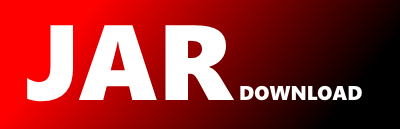
cltool4j.args4j.CalendarParser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cltool4j Show documentation
Show all versions of cltool4j Show documentation
Command-line infrastructure for Java
The newest version!
package cltool4j.args4j;
import java.text.SimpleDateFormat;
import java.util.Calendar;
import java.util.Date;
/**
* {@link ArgumentParser} for {@link Calendar}s. Parses a number of standard (and not-so-standard) date and
* time formats, listed below in {@link SimpleDateFormat} syntax:
*
*
* Dot-separated
*
* - yyyy.MM.dd.HH.mm.ss.SSS.ZZZ
* - yyyy.MM.dd.HH.mm.ss.SSS
* - yyyy.MM.dd.HH.mm.ss
* - yyyy.MM.dd.HH.mm
* - yyyy.MM.dd.HH.mm
*
*
* Dot- and colon-separated, with time
*
* - yyyy.MM.dd HH:mm:ss.SSS ZZZ
* - yyyy.MM.dd HH:mm:ss.SSS
* - yyyy.MM.dd HH:mm:ss
* - yyyy.MM.dd HH:mm
* - MM.dd.yyyy HH:mm:ss
* - MM.dd.yy HH:mm:ss
* - MM.dd HH:mm:ss
* - MM.dd.yyyy HH:mm
* - MM.dd.yy HH:mm
* - MM.dd HH:mm
*
*
* Dot-separated, without time
*
* - yyyy.MM.dd
* - MM.dd.yyyy
* - MM.dd.yy
* - MM.dd
*
*
* Slash-separated, with time
*
* - MM/dd/yyyy HH:mm:ss
* - MM/dd/yy HH:mm:ss
* - MM/dd HH:mm:ss
* - MM/dd/yyyy HH:mm
* - MM/dd/yy HH:mm
* - MM/dd HH:mm
*
*
* Slash-separated, without time
*
* - yyyy/MM/dd
* - MM/dd/yyyy
* - MM/dd/yy
* - MM/dd
*
*
* @author Aaron Dunlop
*
*/
public class CalendarParser extends ArgumentParser {
protected final static SimpleDateFormat COMMANDLINE_DATE_FORMATS[] = new SimpleDateFormat[] {
// Dash-separated, without time
new SimpleDateFormat("yyyy-MM-dd"),
new SimpleDateFormat("MM-dd-yyyy"),
new SimpleDateFormat("MM-dd-yy"),
new SimpleDateFormat("MM-dd"),
// Dash-separated, with time
new SimpleDateFormat("MM-dd-yyyy HH:mm:ss"),
new SimpleDateFormat("MM-dd-yy HH:mm:ss"),
new SimpleDateFormat("MM-dd HH:mm:ss"),
new SimpleDateFormat("MM-dd-yyyy HH:mm"),
new SimpleDateFormat("MM-dd-yy HH:mm"),
new SimpleDateFormat("MM-dd HH:mm"),
// Dot-separated, with time
new SimpleDateFormat("yyyy.MM.dd.HH.mm.ss.SSS.ZZZ"),
new SimpleDateFormat("yyyy.MM.dd.HH.mm.ss.SSS"),
new SimpleDateFormat("yyyy.MM.dd.HH.mm.ss"),
new SimpleDateFormat("yyyy.MM.dd.HH.mm"),
new SimpleDateFormat("yyyy.MM.dd.HH.mm"),
// Dot- and colon-separated, with time
new SimpleDateFormat("yyyy.MM.dd HH:mm:ss.SSS ZZZ"),
new SimpleDateFormat("yyyy.MM.dd HH:mm:ss.SSS"), new SimpleDateFormat("yyyy.MM.dd HH:mm:ss"),
new SimpleDateFormat("yyyy.MM.dd HH:mm"),
new SimpleDateFormat("MM.dd.yyyy HH:mm:ss"),
new SimpleDateFormat("MM.dd.yy HH:mm:ss"),
new SimpleDateFormat("MM.dd HH:mm:ss"),
new SimpleDateFormat("MM.dd.yyyy HH:mm"),
new SimpleDateFormat("MM.dd.yy HH:mm"),
new SimpleDateFormat("MM.dd HH:mm"),
// Dot-separated, without time
new SimpleDateFormat("yyyy.MM.dd"),
new SimpleDateFormat("MM.dd.yyyy"),
new SimpleDateFormat("MM.dd.yy"),
new SimpleDateFormat("MM.dd"),
// Slash-separated, with time
new SimpleDateFormat("MM/dd/yyyy HH:mm:ss"), new SimpleDateFormat("MM/dd/yy HH:mm:ss"),
new SimpleDateFormat("MM/dd HH:mm:ss"), new SimpleDateFormat("MM/dd/yyyy HH:mm"),
new SimpleDateFormat("MM/dd/yy HH:mm"), new SimpleDateFormat("MM/dd HH:mm"),
// Slash-separated, without time
new SimpleDateFormat("yyyy/MM/dd"), new SimpleDateFormat("MM/dd/yyyy"),
new SimpleDateFormat("MM/dd/yy"), new SimpleDateFormat("MM/dd") };
static {
for (final SimpleDateFormat formatter : COMMANDLINE_DATE_FORMATS) {
formatter.setLenient(false);
}
}
public static Calendar parseDate(final String arg) {
final Calendar c = Calendar.getInstance();
final int year = c.get(Calendar.YEAR);
for (final SimpleDateFormat dateFormat : COMMANDLINE_DATE_FORMATS) {
try {
final Date d = dateFormat.parse(arg);
c.setTime(d);
if (c.get(Calendar.YEAR) == 1970) {
c.set(Calendar.YEAR, year);
}
return c;
} catch (final java.text.ParseException ignore) {
}
}
throw new IllegalArgumentException();
}
@Override
public Calendar parse(final String arg) throws IllegalArgumentException {
return parseDate(arg);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy