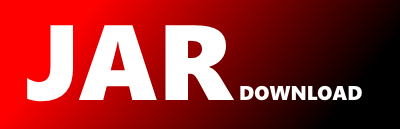
com.google.cloud.tpu.v2alpha1.TpuClient Maven / Gradle / Ivy
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.tpu.v2alpha1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.tpu.v2alpha1.stub.TpuStub;
import com.google.cloud.tpu.v2alpha1.stub.TpuStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.longrunning.Operation;
import com.google.longrunning.OperationsClient;
import com.google.protobuf.Empty;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Manages TPU nodes and other resources
*
* TPU API v2alpha1
*
*
This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* NodeName name = NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]");
* Node response = tpuClient.getNode(name);
* }
* }
*
* Note: close() needs to be called on the TpuClient object to clean up resources such as
* threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* ListNodes
* Lists nodes.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listNodes(ListNodesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listNodes(LocationName parent)
*
listNodes(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listNodesPagedCallable()
*
listNodesCallable()
*
*
*
*
* GetNode
* Gets the details of a node.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getNode(GetNodeRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getNode(NodeName name)
*
getNode(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getNodeCallable()
*
*
*
*
* CreateNode
* Creates a node.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createNodeAsync(CreateNodeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createNodeAsync(LocationName parent, Node node, String nodeId)
*
createNodeAsync(String parent, Node node, String nodeId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createNodeOperationCallable()
*
createNodeCallable()
*
*
*
*
* DeleteNode
* Deletes a node.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteNodeAsync(DeleteNodeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteNodeAsync(NodeName name)
*
deleteNodeAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteNodeOperationCallable()
*
deleteNodeCallable()
*
*
*
*
* StopNode
* Stops a node. This operation is only available with single TPU nodes.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* stopNodeAsync(StopNodeRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* stopNodeOperationCallable()
*
stopNodeCallable()
*
*
*
*
* StartNode
* Starts a node.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* startNodeAsync(StartNodeRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* startNodeOperationCallable()
*
startNodeCallable()
*
*
*
*
* UpdateNode
* Updates the configurations of a node.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateNodeAsync(UpdateNodeRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateNodeAsync(Node node, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateNodeOperationCallable()
*
updateNodeCallable()
*
*
*
*
* ListQueuedResources
* Lists queued resources.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listQueuedResources(ListQueuedResourcesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listQueuedResources(LocationName parent)
*
listQueuedResources(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listQueuedResourcesPagedCallable()
*
listQueuedResourcesCallable()
*
*
*
*
* GetQueuedResource
* Gets details of a queued resource.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getQueuedResource(GetQueuedResourceRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getQueuedResource(QueuedResourceName name)
*
getQueuedResource(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getQueuedResourceCallable()
*
*
*
*
* CreateQueuedResource
* Creates a QueuedResource TPU instance.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createQueuedResourceAsync(CreateQueuedResourceRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createQueuedResourceAsync(LocationName parent, QueuedResource queuedResource, String queuedResourceId)
*
createQueuedResourceAsync(String parent, QueuedResource queuedResource, String queuedResourceId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createQueuedResourceOperationCallable()
*
createQueuedResourceCallable()
*
*
*
*
* DeleteQueuedResource
* Deletes a QueuedResource TPU instance.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteQueuedResourceAsync(DeleteQueuedResourceRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteQueuedResourceAsync(QueuedResourceName name)
*
deleteQueuedResourceAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteQueuedResourceOperationCallable()
*
deleteQueuedResourceCallable()
*
*
*
*
* ResetQueuedResource
* Resets a QueuedResource TPU instance
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* resetQueuedResourceAsync(ResetQueuedResourceRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* resetQueuedResourceAsync(QueuedResourceName name)
*
resetQueuedResourceAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* resetQueuedResourceOperationCallable()
*
resetQueuedResourceCallable()
*
*
*
*
* GenerateServiceIdentity
* Generates the Cloud TPU service identity for the project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* generateServiceIdentity(GenerateServiceIdentityRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* generateServiceIdentityCallable()
*
*
*
*
* ListAcceleratorTypes
* Lists accelerator types supported by this API.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listAcceleratorTypes(ListAcceleratorTypesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listAcceleratorTypes(LocationName parent)
*
listAcceleratorTypes(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listAcceleratorTypesPagedCallable()
*
listAcceleratorTypesCallable()
*
*
*
*
* GetAcceleratorType
* Gets AcceleratorType.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getAcceleratorType(GetAcceleratorTypeRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getAcceleratorType(AcceleratorTypeName name)
*
getAcceleratorType(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getAcceleratorTypeCallable()
*
*
*
*
* ListRuntimeVersions
* Lists runtime versions supported by this API.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listRuntimeVersions(ListRuntimeVersionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listRuntimeVersions(LocationName parent)
*
listRuntimeVersions(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listRuntimeVersionsPagedCallable()
*
listRuntimeVersionsCallable()
*
*
*
*
* GetRuntimeVersion
* Gets a runtime version.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getRuntimeVersion(GetRuntimeVersionRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getRuntimeVersion(RuntimeVersionName name)
*
getRuntimeVersion(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getRuntimeVersionCallable()
*
*
*
*
* GetGuestAttributes
* Retrieves the guest attributes for the node.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getGuestAttributes(GetGuestAttributesRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getGuestAttributesCallable()
*
*
*
*
* SimulateMaintenanceEvent
* Simulates a maintenance event.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* simulateMaintenanceEventAsync(SimulateMaintenanceEventRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* simulateMaintenanceEventOperationCallable()
*
simulateMaintenanceEventCallable()
*
*
*
*
* ListLocations
* Lists information about the supported locations for this service.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listLocations(ListLocationsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listLocationsPagedCallable()
*
listLocationsCallable()
*
*
*
*
* GetLocation
* Gets information about a location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getLocation(GetLocationRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getLocationCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of TpuSettings to create(). For
* example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* TpuSettings tpuSettings =
* TpuSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* TpuClient tpuClient = TpuClient.create(tpuSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* TpuSettings tpuSettings = TpuSettings.newBuilder().setEndpoint(myEndpoint).build();
* TpuClient tpuClient = TpuClient.create(tpuSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@BetaApi
@Generated("by gapic-generator-java")
public class TpuClient implements BackgroundResource {
private final TpuSettings settings;
private final TpuStub stub;
private final OperationsClient operationsClient;
/** Constructs an instance of TpuClient with default settings. */
public static final TpuClient create() throws IOException {
return create(TpuSettings.newBuilder().build());
}
/**
* Constructs an instance of TpuClient, using the given settings. The channels are created based
* on the settings passed in, or defaults for any settings that are not set.
