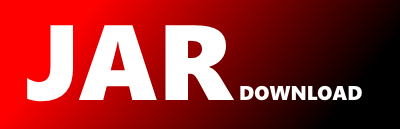
com.google.cloud.workstations.v1.WorkstationsClient Maven / Gradle / Ivy
Show all versions of google-cloud-workstations Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.workstations.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.core.BetaApi;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.httpjson.longrunning.OperationsClient;
import com.google.api.gax.longrunning.OperationFuture;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.OperationCallable;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.cloud.workstations.v1.stub.WorkstationsStub;
import com.google.cloud.workstations.v1.stub.WorkstationsStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.longrunning.Operation;
import com.google.protobuf.FieldMask;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Service for interacting with Cloud Workstations.
*
* This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationClusterName name =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]");
* WorkstationCluster response = workstationsClient.getWorkstationCluster(name);
* }
* }
*
* Note: close() needs to be called on the WorkstationsClient object to clean up resources such
* as threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* GetWorkstationCluster
* Returns the requested workstation cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getWorkstationCluster(GetWorkstationClusterRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getWorkstationCluster(WorkstationClusterName name)
*
getWorkstationCluster(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getWorkstationClusterCallable()
*
*
*
*
* ListWorkstationClusters
* Returns all workstation clusters in the specified location.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listWorkstationClusters(ListWorkstationClustersRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listWorkstationClusters(LocationName parent)
*
listWorkstationClusters(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listWorkstationClustersPagedCallable()
*
listWorkstationClustersCallable()
*
*
*
*
* CreateWorkstationCluster
* Creates a new workstation cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createWorkstationClusterAsync(CreateWorkstationClusterRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createWorkstationClusterAsync(LocationName parent, WorkstationCluster workstationCluster, String workstationClusterId)
*
createWorkstationClusterAsync(String parent, WorkstationCluster workstationCluster, String workstationClusterId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createWorkstationClusterOperationCallable()
*
createWorkstationClusterCallable()
*
*
*
*
* UpdateWorkstationCluster
* Updates an existing workstation cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateWorkstationClusterAsync(UpdateWorkstationClusterRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateWorkstationClusterAsync(WorkstationCluster workstationCluster, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateWorkstationClusterOperationCallable()
*
updateWorkstationClusterCallable()
*
*
*
*
* DeleteWorkstationCluster
* Deletes the specified workstation cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteWorkstationClusterAsync(DeleteWorkstationClusterRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteWorkstationClusterAsync(WorkstationClusterName name)
*
deleteWorkstationClusterAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteWorkstationClusterOperationCallable()
*
deleteWorkstationClusterCallable()
*
*
*
*
* GetWorkstationConfig
* Returns the requested workstation configuration.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getWorkstationConfig(GetWorkstationConfigRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getWorkstationConfig(WorkstationConfigName name)
*
getWorkstationConfig(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getWorkstationConfigCallable()
*
*
*
*
* ListWorkstationConfigs
* Returns all workstation configurations in the specified cluster.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listWorkstationConfigs(ListWorkstationConfigsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listWorkstationConfigs(WorkstationClusterName parent)
*
listWorkstationConfigs(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listWorkstationConfigsPagedCallable()
*
listWorkstationConfigsCallable()
*
*
*
*
* ListUsableWorkstationConfigs
* Returns all workstation configurations in the specified cluster on which the caller has the "workstations.workstation.create" permission.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listUsableWorkstationConfigs(ListUsableWorkstationConfigsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listUsableWorkstationConfigs(WorkstationClusterName parent)
*
listUsableWorkstationConfigs(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listUsableWorkstationConfigsPagedCallable()
*
listUsableWorkstationConfigsCallable()
*
*
*
*
* CreateWorkstationConfig
* Creates a new workstation configuration.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createWorkstationConfigAsync(CreateWorkstationConfigRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createWorkstationConfigAsync(WorkstationClusterName parent, WorkstationConfig workstationConfig, String workstationConfigId)
*
createWorkstationConfigAsync(String parent, WorkstationConfig workstationConfig, String workstationConfigId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createWorkstationConfigOperationCallable()
*
createWorkstationConfigCallable()
*
*
*
*
* UpdateWorkstationConfig
* Updates an existing workstation configuration.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateWorkstationConfigAsync(UpdateWorkstationConfigRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateWorkstationConfigAsync(WorkstationConfig workstationConfig, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateWorkstationConfigOperationCallable()
*
updateWorkstationConfigCallable()
*
*
*
*
* DeleteWorkstationConfig
* Deletes the specified workstation configuration.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteWorkstationConfigAsync(DeleteWorkstationConfigRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteWorkstationConfigAsync(WorkstationConfigName name)
*
deleteWorkstationConfigAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteWorkstationConfigOperationCallable()
*
deleteWorkstationConfigCallable()
*
*
*
*
* GetWorkstation
* Returns the requested workstation.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getWorkstation(GetWorkstationRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getWorkstation(WorkstationName name)
*
getWorkstation(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getWorkstationCallable()
*
*
*
*
* ListWorkstations
* Returns all Workstations using the specified workstation configuration.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listWorkstations(ListWorkstationsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listWorkstations(WorkstationConfigName parent)
*
listWorkstations(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listWorkstationsPagedCallable()
*
listWorkstationsCallable()
*
*
*
*
* ListUsableWorkstations
* Returns all workstations using the specified workstation configuration on which the caller has the "workstations.workstations.use" permission.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listUsableWorkstations(ListUsableWorkstationsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listUsableWorkstations(WorkstationConfigName parent)
*
listUsableWorkstations(String parent)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listUsableWorkstationsPagedCallable()
*
listUsableWorkstationsCallable()
*
*
*
*
* CreateWorkstation
* Creates a new workstation.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createWorkstationAsync(CreateWorkstationRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* createWorkstationAsync(WorkstationConfigName parent, Workstation workstation, String workstationId)
*
createWorkstationAsync(String parent, Workstation workstation, String workstationId)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createWorkstationOperationCallable()
*
createWorkstationCallable()
*
*
*
*
* UpdateWorkstation
* Updates an existing workstation.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateWorkstationAsync(UpdateWorkstationRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* updateWorkstationAsync(Workstation workstation, FieldMask updateMask)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateWorkstationOperationCallable()
*
updateWorkstationCallable()
*
*
*
*
* DeleteWorkstation
* Deletes the specified workstation.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteWorkstationAsync(DeleteWorkstationRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* deleteWorkstationAsync(WorkstationName name)
*
deleteWorkstationAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteWorkstationOperationCallable()
*
deleteWorkstationCallable()
*
*
*
*
* StartWorkstation
* Starts running a workstation so that users can connect to it.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* startWorkstationAsync(StartWorkstationRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* startWorkstationAsync(WorkstationName name)
*
startWorkstationAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* startWorkstationOperationCallable()
*
startWorkstationCallable()
*
*
*
*
* StopWorkstation
* Stops running a workstation, reducing costs.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* stopWorkstationAsync(StopWorkstationRequest request)
*
* Methods that return long-running operations have "Async" method variants that return `OperationFuture`, which is used to track polling of the service.
