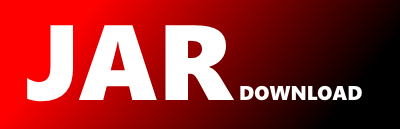
com.google.code.facebookapi.schema.Listing Maven / Gradle / Ivy
Show all versions of facebook-java-api-schema Show documentation
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, vhudson-jaxb-ri-2.1-661
// See http://java.sun.com/xml/jaxb
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2009.02.15 at 07:02:48 PM GMT-08:00
//
package com.google.code.facebookapi.schema;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlType;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.apache.commons.lang.builder.ToStringBuilder;
import org.jvnet.jaxb2_commons.lang.Equals;
import org.jvnet.jaxb2_commons.lang.HashCode;
import org.jvnet.jaxb2_commons.lang.ToString;
import org.jvnet.jaxb2_commons.lang.builder.JAXBEqualsBuilder;
import org.jvnet.jaxb2_commons.lang.builder.JAXBHashCodeBuilder;
import org.jvnet.jaxb2_commons.lang.builder.JAXBToStringBuilder;
/**
* Java class for listing complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="listing">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="listing_id" type="{http://api.facebook.com/1.0/}lid"/>
* <element name="url" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="title" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="description" type="{http://www.w3.org/2001/XMLSchema}string"/>
* <element name="price" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* <element name="poster" type="{http://api.facebook.com/1.0/}uid"/>
* <element name="update_time" type="{http://api.facebook.com/1.0/}time"/>
* <element name="category" type="{http://api.facebook.com/1.0/}marketplace_category"/>
* <element name="subcategory" type="{http://api.facebook.com/1.0/}marketplace_subcategory"/>
* <element name="image_urls" minOccurs="0">
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence maxOccurs="unbounded" minOccurs="0">
* <element name="image_urls_elt" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="list" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </restriction>
* </complexContent>
* </complexType>
* </element>
* <element name="condition" type="{http://www.w3.org/2001/XMLSchema}int" minOccurs="0"/>
* <element name="isbn" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="num_beds" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="num_maths" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="dogs" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="cats" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="smoking" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="square_footage" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="street" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="crossstreet" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="postal" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="rent" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="pay" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="full" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="intern" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="summer" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="nonprofit" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="pay_type" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "listing", propOrder = {
"listingId",
"url",
"title",
"description",
"price",
"poster",
"updateTime",
"category",
"subcategory",
"imageUrls",
"condition",
"isbn",
"numBeds",
"numMaths",
"dogs",
"cats",
"smoking",
"squareFootage",
"street",
"crossstreet",
"postal",
"rent",
"pay",
"full",
"intern",
"summer",
"nonprofit",
"payType"
})
public class Listing
implements Equals, HashCode, ToString
{
@XmlElement(name = "listing_id")
protected long listingId;
@XmlElement(required = true)
protected String url;
@XmlElement(required = true)
protected String title;
@XmlElement(required = true)
protected String description;
protected BigDecimal price;
protected long poster;
@XmlElement(name = "update_time")
protected long updateTime;
@XmlElement(required = true)
protected String category;
@XmlElement(required = true)
protected String subcategory;
@XmlElement(name = "image_urls")
protected Listing.ImageUrls imageUrls;
protected Integer condition;
protected String isbn;
@XmlElement(name = "num_beds")
protected String numBeds;
@XmlElement(name = "num_maths")
protected String numMaths;
protected String dogs;
protected String cats;
protected String smoking;
@XmlElement(name = "square_footage")
protected String squareFootage;
protected String street;
protected String crossstreet;
protected String postal;
protected String rent;
protected String pay;
protected String full;
protected String intern;
protected String summer;
protected String nonprofit;
@XmlElement(name = "pay_type")
protected String payType;
/**
* Gets the value of the listingId property.
*
*/
public long getListingId() {
return listingId;
}
/**
* Sets the value of the listingId property.
*
*/
public void setListingId(long value) {
this.listingId = value;
}
/**
* Gets the value of the url property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getUrl() {
return url;
}
/**
* Sets the value of the url property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setUrl(String value) {
this.url = value;
}
/**
* Gets the value of the title property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTitle() {
return title;
}
/**
* Sets the value of the title property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTitle(String value) {
this.title = value;
}
/**
* Gets the value of the description property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDescription() {
return description;
}
/**
* Sets the value of the description property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDescription(String value) {
this.description = value;
}
/**
* Gets the value of the price property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getPrice() {
return price;
}
/**
* Sets the value of the price property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setPrice(BigDecimal value) {
this.price = value;
}
/**
* Gets the value of the poster property.
*
*/
public long getPoster() {
return poster;
}
/**
* Sets the value of the poster property.
*
*/
public void setPoster(long value) {
this.poster = value;
}
/**
* Gets the value of the updateTime property.
