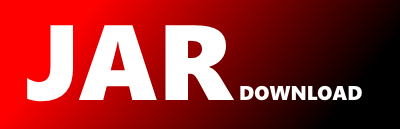
com.google.code.facebookapi.schema.ApplicationGetPublicInfoResponse Maven / Gradle / Ivy
Show all versions of facebook-java-api-schema Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-661
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2009.02.15 at 07:02:48 PM GMT-08:00
//
package com.google.code.facebookapi.schema;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.builder.JAXBEqualsBuilder;
import org.jvnet.jaxb2_commons.lang.builder.JAXBHashCodeBuilder;
import org.jvnet.jaxb2_commons.lang.builder.JAXBToStringBuilder;
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="app_id" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="api_key" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="canvas_name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="display_name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="icon_url" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="logo_url" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="developers">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence maxOccurs="unbounded" minOccurs="0">
* <element name="developer_info" type="{http://api.facebook.com/1.0/}developer_info" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="list" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="company_name" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="daily_active_users" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="weekly_active_users" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="monthly_active_users" type="{http://www.w3.org/2001/XMLSchema}long"/>
* <element name="daily_active_percentage" type="{http://www.w3.org/2001/XMLSchema}int"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"appId",
"apiKey",
"canvasName",
"displayName",
"iconUrl",
"logoUrl",
"developers",
"companyName",
"description",
"dailyActiveUsers",
"weeklyActiveUsers",
"monthlyActiveUsers",
"dailyActivePercentage"
})
@XmlRootElement(name = "application_getPublicInfo_response")
public class ApplicationGetPublicInfoResponse
implements Equals, HashCode, ToString
{
@XmlElement(name = "app_id")
protected long appId;
@XmlElement(name = "api_key", required = true)
protected String apiKey;
@XmlElement(name = "canvas_name", required = true)
protected String canvasName;
@XmlElement(name = "display_name", required = true)
protected String displayName;
@XmlElement(name = "icon_url", required = true)
protected String iconUrl;
@XmlElement(name = "logo_url", required = true)
protected String logoUrl;
@XmlElement(required = true)
protected ApplicationGetPublicInfoResponse.Developers developers;
@XmlElement(name = "company_name", required = true)
protected String companyName;
@XmlElement(required = true)
protected String description;
@XmlElement(name = "daily_active_users")
protected long dailyActiveUsers;
@XmlElement(name = "weekly_active_users")
protected long weeklyActiveUsers;
@XmlElement(name = "monthly_active_users")
protected long monthlyActiveUsers;
@XmlElement(name = "daily_active_percentage")
protected int dailyActivePercentage;
/**
* Gets the value of the appId property.
*
*/
public long getAppId() {
return appId;
}
/**
* Sets the value of the appId property.
*
*/
public void setAppId(long value) {
this.appId = value;
}
/**
* Gets the value of the apiKey property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getApiKey() {
return apiKey;
}
/**
* Sets the value of the apiKey property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setApiKey(String value) {
this.apiKey = value;
}
/**
* Gets the value of the canvasName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCanvasName() {
return canvasName;
}
/**
* Sets the value of the canvasName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCanvasName(String value) {
this.canvasName = value;
}
/**
* Gets the value of the displayName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDisplayName() {
return displayName;
}
/**
* Sets the value of the displayName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDisplayName(String value) {
this.displayName = value;
}
/**
* Gets the value of the iconUrl property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIconUrl() {
return iconUrl;
}
/**
* Sets the value of the iconUrl property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIconUrl(String value) {
this.iconUrl = value;
}
/**
* Gets the value of the logoUrl property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLogoUrl() {
return logoUrl;
}
/**
* Sets the value of the logoUrl property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLogoUrl(String value) {
this.logoUrl = value;
}
/**
* Gets the value of the developers property.
*
* @return
* possible object is
* {@link ApplicationGetPublicInfoResponse.Developers }
*
*/
public ApplicationGetPublicInfoResponse.Developers getDevelopers() {
return developers;
}
/**
* Sets the value of the developers property.
*
* @param value
* allowed object is
* {@link ApplicationGetPublicInfoResponse.Developers }
*
*/
public void setDevelopers(ApplicationGetPublicInfoResponse.Developers value) {
this.developers = value;
}
/**
* Gets the value of the companyName property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCompanyName() {
return companyName;
}
/**
* Sets the value of the companyName property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCompanyName(String value) {
this.companyName = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the dailyActiveUsers property.
