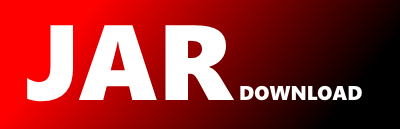
com.google.cloud.iam.admin.v1.IAMClient Maven / Gradle / Ivy
Show all versions of google-iam-admin Show documentation
/*
* Copyright 2024 Google LLC
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* https://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.google.cloud.iam.admin.v1;
import com.google.api.core.ApiFuture;
import com.google.api.core.ApiFutures;
import com.google.api.gax.core.BackgroundResource;
import com.google.api.gax.paging.AbstractFixedSizeCollection;
import com.google.api.gax.paging.AbstractPage;
import com.google.api.gax.paging.AbstractPagedListResponse;
import com.google.api.gax.rpc.PageContext;
import com.google.api.gax.rpc.UnaryCallable;
import com.google.api.resourcenames.ResourceName;
import com.google.cloud.iam.admin.v1.stub.IAMStub;
import com.google.cloud.iam.admin.v1.stub.IAMStubSettings;
import com.google.common.util.concurrent.MoreExecutors;
import com.google.iam.admin.v1.CreateRoleRequest;
import com.google.iam.admin.v1.CreateServiceAccountKeyRequest;
import com.google.iam.admin.v1.CreateServiceAccountRequest;
import com.google.iam.admin.v1.DeleteRoleRequest;
import com.google.iam.admin.v1.DeleteServiceAccountKeyRequest;
import com.google.iam.admin.v1.DeleteServiceAccountRequest;
import com.google.iam.admin.v1.DisableServiceAccountKeyRequest;
import com.google.iam.admin.v1.DisableServiceAccountRequest;
import com.google.iam.admin.v1.EnableServiceAccountKeyRequest;
import com.google.iam.admin.v1.EnableServiceAccountRequest;
import com.google.iam.admin.v1.GetRoleRequest;
import com.google.iam.admin.v1.GetServiceAccountKeyRequest;
import com.google.iam.admin.v1.GetServiceAccountRequest;
import com.google.iam.admin.v1.KeyName;
import com.google.iam.admin.v1.LintPolicyRequest;
import com.google.iam.admin.v1.LintPolicyResponse;
import com.google.iam.admin.v1.ListRolesRequest;
import com.google.iam.admin.v1.ListRolesResponse;
import com.google.iam.admin.v1.ListServiceAccountKeysRequest;
import com.google.iam.admin.v1.ListServiceAccountKeysResponse;
import com.google.iam.admin.v1.ListServiceAccountsRequest;
import com.google.iam.admin.v1.ListServiceAccountsResponse;
import com.google.iam.admin.v1.PatchServiceAccountRequest;
import com.google.iam.admin.v1.Permission;
import com.google.iam.admin.v1.ProjectName;
import com.google.iam.admin.v1.QueryAuditableServicesRequest;
import com.google.iam.admin.v1.QueryAuditableServicesResponse;
import com.google.iam.admin.v1.QueryGrantableRolesRequest;
import com.google.iam.admin.v1.QueryGrantableRolesResponse;
import com.google.iam.admin.v1.QueryTestablePermissionsRequest;
import com.google.iam.admin.v1.QueryTestablePermissionsResponse;
import com.google.iam.admin.v1.Role;
import com.google.iam.admin.v1.ServiceAccount;
import com.google.iam.admin.v1.ServiceAccountKey;
import com.google.iam.admin.v1.ServiceAccountKeyAlgorithm;
import com.google.iam.admin.v1.ServiceAccountName;
import com.google.iam.admin.v1.ServiceAccountPrivateKeyType;
import com.google.iam.admin.v1.ServiceAccountPublicKeyType;
import com.google.iam.admin.v1.SignBlobRequest;
import com.google.iam.admin.v1.SignBlobResponse;
import com.google.iam.admin.v1.SignJwtRequest;
import com.google.iam.admin.v1.SignJwtResponse;
import com.google.iam.admin.v1.UndeleteRoleRequest;
import com.google.iam.admin.v1.UndeleteServiceAccountRequest;
import com.google.iam.admin.v1.UndeleteServiceAccountResponse;
import com.google.iam.admin.v1.UpdateRoleRequest;
import com.google.iam.admin.v1.UploadServiceAccountKeyRequest;
import com.google.iam.v1.GetIamPolicyRequest;
import com.google.iam.v1.Policy;
import com.google.iam.v1.SetIamPolicyRequest;
import com.google.iam.v1.TestIamPermissionsRequest;
import com.google.iam.v1.TestIamPermissionsResponse;
import com.google.protobuf.ByteString;
import com.google.protobuf.Empty;
import java.io.IOException;
import java.util.List;
import java.util.concurrent.TimeUnit;
import javax.annotation.Generated;
// AUTO-GENERATED DOCUMENTATION AND CLASS.
/**
* Service Description: Creates and manages Identity and Access Management (IAM) resources.
*
* You can use this service to work with all of the following resources:
*
*
* - **Service accounts**, which identify an application or a virtual machine
* (VM) instance rather than a person
*
- **Service account keys**, which service accounts use to authenticate with
* Google APIs
*
- **IAM policies for service accounts**, which specify the roles that a
* principal has for the service account
*
- **IAM custom roles**, which help you limit the number of permissions that
* you grant to principals
*
*
* In addition, you can use this service to complete the following tasks, among others:
*
*
* - Test whether a service account can use specific permissions
*
- Check which roles you can grant for a specific resource
*
- Lint, or validate, condition expressions in an IAM policy
*
*
* When you read data from the IAM API, each read is eventually consistent. In other words, if
* you write data with the IAM API, then immediately read that data, the read operation might return
* an older version of the data. To deal with this behavior, your application can retry the request
* with truncated exponential backoff.
*
*
In contrast, writing data to the IAM API is sequentially consistent. In other words, write
* operations are always processed in the order in which they were received.
*
*
This class provides the ability to make remote calls to the backing service through method
* calls that map to API methods. Sample code to get started:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ServiceAccountName name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* ServiceAccount response = iAMClient.getServiceAccount(name);
* }
* }
*
* Note: close() needs to be called on the IAMClient object to clean up resources such as
* threads. In the example above, try-with-resources is used, which automatically calls close().
*
*
* Methods
*
* Method
* Description
* Method Variants
*
*
* ListServiceAccounts
* Lists every [ServiceAccount][google.iam.admin.v1.ServiceAccount] that belongs to a specific project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listServiceAccounts(ListServiceAccountsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listServiceAccounts(ProjectName name)
*
listServiceAccounts(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listServiceAccountsPagedCallable()
*
listServiceAccountsCallable()
*
*
*
*
* GetServiceAccount
* Gets a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getServiceAccount(GetServiceAccountRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getServiceAccount(ServiceAccountName name)
*
getServiceAccount(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getServiceAccountCallable()
*
*
*
*
* CreateServiceAccount
* Creates a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createServiceAccount(CreateServiceAccountRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createServiceAccount(ProjectName name, String accountId, ServiceAccount serviceAccount)
*
createServiceAccount(String name, String accountId, ServiceAccount serviceAccount)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createServiceAccountCallable()
*
*
*
*
* UpdateServiceAccount
* **Note:** We are in the process of deprecating this method. Use [PatchServiceAccount][google.iam.admin.v1.IAM.PatchServiceAccount] instead.
*
Updates a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
You can update only the `display_name` field.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateServiceAccount(ServiceAccount request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateServiceAccountCallable()
*
*
*
*
* PatchServiceAccount
* Patches a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* patchServiceAccount(PatchServiceAccountRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* patchServiceAccountCallable()
*
*
*
*
* DeleteServiceAccount
* Deletes a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
**Warning:** After you delete a service account, you might not be able to undelete it. If you know that you need to re-enable the service account in the future, use [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] instead.
*
If you delete a service account, IAM permanently removes the service account 30 days later. Google Cloud cannot recover the service account after it is permanently removed, even if you file a support request.
*
To help avoid unplanned outages, we recommend that you disable the service account before you delete it. Use [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] to disable the service account, then wait at least 24 hours and watch for unintended consequences. If there are no unintended consequences, you can delete the service account.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteServiceAccount(DeleteServiceAccountRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteServiceAccount(ServiceAccountName name)
*
deleteServiceAccount(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteServiceAccountCallable()
*
*
*
*
* UndeleteServiceAccount
* Restores a deleted [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
**Important:** It is not always possible to restore a deleted service account. Use this method only as a last resort.
*
After you delete a service account, IAM permanently removes the service account 30 days later. There is no way to restore a deleted service account that has been permanently removed.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* undeleteServiceAccount(UndeleteServiceAccountRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* undeleteServiceAccountCallable()
*
*
*
*
* EnableServiceAccount
* Enables a [ServiceAccount][google.iam.admin.v1.ServiceAccount] that was disabled by [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount].
*
If the service account is already enabled, then this method has no effect.
*
If the service account was disabled by other means—for example, if Google disabled the service account because it was compromised—you cannot use this method to enable the service account.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* enableServiceAccount(EnableServiceAccountRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* enableServiceAccountCallable()
*
*
*
*
* DisableServiceAccount
* Disables a [ServiceAccount][google.iam.admin.v1.ServiceAccount] immediately.
*
If an application uses the service account to authenticate, that application can no longer call Google APIs or access Google Cloud resources. Existing access tokens for the service account are rejected, and requests for new access tokens will fail.
*
To re-enable the service account, use [EnableServiceAccount][google.iam.admin.v1.IAM.EnableServiceAccount]. After you re-enable the service account, its existing access tokens will be accepted, and you can request new access tokens.
*
To help avoid unplanned outages, we recommend that you disable the service account before you delete it. Use this method to disable the service account, then wait at least 24 hours and watch for unintended consequences. If there are no unintended consequences, you can delete the service account with [DeleteServiceAccount][google.iam.admin.v1.IAM.DeleteServiceAccount].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* disableServiceAccount(DisableServiceAccountRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* disableServiceAccountCallable()
*
*
*
*
* ListServiceAccountKeys
* Lists every [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey] for a service account.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listServiceAccountKeys(ListServiceAccountKeysRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* listServiceAccountKeys(ServiceAccountName name, List<ListServiceAccountKeysRequest.KeyType> keyTypes)
*
listServiceAccountKeys(String name, List<ListServiceAccountKeysRequest.KeyType> keyTypes)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listServiceAccountKeysCallable()
*
*
*
*
* GetServiceAccountKey
* Gets a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getServiceAccountKey(GetServiceAccountKeyRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getServiceAccountKey(KeyName name, ServiceAccountPublicKeyType publicKeyType)
*
getServiceAccountKey(String name, ServiceAccountPublicKeyType publicKeyType)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getServiceAccountKeyCallable()
*
*
*
*
* CreateServiceAccountKey
* Creates a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createServiceAccountKey(CreateServiceAccountKeyRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* createServiceAccountKey(ServiceAccountName name, ServiceAccountPrivateKeyType privateKeyType, ServiceAccountKeyAlgorithm keyAlgorithm)
*
createServiceAccountKey(String name, ServiceAccountPrivateKeyType privateKeyType, ServiceAccountKeyAlgorithm keyAlgorithm)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createServiceAccountKeyCallable()
*
*
*
*
* UploadServiceAccountKey
* Uploads the public key portion of a key pair that you manage, and associates the public key with a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
After you upload the public key, you can use the private key from the key pair as a service account key.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* uploadServiceAccountKey(UploadServiceAccountKeyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* uploadServiceAccountKeyCallable()
*
*
*
*
* DeleteServiceAccountKey
* Deletes a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. Deleting a service account key does not revoke short-lived credentials that have been issued based on the service account key.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteServiceAccountKey(DeleteServiceAccountKeyRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* deleteServiceAccountKey(KeyName name)
*
deleteServiceAccountKey(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteServiceAccountKeyCallable()
*
*
*
*
* DisableServiceAccountKey
* Disable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. A disabled service account key can be re-enabled with [EnableServiceAccountKey][google.iam.admin.v1.IAM.EnableServiceAccountKey].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* disableServiceAccountKey(DisableServiceAccountKeyRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* disableServiceAccountKey(KeyName name)
*
disableServiceAccountKey(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* disableServiceAccountKeyCallable()
*
*
*
*
* EnableServiceAccountKey
* Enable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* enableServiceAccountKey(EnableServiceAccountKeyRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* enableServiceAccountKey(KeyName name)
*
enableServiceAccountKey(String name)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* enableServiceAccountKeyCallable()
*
*
*
*
* SignBlob
* **Note:** This method is deprecated. Use the [`signBlob`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signBlob) method in the IAM Service Account Credentials API instead. If you currently use this method, see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for instructions.