*/
public static final TpuClient create(TpuSettings settings) throws IOException {
return new TpuClient(settings);
}
/**
* Constructs an instance of TpuClient, using the given stub for making calls. This is for
* advanced usage - prefer using create(TpuSettings).
*/
public static final TpuClient create(TpuStub stub) {
return new TpuClient(stub);
}
/**
* Constructs an instance of TpuClient, using the given settings. This is protected so that it is
* easy to make a subclass, but otherwise, the static factory methods should be preferred.
*/
protected TpuClient(TpuSettings settings) throws IOException {
this.settings = settings;
this.stub = ((TpuStubSettings) settings.getStubSettings()).createStub();
this.operationsClient = OperationsClient.create(this.stub.getOperationsStub());
}
protected TpuClient(TpuStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient = OperationsClient.create(this.stub.getOperationsStub());
}
public final TpuSettings getSettings() {
return settings;
}
public TpuStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final OperationsClient getOperationsClient() {
return operationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists nodes.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (Node element : tpuClient.listNodes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListNodesPagedResponse listNodes(LocationName parent) {
ListNodesRequest request =
ListNodesRequest.newBuilder().setParent(parent == null ? null : parent.toString()).build();
return listNodes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists nodes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (Node element : tpuClient.listNodes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListNodesPagedResponse listNodes(String parent) {
ListNodesRequest request = ListNodesRequest.newBuilder().setParent(parent).build();
return listNodes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists nodes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListNodesRequest request =
* ListNodesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Node element : tpuClient.listNodes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListNodesPagedResponse listNodes(ListNodesRequest request) {
return listNodesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists nodes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListNodesRequest request =
* ListNodesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future = tpuClient.listNodesPagedCallable().futureCall(request);
* // Do something.
* for (Node element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listNodesPagedCallable() {
return stub.listNodesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists nodes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListNodesRequest request =
* ListNodesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListNodesResponse response = tpuClient.listNodesCallable().call(request);
* for (Node element : response.getNodesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listNodesCallable() {
return stub.listNodesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* NodeName name = NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]");
* Node response = tpuClient.getNode(name);
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Node getNode(NodeName name) {
GetNodeRequest request =
GetNodeRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getNode(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String name = NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString();
* Node response = tpuClient.getNode(name);
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Node getNode(String name) {
GetNodeRequest request = GetNodeRequest.newBuilder().setName(name).build();
return getNode(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetNodeRequest request =
* GetNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .build();
* Node response = tpuClient.getNode(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Node getNode(GetNodeRequest request) {
return getNodeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the details of a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetNodeRequest request =
* GetNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .build();
* ApiFuture future = tpuClient.getNodeCallable().futureCall(request);
* // Do something.
* Node response = future.get();
* }
* }
*/
public final UnaryCallable getNodeCallable() {
return stub.getNodeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* Node node = Node.newBuilder().build();
* String nodeId = "nodeId-1040171331";
* Node response = tpuClient.createNodeAsync(parent, node, nodeId).get();
* }
* }
*
* @param parent Required. The parent resource name.
* @param node Required. The node.
* @param nodeId The unqualified resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createNodeAsync(
LocationName parent, Node node, String nodeId) {
CreateNodeRequest request =
CreateNodeRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setNode(node)
.setNodeId(nodeId)
.build();
return createNodeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* Node node = Node.newBuilder().build();
* String nodeId = "nodeId-1040171331";
* Node response = tpuClient.createNodeAsync(parent, node, nodeId).get();
* }
* }
*
* @param parent Required. The parent resource name.
* @param node Required. The node.