*
* stopWorkstationAsync(WorkstationName name)
*
stopWorkstationAsync(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* stopWorkstationOperationCallable()
*
stopWorkstationCallable()
*
*
*
*
* GenerateAccessToken
* Returns a short-lived credential that can be used to send authenticated and authorized traffic to a workstation.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* generateAccessToken(GenerateAccessTokenRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* generateAccessToken(WorkstationName workstation)
*
generateAccessToken(String workstation)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* generateAccessTokenCallable()
*
*
*
*
* SetIamPolicy
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* setIamPolicy(SetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* setIamPolicyCallable()
*
*
*
*
* GetIamPolicy
* Gets the access control policy for a resource. Returns an empty policyif the resource exists and does not have a policy set.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getIamPolicy(GetIamPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getIamPolicyCallable()
*
*
*
*
* TestIamPermissions
* Returns permissions that a caller has on the specified resource. If theresource does not exist, this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
Note: This operation is designed to be used for buildingpermission-aware UIs and command-line tools, not for authorizationchecking. This operation may "fail open" without warning.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* testIamPermissions(TestIamPermissionsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* testIamPermissionsCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of WorkstationsSettings to
* create(). For example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* WorkstationsSettings workstationsSettings =
* WorkstationsSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* WorkstationsClient workstationsClient = WorkstationsClient.create(workstationsSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* WorkstationsSettings workstationsSettings =
* WorkstationsSettings.newBuilder().setEndpoint(myEndpoint).build();
* WorkstationsClient workstationsClient = WorkstationsClient.create(workstationsSettings);
* }
*
* To use REST (HTTP1.1/JSON) transport (instead of gRPC) for sending and receiving requests over
* the wire:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* WorkstationsSettings workstationsSettings = WorkstationsSettings.newHttpJsonBuilder().build();
* WorkstationsClient workstationsClient = WorkstationsClient.create(workstationsSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class WorkstationsClient implements BackgroundResource {
private final WorkstationsSettings settings;
private final WorkstationsStub stub;
private final OperationsClient httpJsonOperationsClient;
private final com.google.longrunning.OperationsClient operationsClient;
/** Constructs an instance of WorkstationsClient with default settings. */
public static final WorkstationsClient create() throws IOException {
return create(WorkstationsSettings.newBuilder().build());
}
/**
* Constructs an instance of WorkstationsClient, using the given settings. The channels are
* created based on the settings passed in, or defaults for any settings that are not set.
*/
public static final WorkstationsClient create(WorkstationsSettings settings) throws IOException {
return new WorkstationsClient(settings);
}
/**
* Constructs an instance of WorkstationsClient, using the given stub for making calls. This is
* for advanced usage - prefer using create(WorkstationsSettings).
*/
public static final WorkstationsClient create(WorkstationsStub stub) {
return new WorkstationsClient(stub);
}
/**
* Constructs an instance of WorkstationsClient, using the given settings. This is protected so
* that it is easy to make a subclass, but otherwise, the static factory methods should be
* preferred.
*/
protected WorkstationsClient(WorkstationsSettings settings) throws IOException {
this.settings = settings;
this.stub = ((WorkstationsStubSettings) settings.getStubSettings()).createStub();
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
protected WorkstationsClient(WorkstationsStub stub) {
this.settings = null;
this.stub = stub;
this.operationsClient =
com.google.longrunning.OperationsClient.create(this.stub.getOperationsStub());
this.httpJsonOperationsClient = OperationsClient.create(this.stub.getHttpJsonOperationsStub());
}
public final WorkstationsSettings getSettings() {
return settings;
}
public WorkstationsStub getStub() {
return stub;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
public final com.google.longrunning.OperationsClient getOperationsClient() {
return operationsClient;
}
/**
* Returns the OperationsClient that can be used to query the status of a long-running operation
* returned by another API method call.
*/
@BetaApi
public final OperationsClient getHttpJsonOperationsClient() {
return httpJsonOperationsClient;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation cluster.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationClusterName name =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]");
* WorkstationCluster response = workstationsClient.getWorkstationCluster(name);
* }
* }
*
* @param name Required. Name of the requested resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final WorkstationCluster getWorkstationCluster(WorkstationClusterName name) {
GetWorkstationClusterRequest request =
GetWorkstationClusterRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getWorkstationCluster(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String name =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]").toString();
* WorkstationCluster response = workstationsClient.getWorkstationCluster(name);
* }
* }
*
* @param name Required. Name of the requested resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final WorkstationCluster getWorkstationCluster(String name) {
GetWorkstationClusterRequest request =
GetWorkstationClusterRequest.newBuilder().setName(name).build();
return getWorkstationCluster(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GetWorkstationClusterRequest request =
* GetWorkstationClusterRequest.newBuilder()
* .setName(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .build();
* WorkstationCluster response = workstationsClient.getWorkstationCluster(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final WorkstationCluster getWorkstationCluster(GetWorkstationClusterRequest request) {
return getWorkstationClusterCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GetWorkstationClusterRequest request =
* GetWorkstationClusterRequest.newBuilder()
* .setName(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .build();
* ApiFuture future =
* workstationsClient.getWorkstationClusterCallable().futureCall(request);
* // Do something.
* WorkstationCluster response = future.get();
* }
* }
*/
public final UnaryCallable
getWorkstationClusterCallable() {
return stub.getWorkstationClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation clusters in the specified location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* for (WorkstationCluster element :
* workstationsClient.listWorkstationClusters(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListWorkstationClustersPagedResponse listWorkstationClusters(LocationName parent) {
ListWorkstationClustersRequest request =
ListWorkstationClustersRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listWorkstationClusters(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation clusters in the specified location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* for (WorkstationCluster element :
* workstationsClient.listWorkstationClusters(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListWorkstationClustersPagedResponse listWorkstationClusters(String parent) {
ListWorkstationClustersRequest request =
ListWorkstationClustersRequest.newBuilder().setParent(parent).build();
return listWorkstationClusters(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation clusters in the specified location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListWorkstationClustersRequest request =
* ListWorkstationClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (WorkstationCluster element :
* workstationsClient.listWorkstationClusters(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListWorkstationClustersPagedResponse listWorkstationClusters(
ListWorkstationClustersRequest request) {
return listWorkstationClustersPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation clusters in the specified location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListWorkstationClustersRequest request =
* ListWorkstationClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* workstationsClient.listWorkstationClustersPagedCallable().futureCall(request);
* // Do something.
* for (WorkstationCluster element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listWorkstationClustersPagedCallable() {
return stub.listWorkstationClustersPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation clusters in the specified location.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListWorkstationClustersRequest request =
* ListWorkstationClustersRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListWorkstationClustersResponse response =
* workstationsClient.listWorkstationClustersCallable().call(request);
* for (WorkstationCluster element : response.getWorkstationClustersList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listWorkstationClustersCallable() {
return stub.listWorkstationClustersCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* LocationName parent = LocationName.of("[PROJECT]", "[LOCATION]");
* WorkstationCluster workstationCluster = WorkstationCluster.newBuilder().build();
* String workstationClusterId = "workstationClusterId351421170";
* WorkstationCluster response =
* workstationsClient
* .createWorkstationClusterAsync(parent, workstationCluster, workstationClusterId)
* .get();
* }
* }
*
* @param parent Required. Parent resource name.
* @param workstationCluster Required. Workstation cluster to create.
* @param workstationClusterId Required. ID to use for the workstation cluster.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createWorkstationClusterAsync(
LocationName parent, WorkstationCluster workstationCluster, String workstationClusterId) {
CreateWorkstationClusterRequest request =
CreateWorkstationClusterRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setWorkstationCluster(workstationCluster)
.setWorkstationClusterId(workstationClusterId)
.build();
return createWorkstationClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String parent = LocationName.of("[PROJECT]", "[LOCATION]").toString();
* WorkstationCluster workstationCluster = WorkstationCluster.newBuilder().build();
* String workstationClusterId = "workstationClusterId351421170";
* WorkstationCluster response =
* workstationsClient
* .createWorkstationClusterAsync(parent, workstationCluster, workstationClusterId)
* .get();
* }
* }
*
* @param parent Required. Parent resource name.