*
*/
public long getDailyActiveUsers() {
return dailyActiveUsers;
}
/**
* Sets the value of the dailyActiveUsers property.
*
*/
public void setDailyActiveUsers(long value) {
this.dailyActiveUsers = value;
}
/**
* Gets the value of the weeklyActiveUsers property.
*
*/
public long getWeeklyActiveUsers() {
return weeklyActiveUsers;
}
/**
* Sets the value of the weeklyActiveUsers property.
*
*/
public void setWeeklyActiveUsers(long value) {
this.weeklyActiveUsers = value;
}
/**
* Gets the value of the monthlyActiveUsers property.
*
*/
public long getMonthlyActiveUsers() {
return monthlyActiveUsers;
}
/**
* Sets the value of the monthlyActiveUsers property.
*
*/
public void setMonthlyActiveUsers(long value) {
this.monthlyActiveUsers = value;
}
/**
* Gets the value of the dailyActivePercentage property.
*
*/
public int getDailyActivePercentage() {
return dailyActivePercentage;
}
/**
* Sets the value of the dailyActivePercentage property.
*
*/
public void setDailyActivePercentage(int value) {
this.dailyActivePercentage = value;
}
public void toString(ToStringBuilder toStringBuilder) {
{
long theAppId;
theAppId = this.getAppId();
toStringBuilder.append("appId", theAppId);
}
{
String theApiKey;
theApiKey = this.getApiKey();
toStringBuilder.append("apiKey", theApiKey);
}
{
String theCanvasName;
theCanvasName = this.getCanvasName();
toStringBuilder.append("canvasName", theCanvasName);
}
{
String theDisplayName;
theDisplayName = this.getDisplayName();
toStringBuilder.append("displayName", theDisplayName);
}
{
String theIconUrl;
theIconUrl = this.getIconUrl();
toStringBuilder.append("iconUrl", theIconUrl);
}
{
String theLogoUrl;
theLogoUrl = this.getLogoUrl();
toStringBuilder.append("logoUrl", theLogoUrl);
}
{
ApplicationGetPublicInfoResponse.Developers theDevelopers;
theDevelopers = this.getDevelopers();
toStringBuilder.append("developers", theDevelopers);
}
{
String theCompanyName;
theCompanyName = this.getCompanyName();
toStringBuilder.append("companyName", theCompanyName);
}
{
String theDescription;
theDescription = this.getDescription();
toStringBuilder.append("description", theDescription);
}
{
long theDailyActiveUsers;
theDailyActiveUsers = this.getDailyActiveUsers();
toStringBuilder.append("dailyActiveUsers", theDailyActiveUsers);
}
{
long theWeeklyActiveUsers;
theWeeklyActiveUsers = this.getWeeklyActiveUsers();
toStringBuilder.append("weeklyActiveUsers", theWeeklyActiveUsers);
}
{
long theMonthlyActiveUsers;
theMonthlyActiveUsers = this.getMonthlyActiveUsers();
toStringBuilder.append("monthlyActiveUsers", theMonthlyActiveUsers);
}
{
int theDailyActivePercentage;
theDailyActivePercentage = this.getDailyActivePercentage();
toStringBuilder.append("dailyActivePercentage", theDailyActivePercentage);
}
}
public String toString() {
final ToStringBuilder toStringBuilder = new JAXBToStringBuilder(this);
toString(toStringBuilder);
return toStringBuilder.toString();
}
public void equals(Object object, EqualsBuilder equalsBuilder) {
if (!(object instanceof ApplicationGetPublicInfoResponse)) {
equalsBuilder.appendSuper(false);
return ;
}
if (this == object) {
return ;
}
final ApplicationGetPublicInfoResponse that = ((ApplicationGetPublicInfoResponse) object);
equalsBuilder.append(this.getAppId(), that.getAppId());
equalsBuilder.append(this.getApiKey(), that.getApiKey());
equalsBuilder.append(this.getCanvasName(), that.getCanvasName());
equalsBuilder.append(this.getDisplayName(), that.getDisplayName());
equalsBuilder.append(this.getIconUrl(), that.getIconUrl());
equalsBuilder.append(this.getLogoUrl(), that.getLogoUrl());
equalsBuilder.append(this.getDevelopers(), that.getDevelopers());
equalsBuilder.append(this.getCompanyName(), that.getCompanyName());
equalsBuilder.append(this.getDescription(), that.getDescription());
equalsBuilder.append(this.getDailyActiveUsers(), that.getDailyActiveUsers());
equalsBuilder.append(this.getWeeklyActiveUsers(), that.getWeeklyActiveUsers());