*
Signs a blob using the system-managed private key for a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* signBlob(SignBlobRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* signBlob(ServiceAccountName name, ByteString bytesToSign)
*
signBlob(String name, ByteString bytesToSign)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* signBlobCallable()
*
*
*
*
* SignJwt
* **Note:** This method is deprecated. Use the [`signJwt`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signJwt) method in the IAM Service Account Credentials API instead. If you currently use this method, see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for instructions.
*
Signs a JSON Web Token (JWT) using the system-managed private key for a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* signJwt(SignJwtRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* signJwt(ServiceAccountName name, String payload)
*
signJwt(String name, String payload)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* signJwtCallable()
*
*
*
*
* GetIamPolicy
* Gets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount]. This IAM policy specifies which principals have access to the service account.
*
This method does not tell you whether the service account has been granted any roles on other resources. To check whether a service account has role grants on a resource, use the `getIamPolicy` method for that resource. For example, to view the role grants for a project, call the Resource Manager API's [`projects.getIamPolicy`](https://cloud.google.com/resource-manager/reference/rest/v1/projects/getIamPolicy) method.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getIamPolicy(GetIamPolicyRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* getIamPolicy(ResourceName resource)
*
getIamPolicy(String resource)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getIamPolicyCallable()
*
*
*
*
* SetIamPolicy
* Sets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
Use this method to grant or revoke access to the service account. For example, you could grant a principal the ability to impersonate the service account.
*
This method does not enable the service account to access other resources. To grant roles to a service account on a resource, follow these steps:
*
1. Call the resource's `getIamPolicy` method to get its current IAM policy. 2. Edit the policy so that it binds the service account to an IAM role for the resource. 3. Call the resource's `setIamPolicy` method to update its IAM policy.
*
For detailed instructions, see [Manage access to project, folders, and organizations](https://cloud.google.com/iam/help/service-accounts/granting-access-to-service-accounts) or [Manage access to other resources](https://cloud.google.com/iam/help/access/manage-other-resources).
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* setIamPolicy(SetIamPolicyRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* setIamPolicy(ResourceName resource, Policy policy)
*
setIamPolicy(String resource, Policy policy)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* setIamPolicyCallable()
*
*
*
*
* TestIamPermissions
* Tests whether the caller has the specified permissions on a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* testIamPermissions(TestIamPermissionsRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* testIamPermissions(ResourceName resource, List<String> permissions)
*
testIamPermissions(String resource, List<String> permissions)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* testIamPermissionsCallable()
*
*
*
*
* QueryGrantableRoles
* Lists roles that can be granted on a Google Cloud resource. A role is grantable if the IAM policy for the resource can contain bindings to the role.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* queryGrantableRoles(QueryGrantableRolesRequest request)
*
* "Flattened" method variants have converted the fields of the request object into function parameters to enable multiple ways to call the same method.
*
* queryGrantableRoles(String fullResourceName)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* queryGrantableRolesPagedCallable()
*
queryGrantableRolesCallable()
*
*
*
*
* ListRoles
* Lists every predefined [Role][google.iam.admin.v1.Role] that IAM supports, or every custom role that is defined for an organization or project.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* listRoles(ListRolesRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* listRolesPagedCallable()
*
listRolesCallable()
*
*
*
*
* GetRole
* Gets the definition of a [Role][google.iam.admin.v1.Role].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* getRole(GetRoleRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* getRoleCallable()
*
*
*
*
* CreateRole
* Creates a new custom [Role][google.iam.admin.v1.Role].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* createRole(CreateRoleRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* createRoleCallable()
*
*
*
*
* UpdateRole
* Updates the definition of a custom [Role][google.iam.admin.v1.Role].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* updateRole(UpdateRoleRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* updateRoleCallable()
*
*
*
*
* DeleteRole
* Deletes a custom [Role][google.iam.admin.v1.Role].
*
When you delete a custom role, the following changes occur immediately:
*
* - You cannot bind a principal to the custom role in an IAM [Policy][google.iam.v1.Policy].
*
- Existing bindings to the custom role are not changed, but they have no effect.
*
- By default, the response from [ListRoles][google.iam.admin.v1.IAM.ListRoles] does not include the custom role.
*
* You have 7 days to undelete the custom role. After 7 days, the following changes occur:
*
* - The custom role is permanently deleted and cannot be recovered.
*
- If an IAM policy contains a binding to the custom role, the binding is permanently removed.
*
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* deleteRole(DeleteRoleRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* deleteRoleCallable()
*
*
*
*
* UndeleteRole
* Undeletes a custom [Role][google.iam.admin.v1.Role].
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* undeleteRole(UndeleteRoleRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* undeleteRoleCallable()
*
*
*
*
* QueryTestablePermissions
* Lists every permission that you can test on a resource. A permission is testable if you can check whether a principal has that permission on the resource.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* queryTestablePermissions(QueryTestablePermissionsRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* queryTestablePermissionsPagedCallable()
*
queryTestablePermissionsCallable()
*
*
*
*
* QueryAuditableServices
* Returns a list of services that allow you to opt into audit logs that are not generated by default.
*
To learn more about audit logs, see the [Logging documentation](https://cloud.google.com/logging/docs/audit).
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* queryAuditableServices(QueryAuditableServicesRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* queryAuditableServicesCallable()
*
*
*
*
* LintPolicy
* Lints, or validates, an IAM policy. Currently checks the [google.iam.v1.Binding.condition][google.iam.v1.Binding.condition] field, which contains a condition expression for a role binding.
*
Successful calls to this method always return an HTTP `200 OK` status code, even if the linter detects an issue in the IAM policy.
*
* Request object method variants only take one parameter, a request object, which must be constructed before the call.
*
* lintPolicy(LintPolicyRequest request)
*
* Callable method variants take no parameters and return an immutable API callable object, which can be used to initiate calls to the service.
*
* lintPolicyCallable()
*
*
*
*
*
* See the individual methods for example code.
*
*
Many parameters require resource names to be formatted in a particular way. To assist with
* these names, this class includes a format method for each type of name, and additionally a parse
* method to extract the individual identifiers contained within names that are returned.
*
*
This class can be customized by passing in a custom instance of IAMSettings to create(). For
* example:
*
*
To customize credentials:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* IAMSettings iAMSettings =
* IAMSettings.newBuilder()
* .setCredentialsProvider(FixedCredentialsProvider.create(myCredentials))
* .build();
* IAMClient iAMClient = IAMClient.create(iAMSettings);
* }
*
* To customize the endpoint:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* IAMSettings iAMSettings = IAMSettings.newBuilder().setEndpoint(myEndpoint).build();
* IAMClient iAMClient = IAMClient.create(iAMSettings);
* }
*
* Please refer to the GitHub repository's samples for more quickstart code snippets.
*/
@Generated("by gapic-generator-java")
public class IAMClient implements BackgroundResource {
private final IAMSettings settings;
private final IAMStub stub;
/** Constructs an instance of IAMClient with default settings. */
public static final IAMClient create() throws IOException {
return create(IAMSettings.newBuilder().build());
}
/**
* Constructs an instance of IAMClient, using the given settings. The channels are created based
* on the settings passed in, or defaults for any settings that are not set.
*/
public static final IAMClient create(IAMSettings settings) throws IOException {
return new IAMClient(settings);
}
/**
* Constructs an instance of IAMClient, using the given stub for making calls. This is for
* advanced usage - prefer using create(IAMSettings).
*/
public static final IAMClient create(IAMStub stub) {
return new IAMClient(stub);
}
/**
* Constructs an instance of IAMClient, using the given settings. This is protected so that it is
* easy to make a subclass, but otherwise, the static factory methods should be preferred.
*/
protected IAMClient(IAMSettings settings) throws IOException {
this.settings = settings;
this.stub = ((IAMStubSettings) settings.getStubSettings()).createStub();
}
protected IAMClient(IAMStub stub) {
this.settings = null;
this.stub = stub;
}
public final IAMSettings getSettings() {
return settings;
}
public IAMStub getStub() {
return stub;
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every [ServiceAccount][google.iam.admin.v1.ServiceAccount] that belongs to a specific
* project.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ProjectName name = ProjectName.of("[PROJECT]");
* for (ServiceAccount element : iAMClient.listServiceAccounts(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The resource name of the project associated with the service accounts,
* such as `projects/my-project-123`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServiceAccountsPagedResponse listServiceAccounts(ProjectName name) {
ListServiceAccountsRequest request =
ListServiceAccountsRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return listServiceAccounts(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every [ServiceAccount][google.iam.admin.v1.ServiceAccount] that belongs to a specific
* project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = ProjectName.of("[PROJECT]").toString();
* for (ServiceAccount element : iAMClient.listServiceAccounts(name).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param name Required. The resource name of the project associated with the service accounts,
* such as `projects/my-project-123`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServiceAccountsPagedResponse listServiceAccounts(String name) {
ListServiceAccountsRequest request =
ListServiceAccountsRequest.newBuilder().setName(name).build();
return listServiceAccounts(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every [ServiceAccount][google.iam.admin.v1.ServiceAccount] that belongs to a specific
* project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ListServiceAccountsRequest request =
* ListServiceAccountsRequest.newBuilder()
* .setName(ProjectName.of("[PROJECT]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (ServiceAccount element : iAMClient.listServiceAccounts(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServiceAccountsPagedResponse listServiceAccounts(
ListServiceAccountsRequest request) {
return listServiceAccountsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every [ServiceAccount][google.iam.admin.v1.ServiceAccount] that belongs to a specific
* project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ListServiceAccountsRequest request =
* ListServiceAccountsRequest.newBuilder()
* .setName(ProjectName.of("[PROJECT]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* iAMClient.listServiceAccountsPagedCallable().futureCall(request);
* // Do something.