* @param workstationCluster Required. Workstation cluster to create.
* @param workstationClusterId Required. ID to use for the workstation cluster.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createWorkstationClusterAsync(
String parent, WorkstationCluster workstationCluster, String workstationClusterId) {
CreateWorkstationClusterRequest request =
CreateWorkstationClusterRequest.newBuilder()
.setParent(parent)
.setWorkstationCluster(workstationCluster)
.setWorkstationClusterId(workstationClusterId)
.build();
return createWorkstationClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* CreateWorkstationClusterRequest request =
* CreateWorkstationClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setWorkstationClusterId("workstationClusterId351421170")
* .setWorkstationCluster(WorkstationCluster.newBuilder().build())
* .setValidateOnly(true)
* .build();
* WorkstationCluster response = workstationsClient.createWorkstationClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createWorkstationClusterAsync(
CreateWorkstationClusterRequest request) {
return createWorkstationClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* CreateWorkstationClusterRequest request =
* CreateWorkstationClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setWorkstationClusterId("workstationClusterId351421170")
* .setWorkstationCluster(WorkstationCluster.newBuilder().build())
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* workstationsClient.createWorkstationClusterOperationCallable().futureCall(request);
* // Do something.
* WorkstationCluster response = future.get();
* }
* }
*/
public final OperationCallable<
CreateWorkstationClusterRequest, WorkstationCluster, OperationMetadata>
createWorkstationClusterOperationCallable() {
return stub.createWorkstationClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* CreateWorkstationClusterRequest request =
* CreateWorkstationClusterRequest.newBuilder()
* .setParent(LocationName.of("[PROJECT]", "[LOCATION]").toString())
* .setWorkstationClusterId("workstationClusterId351421170")
* .setWorkstationCluster(WorkstationCluster.newBuilder().build())
* .setValidateOnly(true)
* .build();
* ApiFuture future =
* workstationsClient.createWorkstationClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
createWorkstationClusterCallable() {
return stub.createWorkstationClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationCluster workstationCluster = WorkstationCluster.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* WorkstationCluster response =
* workstationsClient.updateWorkstationClusterAsync(workstationCluster, updateMask).get();
* }
* }
*
* @param workstationCluster Required. Workstation cluster to update.
* @param updateMask Required. Mask that specifies which fields in the workstation cluster should
* be updated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateWorkstationClusterAsync(
WorkstationCluster workstationCluster, FieldMask updateMask) {
UpdateWorkstationClusterRequest request =
UpdateWorkstationClusterRequest.newBuilder()
.setWorkstationCluster(workstationCluster)
.setUpdateMask(updateMask)
.build();
return updateWorkstationClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* UpdateWorkstationClusterRequest request =
* UpdateWorkstationClusterRequest.newBuilder()
* .setWorkstationCluster(WorkstationCluster.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* WorkstationCluster response = workstationsClient.updateWorkstationClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateWorkstationClusterAsync(
UpdateWorkstationClusterRequest request) {
return updateWorkstationClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* UpdateWorkstationClusterRequest request =
* UpdateWorkstationClusterRequest.newBuilder()
* .setWorkstationCluster(WorkstationCluster.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* OperationFuture future =
* workstationsClient.updateWorkstationClusterOperationCallable().futureCall(request);
* // Do something.
* WorkstationCluster response = future.get();
* }
* }
*/
public final OperationCallable<
UpdateWorkstationClusterRequest, WorkstationCluster, OperationMetadata>
updateWorkstationClusterOperationCallable() {
return stub.updateWorkstationClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* UpdateWorkstationClusterRequest request =
* UpdateWorkstationClusterRequest.newBuilder()
* .setWorkstationCluster(WorkstationCluster.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* ApiFuture future =
* workstationsClient.updateWorkstationClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
updateWorkstationClusterCallable() {
return stub.updateWorkstationClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationClusterName name =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]");
* WorkstationCluster response = workstationsClient.deleteWorkstationClusterAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation cluster to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteWorkstationClusterAsync(
WorkstationClusterName name) {
DeleteWorkstationClusterRequest request =
DeleteWorkstationClusterRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteWorkstationClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String name =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]").toString();
* WorkstationCluster response = workstationsClient.deleteWorkstationClusterAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation cluster to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteWorkstationClusterAsync(
String name) {
DeleteWorkstationClusterRequest request =
DeleteWorkstationClusterRequest.newBuilder().setName(name).build();
return deleteWorkstationClusterAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* DeleteWorkstationClusterRequest request =
* DeleteWorkstationClusterRequest.newBuilder()
* .setName(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* WorkstationCluster response = workstationsClient.deleteWorkstationClusterAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteWorkstationClusterAsync(
DeleteWorkstationClusterRequest request) {
return deleteWorkstationClusterOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* DeleteWorkstationClusterRequest request =
* DeleteWorkstationClusterRequest.newBuilder()
* .setName(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* OperationFuture future =
* workstationsClient.deleteWorkstationClusterOperationCallable().futureCall(request);
* // Do something.
* WorkstationCluster response = future.get();
* }
* }
*/
public final OperationCallable<
DeleteWorkstationClusterRequest, WorkstationCluster, OperationMetadata>
deleteWorkstationClusterOperationCallable() {
return stub.deleteWorkstationClusterOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* DeleteWorkstationClusterRequest request =
* DeleteWorkstationClusterRequest.newBuilder()
* .setName(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* ApiFuture future =
* workstationsClient.deleteWorkstationClusterCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
deleteWorkstationClusterCallable() {
return stub.deleteWorkstationClusterCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationConfigName name =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]");
* WorkstationConfig response = workstationsClient.getWorkstationConfig(name);
* }
* }
*
* @param name Required. Name of the requested resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final WorkstationConfig getWorkstationConfig(WorkstationConfigName name) {
GetWorkstationConfigRequest request =
GetWorkstationConfigRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getWorkstationConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String name =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]")
* .toString();
* WorkstationConfig response = workstationsClient.getWorkstationConfig(name);
* }
* }
*
* @param name Required. Name of the requested resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final WorkstationConfig getWorkstationConfig(String name) {
GetWorkstationConfigRequest request =
GetWorkstationConfigRequest.newBuilder().setName(name).build();
return getWorkstationConfig(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GetWorkstationConfigRequest request =
* GetWorkstationConfigRequest.newBuilder()
* .setName(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .build();
* WorkstationConfig response = workstationsClient.getWorkstationConfig(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final WorkstationConfig getWorkstationConfig(GetWorkstationConfigRequest request) {
return getWorkstationConfigCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GetWorkstationConfigRequest request =
* GetWorkstationConfigRequest.newBuilder()
* .setName(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .build();
* ApiFuture future =
* workstationsClient.getWorkstationConfigCallable().futureCall(request);
* // Do something.
