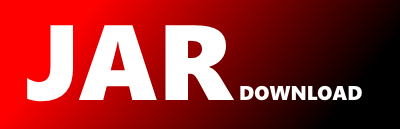
com.google.cloud.tpu.v2alpha1.stub.TpuStub Maven / Gradle / Ivy
Show all versions of google-cloud-tpu Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.tpu.v2alpha1.stub;
import static com.google.cloud.tpu.v2alpha1.TpuClient.ListAcceleratorTypesPagedResponse;
import static com.google.cloud.tpu.v2alpha1.TpuClient.ListLocationsPagedResponse;
import static com.google.cloud.tpu.v2alpha1.TpuClient.ListNodesPagedResponse;
import static com.google.cloud.tpu.v2alpha1.TpuClient.ListQueuedResourcesPagedResponse;
import static com.google.cloud.tpu.v2alpha1.TpuClient.ListRuntimeVersionsPagedResponse;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.location.GetLocationRequest;
import com.google.cloud.location.ListLocationsRequest;
import com.google.cloud.location.ListLocationsResponse;
import com.google.cloud.location.Location;
import com.google.cloud.tpu.v2alpha1.AcceleratorType;
import com.google.cloud.tpu.v2alpha1.CreateNodeRequest;
import com.google.cloud.tpu.v2alpha1.CreateQueuedResourceRequest;
import com.google.cloud.tpu.v2alpha1.DeleteNodeRequest;
import com.google.cloud.tpu.v2alpha1.DeleteQueuedResourceRequest;
import com.google.cloud.tpu.v2alpha1.GenerateServiceIdentityRequest;
import com.google.cloud.tpu.v2alpha1.GenerateServiceIdentityResponse;
import com.google.cloud.tpu.v2alpha1.GetAcceleratorTypeRequest;
import com.google.cloud.tpu.v2alpha1.GetGuestAttributesRequest;
import com.google.cloud.tpu.v2alpha1.GetGuestAttributesResponse;
import com.google.cloud.tpu.v2alpha1.GetNodeRequest;
import com.google.cloud.tpu.v2alpha1.GetQueuedResourceRequest;
import com.google.cloud.tpu.v2alpha1.GetRuntimeVersionRequest;
import com.google.cloud.tpu.v2alpha1.ListAcceleratorTypesRequest;
import com.google.cloud.tpu.v2alpha1.ListAcceleratorTypesResponse;
import com.google.cloud.tpu.v2alpha1.ListNodesRequest;
import com.google.cloud.tpu.v2alpha1.ListNodesResponse;
import com.google.cloud.tpu.v2alpha1.ListQueuedResourcesRequest;
import com.google.cloud.tpu.v2alpha1.ListQueuedResourcesResponse;
import com.google.cloud.tpu.v2alpha1.ListRuntimeVersionsRequest;
import com.google.cloud.tpu.v2alpha1.ListRuntimeVersionsResponse;
import com.google.cloud.tpu.v2alpha1.Node;
import com.google.cloud.tpu.v2alpha1.OperationMetadata;
import com.google.cloud.tpu.v2alpha1.QueuedResource;
import com.google.cloud.tpu.v2alpha1.ResetQueuedResourceRequest;
import com.google.cloud.tpu.v2alpha1.RuntimeVersion;
import com.google.cloud.tpu.v2alpha1.SimulateMaintenanceEventRequest;
import com.google.cloud.tpu.v2alpha1.StartNodeRequest;
import com.google.cloud.tpu.v2alpha1.StopNodeRequest;
import com.google.cloud.tpu.v2alpha1.UpdateNodeRequest;
import com.google.longrunning.Operation;
import com.google.longrunning.stub.OperationsStub;
import com.google.protobuf.Empty;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Base stub class for the Tpu service API.
*
* This class is for advanced usage and reflects the underlying API directly.