* for (ServiceAccount element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
listServiceAccountsPagedCallable() {
return stub.listServiceAccountsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every [ServiceAccount][google.iam.admin.v1.ServiceAccount] that belongs to a specific
* project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ListServiceAccountsRequest request =
* ListServiceAccountsRequest.newBuilder()
* .setName(ProjectName.of("[PROJECT]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* ListServiceAccountsResponse response =
* iAMClient.listServiceAccountsCallable().call(request);
* for (ServiceAccount element : response.getAccountsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
listServiceAccountsCallable() {
return stub.listServiceAccountsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ServiceAccountName name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* ServiceAccount response = iAMClient.getServiceAccount(name);
* }
* }
*
* @param name Required. The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccount getServiceAccount(ServiceAccountName name) {
GetServiceAccountRequest request =
GetServiceAccountRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
return getServiceAccount(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString();
* ServiceAccount response = iAMClient.getServiceAccount(name);
* }
* }
*
* @param name Required. The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccount getServiceAccount(String name) {
GetServiceAccountRequest request = GetServiceAccountRequest.newBuilder().setName(name).build();
return getServiceAccount(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* GetServiceAccountRequest request =
* GetServiceAccountRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .build();
* ServiceAccount response = iAMClient.getServiceAccount(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccount getServiceAccount(GetServiceAccountRequest request) {
return getServiceAccountCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* GetServiceAccountRequest request =
* GetServiceAccountRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .build();
* ApiFuture future = iAMClient.getServiceAccountCallable().futureCall(request);
* // Do something.
* ServiceAccount response = future.get();
* }
* }
*/
public final UnaryCallable getServiceAccountCallable() {
return stub.getServiceAccountCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ProjectName name = ProjectName.of("[PROJECT]");
* String accountId = "accountId-1827029976";
* ServiceAccount serviceAccount = ServiceAccount.newBuilder().build();
* ServiceAccount response = iAMClient.createServiceAccount(name, accountId, serviceAccount);
* }
* }
*
* @param name Required. The resource name of the project associated with the service accounts,
* such as `projects/my-project-123`.
* @param accountId Required. The account id that is used to generate the service account email
* address and a stable unique id. It is unique within a project, must be 6-30 characters
* long, and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])` to comply with
* RFC1035.
* @param serviceAccount The [ServiceAccount][google.iam.admin.v1.ServiceAccount] resource to
* create. Currently, only the following values are user assignable: `display_name` and
* `description`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccount createServiceAccount(
ProjectName name, String accountId, ServiceAccount serviceAccount) {
CreateServiceAccountRequest request =
CreateServiceAccountRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setAccountId(accountId)
.setServiceAccount(serviceAccount)
.build();
return createServiceAccount(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = ProjectName.of("[PROJECT]").toString();
* String accountId = "accountId-1827029976";
* ServiceAccount serviceAccount = ServiceAccount.newBuilder().build();
* ServiceAccount response = iAMClient.createServiceAccount(name, accountId, serviceAccount);
* }
* }
*
* @param name Required. The resource name of the project associated with the service accounts,
* such as `projects/my-project-123`.
* @param accountId Required. The account id that is used to generate the service account email
* address and a stable unique id. It is unique within a project, must be 6-30 characters
* long, and match the regular expression `[a-z]([-a-z0-9]*[a-z0-9])` to comply with
* RFC1035.
* @param serviceAccount The [ServiceAccount][google.iam.admin.v1.ServiceAccount] resource to
* create. Currently, only the following values are user assignable: `display_name` and
* `description`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccount createServiceAccount(
String name, String accountId, ServiceAccount serviceAccount) {
CreateServiceAccountRequest request =
CreateServiceAccountRequest.newBuilder()
.setName(name)
.setAccountId(accountId)
.setServiceAccount(serviceAccount)
.build();
return createServiceAccount(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* CreateServiceAccountRequest request =
* CreateServiceAccountRequest.newBuilder()
* .setName(ProjectName.of("[PROJECT]").toString())
* .setAccountId("accountId-1827029976")
* .setServiceAccount(ServiceAccount.newBuilder().build())
* .build();
* ServiceAccount response = iAMClient.createServiceAccount(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccount createServiceAccount(CreateServiceAccountRequest request) {
return createServiceAccountCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* CreateServiceAccountRequest request =
* CreateServiceAccountRequest.newBuilder()
* .setName(ProjectName.of("[PROJECT]").toString())
* .setAccountId("accountId-1827029976")
* .setServiceAccount(ServiceAccount.newBuilder().build())
* .build();
* ApiFuture future =
* iAMClient.createServiceAccountCallable().futureCall(request);
* // Do something.
* ServiceAccount response = future.get();
* }
* }
*/
public final UnaryCallable
createServiceAccountCallable() {
return stub.createServiceAccountCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** We are in the process of deprecating this method. Use
* [PatchServiceAccount][google.iam.admin.v1.IAM.PatchServiceAccount] instead.
*
* Updates a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
You can update only the `display_name` field.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ServiceAccount request =
* ServiceAccount.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setProjectId("projectId-894832108")
* .setUniqueId("uniqueId-294460212")
* .setEmail("email96619420")
* .setDisplayName("displayName1714148973")
* .setEtag(ByteString.EMPTY)
* .setDescription("description-1724546052")
* .setOauth2ClientId("oauth2ClientId-1210797087")
* .setDisabled(true)
* .build();
* ServiceAccount response = iAMClient.updateServiceAccount(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccount updateServiceAccount(ServiceAccount request) {
return updateServiceAccountCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** We are in the process of deprecating this method. Use
* [PatchServiceAccount][google.iam.admin.v1.IAM.PatchServiceAccount] instead.
*
* Updates a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
You can update only the `display_name` field.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ServiceAccount request =
* ServiceAccount.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setProjectId("projectId-894832108")
* .setUniqueId("uniqueId-294460212")
* .setEmail("email96619420")
* .setDisplayName("displayName1714148973")
* .setEtag(ByteString.EMPTY)
* .setDescription("description-1724546052")
* .setOauth2ClientId("oauth2ClientId-1210797087")
* .setDisabled(true)
* .build();
* ApiFuture future =
* iAMClient.updateServiceAccountCallable().futureCall(request);
* // Do something.
* ServiceAccount response = future.get();
* }
* }
*/
public final UnaryCallable updateServiceAccountCallable() {
return stub.updateServiceAccountCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Patches a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* PatchServiceAccountRequest request =
* PatchServiceAccountRequest.newBuilder()
* .setServiceAccount(ServiceAccount.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ServiceAccount response = iAMClient.patchServiceAccount(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccount patchServiceAccount(PatchServiceAccountRequest request) {
return patchServiceAccountCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Patches a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* PatchServiceAccountRequest request =
* PatchServiceAccountRequest.newBuilder()
* .setServiceAccount(ServiceAccount.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future =
* iAMClient.patchServiceAccountCallable().futureCall(request);
* // Do something.
* ServiceAccount response = future.get();
* }
* }
*/
public final UnaryCallable
patchServiceAccountCallable() {
return stub.patchServiceAccountCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* **Warning:** After you delete a service account, you might not be able to
* undelete it. If you know that you need to re-enable the service account in the future, use
* [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] instead.
*
*
If you delete a service account, IAM permanently removes the service account 30 days later.
* Google Cloud cannot recover the service account after it is permanently removed, even if you
* file a support request.
*
*
To help avoid unplanned outages, we recommend that you disable the service account before
* you delete it. Use [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] to
* disable the service account, then wait at least 24 hours and watch for unintended consequences.
* If there are no unintended consequences, you can delete the service account.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ServiceAccountName name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* iAMClient.deleteServiceAccount(name);
* }
* }
*
* @param name Required. The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteServiceAccount(ServiceAccountName name) {
DeleteServiceAccountRequest request =
DeleteServiceAccountRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
deleteServiceAccount(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* **Warning:** After you delete a service account, you might not be able to
* undelete it. If you know that you need to re-enable the service account in the future, use
* [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] instead.
*
*
If you delete a service account, IAM permanently removes the service account 30 days later.
* Google Cloud cannot recover the service account after it is permanently removed, even if you
* file a support request.
*
*
To help avoid unplanned outages, we recommend that you disable the service account before
* you delete it. Use [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] to
* disable the service account, then wait at least 24 hours and watch for unintended consequences.
* If there are no unintended consequences, you can delete the service account.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString();
* iAMClient.deleteServiceAccount(name);
* }
* }
*
* @param name Required. The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteServiceAccount(String name) {
DeleteServiceAccountRequest request =
DeleteServiceAccountRequest.newBuilder().setName(name).build();
deleteServiceAccount(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* **Warning:** After you delete a service account, you might not be able to
* undelete it. If you know that you need to re-enable the service account in the future, use
* [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] instead.
*
*
If you delete a service account, IAM permanently removes the service account 30 days later.
* Google Cloud cannot recover the service account after it is permanently removed, even if you
* file a support request.
*
*
To help avoid unplanned outages, we recommend that you disable the service account before
* you delete it. Use [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] to
* disable the service account, then wait at least 24 hours and watch for unintended consequences.
* If there are no unintended consequences, you can delete the service account.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DeleteServiceAccountRequest request =
* DeleteServiceAccountRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .build();
* iAMClient.deleteServiceAccount(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteServiceAccount(DeleteServiceAccountRequest request) {
deleteServiceAccountCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* **Warning:** After you delete a service account, you might not be able to
* undelete it. If you know that you need to re-enable the service account in the future, use
* [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] instead.
*
*
If you delete a service account, IAM permanently removes the service account 30 days later.
* Google Cloud cannot recover the service account after it is permanently removed, even if you
* file a support request.
*
*
To help avoid unplanned outages, we recommend that you disable the service account before
* you delete it. Use [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount] to
* disable the service account, then wait at least 24 hours and watch for unintended consequences.
* If there are no unintended consequences, you can delete the service account.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DeleteServiceAccountRequest request =
* DeleteServiceAccountRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .build();
* ApiFuture future = iAMClient.deleteServiceAccountCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable deleteServiceAccountCallable() {
return stub.deleteServiceAccountCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restores a deleted [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* **Important:** It is not always possible to restore a deleted service
* account. Use this method only as a last resort.
*
*
After you delete a service account, IAM permanently removes the service account 30 days
* later. There is no way to restore a deleted service account that has been permanently removed.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* UndeleteServiceAccountRequest request =
* UndeleteServiceAccountRequest.newBuilder().setName("name3373707").build();
* UndeleteServiceAccountResponse response = iAMClient.undeleteServiceAccount(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final UndeleteServiceAccountResponse undeleteServiceAccount(
UndeleteServiceAccountRequest request) {
return undeleteServiceAccountCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Restores a deleted [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* **Important:** It is not always possible to restore a deleted service
* account. Use this method only as a last resort.
*
*
After you delete a service account, IAM permanently removes the service account 30 days
* later. There is no way to restore a deleted service account that has been permanently removed.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* UndeleteServiceAccountRequest request =
* UndeleteServiceAccountRequest.newBuilder().setName("name3373707").build();
* ApiFuture future =
* iAMClient.undeleteServiceAccountCallable().futureCall(request);
* // Do something.
* UndeleteServiceAccountResponse response = future.get();
* }
* }
*/
public final UnaryCallable
undeleteServiceAccountCallable() {
return stub.undeleteServiceAccountCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enables a [ServiceAccount][google.iam.admin.v1.ServiceAccount] that was disabled by
* [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount].
*
* If the service account is already enabled, then this method has no effect.