* WorkstationConfig response = future.get();
* }
* }
*/
public final UnaryCallable
getWorkstationConfigCallable() {
return stub.getWorkstationConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationClusterName parent =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]");
* for (WorkstationConfig element :
* workstationsClient.listWorkstationConfigs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListWorkstationConfigsPagedResponse listWorkstationConfigs(
WorkstationClusterName parent) {
ListWorkstationConfigsRequest request =
ListWorkstationConfigsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listWorkstationConfigs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String parent =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]").toString();
* for (WorkstationConfig element :
* workstationsClient.listWorkstationConfigs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListWorkstationConfigsPagedResponse listWorkstationConfigs(String parent) {
ListWorkstationConfigsRequest request =
ListWorkstationConfigsRequest.newBuilder().setParent(parent).build();
return listWorkstationConfigs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListWorkstationConfigsRequest request =
* ListWorkstationConfigsRequest.newBuilder()
* .setParent(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (WorkstationConfig element :
* workstationsClient.listWorkstationConfigs(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListWorkstationConfigsPagedResponse listWorkstationConfigs(
ListWorkstationConfigsRequest request) {
return listWorkstationConfigsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListWorkstationConfigsRequest request =
* ListWorkstationConfigsRequest.newBuilder()
* .setParent(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* workstationsClient.listWorkstationConfigsPagedCallable().futureCall(request);
* // Do something.
* for (WorkstationConfig element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listWorkstationConfigsPagedCallable() {
return stub.listWorkstationConfigsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListWorkstationConfigsRequest request =
* ListWorkstationConfigsRequest.newBuilder()
* .setParent(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListWorkstationConfigsResponse response =
* workstationsClient.listWorkstationConfigsCallable().call(request);
* for (WorkstationConfig element : response.getWorkstationConfigsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listWorkstationConfigsCallable() {
return stub.listWorkstationConfigsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster on which the caller has the
* "workstations.workstation.create" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationClusterName parent =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]");
* for (WorkstationConfig element :
* workstationsClient.listUsableWorkstationConfigs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListUsableWorkstationConfigsPagedResponse listUsableWorkstationConfigs(
WorkstationClusterName parent) {
ListUsableWorkstationConfigsRequest request =
ListUsableWorkstationConfigsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listUsableWorkstationConfigs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster on which the caller has the
* "workstations.workstation.create" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String parent =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]").toString();
* for (WorkstationConfig element :
* workstationsClient.listUsableWorkstationConfigs(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListUsableWorkstationConfigsPagedResponse listUsableWorkstationConfigs(
String parent) {
ListUsableWorkstationConfigsRequest request =
ListUsableWorkstationConfigsRequest.newBuilder().setParent(parent).build();
return listUsableWorkstationConfigs(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster on which the caller has the
* "workstations.workstation.create" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListUsableWorkstationConfigsRequest request =
* ListUsableWorkstationConfigsRequest.newBuilder()
* .setParent(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (WorkstationConfig element :
* workstationsClient.listUsableWorkstationConfigs(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListUsableWorkstationConfigsPagedResponse listUsableWorkstationConfigs(
ListUsableWorkstationConfigsRequest request) {
return listUsableWorkstationConfigsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster on which the caller has the
* "workstations.workstation.create" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListUsableWorkstationConfigsRequest request =
* ListUsableWorkstationConfigsRequest.newBuilder()
* .setParent(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* workstationsClient.listUsableWorkstationConfigsPagedCallable().futureCall(request);
* // Do something.
* for (WorkstationConfig element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable<
ListUsableWorkstationConfigsRequest, ListUsableWorkstationConfigsPagedResponse>
listUsableWorkstationConfigsPagedCallable() {
return stub.listUsableWorkstationConfigsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstation configurations in the specified cluster on which the caller has the
* "workstations.workstation.create" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListUsableWorkstationConfigsRequest request =
* ListUsableWorkstationConfigsRequest.newBuilder()
* .setParent(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListUsableWorkstationConfigsResponse response =
* workstationsClient.listUsableWorkstationConfigsCallable().call(request);
* for (WorkstationConfig element : response.getWorkstationConfigsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable<
ListUsableWorkstationConfigsRequest, ListUsableWorkstationConfigsResponse>
listUsableWorkstationConfigsCallable() {
return stub.listUsableWorkstationConfigsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationClusterName parent =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]");
* WorkstationConfig workstationConfig = WorkstationConfig.newBuilder().build();
* String workstationConfigId = "workstationConfigId798542368";
* WorkstationConfig response =
* workstationsClient
* .createWorkstationConfigAsync(parent, workstationConfig, workstationConfigId)
* .get();
* }
* }
*
* @param parent Required. Parent resource name.
* @param workstationConfig Required. Config to create.
* @param workstationConfigId Required. ID to use for the workstation configuration.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createWorkstationConfigAsync(
WorkstationClusterName parent,
WorkstationConfig workstationConfig,
String workstationConfigId) {
CreateWorkstationConfigRequest request =
CreateWorkstationConfigRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setWorkstationConfig(workstationConfig)
.setWorkstationConfigId(workstationConfigId)
.build();
return createWorkstationConfigAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String parent =
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]").toString();
* WorkstationConfig workstationConfig = WorkstationConfig.newBuilder().build();
* String workstationConfigId = "workstationConfigId798542368";
* WorkstationConfig response =
* workstationsClient
* .createWorkstationConfigAsync(parent, workstationConfig, workstationConfigId)
* .get();
* }
* }
*
* @param parent Required. Parent resource name.
* @param workstationConfig Required. Config to create.
* @param workstationConfigId Required. ID to use for the workstation configuration.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createWorkstationConfigAsync(
String parent, WorkstationConfig workstationConfig, String workstationConfigId) {
CreateWorkstationConfigRequest request =
CreateWorkstationConfigRequest.newBuilder()
.setParent(parent)
.setWorkstationConfig(workstationConfig)
.setWorkstationConfigId(workstationConfigId)
.build();
return createWorkstationConfigAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* CreateWorkstationConfigRequest request =
* CreateWorkstationConfigRequest.newBuilder()
* .setParent(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setWorkstationConfigId("workstationConfigId798542368")
* .setWorkstationConfig(WorkstationConfig.newBuilder().build())
* .setValidateOnly(true)
* .build();
* WorkstationConfig response = workstationsClient.createWorkstationConfigAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createWorkstationConfigAsync(
CreateWorkstationConfigRequest request) {
return createWorkstationConfigOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* CreateWorkstationConfigRequest request =
* CreateWorkstationConfigRequest.newBuilder()
* .setParent(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setWorkstationConfigId("workstationConfigId798542368")
* .setWorkstationConfig(WorkstationConfig.newBuilder().build())
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* workstationsClient.createWorkstationConfigOperationCallable().futureCall(request);
* // Do something.
* WorkstationConfig response = future.get();
* }
* }
*/
public final OperationCallable<
CreateWorkstationConfigRequest, WorkstationConfig, OperationMetadata>
createWorkstationConfigOperationCallable() {
return stub.createWorkstationConfigOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* CreateWorkstationConfigRequest request =
* CreateWorkstationConfigRequest.newBuilder()
* .setParent(
* WorkstationClusterName.of("[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]")
* .toString())
* .setWorkstationConfigId("workstationConfigId798542368")
* .setWorkstationConfig(WorkstationConfig.newBuilder().build())
* .setValidateOnly(true)
* .build();
* ApiFuture future =
* workstationsClient.createWorkstationConfigCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
createWorkstationConfigCallable() {
return stub.createWorkstationConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationConfig workstationConfig = WorkstationConfig.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* WorkstationConfig response =
* workstationsClient.updateWorkstationConfigAsync(workstationConfig, updateMask).get();
* }
* }
*
* @param workstationConfig Required. Config to update.
* @param updateMask Required. Mask specifying which fields in the workstation configuration
* should be updated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateWorkstationConfigAsync(
WorkstationConfig workstationConfig, FieldMask updateMask) {
UpdateWorkstationConfigRequest request =
UpdateWorkstationConfigRequest.newBuilder()
.setWorkstationConfig(workstationConfig)
.setUpdateMask(updateMask)
.build();
return updateWorkstationConfigAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* UpdateWorkstationConfigRequest request =
* UpdateWorkstationConfigRequest.newBuilder()
* .setWorkstationConfig(WorkstationConfig.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* WorkstationConfig response = workstationsClient.updateWorkstationConfigAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateWorkstationConfigAsync(
UpdateWorkstationConfigRequest request) {
return updateWorkstationConfigOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* UpdateWorkstationConfigRequest request =
* UpdateWorkstationConfigRequest.newBuilder()
* .setWorkstationConfig(WorkstationConfig.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* OperationFuture future =
* workstationsClient.updateWorkstationConfigOperationCallable().futureCall(request);
* // Do something.
* WorkstationConfig response = future.get();
* }
* }
*/
public final OperationCallable<
UpdateWorkstationConfigRequest, WorkstationConfig, OperationMetadata>
updateWorkstationConfigOperationCallable() {
return stub.updateWorkstationConfigOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* UpdateWorkstationConfigRequest request =
* UpdateWorkstationConfigRequest.newBuilder()
* .setWorkstationConfig(WorkstationConfig.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* ApiFuture future =
* workstationsClient.updateWorkstationConfigCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
updateWorkstationConfigCallable() {
return stub.updateWorkstationConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationConfigName name =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]");
* WorkstationConfig response = workstationsClient.deleteWorkstationConfigAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation configuration to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteWorkstationConfigAsync(
WorkstationConfigName name) {
DeleteWorkstationConfigRequest request =
DeleteWorkstationConfigRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteWorkstationConfigAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String name =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]")
* .toString();
* WorkstationConfig response = workstationsClient.deleteWorkstationConfigAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation configuration to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteWorkstationConfigAsync(
String name) {
DeleteWorkstationConfigRequest request =
DeleteWorkstationConfigRequest.newBuilder().setName(name).build();
return deleteWorkstationConfigAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* DeleteWorkstationConfigRequest request =
* DeleteWorkstationConfigRequest.newBuilder()
* .setName(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* WorkstationConfig response = workstationsClient.deleteWorkstationConfigAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteWorkstationConfigAsync(
DeleteWorkstationConfigRequest request) {
return deleteWorkstationConfigOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* DeleteWorkstationConfigRequest request =
* DeleteWorkstationConfigRequest.newBuilder()
* .setName(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* OperationFuture future =
* workstationsClient.deleteWorkstationConfigOperationCallable().futureCall(request);
* // Do something.
* WorkstationConfig response = future.get();
* }
* }
*/
public final OperationCallable<
DeleteWorkstationConfigRequest, WorkstationConfig, OperationMetadata>
deleteWorkstationConfigOperationCallable() {
return stub.deleteWorkstationConfigOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* DeleteWorkstationConfigRequest request =
* DeleteWorkstationConfigRequest.newBuilder()
* .setName(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .setForce(true)
* .build();
* ApiFuture future =
* workstationsClient.deleteWorkstationConfigCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable
deleteWorkstationConfigCallable() {
return stub.deleteWorkstationConfigCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationName name =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]");
* Workstation response = workstationsClient.getWorkstation(name);
* }
* }
*
* @param name Required. Name of the requested resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Workstation getWorkstation(WorkstationName name) {
GetWorkstationRequest request =
GetWorkstationRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return getWorkstation(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String name =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString();
* Workstation response = workstationsClient.getWorkstation(name);
* }
* }
*
* @param name Required. Name of the requested resource.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Workstation getWorkstation(String name) {
GetWorkstationRequest request = GetWorkstationRequest.newBuilder().setName(name).build();
return getWorkstation(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GetWorkstationRequest request =
* GetWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .build();
* Workstation response = workstationsClient.getWorkstation(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Workstation getWorkstation(GetWorkstationRequest request) {
return getWorkstationCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns the requested workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GetWorkstationRequest request =
* GetWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .build();
* ApiFuture future =
* workstationsClient.getWorkstationCallable().futureCall(request);
* // Do something.
* Workstation response = future.get();
* }
* }
*/
public final UnaryCallable getWorkstationCallable() {
return stub.getWorkstationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all Workstations using the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationConfigName parent =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]");
* for (Workstation element : workstationsClient.listWorkstations(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListWorkstationsPagedResponse listWorkstations(WorkstationConfigName parent) {
ListWorkstationsRequest request =
ListWorkstationsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listWorkstations(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all Workstations using the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String parent =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]")
* .toString();
* for (Workstation element : workstationsClient.listWorkstations(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListWorkstationsPagedResponse listWorkstations(String parent) {
ListWorkstationsRequest request =
ListWorkstationsRequest.newBuilder().setParent(parent).build();
return listWorkstations(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all Workstations using the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListWorkstationsRequest request =
* ListWorkstationsRequest.newBuilder()
* .setParent(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Workstation element : workstationsClient.listWorkstations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListWorkstationsPagedResponse listWorkstations(ListWorkstationsRequest request) {
return listWorkstationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all Workstations using the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListWorkstationsRequest request =
* ListWorkstationsRequest.newBuilder()
* .setParent(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* workstationsClient.listWorkstationsPagedCallable().futureCall(request);
* // Do something.
* for (Workstation element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listWorkstationsPagedCallable() {
return stub.listWorkstationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all Workstations using the specified workstation configuration.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListWorkstationsRequest request =
* ListWorkstationsRequest.newBuilder()
* .setParent(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListWorkstationsResponse response =
* workstationsClient.listWorkstationsCallable().call(request);
* for (Workstation element : response.getWorkstationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listWorkstationsCallable() {
return stub.listWorkstationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstations using the specified workstation configuration on which the caller has
* the "workstations.workstations.use" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationConfigName parent =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]");
* for (Workstation element : workstationsClient.listUsableWorkstations(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListUsableWorkstationsPagedResponse listUsableWorkstations(
WorkstationConfigName parent) {
ListUsableWorkstationsRequest request =
ListUsableWorkstationsRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.build();
return listUsableWorkstations(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstations using the specified workstation configuration on which the caller has
* the "workstations.workstations.use" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String parent =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]")
* .toString();
* for (Workstation element : workstationsClient.listUsableWorkstations(parent).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param parent Required. Parent resource name.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListUsableWorkstationsPagedResponse listUsableWorkstations(String parent) {
ListUsableWorkstationsRequest request =
ListUsableWorkstationsRequest.newBuilder().setParent(parent).build();
return listUsableWorkstations(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstations using the specified workstation configuration on which the caller has
* the "workstations.workstations.use" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListUsableWorkstationsRequest request =
* ListUsableWorkstationsRequest.newBuilder()
* .setParent(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Workstation element : workstationsClient.listUsableWorkstations(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListUsableWorkstationsPagedResponse listUsableWorkstations(
ListUsableWorkstationsRequest request) {
return listUsableWorkstationsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstations using the specified workstation configuration on which the caller has
* the "workstations.workstations.use" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListUsableWorkstationsRequest request =
* ListUsableWorkstationsRequest.newBuilder()
* .setParent(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* workstationsClient.listUsableWorkstationsPagedCallable().futureCall(request);
* // Do something.
* for (Workstation element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listUsableWorkstationsPagedCallable() {
return stub.listUsableWorkstationsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns all workstations using the specified workstation configuration on which the caller has
* the "workstations.workstations.use" permission.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* ListUsableWorkstationsRequest request =
* ListUsableWorkstationsRequest.newBuilder()
* .setParent(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListUsableWorkstationsResponse response =
* workstationsClient.listUsableWorkstationsCallable().call(request);
* for (Workstation element : response.getWorkstationsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listUsableWorkstationsCallable() {
return stub.listUsableWorkstationsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationConfigName parent =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]");
* Workstation workstation = Workstation.newBuilder().build();
* String workstationId = "workstationId560540030";
* Workstation response =
* workstationsClient.createWorkstationAsync(parent, workstation, workstationId).get();
* }
* }
*
* @param parent Required. Parent resource name.
* @param workstation Required. Workstation to create.
* @param workstationId Required. ID to use for the workstation.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createWorkstationAsync(
WorkstationConfigName parent, Workstation workstation, String workstationId) {
CreateWorkstationRequest request =
CreateWorkstationRequest.newBuilder()
.setParent(parent == null ? null : parent.toString())
.setWorkstation(workstation)
.setWorkstationId(workstationId)
.build();
return createWorkstationAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String parent =
* WorkstationConfigName.of(
* "[PROJECT]", "[LOCATION]", "[WORKSTATION_CLUSTER]", "[WORKSTATION_CONFIG]")
* .toString();
* Workstation workstation = Workstation.newBuilder().build();
* String workstationId = "workstationId560540030";
* Workstation response =
* workstationsClient.createWorkstationAsync(parent, workstation, workstationId).get();
* }
* }
*
* @param parent Required. Parent resource name.
* @param workstation Required. Workstation to create.
* @param workstationId Required. ID to use for the workstation.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createWorkstationAsync(
String parent, Workstation workstation, String workstationId) {
CreateWorkstationRequest request =
CreateWorkstationRequest.newBuilder()
.setParent(parent)
.setWorkstation(workstation)
.setWorkstationId(workstationId)
.build();
return createWorkstationAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* CreateWorkstationRequest request =
* CreateWorkstationRequest.newBuilder()
* .setParent(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setWorkstationId("workstationId560540030")
* .setWorkstation(Workstation.newBuilder().build())
* .setValidateOnly(true)
* .build();
* Workstation response = workstationsClient.createWorkstationAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture createWorkstationAsync(
CreateWorkstationRequest request) {
return createWorkstationOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* CreateWorkstationRequest request =
* CreateWorkstationRequest.newBuilder()
* .setParent(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setWorkstationId("workstationId560540030")
* .setWorkstation(Workstation.newBuilder().build())
* .setValidateOnly(true)
* .build();
* OperationFuture future =
* workstationsClient.createWorkstationOperationCallable().futureCall(request);
* // Do something.
* Workstation response = future.get();
* }
* }
*/
public final OperationCallable
createWorkstationOperationCallable() {
return stub.createWorkstationOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* CreateWorkstationRequest request =
* CreateWorkstationRequest.newBuilder()
* .setParent(
* WorkstationConfigName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]")
* .toString())
* .setWorkstationId("workstationId560540030")
* .setWorkstation(Workstation.newBuilder().build())
* .setValidateOnly(true)
* .build();
* ApiFuture future =
* workstationsClient.createWorkstationCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable createWorkstationCallable() {
return stub.createWorkstationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* Workstation workstation = Workstation.newBuilder().build();
* FieldMask updateMask = FieldMask.newBuilder().build();
* Workstation response =
* workstationsClient.updateWorkstationAsync(workstation, updateMask).get();
* }
* }
*
* @param workstation Required. Workstation to update.
* @param updateMask Required. Mask specifying which fields in the workstation configuration
* should be updated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateWorkstationAsync(
Workstation workstation, FieldMask updateMask) {
UpdateWorkstationRequest request =
UpdateWorkstationRequest.newBuilder()
.setWorkstation(workstation)
.setUpdateMask(updateMask)
.build();
return updateWorkstationAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* UpdateWorkstationRequest request =
* UpdateWorkstationRequest.newBuilder()
* .setWorkstation(Workstation.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* Workstation response = workstationsClient.updateWorkstationAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture updateWorkstationAsync(
UpdateWorkstationRequest request) {
return updateWorkstationOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* UpdateWorkstationRequest request =
* UpdateWorkstationRequest.newBuilder()
* .setWorkstation(Workstation.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* OperationFuture future =
* workstationsClient.updateWorkstationOperationCallable().futureCall(request);
* // Do something.
* Workstation response = future.get();
* }
* }
*/
public final OperationCallable
updateWorkstationOperationCallable() {
return stub.updateWorkstationOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates an existing workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* UpdateWorkstationRequest request =
* UpdateWorkstationRequest.newBuilder()
* .setWorkstation(Workstation.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .setValidateOnly(true)
* .setAllowMissing(true)
* .build();
* ApiFuture future =
* workstationsClient.updateWorkstationCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable updateWorkstationCallable() {
return stub.updateWorkstationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationName name =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]");
* Workstation response = workstationsClient.deleteWorkstationAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteWorkstationAsync(
WorkstationName name) {
DeleteWorkstationRequest request =
DeleteWorkstationRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return deleteWorkstationAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String name =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString();
* Workstation response = workstationsClient.deleteWorkstationAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation to delete.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteWorkstationAsync(String name) {
DeleteWorkstationRequest request = DeleteWorkstationRequest.newBuilder().setName(name).build();
return deleteWorkstationAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* DeleteWorkstationRequest request =
* DeleteWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* Workstation response = workstationsClient.deleteWorkstationAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture deleteWorkstationAsync(
DeleteWorkstationRequest request) {
return deleteWorkstationOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* DeleteWorkstationRequest request =
* DeleteWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* OperationFuture future =
* workstationsClient.deleteWorkstationOperationCallable().futureCall(request);
* // Do something.
* Workstation response = future.get();
* }
* }
*/
public final OperationCallable
deleteWorkstationOperationCallable() {
return stub.deleteWorkstationOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes the specified workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* DeleteWorkstationRequest request =
* DeleteWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* ApiFuture future =
* workstationsClient.deleteWorkstationCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable deleteWorkstationCallable() {
return stub.deleteWorkstationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts running a workstation so that users can connect to it.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationName name =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]");
* Workstation response = workstationsClient.startWorkstationAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation to start.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture startWorkstationAsync(
WorkstationName name) {
StartWorkstationRequest request =
StartWorkstationRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return startWorkstationAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts running a workstation so that users can connect to it.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String name =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString();
* Workstation response = workstationsClient.startWorkstationAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation to start.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture startWorkstationAsync(String name) {
StartWorkstationRequest request = StartWorkstationRequest.newBuilder().setName(name).build();
return startWorkstationAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts running a workstation so that users can connect to it.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* StartWorkstationRequest request =
* StartWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* Workstation response = workstationsClient.startWorkstationAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture startWorkstationAsync(
StartWorkstationRequest request) {
return startWorkstationOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts running a workstation so that users can connect to it.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* StartWorkstationRequest request =
* StartWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* OperationFuture future =
* workstationsClient.startWorkstationOperationCallable().futureCall(request);
* // Do something.