*/
@BetaApi
@Generated("by gapic-generator-java")
public abstract class TpuStub implements BackgroundResource {
public OperationsStub getOperationsStub() {
throw new UnsupportedOperationException("Not implemented: getOperationsStub()");
}
public UnaryCallable listNodesPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listNodesPagedCallable()");
}
public UnaryCallable listNodesCallable() {
throw new UnsupportedOperationException("Not implemented: listNodesCallable()");
}
public UnaryCallable getNodeCallable() {
throw new UnsupportedOperationException("Not implemented: getNodeCallable()");
}
public OperationCallable
createNodeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: createNodeOperationCallable()");
}
public UnaryCallable createNodeCallable() {
throw new UnsupportedOperationException("Not implemented: createNodeCallable()");
}
public OperationCallable
deleteNodeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: deleteNodeOperationCallable()");
}
public UnaryCallable deleteNodeCallable() {
throw new UnsupportedOperationException("Not implemented: deleteNodeCallable()");
}
public OperationCallable stopNodeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: stopNodeOperationCallable()");
}
public UnaryCallable stopNodeCallable() {
throw new UnsupportedOperationException("Not implemented: stopNodeCallable()");
}
public OperationCallable startNodeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: startNodeOperationCallable()");
}
public UnaryCallable startNodeCallable() {
throw new UnsupportedOperationException("Not implemented: startNodeCallable()");
}
public OperationCallable
updateNodeOperationCallable() {
throw new UnsupportedOperationException("Not implemented: updateNodeOperationCallable()");
}
public UnaryCallable updateNodeCallable() {
throw new UnsupportedOperationException("Not implemented: updateNodeCallable()");
}
public UnaryCallable
listQueuedResourcesPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listQueuedResourcesPagedCallable()");
}
public UnaryCallable
listQueuedResourcesCallable() {
throw new UnsupportedOperationException("Not implemented: listQueuedResourcesCallable()");
}
public UnaryCallable getQueuedResourceCallable() {
throw new UnsupportedOperationException("Not implemented: getQueuedResourceCallable()");
}
public OperationCallable
createQueuedResourceOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: createQueuedResourceOperationCallable()");
}
public UnaryCallable createQueuedResourceCallable() {
throw new UnsupportedOperationException("Not implemented: createQueuedResourceCallable()");
}
public OperationCallable
deleteQueuedResourceOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: deleteQueuedResourceOperationCallable()");
}
public UnaryCallable deleteQueuedResourceCallable() {
throw new UnsupportedOperationException("Not implemented: deleteQueuedResourceCallable()");
}
public OperationCallable
resetQueuedResourceOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: resetQueuedResourceOperationCallable()");
}
public UnaryCallable resetQueuedResourceCallable() {
throw new UnsupportedOperationException("Not implemented: resetQueuedResourceCallable()");
}
public UnaryCallable
generateServiceIdentityCallable() {
throw new UnsupportedOperationException("Not implemented: generateServiceIdentityCallable()");
}
public UnaryCallable
listAcceleratorTypesPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listAcceleratorTypesPagedCallable()");
}
public UnaryCallable
listAcceleratorTypesCallable() {
throw new UnsupportedOperationException("Not implemented: listAcceleratorTypesCallable()");
}
public UnaryCallable getAcceleratorTypeCallable() {
throw new UnsupportedOperationException("Not implemented: getAcceleratorTypeCallable()");
}
public UnaryCallable
listRuntimeVersionsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listRuntimeVersionsPagedCallable()");
}
public UnaryCallable
listRuntimeVersionsCallable() {
throw new UnsupportedOperationException("Not implemented: listRuntimeVersionsCallable()");
}
public UnaryCallable getRuntimeVersionCallable() {
throw new UnsupportedOperationException("Not implemented: getRuntimeVersionCallable()");
}
public UnaryCallable
getGuestAttributesCallable() {
throw new UnsupportedOperationException("Not implemented: getGuestAttributesCallable()");
}
public OperationCallable
simulateMaintenanceEventOperationCallable() {
throw new UnsupportedOperationException(
"Not implemented: simulateMaintenanceEventOperationCallable()");
}
public UnaryCallable
simulateMaintenanceEventCallable() {
throw new UnsupportedOperationException("Not implemented: simulateMaintenanceEventCallable()");
}
public UnaryCallable
listLocationsPagedCallable() {
throw new UnsupportedOperationException("Not implemented: listLocationsPagedCallable()");
}
public UnaryCallable listLocationsCallable() {
throw new UnsupportedOperationException("Not implemented: listLocationsCallable()");
}
public UnaryCallable getLocationCallable() {
throw new UnsupportedOperationException("Not implemented: getLocationCallable()");
}
@Override
public abstract void close();
}