*
*
If the service account was disabled by other means—for example, if Google disabled the
* service account because it was compromised—you cannot use this method to enable the service
* account.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* EnableServiceAccountRequest request =
* EnableServiceAccountRequest.newBuilder().setName("name3373707").build();
* iAMClient.enableServiceAccount(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void enableServiceAccount(EnableServiceAccountRequest request) {
enableServiceAccountCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enables a [ServiceAccount][google.iam.admin.v1.ServiceAccount] that was disabled by
* [DisableServiceAccount][google.iam.admin.v1.IAM.DisableServiceAccount].
*
* If the service account is already enabled, then this method has no effect.
*
*
If the service account was disabled by other means—for example, if Google disabled the
* service account because it was compromised—you cannot use this method to enable the service
* account.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* EnableServiceAccountRequest request =
* EnableServiceAccountRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = iAMClient.enableServiceAccountCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable enableServiceAccountCallable() {
return stub.enableServiceAccountCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disables a [ServiceAccount][google.iam.admin.v1.ServiceAccount] immediately.
*
* If an application uses the service account to authenticate, that application can no longer
* call Google APIs or access Google Cloud resources. Existing access tokens for the service
* account are rejected, and requests for new access tokens will fail.
*
*
To re-enable the service account, use
* [EnableServiceAccount][google.iam.admin.v1.IAM.EnableServiceAccount]. After you re-enable the
* service account, its existing access tokens will be accepted, and you can request new access
* tokens.
*
*
To help avoid unplanned outages, we recommend that you disable the service account before
* you delete it. Use this method to disable the service account, then wait at least 24 hours and
* watch for unintended consequences. If there are no unintended consequences, you can delete the
* service account with [DeleteServiceAccount][google.iam.admin.v1.IAM.DeleteServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DisableServiceAccountRequest request =
* DisableServiceAccountRequest.newBuilder().setName("name3373707").build();
* iAMClient.disableServiceAccount(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void disableServiceAccount(DisableServiceAccountRequest request) {
disableServiceAccountCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disables a [ServiceAccount][google.iam.admin.v1.ServiceAccount] immediately.
*
* If an application uses the service account to authenticate, that application can no longer
* call Google APIs or access Google Cloud resources. Existing access tokens for the service
* account are rejected, and requests for new access tokens will fail.
*
*
To re-enable the service account, use
* [EnableServiceAccount][google.iam.admin.v1.IAM.EnableServiceAccount]. After you re-enable the
* service account, its existing access tokens will be accepted, and you can request new access
* tokens.
*
*
To help avoid unplanned outages, we recommend that you disable the service account before
* you delete it. Use this method to disable the service account, then wait at least 24 hours and
* watch for unintended consequences. If there are no unintended consequences, you can delete the
* service account with [DeleteServiceAccount][google.iam.admin.v1.IAM.DeleteServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DisableServiceAccountRequest request =
* DisableServiceAccountRequest.newBuilder().setName("name3373707").build();
* ApiFuture future = iAMClient.disableServiceAccountCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable disableServiceAccountCallable() {
return stub.disableServiceAccountCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey] for a service account.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ServiceAccountName name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* List keyTypes = new ArrayList<>();
* ListServiceAccountKeysResponse response = iAMClient.listServiceAccountKeys(name, keyTypes);
* }
* }
*
* @param name Required. The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`.
* Using `-` as a wildcard for the `PROJECT_ID`, will infer the project from the account.
* The `ACCOUNT` value can be the `email` address or the `unique_id` of the service account.
* @param keyTypes Filters the types of keys the user wants to include in the list response.
* Duplicate key types are not allowed. If no key type is provided, all keys are returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServiceAccountKeysResponse listServiceAccountKeys(
ServiceAccountName name, List keyTypes) {
ListServiceAccountKeysRequest request =
ListServiceAccountKeysRequest.newBuilder()
.setName(name == null ? null : name.toString())
.addAllKeyTypes(keyTypes)
.build();
return listServiceAccountKeys(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey] for a service account.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString();
* List keyTypes = new ArrayList<>();
* ListServiceAccountKeysResponse response = iAMClient.listServiceAccountKeys(name, keyTypes);
* }
* }
*
* @param name Required. The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`.
* Using `-` as a wildcard for the `PROJECT_ID`, will infer the project from the account.
* The `ACCOUNT` value can be the `email` address or the `unique_id` of the service account.
* @param keyTypes Filters the types of keys the user wants to include in the list response.
* Duplicate key types are not allowed. If no key type is provided, all keys are returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServiceAccountKeysResponse listServiceAccountKeys(
String name, List keyTypes) {
ListServiceAccountKeysRequest request =
ListServiceAccountKeysRequest.newBuilder().setName(name).addAllKeyTypes(keyTypes).build();
return listServiceAccountKeys(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey] for a service account.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ListServiceAccountKeysRequest request =
* ListServiceAccountKeysRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .addAllKeyTypes(new ArrayList())
* .build();
* ListServiceAccountKeysResponse response = iAMClient.listServiceAccountKeys(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListServiceAccountKeysResponse listServiceAccountKeys(
ListServiceAccountKeysRequest request) {
return listServiceAccountKeysCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey] for a service account.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ListServiceAccountKeysRequest request =
* ListServiceAccountKeysRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .addAllKeyTypes(new ArrayList())
* .build();
* ApiFuture future =
* iAMClient.listServiceAccountKeysCallable().futureCall(request);
* // Do something.
* ListServiceAccountKeysResponse response = future.get();
* }
* }
*/
public final UnaryCallable
listServiceAccountKeysCallable() {
return stub.listServiceAccountKeysCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* KeyName name = KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]");
* ServiceAccountPublicKeyType publicKeyType = ServiceAccountPublicKeyType.forNumber(0);
* ServiceAccountKey response = iAMClient.getServiceAccountKey(name, publicKeyType);
* }
* }
*
* @param name Required. The resource name of the service account key in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}/keys/{key}`.
* Using `-` as a wildcard for the `PROJECT_ID` will infer the project from the account.
* The `ACCOUNT` value can be the `email` address or the `unique_id` of the service account.
* @param publicKeyType Optional. The output format of the public key. The default is `TYPE_NONE`,
* which means that the public key is not returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccountKey getServiceAccountKey(
KeyName name, ServiceAccountPublicKeyType publicKeyType) {
GetServiceAccountKeyRequest request =
GetServiceAccountKeyRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setPublicKeyType(publicKeyType)
.build();
return getServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString();
* ServiceAccountPublicKeyType publicKeyType = ServiceAccountPublicKeyType.forNumber(0);
* ServiceAccountKey response = iAMClient.getServiceAccountKey(name, publicKeyType);
* }
* }
*
* @param name Required. The resource name of the service account key in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}/keys/{key}`.
* Using `-` as a wildcard for the `PROJECT_ID` will infer the project from the account.
* The `ACCOUNT` value can be the `email` address or the `unique_id` of the service account.
* @param publicKeyType Optional. The output format of the public key. The default is `TYPE_NONE`,
* which means that the public key is not returned.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccountKey getServiceAccountKey(
String name, ServiceAccountPublicKeyType publicKeyType) {
GetServiceAccountKeyRequest request =
GetServiceAccountKeyRequest.newBuilder()
.setName(name)
.setPublicKeyType(publicKeyType)
.build();
return getServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* GetServiceAccountKeyRequest request =
* GetServiceAccountKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString())
* .setPublicKeyType(ServiceAccountPublicKeyType.forNumber(0))
* .build();
* ServiceAccountKey response = iAMClient.getServiceAccountKey(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccountKey getServiceAccountKey(GetServiceAccountKeyRequest request) {
return getServiceAccountKeyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* GetServiceAccountKeyRequest request =
* GetServiceAccountKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString())
* .setPublicKeyType(ServiceAccountPublicKeyType.forNumber(0))
* .build();
* ApiFuture future =
* iAMClient.getServiceAccountKeyCallable().futureCall(request);
* // Do something.
* ServiceAccountKey response = future.get();
* }
* }
*/
public final UnaryCallable
getServiceAccountKeyCallable() {
return stub.getServiceAccountKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ServiceAccountName name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* ServiceAccountPrivateKeyType privateKeyType = ServiceAccountPrivateKeyType.forNumber(0);
* ServiceAccountKeyAlgorithm keyAlgorithm = ServiceAccountKeyAlgorithm.forNumber(0);
* ServiceAccountKey response =
* iAMClient.createServiceAccountKey(name, privateKeyType, keyAlgorithm);
* }
* }
*
* @param name Required. The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @param privateKeyType The output format of the private key. The default value is
* `TYPE_GOOGLE_CREDENTIALS_FILE`, which is the Google Credentials File format.
* @param keyAlgorithm Which type of key and algorithm to use for the key. The default is
* currently a 2K RSA key. However this may change in the future.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccountKey createServiceAccountKey(
ServiceAccountName name,
ServiceAccountPrivateKeyType privateKeyType,
ServiceAccountKeyAlgorithm keyAlgorithm) {
CreateServiceAccountKeyRequest request =
CreateServiceAccountKeyRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setPrivateKeyType(privateKeyType)
.setKeyAlgorithm(keyAlgorithm)
.build();
return createServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString();
* ServiceAccountPrivateKeyType privateKeyType = ServiceAccountPrivateKeyType.forNumber(0);
* ServiceAccountKeyAlgorithm keyAlgorithm = ServiceAccountKeyAlgorithm.forNumber(0);
* ServiceAccountKey response =
* iAMClient.createServiceAccountKey(name, privateKeyType, keyAlgorithm);
* }
* }
*
* @param name Required. The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @param privateKeyType The output format of the private key. The default value is
* `TYPE_GOOGLE_CREDENTIALS_FILE`, which is the Google Credentials File format.
* @param keyAlgorithm Which type of key and algorithm to use for the key. The default is
* currently a 2K RSA key. However this may change in the future.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccountKey createServiceAccountKey(
String name,
ServiceAccountPrivateKeyType privateKeyType,
ServiceAccountKeyAlgorithm keyAlgorithm) {
CreateServiceAccountKeyRequest request =
CreateServiceAccountKeyRequest.newBuilder()
.setName(name)
.setPrivateKeyType(privateKeyType)
.setKeyAlgorithm(keyAlgorithm)
.build();
return createServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* CreateServiceAccountKeyRequest request =
* CreateServiceAccountKeyRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setPrivateKeyType(ServiceAccountPrivateKeyType.forNumber(0))
* .setKeyAlgorithm(ServiceAccountKeyAlgorithm.forNumber(0))
* .build();
* ServiceAccountKey response = iAMClient.createServiceAccountKey(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccountKey createServiceAccountKey(CreateServiceAccountKeyRequest request) {
return createServiceAccountKeyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* CreateServiceAccountKeyRequest request =
* CreateServiceAccountKeyRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setPrivateKeyType(ServiceAccountPrivateKeyType.forNumber(0))
* .setKeyAlgorithm(ServiceAccountKeyAlgorithm.forNumber(0))
* .build();
* ApiFuture future =
* iAMClient.createServiceAccountKeyCallable().futureCall(request);
* // Do something.