* Workstation response = future.get();
* }
* }
*/
public final OperationCallable
startWorkstationOperationCallable() {
return stub.startWorkstationOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Starts running a workstation so that users can connect to it.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* StartWorkstationRequest request =
* StartWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* ApiFuture future =
* workstationsClient.startWorkstationCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable startWorkstationCallable() {
return stub.startWorkstationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops running a workstation, reducing costs.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationName name =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]");
* Workstation response = workstationsClient.stopWorkstationAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation to stop.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture stopWorkstationAsync(
WorkstationName name) {
StopWorkstationRequest request =
StopWorkstationRequest.newBuilder().setName(name == null ? null : name.toString()).build();
return stopWorkstationAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops running a workstation, reducing costs.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String name =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString();
* Workstation response = workstationsClient.stopWorkstationAsync(name).get();
* }
* }
*
* @param name Required. Name of the workstation to stop.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture stopWorkstationAsync(String name) {
StopWorkstationRequest request = StopWorkstationRequest.newBuilder().setName(name).build();
return stopWorkstationAsync(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops running a workstation, reducing costs.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* StopWorkstationRequest request =
* StopWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* Workstation response = workstationsClient.stopWorkstationAsync(request).get();
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final OperationFuture stopWorkstationAsync(
StopWorkstationRequest request) {
return stopWorkstationOperationCallable().futureCall(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops running a workstation, reducing costs.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* StopWorkstationRequest request =
* StopWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* OperationFuture future =
* workstationsClient.stopWorkstationOperationCallable().futureCall(request);
* // Do something.
* Workstation response = future.get();
* }
* }
*/
public final OperationCallable
stopWorkstationOperationCallable() {
return stub.stopWorkstationOperationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Stops running a workstation, reducing costs.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* StopWorkstationRequest request =
* StopWorkstationRequest.newBuilder()
* .setName(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setValidateOnly(true)
* .setEtag("etag3123477")
* .build();
* ApiFuture future =
* workstationsClient.stopWorkstationCallable().futureCall(request);
* // Do something.
* Operation response = future.get();
* }
* }
*/
public final UnaryCallable stopWorkstationCallable() {
return stub.stopWorkstationCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a short-lived credential that can be used to send authenticated and authorized traffic
* to a workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* WorkstationName workstation =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]");
* GenerateAccessTokenResponse response = workstationsClient.generateAccessToken(workstation);
* }
* }
*
* @param workstation Required. Name of the workstation for which the access token should be
* generated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAccessTokenResponse generateAccessToken(WorkstationName workstation) {
GenerateAccessTokenRequest request =
GenerateAccessTokenRequest.newBuilder()
.setWorkstation(workstation == null ? null : workstation.toString())
.build();
return generateAccessToken(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a short-lived credential that can be used to send authenticated and authorized traffic
* to a workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* String workstation =
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString();
* GenerateAccessTokenResponse response = workstationsClient.generateAccessToken(workstation);
* }
* }
*
* @param workstation Required. Name of the workstation for which the access token should be
* generated.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAccessTokenResponse generateAccessToken(String workstation) {
GenerateAccessTokenRequest request =
GenerateAccessTokenRequest.newBuilder().setWorkstation(workstation).build();
return generateAccessToken(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a short-lived credential that can be used to send authenticated and authorized traffic
* to a workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GenerateAccessTokenRequest request =
* GenerateAccessTokenRequest.newBuilder()
* .setWorkstation(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .build();
* GenerateAccessTokenResponse response = workstationsClient.generateAccessToken(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final GenerateAccessTokenResponse generateAccessToken(GenerateAccessTokenRequest request) {
return generateAccessTokenCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a short-lived credential that can be used to send authenticated and authorized traffic
* to a workstation.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GenerateAccessTokenRequest request =
* GenerateAccessTokenRequest.newBuilder()
* .setWorkstation(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .build();
* ApiFuture future =
* workstationsClient.generateAccessTokenCallable().futureCall(request);
* // Do something.
* GenerateAccessTokenResponse response = future.get();
* }
* }
*/
public final UnaryCallable
generateAccessTokenCallable() {
return stub.generateAccessTokenCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Policy response = workstationsClient.setIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(SetIamPolicyRequest request) {
return setIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the access control policy on the specified resource. Replacesany existing policy.
*
* Can return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED`errors.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = workstationsClient.setIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable setIamPolicyCallable() {
return stub.setIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* Policy response = workstationsClient.getIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(GetIamPolicyRequest request) {
return getIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the access control policy for a resource. Returns an empty policyif the resource exists
* and does not have a policy set.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* ApiFuture future = workstationsClient.getIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable getIamPolicyCallable() {
return stub.getIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .addAllPermissions(new ArrayList())
* .build();
* TestIamPermissionsResponse response = workstationsClient.testIamPermissions(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(TestIamPermissionsRequest request) {
return testIamPermissionsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns permissions that a caller has on the specified resource. If theresource does not exist,
* this will return an empty set ofpermissions, not a `NOT_FOUND` error.
*
* Note: This operation is designed to be used for buildingpermission-aware UIs and
* command-line tools, not for authorizationchecking. This operation may "fail open" without
* warning.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (WorkstationsClient workstationsClient = WorkstationsClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(
* WorkstationName.of(
* "[PROJECT]",
* "[LOCATION]",
* "[WORKSTATION_CLUSTER]",
* "[WORKSTATION_CONFIG]",
* "[WORKSTATION]")
* .toString())
* .addAllPermissions(new ArrayList())
* .build();
* ApiFuture future =
* workstationsClient.testIamPermissionsCallable().futureCall(request);
* // Do something.