equalsBuilder.append(this.getMonthlyActiveUsers(), that.getMonthlyActiveUsers());
equalsBuilder.append(this.getDailyActivePercentage(), that.getDailyActivePercentage());
}
public boolean equals(Object object) {
if (!(object instanceof ApplicationGetPublicInfoResponse)) {
return false;
}
if (this == object) {
return true;
}
final EqualsBuilder equalsBuilder = new JAXBEqualsBuilder();
equals(object, equalsBuilder);
return equalsBuilder.isEquals();
}
public void hashCode(HashCodeBuilder hashCodeBuilder) {
hashCodeBuilder.append(this.getAppId());
hashCodeBuilder.append(this.getApiKey());
hashCodeBuilder.append(this.getCanvasName());
hashCodeBuilder.append(this.getDisplayName());
hashCodeBuilder.append(this.getIconUrl());
hashCodeBuilder.append(this.getLogoUrl());
hashCodeBuilder.append(this.getDevelopers());
hashCodeBuilder.append(this.getCompanyName());
hashCodeBuilder.append(this.getDescription());
hashCodeBuilder.append(this.getDailyActiveUsers());
hashCodeBuilder.append(this.getWeeklyActiveUsers());
hashCodeBuilder.append(this.getMonthlyActiveUsers());
hashCodeBuilder.append(this.getDailyActivePercentage());
}
public int hashCode() {
final HashCodeBuilder hashCodeBuilder = new JAXBHashCodeBuilder();
hashCode(hashCodeBuilder);
return hashCodeBuilder.toHashCode();
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence maxOccurs="unbounded" minOccurs="0">
* <element name="developer_info" type="{http://api.facebook.com/1.0/}developer_info" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="list" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"developerInfo"
})
public static class Developers
implements Equals, HashCode, ToString
{
@XmlElement(name = "developer_info")
protected List developerInfo;
@XmlAttribute
protected Boolean list;
/**
* Gets the value of the developerInfo property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the developerInfo property.
*
*
* For example, to add a new item, do as follows:
*
* getDeveloperInfo().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link DeveloperInfo }
*
*
*/
public List getDeveloperInfo() {
if (developerInfo == null) {
developerInfo = new ArrayList();
}
return this.developerInfo;
}
/**
* Gets the value of the list property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isList() {
return list;
}
/**
* Sets the value of the list property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setList(Boolean value) {
this.list = value;
}
public void toString(ToStringBuilder toStringBuilder) {
{
List theDeveloperInfo;
theDeveloperInfo = this.getDeveloperInfo();
toStringBuilder.append("developerInfo", theDeveloperInfo);
}
{
Boolean theList;
theList = this.isList();
toStringBuilder.append("list", theList);
}
}
public String toString() {
final ToStringBuilder toStringBuilder = new JAXBToStringBuilder(this);
toString(toStringBuilder);
return toStringBuilder.toString();
}
public void equals(Object object, EqualsBuilder equalsBuilder) {
if (!(object instanceof ApplicationGetPublicInfoResponse.Developers)) {
equalsBuilder.appendSuper(false);
return ;
}
if (this == object) {
return ;
}
final ApplicationGetPublicInfoResponse.Developers that = ((ApplicationGetPublicInfoResponse.Developers) object);
equalsBuilder.append(this.getDeveloperInfo(), that.getDeveloperInfo());
equalsBuilder.append(this.isList(), that.isList());
}
public boolean equals(Object object) {
if (!(object instanceof ApplicationGetPublicInfoResponse.Developers)) {
return false;
}
if (this == object) {
return true;
}
final EqualsBuilder equalsBuilder = new JAXBEqualsBuilder();
equals(object, equalsBuilder);
return equalsBuilder.isEquals();
}
public void hashCode(HashCodeBuilder hashCodeBuilder) {
hashCodeBuilder.append(this.getDeveloperInfo());
hashCodeBuilder.append(this.isList());
}
public int hashCode() {
final HashCodeBuilder hashCodeBuilder = new JAXBHashCodeBuilder();
hashCode(hashCodeBuilder);
return hashCodeBuilder.toHashCode();
}
}
}