* ServiceAccountKey response = future.get();
* }
* }
*/
public final UnaryCallable
createServiceAccountKeyCallable() {
return stub.createServiceAccountKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Uploads the public key portion of a key pair that you manage, and associates the public key
* with a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* After you upload the public key, you can use the private key from the key pair as a service
* account key.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* UploadServiceAccountKeyRequest request =
* UploadServiceAccountKeyRequest.newBuilder()
* .setName("name3373707")
* .setPublicKeyData(ByteString.EMPTY)
* .build();
* ServiceAccountKey response = iAMClient.uploadServiceAccountKey(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ServiceAccountKey uploadServiceAccountKey(UploadServiceAccountKeyRequest request) {
return uploadServiceAccountKeyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Uploads the public key portion of a key pair that you manage, and associates the public key
* with a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* After you upload the public key, you can use the private key from the key pair as a service
* account key.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* UploadServiceAccountKeyRequest request =
* UploadServiceAccountKeyRequest.newBuilder()
* .setName("name3373707")
* .setPublicKeyData(ByteString.EMPTY)
* .build();
* ApiFuture future =
* iAMClient.uploadServiceAccountKeyCallable().futureCall(request);
* // Do something.
* ServiceAccountKey response = future.get();
* }
* }
*/
public final UnaryCallable
uploadServiceAccountKeyCallable() {
return stub.uploadServiceAccountKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. Deleting a service
* account key does not revoke short-lived credentials that have been issued based on the service
* account key.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* KeyName name = KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]");
* iAMClient.deleteServiceAccountKey(name);
* }
* }
*
* @param name Required. The resource name of the service account key in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}/keys/{key}`. Using `-` as a wildcard for
* the `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteServiceAccountKey(KeyName name) {
DeleteServiceAccountKeyRequest request =
DeleteServiceAccountKeyRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
deleteServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. Deleting a service
* account key does not revoke short-lived credentials that have been issued based on the service
* account key.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString();
* iAMClient.deleteServiceAccountKey(name);
* }
* }
*
* @param name Required. The resource name of the service account key in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}/keys/{key}`. Using `-` as a wildcard for
* the `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteServiceAccountKey(String name) {
DeleteServiceAccountKeyRequest request =
DeleteServiceAccountKeyRequest.newBuilder().setName(name).build();
deleteServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. Deleting a service
* account key does not revoke short-lived credentials that have been issued based on the service
* account key.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DeleteServiceAccountKeyRequest request =
* DeleteServiceAccountKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString())
* .build();
* iAMClient.deleteServiceAccountKey(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void deleteServiceAccountKey(DeleteServiceAccountKeyRequest request) {
deleteServiceAccountKeyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. Deleting a service
* account key does not revoke short-lived credentials that have been issued based on the service
* account key.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DeleteServiceAccountKeyRequest request =
* DeleteServiceAccountKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString())
* .build();
* ApiFuture future = iAMClient.deleteServiceAccountKeyCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable
deleteServiceAccountKeyCallable() {
return stub.deleteServiceAccountKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. A disabled service
* account key can be re-enabled with
* [EnableServiceAccountKey][google.iam.admin.v1.IAM.EnableServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* KeyName name = KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]");
* iAMClient.disableServiceAccountKey(name);
* }
* }
*
* @param name Required. The resource name of the service account key in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}/keys/{key}`.
* Using `-` as a wildcard for the `PROJECT_ID` will infer the project from the account.
* The `ACCOUNT` value can be the `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void disableServiceAccountKey(KeyName name) {
DisableServiceAccountKeyRequest request =
DisableServiceAccountKeyRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
disableServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. A disabled service
* account key can be re-enabled with
* [EnableServiceAccountKey][google.iam.admin.v1.IAM.EnableServiceAccountKey].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString();
* iAMClient.disableServiceAccountKey(name);
* }
* }
*
* @param name Required. The resource name of the service account key in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}/keys/{key}`.
* Using `-` as a wildcard for the `PROJECT_ID` will infer the project from the account.
* The `ACCOUNT` value can be the `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void disableServiceAccountKey(String name) {
DisableServiceAccountKeyRequest request =
DisableServiceAccountKeyRequest.newBuilder().setName(name).build();
disableServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. A disabled service
* account key can be re-enabled with
* [EnableServiceAccountKey][google.iam.admin.v1.IAM.EnableServiceAccountKey].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DisableServiceAccountKeyRequest request =
* DisableServiceAccountKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString())
* .build();
* iAMClient.disableServiceAccountKey(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void disableServiceAccountKey(DisableServiceAccountKeyRequest request) {
disableServiceAccountKeyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Disable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey]. A disabled service
* account key can be re-enabled with
* [EnableServiceAccountKey][google.iam.admin.v1.IAM.EnableServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DisableServiceAccountKeyRequest request =
* DisableServiceAccountKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString())
* .build();
* ApiFuture future = iAMClient.disableServiceAccountKeyCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable
disableServiceAccountKeyCallable() {
return stub.disableServiceAccountKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* KeyName name = KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]");
* iAMClient.enableServiceAccountKey(name);
* }
* }
*
* @param name Required. The resource name of the service account key in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}/keys/{key}`.
* Using `-` as a wildcard for the `PROJECT_ID` will infer the project from the account.
* The `ACCOUNT` value can be the `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void enableServiceAccountKey(KeyName name) {
EnableServiceAccountKeyRequest request =
EnableServiceAccountKeyRequest.newBuilder()
.setName(name == null ? null : name.toString())
.build();
enableServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString();
* iAMClient.enableServiceAccountKey(name);
* }
* }
*
* @param name Required. The resource name of the service account key in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}/keys/{key}`.
* Using `-` as a wildcard for the `PROJECT_ID` will infer the project from the account.
* The `ACCOUNT` value can be the `email` address or the `unique_id` of the service account.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void enableServiceAccountKey(String name) {
EnableServiceAccountKeyRequest request =
EnableServiceAccountKeyRequest.newBuilder().setName(name).build();
enableServiceAccountKey(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* EnableServiceAccountKeyRequest request =
* EnableServiceAccountKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString())
* .build();
* iAMClient.enableServiceAccountKey(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final void enableServiceAccountKey(EnableServiceAccountKeyRequest request) {
enableServiceAccountKeyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Enable a [ServiceAccountKey][google.iam.admin.v1.ServiceAccountKey].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* EnableServiceAccountKeyRequest request =
* EnableServiceAccountKeyRequest.newBuilder()
* .setName(KeyName.of("[PROJECT]", "[SERVICE_ACCOUNT]", "[KEY]").toString())
* .build();
* ApiFuture future = iAMClient.enableServiceAccountKeyCallable().futureCall(request);
* // Do something.
* future.get();
* }
* }
*/
public final UnaryCallable
enableServiceAccountKeyCallable() {
return stub.enableServiceAccountKeyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** This method is deprecated. Use the
* [`signBlob`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signBlob)
* method in the IAM Service Account Credentials API instead. If you currently use this method,
* see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for
* instructions.
*
* Signs a blob using the system-managed private key for a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ServiceAccountName name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* ByteString bytesToSign = ByteString.EMPTY;
* SignBlobResponse response = iAMClient.signBlob(name, bytesToSign);
* }
* }
*
* @param name Required. Deprecated. [Migrate to Service Account Credentials
* API](https://cloud.google.com/iam/help/credentials/migrate-api).
* The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @param bytesToSign Required. Deprecated. [Migrate to Service Account Credentials
* API](https://cloud.google.com/iam/help/credentials/migrate-api).
*
The bytes to sign.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
* @deprecated This method is deprecated and will be removed in the next major version update.
*/
@Deprecated
public final SignBlobResponse signBlob(ServiceAccountName name, ByteString bytesToSign) {
SignBlobRequest request =
SignBlobRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setBytesToSign(bytesToSign)
.build();
return signBlob(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** This method is deprecated. Use the
* [`signBlob`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signBlob)
* method in the IAM Service Account Credentials API instead. If you currently use this method,
* see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for
* instructions.
*
*
Signs a blob using the system-managed private key for a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString();
* ByteString bytesToSign = ByteString.EMPTY;
* SignBlobResponse response = iAMClient.signBlob(name, bytesToSign);
* }
* }
*
* @param name Required. Deprecated. [Migrate to Service Account Credentials
* API](https://cloud.google.com/iam/help/credentials/migrate-api).
* The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @param bytesToSign Required. Deprecated. [Migrate to Service Account Credentials
* API](https://cloud.google.com/iam/help/credentials/migrate-api).
*
The bytes to sign.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
* @deprecated This method is deprecated and will be removed in the next major version update.
*/
@Deprecated
public final SignBlobResponse signBlob(String name, ByteString bytesToSign) {
SignBlobRequest request =
SignBlobRequest.newBuilder().setName(name).setBytesToSign(bytesToSign).build();
return signBlob(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** This method is deprecated. Use the
* [`signBlob`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signBlob)
* method in the IAM Service Account Credentials API instead. If you currently use this method,
* see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for
* instructions.
*
*
Signs a blob using the system-managed private key for a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* SignBlobRequest request =
* SignBlobRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setBytesToSign(ByteString.EMPTY)
* .build();
* SignBlobResponse response = iAMClient.signBlob(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
* @deprecated This method is deprecated and will be removed in the next major version update.
*/
@Deprecated
public final SignBlobResponse signBlob(SignBlobRequest request) {
return signBlobCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** This method is deprecated. Use the
* [`signBlob`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signBlob)
* method in the IAM Service Account Credentials API instead. If you currently use this method,
* see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for
* instructions.
*
* Signs a blob using the system-managed private key for a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* SignBlobRequest request =
* SignBlobRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setBytesToSign(ByteString.EMPTY)
* .build();
* ApiFuture future = iAMClient.signBlobCallable().futureCall(request);
* // Do something.
* SignBlobResponse response = future.get();
* }
* }
*
* @deprecated This method is deprecated and will be removed in the next major version update.
*/
@Deprecated
public final UnaryCallable signBlobCallable() {
return stub.signBlobCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** This method is deprecated. Use the
* [`signJwt`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signJwt)
* method in the IAM Service Account Credentials API instead. If you currently use this method,
* see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for
* instructions.
*
* Signs a JSON Web Token (JWT) using the system-managed private key for a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ServiceAccountName name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* String payload = "payload-786701938";
* SignJwtResponse response = iAMClient.signJwt(name, payload);
* }
* }
*
* @param name Required. Deprecated. [Migrate to Service Account Credentials
* API](https://cloud.google.com/iam/help/credentials/migrate-api).
* The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @param payload Required. Deprecated. [Migrate to Service Account Credentials
* API](https://cloud.google.com/iam/help/credentials/migrate-api).
*
The JWT payload to sign. Must be a serialized JSON object that contains a JWT Claims
* Set. For example: `{"sub": "user{@literal @}example.com", "iat": 313435}`
*
If the JWT Claims Set contains an expiration time (`exp`) claim, it must be an integer
* timestamp that is not in the past and no more than 12 hours in the future.
*
If the JWT Claims Set does not contain an expiration time (`exp`) claim, this claim is
* added automatically, with a timestamp that is 1 hour in the future.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
* @deprecated This method is deprecated and will be removed in the next major version update.
*/
@Deprecated
public final SignJwtResponse signJwt(ServiceAccountName name, String payload) {
SignJwtRequest request =
SignJwtRequest.newBuilder()
.setName(name == null ? null : name.toString())
.setPayload(payload)
.build();
return signJwt(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** This method is deprecated. Use the
* [`signJwt`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signJwt)
* method in the IAM Service Account Credentials API instead. If you currently use this method,
* see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for
* instructions.
