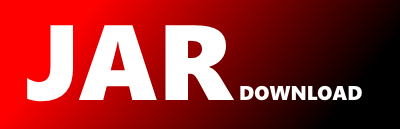
com.google.cloud.pubsublite.beam.AutoValue_PublisherOptions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pubsublite-beam-io Show documentation
Show all versions of pubsublite-beam-io Show documentation
Beam IO for Google Cloud Pub/Sub Lite
package com.google.cloud.pubsublite.beam;
import com.google.cloud.pubsublite.TopicPath;
import javax.annotation.Generated;
import org.checkerframework.checker.nullness.qual.Nullable;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_PublisherOptions extends PublisherOptions {
private final TopicPath topicPath;
private final @Nullable SerializableSupplier
© 2015 - 2024 Weber Informatics LLC | Privacy Policy