* @param nodeId The unqualified resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createNodeAsync(
String parent, Node node, String nodeId) {
CreateNodeRequest request =
CreateNodeRequest.newBuilder().setParent(parent).setNode(node).setNodeId(nodeId).build();
return createNodeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* CreateNodeRequest request =
* CreateNodeRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setNodeId("nodeId-1040171331")
* .setNode(Node.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* Node response = tpuClient.createNodeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createNodeAsync(CreateNodeRequest request) {
return createNodeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* CreateNodeRequest request =
* CreateNodeRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setNodeId("nodeId-1040171331")
* .setNode(Node.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* tpuClient.createNodeOperationCallable().futureCall(request);
* // Do something.
* Node response = future.get();
* }
* }
*/
public final OperationCallable
createNodeOperationCallable() {
return stub.createNodeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* CreateNodeRequest request =
* CreateNodeRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setNodeId("nodeId-1040171331")
* .setNode(Node.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = tpuClient.createNodeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createNodeCallable() {
return stub.createNodeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* NodeName name = NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]");
* tpuClient.deleteNodeAsync(name).get();
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteNodeAsync(NodeName name) {
DeleteNodeRequest request =
DeleteNodeRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return deleteNodeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String name = NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString();
* tpuClient.deleteNodeAsync(name).get();
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteNodeAsync(String name) {
DeleteNodeRequest request = DeleteNodeRequest.newBuilder().setName(name).build();
return deleteNodeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* DeleteNodeRequest request =
* DeleteNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .setRequestId("requestId693933066")
* .build();
* tpuClient.deleteNodeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteNodeAsync(
DeleteNodeRequest request) {
return deleteNodeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* DeleteNodeRequest request =
* DeleteNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* tpuClient.deleteNodeOperationCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final OperationCallable
deleteNodeOperationCallable() {
return stub.deleteNodeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* DeleteNodeRequest request =
* DeleteNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = tpuClient.deleteNodeCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteNodeCallable() {
return stub.deleteNodeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops a node. This operation is only available with single TPU nodes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* StopNodeRequest request =
* StopNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .build();
* Node response = tpuClient.stopNodeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture stopNodeAsync(StopNodeRequest request) {
return stopNodeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops a node. This operation is only available with single TPU nodes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* StopNodeRequest request =
* StopNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .build();
* OperationFuture future =
* tpuClient.stopNodeOperationCallable().futureCall(request);
* // Do something.
* Node response = future.get();
* }
* }
*/
public final OperationCallable
stopNodeOperationCallable() {
return stub.stopNodeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops a node. This operation is only available with single TPU nodes.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* StopNodeRequest request =
* StopNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .build();
* ApiFuture future = tpuClient.stopNodeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable stopNodeCallable() {
return stub.stopNodeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* StartNodeRequest request =
* StartNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .build();
* Node response = tpuClient.startNodeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture startNodeAsync(StartNodeRequest request) {
return startNodeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* StartNodeRequest request =
* StartNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .build();
* OperationFuture future =
* tpuClient.startNodeOperationCallable().futureCall(request);
* // Do something.
* Node response = future.get();
* }
* }
*/
public final OperationCallable
startNodeOperationCallable() {
return stub.startNodeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* StartNodeRequest request =
* StartNodeRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .build();
* ApiFuture future = tpuClient.startNodeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable startNodeCallable() {
return stub.startNodeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the configurations of a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* Node node = Node.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Node response = tpuClient.updateNodeAsync(node, updateMask).get();
* }
* }
*
* @param node Required. The node. Only fields specified in update_mask are updated.
* @param updateMask Required. Mask of fields from [Node][Tpu.Node] to update. Supported fields:
* [description, tags, labels, metadata, network_config.enable_external_ips].
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateNodeAsync(
Node node, FieldMask updateMask) {
UpdateNodeRequest request =
UpdateNodeRequest.newBuilder().setNode(node).setUpdateMask(updateMask).build();
return updateNodeAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the configurations of a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* UpdateNodeRequest request =
* UpdateNodeRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setNode(Node.newBuilder().build())
* .build();
* Node response = tpuClient.updateNodeAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateNodeAsync(UpdateNodeRequest request) {
return updateNodeOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the configurations of a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* UpdateNodeRequest request =
* UpdateNodeRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setNode(Node.newBuilder().build())
* .build();
* OperationFuture future =
* tpuClient.updateNodeOperationCallable().futureCall(request);
* // Do something.
* Node response = future.get();
* }
* }
*/
public final OperationCallable
updateNodeOperationCallable() {
return stub.updateNodeOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the configurations of a node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* UpdateNodeRequest request =
* UpdateNodeRequest.newBuilder()
* .setUpdateMask(FieldMask.newBuilder().build())
* .setNode(Node.newBuilder().build())
* .build();
* ApiFuture future = tpuClient.updateNodeCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateNodeCallable() {
return stub.updateNodeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists queued resources.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (QueuedResource element : tpuClient.listQueuedResources(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListQueuedResourcesPagedResponse listQueuedResources(LocationName parent) {
ListQueuedResourcesRequest request =
ListQueuedResourcesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listQueuedResources(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists queued resources.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (QueuedResource element : tpuClient.listQueuedResources(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListQueuedResourcesPagedResponse listQueuedResources(String parent) {
ListQueuedResourcesRequest request =
ListQueuedResourcesRequest.newBuilder().setParent(parent).build();
return listQueuedResources(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists queued resources.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListQueuedResourcesRequest request =
* ListQueuedResourcesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (QueuedResource element : tpuClient.listQueuedResources(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListQueuedResourcesPagedResponse listQueuedResources(
ListQueuedResourcesRequest request) {
return listQueuedResourcesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists queued resources.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListQueuedResourcesRequest request =
* ListQueuedResourcesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* tpuClient.listQueuedResourcesPagedCallable().futureCall(request);
* // Do something.
* for (QueuedResource element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listQueuedResourcesPagedCallable() {
return stub.listQueuedResourcesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists queued resources.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListQueuedResourcesRequest request =
* ListQueuedResourcesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListQueuedResourcesResponse response =
* tpuClient.listQueuedResourcesCallable().call(request);
* for (QueuedResource element : response.getQueuedResourcesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listQueuedResourcesCallable() {
return stub.listQueuedResourcesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a queued resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* QueuedResourceName name =
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]");
* QueuedResource response = tpuClient.getQueuedResource(name);
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueuedResource getQueuedResource(QueuedResourceName name) {
GetQueuedResourceRequest request =
GetQueuedResourceRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getQueuedResource(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a queued resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String name =
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString();
* QueuedResource response = tpuClient.getQueuedResource(name);
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueuedResource getQueuedResource(String name) {
GetQueuedResourceRequest request = GetQueuedResourceRequest.newBuilder().setName(name).build();
return getQueuedResource(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a queued resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetQueuedResourceRequest request =
* GetQueuedResourceRequest.newBuilder()
* .setName(
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString())
* .build();
* QueuedResource response = tpuClient.getQueuedResource(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueuedResource getQueuedResource(GetQueuedResourceRequest request) {
return getQueuedResourceCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets details of a queued resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetQueuedResourceRequest request =
* GetQueuedResourceRequest.newBuilder()
* .setName(
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString())
* .build();
* ApiFuture future = tpuClient.getQueuedResourceCallable().futureCall(request);
* // Do something.
* QueuedResource response = future.get();
* }
* }
*/
public final UnaryCallable getQueuedResourceCallable() {
return stub.getQueuedResourceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* QueuedResource queuedResource = QueuedResource.newBuilder().build();
* String queuedResourceId = "queuedResourceId437646236";
* QueuedResource response =
* tpuClient.createQueuedResourceAsync(parent, queuedResource, queuedResourceId).get();
* }
* }
*
* @param parent Required. The parent resource name.
* @param queuedResource Required. The queued resource.
* @param queuedResourceId The unqualified resource name. Should follow the `^[A-Za-z0-9_.~+%-]+$`
* regex format.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createQueuedResourceAsync(
LocationName parent, QueuedResource queuedResource, String queuedResourceId) {
CreateQueuedResourceRequest request =
CreateQueuedResourceRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setQueuedResource(queuedResource)
.setQueuedResourceId(queuedResourceId)
.build();
return createQueuedResourceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* QueuedResource queuedResource = QueuedResource.newBuilder().build();
* String queuedResourceId = "queuedResourceId437646236";
* QueuedResource response =
* tpuClient.createQueuedResourceAsync(parent, queuedResource, queuedResourceId).get();
* }
* }
*
* @param parent Required. The parent resource name.
* @param queuedResource Required. The queued resource.
* @param queuedResourceId The unqualified resource name. Should follow the `^[A-Za-z0-9_.~+%-]+$`
* regex format.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createQueuedResourceAsync(
String parent, QueuedResource queuedResource, String queuedResourceId) {
CreateQueuedResourceRequest request =
CreateQueuedResourceRequest.newBuilder()
.setParent(parent)
.setQueuedResource(queuedResource)
.setQueuedResourceId(queuedResourceId)
.build();
return createQueuedResourceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* CreateQueuedResourceRequest request =
* CreateQueuedResourceRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setQueuedResourceId("queuedResourceId437646236")
* .setQueuedResource(QueuedResource.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* QueuedResource response = tpuClient.createQueuedResourceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createQueuedResourceAsync(
CreateQueuedResourceRequest request) {
return createQueuedResourceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* CreateQueuedResourceRequest request =
* CreateQueuedResourceRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setQueuedResourceId("queuedResourceId437646236")
* .setQueuedResource(QueuedResource.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* OperationFuture future =
* tpuClient.createQueuedResourceOperationCallable().futureCall(request);
* // Do something.
* QueuedResource response = future.get();
* }
* }
*/
public final OperationCallable
createQueuedResourceOperationCallable() {
return stub.createQueuedResourceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* CreateQueuedResourceRequest request =
* CreateQueuedResourceRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setQueuedResourceId("queuedResourceId437646236")
* .setQueuedResource(QueuedResource.newBuilder().build())
* .setRequestId("requestId693933066")
* .build();
* ApiFuture future = tpuClient.createQueuedResourceCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
createQueuedResourceCallable() {
return stub.createQueuedResourceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* QueuedResourceName name =
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]");
* QueuedResource response = tpuClient.deleteQueuedResourceAsync(name).get();
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteQueuedResourceAsync(
QueuedResourceName name) {
DeleteQueuedResourceRequest request =
DeleteQueuedResourceRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteQueuedResourceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String name =
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString();
* QueuedResource response = tpuClient.deleteQueuedResourceAsync(name).get();
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteQueuedResourceAsync(
String name) {
DeleteQueuedResourceRequest request =
DeleteQueuedResourceRequest.newBuilder().setName(name).build();
return deleteQueuedResourceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* DeleteQueuedResourceRequest request =
* DeleteQueuedResourceRequest.newBuilder()
* .setName(
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* QueuedResource response = tpuClient.deleteQueuedResourceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteQueuedResourceAsync(
DeleteQueuedResourceRequest request) {
return deleteQueuedResourceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* DeleteQueuedResourceRequest request =
* DeleteQueuedResourceRequest.newBuilder()
* .setName(
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* OperationFuture future =
* tpuClient.deleteQueuedResourceOperationCallable().futureCall(request);
* // Do something.
* QueuedResource response = future.get();
* }
* }
*/
public final OperationCallable
deleteQueuedResourceOperationCallable() {
return stub.deleteQueuedResourceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a QueuedResource TPU instance.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* DeleteQueuedResourceRequest request =
* DeleteQueuedResourceRequest.newBuilder()
* .setName(
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString())
* .setRequestId("requestId693933066")
* .setForce(true)
* .build();
* ApiFuture future = tpuClient.deleteQueuedResourceCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
deleteQueuedResourceCallable() {
return stub.deleteQueuedResourceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resets a QueuedResource TPU instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* QueuedResourceName name =
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]");
* QueuedResource response = tpuClient.resetQueuedResourceAsync(name).get();
* }
* }
*
* @param name Required. The name of the queued resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture resetQueuedResourceAsync(
QueuedResourceName name) {
ResetQueuedResourceRequest request =
ResetQueuedResourceRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return resetQueuedResourceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resets a QueuedResource TPU instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String name =
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString();
* QueuedResource response = tpuClient.resetQueuedResourceAsync(name).get();
* }
* }
*
* @param name Required. The name of the queued resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture resetQueuedResourceAsync(
String name) {
ResetQueuedResourceRequest request =
ResetQueuedResourceRequest.newBuilder().setName(name).build();
return resetQueuedResourceAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resets a QueuedResource TPU instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ResetQueuedResourceRequest request =
* ResetQueuedResourceRequest.newBuilder()
* .setName(
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString())
* .build();
* QueuedResource response = tpuClient.resetQueuedResourceAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture resetQueuedResourceAsync(
ResetQueuedResourceRequest request) {
return resetQueuedResourceOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resets a QueuedResource TPU instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ResetQueuedResourceRequest request =
* ResetQueuedResourceRequest.newBuilder()
* .setName(
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString())
* .build();
* OperationFuture future =
* tpuClient.resetQueuedResourceOperationCallable().futureCall(request);
* // Do something.
* QueuedResource response = future.get();
* }
* }
*/
public final OperationCallable
resetQueuedResourceOperationCallable() {
return stub.resetQueuedResourceOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Resets a QueuedResource TPU instance
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ResetQueuedResourceRequest request =
* ResetQueuedResourceRequest.newBuilder()
* .setName(
* QueuedResourceName.of("[PROJECT]", "[LOCATION]", "[QUEUED_RESOURCE]").toString())
* .build();
* ApiFuture future = tpuClient.resetQueuedResourceCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable resetQueuedResourceCallable() {
return stub.resetQueuedResourceCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generates the Cloud TPU service identity for the project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GenerateServiceIdentityRequest request =
* GenerateServiceIdentityRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .build();
* GenerateServiceIdentityResponse response = tpuClient.generateServiceIdentity(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateServiceIdentityResponse generateServiceIdentity(
GenerateServiceIdentityRequest request) {
return generateServiceIdentityCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Generates the Cloud TPU service identity for the project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GenerateServiceIdentityRequest request =
* GenerateServiceIdentityRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .build();
* ApiFuture future =
* tpuClient.generateServiceIdentityCallable().futureCall(request);
* // Do something.
* GenerateServiceIdentityResponse response = future.get();
* }
* }
*/
public final UnaryCallable
generateServiceIdentityCallable() {
return stub.generateServiceIdentityCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists accelerator types supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (AcceleratorType element : tpuClient.listAcceleratorTypes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAcceleratorTypesPagedResponse listAcceleratorTypes(LocationName parent) {
ListAcceleratorTypesRequest request =
ListAcceleratorTypesRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listAcceleratorTypes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists accelerator types supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (AcceleratorType element : tpuClient.listAcceleratorTypes(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAcceleratorTypesPagedResponse listAcceleratorTypes(String parent) {
ListAcceleratorTypesRequest request =
ListAcceleratorTypesRequest.newBuilder().setParent(parent).build();
return listAcceleratorTypes(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists accelerator types supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListAcceleratorTypesRequest request =
* ListAcceleratorTypesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (AcceleratorType element : tpuClient.listAcceleratorTypes(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListAcceleratorTypesPagedResponse listAcceleratorTypes(
ListAcceleratorTypesRequest request) {
return listAcceleratorTypesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists accelerator types supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListAcceleratorTypesRequest request =
* ListAcceleratorTypesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* tpuClient.listAcceleratorTypesPagedCallable().futureCall(request);
* // Do something.