*
*/
public long getUpdateTime() {
return updateTime;
}
/**
* Sets the value of the updateTime property.
*
*/
public void setUpdateTime(long value) {
this.updateTime = value;
}
/**
* Gets the value of the category property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCategory() {
return category;
}
/**
* Sets the value of the category property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCategory(String value) {
this.category = value;
}
/**
* Gets the value of the subcategory property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSubcategory() {
return subcategory;
}
/**
* Sets the value of the subcategory property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSubcategory(String value) {
this.subcategory = value;
}
/**
* Gets the value of the imageUrls property.
*
* @return
* possible object is
* {@link Listing.ImageUrls }
*
*/
public Listing.ImageUrls getImageUrls() {
return imageUrls;
}
/**
* Sets the value of the imageUrls property.
*
* @param value
* allowed object is
* {@link Listing.ImageUrls }
*
*/
public void setImageUrls(Listing.ImageUrls value) {
this.imageUrls = value;
}
/**
* Gets the value of the condition property.
*
* @return
* possible object is
* {@link Integer }
*
*/
public Integer getCondition() {
return condition;
}
/**
* Sets the value of the condition property.
*
* @param value
* allowed object is
* {@link Integer }
*
*/
public void setCondition(Integer value) {
this.condition = value;
}
/**
* Gets the value of the isbn property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIsbn() {
return isbn;
}
/**
* Sets the value of the isbn property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIsbn(String value) {
this.isbn = value;
}
/**
* Gets the value of the numBeds property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNumBeds() {
return numBeds;
}
/**
* Sets the value of the numBeds property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNumBeds(String value) {
this.numBeds = value;
}
/**
* Gets the value of the numMaths property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNumMaths() {
return numMaths;
}
/**
* Sets the value of the numMaths property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNumMaths(String value) {
this.numMaths = value;
}
/**
* Gets the value of the dogs property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getDogs() {
return dogs;
}
/**
* Sets the value of the dogs property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setDogs(String value) {
this.dogs = value;
}
/**
* Gets the value of the cats property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCats() {
return cats;
}
/**
* Sets the value of the cats property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCats(String value) {
this.cats = value;
}
/**
* Gets the value of the smoking property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSmoking() {
return smoking;
}
/**
* Sets the value of the smoking property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSmoking(String value) {
this.smoking = value;
}
/**
* Gets the value of the squareFootage property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSquareFootage() {
return squareFootage;
}
/**
* Sets the value of the squareFootage property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSquareFootage(String value) {
this.squareFootage = value;
}
/**
* Gets the value of the street property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getStreet() {
return street;
}
/**
* Sets the value of the street property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setStreet(String value) {
this.street = value;
}
/**
* Gets the value of the crossstreet property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCrossstreet() {
return crossstreet;
}
/**
* Sets the value of the crossstreet property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCrossstreet(String value) {
this.crossstreet = value;
}
/**
* Gets the value of the postal property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPostal() {
return postal;
}
/**
* Sets the value of the postal property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPostal(String value) {
this.postal = value;
}
/**
* Gets the value of the rent property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getRent() {
return rent;
}
/**
* Sets the value of the rent property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setRent(String value) {
this.rent = value;
}
/**
* Gets the value of the pay property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPay() {
return pay;
}
/**
* Sets the value of the pay property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPay(String value) {
this.pay = value;
}
/**
* Gets the value of the full property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getFull() {
return full;
}
/**
* Sets the value of the full property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setFull(String value) {
this.full = value;
}
/**
* Gets the value of the intern property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getIntern() {
return intern;
}
/**
