLOYMENT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.getHydratedDeploymentCallable().futureCall(request);
* // Do something.
* HydratedDeployment response = future.get();
* }
* }
*/
public final UnaryCallable
getHydratedDeploymentCallable() {
return stub.getHydratedDeploymentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all hydrated deployments present under a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* DeploymentName parent =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]");
* for (HydratedDeployment element :
* telcoAutomationClient.listHydratedDeployments(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The deployment managing the hydrated deployments.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListHydratedDeploymentsPagedResponse listHydratedDeployments(DeploymentName parent) {
ListHydratedDeploymentsRequest request =
ListHydratedDeploymentsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listHydratedDeployments(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all hydrated deployments present under a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String parent =
* DeploymentName.of("[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString();
* for (HydratedDeployment element :
* telcoAutomationClient.listHydratedDeployments(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The deployment managing the hydrated deployments.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListHydratedDeploymentsPagedResponse listHydratedDeployments(String parent) {
ListHydratedDeploymentsRequest request =
ListHydratedDeploymentsRequest.newBuilder().setParent(parent).build();
return listHydratedDeployments(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all hydrated deployments present under a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListHydratedDeploymentsRequest request =
* ListHydratedDeploymentsRequest.newBuilder()
* .setParent(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (HydratedDeployment element :
* telcoAutomationClient.listHydratedDeployments(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListHydratedDeploymentsPagedResponse listHydratedDeployments(
ListHydratedDeploymentsRequest request) {
return listHydratedDeploymentsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all hydrated deployments present under a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListHydratedDeploymentsRequest request =
* ListHydratedDeploymentsRequest.newBuilder()
* .setParent(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* telcoAutomationClient.listHydratedDeploymentsPagedCallable().futureCall(request);
* // Do something.
* for (HydratedDeployment element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listHydratedDeploymentsPagedCallable() {
return stub.listHydratedDeploymentsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* List all hydrated deployments present under a deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListHydratedDeploymentsRequest request =
* ListHydratedDeploymentsRequest.newBuilder()
* .setParent(
* DeploymentName.of(
* "[PROJECT]", "[LOCATION]", "[ORCHESTRATION_CLUSTER]", "[DEPLOYMENT]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListHydratedDeploymentsResponse response =
* telcoAutomationClient.listHydratedDeploymentsCallable().call(request);
* for (HydratedDeployment element : response.getHydratedDeploymentsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listHydratedDeploymentsCallable() {
return stub.listHydratedDeploymentsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a hydrated deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* HydratedDeployment hydratedDeployment = HydratedDeployment.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* HydratedDeployment response =
* telcoAutomationClient.updateHydratedDeployment(hydratedDeployment, updateMask);
* }
* }
*
* @param hydratedDeployment Required. The hydrated deployment to update.
* @param updateMask Required. The list of fields to update. Update mask supports a special value
* `*` which fully replaces (equivalent to PUT) the resource provided.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HydratedDeployment updateHydratedDeployment(
HydratedDeployment hydratedDeployment, FieldMask updateMask) {
UpdateHydratedDeploymentRequest request =
UpdateHydratedDeploymentRequest.newBuilder()
.setHydratedDeployment(hydratedDeployment)
.setUpdateMask(updateMask)
.build();
return updateHydratedDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a hydrated deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* UpdateHydratedDeploymentRequest request =
* UpdateHydratedDeploymentRequest.newBuilder()
* .setHydratedDeployment(HydratedDeployment.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* HydratedDeployment response = telcoAutomationClient.updateHydratedDeployment(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HydratedDeployment updateHydratedDeployment(
UpdateHydratedDeploymentRequest request) {
return updateHydratedDeploymentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates a hydrated deployment.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* UpdateHydratedDeploymentRequest request =
* UpdateHydratedDeploymentRequest.newBuilder()
* .setHydratedDeployment(HydratedDeployment.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* telcoAutomationClient.updateHydratedDeploymentCallable().futureCall(request);
* // Do something.