* for (AcceleratorType element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listAcceleratorTypesPagedCallable() {
return stub.listAcceleratorTypesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists accelerator types supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListAcceleratorTypesRequest request =
* ListAcceleratorTypesRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListAcceleratorTypesResponse response =
* tpuClient.listAcceleratorTypesCallable().call(request);
* for (AcceleratorType element : response.getAcceleratorTypesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listAcceleratorTypesCallable() {
return stub.listAcceleratorTypesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets AcceleratorType.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* AcceleratorTypeName name =
* AcceleratorTypeName.of("[PROJECT]", "[LOCATION]", "[ACCELERATOR_TYPE]");
* AcceleratorType response = tpuClient.getAcceleratorType(name);
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AcceleratorType getAcceleratorType(AcceleratorTypeName name) {
GetAcceleratorTypeRequest request =
GetAcceleratorTypeRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getAcceleratorType(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets AcceleratorType.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String name =
* AcceleratorTypeName.of("[PROJECT]", "[LOCATION]", "[ACCELERATOR_TYPE]").toString();
* AcceleratorType response = tpuClient.getAcceleratorType(name);
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AcceleratorType getAcceleratorType(String name) {
GetAcceleratorTypeRequest request =
GetAcceleratorTypeRequest.newBuilder().setName(name).build();
return getAcceleratorType(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets AcceleratorType.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetAcceleratorTypeRequest request =
* GetAcceleratorTypeRequest.newBuilder()
* .setName(
* AcceleratorTypeName.of("[PROJECT]", "[LOCATION]", "[ACCELERATOR_TYPE]")
* .toString())
* .build();
* AcceleratorType response = tpuClient.getAcceleratorType(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final AcceleratorType getAcceleratorType(GetAcceleratorTypeRequest request) {
return getAcceleratorTypeCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets AcceleratorType.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetAcceleratorTypeRequest request =
* GetAcceleratorTypeRequest.newBuilder()
* .setName(
* AcceleratorTypeName.of("[PROJECT]", "[LOCATION]", "[ACCELERATOR_TYPE]")
* .toString())
* .build();
* ApiFuture future =
* tpuClient.getAcceleratorTypeCallable().futureCall(request);
* // Do something.
* AcceleratorType response = future.get();
* }
* }
*/
public final UnaryCallable
getAcceleratorTypeCallable() {
return stub.getAcceleratorTypeCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists runtime versions supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (RuntimeVersion element : tpuClient.listRuntimeVersions(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRuntimeVersionsPagedResponse listRuntimeVersions(LocationName parent) {
ListRuntimeVersionsRequest request =
ListRuntimeVersionsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listRuntimeVersions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists runtime versions supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (RuntimeVersion element : tpuClient.listRuntimeVersions(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. The parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRuntimeVersionsPagedResponse listRuntimeVersions(String parent) {
ListRuntimeVersionsRequest request =
ListRuntimeVersionsRequest.newBuilder().setParent(parent).build();
return listRuntimeVersions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists runtime versions supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListRuntimeVersionsRequest request =
* ListRuntimeVersionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* for (RuntimeVersion element : tpuClient.listRuntimeVersions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRuntimeVersionsPagedResponse listRuntimeVersions(
ListRuntimeVersionsRequest request) {
return listRuntimeVersionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists runtime versions supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListRuntimeVersionsRequest request =
* ListRuntimeVersionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* ApiFuture future =
* tpuClient.listRuntimeVersionsPagedCallable().futureCall(request);
* // Do something.
* for (RuntimeVersion element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listRuntimeVersionsPagedCallable() {
return stub.listRuntimeVersionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists runtime versions supported by this API.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListRuntimeVersionsRequest request =
* ListRuntimeVersionsRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setFilter("filter-1274492040")
* .setOrderBy("orderBy-1207110587")
* .build();
* while (true) {
* ListRuntimeVersionsResponse response =
* tpuClient.listRuntimeVersionsCallable().call(request);
* for (RuntimeVersion element : response.getRuntimeVersionsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listRuntimeVersionsCallable() {
return stub.listRuntimeVersionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a runtime version.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* RuntimeVersionName name =
* RuntimeVersionName.of("[PROJECT]", "[LOCATION]", "[RUNTIME_VERSION]");
* RuntimeVersion response = tpuClient.getRuntimeVersion(name);
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RuntimeVersion getRuntimeVersion(RuntimeVersionName name) {
GetRuntimeVersionRequest request =
GetRuntimeVersionRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getRuntimeVersion(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a runtime version.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* String name =
* RuntimeVersionName.of("[PROJECT]", "[LOCATION]", "[RUNTIME_VERSION]").toString();
* RuntimeVersion response = tpuClient.getRuntimeVersion(name);
* }
* }
*
* @param name Required. The resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RuntimeVersion getRuntimeVersion(String name) {
GetRuntimeVersionRequest request = GetRuntimeVersionRequest.newBuilder().setName(name).build();
return getRuntimeVersion(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a runtime version.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetRuntimeVersionRequest request =
* GetRuntimeVersionRequest.newBuilder()
* .setName(
* RuntimeVersionName.of("[PROJECT]", "[LOCATION]", "[RUNTIME_VERSION]").toString())
* .build();
* RuntimeVersion response = tpuClient.getRuntimeVersion(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final RuntimeVersion getRuntimeVersion(GetRuntimeVersionRequest request) {
return getRuntimeVersionCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a runtime version.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetRuntimeVersionRequest request =
* GetRuntimeVersionRequest.newBuilder()
* .setName(
* RuntimeVersionName.of("[PROJECT]", "[LOCATION]", "[RUNTIME_VERSION]").toString())
* .build();
* ApiFuture future = tpuClient.getRuntimeVersionCallable().futureCall(request);
* // Do something.