* Sets the value of the intern property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setIntern(String value) {
this.intern = value;
}
/**
* Gets the value of the summer property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getSummer() {
return summer;
}
/**
* Sets the value of the summer property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setSummer(String value) {
this.summer = value;
}
/**
* Gets the value of the nonprofit property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getNonprofit() {
return nonprofit;
}
/**
* Sets the value of the nonprofit property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setNonprofit(String value) {
this.nonprofit = value;
}
/**
* Gets the value of the payType property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getPayType() {
return payType;
}
/**
* Sets the value of the payType property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPayType(String value) {
this.payType = value;
}
public void toString(ToStringBuilder toStringBuilder) {
{
long theListingId;
theListingId = this.getListingId();
toStringBuilder.append("listingId", theListingId);
}
{
String theUrl;
theUrl = this.getUrl();
toStringBuilder.append("url", theUrl);
}
{
String theTitle;
theTitle = this.getTitle();
toStringBuilder.append("title", theTitle);
}
{
String theDescription;
theDescription = this.getDescription();
toStringBuilder.append("description", theDescription);
}
{
BigDecimal thePrice;
thePrice = this.getPrice();
toStringBuilder.append("price", thePrice);
}
{
long thePoster;
thePoster = this.getPoster();
toStringBuilder.append("poster", thePoster);
}
{
long theUpdateTime;
theUpdateTime = this.getUpdateTime();
toStringBuilder.append("updateTime", theUpdateTime);
}
{
String theCategory;
theCategory = this.getCategory();
toStringBuilder.append("category", theCategory);
}
{
String theSubcategory;
theSubcategory = this.getSubcategory();
toStringBuilder.append("subcategory", theSubcategory);
}
{
Listing.ImageUrls theImageUrls;
theImageUrls = this.getImageUrls();
toStringBuilder.append("imageUrls", theImageUrls);
}
{
Integer theCondition;
theCondition = this.getCondition();
toStringBuilder.append("condition", theCondition);
}
{
String theIsbn;
theIsbn = this.getIsbn();
toStringBuilder.append("isbn", theIsbn);
}
{
String theNumBeds;
theNumBeds = this.getNumBeds();
toStringBuilder.append("numBeds", theNumBeds);
}
{
String theNumMaths;
theNumMaths = this.getNumMaths();
toStringBuilder.append("numMaths", theNumMaths);
}
{
String theDogs;
theDogs = this.getDogs();
toStringBuilder.append("dogs", theDogs);
}
{
String theCats;
theCats = this.getCats();
toStringBuilder.append("cats", theCats);
}
{
String theSmoking;
theSmoking = this.getSmoking();
toStringBuilder.append("smoking", theSmoking);
}
{
String theSquareFootage;
theSquareFootage = this.getSquareFootage();
toStringBuilder.append("squareFootage", theSquareFootage);
}
{
String theStreet;
theStreet = this.getStreet();
toStringBuilder.append("street", theStreet);
}
{
String theCrossstreet;
theCrossstreet = this.getCrossstreet();
toStringBuilder.append("crossstreet", theCrossstreet);
}
{
String thePostal;
thePostal = this.getPostal();
toStringBuilder.append("postal", thePostal);
}
{
String theRent;
theRent = this.getRent();
toStringBuilder.append("rent", theRent);
}
{
String thePay;
thePay = this.getPay();
toStringBuilder.append("pay", thePay);
}
{
String theFull;
theFull = this.getFull();
toStringBuilder.append("full", theFull);
}
{
String theIntern;
theIntern = this.getIntern();
toStringBuilder.append("intern", theIntern);
}
{
String theSummer;
theSummer = this.getSummer();
toStringBuilder.append("summer", theSummer);
}
{
String theNonprofit;
theNonprofit = this.getNonprofit();
toStringBuilder.append("nonprofit", theNonprofit);
}
{
String thePayType;
thePayType = this.getPayType();
toStringBuilder.append("payType", thePayType);
}
}
public String toString() {
final ToStringBuilder toStringBuilder = new JAXBToStringBuilder(this);
toString(toStringBuilder);
return toStringBuilder.toString();
}
public void equals(Object object, EqualsBuilder equalsBuilder) {
if (!(object instanceof Listing)) {
equalsBuilder.appendSuper(false);
return ;
}
if (this == object) {
return ;
}
final Listing that = ((Listing) object);
equalsBuilder.append(this.getListingId(), that.getListingId());
equalsBuilder.append(this.getUrl(), that.getUrl());
equalsBuilder.append(this.getTitle(), that.getTitle());
equalsBuilder.append(this.getDescription(), that.getDescription());
equalsBuilder.append(this.getPrice(), that.getPrice());
equalsBuilder.append(this.getPoster(), that.getPoster());
equalsBuilder.append(this.getUpdateTime(), that.getUpdateTime());
equalsBuilder.append(this.getCategory(), that.getCategory());
equalsBuilder.append(this.getSubcategory(), that.getSubcategory());
equalsBuilder.append(this.getImageUrls(), that.getImageUrls());
equalsBuilder.append(this.getCondition(), that.getCondition());
equalsBuilder.append(this.getIsbn(), that.getIsbn());
equalsBuilder.append(this.getNumBeds(), that.getNumBeds());
equalsBuilder.append(this.getNumMaths(), that.getNumMaths());
equalsBuilder.append(this.getDogs(), that.getDogs());