* HydratedDeployment response = future.get();
* }
* }
*/
public final UnaryCallable
updateHydratedDeploymentCallable() {
return stub.updateHydratedDeploymentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Applies a hydrated deployment to a workload cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* HydratedDeploymentName name =
* HydratedDeploymentName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[ORCHESTRATION_CLUSTER]",
* "[DEPLOYMENT]",
* "[HYDRATED_DEPLOYMENT]");
* HydratedDeployment response = telcoAutomationClient.applyHydratedDeployment(name);
* }
* }
*
* @param name Required. The name of the hydrated deployment to apply.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HydratedDeployment applyHydratedDeployment(HydratedDeploymentName name) {
ApplyHydratedDeploymentRequest request =
ApplyHydratedDeploymentRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return applyHydratedDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Applies a hydrated deployment to a workload cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* String name =
* HydratedDeploymentName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[ORCHESTRATION_CLUSTER]",
* "[DEPLOYMENT]",
* "[HYDRATED_DEPLOYMENT]")
* .toString();
* HydratedDeployment response = telcoAutomationClient.applyHydratedDeployment(name);
* }
* }
*
* @param name Required. The name of the hydrated deployment to apply.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HydratedDeployment applyHydratedDeployment(String name) {
ApplyHydratedDeploymentRequest request =
ApplyHydratedDeploymentRequest.newBuilder().setName(name).build();
return applyHydratedDeployment(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Applies a hydrated deployment to a workload cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ApplyHydratedDeploymentRequest request =
* ApplyHydratedDeploymentRequest.newBuilder()
* .setName(
* HydratedDeploymentName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[ORCHESTRATION_CLUSTER]",
* "[DEPLOYMENT]",
* "[HYDRATED_DEPLOYMENT]")
* .toString())
* .build();
* HydratedDeployment response = telcoAutomationClient.applyHydratedDeployment(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final HydratedDeployment applyHydratedDeployment(ApplyHydratedDeploymentRequest request) {
return applyHydratedDeploymentCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Applies a hydrated deployment to a workload cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ApplyHydratedDeploymentRequest request =
* ApplyHydratedDeploymentRequest.newBuilder()
* .setName(
* HydratedDeploymentName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[ORCHESTRATION_CLUSTER]",
* "[DEPLOYMENT]",
* "[HYDRATED_DEPLOYMENT]")
* .toString())
* .build();
* ApiFuture future =
* telcoAutomationClient.applyHydratedDeploymentCallable().futureCall(request);
* // Do something.
* HydratedDeployment response = future.get();
* }
* }
*/
public final UnaryCallable
applyHydratedDeploymentCallable() {
return stub.applyHydratedDeploymentCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : telcoAutomationClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* telcoAutomationClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response =
* telcoAutomationClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = telcoAutomationClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TelcoAutomationClient telcoAutomationClient = TelcoAutomationClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = telcoAutomationClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListOrchestrationClustersPagedResponse
extends AbstractPagedListResponse<
ListOrchestrationClustersRequest,
ListOrchestrationClustersResponse,
OrchestrationCluster,
ListOrchestrationClustersPage,
ListOrchestrationClustersFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListOrchestrationClustersRequest,
ListOrchestrationClustersResponse,
OrchestrationCluster>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListOrchestrationClustersPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListOrchestrationClustersPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListOrchestrationClustersPagedResponse(ListOrchestrationClustersPage page) {
super(page, ListOrchestrationClustersFixedSizeCollection.createEmptyCollection());
}
}
public static class ListOrchestrationClustersPage