* TestIamPermissionsResponse response = future.get();
* }
* }
*/
public final UnaryCallable
testIamPermissionsCallable() {
return stub.testIamPermissionsCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListWorkstationClustersPagedResponse
extends AbstractPagedListResponse<
ListWorkstationClustersRequest,
ListWorkstationClustersResponse,
WorkstationCluster,
ListWorkstationClustersPage,
ListWorkstationClustersFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListWorkstationClustersRequest, ListWorkstationClustersResponse, WorkstationCluster>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListWorkstationClustersPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListWorkstationClustersPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListWorkstationClustersPagedResponse(ListWorkstationClustersPage page) {
super(page, ListWorkstationClustersFixedSizeCollection.createEmptyCollection());
}
}
public static class ListWorkstationClustersPage
extends AbstractPage<
ListWorkstationClustersRequest,
ListWorkstationClustersResponse,
WorkstationCluster,
ListWorkstationClustersPage> {
private ListWorkstationClustersPage(
PageContext<
ListWorkstationClustersRequest, ListWorkstationClustersResponse, WorkstationCluster>
context,
ListWorkstationClustersResponse response) {
super(context, response);
}
private static ListWorkstationClustersPage createEmptyPage() {
return new ListWorkstationClustersPage(null, null);
}
@Override
protected ListWorkstationClustersPage createPage(
PageContext<
ListWorkstationClustersRequest, ListWorkstationClustersResponse, WorkstationCluster>
context,
ListWorkstationClustersResponse response) {
return new ListWorkstationClustersPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListWorkstationClustersRequest, ListWorkstationClustersResponse, WorkstationCluster>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListWorkstationClustersFixedSizeCollection
extends AbstractFixedSizeCollection<
ListWorkstationClustersRequest,
ListWorkstationClustersResponse,
WorkstationCluster,
ListWorkstationClustersPage,
ListWorkstationClustersFixedSizeCollection> {
private ListWorkstationClustersFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListWorkstationClustersFixedSizeCollection createEmptyCollection() {
return new ListWorkstationClustersFixedSizeCollection(null, 0);
}
@Override
protected ListWorkstationClustersFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListWorkstationClustersFixedSizeCollection(pages, collectionSize);
}
}
public static class ListWorkstationConfigsPagedResponse
extends AbstractPagedListResponse<
ListWorkstationConfigsRequest,
ListWorkstationConfigsResponse,
WorkstationConfig,
ListWorkstationConfigsPage,
ListWorkstationConfigsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListWorkstationConfigsRequest, ListWorkstationConfigsResponse, WorkstationConfig>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListWorkstationConfigsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListWorkstationConfigsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListWorkstationConfigsPagedResponse(ListWorkstationConfigsPage page) {
super(page, ListWorkstationConfigsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListWorkstationConfigsPage
extends AbstractPage<
ListWorkstationConfigsRequest,
ListWorkstationConfigsResponse,
WorkstationConfig,
ListWorkstationConfigsPage> {
private ListWorkstationConfigsPage(
PageContext<
ListWorkstationConfigsRequest, ListWorkstationConfigsResponse, WorkstationConfig>
context,
ListWorkstationConfigsResponse response) {
super(context, response);
}
private static ListWorkstationConfigsPage createEmptyPage() {
return new ListWorkstationConfigsPage(null, null);
}
@Override
protected ListWorkstationConfigsPage createPage(
PageContext<
ListWorkstationConfigsRequest, ListWorkstationConfigsResponse, WorkstationConfig>
context,
ListWorkstationConfigsResponse response) {
return new ListWorkstationConfigsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListWorkstationConfigsRequest, ListWorkstationConfigsResponse, WorkstationConfig>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListWorkstationConfigsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListWorkstationConfigsRequest,
ListWorkstationConfigsResponse,
WorkstationConfig,
ListWorkstationConfigsPage,
ListWorkstationConfigsFixedSizeCollection> {
private ListWorkstationConfigsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListWorkstationConfigsFixedSizeCollection createEmptyCollection() {
return new ListWorkstationConfigsFixedSizeCollection(null, 0);
}
@Override
protected ListWorkstationConfigsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListWorkstationConfigsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListUsableWorkstationConfigsPagedResponse
extends AbstractPagedListResponse<
ListUsableWorkstationConfigsRequest,
ListUsableWorkstationConfigsResponse,
WorkstationConfig,
ListUsableWorkstationConfigsPage,
ListUsableWorkstationConfigsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext<
ListUsableWorkstationConfigsRequest,
ListUsableWorkstationConfigsResponse,
WorkstationConfig>
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListUsableWorkstationConfigsPage.createEmptyPage()
.createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListUsableWorkstationConfigsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListUsableWorkstationConfigsPagedResponse(ListUsableWorkstationConfigsPage page) {
super(page, ListUsableWorkstationConfigsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListUsableWorkstationConfigsPage
extends AbstractPage<
ListUsableWorkstationConfigsRequest,
ListUsableWorkstationConfigsResponse,
WorkstationConfig,
ListUsableWorkstationConfigsPage> {
private ListUsableWorkstationConfigsPage(
PageContext<
ListUsableWorkstationConfigsRequest,
ListUsableWorkstationConfigsResponse,
WorkstationConfig>
context,
ListUsableWorkstationConfigsResponse response) {
super(context, response);
}
private static ListUsableWorkstationConfigsPage createEmptyPage() {
return new ListUsableWorkstationConfigsPage(null, null);
}
@Override
protected ListUsableWorkstationConfigsPage createPage(
PageContext<
ListUsableWorkstationConfigsRequest,
ListUsableWorkstationConfigsResponse,
WorkstationConfig>
context,
ListUsableWorkstationConfigsResponse response) {
return new ListUsableWorkstationConfigsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext<
ListUsableWorkstationConfigsRequest,
ListUsableWorkstationConfigsResponse,
WorkstationConfig>
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListUsableWorkstationConfigsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListUsableWorkstationConfigsRequest,
ListUsableWorkstationConfigsResponse,
WorkstationConfig,
ListUsableWorkstationConfigsPage,
ListUsableWorkstationConfigsFixedSizeCollection> {
private ListUsableWorkstationConfigsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListUsableWorkstationConfigsFixedSizeCollection createEmptyCollection() {
return new ListUsableWorkstationConfigsFixedSizeCollection(null, 0);
}
@Override
protected ListUsableWorkstationConfigsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListUsableWorkstationConfigsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListWorkstationsPagedResponse
extends AbstractPagedListResponse<
ListWorkstationsRequest,
ListWorkstationsResponse,
Workstation,
ListWorkstationsPage,
ListWorkstationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListWorkstationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListWorkstationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListWorkstationsPagedResponse(ListWorkstationsPage page) {
super(page, ListWorkstationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListWorkstationsPage
extends AbstractPage<
ListWorkstationsRequest, ListWorkstationsResponse, Workstation, ListWorkstationsPage> {
private ListWorkstationsPage(
PageContext context,
ListWorkstationsResponse response) {
super(context, response);
}
private static ListWorkstationsPage createEmptyPage() {
return new ListWorkstationsPage(null, null);
}
@Override
protected ListWorkstationsPage createPage(
PageContext context,
ListWorkstationsResponse response) {
return new ListWorkstationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListWorkstationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListWorkstationsRequest,
ListWorkstationsResponse,
Workstation,
ListWorkstationsPage,
ListWorkstationsFixedSizeCollection> {
private ListWorkstationsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListWorkstationsFixedSizeCollection createEmptyCollection() {
return new ListWorkstationsFixedSizeCollection(null, 0);
}
@Override
protected ListWorkstationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListWorkstationsFixedSizeCollection(pages, collectionSize);
}
}
public static class ListUsableWorkstationsPagedResponse
extends AbstractPagedListResponse<
ListUsableWorkstationsRequest,
ListUsableWorkstationsResponse,
Workstation,
ListUsableWorkstationsPage,
ListUsableWorkstationsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListUsableWorkstationsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListUsableWorkstationsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListUsableWorkstationsPagedResponse(ListUsableWorkstationsPage page) {
super(page, ListUsableWorkstationsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListUsableWorkstationsPage
extends AbstractPage<
ListUsableWorkstationsRequest,
ListUsableWorkstationsResponse,
Workstation,
ListUsableWorkstationsPage> {
private ListUsableWorkstationsPage(
PageContext
context,
ListUsableWorkstationsResponse response) {
super(context, response);
}
private static ListUsableWorkstationsPage createEmptyPage() {
return new ListUsableWorkstationsPage(null, null);
}
@Override
protected ListUsableWorkstationsPage createPage(
PageContext
context,
ListUsableWorkstationsResponse response) {
return new ListUsableWorkstationsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListUsableWorkstationsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListUsableWorkstationsRequest,
ListUsableWorkstationsResponse,
Workstation,
ListUsableWorkstationsPage,
ListUsableWorkstationsFixedSizeCollection> {
private ListUsableWorkstationsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListUsableWorkstationsFixedSizeCollection createEmptyCollection() {
return new ListUsableWorkstationsFixedSizeCollection(null, 0);
}
@Override
protected ListUsableWorkstationsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListUsableWorkstationsFixedSizeCollection(pages, collectionSize);
}
}
}