*
*
Signs a JSON Web Token (JWT) using the system-managed private key for a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String name = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString();
* String payload = "payload-786701938";
* SignJwtResponse response = iAMClient.signJwt(name, payload);
* }
* }
*
* @param name Required. Deprecated. [Migrate to Service Account Credentials
* API](https://cloud.google.com/iam/help/credentials/migrate-api).
* The resource name of the service account in the following format:
* `projects/{PROJECT_ID}/serviceAccounts/{ACCOUNT}`. Using `-` as a wildcard for the
* `PROJECT_ID` will infer the project from the account. The `ACCOUNT` value can be the
* `email` address or the `unique_id` of the service account.
* @param payload Required. Deprecated. [Migrate to Service Account Credentials
* API](https://cloud.google.com/iam/help/credentials/migrate-api).
*
The JWT payload to sign. Must be a serialized JSON object that contains a JWT Claims
* Set. For example: `{"sub": "user{@literal @}example.com", "iat": 313435}`
*
If the JWT Claims Set contains an expiration time (`exp`) claim, it must be an integer
* timestamp that is not in the past and no more than 12 hours in the future.
*
If the JWT Claims Set does not contain an expiration time (`exp`) claim, this claim is
* added automatically, with a timestamp that is 1 hour in the future.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
* @deprecated This method is deprecated and will be removed in the next major version update.
*/
@Deprecated
public final SignJwtResponse signJwt(String name, String payload) {
SignJwtRequest request = SignJwtRequest.newBuilder().setName(name).setPayload(payload).build();
return signJwt(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** This method is deprecated. Use the
* [`signJwt`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signJwt)
* method in the IAM Service Account Credentials API instead. If you currently use this method,
* see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for
* instructions.
*
*
Signs a JSON Web Token (JWT) using the system-managed private key for a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* SignJwtRequest request =
* SignJwtRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setPayload("payload-786701938")
* .build();
* SignJwtResponse response = iAMClient.signJwt(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
* @deprecated This method is deprecated and will be removed in the next major version update.
*/
@Deprecated
public final SignJwtResponse signJwt(SignJwtRequest request) {
return signJwtCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* **Note:** This method is deprecated. Use the
* [`signJwt`](https://cloud.google.com/iam/help/rest-credentials/v1/projects.serviceAccounts/signJwt)
* method in the IAM Service Account Credentials API instead. If you currently use this method,
* see the [migration guide](https://cloud.google.com/iam/help/credentials/migrate-api) for
* instructions.
*
* Signs a JSON Web Token (JWT) using the system-managed private key for a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* SignJwtRequest request =
* SignJwtRequest.newBuilder()
* .setName(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setPayload("payload-786701938")
* .build();
* ApiFuture future = iAMClient.signJwtCallable().futureCall(request);
* // Do something.
* SignJwtResponse response = future.get();
* }
* }
*
* @deprecated This method is deprecated and will be removed in the next major version update.
*/
@Deprecated
public final UnaryCallable signJwtCallable() {
return stub.signJwtCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
* This IAM policy specifies which principals have access to the service account.
*
* This method does not tell you whether the service account has been granted any roles on
* other resources. To check whether a service account has role grants on a resource, use the
* `getIamPolicy` method for that resource. For example, to view the role grants for a project,
* call the Resource Manager API's
* [`projects.getIamPolicy`](https://cloud.google.com/resource-manager/reference/rest/v1/projects/getIamPolicy)
* method.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ResourceName resource = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* Policy response = iAMClient.getIamPolicy(resource);
* }
* }
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the
* operation documentation for the appropriate value for this field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(ResourceName resource) {
GetIamPolicyRequest request =
GetIamPolicyRequest.newBuilder()
.setResource(resource == null ? null : resource.toString())
.build();
return getIamPolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
* This IAM policy specifies which principals have access to the service account.
*
* This method does not tell you whether the service account has been granted any roles on
* other resources. To check whether a service account has role grants on a resource, use the
* `getIamPolicy` method for that resource. For example, to view the role grants for a project,
* call the Resource Manager API's
* [`projects.getIamPolicy`](https://cloud.google.com/resource-manager/reference/rest/v1/projects/getIamPolicy)
* method.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String resource = ProjectName.of("[PROJECT]").toString();
* Policy response = iAMClient.getIamPolicy(resource);
* }
* }
*
* @param resource REQUIRED: The resource for which the policy is being requested. See the
* operation documentation for the appropriate value for this field.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(String resource) {
GetIamPolicyRequest request = GetIamPolicyRequest.newBuilder().setResource(resource).build();
return getIamPolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
* This IAM policy specifies which principals have access to the service account.
*
* This method does not tell you whether the service account has been granted any roles on
* other resources. To check whether a service account has role grants on a resource, use the
* `getIamPolicy` method for that resource. For example, to view the role grants for a project,
* call the Resource Manager API's
* [`projects.getIamPolicy`](https://cloud.google.com/resource-manager/reference/rest/v1/projects/getIamPolicy)
* method.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* Policy response = iAMClient.getIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy getIamPolicy(GetIamPolicyRequest request) {
return getIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
* This IAM policy specifies which principals have access to the service account.
*
* This method does not tell you whether the service account has been granted any roles on
* other resources. To check whether a service account has role grants on a resource, use the
* `getIamPolicy` method for that resource. For example, to view the role grants for a project,
* call the Resource Manager API's
* [`projects.getIamPolicy`](https://cloud.google.com/resource-manager/reference/rest/v1/projects/getIamPolicy)
* method.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* GetIamPolicyRequest request =
* GetIamPolicyRequest.newBuilder()
* .setResource(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setOptions(GetPolicyOptions.newBuilder().build())
* .build();
* ApiFuture future = iAMClient.getIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable getIamPolicyCallable() {
return stub.getIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Use this method to grant or revoke access to the service account. For example, you could
* grant a principal the ability to impersonate the service account.
*
*
This method does not enable the service account to access other resources. To grant roles to
* a service account on a resource, follow these steps:
*
*
1. Call the resource's `getIamPolicy` method to get its current IAM policy. 2. Edit the
* policy so that it binds the service account to an IAM role for the resource. 3. Call the
* resource's `setIamPolicy` method to update its IAM policy.
*
*
For detailed instructions, see [Manage access to project, folders, and
* organizations](https://cloud.google.com/iam/help/service-accounts/granting-access-to-service-accounts)
* or [Manage access to other
* resources](https://cloud.google.com/iam/help/access/manage-other-resources).
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ResourceName resource = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* Policy policy = Policy.newBuilder().build();
* Policy response = iAMClient.setIamPolicy(resource, policy);
* }
* }
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the
* operation documentation for the appropriate value for this field.
* @param policy REQUIRED: The complete policy to be applied to the `resource`. The size of the
* policy is limited to a few 10s of KB. An empty policy is a valid policy but certain Cloud
* Platform services (such as Projects) might reject them.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(ResourceName resource, Policy policy) {
SetIamPolicyRequest request =
SetIamPolicyRequest.newBuilder()
.setResource(resource == null ? null : resource.toString())
.setPolicy(policy)
.build();
return setIamPolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Use this method to grant or revoke access to the service account. For example, you could
* grant a principal the ability to impersonate the service account.
*
*
This method does not enable the service account to access other resources. To grant roles to
* a service account on a resource, follow these steps:
*
*
1. Call the resource's `getIamPolicy` method to get its current IAM policy. 2. Edit the
* policy so that it binds the service account to an IAM role for the resource. 3. Call the
* resource's `setIamPolicy` method to update its IAM policy.
*
*
For detailed instructions, see [Manage access to project, folders, and
* organizations](https://cloud.google.com/iam/help/service-accounts/granting-access-to-service-accounts)
* or [Manage access to other
* resources](https://cloud.google.com/iam/help/access/manage-other-resources).
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String resource = ProjectName.of("[PROJECT]").toString();
* Policy policy = Policy.newBuilder().build();
* Policy response = iAMClient.setIamPolicy(resource, policy);
* }
* }
*
* @param resource REQUIRED: The resource for which the policy is being specified. See the
* operation documentation for the appropriate value for this field.
* @param policy REQUIRED: The complete policy to be applied to the `resource`. The size of the
* policy is limited to a few 10s of KB. An empty policy is a valid policy but certain Cloud
* Platform services (such as Projects) might reject them.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(String resource, Policy policy) {
SetIamPolicyRequest request =
SetIamPolicyRequest.newBuilder().setResource(resource).setPolicy(policy).build();
return setIamPolicy(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Use this method to grant or revoke access to the service account. For example, you could
* grant a principal the ability to impersonate the service account.
*
*
This method does not enable the service account to access other resources. To grant roles to
* a service account on a resource, follow these steps:
*
*
1. Call the resource's `getIamPolicy` method to get its current IAM policy. 2. Edit the
* policy so that it binds the service account to an IAM role for the resource. 3. Call the
* resource's `setIamPolicy` method to update its IAM policy.
*
*
For detailed instructions, see [Manage access to project, folders, and
* organizations](https://cloud.google.com/iam/help/service-accounts/granting-access-to-service-accounts)
* or [Manage access to other
* resources](https://cloud.google.com/iam/help/access/manage-other-resources).
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Policy response = iAMClient.setIamPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Policy setIamPolicy(SetIamPolicyRequest request) {
return setIamPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Sets the IAM policy that is attached to a [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Use this method to grant or revoke access to the service account. For example, you could
* grant a principal the ability to impersonate the service account.
*
*
This method does not enable the service account to access other resources. To grant roles to
* a service account on a resource, follow these steps:
*
*
1. Call the resource's `getIamPolicy` method to get its current IAM policy. 2. Edit the
* policy so that it binds the service account to an IAM role for the resource. 3. Call the
* resource's `setIamPolicy` method to update its IAM policy.
*
*
For detailed instructions, see [Manage access to project, folders, and
* organizations](https://cloud.google.com/iam/help/service-accounts/granting-access-to-service-accounts)
* or [Manage access to other
* resources](https://cloud.google.com/iam/help/access/manage-other-resources).
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* SetIamPolicyRequest request =
* SetIamPolicyRequest.newBuilder()
* .setResource(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .setPolicy(Policy.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = iAMClient.setIamPolicyCallable().futureCall(request);
* // Do something.
* Policy response = future.get();
* }
* }
*/
public final UnaryCallable setIamPolicyCallable() {
return stub.setIamPolicyCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tests whether the caller has the specified permissions on a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ResourceName resource = ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]");
* List permissions = new ArrayList<>();
* TestIamPermissionsResponse response = iAMClient.testIamPermissions(resource, permissions);
* }
* }
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
* @param permissions The set of permissions to check for the `resource`. Permissions with
* wildcards (such as '*' or 'storage.*') are not allowed. For more information see
* [IAM Overview](https://cloud.google.com/iam/docs/overview#permissions).
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(
ResourceName resource, List permissions) {
TestIamPermissionsRequest request =
TestIamPermissionsRequest.newBuilder()
.setResource(resource == null ? null : resource.toString())
.addAllPermissions(permissions)
.build();
return testIamPermissions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tests whether the caller has the specified permissions on a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String resource = ProjectName.of("[PROJECT]").toString();
* List permissions = new ArrayList<>();
* TestIamPermissionsResponse response = iAMClient.testIamPermissions(resource, permissions);
* }
* }
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See the
* operation documentation for the appropriate value for this field.