extends AbstractPage<
ListOrchestrationClustersRequest,
ListOrchestrationClustersResponse,
OrchestrationCluster,
ListOrchestrationClustersPage> {
private ListOrchestrationClustersPage(
PageContext<
ListOrchestrationClustersRequest,
ListOrchestrationClustersResponse,
OrchestrationCluster>
context,
ListOrchestrationClustersResponse response) {
super(context, response);
}
private static ListOrchestrationClustersPage createEmptyPage() {
return new ListOrchestrationClustersPage(null, null);
}
@Override
protected ListOrchestrationClustersPage createPage(
PageContext<
ListOrchestrationClustersRequest,
ListOrchestrationClustersResponse,
OrchestrationCluster>
context,
ListOrchestrationClustersResponse response) {
return new ListOrchestrationClustersPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListOrchestrationClustersRequest,
ListOrchestrationClustersResponse,
OrchestrationCluster>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListOrchestrationClustersFixedSizeCollection
extends AbstractFixedSizeCollection<
ListOrchestrationClustersRequest,
ListOrchestrationClustersResponse,
OrchestrationCluster,
ListOrchestrationClustersPage,
ListOrchestrationClustersFixedSizeCollection> {
private ListOrchestrationClustersFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListOrchestrationClustersFixedSizeCollection createEmptyCollection() {
return new ListOrchestrationClustersFixedSizeCollection(null, 0);
}
@Override
protected ListOrchestrationClustersFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListOrchestrationClustersFixedSizeCollection(pages, collectionSize);
}
}
public static class ListEdgeSlmsPagedResponse
extends AbstractPagedListResponse<
ListEdgeSlmsRequest,
ListEdgeSlmsResponse,
EdgeSlm,
ListEdgeSlmsPage,
ListEdgeSlmsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListEdgeSlmsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListEdgeSlmsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListEdgeSlmsPagedResponse(ListEdgeSlmsPage page) {
super(page, ListEdgeSlmsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListEdgeSlmsPage
extends AbstractPage {
private ListEdgeSlmsPage(
PageContext context,
ListEdgeSlmsResponse response) {
super(context, response);
}
private static ListEdgeSlmsPage createEmptyPage() {
return new ListEdgeSlmsPage(null, null);
}
@Override
protected ListEdgeSlmsPage createPage(
PageContext context,
ListEdgeSlmsResponse response) {
return new ListEdgeSlmsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListEdgeSlmsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListEdgeSlmsRequest,
ListEdgeSlmsResponse,
EdgeSlm,
ListEdgeSlmsPage,
ListEdgeSlmsFixedSizeCollection> {
private ListEdgeSlmsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListEdgeSlmsFixedSizeCollection createEmptyCollection() {
return new ListEdgeSlmsFixedSizeCollection(null, 0);
}
@Override
protected ListEdgeSlmsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListEdgeSlmsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListBlueprintsPagedResponse
extends AbstractPagedListResponse<
ListBlueprintsRequest,
ListBlueprintsResponse,
Blueprint,
ListBlueprintsPage,
ListBlueprintsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListBlueprintsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListBlueprintsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListBlueprintsPagedResponse(ListBlueprintsPage page) {
super(page, ListBlueprintsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListBlueprintsPage
extends AbstractPage<
ListBlueprintsRequest, ListBlueprintsResponse, Blueprint, ListBlueprintsPage> {
private ListBlueprintsPage(
PageContext context,
ListBlueprintsResponse response) {
super(context, response);
}
private static ListBlueprintsPage createEmptyPage() {
return new ListBlueprintsPage(null, null);
}
@Override
protected ListBlueprintsPage createPage(
PageContext context,
ListBlueprintsResponse response) {
return new ListBlueprintsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListBlueprintsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListBlueprintsRequest,
ListBlueprintsResponse,
Blueprint,
ListBlueprintsPage,
ListBlueprintsFixedSizeCollection> {
private ListBlueprintsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListBlueprintsFixedSizeCollection createEmptyCollection() {
return new ListBlueprintsFixedSizeCollection(null, 0);