equalsBuilder.append(this.getCats(), that.getCats());
equalsBuilder.append(this.getSmoking(), that.getSmoking());
equalsBuilder.append(this.getSquareFootage(), that.getSquareFootage());
equalsBuilder.append(this.getStreet(), that.getStreet());
equalsBuilder.append(this.getCrossstreet(), that.getCrossstreet());
equalsBuilder.append(this.getPostal(), that.getPostal());
equalsBuilder.append(this.getRent(), that.getRent());
equalsBuilder.append(this.getPay(), that.getPay());
equalsBuilder.append(this.getFull(), that.getFull());
equalsBuilder.append(this.getIntern(), that.getIntern());
equalsBuilder.append(this.getSummer(), that.getSummer());
equalsBuilder.append(this.getNonprofit(), that.getNonprofit());
equalsBuilder.append(this.getPayType(), that.getPayType());
}
public boolean equals(Object object) {
if (!(object instanceof Listing)) {
return false;
}
if (this == object) {
return true;
}
final EqualsBuilder equalsBuilder = new JAXBEqualsBuilder();
equals(object, equalsBuilder);
return equalsBuilder.isEquals();
}
public void hashCode(HashCodeBuilder hashCodeBuilder) {
hashCodeBuilder.append(this.getListingId());
hashCodeBuilder.append(this.getUrl());
hashCodeBuilder.append(this.getTitle());
hashCodeBuilder.append(this.getDescription());
hashCodeBuilder.append(this.getPrice());
hashCodeBuilder.append(this.getPoster());
hashCodeBuilder.append(this.getUpdateTime());
hashCodeBuilder.append(this.getCategory());
hashCodeBuilder.append(this.getSubcategory());
hashCodeBuilder.append(this.getImageUrls());
hashCodeBuilder.append(this.getCondition());
hashCodeBuilder.append(this.getIsbn());
hashCodeBuilder.append(this.getNumBeds());
hashCodeBuilder.append(this.getNumMaths());
hashCodeBuilder.append(this.getDogs());
hashCodeBuilder.append(this.getCats());
hashCodeBuilder.append(this.getSmoking());
hashCodeBuilder.append(this.getSquareFootage());
hashCodeBuilder.append(this.getStreet());
hashCodeBuilder.append(this.getCrossstreet());
hashCodeBuilder.append(this.getPostal());
hashCodeBuilder.append(this.getRent());
hashCodeBuilder.append(this.getPay());
hashCodeBuilder.append(this.getFull());
hashCodeBuilder.append(this.getIntern());
hashCodeBuilder.append(this.getSummer());
hashCodeBuilder.append(this.getNonprofit());
hashCodeBuilder.append(this.getPayType());
}
public int hashCode() {
final HashCodeBuilder hashCodeBuilder = new JAXBHashCodeBuilder();
hashCode(hashCodeBuilder);
return hashCodeBuilder.toHashCode();
}
/**
* Java class for anonymous complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType>
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence maxOccurs="unbounded" minOccurs="0">
* <element name="image_urls_elt" type="{http://www.w3.org/2001/XMLSchema}string" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* <attribute name="list" type="{http://www.w3.org/2001/XMLSchema}boolean" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"imageUrlsElt"
})
public static class ImageUrls
implements Equals, HashCode, ToString
{
@XmlElement(name = "image_urls_elt")
protected List imageUrlsElt;
@XmlAttribute
protected Boolean list;
/**
* Gets the value of the imageUrlsElt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the imageUrlsElt property.
*
*
* For example, to add a new item, do as follows:
*
* getImageUrlsElt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link String }
*
*
*/
public List getImageUrlsElt() {
if (imageUrlsElt == null) {
imageUrlsElt = new ArrayList();
}
return this.imageUrlsElt;
}
/**
* Gets the value of the list property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isList() {
return list;
}
/**
* Sets the value of the list property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setList(Boolean value) {
this.list = value;
}
public void toString(ToStringBuilder toStringBuilder) {
{
List theImageUrlsElt;
theImageUrlsElt = this.getImageUrlsElt();
toStringBuilder.append("imageUrlsElt", theImageUrlsElt);
}
{
Boolean theList;
theList = this.isList();
toStringBuilder.append("list", theList);
}
}
public String toString() {
final ToStringBuilder toStringBuilder = new JAXBToStringBuilder(this);
toString(toStringBuilder);
return toStringBuilder.toString();
}
public void equals(Object object, EqualsBuilder equalsBuilder) {
if (!(object instanceof Listing.ImageUrls)) {
equalsBuilder.appendSuper(false);
return ;
}
if (this == object) {
return ;
}
final Listing.ImageUrls that = ((Listing.ImageUrls) object);
equalsBuilder.append(this.getImageUrlsElt(), that.getImageUrlsElt());
equalsBuilder.append(this.isList(), that.isList());
}
public boolean equals(Object object) {
if (!(object instanceof Listing.ImageUrls)) {
return false;
}
if (this == object) {
return true;
}
final EqualsBuilder equalsBuilder = new JAXBEqualsBuilder();
equals(object, equalsBuilder);
return equalsBuilder.isEquals();
}
public void hashCode(HashCodeBuilder hashCodeBuilder) {
hashCodeBuilder.append(this.getImageUrlsElt());
hashCodeBuilder.append(this.isList());
}
public int hashCode() {
final HashCodeBuilder hashCodeBuilder = new JAXBHashCodeBuilder();
hashCode(hashCodeBuilder);
return hashCodeBuilder.toHashCode();
}
}
}