* @param permissions The set of permissions to check for the `resource`. Permissions with
* wildcards (such as '*' or 'storage.*') are not allowed. For more information see
* [IAM Overview](https://cloud.google.com/iam/docs/overview#permissions).
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(
String resource, List permissions) {
TestIamPermissionsRequest request =
TestIamPermissionsRequest.newBuilder()
.setResource(resource)
.addAllPermissions(permissions)
.build();
return testIamPermissions(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tests whether the caller has the specified permissions on a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* TestIamPermissionsResponse response = iAMClient.testIamPermissions(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final TestIamPermissionsResponse testIamPermissions(TestIamPermissionsRequest request) {
return testIamPermissionsCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Tests whether the caller has the specified permissions on a
* [ServiceAccount][google.iam.admin.v1.ServiceAccount].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* TestIamPermissionsRequest request =
* TestIamPermissionsRequest.newBuilder()
* .setResource(ServiceAccountName.of("[PROJECT]", "[SERVICE_ACCOUNT]").toString())
* .addAllPermissions(new ArrayList())
* .build();
* ApiFuture future =
* iAMClient.testIamPermissionsCallable().futureCall(request);
* // Do something.
* TestIamPermissionsResponse response = future.get();
* }
* }
*/
public final UnaryCallable
testIamPermissionsCallable() {
return stub.testIamPermissionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists roles that can be granted on a Google Cloud resource. A role is grantable if the IAM
* policy for the resource can contain bindings to the role.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* String fullResourceName = "fullResourceName-853732376";
* for (Role element : iAMClient.queryGrantableRoles(fullResourceName).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param fullResourceName Required. The full resource name to query from the list of grantable
* roles.
* The name follows the Google Cloud Platform resource format. For example, a Cloud
* Platform project with id `my-project` will be named
* `//cloudresourcemanager.googleapis.com/projects/my-project`.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueryGrantableRolesPagedResponse queryGrantableRoles(String fullResourceName) {
QueryGrantableRolesRequest request =
QueryGrantableRolesRequest.newBuilder().setFullResourceName(fullResourceName).build();
return queryGrantableRoles(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists roles that can be granted on a Google Cloud resource. A role is grantable if the IAM
* policy for the resource can contain bindings to the role.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* QueryGrantableRolesRequest request =
* QueryGrantableRolesRequest.newBuilder()
* .setFullResourceName("fullResourceName-853732376")
* .setView(RoleView.forNumber(0))
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Role element : iAMClient.queryGrantableRoles(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueryGrantableRolesPagedResponse queryGrantableRoles(
QueryGrantableRolesRequest request) {
return queryGrantableRolesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists roles that can be granted on a Google Cloud resource. A role is grantable if the IAM
* policy for the resource can contain bindings to the role.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* QueryGrantableRolesRequest request =
* QueryGrantableRolesRequest.newBuilder()
* .setFullResourceName("fullResourceName-853732376")
* .setView(RoleView.forNumber(0))
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future = iAMClient.queryGrantableRolesPagedCallable().futureCall(request);
* // Do something.
* for (Role element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
queryGrantableRolesPagedCallable() {
return stub.queryGrantableRolesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists roles that can be granted on a Google Cloud resource. A role is grantable if the IAM
* policy for the resource can contain bindings to the role.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* QueryGrantableRolesRequest request =
* QueryGrantableRolesRequest.newBuilder()
* .setFullResourceName("fullResourceName-853732376")
* .setView(RoleView.forNumber(0))
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* QueryGrantableRolesResponse response =
* iAMClient.queryGrantableRolesCallable().call(request);
* for (Role element : response.getRolesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
queryGrantableRolesCallable() {
return stub.queryGrantableRolesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every predefined [Role][google.iam.admin.v1.Role] that IAM supports, or every custom role
* that is defined for an organization or project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ListRolesRequest request =
* ListRolesRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setView(RoleView.forNumber(0))
* .setShowDeleted(true)
* .build();
* for (Role element : iAMClient.listRoles(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final ListRolesPagedResponse listRoles(ListRolesRequest request) {
return listRolesPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every predefined [Role][google.iam.admin.v1.Role] that IAM supports, or every custom role
* that is defined for an organization or project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ListRolesRequest request =
* ListRolesRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setView(RoleView.forNumber(0))
* .setShowDeleted(true)
* .build();
* ApiFuture future = iAMClient.listRolesPagedCallable().futureCall(request);
* // Do something.
* for (Role element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable listRolesPagedCallable() {
return stub.listRolesPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every predefined [Role][google.iam.admin.v1.Role] that IAM supports, or every custom role
* that is defined for an organization or project.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* ListRolesRequest request =
* ListRolesRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .setView(RoleView.forNumber(0))
* .setShowDeleted(true)
* .build();
* while (true) {
* ListRolesResponse response = iAMClient.listRolesCallable().call(request);
* for (Role element : response.getRolesList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable listRolesCallable() {
return stub.listRolesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the definition of a [Role][google.iam.admin.v1.Role].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* GetRoleRequest request =
* GetRoleRequest.newBuilder().setName("GetRoleRequest84528163".toString()).build();
* Role response = iAMClient.getRole(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Role getRole(GetRoleRequest request) {
return getRoleCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Gets the definition of a [Role][google.iam.admin.v1.Role].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* GetRoleRequest request =
* GetRoleRequest.newBuilder().setName("GetRoleRequest84528163".toString()).build();
* ApiFuture future = iAMClient.getRoleCallable().futureCall(request);
* // Do something.
* Role response = future.get();
* }
* }
*/
public final UnaryCallable getRoleCallable() {
return stub.getRoleCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new custom [Role][google.iam.admin.v1.Role].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* CreateRoleRequest request =
* CreateRoleRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setRoleId("roleId-925416399")
* .setRole(Role.newBuilder().build())
* .build();
* Role response = iAMClient.createRole(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Role createRole(CreateRoleRequest request) {
return createRoleCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Creates a new custom [Role][google.iam.admin.v1.Role].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* CreateRoleRequest request =
* CreateRoleRequest.newBuilder()
* .setParent(ProjectName.of("[PROJECT]").toString())
* .setRoleId("roleId-925416399")
* .setRole(Role.newBuilder().build())
* .build();
* ApiFuture future = iAMClient.createRoleCallable().futureCall(request);
* // Do something.
* Role response = future.get();
* }
* }
*/
public final UnaryCallable createRoleCallable() {
return stub.createRoleCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the definition of a custom [Role][google.iam.admin.v1.Role].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* UpdateRoleRequest request =
* UpdateRoleRequest.newBuilder()
* .setName("UpdateRoleRequest230834320".toString())
* .setRole(Role.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* Role response = iAMClient.updateRole(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Role updateRole(UpdateRoleRequest request) {
return updateRoleCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Updates the definition of a custom [Role][google.iam.admin.v1.Role].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* UpdateRoleRequest request =
* UpdateRoleRequest.newBuilder()
* .setName("UpdateRoleRequest230834320".toString())
* .setRole(Role.newBuilder().build())
* .setUpdateMask(FieldMask.newBuilder().build())
* .build();
* ApiFuture future = iAMClient.updateRoleCallable().futureCall(request);
* // Do something.
* Role response = future.get();
* }
* }
*/
public final UnaryCallable updateRoleCallable() {
return stub.updateRoleCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a custom [Role][google.iam.admin.v1.Role].
*
* When you delete a custom role, the following changes occur immediately:
*
*
* - You cannot bind a principal to the custom role in an IAM [Policy][google.iam.v1.Policy].
*
- Existing bindings to the custom role are not changed, but they have no effect.
*
- By default, the response from [ListRoles][google.iam.admin.v1.IAM.ListRoles] does not
* include the custom role.
*
*
* You have 7 days to undelete the custom role. After 7 days, the following changes occur:
*
*
* - The custom role is permanently deleted and cannot be recovered.
*
- If an IAM policy contains a binding to the custom role, the binding is permanently
* removed.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DeleteRoleRequest request =
* DeleteRoleRequest.newBuilder()
* .setName("DeleteRoleRequest1468559982".toString())
* .setEtag(ByteString.EMPTY)
* .build();
* Role response = iAMClient.deleteRole(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Role deleteRole(DeleteRoleRequest request) {
return deleteRoleCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Deletes a custom [Role][google.iam.admin.v1.Role].
*
* When you delete a custom role, the following changes occur immediately:
*
*
* - You cannot bind a principal to the custom role in an IAM [Policy][google.iam.v1.Policy].
*
- Existing bindings to the custom role are not changed, but they have no effect.
*
- By default, the response from [ListRoles][google.iam.admin.v1.IAM.ListRoles] does not
* include the custom role.
*
*
* You have 7 days to undelete the custom role. After 7 days, the following changes occur:
*
*
* - The custom role is permanently deleted and cannot be recovered.
*
- If an IAM policy contains a binding to the custom role, the binding is permanently
* removed.
*
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* DeleteRoleRequest request =
* DeleteRoleRequest.newBuilder()
* .setName("DeleteRoleRequest1468559982".toString())
* .setEtag(ByteString.EMPTY)
* .build();
* ApiFuture future = iAMClient.deleteRoleCallable().futureCall(request);
* // Do something.
* Role response = future.get();
* }
* }
*/
public final UnaryCallable deleteRoleCallable() {
return stub.deleteRoleCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Undeletes a custom [Role][google.iam.admin.v1.Role].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* UndeleteRoleRequest request =
* UndeleteRoleRequest.newBuilder()
* .setName("UndeleteRoleRequest755355893".toString())
* .setEtag(ByteString.EMPTY)
* .build();
* Role response = iAMClient.undeleteRole(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final Role undeleteRole(UndeleteRoleRequest request) {
return undeleteRoleCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Undeletes a custom [Role][google.iam.admin.v1.Role].
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* UndeleteRoleRequest request =
* UndeleteRoleRequest.newBuilder()
* .setName("UndeleteRoleRequest755355893".toString())
* .setEtag(ByteString.EMPTY)
* .build();
* ApiFuture future = iAMClient.undeleteRoleCallable().futureCall(request);
* // Do something.
* Role response = future.get();
* }
* }
*/
public final UnaryCallable undeleteRoleCallable() {
return stub.undeleteRoleCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every permission that you can test on a resource. A permission is testable if you can
* check whether a principal has that permission on the resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* QueryTestablePermissionsRequest request =
* QueryTestablePermissionsRequest.newBuilder()
* .setFullResourceName("fullResourceName-853732376")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* for (Permission element : iAMClient.queryTestablePermissions(request).iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueryTestablePermissionsPagedResponse queryTestablePermissions(
QueryTestablePermissionsRequest request) {
return queryTestablePermissionsPagedCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every permission that you can test on a resource. A permission is testable if you can
* check whether a principal has that permission on the resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* QueryTestablePermissionsRequest request =
* QueryTestablePermissionsRequest.newBuilder()
* .setFullResourceName("fullResourceName-853732376")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* ApiFuture future =
* iAMClient.queryTestablePermissionsPagedCallable().futureCall(request);
* // Do something.
* for (Permission element : future.get().iterateAll()) {
* // doThingsWith(element);
* }
* }
* }
*/
public final UnaryCallable
queryTestablePermissionsPagedCallable() {
return stub.queryTestablePermissionsPagedCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lists every permission that you can test on a resource. A permission is testable if you can
* check whether a principal has that permission on the resource.