}
@Override
protected ListBlueprintsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListBlueprintsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListBlueprintRevisionsPagedResponse
extends AbstractPagedListResponse<
ListBlueprintRevisionsRequest,
ListBlueprintRevisionsResponse,
Blueprint,
ListBlueprintRevisionsPage,
ListBlueprintRevisionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListBlueprintRevisionsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListBlueprintRevisionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListBlueprintRevisionsPagedResponse(ListBlueprintRevisionsPage page) {
super(page, ListBlueprintRevisionsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListBlueprintRevisionsPage
extends AbstractPage<
ListBlueprintRevisionsRequest,
ListBlueprintRevisionsResponse,
Blueprint,
ListBlueprintRevisionsPage> {
private ListBlueprintRevisionsPage(
PageContext
context,
ListBlueprintRevisionsResponse response) {
super(context, response);
}
private static ListBlueprintRevisionsPage createEmptyPage() {
return new ListBlueprintRevisionsPage(null, null);
}
@Override
protected ListBlueprintRevisionsPage createPage(
PageContext
context,
ListBlueprintRevisionsResponse response) {
return new ListBlueprintRevisionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListBlueprintRevisionsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListBlueprintRevisionsRequest,
ListBlueprintRevisionsResponse,
Blueprint,
ListBlueprintRevisionsPage,
ListBlueprintRevisionsFixedSizeCollection> {
private ListBlueprintRevisionsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListBlueprintRevisionsFixedSizeCollection createEmptyCollection() {
return new ListBlueprintRevisionsFixedSizeCollection(null, 0);
}
@Override
protected ListBlueprintRevisionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListBlueprintRevisionsFixedSizeCollection(pages, collectionSize);
}
}
public static class SearchBlueprintRevisionsPagedResponse
extends AbstractPagedListResponse<
SearchBlueprintRevisionsRequest,
SearchBlueprintRevisionsResponse,
Blueprint,
SearchBlueprintRevisionsPage,
SearchBlueprintRevisionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
SearchBlueprintRevisionsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new SearchBlueprintRevisionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private SearchBlueprintRevisionsPagedResponse(SearchBlueprintRevisionsPage page) {
super(page, SearchBlueprintRevisionsFixedSizeCollection.createEmptyCollection());
}
}
public static class SearchBlueprintRevisionsPage
extends AbstractPage<
SearchBlueprintRevisionsRequest,
SearchBlueprintRevisionsResponse,
Blueprint,
SearchBlueprintRevisionsPage> {
private SearchBlueprintRevisionsPage(
PageContext
context,
SearchBlueprintRevisionsResponse response) {
super(context, response);
}
private static SearchBlueprintRevisionsPage createEmptyPage() {
return new SearchBlueprintRevisionsPage(null, null);
}
@Override
protected SearchBlueprintRevisionsPage createPage(
PageContext
context,
SearchBlueprintRevisionsResponse response) {
return new SearchBlueprintRevisionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class SearchBlueprintRevisionsFixedSizeCollection
extends AbstractFixedSizeCollection<
SearchBlueprintRevisionsRequest,
SearchBlueprintRevisionsResponse,
Blueprint,
SearchBlueprintRevisionsPage,
SearchBlueprintRevisionsFixedSizeCollection> {
private SearchBlueprintRevisionsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static SearchBlueprintRevisionsFixedSizeCollection createEmptyCollection() {
return new SearchBlueprintRevisionsFixedSizeCollection(null, 0);
}
@Override
protected SearchBlueprintRevisionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new SearchBlueprintRevisionsFixedSizeCollection(pages, collectionSize);
}
}
public static class SearchDeploymentRevisionsPagedResponse
extends AbstractPagedListResponse<
SearchDeploymentRevisionsRequest,
SearchDeploymentRevisionsResponse,
Deployment,
SearchDeploymentRevisionsPage,
SearchDeploymentRevisionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
SearchDeploymentRevisionsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new SearchDeploymentRevisionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private SearchDeploymentRevisionsPagedResponse(SearchDeploymentRevisionsPage page) {