* RuntimeVersion response = future.get();
* }
* }
*/
public final UnaryCallable getRuntimeVersionCallable() {
return stub.getRuntimeVersionCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the guest attributes for the node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetGuestAttributesRequest request =
* GetGuestAttributesRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .setQueryPath("queryPath-1807004403")
* .addAllWorkerIds(new ArrayList())
* .build();
* GetGuestAttributesResponse response = tpuClient.getGuestAttributes(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GetGuestAttributesResponse getGuestAttributes(GetGuestAttributesRequest request) {
return getGuestAttributesCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Retrieves the guest attributes for the node.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetGuestAttributesRequest request =
* GetGuestAttributesRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .setQueryPath("queryPath-1807004403")
* .addAllWorkerIds(new ArrayList())
* .build();
* ApiFuture future =
* tpuClient.getGuestAttributesCallable().futureCall(request);
* // Do something.
* GetGuestAttributesResponse response = future.get();
* }
* }
*/
public final UnaryCallable
getGuestAttributesCallable() {
return stub.getGuestAttributesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Simulates a maintenance event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* SimulateMaintenanceEventRequest request =
* SimulateMaintenanceEventRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .addAllWorkerIds(new ArrayList())
* .build();
* Node response = tpuClient.simulateMaintenanceEventAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture simulateMaintenanceEventAsync(
SimulateMaintenanceEventRequest request) {
return simulateMaintenanceEventOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Simulates a maintenance event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* SimulateMaintenanceEventRequest request =
* SimulateMaintenanceEventRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .addAllWorkerIds(new ArrayList())
* .build();
* OperationFuture future =
* tpuClient.simulateMaintenanceEventOperationCallable().futureCall(request);
* // Do something.
* Node response = future.get();
* }
* }
*/
public final OperationCallable
simulateMaintenanceEventOperationCallable() {
return stub.simulateMaintenanceEventOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Simulates a maintenance event.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* SimulateMaintenanceEventRequest request =
* SimulateMaintenanceEventRequest.newBuilder()
* .setName(NodeName.of("[PROJECT]", "[LOCATION]", "[NODE]").toString())
* .addAllWorkerIds(new ArrayList())
* .build();
* ApiFuture future =
* tpuClient.simulateMaintenanceEventCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
simulateMaintenanceEventCallable() {
return stub.simulateMaintenanceEventCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Location element : tpuClient.listLocations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListLocationsPagedResponse listLocations(ListLocationsRequest request) {
return listLocationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future = tpuClient.listLocationsPagedCallable().futureCall(request);
* // Do something.
* for (Location element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listLocationsPagedCallable() {
return stub.listLocationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists information about the supported locations for this service.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* ListLocationsRequest request =
* ListLocationsRequest.newBuilder()
* .setName("name3373707")
* .setFilter("filter-1274492040")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListLocationsResponse response = tpuClient.listLocationsCallable().call(request);
* for (Location element : response.getLocationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listLocationsCallable() {
return stub.listLocationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* Location response = tpuClient.getLocation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Location getLocation(GetLocationRequest request) {
return getLocationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets information about a location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (TpuClient tpuClient = TpuClient.create()) {
* GetLocationRequest request = GetLocationRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = tpuClient.getLocationCallable().futureCall(request);
* // Do something.
* Location response = future.get();
* }
* }
*/
public final UnaryCallable getLocationCallable() {
return stub.getLocationCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListNodesPagedResponse
extends AbstractPagedListResponse<
ListNodesRequest, ListNodesResponse, Node, ListNodesPage, ListNodesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListNodesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListNodesPagedResponse(input), MoreExecutors.directExecutor());
}
private ListNodesPagedResponse(ListNodesPage page) {
super(page, ListNodesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListNodesPage
extends AbstractPage {
private ListNodesPage(
PageContext context,
ListNodesResponse response) {
super(context, response);
}
private static ListNodesPage createEmptyPage() {
return new ListNodesPage(null, null);
}
@Override
protected ListNodesPage createPage(
PageContext context,
ListNodesResponse response) {
return new ListNodesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListNodesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListNodesRequest, ListNodesResponse, Node, ListNodesPage, ListNodesFixedSizeCollection> {
private ListNodesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListNodesFixedSizeCollection createEmptyCollection() {
return new ListNodesFixedSizeCollection(null, 0);
}
@Override
protected ListNodesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListNodesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListQueuedResourcesPagedResponse
extends AbstractPagedListResponse<
ListQueuedResourcesRequest,
ListQueuedResourcesResponse,
QueuedResource,
ListQueuedResourcesPage,
ListQueuedResourcesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListQueuedResourcesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListQueuedResourcesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListQueuedResourcesPagedResponse(ListQueuedResourcesPage page) {
super(page, ListQueuedResourcesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListQueuedResourcesPage
extends AbstractPage<
ListQueuedResourcesRequest,
ListQueuedResourcesResponse,
QueuedResource,
ListQueuedResourcesPage> {
private ListQueuedResourcesPage(
PageContext
context,
ListQueuedResourcesResponse response) {
super(context, response);
}
private static ListQueuedResourcesPage createEmptyPage() {
return new ListQueuedResourcesPage(null, null);
}
@Override
protected ListQueuedResourcesPage createPage(
PageContext
context,
ListQueuedResourcesResponse response) {
return new ListQueuedResourcesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListQueuedResourcesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListQueuedResourcesRequest,
ListQueuedResourcesResponse,
QueuedResource,
ListQueuedResourcesPage,
ListQueuedResourcesFixedSizeCollection> {
private ListQueuedResourcesFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListQueuedResourcesFixedSizeCollection createEmptyCollection() {
return new ListQueuedResourcesFixedSizeCollection(null, 0);
}
@Override
protected ListQueuedResourcesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListQueuedResourcesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListAcceleratorTypesPagedResponse
extends AbstractPagedListResponse<
ListAcceleratorTypesRequest,
ListAcceleratorTypesResponse,
AcceleratorType,
ListAcceleratorTypesPage,
ListAcceleratorTypesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListAcceleratorTypesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListAcceleratorTypesPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListAcceleratorTypesPagedResponse(ListAcceleratorTypesPage page) {
super(page, ListAcceleratorTypesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListAcceleratorTypesPage
extends AbstractPage<
ListAcceleratorTypesRequest,
ListAcceleratorTypesResponse,
AcceleratorType,
ListAcceleratorTypesPage> {
private ListAcceleratorTypesPage(
PageContext
context,
ListAcceleratorTypesResponse response) {
super(context, response);
}
private static ListAcceleratorTypesPage createEmptyPage() {
return new ListAcceleratorTypesPage(null, null);
}
@Override
protected ListAcceleratorTypesPage createPage(
PageContext
context,
ListAcceleratorTypesResponse response) {
return new ListAcceleratorTypesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListAcceleratorTypesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListAcceleratorTypesRequest,
ListAcceleratorTypesResponse,
AcceleratorType,
ListAcceleratorTypesPage,
ListAcceleratorTypesFixedSizeCollection> {
private ListAcceleratorTypesFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListAcceleratorTypesFixedSizeCollection createEmptyCollection() {
return new ListAcceleratorTypesFixedSizeCollection(null, 0);
}
@Override
protected ListAcceleratorTypesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListAcceleratorTypesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListRuntimeVersionsPagedResponse
extends AbstractPagedListResponse<
ListRuntimeVersionsRequest,
ListRuntimeVersionsResponse,
RuntimeVersion,
ListRuntimeVersionsPage,
ListRuntimeVersionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListRuntimeVersionsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListRuntimeVersionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListRuntimeVersionsPagedResponse(ListRuntimeVersionsPage page) {
super(page, ListRuntimeVersionsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListRuntimeVersionsPage
extends AbstractPage<
ListRuntimeVersionsRequest,
ListRuntimeVersionsResponse,
RuntimeVersion,
ListRuntimeVersionsPage> {
private ListRuntimeVersionsPage(
PageContext
context,
ListRuntimeVersionsResponse response) {
super(context, response);
}
private static ListRuntimeVersionsPage createEmptyPage() {
return new ListRuntimeVersionsPage(null, null);
}
@Override
protected ListRuntimeVersionsPage createPage(
PageContext
context,
ListRuntimeVersionsResponse response) {
return new ListRuntimeVersionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListRuntimeVersionsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListRuntimeVersionsRequest,
ListRuntimeVersionsResponse,
RuntimeVersion,
ListRuntimeVersionsPage,
ListRuntimeVersionsFixedSizeCollection> {
private ListRuntimeVersionsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListRuntimeVersionsFixedSizeCollection createEmptyCollection() {
return new ListRuntimeVersionsFixedSizeCollection(null, 0);
}
@Override
protected ListRuntimeVersionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListRuntimeVersionsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListLocationsPagedResponse
extends AbstractPagedListResponse<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListLocationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListLocationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListLocationsPagedResponse(ListLocationsPage page) {
super(page, ListLocationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListLocationsPage
extends AbstractPage<
ListLocationsRequest, ListLocationsResponse, Location, ListLocationsPage> {
private ListLocationsPage(
PageContext context,
ListLocationsResponse response) {
super(context, response);
}
private static ListLocationsPage createEmptyPage() {
return new ListLocationsPage(null, null);
}
@Override
protected ListLocationsPage createPage(
PageContext context,
ListLocationsResponse response) {
return new ListLocationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListLocationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListLocationsRequest,
ListLocationsResponse,
Location,
ListLocationsPage,
ListLocationsFixedSizeCollection> {
private ListLocationsFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListLocationsFixedSizeCollection createEmptyCollection() {
return new ListLocationsFixedSizeCollection(null, 0);
}
@Override
protected ListLocationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListLocationsFixedSizeCollection(pages, collectionSize);
}
}
}