*
* Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* QueryTestablePermissionsRequest request =
* QueryTestablePermissionsRequest.newBuilder()
* .setFullResourceName("fullResourceName-853732376")
* .setPageSize(883849137)
* .setPageToken("pageToken873572522")
* .build();
* while (true) {
* QueryTestablePermissionsResponse response =
* iAMClient.queryTestablePermissionsCallable().call(request);
* for (Permission element : response.getPermissionsList()) {
* // doThingsWith(element);
* }
* String nextPageToken = response.getNextPageToken();
* if (!Strings.isNullOrEmpty(nextPageToken)) {
* request = request.toBuilder().setPageToken(nextPageToken).build();
* } else {
* break;
* }
* }
* }
* }
*/
public final UnaryCallable
queryTestablePermissionsCallable() {
return stub.queryTestablePermissionsCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of services that allow you to opt into audit logs that are not generated by
* default.
*
* To learn more about audit logs, see the [Logging
* documentation](https://cloud.google.com/logging/docs/audit).
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* QueryAuditableServicesRequest request =
* QueryAuditableServicesRequest.newBuilder()
* .setFullResourceName("fullResourceName-853732376")
* .build();
* QueryAuditableServicesResponse response = iAMClient.queryAuditableServices(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final QueryAuditableServicesResponse queryAuditableServices(
QueryAuditableServicesRequest request) {
return queryAuditableServicesCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Returns a list of services that allow you to opt into audit logs that are not generated by
* default.
*
* To learn more about audit logs, see the [Logging
* documentation](https://cloud.google.com/logging/docs/audit).
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* QueryAuditableServicesRequest request =
* QueryAuditableServicesRequest.newBuilder()
* .setFullResourceName("fullResourceName-853732376")
* .build();
* ApiFuture future =
* iAMClient.queryAuditableServicesCallable().futureCall(request);
* // Do something.
* QueryAuditableServicesResponse response = future.get();
* }
* }
*/
public final UnaryCallable
queryAuditableServicesCallable() {
return stub.queryAuditableServicesCallable();
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lints, or validates, an IAM policy. Currently checks the
* [google.iam.v1.Binding.condition][google.iam.v1.Binding.condition] field, which contains a
* condition expression for a role binding.
*
* Successful calls to this method always return an HTTP `200 OK` status code, even if the
* linter detects an issue in the IAM policy.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* LintPolicyRequest request =
* LintPolicyRequest.newBuilder().setFullResourceName("fullResourceName-853732376").build();
* LintPolicyResponse response = iAMClient.lintPolicy(request);
* }
* }
*
* @param request The request object containing all of the parameters for the API call.
* @throws com.google.api.gax.rpc.ApiException if the remote call fails
*/
public final LintPolicyResponse lintPolicy(LintPolicyRequest request) {
return lintPolicyCallable().call(request);
}
// AUTO-GENERATED DOCUMENTATION AND METHOD.
/**
* Lints, or validates, an IAM policy. Currently checks the
* [google.iam.v1.Binding.condition][google.iam.v1.Binding.condition] field, which contains a
* condition expression for a role binding.
*
* Successful calls to this method always return an HTTP `200 OK` status code, even if the
* linter detects an issue in the IAM policy.
*
*
Sample code:
*
*
{@code
* // This snippet has been automatically generated and should be regarded as a code template only.
* // It will require modifications to work:
* // - It may require correct/in-range values for request initialization.
* // - It may require specifying regional endpoints when creating the service client as shown in
* // https://cloud.google.com/java/docs/setup#configure_endpoints_for_the_client_library
* try (IAMClient iAMClient = IAMClient.create()) {
* LintPolicyRequest request =
* LintPolicyRequest.newBuilder().setFullResourceName("fullResourceName-853732376").build();
* ApiFuture future = iAMClient.lintPolicyCallable().futureCall(request);
* // Do something.
* LintPolicyResponse response = future.get();
* }
* }
*/
public final UnaryCallable lintPolicyCallable() {
return stub.lintPolicyCallable();
}
@Override
public final void close() {
stub.close();
}
@Override
public void shutdown() {
stub.shutdown();
}
@Override
public boolean isShutdown() {
return stub.isShutdown();
}
@Override
public boolean isTerminated() {
return stub.isTerminated();
}
@Override
public void shutdownNow() {
stub.shutdownNow();
}
@Override
public boolean awaitTermination(long duration, TimeUnit unit) throws InterruptedException {
return stub.awaitTermination(duration, unit);
}
public static class ListServiceAccountsPagedResponse
extends AbstractPagedListResponse<
ListServiceAccountsRequest,
ListServiceAccountsResponse,
ServiceAccount,
ListServiceAccountsPage,
ListServiceAccountsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListServiceAccountsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new ListServiceAccountsPagedResponse(input),
MoreExecutors.directExecutor());
}
private ListServiceAccountsPagedResponse(ListServiceAccountsPage page) {
super(page, ListServiceAccountsFixedSizeCollection.createEmptyCollection());
}
}
public static class ListServiceAccountsPage
extends AbstractPage<
ListServiceAccountsRequest,
ListServiceAccountsResponse,
ServiceAccount,
ListServiceAccountsPage> {
private ListServiceAccountsPage(
PageContext
context,
ListServiceAccountsResponse response) {
super(context, response);
}
private static ListServiceAccountsPage createEmptyPage() {
return new ListServiceAccountsPage(null, null);
}
@Override
protected ListServiceAccountsPage createPage(
PageContext
context,
ListServiceAccountsResponse response) {
return new ListServiceAccountsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListServiceAccountsFixedSizeCollection
extends AbstractFixedSizeCollection<
ListServiceAccountsRequest,
ListServiceAccountsResponse,
ServiceAccount,
ListServiceAccountsPage,
ListServiceAccountsFixedSizeCollection> {
private ListServiceAccountsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListServiceAccountsFixedSizeCollection createEmptyCollection() {
return new ListServiceAccountsFixedSizeCollection(null, 0);
}
@Override
protected ListServiceAccountsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListServiceAccountsFixedSizeCollection(pages, collectionSize);
}
}
public static class QueryGrantableRolesPagedResponse
extends AbstractPagedListResponse<
QueryGrantableRolesRequest,
QueryGrantableRolesResponse,
Role,
QueryGrantableRolesPage,
QueryGrantableRolesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
QueryGrantableRolesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new QueryGrantableRolesPagedResponse(input),
MoreExecutors.directExecutor());
}
private QueryGrantableRolesPagedResponse(QueryGrantableRolesPage page) {
super(page, QueryGrantableRolesFixedSizeCollection.createEmptyCollection());
}
}
public static class QueryGrantableRolesPage
extends AbstractPage<
QueryGrantableRolesRequest, QueryGrantableRolesResponse, Role, QueryGrantableRolesPage> {
private QueryGrantableRolesPage(
PageContext context,
QueryGrantableRolesResponse response) {
super(context, response);
}
private static QueryGrantableRolesPage createEmptyPage() {
return new QueryGrantableRolesPage(null, null);
}
@Override
protected QueryGrantableRolesPage createPage(
PageContext context,
QueryGrantableRolesResponse response) {
return new QueryGrantableRolesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class QueryGrantableRolesFixedSizeCollection
extends AbstractFixedSizeCollection<
QueryGrantableRolesRequest,
QueryGrantableRolesResponse,
Role,
QueryGrantableRolesPage,
QueryGrantableRolesFixedSizeCollection> {
private QueryGrantableRolesFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static QueryGrantableRolesFixedSizeCollection createEmptyCollection() {
return new QueryGrantableRolesFixedSizeCollection(null, 0);
}
@Override
protected QueryGrantableRolesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new QueryGrantableRolesFixedSizeCollection(pages, collectionSize);
}
}
public static class ListRolesPagedResponse
extends AbstractPagedListResponse<
ListRolesRequest, ListRolesResponse, Role, ListRolesPage, ListRolesFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext context,
ApiFuture futureResponse) {
ApiFuture futurePage =
ListRolesPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage, input -> new ListRolesPagedResponse(input), MoreExecutors.directExecutor());
}
private ListRolesPagedResponse(ListRolesPage page) {
super(page, ListRolesFixedSizeCollection.createEmptyCollection());
}
}
public static class ListRolesPage
extends AbstractPage {
private ListRolesPage(
PageContext context,
ListRolesResponse response) {
super(context, response);
}
private static ListRolesPage createEmptyPage() {
return new ListRolesPage(null, null);
}
@Override
protected ListRolesPage createPage(
PageContext context,
ListRolesResponse response) {
return new ListRolesPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class ListRolesFixedSizeCollection
extends AbstractFixedSizeCollection<
ListRolesRequest, ListRolesResponse, Role, ListRolesPage, ListRolesFixedSizeCollection> {
private ListRolesFixedSizeCollection(List pages, int collectionSize) {
super(pages, collectionSize);
}
private static ListRolesFixedSizeCollection createEmptyCollection() {
return new ListRolesFixedSizeCollection(null, 0);
}
@Override
protected ListRolesFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new ListRolesFixedSizeCollection(pages, collectionSize);
}
}
public static class QueryTestablePermissionsPagedResponse
extends AbstractPagedListResponse<
QueryTestablePermissionsRequest,
QueryTestablePermissionsResponse,
Permission,
QueryTestablePermissionsPage,
QueryTestablePermissionsFixedSizeCollection> {
public static ApiFuture createAsync(
PageContext
context,
ApiFuture futureResponse) {
ApiFuture futurePage =
QueryTestablePermissionsPage.createEmptyPage().createPageAsync(context, futureResponse);
return ApiFutures.transform(
futurePage,
input -> new QueryTestablePermissionsPagedResponse(input),
MoreExecutors.directExecutor());
}
private QueryTestablePermissionsPagedResponse(QueryTestablePermissionsPage page) {
super(page, QueryTestablePermissionsFixedSizeCollection.createEmptyCollection());
}
}
public static class QueryTestablePermissionsPage
extends AbstractPage<
QueryTestablePermissionsRequest,
QueryTestablePermissionsResponse,
Permission,
QueryTestablePermissionsPage> {
private QueryTestablePermissionsPage(
PageContext
context,
QueryTestablePermissionsResponse response) {
super(context, response);
}
private static QueryTestablePermissionsPage createEmptyPage() {
return new QueryTestablePermissionsPage(null, null);
}
@Override
protected QueryTestablePermissionsPage createPage(
PageContext
context,
QueryTestablePermissionsResponse response) {
return new QueryTestablePermissionsPage(context, response);
}
@Override
public ApiFuture createPageAsync(
PageContext
context,
ApiFuture futureResponse) {
return super.createPageAsync(context, futureResponse);
}
}
public static class QueryTestablePermissionsFixedSizeCollection
extends AbstractFixedSizeCollection<
QueryTestablePermissionsRequest,
QueryTestablePermissionsResponse,
Permission,
QueryTestablePermissionsPage,
QueryTestablePermissionsFixedSizeCollection> {
private QueryTestablePermissionsFixedSizeCollection(
List pages, int collectionSize) {
super(pages, collectionSize);
}
private static QueryTestablePermissionsFixedSizeCollection createEmptyCollection() {
return new QueryTestablePermissionsFixedSizeCollection(null, 0);
}
@Override
protected QueryTestablePermissionsFixedSizeCollection createCollection(
List pages, int collectionSize) {
return new QueryTestablePermissionsFixedSizeCollection(pages, collectionSize);
}
}
}