super(page, SearchDeploymentRevisionsFixedSizeCollection.createEmptyCollection());
}
}
public static class SearchDeploymentRevisionsPage
extends AbstractPage<
SearchDeploymentRevisionsRequest,
SearchDeploymentRevisionsResponse,
Deployment,
SearchDeploymentRevisionsPage> {
private SearchDeploymentRevisionsPage(
PageContext
context,
SearchDeploymentRevisionsResponse response) {
super(context, response);
}
private static SearchDeploymentRevisionsPage createEmptyPage() {
return new SearchDeploymentRevisionsPage(null, null);
}
@Override
protected SearchDeploymentRevisionsPage createPage(
PageContext
context,
SearchDeploymentRevisionsResponse response) {
return new SearchDeploymentRevisionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class SearchDeploymentRevisionsFixedSizeCollection
extends AbstractFixedSizeCollection<
SearchDeploymentRevisionsRequest,
SearchDeploymentRevisionsResponse,
Deployment,
SearchDeploymentRevisionsPage,
SearchDeploymentRevisionsFixedSizeCollection> {
private SearchDeploymentRevisionsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static SearchDeploymentRevisionsFixedSizeCollection createEmptyCollection() {
return new SearchDeploymentRevisionsFixedSizeCollection(null, 0);
}
@Override
protected SearchDeploymentRevisionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new SearchDeploymentRevisionsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListPublicBlueprintsPagedResponse
extends AbstractPagedListResponse<
ListPublicBlueprintsRequest,
ListPublicBlueprintsResponse,
PublicBlueprint,
ListPublicBlueprintsPage,
ListPublicBlueprintsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListPublicBlueprintsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListPublicBlueprintsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListPublicBlueprintsPagedResponse(ListPublicBlueprintsPage page) {
super(page, ListPublicBlueprintsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListPublicBlueprintsPage
extends AbstractPage<
ListPublicBlueprintsRequest,
ListPublicBlueprintsResponse,
PublicBlueprint,
ListPublicBlueprintsPage> {
private ListPublicBlueprintsPage(
PageContext
context,
ListPublicBlueprintsResponse response) {
super(context, response);
}
private static ListPublicBlueprintsPage createEmptyPage() {
return new ListPublicBlueprintsPage(null, null);
}
@Override
protected ListPublicBlueprintsPage createPage(
PageContext
context,
ListPublicBlueprintsResponse response) {
return new ListPublicBlueprintsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListPublicBlueprintsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListPublicBlueprintsRequest,
ListPublicBlueprintsResponse,
PublicBlueprint,
ListPublicBlueprintsPage,
ListPublicBlueprintsFixedSizeCollection> {
private ListPublicBlueprintsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListPublicBlueprintsFixedSizeCollection createEmptyCollection() {
return new ListPublicBlueprintsFixedSizeCollection(null, 0);
}
@Override
protected ListPublicBlueprintsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListPublicBlueprintsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListDeploymentsPagedResponse
extends AbstractPagedListResponse<
ListDeploymentsRequest,
ListDeploymentsResponse,
Deployment,
ListDeploymentsPage,
ListDeploymentsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListDeploymentsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListDeploymentsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListDeploymentsPagedResponse(ListDeploymentsPage page) {
super(page, ListDeploymentsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListDeploymentsPage
extends AbstractPage<
ListDeploymentsRequest, ListDeploymentsResponse, Deployment, ListDeploymentsPage> {
private ListDeploymentsPage(
PageContext context,
ListDeploymentsResponse response) {
super(context, response);
}
private static ListDeploymentsPage createEmptyPage() {
return new ListDeploymentsPage(null, null);
}
@Override
protected ListDeploymentsPage createPage(
PageContext context,
ListDeploymentsResponse response) {
return new ListDeploymentsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListDeploymentsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListDeploymentsRequest,
ListDeploymentsResponse,
Deployment,
ListDeploymentsPage,
ListDeploymentsFixedSizeCollection> {
private ListDeploymentsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListDeploymentsFixedSizeCollection createEmptyCollection() {
return new ListDeploymentsFixedSizeCollection(null, 0);
}
@Override
protected ListDeploymentsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListDeploymentsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListDeploymentRevisionsPagedResponse
extends AbstractPagedListResponse<
ListDeploymentRevisionsRequest,
ListDeploymentRevisionsResponse,
Deployment,
ListDeploymentRevisionsPage,
ListDeploymentRevisionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListDeploymentRevisionsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListDeploymentRevisionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListDeploymentRevisionsPagedResponse(ListDeploymentRevisionsPage page) {
super(page, ListDeploymentRevisionsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListDeploymentRevisionsPage
extends AbstractPage<
ListDeploymentRevisionsRequest,
ListDeploymentRevisionsResponse,
Deployment,
ListDeploymentRevisionsPage> {
private ListDeploymentRevisionsPage(
PageContext
context,
ListDeploymentRevisionsResponse response) {
super(context, response);
}
private static ListDeploymentRevisionsPage createEmptyPage() {
return new ListDeploymentRevisionsPage(null, null);
}
@Override
protected ListDeploymentRevisionsPage createPage(
PageContext
context,
ListDeploymentRevisionsResponse response) {
return new ListDeploymentRevisionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListDeploymentRevisionsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListDeploymentRevisionsRequest,
ListDeploymentRevisionsResponse,
Deployment,
ListDeploymentRevisionsPage,
ListDeploymentRevisionsFixedSizeCollection> {
private ListDeploymentRevisionsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListDeploymentRevisionsFixedSizeCollection createEmptyCollection() {
return new ListDeploymentRevisionsFixedSizeCollection(null, 0);
}
@Override
protected ListDeploymentRevisionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListDeploymentRevisionsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListHydratedDeploymentsPagedResponse
extends AbstractPagedListResponse<
ListHydratedDeploymentsRequest,
ListHydratedDeploymentsResponse,
HydratedDeployment,
ListHydratedDeploymentsPage,
ListHydratedDeploymentsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListHydratedDeploymentsRequest, ListHydratedDeploymentsResponse, HydratedDeployment>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListHydratedDeploymentsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListHydratedDeploymentsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListHydratedDeploymentsPagedResponse(ListHydratedDeploymentsPage page) {
super(page, ListHydratedDeploymentsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListHydratedDeploymentsPage
extends AbstractPage<
ListHydratedDeploymentsRequest,
ListHydratedDeploymentsResponse,
HydratedDeployment,
ListHydratedDeploymentsPage> {
private ListHydratedDeploymentsPage(
PageContext<
ListHydratedDeploymentsRequest, ListHydratedDeploymentsResponse, HydratedDeployment>
context,
ListHydratedDeploymentsResponse response) {
super(context, response);
}
private static ListHydratedDeploymentsPage createEmptyPage() {
return new ListHydratedDeploymentsPage(null, null);
}
@Override
protected ListHydratedDeploymentsPage createPage(
PageContext<
ListHydratedDeploymentsRequest, ListHydratedDeploymentsResponse, HydratedDeployment>
context,
ListHydratedDeploymentsResponse response) {
return new ListHydratedDeploymentsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListHydratedDeploymentsRequest, ListHydratedDeploymentsResponse, HydratedDeployment>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListHydratedDeploymentsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListHydratedDeploymentsRequest,
ListHydratedDeploymentsResponse,
HydratedDeployment,
ListHydratedDeploymentsPage,
ListHydratedDeploymentsFixedSizeCollection> {
private ListHydratedDeploymentsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListHydratedDeploymentsFixedSizeCollection createEmptyCollection() {
return new ListHydratedDeploymentsFixedSizeCollection(null, 0);
}
@Override
protected ListHydratedDeploymentsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListHydratedDeploymentsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}